To compile a C++ program in Windows, you can use the g++ compiler from the MinGW toolchain by executing the following command in the Command Prompt.
g++ -o outputfilename inputfilename.cpp
Understanding C++ Compilation
What is Compilation?
Compilation is the process through which C++ source code is transformed into machine code, which can be executed by the computer's processor. This process involves several stages:
-
Preprocessing: This stage handles directives like `#include` and `#define`, which are not part of the actual C++ code but help in organizing the code and managing constants.
-
Compilation: The preprocessed code is then converted into assembly code, which is a lower-level representation of the program.
-
Assembly: The assembly code is translated into machine code, which consists of binary instructions that the CPU can understand.
-
Linking: Finally, the machine code is linked with libraries and other resources needed for execution, creating an executable file.
Why Use C++ in Windows?
C++ is a powerful programming language offering a mix of performance and flexibility, making it an ideal choice for various applications on Windows. It allows developers to interact closely with system resources, yielding highly efficient applications. C++ is commonly used in software development for desktop applications, game development, real-time systems, and system software.
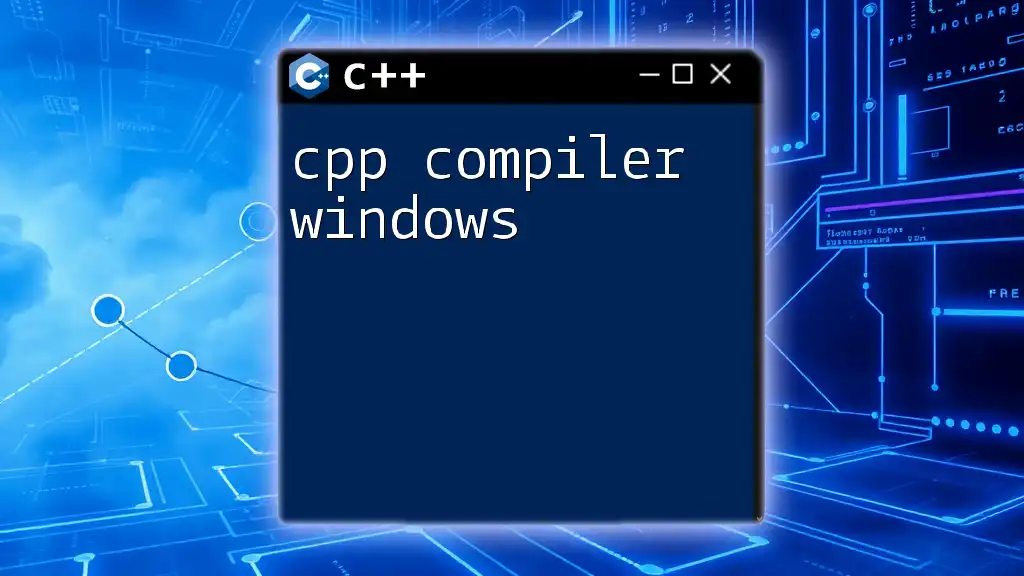
Setting Up Your Environment
Essential Tools Required
To compile C++ in Windows, you need a suitable compiler and, optionally, an Integrated Development Environment (IDE):
-
Compiler Options: Popular choices include Visual Studio's MSVC (Microsoft Visual C++) and MinGW (Minimalist GNU for Windows). Each has its strengths depending on your development needs.
-
IDE Choices: While you can compile C++ using command-line tools, an IDE such as Visual Studio or Code::Blocks offers an integrated environment that simplifies coding, debugging, and compiling processes.
Installing a Compiler
Installing MinGW
- Download the Installer: Go to the [MinGW website](http://www.mingw.org/) and download the latest installer.
- Installation Process: Follow the prompts to install MinGW, ensuring that you select the necessary components (especially the C++ compiler).
- Setting Up Environment Variables:
- Navigate to Control Panel > System and Security > System > Advanced system settings.
- Click on Environment Variables.
- Under System variables, find the Path variable and include the path to your MinGW `bin` directory (e.g., `C:\MinGW\bin`).
Installing Visual Studio
- Download Visual Studio: Go to the [Visual Studio website](https://visualstudio.microsoft.com/) and download the Community version (it's free).
- Installation Process: During installation, select the Desktop development with C++ workload. This ensures you have all necessary components for C++ development.
