`std::vector` is a dynamic array provided by the C++ Standard Library that can grow and shrink in size, allowing efficient insertion and deletion of elements.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.push_back(6); // Adding an element
for (int num : numbers) {
std::cout << num << " "; // Output: 1 2 3 4 5 6
}
return 0;
}
What is std::vector?
`std::vector` is a dynamic array provided by the C++ Standard Library. It can grow and shrink in size automatically as elements are added or removed. This makes it a versatile and powerful tool in C++ programming, offering flexibility compared to static arrays and simpler management than linked lists. The dynamic size feature allows programmers to handle data of varying length more easily.
In comparison to other data structures, `std::vector` offers benefits such as efficient memory management, ease of access, and built-in functions for manipulation that arrays and lists may lack.
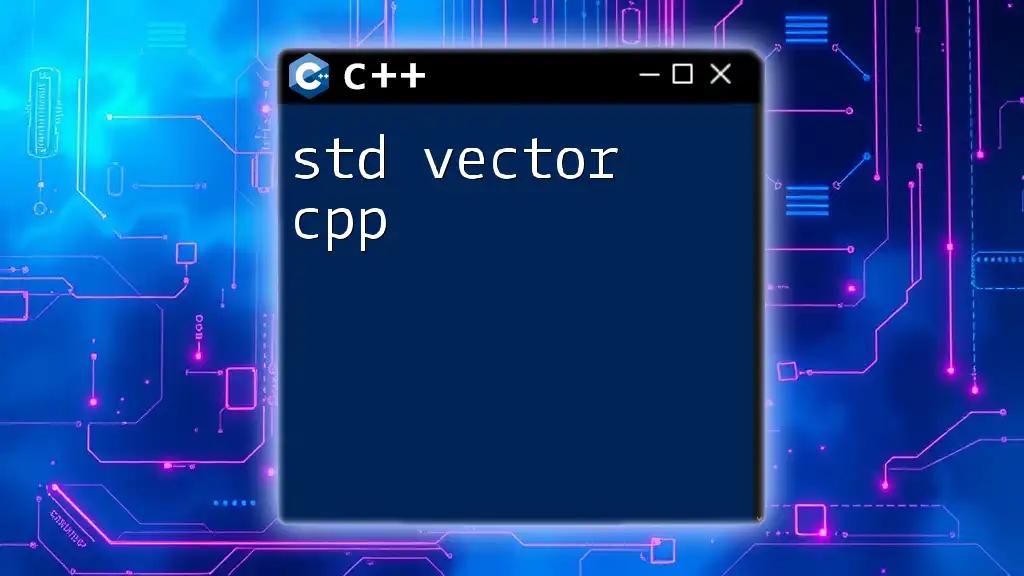
Creating a std::vector
Including Necessary Header Files
Before using `std::vector`, it's essential to include the relevant header file:
#include <vector>
This line ensures that the vector class is available for use in your program.
Declaring a Vector
Declaring a vector is straightforward. Here’s how you can create an empty vector and a vector with initial values:
std::vector<int> myVector; // Empty vector
std::vector<int> initializedVector = {1, 2, 3, 4, 5}; // Initialized with values
In the first example, `myVector` is declared but starts with no elements. In the second example, `initializedVector` is created with five integer elements.
Specifying the Vector Size
You can create a vector of a specific size and assign a default value to each element using the following syntax:
std::vector<int> fixedSizeVector(10, 0); // A vector of size 10, initialized with zeros
This code creates a vector of size 10, where each element is initialized to zero.
