The `print` function in C++ is typically accomplished using the `cout` object from the `iostream` library, which allows you to output text to the console.
Here’s a simple example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the Basics of C++ Print Function
What is the C++ Print Function?
The C++ print function is a fundamental feature of the language that allows programmers to output data to the console. It plays a crucial role in displaying results, debugging code, and interacting with users. By mastering the print function, you gain a powerful tool for communication within your C++ programs.
The Standard Output Stream
In C++, output is commonly directed to the standard output stream, represented by `std::cout`. This is part of the `<iostream>` library, which needs to be included in any program that utilizes output. Understanding how `std::cout` works is essential for effectively using the print function.
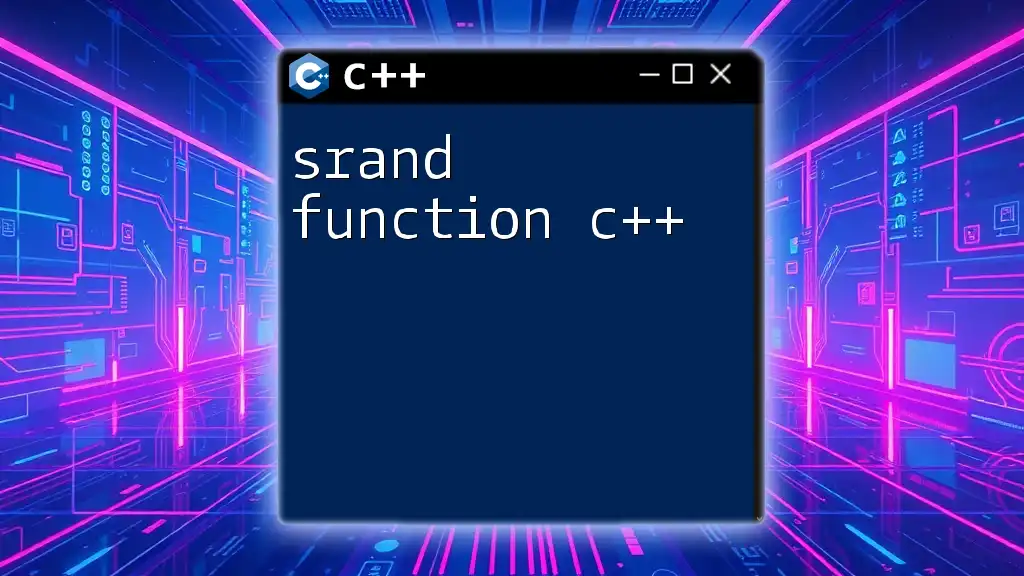
Getting Started with the Print Function in C++
Basic Syntax of the Print Function
To utilize the print function in C++, you typically use the following syntax:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example, `std::cout` sends the string "Hello, World!" to the console. The `<<` operator is used to append input to the output stream, while `std::endl` generates a new line and flushes the output buffer. Each component of this syntax plays a specific role, making it easy to generate output with minimal complexity.
Using Escape Sequences in Output
Escape sequences are special characters that provide formatting options within the output. Common escape sequences in C++ include:
- `\n`: Newline
- `\t`: Horizontal tab
- `\\`: Backslash
Using escape sequences, you can enhance the readability of your output. For instance:
std::cout << "Hello, World!\nWelcome to C++ Programming!\n";
This code snippet will print "Hello, World!" followed by "Welcome to C++ Programming!" on the next line, demonstrating how to manipulate the format for better clarity.
