The `emplace` function in C++ allows you to efficiently insert a new element into a `std::map` by constructing the element in place, thereby avoiding unnecessary copies.
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
myMap.emplace(1, "One");
myMap.emplace(2, "Two");
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
Understanding C++ Maps
What is a Map in C++?
A map in C++ is a collection of key-value pairs, where each key is unique, and it is used for efficient data retrieval. Maps are part of the C++ Standard Library and belong to the family of associative containers. They allow you to store objects in a way that makes accessing them based on a key quick and efficient.
Maps provide functionalities that facilitate various tasks, such as searching for a value associated with a specific key, updating values, and checking for the existence of a key in the map.
Syntax and Structure of a Map
To define a map in C++, you can use the following syntax:
std::map<KeyType, ValueType> mapName;
For example, to define a map that associates integers with strings, you would write:
std::map<int, std::string> myMap;
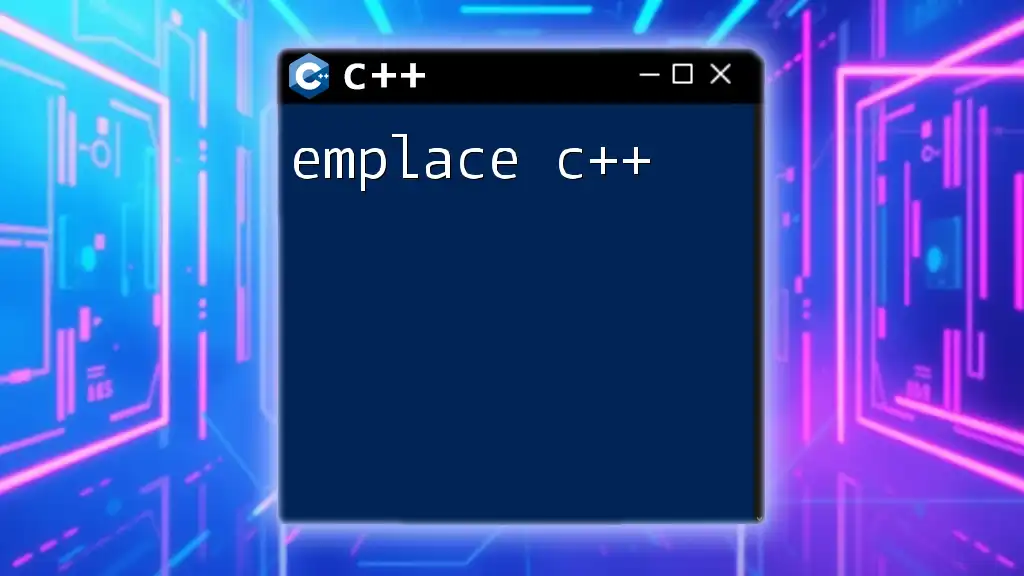
The 'Emplace' Method
What is Emplace?
The `emplace` method is a member function of the map that allows you to insert new key-value pairs into the map. What sets `emplace` apart from the traditional `insert` method is that `emplace` constructs the key-value pair in place, potentially improving performance by eliminating unnecessary copies or moves of objects.
Syntax of map::emplace
The syntax to use the `emplace` method is straightforward:
mapName.emplace(key, value);
For instance:
myMap.emplace(1, "Value One");
This code snippet adds an entry to `myMap` with the key `1` and the associated value `"Value One"`.
