To compile a C++ program using Clang, you can use the following command in your terminal, replacing `filename.cpp` with the name of your source file:
clang++ filename.cpp -o output_executable
What Is Clang?
Clang is a modern compiler for the C, C++, and Objective-C programming languages. It is part of the LLVM project, which provides a robust framework for optimizing and generating code for various architectures. One of the key advantages of Clang is its ability to produce highly optimized binaries while delivering excellent diagnostics and error messages that help developers troubleshoot their code effectively.
Using Clang for C++ development can enhance your coding experience thanks to its speed, flexibility, and extensive tooling. Whether you're a beginner or an experienced programmer, learning to compile C++ with Clang can increase your productivity and code quality.
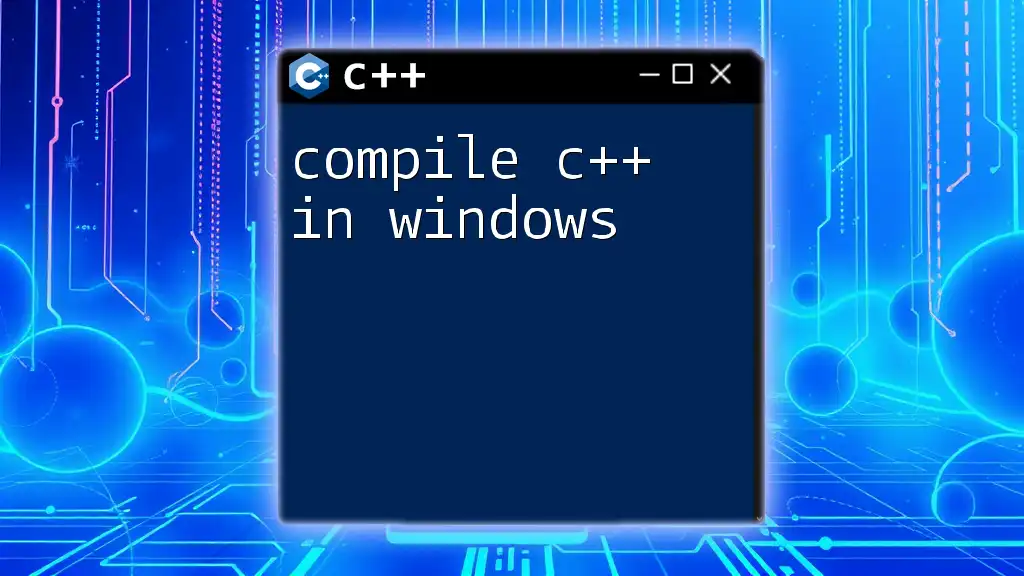
Setting Up Clang
Installing Clang
To get started with Clang, you first need to install it on your machine. Here’s how you can do that based on your operating system:
Windows: The easiest way to install Clang is through a package manager like Chocolatey. If you don’t have Chocolatey installed, you can download it from [the official website](https://chocolatey.org/install). Once installed, execute the following command in your command prompt:
choco install llvm
If you prefer manual installation, you can visit the [LLVM releases page](https://releases.llvm.org/) and download the appropriate installer.
macOS: The preferred method for macOS users is to use Homebrew. If you haven’t installed Homebrew yet, follow the instructions on [the Homebrew website](https://brew.sh). Once Homebrew is set up, run the following command:
brew install llvm
Linux: Installation commands can vary by distribution. For Ubuntu, use:
sudo apt install clang
For Fedora, use:
sudo dnf install clang
Make sure to check your distribution’s package manager for the specific Clang installation command.
Verifying Clang Installation
After installation, it's crucial to confirm that Clang is set up correctly on your system. Open your terminal or command prompt and enter the following command:
clang --version
You should see output containing the version number and build details of Clang. If you encounter any errors, please revisit the installation steps.
