To compile a C++ program on a Mac using the terminal, use the following command, replacing `filename.cpp` with the name of your C++ source file.
g++ filename.cpp -o outputName
Understanding C++ Compilation
What is Compilation?
Compilation is the process of converting human-readable C++ code into machine language that a computer can execute. This involves three main stages: preprocessing, compilation, and linking.
-
Preprocessing: This stage handles directives marked by `#` in the code, such as `#include` for including files and `#define` for macros. It prepares the code for the next step.
-
Compilation: In this phase, the compiler translates the preprocessed code into object code, which is not yet executable.
-
Linking: Finally, the linker combines object files and resolves references to libraries or other symbols, producing an executable file.
Understanding this process helps in efficiently debugging and optimizing your C++ programs.
Why C++ Compilation is Important on Mac?
Using a Mac for C++ development comes with numerous benefits, including robust hardware, a UNIX-based operating system, and excellent development tools. Mastering the C++ compilation process on Mac not only allows for quick development but also enables smooth deployment of applications across Apple devices. Additionally, being familiar with the Mac compile C++ workflow can enhance productivity and reduce time spent troubleshooting.
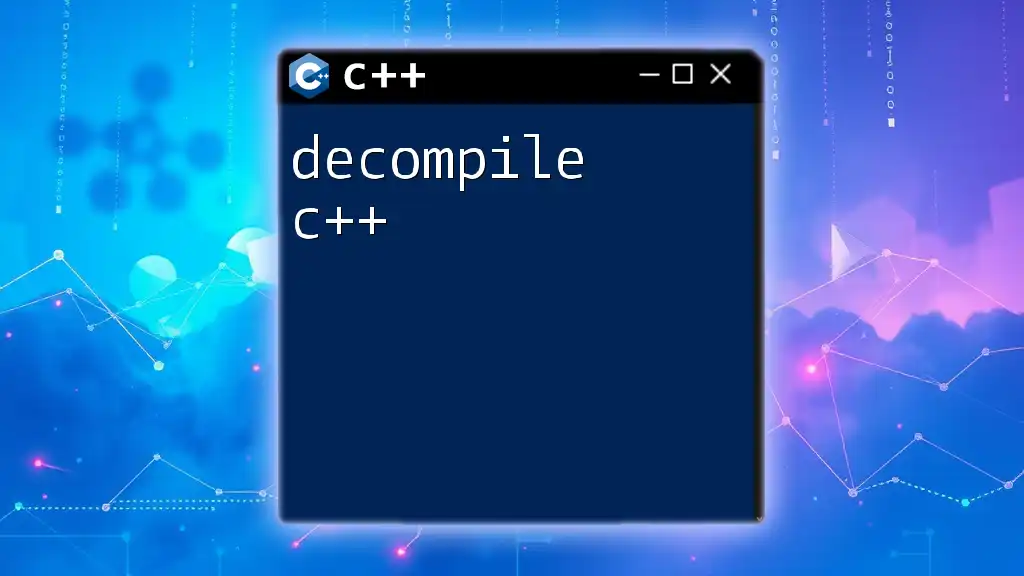
Setting Up Your Mac for C++ Development
Installing Xcode
Xcode is Apple's official integrated development environment (IDE) for macOS. It supports C++ and offers a range of tools for building, debugging, and executing C++ applications.
To install Xcode:
- Open the App Store on your Mac.
- Search for "Xcode" and click Get to download and install it.
- Once Xcode is installed, you can create a new project for your C++ programs.
Xcode provides a user-friendly interface, making it a popular choice among developers.
Command Line Tools for Xcode
In addition to the Xcode IDE, installing Command Line Tools expands your ability to compile C++ code directly from the terminal. To install Command Line Tools:
- Open the Terminal.
- Run the command:
xcode-select --install
- Follow the prompts to complete the installation.
Command Line Tools include essential compilers and file management utilities that are critical for compiling C++ code without a GUI.
Alternative C++ Compilers for Mac
While Xcode's setup is convenient, you also have access to other C++ compilers like Clang and GCC. Here’s a glance at these alternatives:
- Clang: The default C++ compiler on macOS, known for its performance and user-friendly error messages. It is included with Xcode and Command Line Tools.