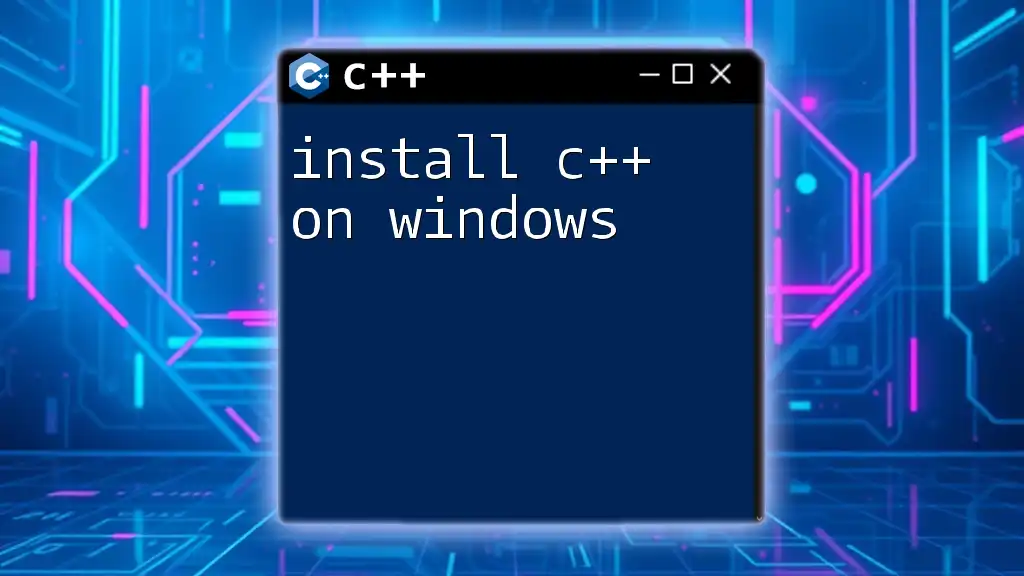
Writing Your First C++ Program
Creating a Simple Program
Now that your environment is set up, it's time to write your first C++ program. Here's a classic example: a simple "Hello, World!" program.
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Explanation:
- `#include <iostream>`: This line includes the input-output stream library, enabling you to use `cout` for console output.
- `using namespace std;`: This helps avoid typing `std::` before every standard library object.
- `int main() { ... }`: This is the main function, where the execution of the program begins.
- `cout << "Hello, World!" << endl;`: This command outputs the string to the console followed by a newline.
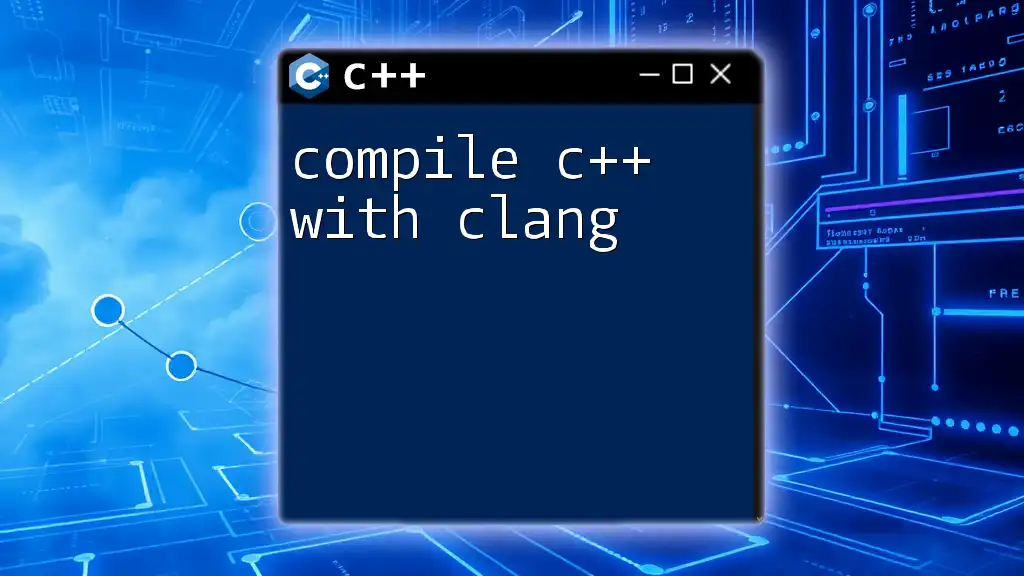
Compiling C++ in Windows
Using the Command Line Compiler
Compiling with MinGW
- Open the Command Prompt.