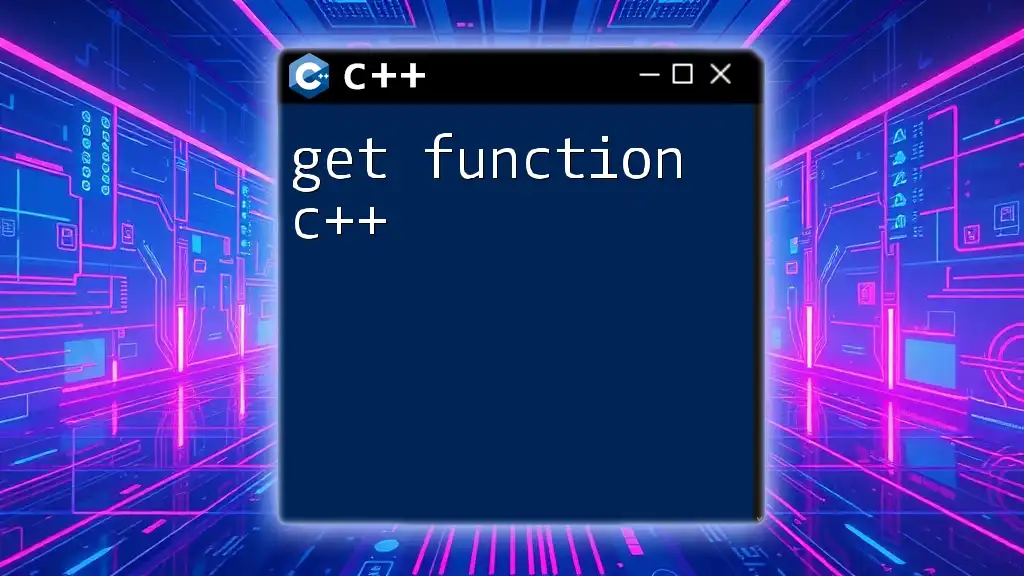
Advanced Usage of the C++ Print Function
Printing Different Data Types
C++ allows you to print various data types seamlessly. This versatility makes the print function even more valuable. For example, consider the following code:
int age = 25;
float height = 5.9;
char initial = 'A';
std::string name = "Alice";
std::cout << "Name: " << name << ", Age: " << age << ", Height: " << height << " feet." << std::endl;
This example illustrates how you can output strings, integers, characters, and floating-point numbers all in a single statement. Such flexibility is essential when dealing with multiple data types in a C++ program.
Formatting Output
Manipulators for Formatting
C++ provides manipulators to format the output whenever needed. Some common manipulators include:
- `std::endl`: Ends the current line and flushes the output buffer.
- `std::setw(int width)`: Sets the width of the next output field.
- `std::fixed`: Sets fixed-point notation for floating-point numbers.
- `std::setprecision(int n)`: Specifies the number of decimal places to display.
Here’s an example showcasing the use of manipulators:
#include <iomanip>
std::cout << std::fixed << std::setprecision(2) << "Height: " << height << " feet." << std::endl;
This code sets the output format to fixed-point notation with two decimal places, making the height display accurate and professional.
Custom Formatting: Using Width and Precision
Output width and precision are instrumental in creating visually appealing outputs. You can control how data appears on the console by combining width settings with precision. For instance:
std::cout << std::setw(10) << std::setprecision(3) << height << std::endl;
By setting width with `std::setw`, you ensure that the number occupies a specific amount of space, which can help align outputs vertically in larger applications.
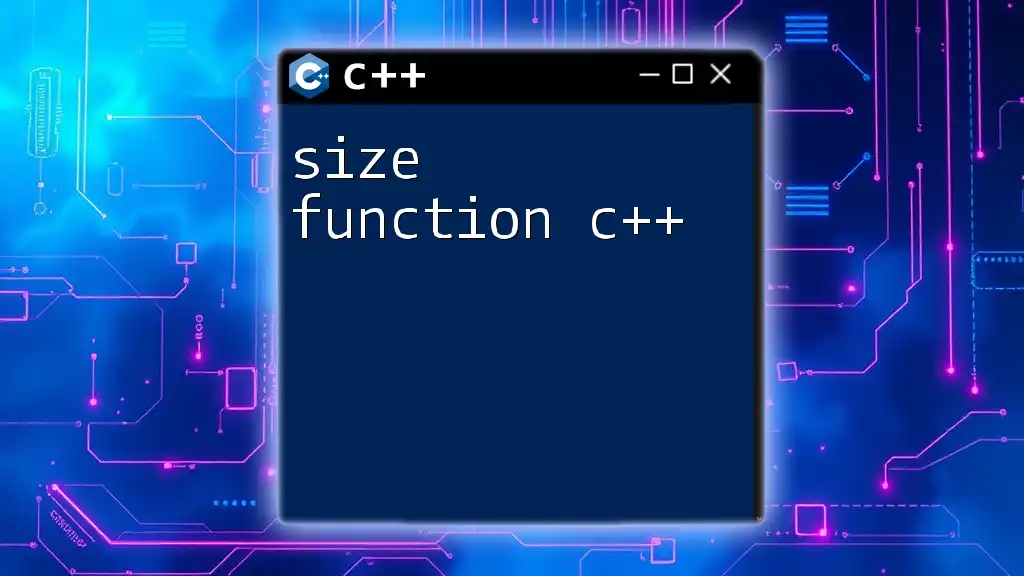
Error Handling with the Print Function
Common Errors When Using Print Functions
While using the print function in C++, developers may encounter several common issues. Examples include:
- Syntax errors caused by typos or incorrect syntax.
