In C++, an `std::vector` is a dynamic array that can resize itself automatically when elements are added or removed, enabling efficient management of a collection of elements.
Here's a simple example of how to declare and initialize an `std::vector`:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5}; // Initializing a vector of integers
for (int num : numbers) {
std::cout << num << " "; // Output: 1 2 3 4 5
}
return 0;
}
Overview of `at()` Function in C++
What is the `at()` Method?
The `at()` method in C++ is a member function of the `std::vector` class used to access elements by their index. Unlike the subscript operator `[]`, which does not perform bounds checking, `at()` checks if the index is valid before accessing the element. This makes `at()` a safer choice when working with vectors, especially for beginners who may not be familiar with potential out-of-bounds errors.
Syntax of the `at()` Method
The basic syntax for using the `at()` method is straightforward:
vector_name.at(index);
Where `vector_name` is the name of your vector and `index` is the position of the element you wish to access.
For example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::cout << "Element at index 2: " << numbers.at(2) << std::endl; // Output: 3
return 0;
}
In this snippet, the `at()` method retrieves the element at index 2, which is 3.
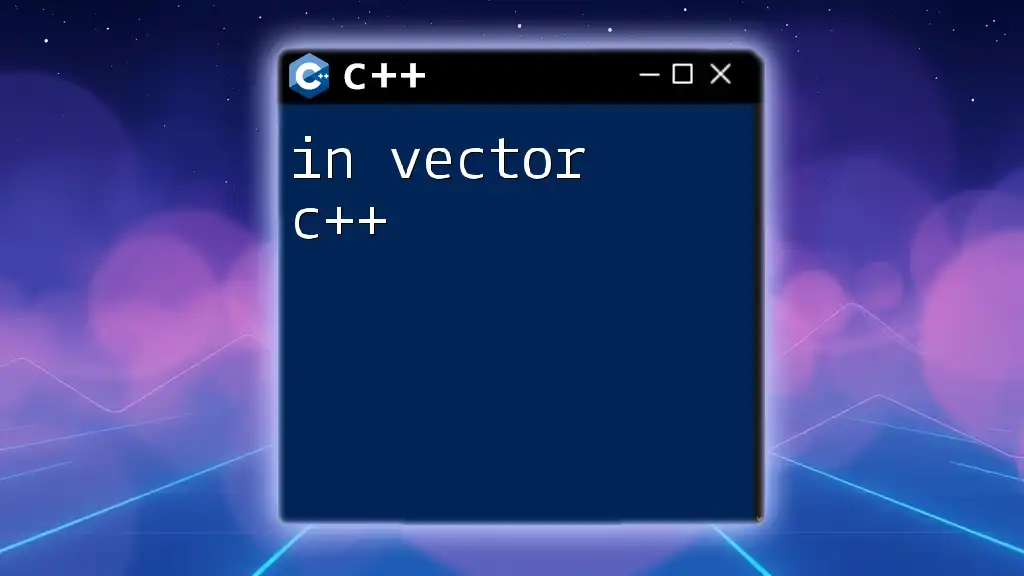
How to Use `at()` with C++ Vectors
Accessing Elements Using `at()`
Using the `at()` method is valuable for accessing elements safely. If you try to access an out-of-bounds index, `at()` will throw a `std::out_of_range` exception, enabling your program to handle such errors gracefully.
Here’s an example demonstrating how to use `at()` to access a vector element:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {10, 20, 30, 40, 50};
std::cout << "Element at index 3: " << numbers.at(3) << std::endl; // Output: 40
return 0;
}
Using `at()` to Modify Elements
You can also use `at()` to modify elements in a vector, just like you would with the subscript operator:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {10, 20, 30, 40, 50};
numbers.at(1) = 25; // Modify the second element
std::cout << "Modified element at index 1: " << numbers.at(1) << std::endl; // Output: 25
return 0;
}
Here, the second element (index 1) is changed from 20 to 25, showcasing how you can update values safely with `at()`.