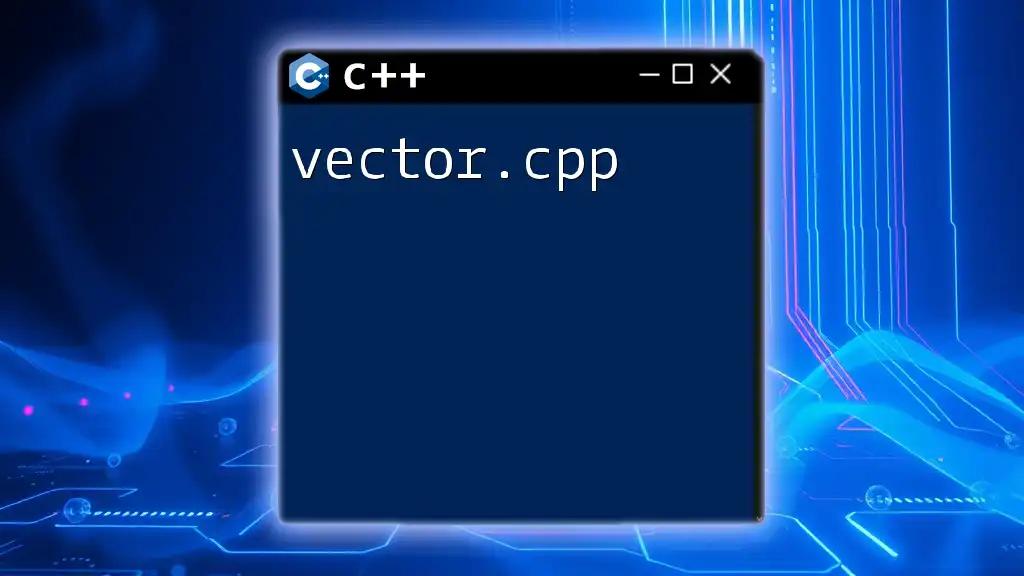
Modifying std::vector Content
Adding Elements to a Vector
To add elements to a vector, you typically use the `push_back()` method:
myVector.push_back(10);
myVector.push_back(20);
This method appends the specified value to the end of the vector. It is efficient, maintaining the order of the elements.
Another option for adding elements at specific positions is using the `insert()` method:
initializedVector.insert(initializedVector.begin() + 2, 99); // Inserts 99 at index 2
In this example, `99` is inserted between the existing elements.
Removing Elements from a Vector
Removing elements can be done using `pop_back()` which removes the last element:
myVector.pop_back(); // Removes the last element
For removing a specific element or a range, you can use `erase()`:
initializedVector.erase(initializedVector.begin() + 1); // Removes the element at index 1
It's important to understand that erasing an element may invalidate iterators pointing to elements following the removed one.
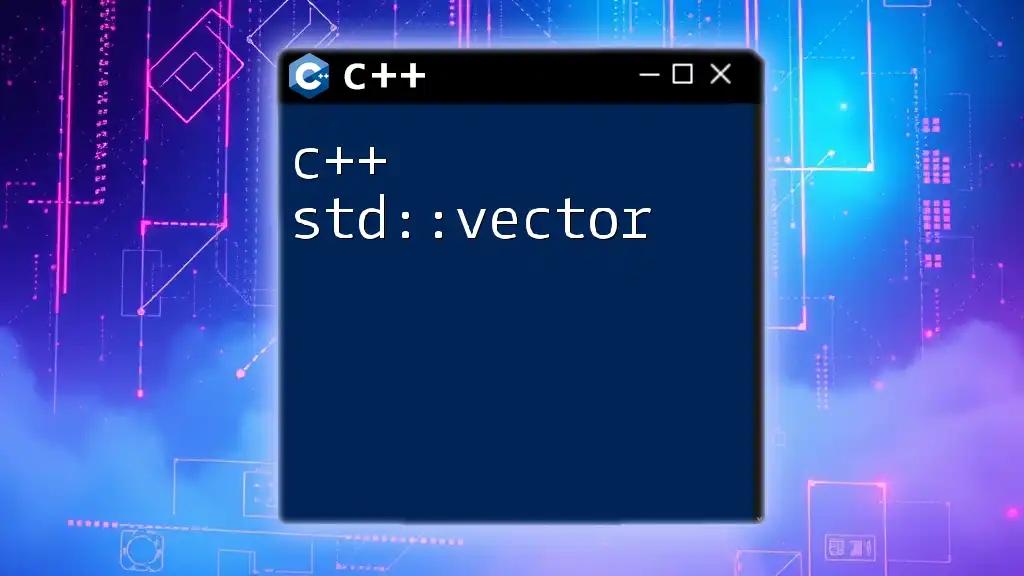
Accessing Vector Elements
Using the Index Operator `[]`
You can access elements directly by their index using the index operator:
int value = initializedVector[0]; // Accesses the first element
However, be cautious with this method, as it does not perform bound checking, potentially leading to undefined behavior if the index is out of range.
