Variadic functions in C++ allow you to create functions that can take a variable number of arguments, enabling more flexible function calls.
Here's a simple code snippet demonstrating how to define and use a variadic function in C++:
#include <iostream>
#include <cstdarg>
void printNumbers(int count, ...) {
va_list args;
va_start(args, count);
for (int i = 0; i < count; ++i) {
std::cout << va_arg(args, int) << " ";
}
va_end(args);
}
int main() {
printNumbers(5, 10, 20, 30, 40, 50); // Outputs: 10 20 30 40 50
return 0;
}
What are Variadic Functions?
Variadic functions are a special type of function that allows you to accept a variable number of arguments. They are particularly useful when you don't know beforehand how many inputs the function will require. In C++, this feature can greatly enhance the flexibility and utility of your functions, enabling dynamic data handling.

Understanding Variadic Parameters in C++
Definition of Variadic Parameters
Variadic parameters are parameters that allow a function to accept a variable number of arguments. Unlike regular parameters, where the number of inputs must be predetermined, variadic parameters use a special syntax to allow for flexibility.
How They Differ from Regular Parameters
In a regular function, the parameters must be declared explicitly. For example, a function that takes two integers would be specified as:
void add(int a, int b);
However, with variadic functions, you may define a function that takes multiple arguments of the same type or different types, fostering greater versatility.
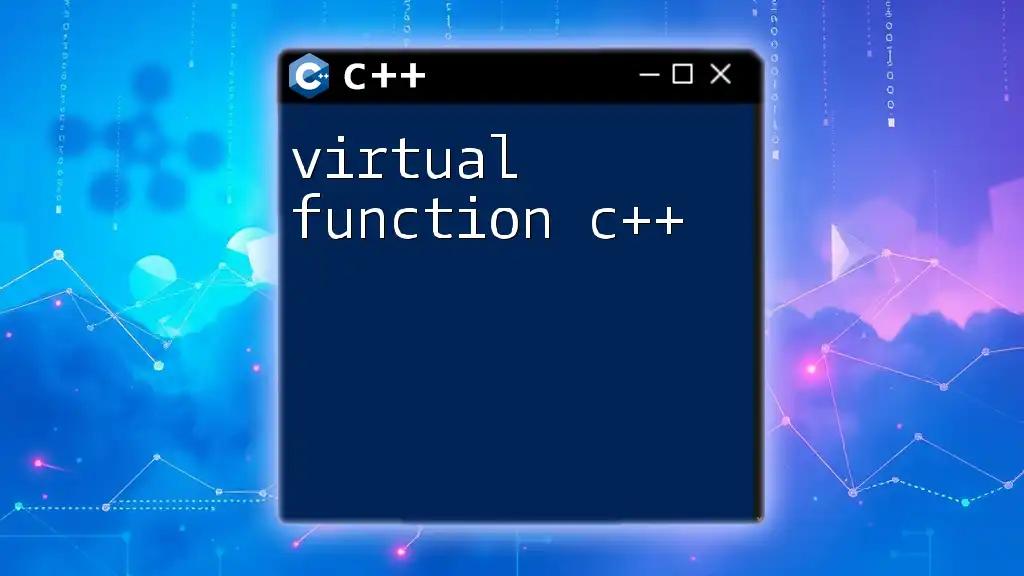
What are Varargs in C++?
The term "varargs" refers to the variable arguments feature that allows for functions to accept a flexible number of arguments. Historically, this feature was mainly associated with the C programming language, but it is also available in C++.
C++ provides mechanisms through the standard library to support variadic functions, which can lead to cleaner and more readable code. While varargs are straightforward to use, they can lead to less type safety and potential runtime errors, prompting the cautious programmer to seek alternatives.