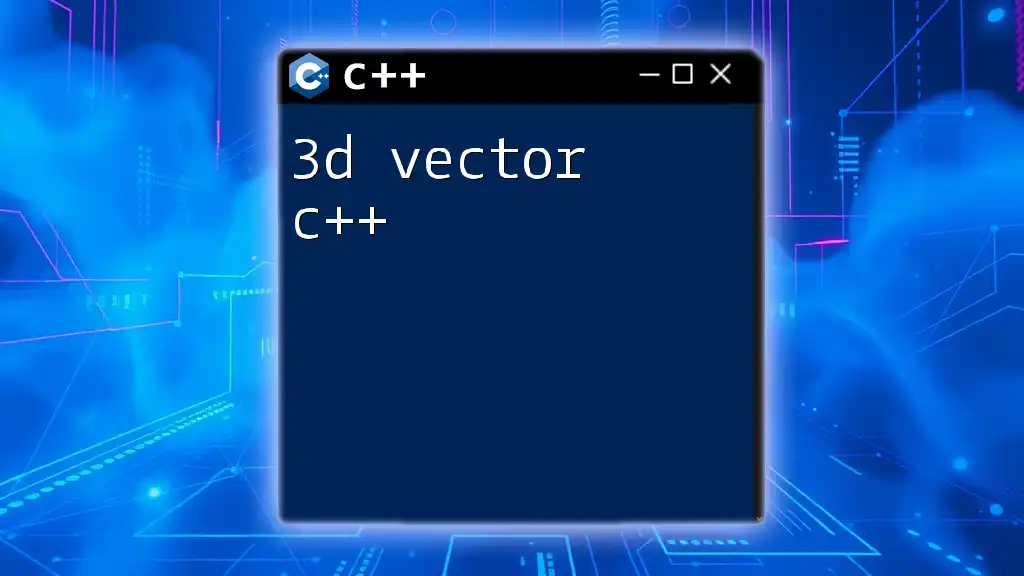
Exception Handling with `at()`
Out-of-Bounds Access
One of the most significant advantages of using `at()` is its built-in exception handling. If you attempt to access an index that exceeds the vector's size, the program will throw an exception rather than causing undefined behavior.
Consider the following example, which demonstrates how to handle such exceptions:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3};
try {
std::cout << numbers.at(10) << std::endl; // Out of bounds
} catch (const std::out_of_range& e) {
std::cerr << "Exception: " << e.what() << std::endl; // Output error message
}
return 0;
}
In this case, attempting to access `numbers.at(10)` will lead to an exception, and the catch block will handle this gracefully by printing a meaningful error message.
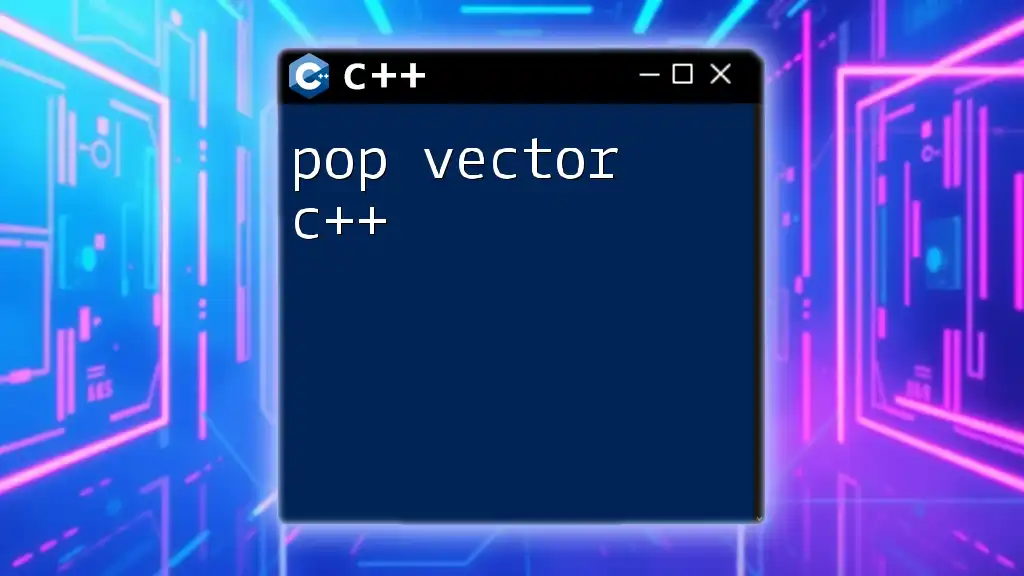
Comparisons: `at()` vs `[]` Operator
Performance Considerations
While `at()` adds a slight overhead due to bounds checking, the difference in performance is often negligible compared to the benefits it offers in terms of safety. The subscript operator `[]`, on the other hand, is slightly faster but poses a higher risk because it does not check whether the index is valid.