- Navigate to the directory where your `.cpp` file is stored using the `cd` command.
- Use the following command to compile your program:
g++ -o hello hello.cpp
In this command:
- `g++` is the GNU C++ compiler.
- `-o hello` specifies the name of the output executable.
- `hello.cpp` is the name of your C++ source file.
Compiling with Visual Studio Command Prompt
- Open the Visual Studio Developer Command Prompt.
- Navigate to your source file's directory.
- Compile your program using:
cl hello.cpp
Note: This command automatically creates an executable named `hello.exe`.
Using an Integrated Development Environment (IDE)
Compiling in Visual Studio
- Open Visual Studio and create a new project.
- Choose Console App and select C++ as the programming language.
- Write or paste your C++ code into the provided editor.
- To compile, click on the Build menu and select Build Solution or press `Ctrl + Shift + B`.
- Run your program using `Ctrl + F5` to see the output in a console window.
Other IDEs
For those using Code::Blocks or Dev-C++, compiling is similarly straightforward. Simply open the IDE, create a new project, write your C++ code, and hit the compile button to generate the executable file.
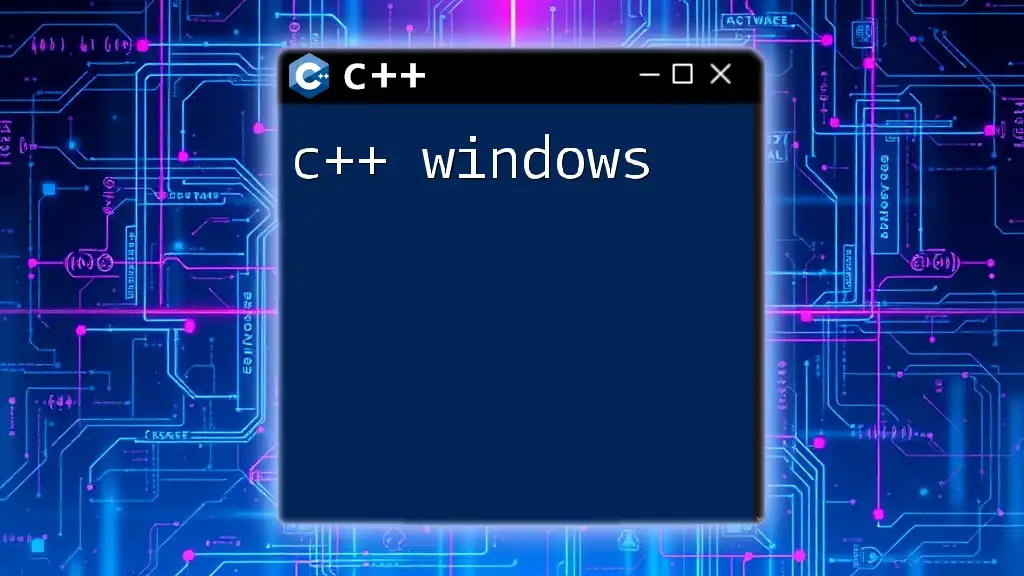
Troubleshooting Compilation Errors
Common Errors and Fixes
Syntax Errors
Syntax errors are among the most common issues when compiling C++. They occur when the code does not conform to the language's grammar. For instance, forgetting a semicolon at the end of a statement will result in a syntax error. The compiler will provide a message, often pointing to the erroneous line, enabling you to correct it.
Linker Errors
Linker errors occur when the compiler can’t find definitions for functions or variables declared in the code. For example, if you declare a function in one file but fail to define it in another, a linker error will arise. Always ensure all necessary modules and libraries are included and correctly referenced.
Best Practices
Adopting best practices can help minimize errors during compilation:
- Keep your filenames simple and consistent.
- Use appropriate compiler flags for debugging, such as `-g` for MinGW, to help identify issues.
- Regularly clean and rebuild your project to ensure outdated files do not cause conflicts.