Using `at()` Method
An alternative way to access elements is through the `at()` method, which provides safety through bounds checking:
int safeValue = initializedVector.at(1); // Accesses the second element
If the index is out of bounds, `at()` throws an `std::out_of_range` exception, making it a more robust option for accessing elements.
Using Iterators
Iterators are a powerful feature of C++ that allow you to traverse a vector:
for (std::vector<int>::iterator it = initializedVector.begin(); it != initializedVector.end(); ++it) {
std::cout << *it << " "; // Outputs each element
}
This method gives you fine control over element access and is preferred in many C++ applications.
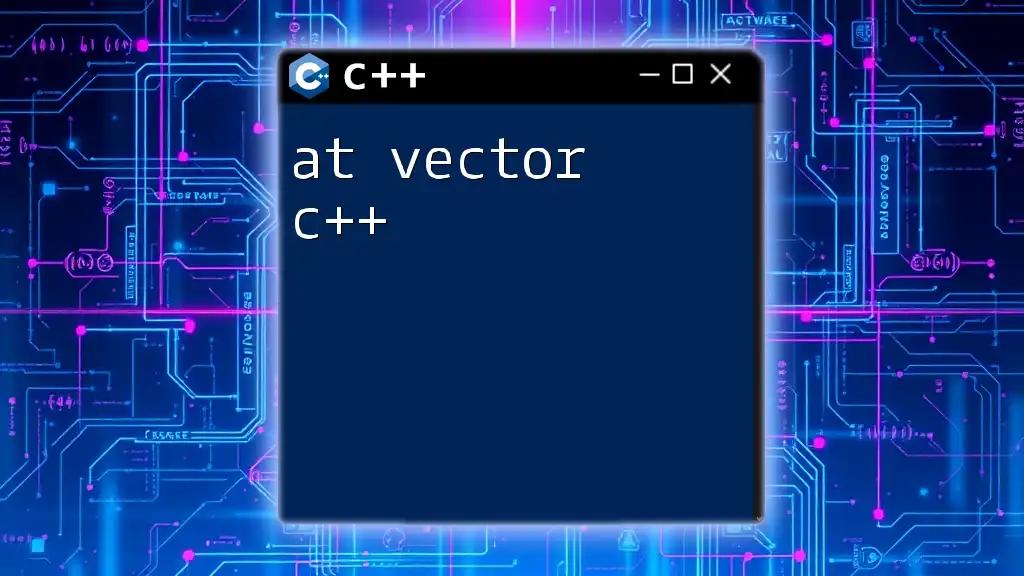
Resizing and Shrinking Vectors
Resizing a Vector
You can change the size of a vector dynamically using the `resize()` method:
initializedVector.resize(8); // Resizes to 8 elements, filling new slots with 0
If the new size is larger than the current, the new elements will be initialized to zero.
Shrinking a Vector
To reduce unused memory, you can call `shrink_to_fit()`:
initializedVector.shrink_to_fit(); // Requests the reduction of capacity to fit the current size
This method is not guaranteed to reduce the size but is a good practice for managing memory effectively.