Syntax of C++ Variadic Functions
The syntax for declaring a variadic function in C++ involves using an ellipsis (`...`) at the end of the parameter list. Here’s a basic example of a simple variadic function that prints multiple integers:
void printNumbers(int count, ...) {
va_list args; // Declare a variable of type va_list
va_start(args, count); // Initialize args to retrieve additional arguments
for (int i = 0; i < count; ++i) {
int num = va_arg(args, int); // Retrieve each argument
std::cout << num << " "; // Print the argument
}
va_end(args); // Clean up
}
This function uses macros from the `<cstdarg>` header to handle the variable arguments, which is essential for variadic functions.

Using Variadic Arguments in Functions
The aforementioned example also highlights how to implement variadic arguments in functions. Let's explore this by creating a simple function that sums a list of integers passed to it:
int sum(int count, ...) {
int total = 0; // Variable to hold the total
va_list args; // Declare a variable of type va_list
va_start(args, count); // Initialize args
for (int i = 0; i < count; ++i) {
total += va_arg(args, int); // Retrieve each integer and add it to total
}
va_end(args); // Clean up
return total; // Return the sum
}
In this function, `va_list`, `va_start`, `va_arg`, and `va_end` are crucial for managing the input arguments. This is a fundamental demonstration of handling variadic arguments in C++.
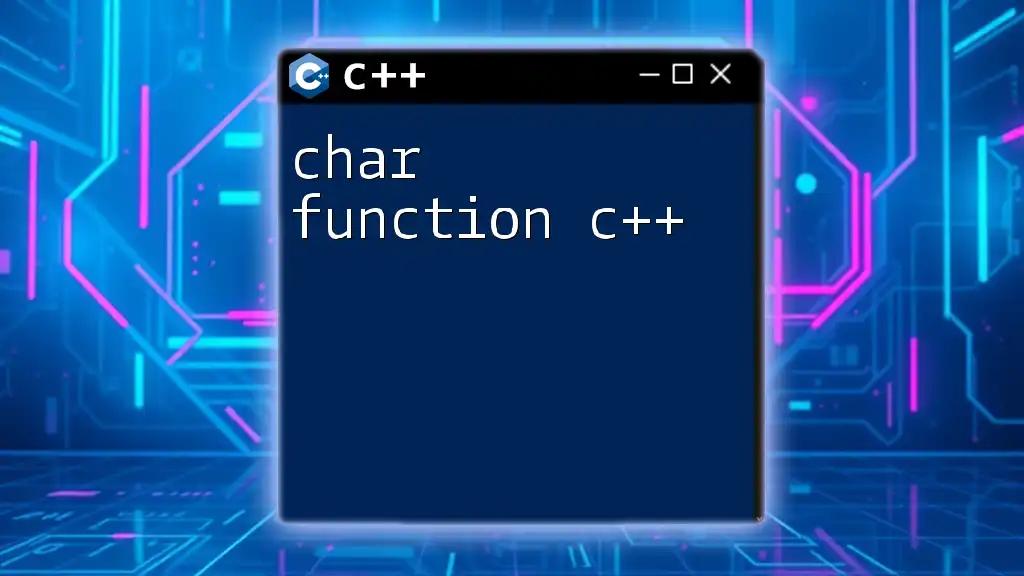
Advanced Techniques with Variadic Functions
Variadic Templates in C++11 and Beyond
Introduced in C++11, variadic templates provide a more type-safe alternative to traditional variadic functions. They allow you to define functions that can accept any number of parameters of varying types.
An example of a variadic template function for printing multiple data types would be:
template<typename T>
void print(const T& value) { // Base case for recursion
std::cout << value << " ";
}
template<typename T, typename... Args>
void print(const T& first, const Args&... rest) { // Recursive case
print(first); // Print the first argument
print(rest...); // Recursively print the rest
}
The recursive nature of this implementation allows for a seamless handling of multiple arguments of potentially differing types.
Handling Different Data Types with Variadic Functions
One of the main advantages of variadic templates is their ability to handle various data types cleanly. This eliminates the drawbacks of type ambiguity present in traditional variadic functions. For illustration, let's consider a scenario where we want to concatenate different types into a single output.
