The `std::function` in C++ is a versatile template class that encapsulates callable objects, such as functions, lambda expressions, or function objects, allowing you to store and pass them as first-class objects.
Here's a simple code snippet demonstrating its use:
#include <iostream>
#include <functional>
int main() {
std::function<int(int, int)> add = [](int a, int b) { return a + b; };
std::cout << "Sum: " << add(3, 4) << std::endl; // Output: Sum: 7
return 0;
}
What is std::function?
`std::function` is a versatile and powerful component of C++ that is part of the Standard Template Library (STL). It serves as a generalized function wrapper, allowing you to store, pass around, and invoke callable entities. This includes regular functions, lambda expressions, bind expressions, or even other function objects.
Why Use std::function?
The prominence of `std::function` in modern C++ programming arises from its flexibility. It allows for type-erasure, meaning you can create a uniform interface to handle a variety of callable objects without worrying about their specific types. This is particularly useful when designing APIs where the exact callable entity may not be known in advance.
In contrast to traditional function pointers, `std::function` offers a type-safe, more user-friendly interface that eliminates many of the limitations associated with the use of function pointers. You can capture state, pass arguments, and even define callables inline with lambdas, enhancing both readability and maintainability.
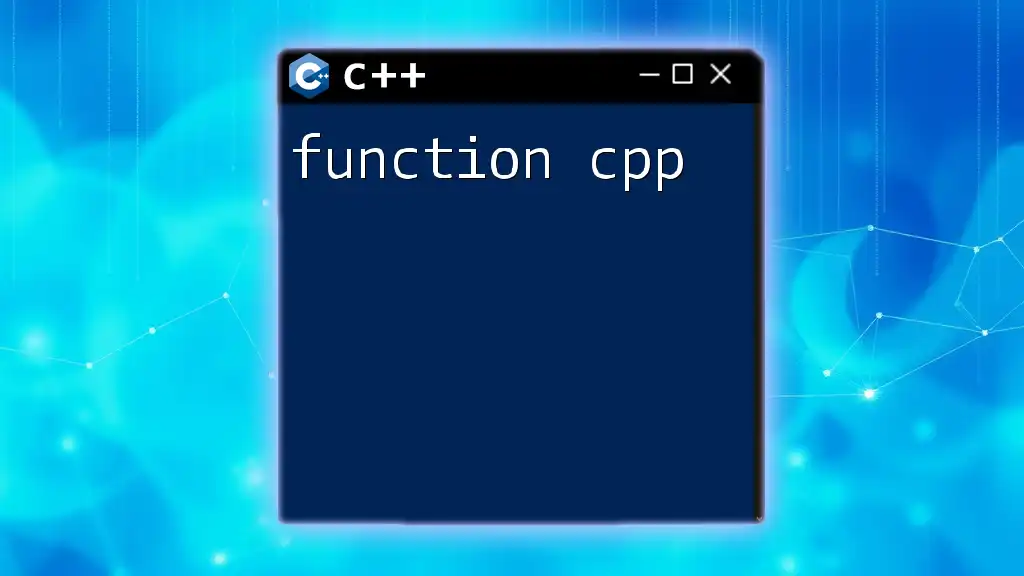
Understanding the Basics of std::function
Syntax of std::function
The basic syntax for declaring a `std::function` involves specifying the return type and the argument types within angle brackets. Here’s a simple example:
#include <functional>
#include <iostream>
std::function<void(int)> func;
In this case, `func` can store any callable that takes an `int` as an argument and has no return value.
How to Include std::function
To use `std::function`, you must include the appropriate header in your program:
#include <functional>
This inclusion is crucial because `std::function` and other related functionalities are defined in the `<functional>` header.
