To compile C++ code, you typically use a command-line tool like `g++` to transform your source code into an executable program. Here's a simple command that demonstrates this process:
g++ -o myprogram mysource.cpp
In this example, `myprogram` is the name of the output executable, and `mysource.cpp` is your C++ source file.
What Does It Mean to Compile Code in C++?
Definition of Compilation
Compilation is the process of converting source code written in a high-level programming language, such as C++, into machine code that can be executed by a computer's CPU. The C++ compiler reads the code files, performs syntax checking, optimizes the code, and then generates an executable file. This compilation process is crucial as it ensures that the program can run efficiently and effectively.
Why Compilation is Necessary
Compiling code is essential for several reasons:
-
Performance: Compiled code typically runs faster than interpreted code because it is converted into machine language. This allows the CPU to execute the instructions directly, without any intermediate interpretation.
-
Error Checking: The compilation process helps identify errors in the source code before execution. This means developers can catch syntax errors, type mismatches, and other potential issues early in the development process.
When comparing compiled languages like C++ with interpreted languages like Python, it becomes clear that compilation enhances performance and allows for static error checking, which is particularly beneficial during the development phase.
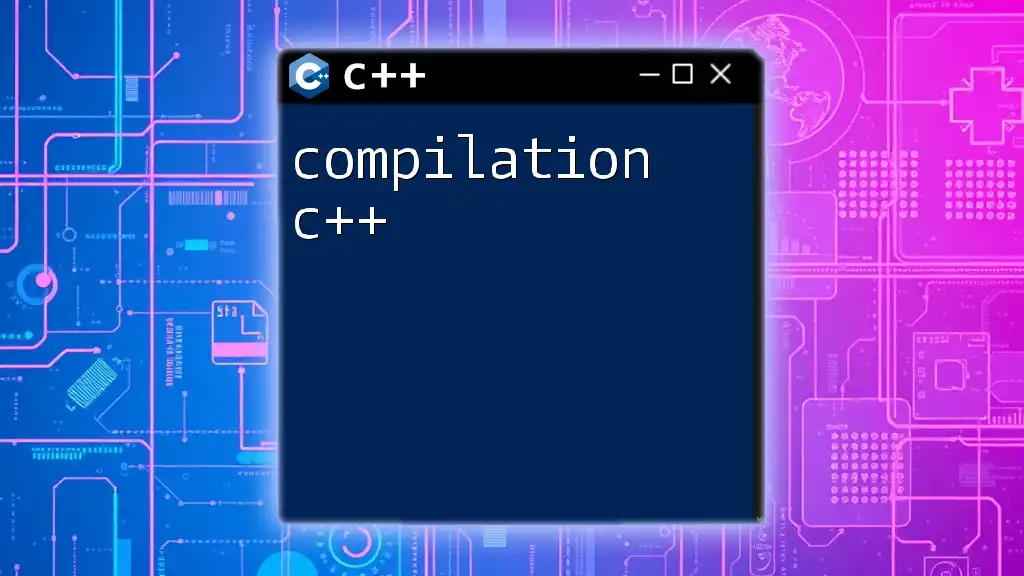
Getting Started with Compiling C++ Code
Setting Up Your C++ Development Environment
To compile C++ code, you first need a suitable development environment. This typically involves selecting a compiler and an Integrated Development Environment (IDE). Some of the most popular C++ compilers include:
-
GCC (GNU Compiler Collection): A widely-used open-source compiler that supports various programming languages, including C++.
-
Clang: Known for its speed and modern architecture, this compiler is part of the LLVM project and provides excellent diagnostics.
-
MSVC (Microsoft Visual C++): A feature-rich IDE and compiler on the Windows platform, part of the Visual Studio suite.
Choose a combination of these tools based on your operating system and project requirements.
Writing Your First C++ Program
After setting up your environment, you can write your first C++ program. Here's a simple "Hello, World!" program to get started:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
This program includes the iostream library to facilitate input and output operations, defines the `main` function as the program's entry point, and prints "Hello, World!" to the console. Understanding this basic structure is fundamental when you begin to compile code in C++.