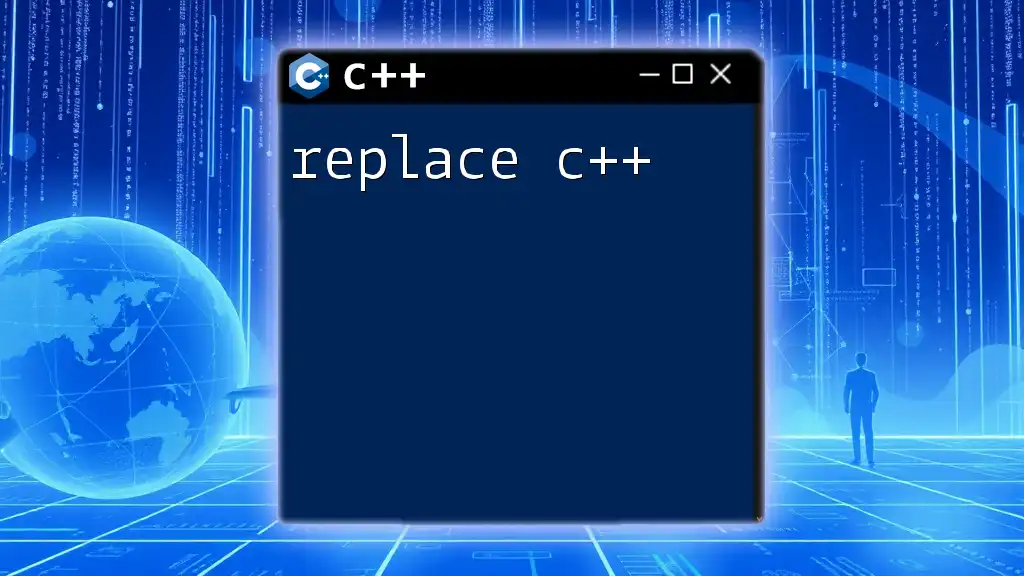
Practical Use of Emplace in C++ Maps
Adding Elements with Emplace
Using `emplace` to add elements to a map is effective and efficient. Here is a basic example:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
myMap.emplace(1, "First");
myMap.emplace(2, "Second");
for(const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
In this snippet, the `emplace` method inserts two entries into `myMap`. The loop iterates through the map, displaying each key-value pair. The output will be:
1: First
2: Second
This illustrates how `emplace` adds entries efficiently.
Using Emplace with Custom Types
You can also use `emplace` to add custom objects to a map. Let's consider a simple struct:
struct Person {
std::string name;
int age;
Person(std::string n, int a) : name(n), age(a) {}
};
Now, let's say you want to store `Person` objects in a map.
std::map<int, Person> personMap;
personMap.emplace(1, Person("Alice", 30));
In this case, `emplace` constructs a `Person` object directly within `personMap`, which leads to increased efficiency as no temporary objects are created.
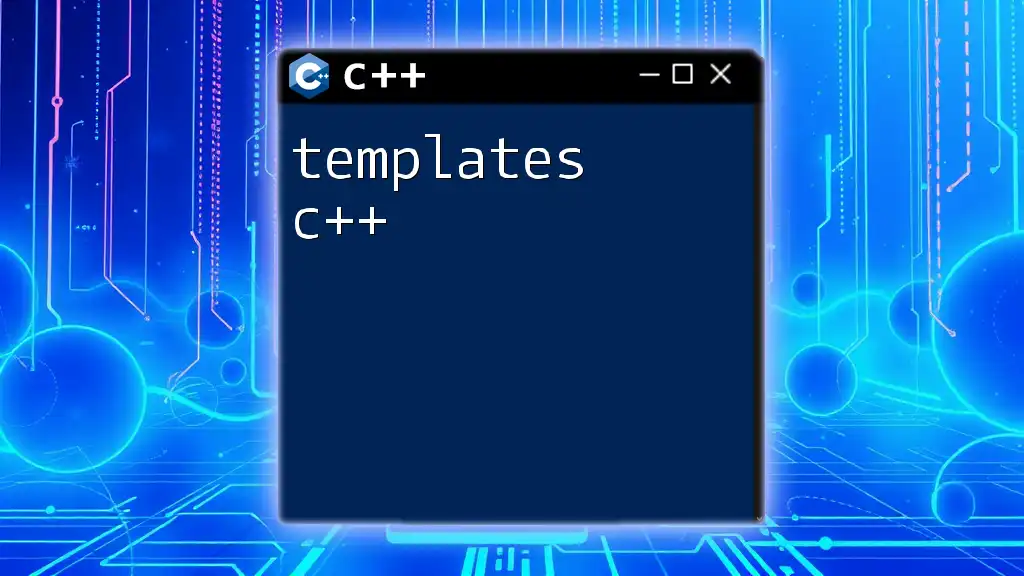
Emplace Back vs Emplace
What is emplace_back?
The `emplace_back` method is specific to vectors in C++ and allows you to add new elements to the end of a vector in-place, similar to how `emplace` works for maps. The main difference lies in the context in which they are used: `emplace_back` is for vectors, while `emplace` applies to maps.
The distinction is essential—using `emplace_back` to add elements to a vector will not behave like `emplace` in a map.