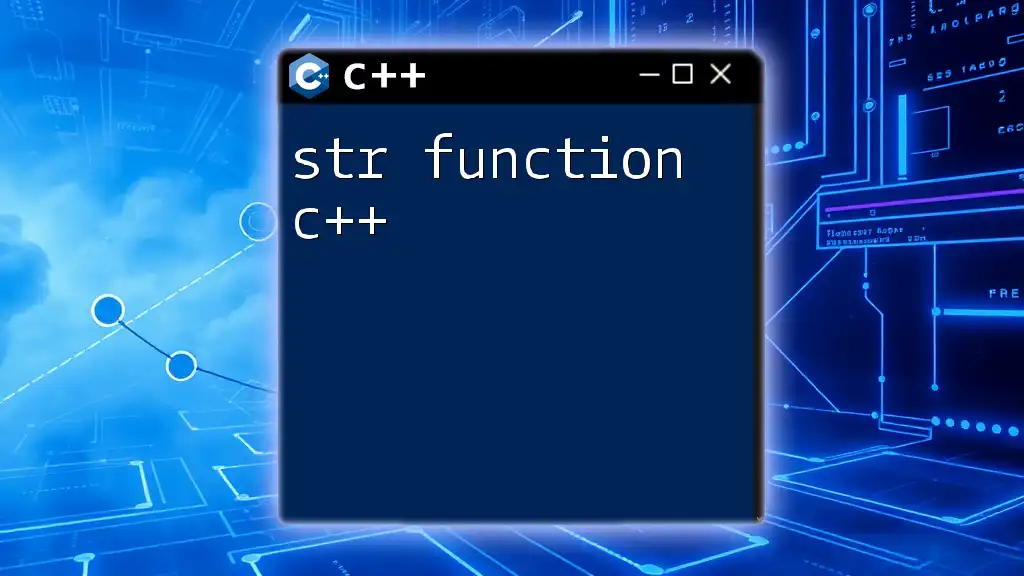
Creating std::function Objects
Instantiating std::function
To create a `std::function` object, you can assign it a callable entity. This can be a regular function, lambda, or any other callable. Here’s how to instantiate a `std::function`:
void myFunction(int x) {
std::cout << "Value: " << x << std::endl;
}
std::function<void(int)> func = myFunction;
func(10); // Output: Value: 10
Using Lambda Functions with std::function
Lambda functions introduce a more concise way to create callable objects in C++. You can directly assign a lambda to a `std::function`. Here’s an example:
std::function<void(int)> lambdaFunc = [](int x) {
std::cout << "Lambda Value: " << x << std::endl;
};
lambdaFunc(20); // Output: Lambda Value: 20
Using lambdas enhances the expressiveness of your code, allowing inline definitions that are both clear and powerful.
Binding Arguments and std::function
You can bind specific arguments to a function using `std::bind`. This can be useful when you want to create a new callable that has some preset parameters. Here’s a demonstration:
#include <functional>
void add(int a, int b) {
std::cout << "Sum: " << (a + b) << std::endl;
}
auto boundFunction = std::bind(add, 5, std::placeholders::_1);
boundFunction(10); // Output: Sum: 15
In this example, `boundFunction` takes one argument, passing it along with the pre-defined `5` to the `add` function.