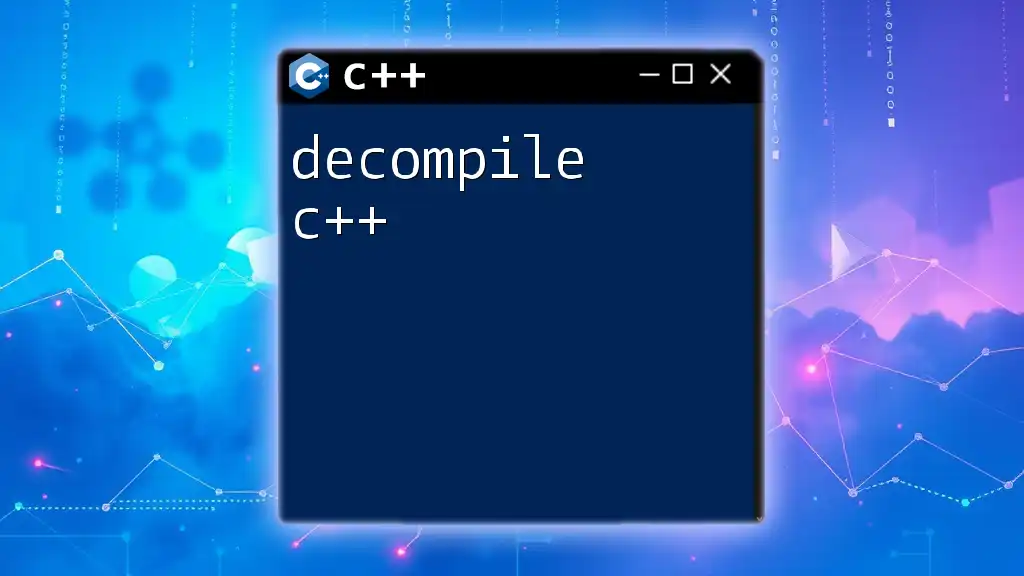
How to Compile C++ Code
Using Command Line Compilation
Compiling C++ code can be done using the command line (terminal). Here’s how:
- Open your terminal.
- Navigate to the directory where your C++ file is located.
- Use the following command to compile your program:
g++ -o hello_world hello_world.cpp
In this command:
- `g++` is the compiler invocation.
- `-o hello_world` specifies the name of the output executable file.
- `hello_world.cpp` is the name of your source code file.
Upon running this command, if there are no errors, you’ll see an executable file created named `hello_world`. If errors are present, the compiler will provide messages to help you troubleshoot the code.
Compiling C++ Code in an IDE
Using an IDE can simplify the process of compiling and running C++ code. In IDEs like Code::Blocks or Visual Studio:
- Open the IDE and create a new C++ project.
- Add a new source file, paste in your C++ code, and save it.
- Use the “Build” or “Compile” option in the IDE menu, usually found in the project settings.
The IDE handles the compilation and provides an output window where you can see error messages if any issues arise.
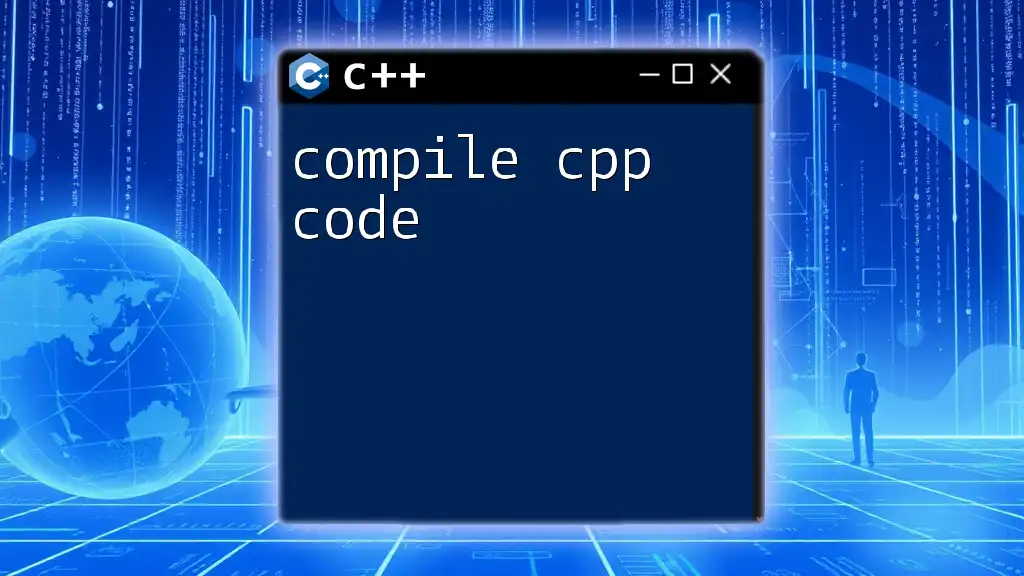
Common Compile Errors and How to Fix Them
Syntax Errors
One of the most common issues faced during compilation are syntax errors. These mistakes occur when the code deviates from the rules of the C++ language. Examples of syntax errors include missing semicolons, unmatched parentheses, or incorrect use of keywords.
