In C++, class functions (also known as methods) are functions that operate on the objects of a class, allowing encapsulation and behavior definition within the class itself.
class Example {
public:
void greet() {
std::cout << "Hello, world!" << std::endl;
}
};
int main() {
Example ex;
ex.greet(); // Calls the greet function
return 0;
}
Understanding C++ Classes
What is a Class in C++?
A class in C++ is a blueprint for creating objects. It encapsulates data for the object and methods to manipulate that data. Classes are fundamental to object-oriented programming in C++.
Consider a simple class definition for a Car:
class Car {
public:
// Attributes
string model;
int year;
// Method
void displayInfo() {
cout << "Model: " << model << ", Year: " << year << endl;
}
};
In this example, the `Car` class has two attributes: `model` and `year`, along with a method called `displayInfo()` which prints the car's details.
Benefits of Using Classes
Using classes provides a plethora of advantages:
- Encapsulation: They allow bundling data and functions that operate on that data, keeping code organized and manageable.
- Reusability: Code can be reused across different projects or parts of the same project, reducing redundancy.
- Improved Maintainability: Changes can be made in one place without affecting the entire system, allowing for easier updates and debugging.
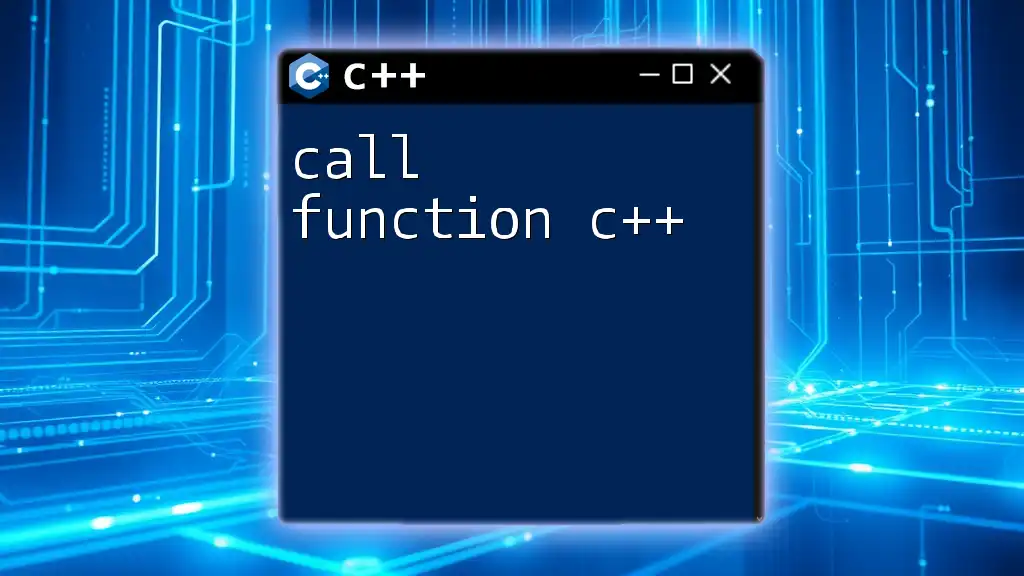
Exploring Functions within Classes
What are Class Functions in C++?
Class functions, or member functions, are functions defined within a class that operate on the objects of that class. Unlike global functions, which are independent, member functions have access to the class's data members.
Here’s an example of a class function:
class Rectangle {
public:
int width, height;
int area() {
return width * height; // Member function calculating area
}
};
Types of Class Functions
Non-static Member Functions
Non-static member functions operate on an instance of a class. They have access to the instance’s attributes, and can modify them.
Example:
class Box {
public:
int length;
void setLength(int l) {
length = l; // Setting the value of an attribute
}
};
Static Member Functions
Static member functions belong to the class rather than any specific object. They do not have access to instance variables or instance methods.
Example of a static function:
class Calculator {
public:
static int add(int a, int b) {
return a + b; // Static member function
}
};
Const Member Functions
Const member functions ensure that the function does not modify any member variables of the object on which it is invoked. This is particularly useful for read-only methods.
Example:
class Point {
public:
int x, y;
int getX() const {
return x; // A const member function
}
};
Friend Functions
Friend functions are not member functions but have access to the private and protected members of a class. They are defined outside the class but are declared as friends inside the class.