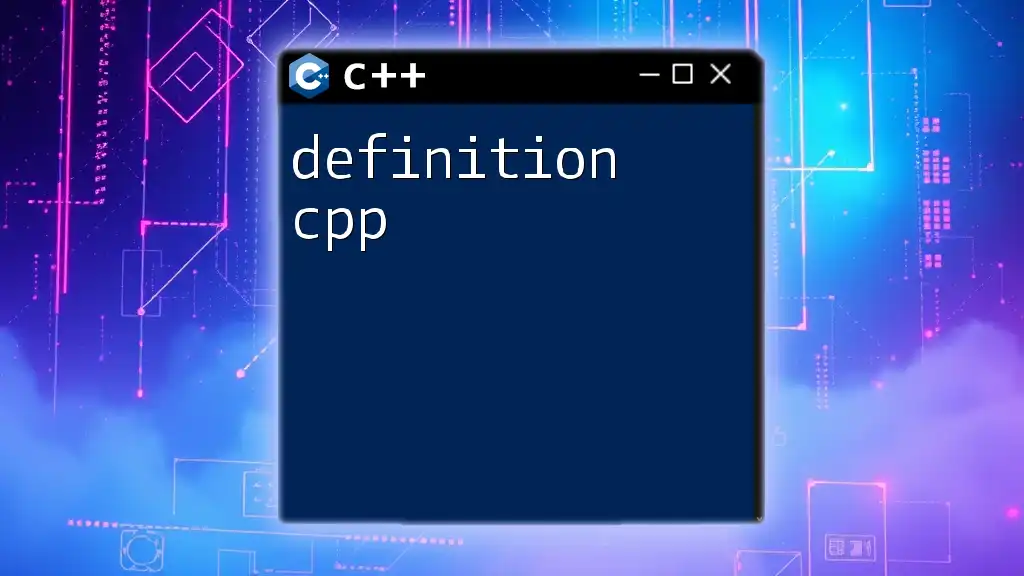
Practical Applications of std::function
std::function in Callbacks
Callbacks are a common use case for `std::function`, enabling a clean separation of event handling or response function from the triggering code. Here’s how to implement a simple callback mechanism:
void executeCallback(std::function<void()> callback) {
std::cout << "Executing callback:" << std::endl;
callback();
}
void myCallback() {
std::cout << "Callback executed!" << std::endl;
}
int main() {
executeCallback(myCallback); // Output: Callback executed!
}
std::function in STL Algorithms
`std::function` integrates seamlessly with STL algorithms, allowing you to use custom operations. For instance, you can use it with `std::for_each`:
#include <vector>
#include <algorithm>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::function<void(int)> print = [](int n) { std::cout << n << " "; };
std::for_each(numbers.begin(), numbers.end(), print);
// Output: 1 2 3 4 5
}
Storing Multiple Functions in Containers
With `std::function`, you can easily store multiple function objects in STL containers like vectors or maps. Here’s how to accomplish this:
#include <vector>
#include <functional>
#include <iostream>
int main() {
std::vector<std::function<void()>> functions;
functions.push_back([]() { std::cout << "Function 1" << std::endl; });
functions.push_back([]() { std::cout << "Function 2" << std::endl; });
for (const auto& func : functions) {
func();
}
// Output:
// Function 1
// Function 2
}
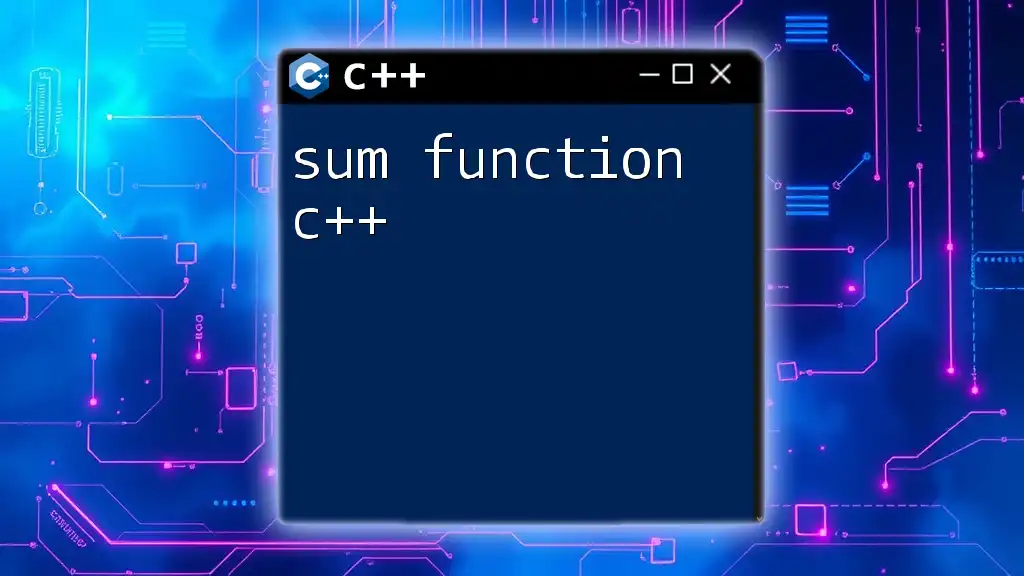
Advanced Usage of std::function
Performance Considerations
While `std::function` offers flexibility, it does introduce some overhead. It has dynamic memory allocation, which may impact performance in time-critical code. To mitigate this, prefer using simpler callable types when performance is paramount, such as lambdas or regular function pointers.
