The average function in C++ calculates the mean of a set of numerical values and can be implemented as follows:
double average(double arr[], int size) {
double sum = 0;
for(int i = 0; i < size; i++) {
sum += arr[i];
}
return sum / size;
}
Understanding the Average Function in C++
What is an Average?
The average, often referred to as the mean, is a statistical measure that represents the central point of a dataset. In programming, calculating the average is crucial for data analysis, performance metrics, and understanding trends. It is widely used in various applications, from academic results to financial forecasting. Therefore, having a solid grasp on how to compute the average is essential for programmers, particularly in C++.
C++ Basics for the Average Function
Before diving into the average function in C++, it's important to understand some basic programming concepts. In C++, a function is a block of code that performs a specific task. It can take inputs (parameters), process them, and return an output. Fundamental concepts like variables, data types, and loops are crucial for implementing an average function effectively.
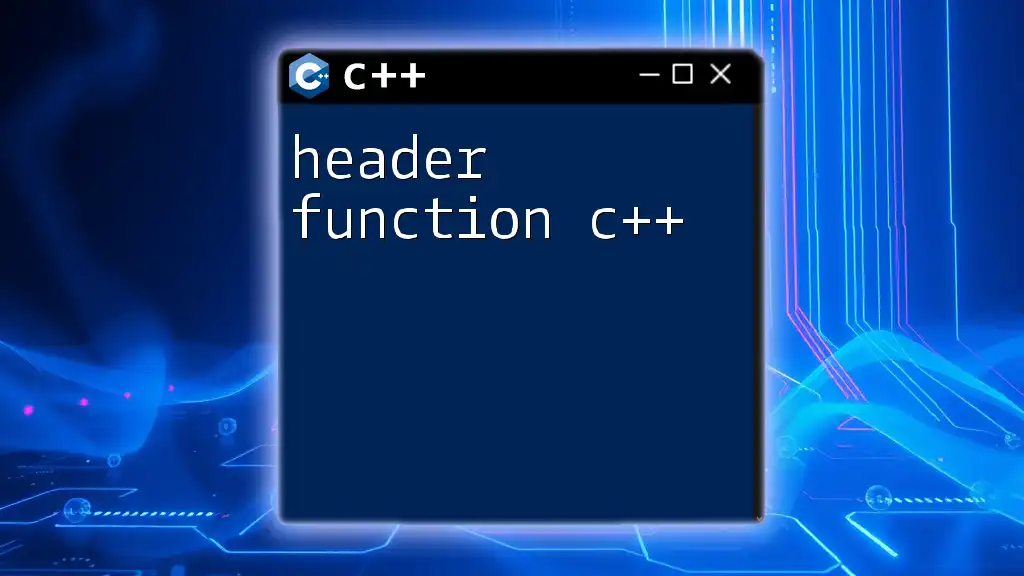
Implementing the Average Function in C++
Writing a Simple Average Function
To calculate the average using a function in C++, it’s essential to define one that takes the sum of values and the count of those values as parameters. Here’s a straightforward implementation of an average function:
double calculateAverage(int sum, int count) {
return static_cast<double>(sum) / count;
}
In this example, the function `calculateAverage` accepts two parameters: `sum` (the total of the numbers) and `count` (the number of items). The function returns the result as a `double` to ensure that the average can have decimal places. Using `static_cast<double>` ensures proper conversion and avoids integer division.
Example: Calculating the Average of an Array
Arrays are often used to store multiple values in C++. Calculating the average from an array involves iterating through its elements to find the sum and then dividing it by the size of the array. Here is how you can implement this:
#include <iostream>
using namespace std;
double calculateArrayAverage(int arr[], int size) {
int sum = 0;
for (int i = 0; i < size; i++) {
sum += arr[i];
}
return static_cast<double>(sum) / size;
}
In this code snippet, `calculateArrayAverage` takes an array and its size as inputs. It initializes a `sum` variable to zero, then uses a `for` loop to iterate over each element of the array, adding each element to the `sum`. Finally, the function returns the average value.
Explanation of the Code
In this example, the use of the `for` loop is crucial for iterating through the array elements. Each element contributes to the total sum, which is then divided by the number of elements. However, it is crucial to ensure that the array is not empty to prevent a division by zero error.