This flexibility comes with optional template specialization, allowing more control over specific types if necessary.

Common Use Cases for Variadic Functions
Variadic functions are widely employed across various applications. Some popular use cases include:
-
Logging Functions: Functions that log different messages, where a varying number of arguments and types may exist, relying on variadic handling for flexibility.
-
Event Handling Systems: Used in GUI applications, where functions may receive different numbers and types of event parameters.
-
Mathematical Computations: For averaging or summing a dynamic list of values, variadic functions enable simplified calculations without static method signatures.
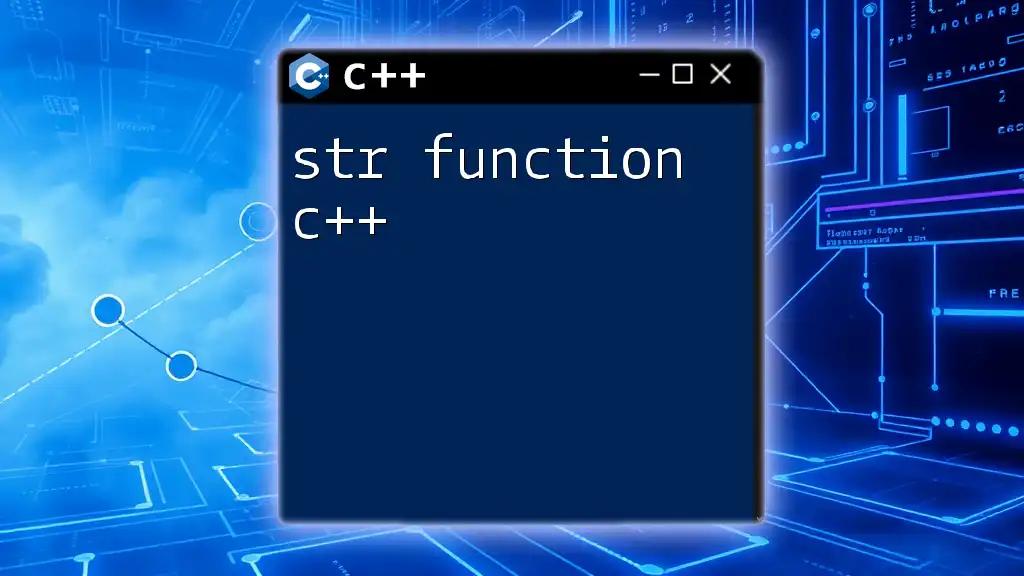
Performance Considerations
While variadic functions add flexibility, they should be used judiciously. Key considerations include:
-
When to Use: Employ variadic functions when you need to accept a diverse range of inputs. Avoid them when clear, type-safe function definitions can improve code clarity.
-
Potential Drawbacks: Variadic functions can lead to runtime errors if argument types are not carefully managed. Practices such as ensuring that the user knows the expected types can help mitigate issues.

Best Practices for Variadic Functions
Ensuring Type Safety
Maintaining type safety is crucial while using variadic functions. You may use mechanisms like `static_assert` within variadic templates to enforce constraints on input types, reducing runtime errors.
Readability and Maintainability
Clear structure enhances maintainability. Here are some tips:
- Use clear naming conventions.
- Include comments to explain complex logic.
- Maintain consistent formatting for readability.