- GCC: The GNU Compiler Collection, a widely used compiler that supports multiple programming languages. You can install it via Homebrew by running:
brew install gcc
Consider your project's requirements and tool preferences when choosing a compiler.
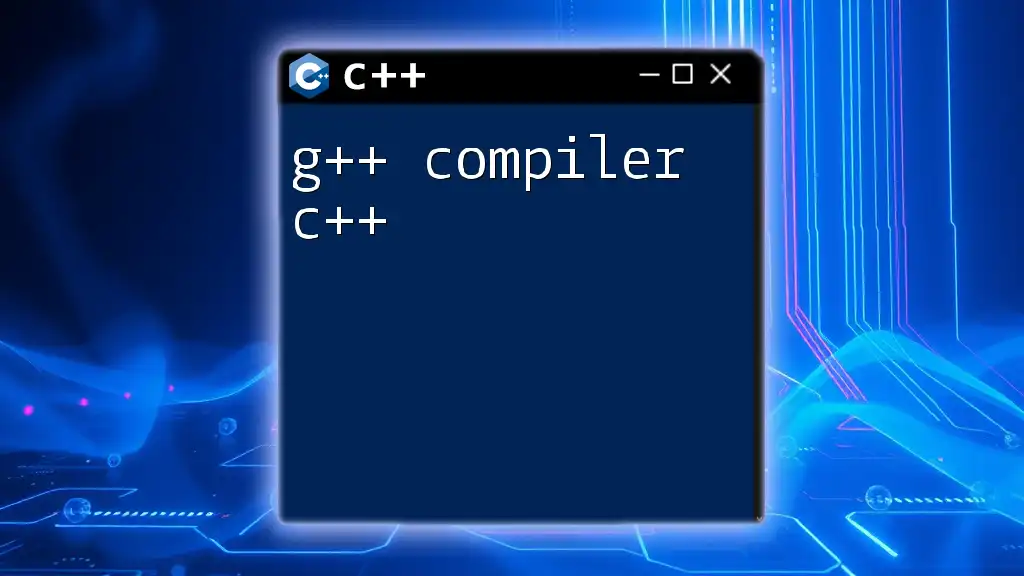
Compiling C++ Code on Mac
Writing Your First C++ Program
To illustrate the compilation process, let's write a simple C++ program:
#include <iostream>
int main() {
std::cout << "Hello, Mac C++ Compiler!" << std::endl;
return 0;
}
This program demonstrates basic syntax in C++, including input/output functionality through the `iostream` library. The `main()` function serves as the entry point of every C++ program.
Compiling C++ with Xcode
To compile your C++ program using Xcode:
- Launch Xcode and create a new project. Select C++ under the macOS section.
- Choose Command Line Tool, and then name your project.
- Input your C++ code in the main file.
- Click the Build button (or use the shortcut Command + B) to compile the program.
- To run the program, click the Run button (Command + R).
Xcode takes care of the compilation and linking stages automatically, allowing you to focus on code development.
Using the Command Line to Compile C++
Alternatively, you can compile C++ code directly from the command line, which offers greater control. Begin by navigating to the directory containing your C++ file using the `cd` command. For example:
cd path/to/your/code
To compile your file named `hello.cpp` using Clang, execute:
clang++ -o hello hello.cpp
Here, `-o hello` specifies the name of the output executable. If the compilation is successful, this command will produce an executable file named `hello`.
To run the compiled program, simply enter:
./hello
This will display the output: `Hello, Mac C++ Compiler!`.