To detect and resolve these errors, always read compiler error messages carefully; they often indicate the line number where the error occurred. Debugging tools within IDEs can also provide real-time feedback and highlight syntax issues as you write code.
Linker Errors
While syntax errors prevent the code from being compiled, linker errors arise when the compiled code cannot link to the necessary libraries or other code elements. For example, if you declare a function in your code but forget to define it, the linker will complain about an "undefined reference."
To resolve linker errors, double-check:
- Whether all functions are defined.
- If you're missing any library linking arguments during compilation.
Debugging Compilation Issues
Debugging compilation issues requires familiarity with your compiler's flags. Using verbose flags like `-Wall` can help produce more detailed error messages, assisting in identifying the issues present in your code.
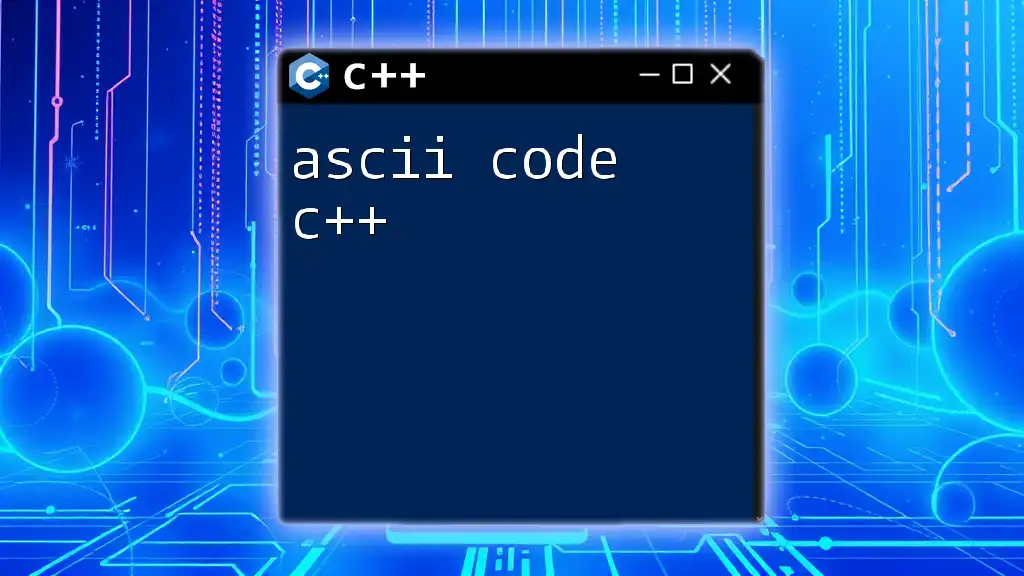
Executing a Compiled C++ Program
Running the Executable File
Once your C++ program is successfully compiled, running the executable file allows you to see your program in action. Follow these steps:
- Open your terminal again.
- Navigate to the directory containing the executable.
- Execute the file using:
./hello_world
This command launches the compiled program. If everything is set up correctly, you should see the output "Hello, World!" on your terminal.
Compiling and Running Code in One Go
For efficiency, you can compile and run your program in a single command using command chaining. For example:
g++ hello_world.cpp && ./a.out
In this command:
- If the compilation is successful, `&&` ensures that the executable runs immediately afterward.
This streamlines your workflow and saves time during the development process.