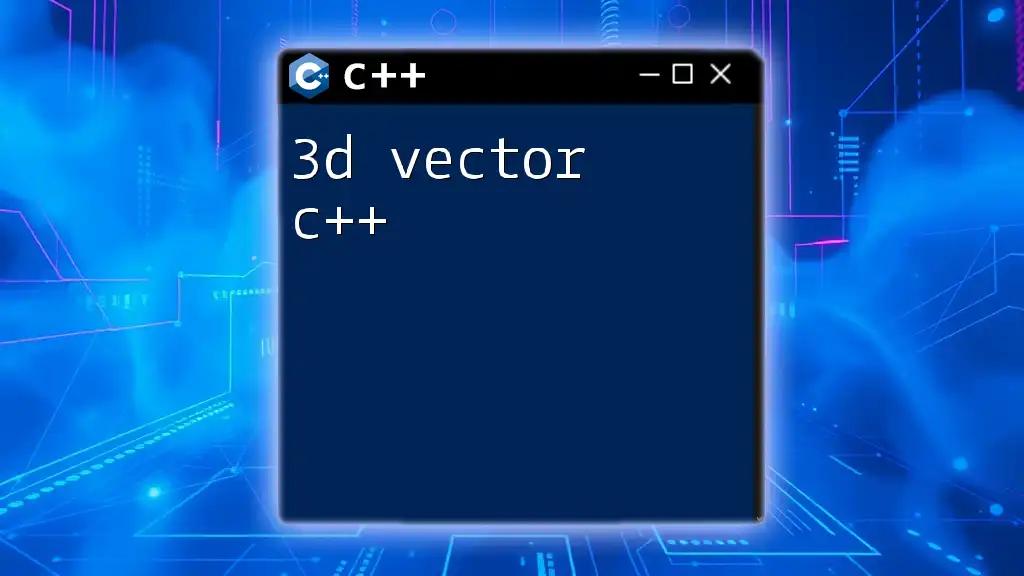
Capacity and Performance of std::vector
Understanding Capacity vs. Size
The size of a vector refers to the number of elements currently in it, while the capacity refers to the total amount of memory allocated for the vector. You can check these values using:
size_t currentSize = initializedVector.size(); // Returns the current number of elements
size_t currentCapacity = initializedVector.capacity(); // Returns the allocated capacity
It's essential to grasp this distinction, especially when performance is a consideration.
Reserve Method
To optimize performance, consider reserving space if you know the number of elements to expect. This prevents multiple memory reallocations:
myVector.reserve(100); // Reserves space for 100 elements
This proactive approach results in better performance during extensive insertions.
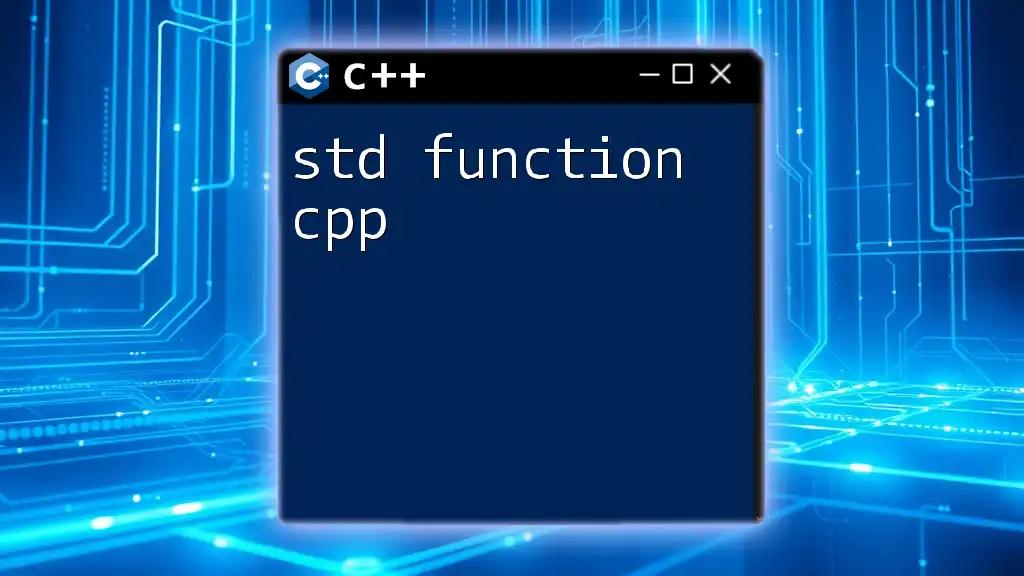
Common Use Cases for std::vector
When to Use std::vector?