Example:
class Box;
class Friend {
public:
void display(Box &b);
};
class Box {
private:
int length;
public:
Box(int l) : length(l) {}
friend void Friend::display(Box &b);
};
void Friend::display(Box &b) {
cout << "Length: " << b.length << endl; // Accessing private member
}
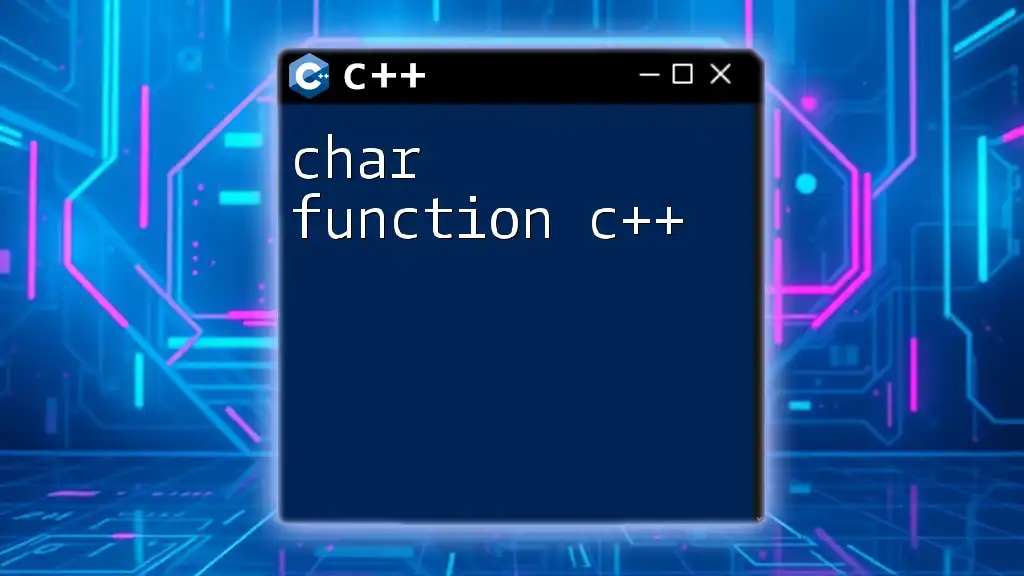
Working with Functions in Classes
Defining Functions in a Class
Defining a function in a class involves specifying the function's return type, name, and parameters (if any). It's essential to maintain clarity in your syntax for better readability.
Example:
class Employee {
public:
string name;
void setName(string empName) {
name = empName; // Setting the value of name
}
};
Calling Class Functions
To call class functions, you first need to create an object of the class. After instantiation, you can access the functions using the dot operator (`.`).
Example:
Employee emp;
emp.setName("John Doe"); // Calling a non-static member function
cout << emp.name << endl; // Outputting the name
For static functions, you call the function using the class name:
int sum = Calculator::add(5, 10); // Calling a static member function
Function Overloading in Classes
C++ allows you to create multiple functions with the same name but different parameters within a class. This is known as function overloading.
To overload member functions, ensure that the parameter lists differ in type or number.