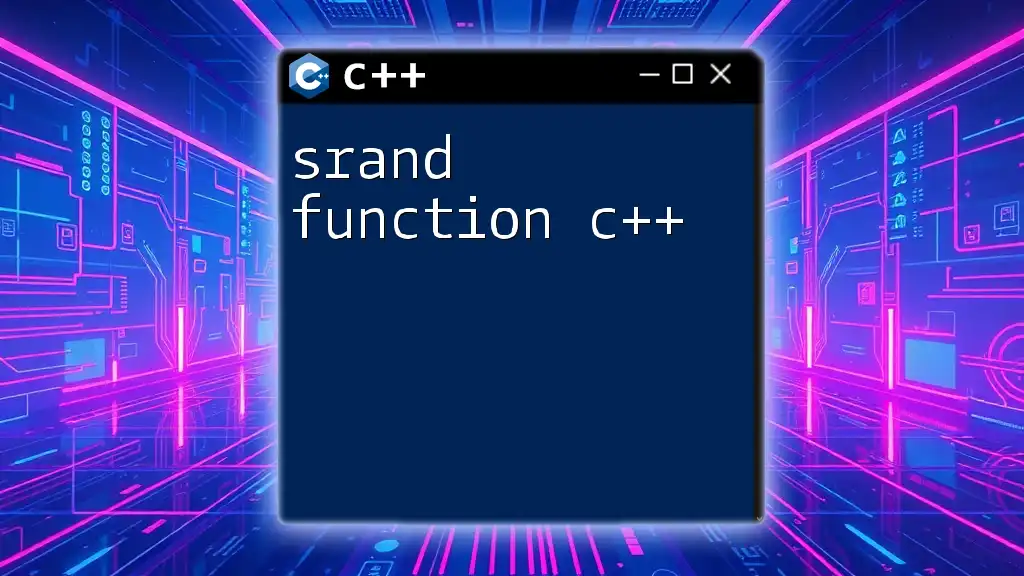
Advanced Average Calculations in C++
Using Vectors for Flexible Average Calculation
While arrays work great, vectors in C++ offer more flexibility and are dynamically resizable. Let's see how you can implement average calculation using vectors:
#include <iostream>
#include <vector>
double calculateVectorAverage(const std::vector<int>& vec) {
int sum = 0;
for (int num : vec) {
sum += num;
}
return static_cast<double>(sum) / vec.size();
}
The `calculateVectorAverage` function takes a vector as input and uses a range-based `for` loop to traverse the elements. This code is cleaner and takes advantage of C++'s powerful Standard Template Library (STL).
Detailed Breakdown of Vector Functionality
Vectors are advantageous because they can grow or shrink in size as needed, meaning you can manage memory more effectively than with static arrays. By passing the vector by reference (`const std::vector<int>&`), we avoid unnecessary copies, improving performance.
Handling User Input for Dynamic Average Calculations
User input can create a more interactive program that calculates averages based on dynamically provided data. Here’s a code snippet illustrating how to take user inputs for calculating an average from an array:
#include <iostream>
#include <vector>
double calculateAverageFromInput() {
int n, num;
std::cout << "Enter number of elements: ";
std::cin >> n;
std::vector<int> numbers;
for (int i = 0; i < n; ++i) {
std::cout << "Enter number " << i + 1 << ": ";
std::cin >> num;
numbers.push_back(num);
}
return calculateVectorAverage(numbers);
}
In this example, the function prompts the user to enter the number of elements and subsequently gathers each number into a vector. Once all numbers are input, the average is calculated using `calculateVectorAverage`.
Explanation of Input Handling
This approach involves prompting the user for data, allowing for dynamic calculations. Programmers should ensure they handle cases where the user provides invalid input or a count of zero to prevent runtime errors or logical issues.