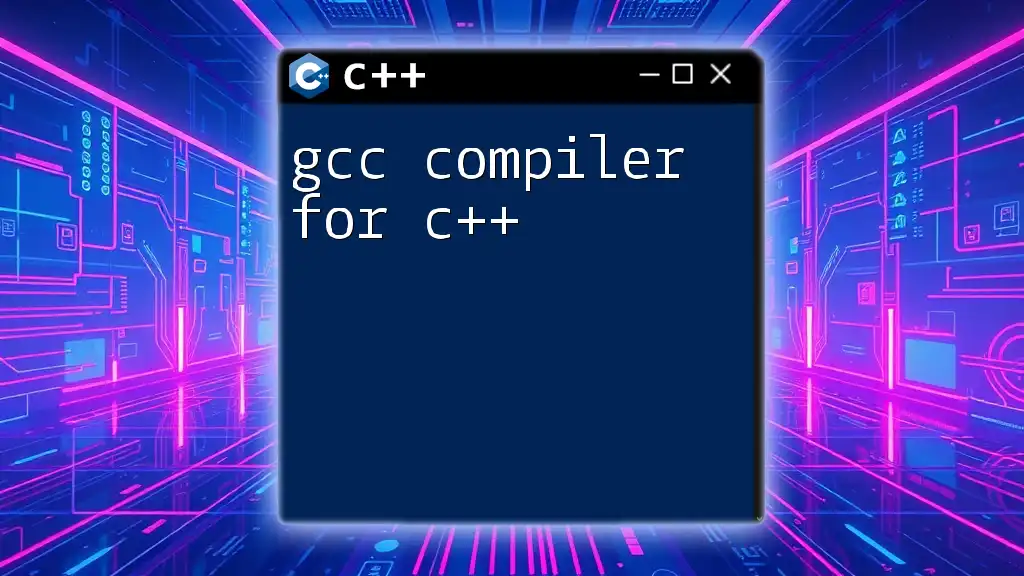
Advanced Compilation Techniques
Using Makefile for Project Management
As your projects grow in size and complexity, managing compilation can become cumbersome. Enter Makefile, a powerful tool that automates the build process for C++ projects by defining rules for compiling various files.
Here's a simple example of a Makefile:
all: hello_world
hello_world: hello_world.o
g++ -o hello_world hello_world.o
hello_world.o: hello_world.cpp
g++ -c hello_world.cpp
clean:
rm -f *.o hello_world
This Makefile defines targets for building and cleaning executable files, making it easier to manage larger projects by automatically handling dependencies and compile commands.
Utilizing Compiler Flags
Understanding and utilizing compiler flags can greatly enhance the efficiency of your code. Commonly used flags include:
- `-O2`: Optimizes the performance of your code.
- `-g`: Generates debugging information, useful if you want to debug the executable later.
- `-Wall`: Enables all compiler's warning messages, helping you write better code by identifying potential issues early.
For instance, to compile with optimization and debugging, you would use:
g++ -O2 -g hello_world.cpp
This command compiles your program with optimizations and includes necessary debugging information.
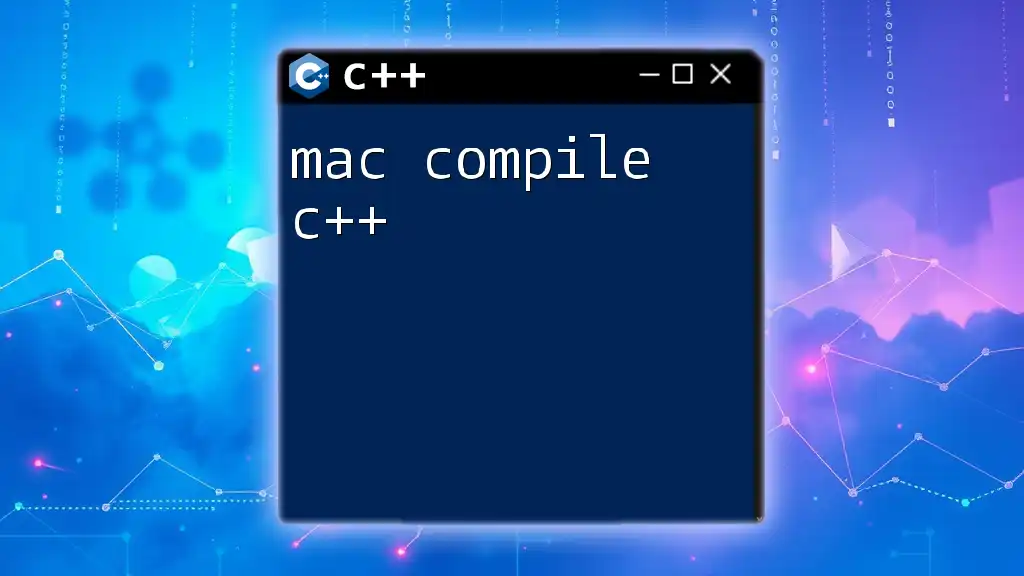
Conclusion
Compiling C++ code is a fundamental skill for any aspiring programmer. Understanding the compilation process, how to set up your environment, and how to troubleshoot common errors not only enhances your coding experience but also helps you create efficient and robust software. As you become more comfortable with compiling and executing C++ programs, don't hesitate to experiment with advanced techniques and tools that can further streamline your workflow.