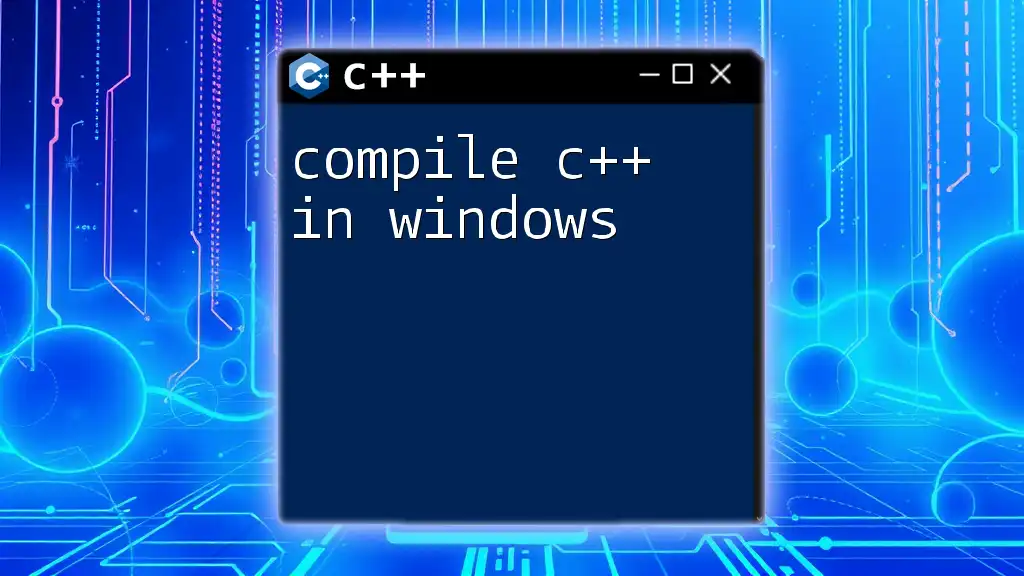
Common Errors and Troubleshooting
Compilation Errors on Mac
When compiling C++ code, you may encounter various errors. Common issues include:
-
Syntax Errors: Mistakes in language syntax (like missing semicolons) can lead to compilation failures. Read the error messages carefully as they indicate the line number and type of error.
-
Linking Errors: These occur when the linker cannot find the necessary libraries or object files. Ensure that all required files are referenced correctly.
To resolve these issues, double-check your code and paths, and consult online documentation for clarity on the errors encountered.
Debugging Your C++ Code
Debugging is essential for identifying and resolving issues within your code. Xcode provides a powerful debugger that allows for step-by-step execution, breakpoints, and variable inspection.
For command-line debugging, you can use tools like `gdb`. To compile with debug information, compile your C++ code using:
clang++ -g -o hello hello.cpp
This will generate debug symbols that can help you trace errors effectively.
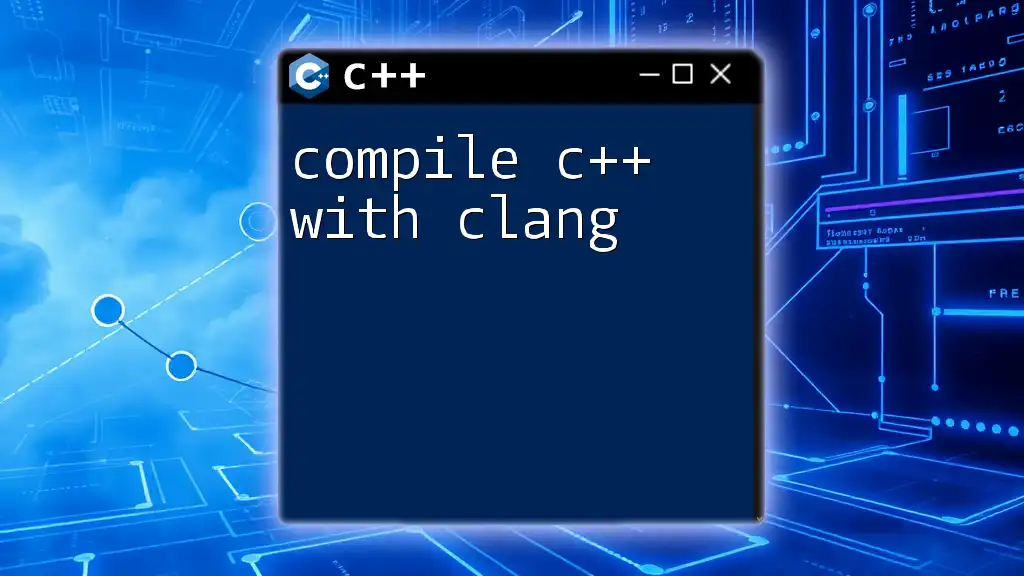
Advanced Compilation Techniques
Linking Multiple C++ Files
When working on larger projects, you often have multiple C++ source files. Linking them together is essential. For instance, suppose you have two source files, `main.cpp` and `helper.cpp`. You can compile and link them using:
clang++ -o myprogram main.cpp helper.cpp
This command will produce an executable named `myprogram`, effectively combining the sources.