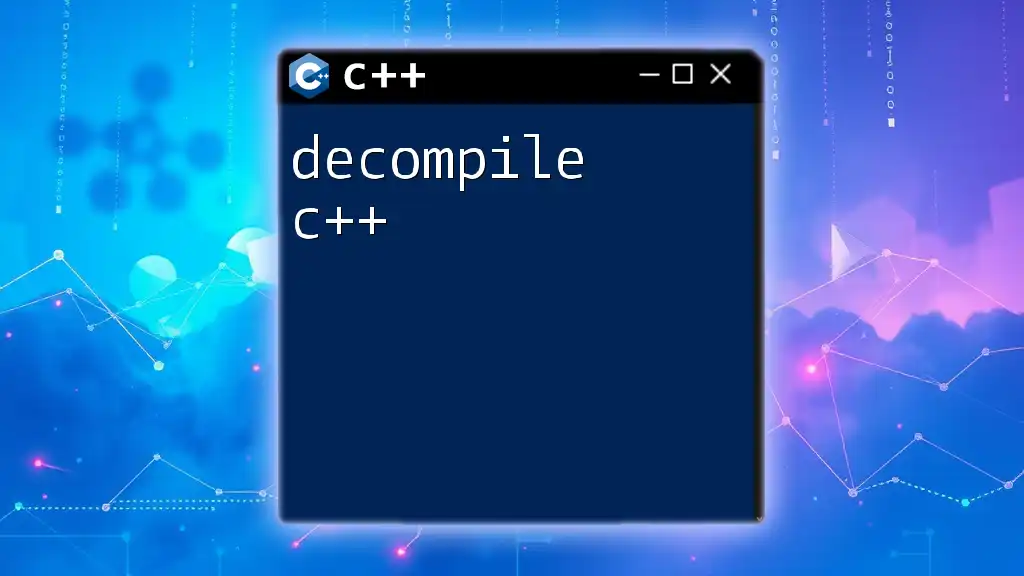
Compiling a Simple C++ Program
Writing Your First C++ Program
Let’s create a simple C++ program to demonstrate compiling with Clang. Open your text editor or IDE, and write the following code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This program includes the standard input-output library and defines a `main` function. When executed, it prints “Hello, World!” to the console.
Using Clang to Compile
Now, let’s compile this C++ program using Clang. Save the code in a file named `hello.cpp`. To compile it, open your terminal or command prompt and navigate to the directory where your file is saved. Run the following command:
clang++ -o hello hello.cpp
Here's a breakdown of this command:
- `clang++`: This is the Clang compiler for C++.
- `-o hello`: This option specifies the name of the output file as `hello`.
- `hello.cpp`: This is the source file we want to compile.
Running the Compiled Program
After a successful compilation, you can run your program. In your terminal, enter:
./hello
You should see the output:
Hello, World!
This confirms that your program has been compiled and executed successfully.
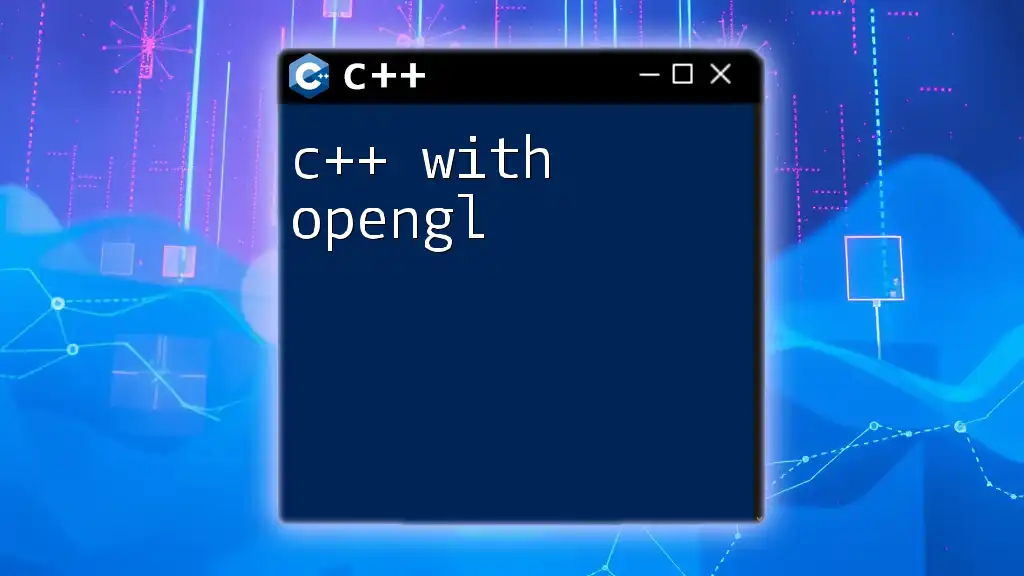
Advanced Clang Compilation Options
Optimizing Your Code
For better performance, you can use optimization flags during the compilation process. Clang offers several optimization levels like `-O1`, `-O2`, and `-O3`, which compile your code with increasing levels of optimization. To use these optimizations, modify your compilation command like this:
clang++ -O2 -o hello hello.cpp
The `-O2` flag provides a good balance between compilation time and performance.
Debugging Your Code
Debugging is an essential part of software development. Including debugging information during compilation can help you with this. Use the `-g` flag as follows:
clang++ -g -o hello debug_example.cpp
This command will generate a binary with debugging symbols that allows you to use tools like `gdb` for step-by-step debugging of your program.
Specifying Standard Versions
C++ evolves over time, introducing new features and improvements. You can specify which version of the C++ standard to use by including the `-std=` flag. For instance, to compile with C++17, use:
clang++ -std=c++17 -o hello hello.cpp
Utilizing the correct standard is crucial for taking advantage of new features and optimizations.
