A mutator function in C++ is a member function that modifies the value of a private data member of a class, commonly known as a setter.
class Example {
private:
int value;
public:
void setValue(int newValue) {
value = newValue;
}
};
What are Mutator Functions in C++?
Definition of Mutator Function
A mutator function, often referred to as a setter function, is a member function of a class that is used to set or modify the value of a private data member. The primary purpose of a mutator function is to provide a controlled way to change the state of an object, thereby adhering to the principle of encapsulation in object-oriented programming.
Importance of Mutators
Mutators are crucial for maintaining encapsulation, which is one of the fundamental concepts of object-oriented programming (OOP). By using mutators, you can ensure that the internal state of an object can only be changed through well-defined interfaces, thereby preventing unauthorized access to the underlying data.
Using a mutator function instead of direct access aids in:
- Data Integrity: Prevents invalid data from being assigned to class members.
- Maintainability: Simplifies future changes—modifications to how data is set can be made inside the mutator without affecting other parts of your codebase.
- Flexibility: Allows additional logic—such as validation—within the mutator, ensuring that only valid data modifies the object's state.
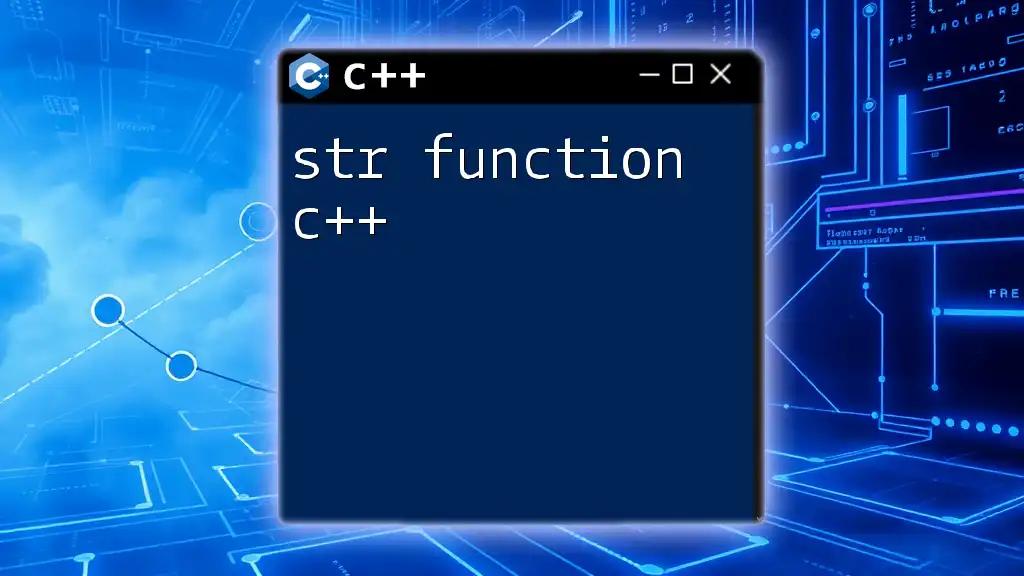
How to Implement Mutator Functions in C++
Basic Structure of a Mutator Function
Implementing a mutator function follows a simple structure. The function typically includes the `void` return type, reflecting that it performs an action rather than returning a value. It accepts parameters necessary to set the private data member.
Here’s a basic example demonstrating this:
class MyClass {
private:
int myValue;
public:
void setMyValue(int value) {
myValue = value;
}
};
Example of a Simple Class with a Mutator
Let's explore a complete example with an `Employee` class that uses mutator functions to set the employee's name and age.
class Employee {
private:
std::string name;
int age;
public:
void setName(const std::string &newName) {
name = newName;
}
void setAge(int newAge) {
if (newAge > 0) { // Simple validation
age = newAge;
}
}
};
Explanation of the Example
In the `Employee` class, we have two private attributes: `name` and `age`. The mutator functions `setName` and `setAge` allow controlled modifications:
- `setName` directly assigns a new name to the `name` member.
- `setAge` checks if the new age is positive before assigning it to ensure the integrity of the `age` attribute.