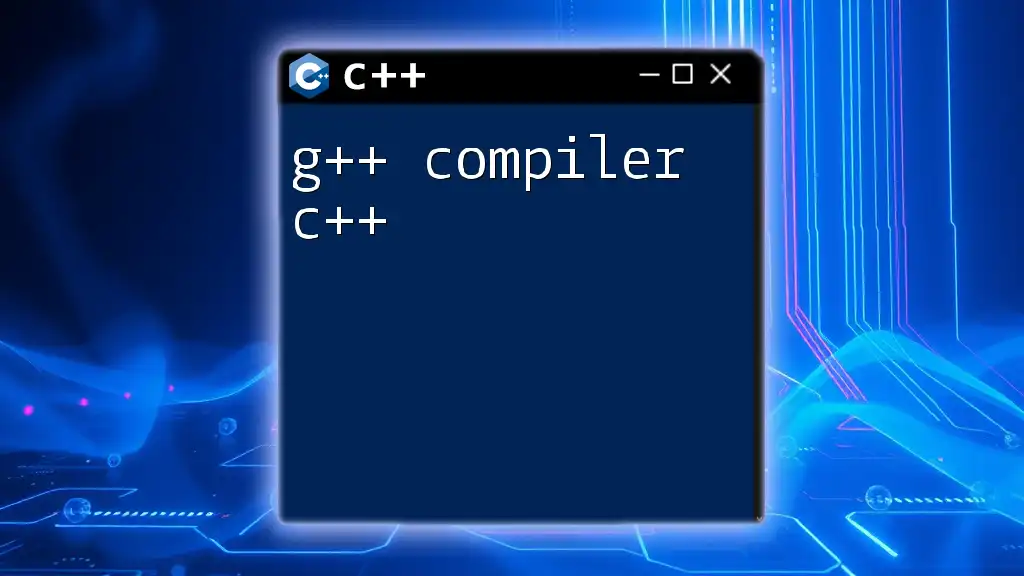
Additional Resources
Online Compilers
For beginners who wish to experiment without setting up a local environment, online compilers offer a great starting point. Popular options include Replit, JDoodle, and OnlineGDB, which allow for immediate coding and execution.
Books and Courses
To deepen your understanding of C++, consider reading classic texts like "The C++ Programming Language" by Bjarne Stroustrup or taking online courses from platforms such as Coursera or Udemy. These resources provide structured learning paths that cover everything from coding basics to advanced concepts.
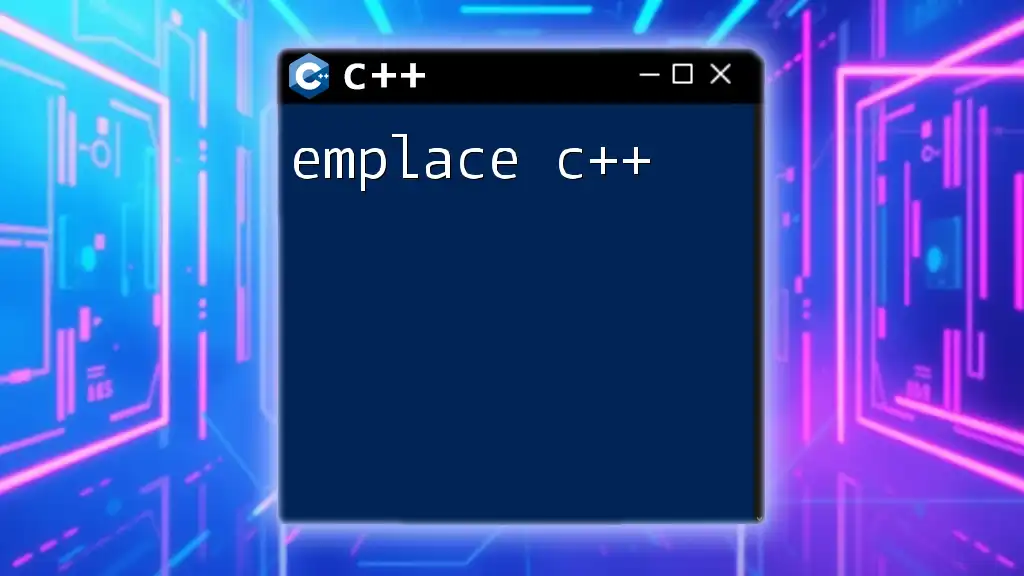
Call to Action
We encourage you to share your experiences with compiling C++ code. What challenges did you face, and how did you overcome them? Dive into coding, experiment with compilation techniques, and enjoy the journey into the world of C++ programming!