The `emplace` function in C++ allows you to construct an object in place within a container, using the provided arguments to directly forward to the constructor, thereby avoiding unnecessary copies.
Here's a code snippet demonstrating its use with `std::vector`:
#include <vector>
#include <iostream>
struct Point {
int x, y;
Point(int x, int y) : x(x), y(y) {}
};
int main() {
std::vector<Point> points;
points.emplace_back(1, 2); // Construct Point(1, 2) directly in the vector.
std::cout << "Point: (" << points[0].x << ", " << points[0].y << ")\n";
return 0;
}
Understanding C++ Emplace
What is emplace? In the context of C++, emplace is a function that allows for the construction of an object in place, directly within a container. It eliminates the need for unnecessary copies or moves, which can enhance performance.
When compared to methods like `push_back` and `insert`, emplace stands out by constructing the element directly at the desired location, rather than creating a temporary object. This can lead to notable performance gains, particularly in situations involving complex or expensive-to-copy objects.
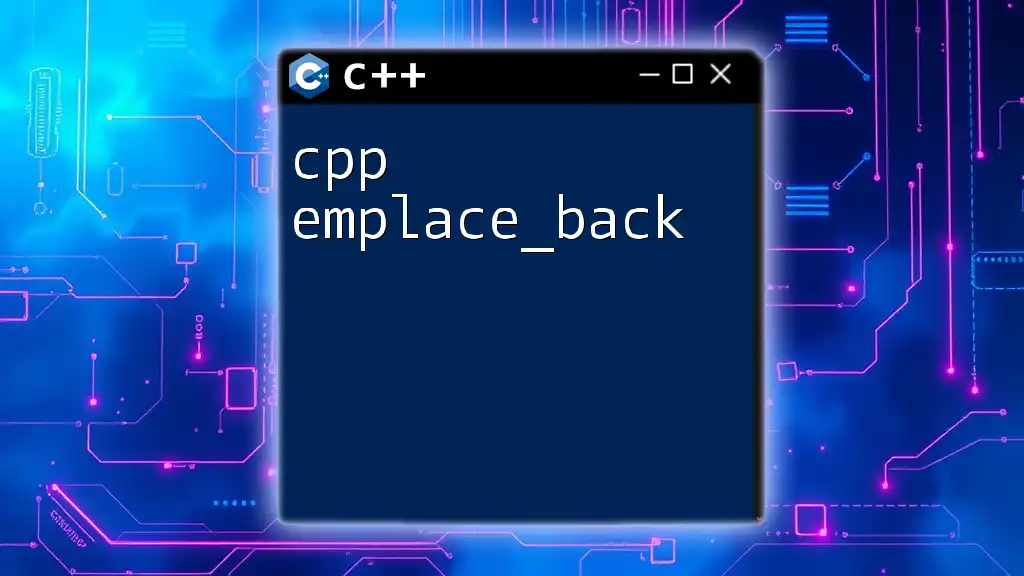
C++ Standard Library Containers
C++ offers a variety of standard library containers, including `std::vector`, `std::unordered_map`, and `std::list`. Each of these containers can leverage emplace to improve performance.
Value Semantics vs. Reference Semantics
It's essential to understand the difference between value semantics and reference semantics when employing emplace. Value semantics create copies of objects, leading to potentially costly operations, while reference semantics allow objects to be referred to without being copied, making operations more efficient.
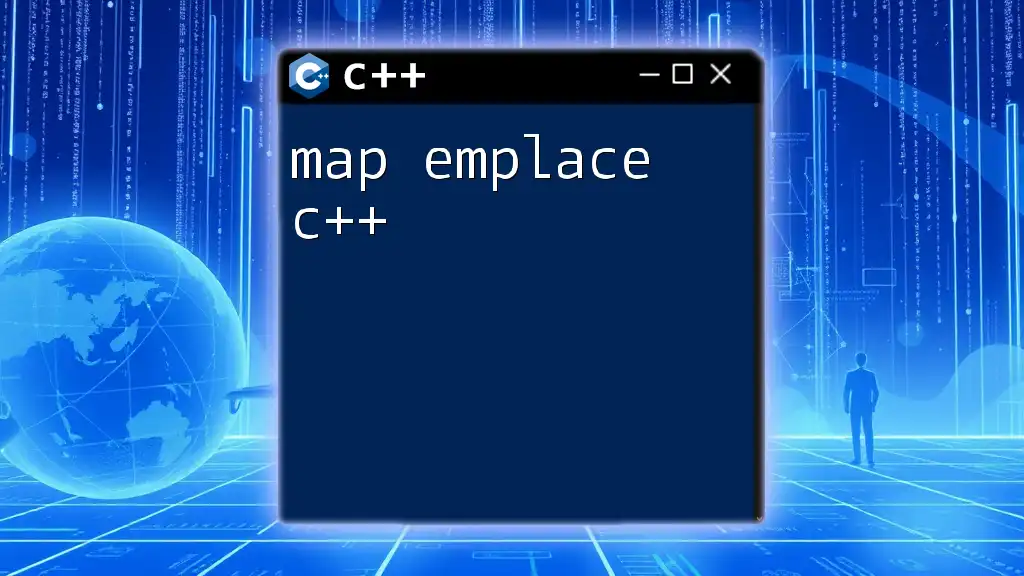
Emplace Versus Insert and Push Back
Push Back vs. Emplace
The `push_back` method is widely known for adding elements to the end of a container. For instance:
std::vector<std::string> myVector;
myVector.push_back("Hello");
This method first constructs a temporary `std::string`, then copies/moves it into the vector, which can lead to unnecessary resource usage.