Example:
class Display {
public:
void show(int i) {
cout << "Integer: " << i << endl;
}
void show(double d) {
cout << "Double: " << d << endl;
}
};
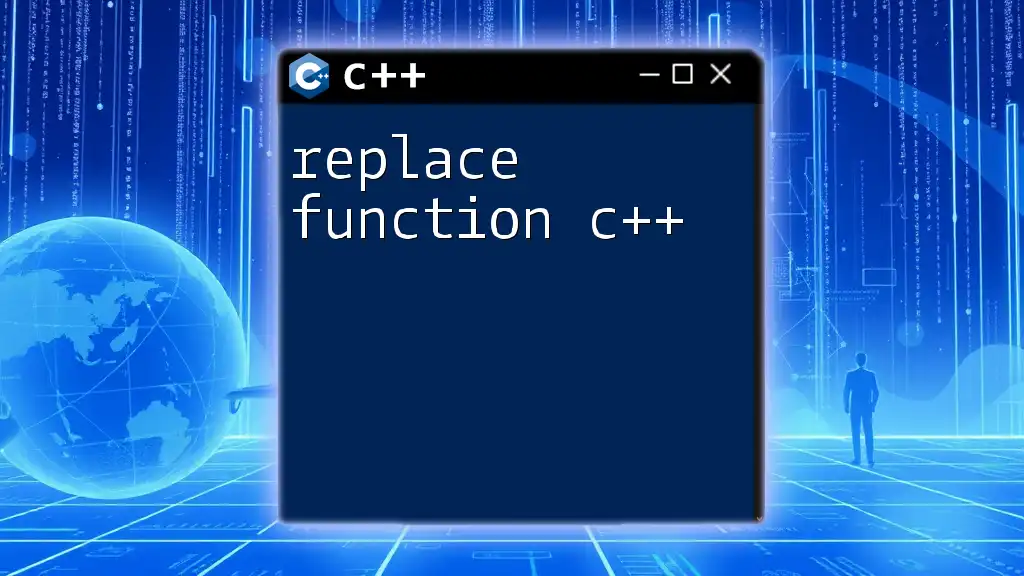
Practical Examples
Example 1: Simple Bank Account Class
Let’s create a simple BankAccount class, which will illustrate member functions clearly:
class BankAccount {
private:
double balance;
public:
BankAccount() : balance(0) {}
void deposit(double amount) {
balance += amount; // Deposit method
}
void withdraw(double amount) {
if (amount > balance) {
cout << "Insufficient funds!" << endl;
} else {
balance -= amount; // Withdraw method
}
}
void displayBalance() const {
cout << "Balance: $" << balance << endl; // Display balance
}
};
Example 2: Rectangle Class with Area Calculation
For a practical area calculation, let’s define a Rectangle class, focusing on class functions:
class Rectangle {
private:
int width, height;
public:
Rectangle(int w, int h) : width(w), height(h) {}
int area() const {
return width * height; // Area calculation
}
void display() const {
cout << "Width: " << width << ", Height: " << height << ", Area: " << area() << endl;
}
};
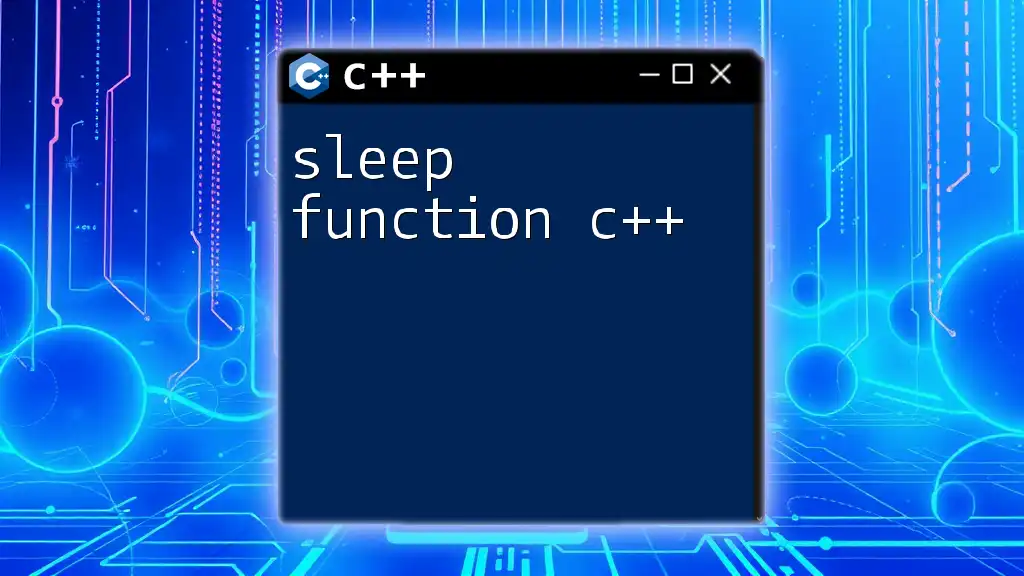
Best Practices for Using Class Functions
Keeping Functions Concise
Strive for short and focused functions. Each function should accomplish a single task, leading to clearer, more maintainable code.
Using Access Specifiers
Utilize access specifiers wisely. Public members are accessible outside the class, whereas private members are hidden. Understanding when to encapsulate data is crucial for maintaining class integrity.
class MyClass {
private:
int secret;
public:
void reveal() {
cout << "Secret: " << secret << endl; // Accessing private member
}
};
Documentation and Comments
The significance of documenting your class functions cannot be overstated. Write clean, understandable comments that clarify the purpose and functionality of each function. This aids both in development and future maintenance.
