In C++, you can compare two vectors using relational operators to check if they are equal, not equal, or if one is less than/greater than the other based on their contents.
Here's a code snippet demonstrating how to compare vectors in C++:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {1, 2, 3};
std::vector<int> vec3 = {4, 5, 6};
// Comparing vectors
if (vec1 == vec2) {
std::cout << "vec1 is equal to vec2" << std::endl;
}
if (vec1 != vec3) {
std::cout << "vec1 is not equal to vec3" << std::endl;
}
if (vec1 < vec3) {
std::cout << "vec1 is less than vec3" << std::endl;
}
return 0;
}
Understanding Vectors in C++
What are Vectors?
Vectors in C++ are a part of the Standard Template Library (STL) and represent a dynamic array that can resize itself automatically when elements are added or removed. Unlike arrays, which have fixed sizes, vectors allow you to manage collections of data more flexibly.
For example, here's how you can create a vector of integers:
#include <vector>
std::vector<int> myVector;
Vectors can hold elements of any data type, including user-defined types, making them a powerful feature in C++.
Basic Operations with Vectors
Understanding basic operations is crucial for effectively using vectors in your programs. Here are some fundamental operations:
- Adding Elements: You can add elements to the end of a vector using the `push_back` method.
- Accessing Elements: Elements can be accessed using the subscript operator `[]`.
- Removing Elements: To remove elements, you can use the `pop_back` method to remove the last element or `erase` for specific positions.
Here’s a simple illustration of each operation in code:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector;
// Adding elements
myVector.push_back(10);
myVector.push_back(20);
// Accessing elements
std::cout << "First element: " << myVector[0] << "\n"; // Outputs 10
// Removing the last element
myVector.pop_back();
// The vector now contains only one element
std::cout << "Size after pop_back: " << myVector.size() << "\n"; // Outputs 1
return 0;
}
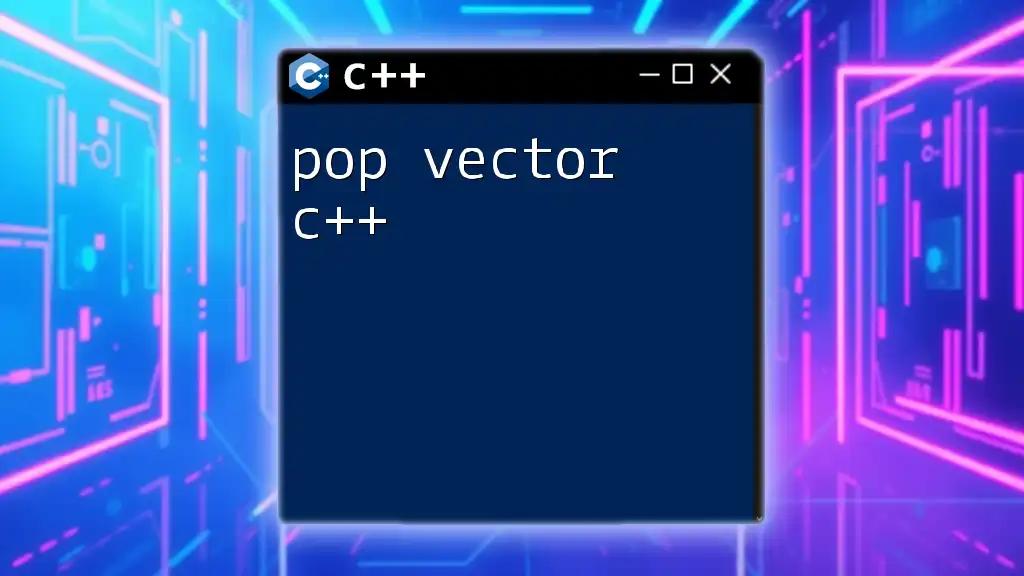
Methods to Compare Vectors in C++
Using Operators
One of the most straightforward ways to compare vectors in C++ is by using relational operators. You can employ `==`, `!=`, `<`, `<=`, `>`, and `>=` directly on vectors. These operators provide a quick way to check if two vectors are equal, or if one vector is less than or greater than another based on lexicographical order.
For example, here’s how you can check for equality:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {1, 2, 3};
if (vec1 == vec2) {
std::cout << "Vectors are equal.\n";
} else {
std::cout << "Vectors are not equal.\n";
}
return 0;
}
Using the `std::equal` Function
Another effective method for comparing vectors is using the `std::equal` function, which is found in the `<algorithm>` header. This function provides flexibility for comparison, allowing you to decide how the elements in the two vectors are compared.
You can check vectors for equality using `std::equal`:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {1, 2, 3};
if (std::equal(vec1.begin(), vec1.end(), vec2.begin())) {
std::cout << "Vectors are equal using std::equal.\n";
} else {
std::cout << "Vectors are not equal using std::equal.\n";
}
return 0;
}
Custom Comparison Functions
In certain situations, you may want to define specific rules for comparing vectors. By creating a custom comparison function, you have the flexibility to compare vectors based on size, specific attributes, or other custom criteria.