When to Use Each Method
Use the `at()` method when:
- You want safety and are concerned about out-of-bounds access.
- You are working in contexts where exceptions can be caught and managed.
On the other hand, the `[]` operator is appropriate when:
- Performance is critical, and you are confident about the index validity.
Here’s an illustrative example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
// Using at() safely
std::cout << "Using at(): " << numbers.at(3) << std::endl; // Output: 4
// Using [] operator (risk of out-of-bounds)
std::cout << "Using []: " << numbers[2] << std::endl; // Output: 3
return 0;
}
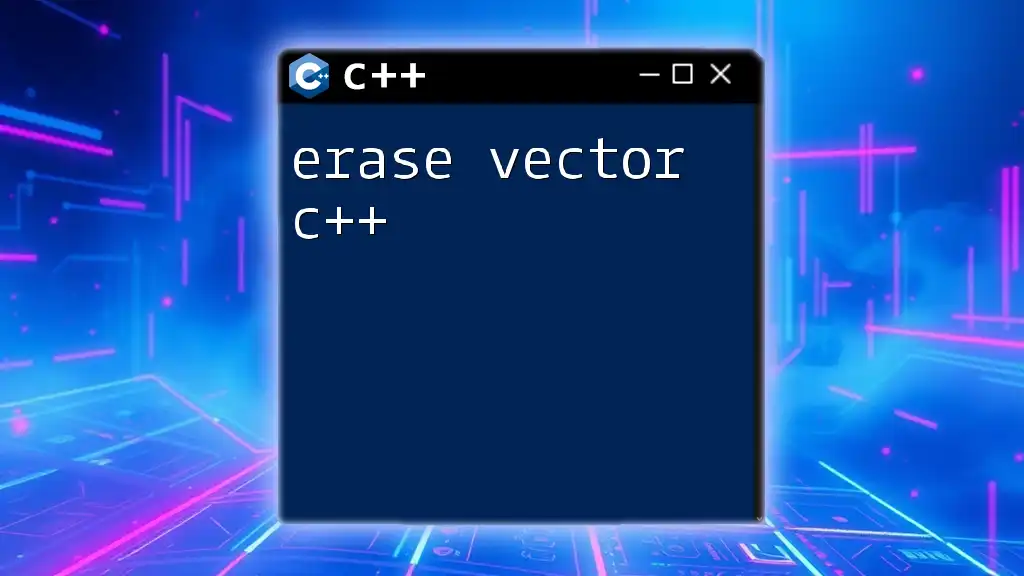
Common Use Cases for `at()`
Practical Scenarios in Programming
In most applications requiring arrays or lists, vectors with the `at()` method can be incredibly useful.
For instance, you might need to iterate over elements safely:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {10, 20, 30, 40};
for (size_t i = 0; i < numbers.size(); ++i) {
std::cout << "Element at index " << i << ": " << numbers.at(i) << std::endl;
}
return 0;
}
This code safely prints each element of the vector using `at()`, avoiding potential out-of-bounds issues.