Using Makefiles for Compilation
Makefiles can streamline the compilation process by automating it. A simple Makefile could look like this:
all: myprogram
myprogram: main.o helper.o
clang++ -o myprogram main.o helper.o
main.o: main.cpp
clang++ -c main.cpp
helper.o: helper.cpp
clang++ -c helper.cpp
In this example, the `all` target is defined to build `myprogram`. The Makefile specifies how to compile each object file and link them together, making building projects simpler and more efficient.
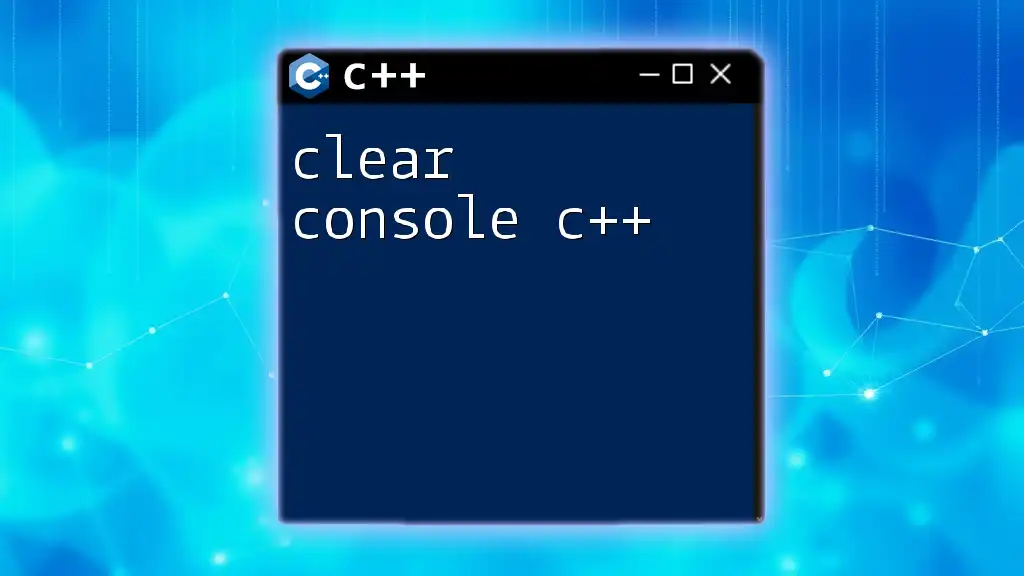
Best Practices for Compiling C++ on Mac
Organizing Your Code
Maintaining a well-structured file organization can significantly improve code manageability. Consider adopting a pattern where you include:
- A src directory for source files
- A bin directory for compiled binaries
- An include directory for header files
This organization not only helps you navigate your codebase but also makes collaboration easier.
Regularly Updating Compiler Tools
Keeping your Xcode and command line tools updated is crucial for leveraging improvements in performance and bug fixes. To check for updates, open the App Store and navigate to the Updates tab, or run:
softwareupdate --all --install --force
Regular updates ensure you have access to the latest features and optimizations in your development environment.