- Type mismatch errors when trying to output unsupported data types.
These issues can hinder compilation and runtime and should be addressed promptly.
Handling Output Errors Gracefully
Handling output errors gracefully can enhance user experience. Utilize error messages to notify users of input or runtime-related issues. By injecting checks and balances into your program, you can improve the robustness of outputs:
if (!std::cout) {
std::cerr << "Output error occurred." << std::endl;
}
This snippet checks if `std::cout` is in a good state and outputs an error message to `std::cerr` if there's a problem.
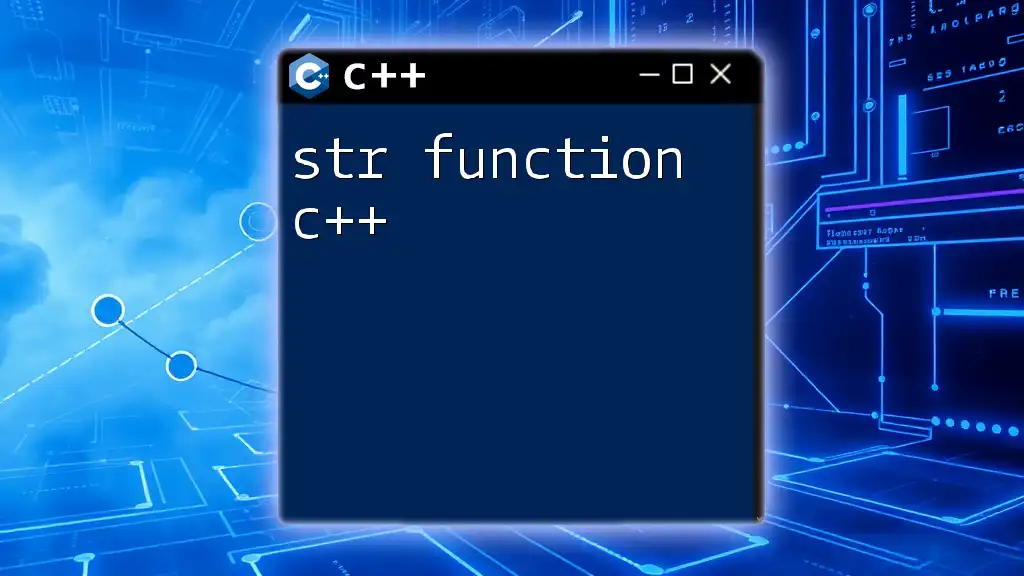
Best Practices for Using the Print Function in C++
Tips for Effective Printing in C++
To maximize the effectiveness of the print function in C++, consider these best practices:
- Keep output concise and meaningful: Ensure messages are easy to understand and relevant to the program's functionality.
- Use comments for clarity: Comment your code to guide others who may read it after you. This is vital for collaboration and maintenance.
- Structure output for better readability: Align and format your output for cleaner presentations, especially when dealing with a lot of data.