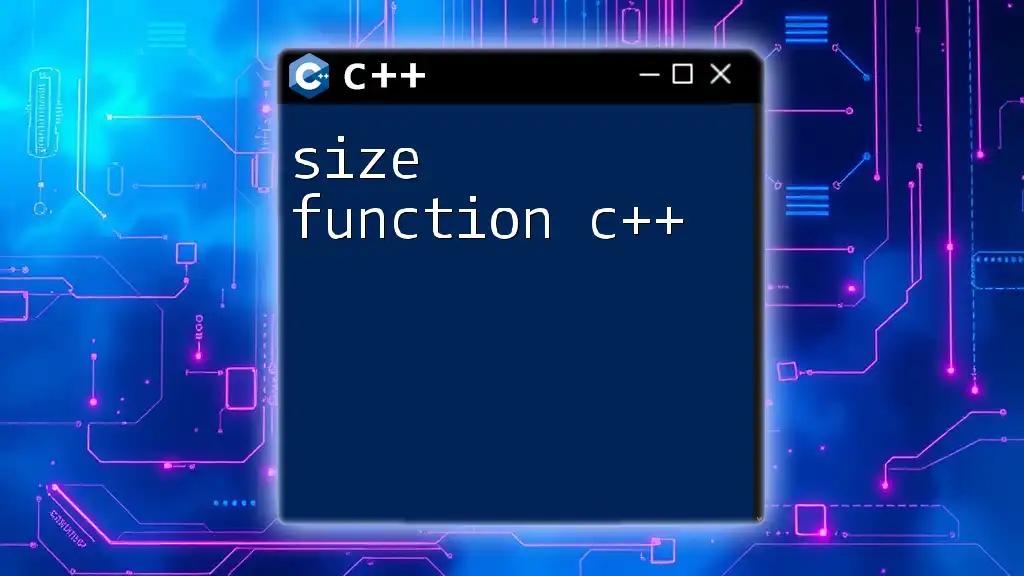
Recap of Key Points
To conclude, variadic functions in C++ represent a powerful programming feature that offers flexibility in handling variable numbers of arguments. By understanding their types, syntax, variations with templates, and best practices, programmers can exploit their advantages while minimizing potential pitfalls.
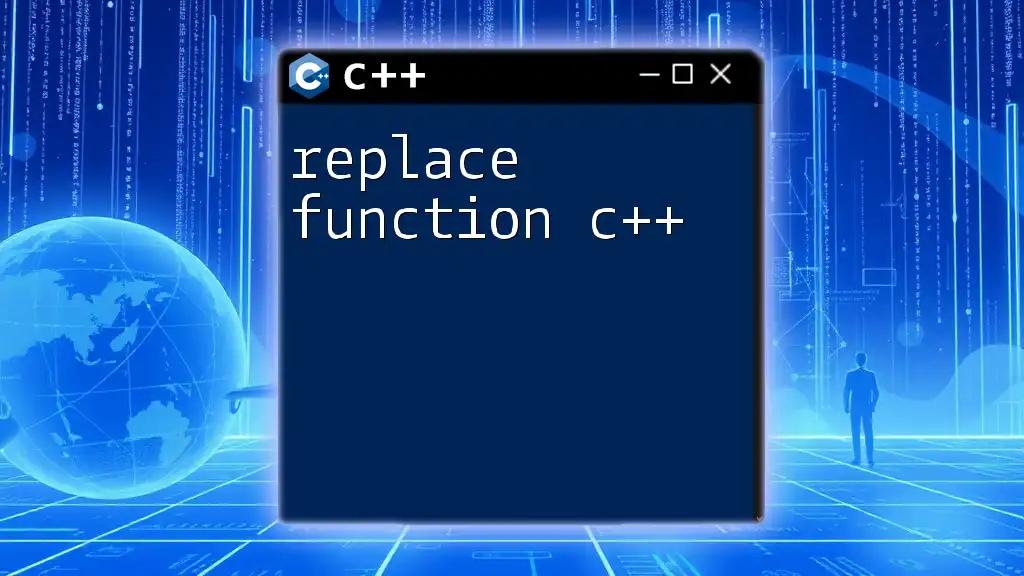
Further Learning Resources
For those eager to expand their knowledge further, consider delving into the recommended materials such as specialized books on C++, online tutorials, and active participation in C++ developer communities and forums. Embrace the learning journey, and keep coding!