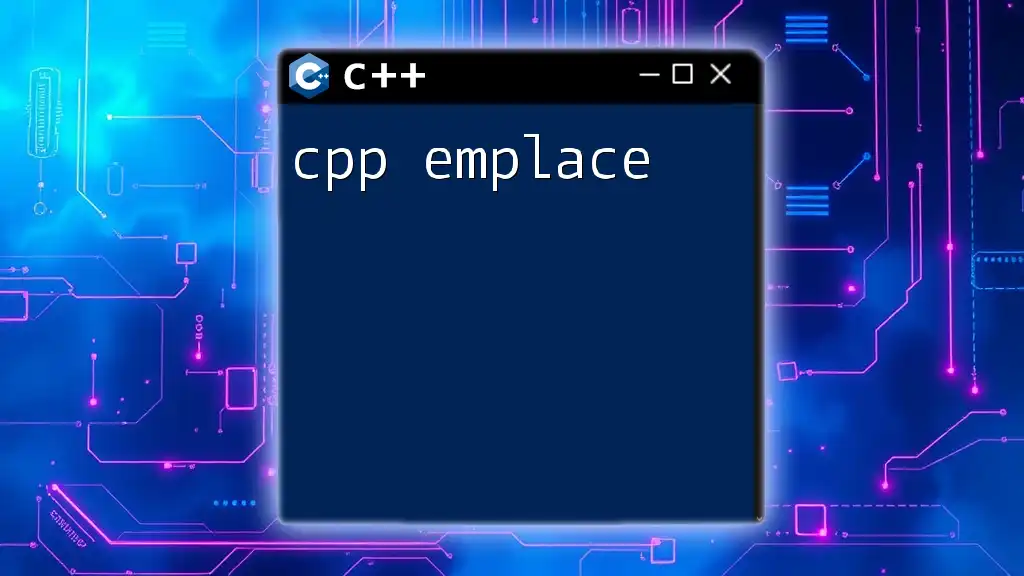
Best Practices for Using Emplace
When to Use Emplace?
Use `emplace` when:
- You want to construct objects directly in the container, saving on copies or moves.
- You are dealing with types that are expensive to copy.
- You aim to write cleaner and more efficient code.
Performance Comparison: Emplace vs Insert
When comparing `emplace` and `insert`, `emplace` may offer performance improvements since it avoids additional copy or move operations. In operations that require many insertions, such as batch inserts, using `emplace` can contribute to faster execution times due to fewer object creations.
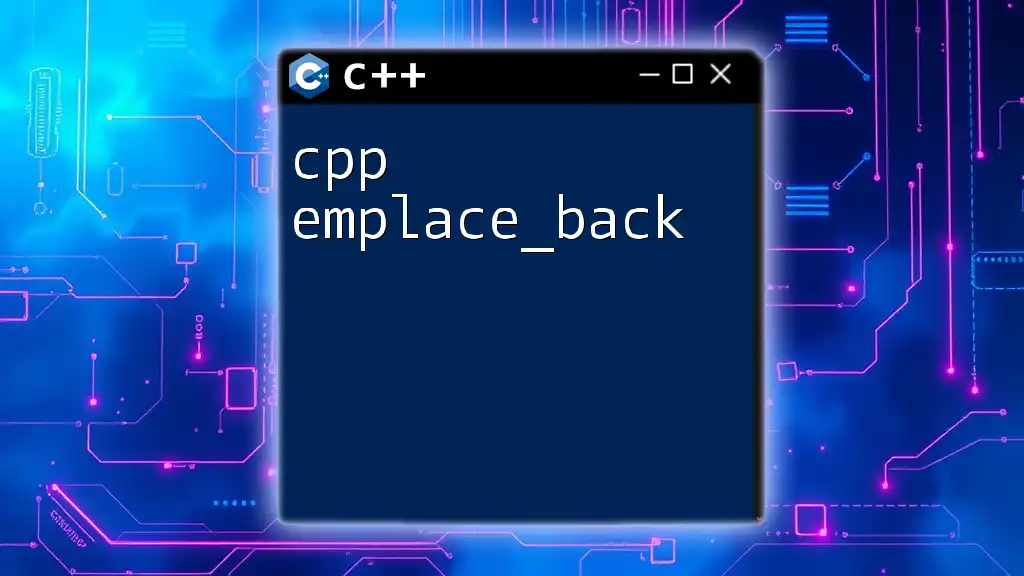
Common Pitfalls and Troubleshooting
Potential Issues with Emplace
While `emplace` is powerful, it can lead to issues if:
- The key type is not handled correctly, which can result in compilation errors.
- You are mistakenly trying to insert a duplicate key, as this will fail.
Debugging Tips
When using `emplace`, ensure you:
- Check types and ensure they match your key-value pair requirements.
- Utilize the debugger to verify the state of the map if unexpected behavior occurs.
- Consider using log statements to track the progress of your insertions.