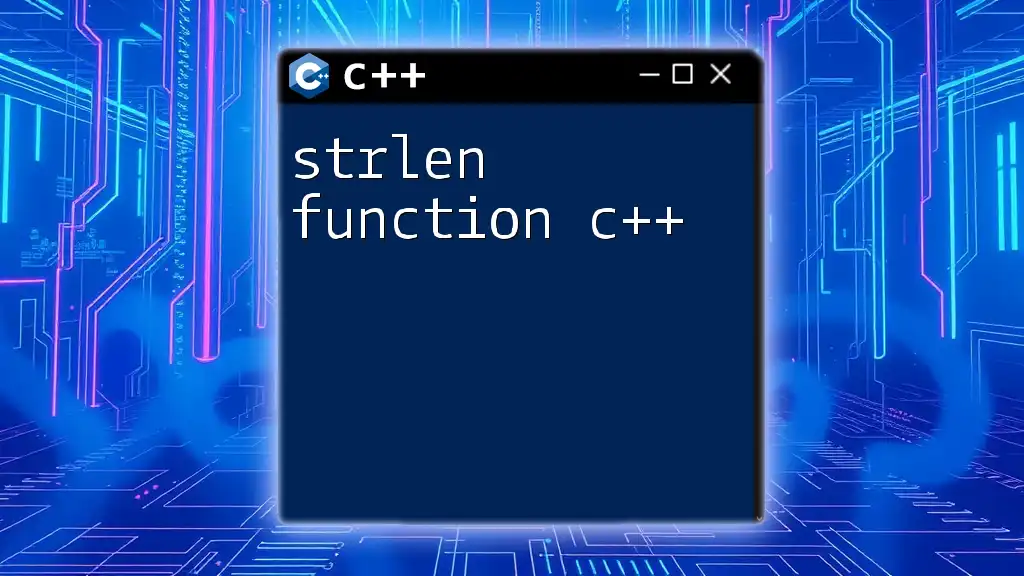
Conclusion
In this comprehensive guide, we explored the print function in C++, starting with its basic syntax and progressing into advanced usage, including formatting and error handling. By mastering these elements, you can write cleaner, more efficient C++ programs that effectively communicate information.
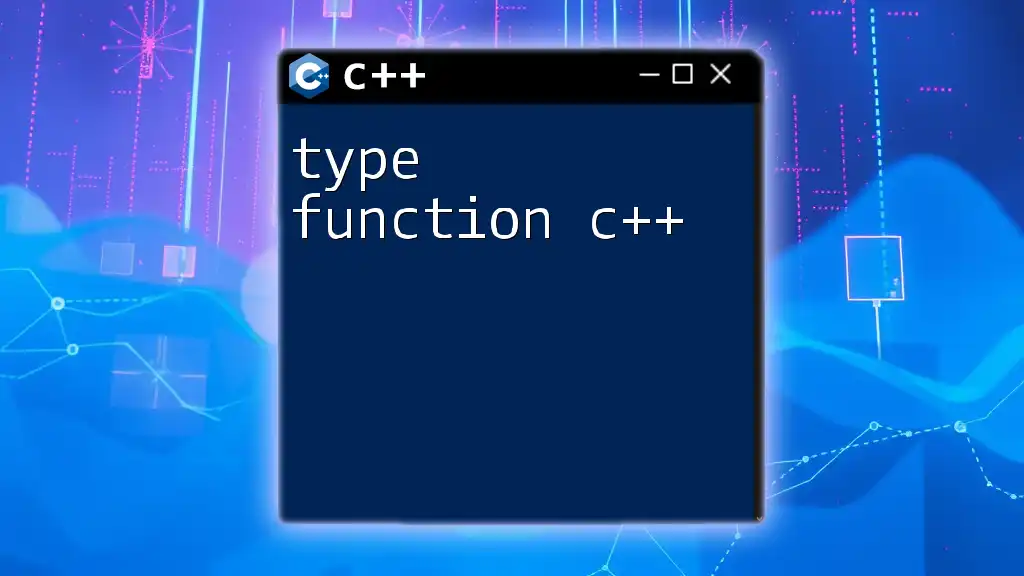
Additional Resources
References and Further Reading
For more in-depth understanding, consult the official C++ documentation at [cplusplus.com](http://www.cplusplus.com) and consider investing in authoritative C++ literature.
Community and Support
Join C++ forums, such as Stack Overflow and Reddit's r/cpp, to seek support and engage with fellow programmers to boost your learning and problem-solving skills.