Mutators vs. Accessors in C++
What are Accessor Functions?
Accessor functions, commonly known as getter functions, are another important type of member function used to retrieve private data members of a class. They complement mutator functions by providing a way for external code to obtain data without directly accessing class fields.
Importance of Accessors
Accessors promote encapsulation by ensuring that the internal representation of an object cannot be changed via external code. By using accessors, you can:
- Safeguard the integrity of data.
- Change the internal implementation without affecting the code that relies on these accessors.
Example of Accessor Functions in C++
Here's how you can also implement accessors for the `Employee` class:
class Employee {
private:
std::string name;
int age;
public:
void setName(const std::string &newName) { name = newName; }
void setAge(int newAge) { if (newAge > 0) age = newAge; }
std::string getName() const { return name; }
int getAge() const { return age; }
};
Accessors and Mutators: The Complete Picture
Together, accessors and mutators establish a clear contract for how the data members of a class should be handled. Accessors allow for safe retrieval, while mutators regulate modifications, which help keep the data consistent and reliable throughout its lifecycle.
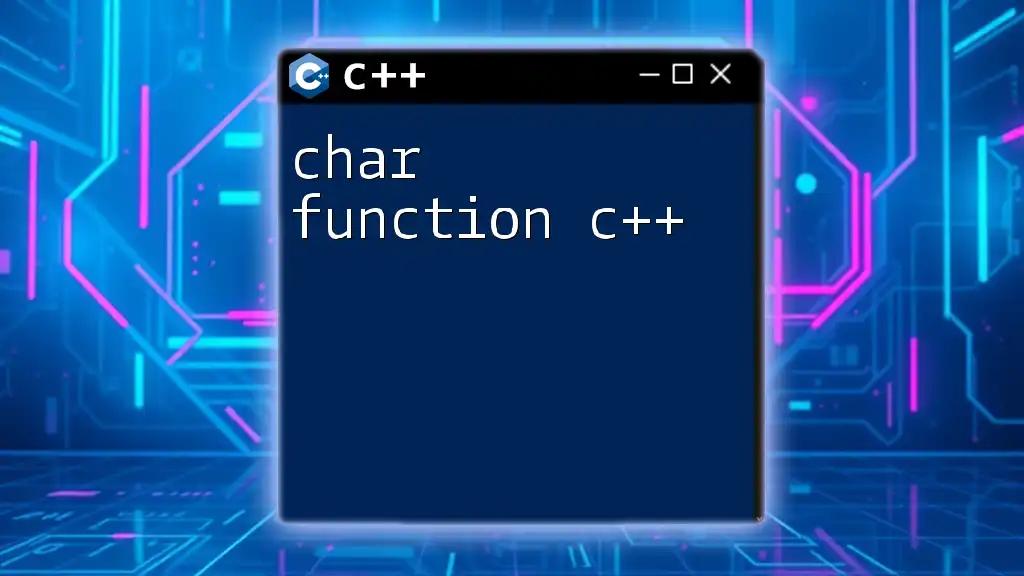
Best Practices for Implementing Mutator Functions
Naming Conventions
When naming your mutator methods, a widely accepted convention is to begin the method names with the prefix "set", followed by the name of the variable. For instance, `setName` and `setAge` are intuitive and immediately indicate their purpose. Following conventions like this increases code readability.
Validation Inside Mutators
Including validation logic within your mutator functions is paramount. By ensuring that the data being set is valid, you prevent potential future errors from propagating through your application. This practice safeguards the integrity of your objects.
Consistency Planning
Make sure your mutator methods remain consistent with the business logic you aim to apply. This consistency leads to fewer bugs and a more maintainable code base.

Common Mistakes to Avoid with Mutator Functions
Direct Variable Access
One of the most significant mistakes in C++ programming is bypassing mutator functions and directly accessing class members. This practice undermines encapsulation, making it easier for invalid or unexpected values to compromise object integrity.
Lack of Validation
Failing to include validation checks within your mutator functions can have dire consequences. If a bad value is assigned, it can lead to logic errors or crashes later in your program's execution timeframe.
Overusing Mutators
While mutators are essential, relying too heavily on them can complicate class design. Aim for simplicity and balance in your classes to avoid convoluted structures that are hard to maintain.
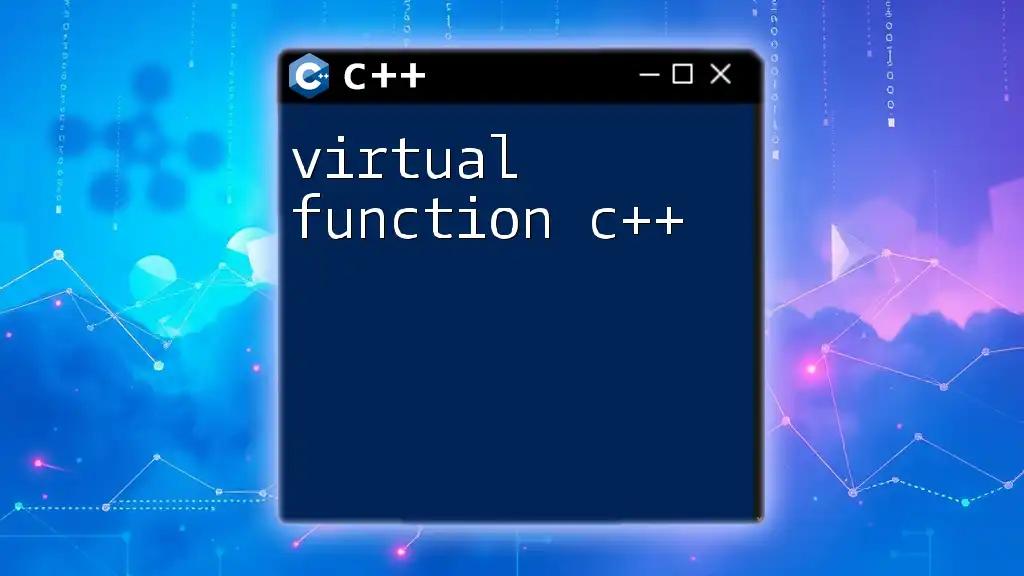
Conclusion
Mutator functions in C++ are vital for creating robust and maintainable code in an object-oriented programming paradigm. They help to ensure that class attributes can only be changed in a controlled manner, providing numerous benefits such as data integrity, flexibility, and adherence to the principles of encapsulation. By understanding and implementing both mutators and accessors effectively, you can significantly improve your C++ programming practices.

Further Reading
For those looking to deepen their understanding of accessors and mutators in C++, consider exploring articles on advanced OOP concepts, joining community forums, and engaging with coding platforms that offer hands-on practice and expert advice. The knowledge you gain will further enrich your software development skills!