Integrating `at()` in Real Applications
In real-world applications, the use of `at()` can be crucial in handling user inputs where index validity might be uncertain. For example, when retrieving data based on user queries or inputs from a database, using `at()` helps ensure that your application remains robust against invalid access attempts.
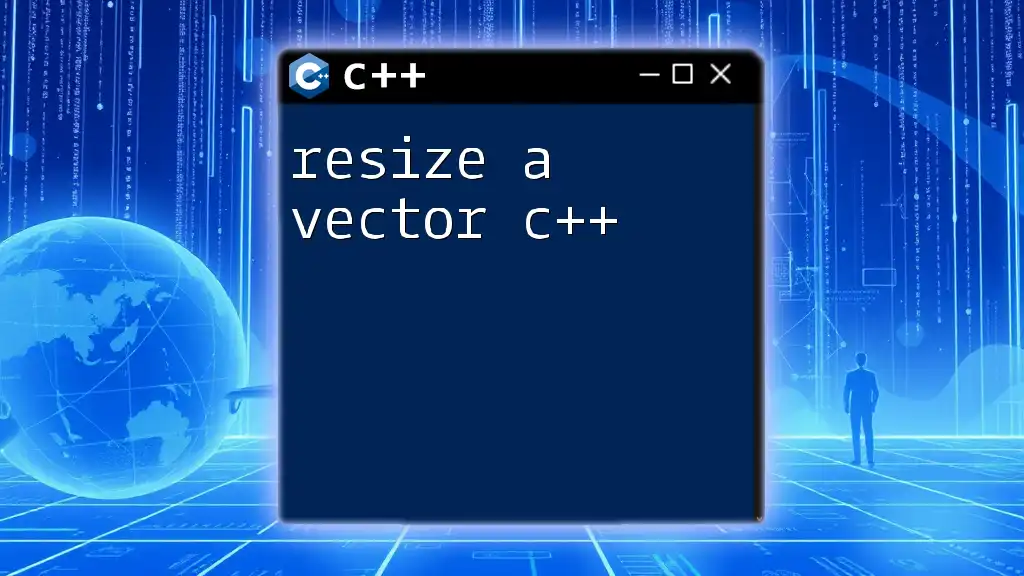
Best Practices for Using `at()`
Tips for Efficient Coding
When working with vectors and the `at()` method, consider the following best practices:
- Always prefer `at()` when user input can affect index values.
- Use exception handling alongside `at()` to provide better user experiences.
Enhancing Readability and Maintenance
Using `at()` contributes to better code readability. By clearly handling cases of out-of-bounds access, your code allows for simpler debugging and maintenance. New developers approaching your codebase can easily see how data access is managed, improving collaboration and understanding.