Here’s an example of a custom comparator function that checks if one vector is smaller than another based on its size:
#include <iostream>
#include <vector>
#include <algorithm>
bool compareVectors(const std::vector<int>& a, const std::vector<int>& b) {
return a.size() < b.size();
}
int main() {
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {1, 2};
if (compareVectors(vec1, vec2)) {
std::cout << "vec1 is smaller than vec2.\n";
} else {
std::cout << "vec1 is not smaller than vec2.\n";
}
return 0;
}
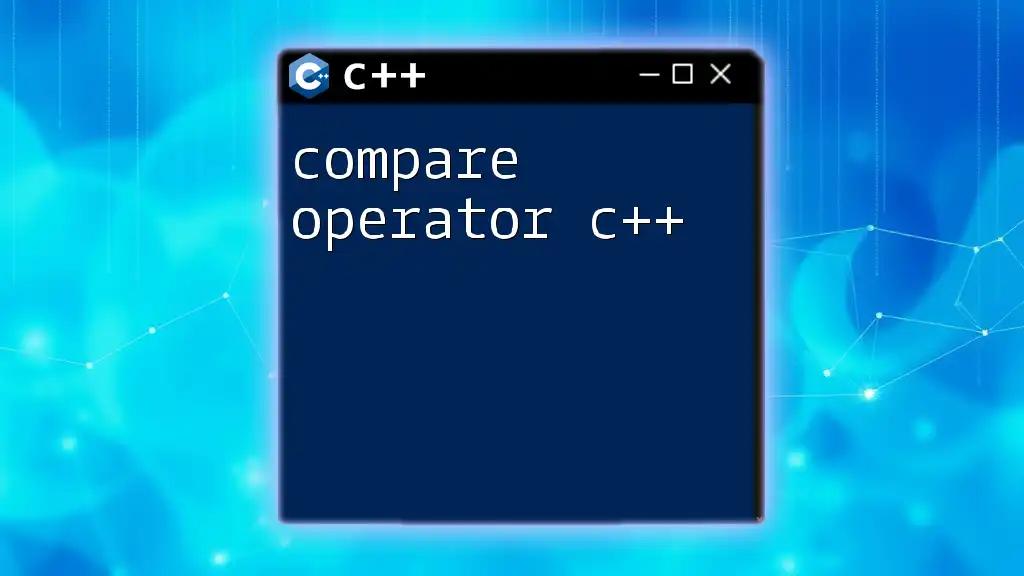
Advanced Vector Comparison Techniques
Comparing Vectors of Different Types
When dealing with vectors containing different data types, you may need to implement type conversions for comparison. This can be done using C++'s built-in type casting features or by designing your custom logic to handle such comparisons correctly.
Performance Considerations
When comparing vectors, especially large ones, be aware of the performance implications of different methods. For instance, using `std::equal` is often more efficient than manual element-by-element comparisons, especially if optimized algorithms are utilized. It’s crucial to consider the context, especially when working with large-scale data.
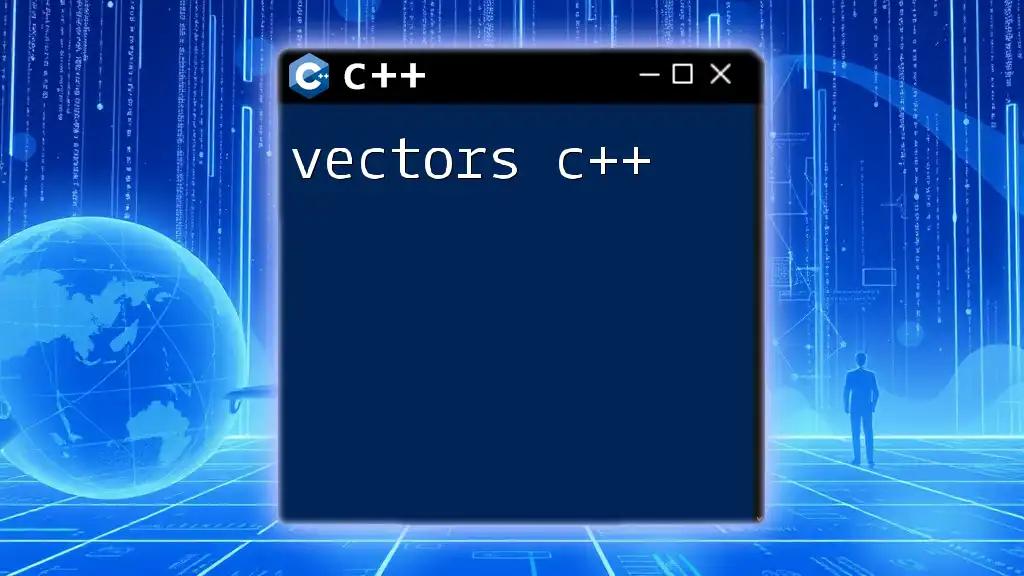
Common Mistakes When Comparing Vectors
Not Considering Vector Size
One frequent mistake is failing to compare the sizes of vectors before checking their elements. If two vectors are of different sizes, directly comparing them can lead to out-of-bounds errors.