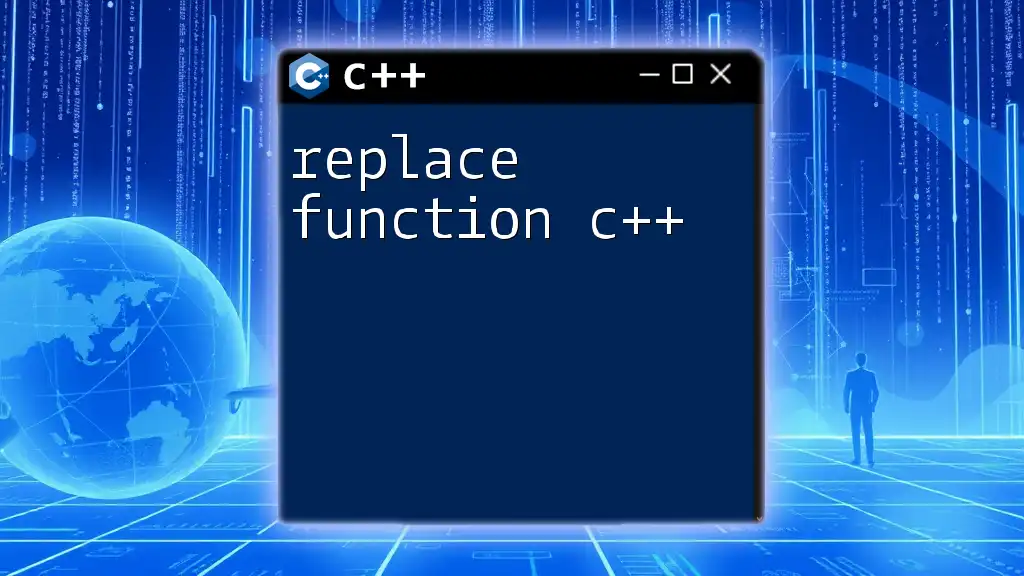
Common Pitfalls and Tips
Common Mistakes in Average Calculation
While calculating averages, it's essential to mitigate common pitfalls:
- Division by Zero Errors: Ensure your count of numbers is not zero before performing the division.
- Using Integer Division: If both numerator and denominator are integers, the result will also be an integer. Always use casting when necessary.
Tips for Accurate Average Calculation
To achieve accurate results while calculating averages in C++, keep these tips in mind:
- Make use of `static_cast<double>` to prevent integer division.
- Always check for an empty array or vector before performing calculations to avoid division by zero.
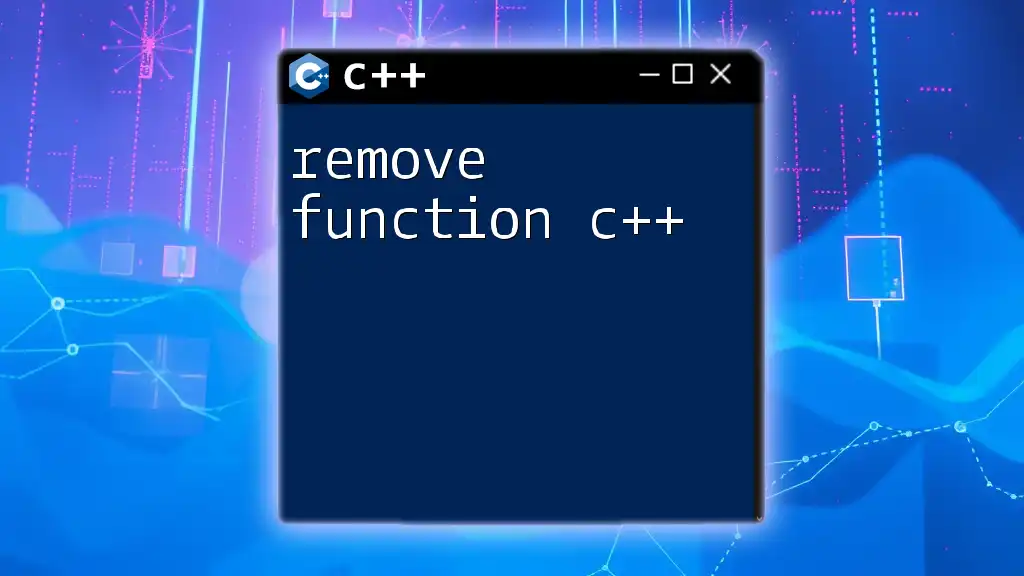
Conclusion
Understanding the average function in C++ opens up a myriad of possibilities for data processing and analysis. Mastering how to calculate averages using both arrays and vectors allows you to efficiently process sets of data. Practicing these concepts will strengthen programming skills and enhance your ability to work with numeric data effectively.
Encourage continued learning by experimenting with writing your own average functions or delving deeper into C++ functions and data analytics techniques. By harnessing these skills, you will be well-equipped to tackle complex programming challenges.