In contrast, using emplace:
std::vector<std::string> myVector;
myVector.emplace_back("Hello");
When using `emplace_back`, the string is constructed directly in the vector's memory space, eliminating the need for a temporary object. This makes emplace generally more efficient than push_back, especially for complex objects.
Insert vs. Emplace
The `insert` method allows elements to be added to a specific position within a container:
std::vector<int> myVector;
myVector.insert(myVector.begin(), 42);
Similar to push_back, insert typically requires constructing a temporary object before it is copied or moved into the destination.
Conversely, using emplace allows direct construction:
std::vector<int> myVector;
myVector.emplace(myVector.begin(), 42);
Here, the integer `42` is constructed directly in the vector’s space, improving performance by avoiding extra copies.
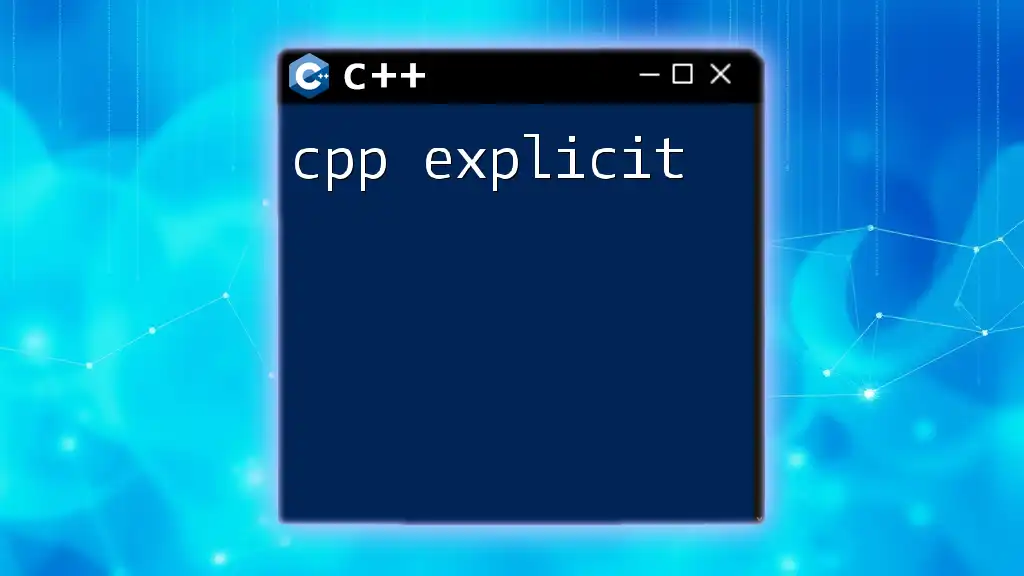
Using Emplace in C++ Containers
Emplacing Elements in std::vector
When working with `std::vector`, emplacing allows for efficient addition of elements.
Example: Basic Emplacement
std::vector<std::pair<int, int>> vec;
vec.emplace_back(1, 2); // Constructs the pair in place
Detailed Breakdown of Arguments: The arguments provided to `emplace_back` are perfectly forwarded to the constructor of the `std::pair`, allowing for efficient in-place creation.
Emplacing Key-Value Pairs in std::unordered_map
Emplace is particularly useful when inserting key-value pairs into a `std::unordered_map`.
Code Example: Using Emplace for Maps
std::unordered_map<int, std::string> map;
map.emplace(1, "One");
In this case, the key-value pair is constructed directly within the map’s internal structure, minimizing memory allocation overhead.
How Emplace Affects Constructor Calls: Emplace utilizes perfect forwarding to pass the arguments to the constructor of the element type, which avoids unnecessary object copies and boosts efficiency.
Emplacing Objects in std::list
Linked lists can also benefit from emplace. Here, it’s about efficiency in the insertion of nodes.
Code Example: Emplacing into List
std::list<int> myList;
myList.emplace_front(5); // Constructs the element in place at front
This method constructs the integer directly at the front of the list, avoiding the overhead associated with node creation and value copy.