Modifying Vectors During Comparison
Another common pitfall is making changes to the vectors while performing a comparison. This can lead to unpredictable behavior, including incorrect comparison results or runtime errors. Always ensure that the vectors remain unchanged during the comparison process.
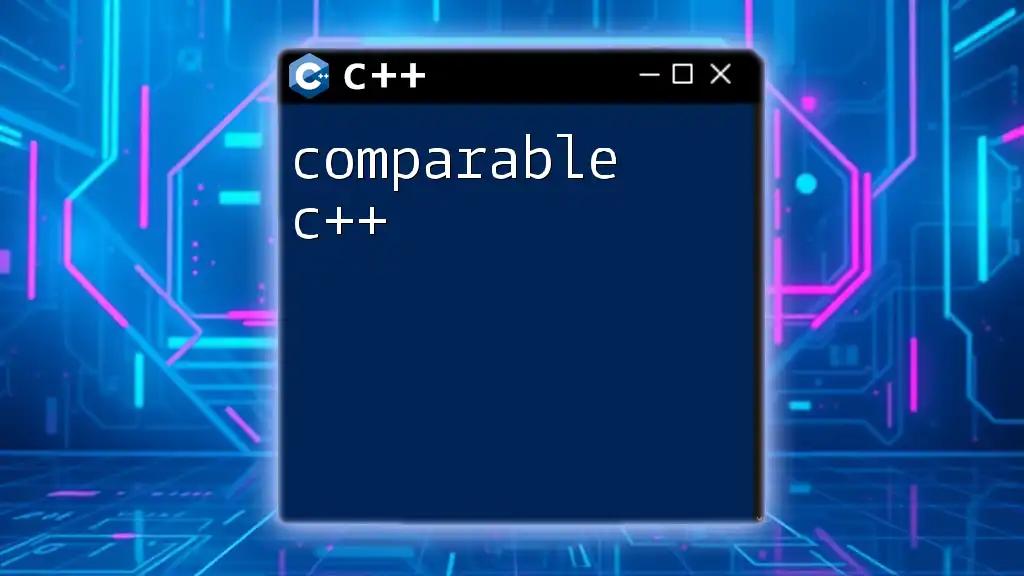
Conclusion
In C++, comparing vectors can be accomplished in various ways, each method serving different needs. From using operators to custom comparison functions, understanding these techniques is essential for effective programming. Practice with the provided examples to reinforce your understanding and build confidence in your ability to compare vectors in C++. As you continue your C++ learning journey, explore more concepts and refine your programming skills to harness the full power of this versatile language.
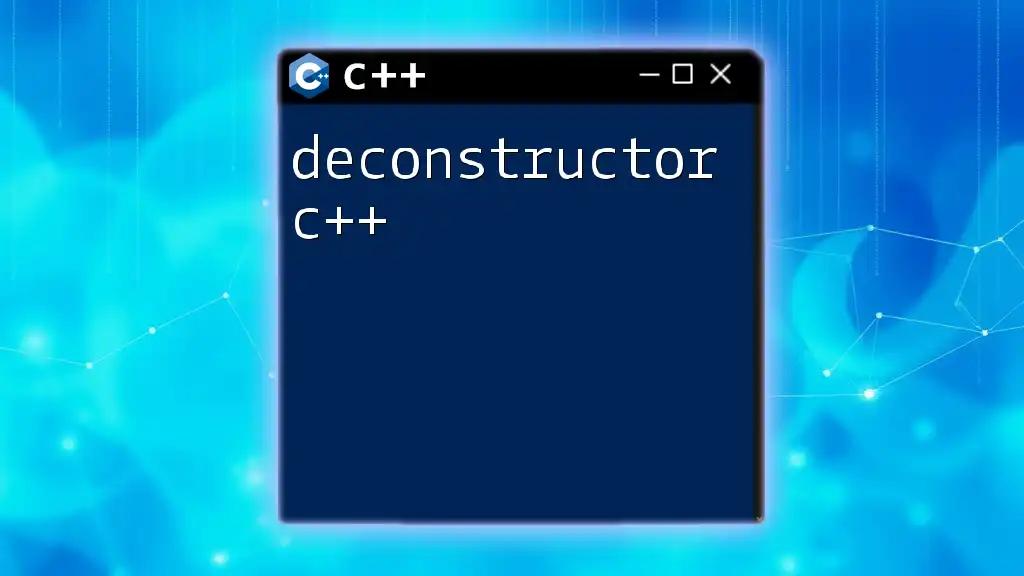
Additional Resources
For continued learning, consider visiting the official C++ documentation and explore recommended books and online courses that delve deeper into STL and vector usage. Happy coding!