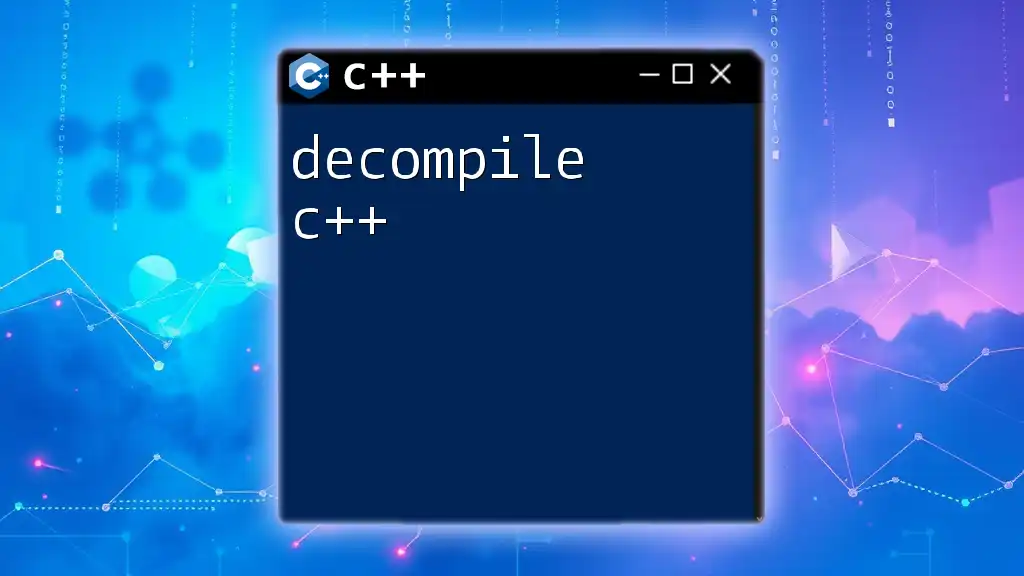
Conclusion
Compiling C++ in Windows is a straightforward process once you have your environment set up correctly. Familiarizing yourself with the tools and methods discussed in this guide will enhance your programming efficiency and help you produce error-free code. Don’t hesitate to experiment with different settings and configurations as you continue your journey in C++ programming.
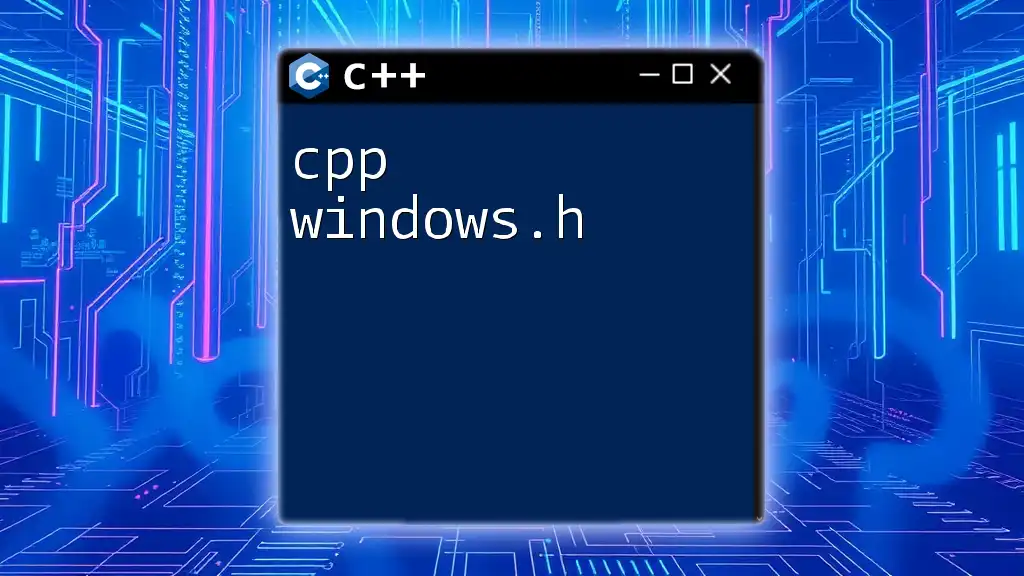
Additional Resources
If you're eager to learn more about C++ programming, consider exploring further readings, online courses, or tutorials that delve into advanced topics, best practices, and real-world applications of C++.