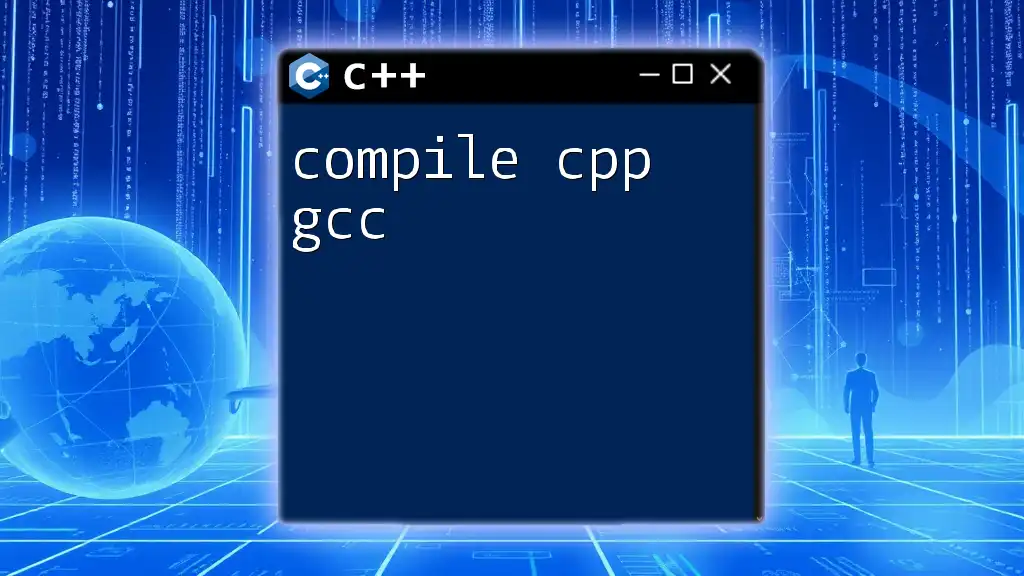
Common Compilation Errors and Solutions
Understanding Error Messages
When compiling your code, you may encounter errors. Clang provides informative error messages, often pointing directly to the source of the issue. For example, a common error could look like:
hello.cpp:1:10: fatal error: 'iostream' file not found
This message indicates that the compiler can't locate the `iostream` header, often due to a missing library or incorrect file paths.
Troubleshooting Tips
- Ensure that all necessary libraries are installed.
- Verify that you are in the correct directory containing your source files.
- Double-check your code for syntax errors and typos, as they often lead to compilation errors.
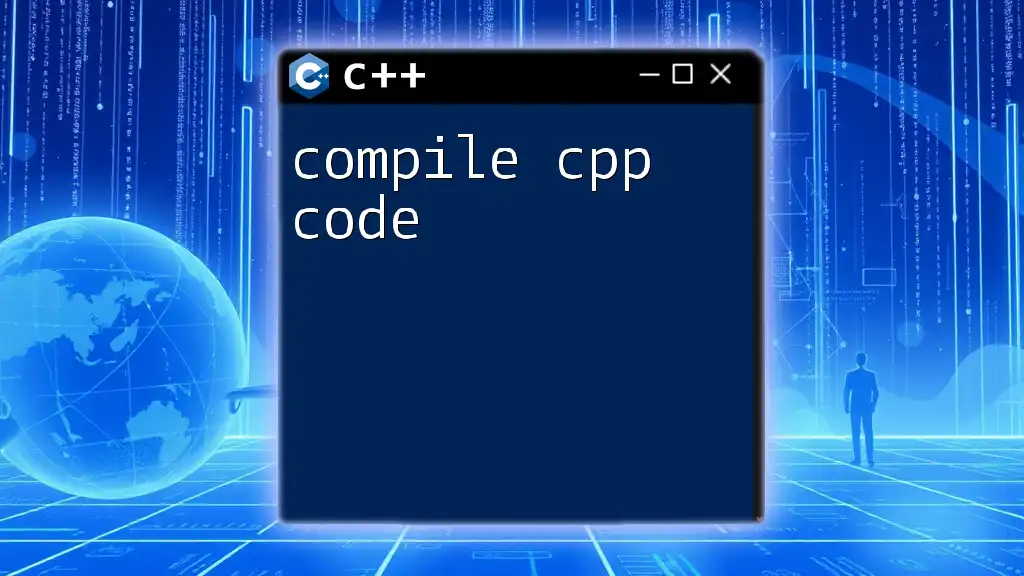
Clang Tooling
Clang Format
Clang Format is a tool that helps enforce consistent code style throughout your project. To format your code automatically, you can run the command:
clang-format -i your_code.cpp
The `-i` flag modifies the file in place, applying formatting rules based on your `.clang-format` configuration file. This practice improves readability and reduces code review time.