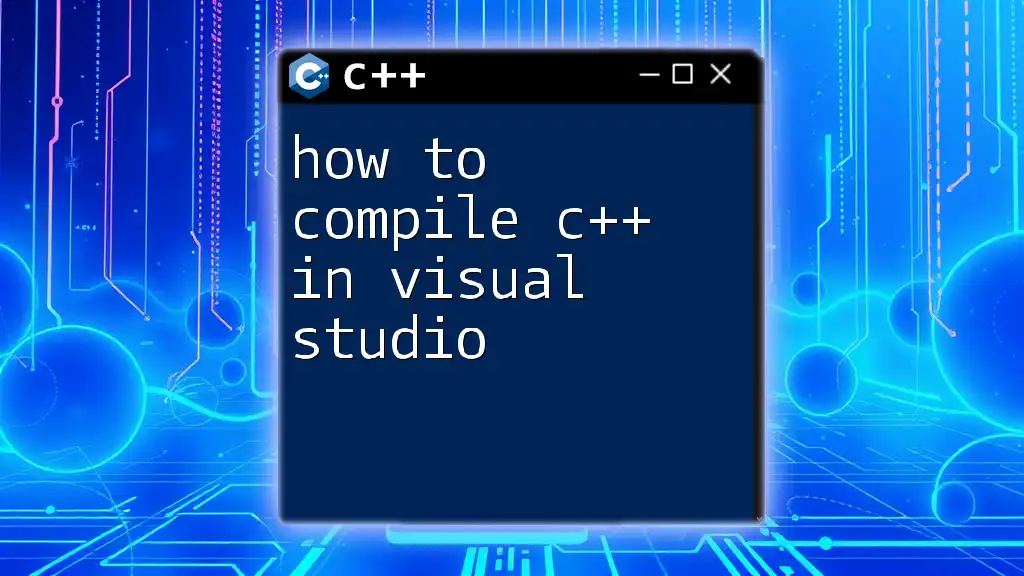
Conclusion
In summary, mastering the process to mac compile C++ can significantly enhance your programming efficiency. With tools like Xcode and command line utilities, you have powerful options at your disposal. As with any skill, practice and exploration are key to becoming proficient in compiling C++ code on Mac. You’re now equipped to start your journey in C++ development!
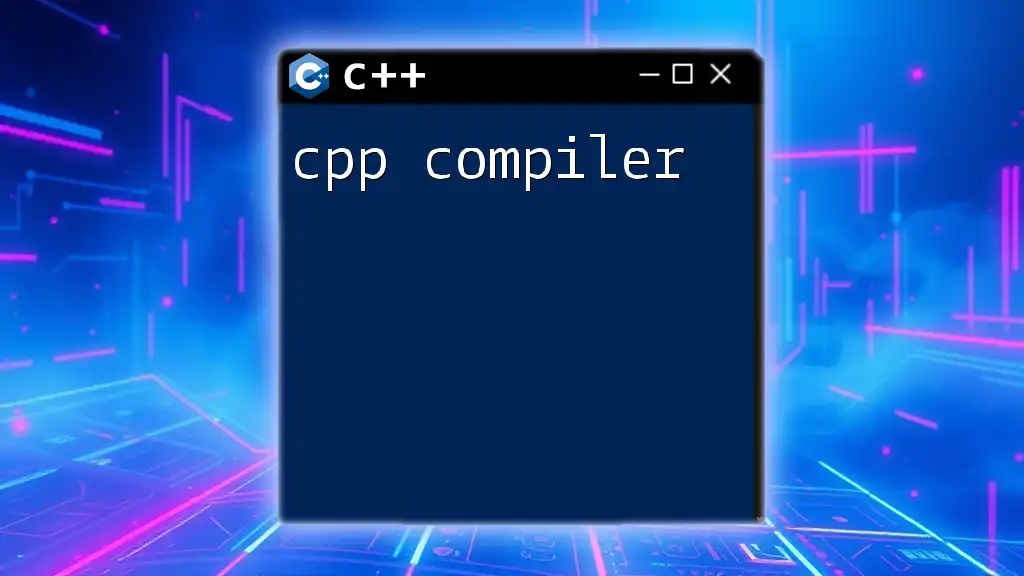
Additional Resources
To deepen your understanding, refer to official documentation for Xcode and command line tools. There are also many excellent books and tutorials available for further learning. Consider exploring these to enhance your programming projects.