`std::vector` is ideal for situations where you need dynamic sizing, easy access to elements, and efficient storage. Common use cases include managing lists of items, implementing stacks or queues, and handling arrays where size changes during runtime.
Comparing std::vector with Other Containers
When deciding between `std::vector` and other containers, consider:
- std::array: Fixed size, no dynamic resizing, but more performance-efficient for known sizes.
- std::list: Ideal for frequent insertions and deletions, but offers slower access times because of sequential access.
Each container has its pros and cons, and choosing the right one depends on specific requirements.
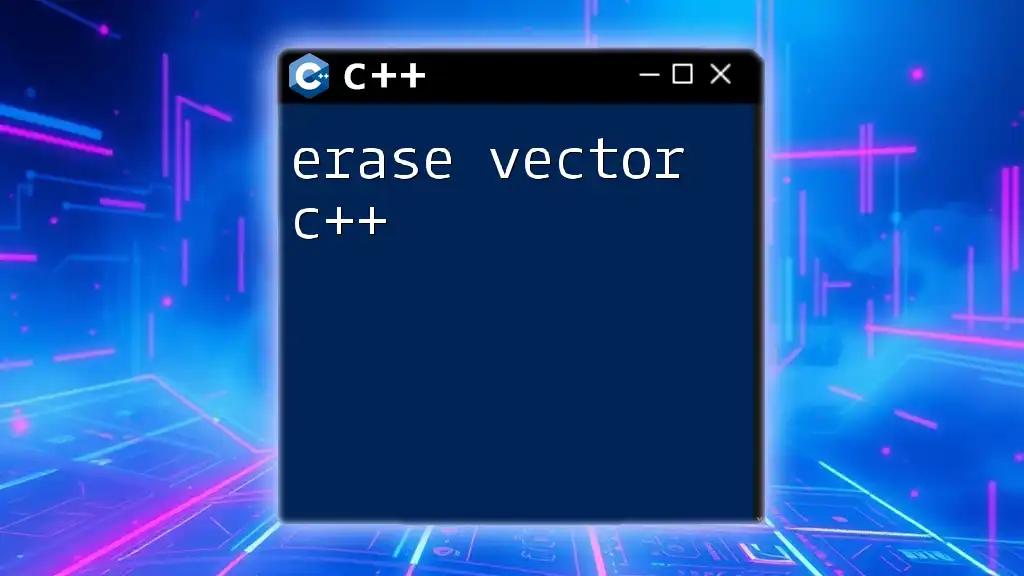
Advanced Features of std::vector
Copying and Assigning Vectors
Vectors can be copied using the assignment operator or copy constructor, ensuring all elements are copied correctly:
std::vector<int> copiedVector = initializedVector; // Copy constructor
This creates a new vector that mirrors the contents of the original.
Vectors of Vectors
You can create a vector of vectors (2D vector) for more complex data structures:
std::vector<std::vector<int>> matrix(3, std::vector<int>(4, 0)); // 3x4 matrix initialized to 0
This example initializes a 3x4 grid where each element is set to zero, suitable for representing matrices or grids.

Conclusion
In summary, `std::vector` is one of the most powerful tools in C++ to handle dynamic arrays. It combines ease of use with powerful features that support effective memory management, element manipulation, and intuitive access patterns.
By understanding how to effectively use `std::vector`, you can enhance your C++ programming skills and write more efficient, cleaner code. Don’t hesitate to explore the additional functionalities of vectors, experiment with the examples provided, and leverage the power of `std::vector` in your projects!
For further exploration, check out the C++ Standard Library documentation and engage with community resources to deepen your understanding and skills.