Clang-Tidy
Clang-Tidy is a tool that provides static code analysis, helping identify potential bugs and code quality issues. You can run it on your code with the following command:
clang-tidy your_code.cpp -- -std=c++17
This will analyze your source file and suggest improvements based on best practices, which can help you write cleaner, more efficient code.
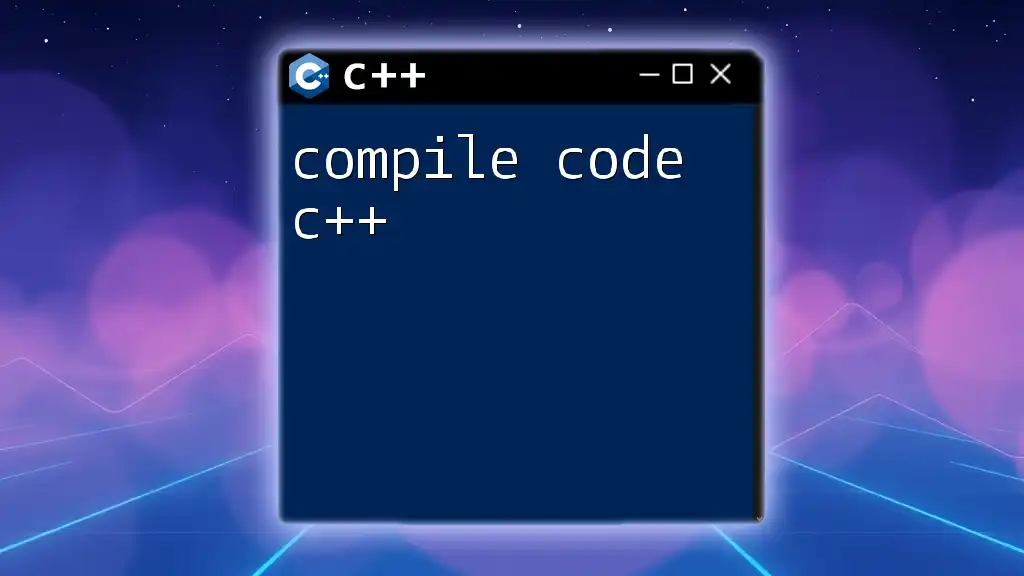
Conclusion
In this guide, we covered how to compile C++ with Clang, walking through installation, basic compilation commands, and advanced options for optimizing and debugging your code. Remember that mastering Clang can significantly enhance your development workflow, thanks to its powerful features and excellent tools.
As you continue your journey, don’t hesitate to practice by writing more complex programs and experimenting with different compilation options. The more you practice, the more proficient you will become. Happy coding!
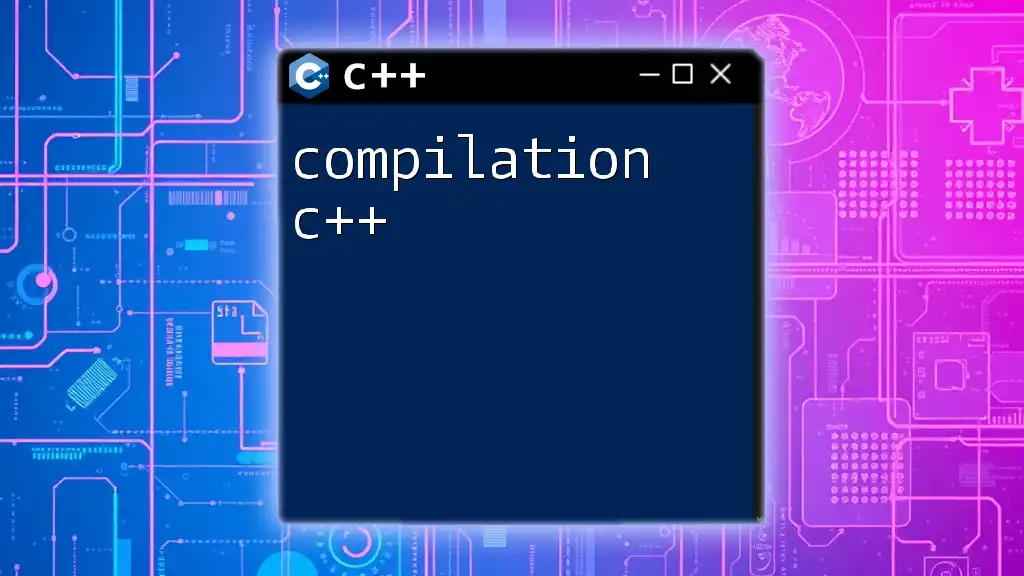
Additional Resources
For further learning, don’t miss exploring the [Clang documentation](https://clang.llvm.org/docs/index.html) and consider reading recommended books or online courses to deepen your understanding of C++ programming and Clang.