std::function vs Other Function Objects
When considering alternatives, `std::bind` and lambdas also provide callable functionality but do so in different ways. `std::function` stands out when you require a uniform way to handle various callable types, while lambda functions can offer better performance due to their lightweight nature in specific scenarios.
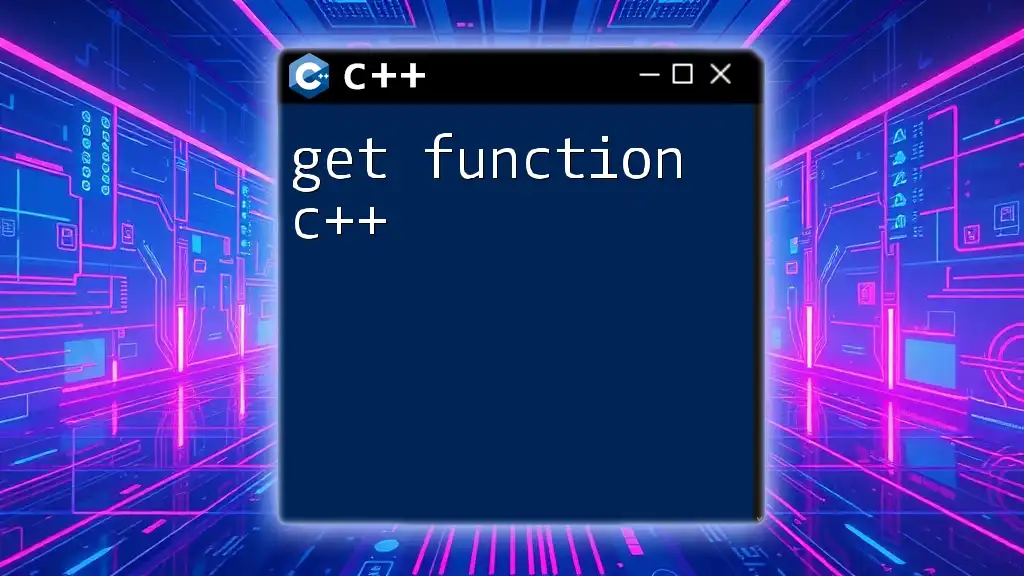
Common Mistakes and Best Practices
Common Pitfalls When Using std::function
One common mistake is failing to consider there can be overhead with using `std::function`, especially in time-sensitive applications. Additionally, if using a lambda that captures large objects, be mindful of the potential performance hit.
Best Practices for Using std::function
To maintain clean and efficient code when using `std::function`:
- Use it when you truly need type erasure. For polymorphic behavior in callable objects, it’s ideal.
- Prefer direct lambdas wherever possible for better performance.
- Avoid unnecessary bindings to reduce overhead.