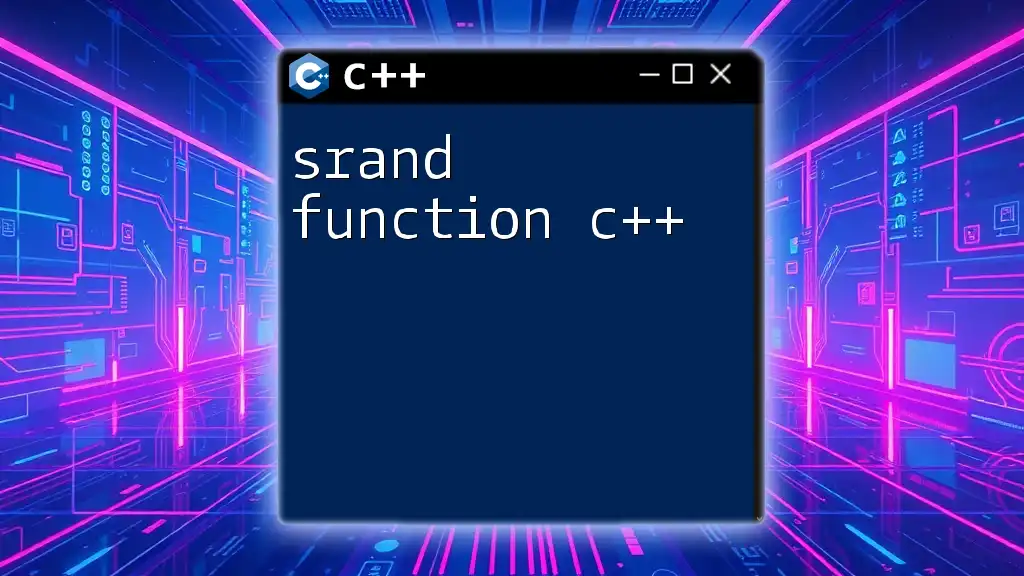
Conclusion
In summary, understanding class functions in C++ is paramount to mastering object-oriented programming. These functions allow you to manipulate object data effectively, encapsulate functionality, and write organized code. Delve into examples, practice continuously, and explore the immense capabilities provided by C++ class functions.
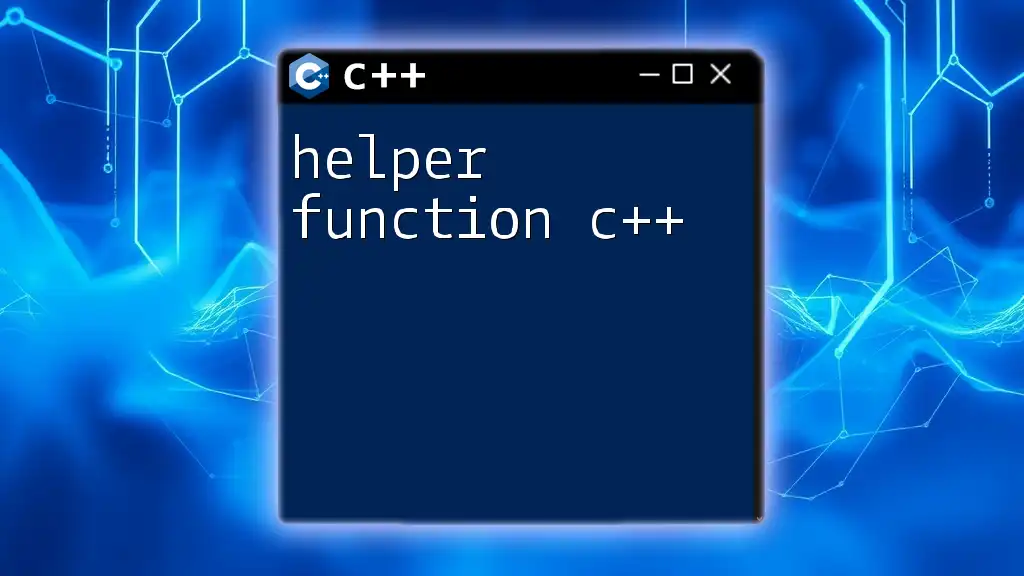
Call to Action
Feel inspired to explore more resources on C++ class functions. Share your experiences and questions in the comments section below; let's learn and grow together in our coding journey!