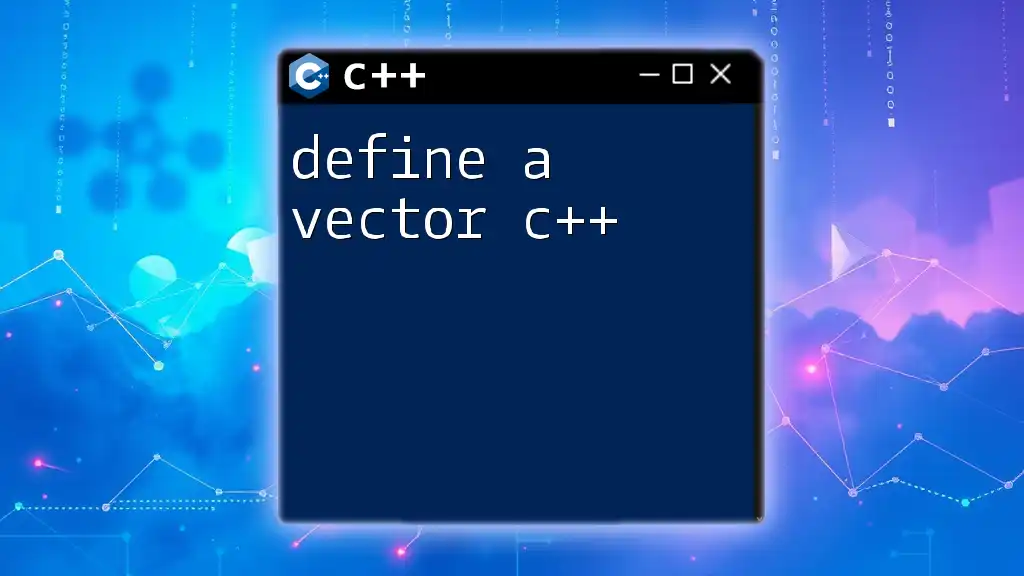
Conclusion
Summary of Key Points
In summary, the `at()` method in C++ vectors provides a safe and efficient way to access and manipulate vector elements. Its built-in error checking prevents common pitfalls associated with out-of-bounds access, making it a preferred choice in many scenarios.
Further Learning Resources
For those seeking to deepen their understanding of C++ vectors and their functionalities, consider diving into these resources:
- C++ Programming Language Textbooks
- Online C++ tutorials and courses
- Documentation and community forums for hands-on support
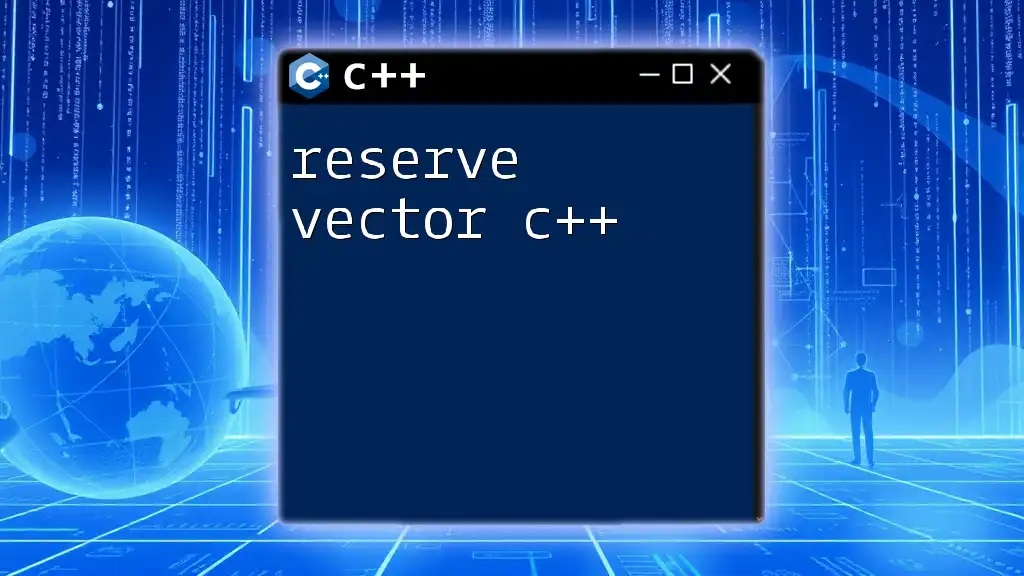
FAQs about C++ Vectors and `at()`
What is the difference between `at()` and `size()`?
The `at()` method is used to access elements at a specific index, while `size()` returns the total number of elements in the vector.
Can `at()` be used with empty vectors?
Yes, `at()` can be attempted with empty vectors but will throw `std::out_of_range` exceptions if you try to access an index (like `0`) on an empty vector.
Is it safe to use `[]` instead of `at()`?
Using `[]` is safe if you are confident that the index is within bounds; otherwise, it could lead to undefined behavior. For safer access, prefer using `at()`.