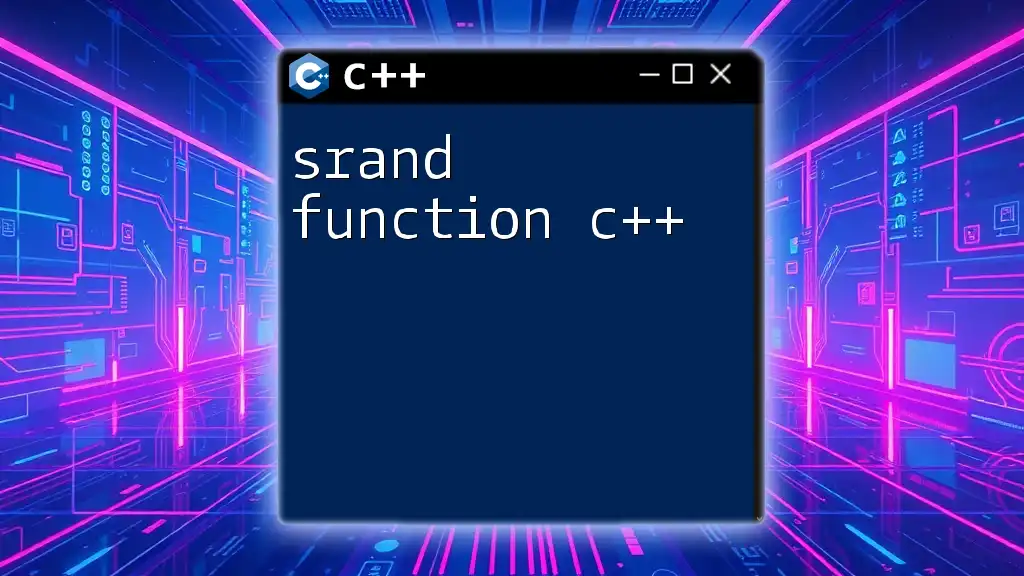
Conclusion
`std::function` is an invaluable tool in C++, bridging the gap between diverse callable types with its flexible and type-safe interface. Mastering it can significantly enhance your programming capabilities, providing greater abstraction and modularity to your code.
Resources for Further Learning
For those looking to deepen their understanding of `std::function`, consider exploring online C++ tutorials, documentation, or advanced programming books that cover the Standard Template Library in greater detail. Engaging with real-world projects can also expedite your learning process.
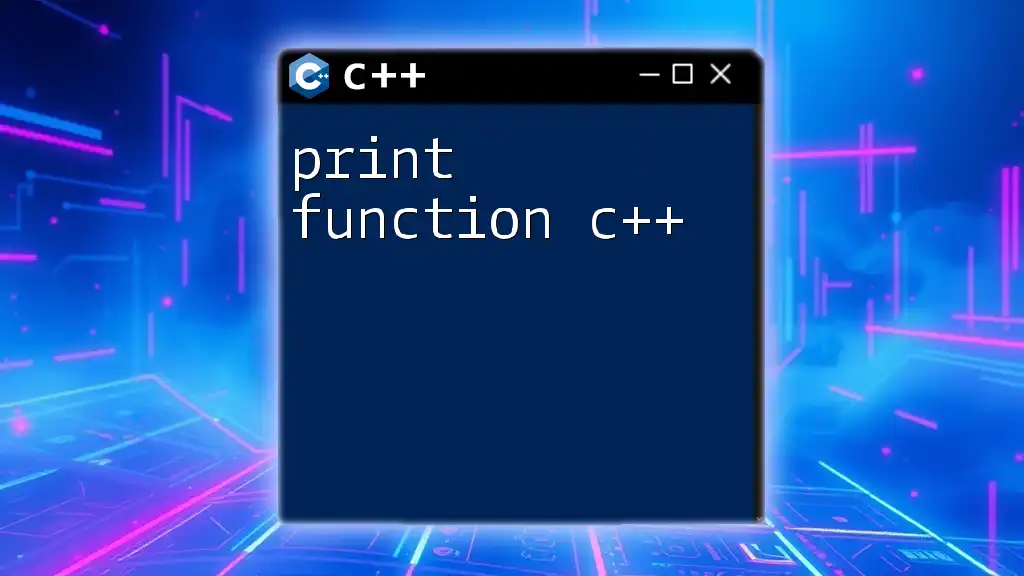
Call to Action
To truly grasp the power of `std::function`, start experimenting with it in your own projects. Implement callbacks, leverage it in STL algorithms, or store multiple callable types in containers. The more you practice, the more intuitive its use will become!