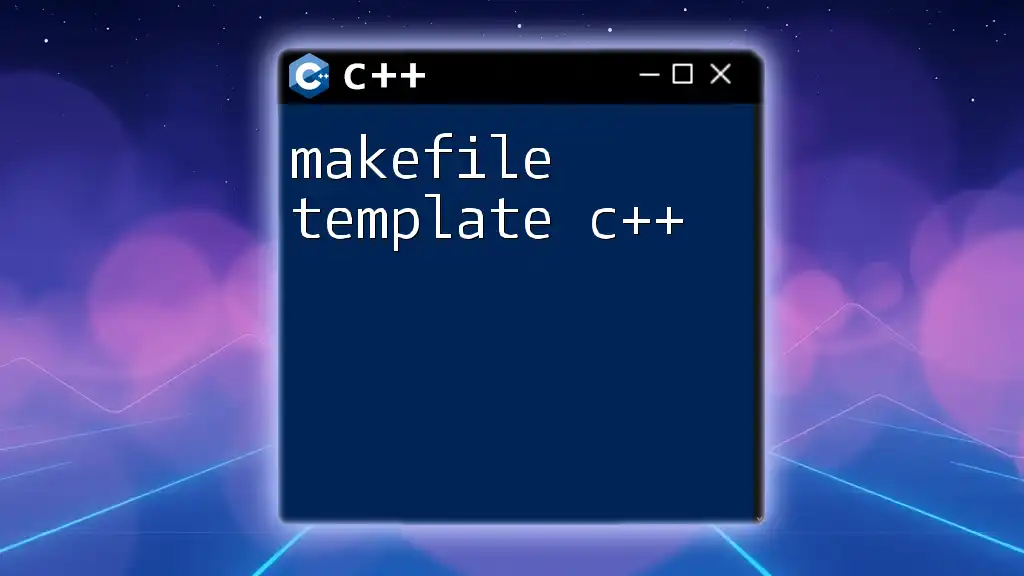
Conclusion
Understanding `map emplace c++` is essential for efficient map usage in your C++ applications. By taking full advantage of the `emplace` method, you can streamline your code, reduce unnecessary overhead, and enjoy optimal performance while managing key-value pairs in maps. Practice with the examples provided, and experiment with your implementations to become proficient in this powerful feature!
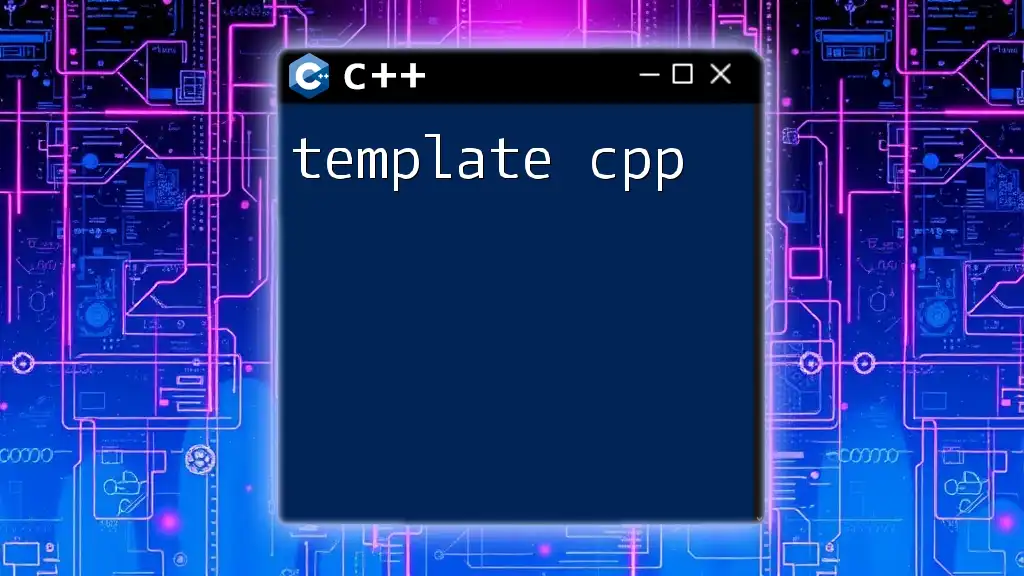
Additional Resources
- Consider exploring recommended books for further learning in C++, such as "Effective C++" by Scott Meyers.
- Online courses or tutorials can provide hands-on practice with C++ maps and `emplace`.
- For official documentation, visit [cppreference.com](https://en.cppreference.com/w/cpp/container/map) for comprehensive details on maps and their methods.