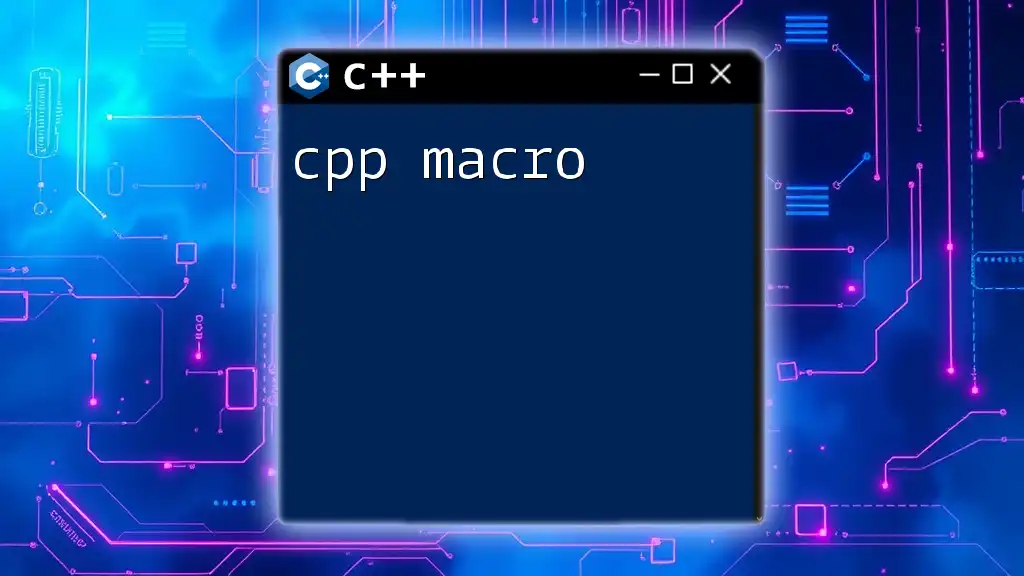
Performance Implications of Emplace
Memory Allocation and Efficiency
Using emplace notably reduces memory allocation and copying overhead, especially for complex objects that might otherwise need to be duplicated. By constructing objects directly within the container, significant performance improvements can be realized, particularly in performance-sensitive applications.
Best Practices for Using Emplace
-
When to Prefer Emplace:
- Use emplace when you need to create an object directly in a container and want to avoid the overhead of temporary objects.
- If you're dealing with user-defined types or complex objects that have non-trivial constructors or destructors, emplace is the way to go.
-
Situations That Benefit from Using Emplace:
- Performance-critical sections of code.
- Large data sets where memory management is a concern.
- Containers holding objects that are expensive to copy/move.
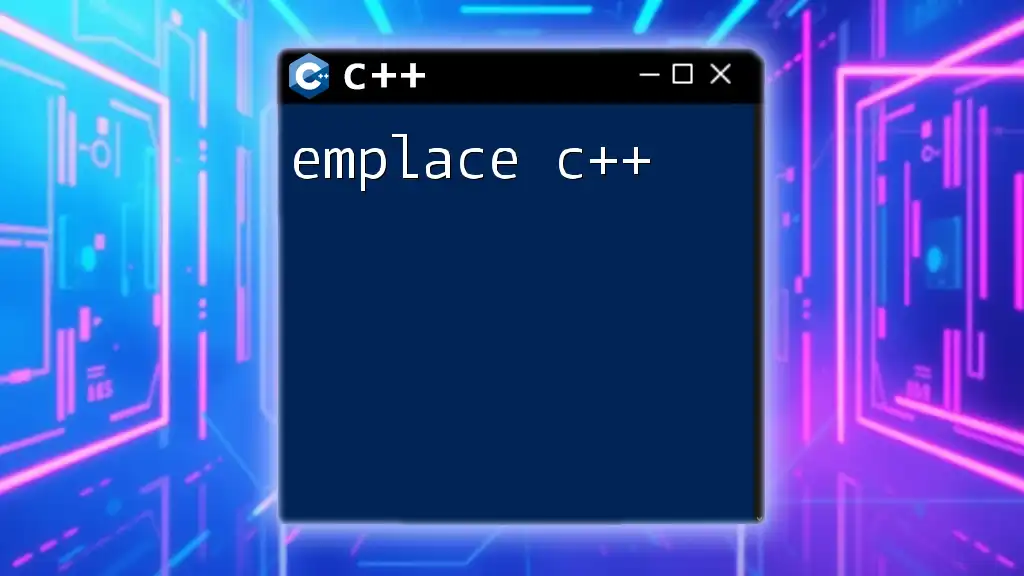
Conclusion
In summary, the use of `cpp emplace` can lead to significant performance enhancements by allowing for in-place object construction within containers. This guide has provided insights into the benefits of emplace over traditional methods like `push_back` and `insert`, along with practical examples. Practicing these concepts will solidify your understanding and efficiency in using C++. Embrace emplace, and elevate your C++ programming skills!
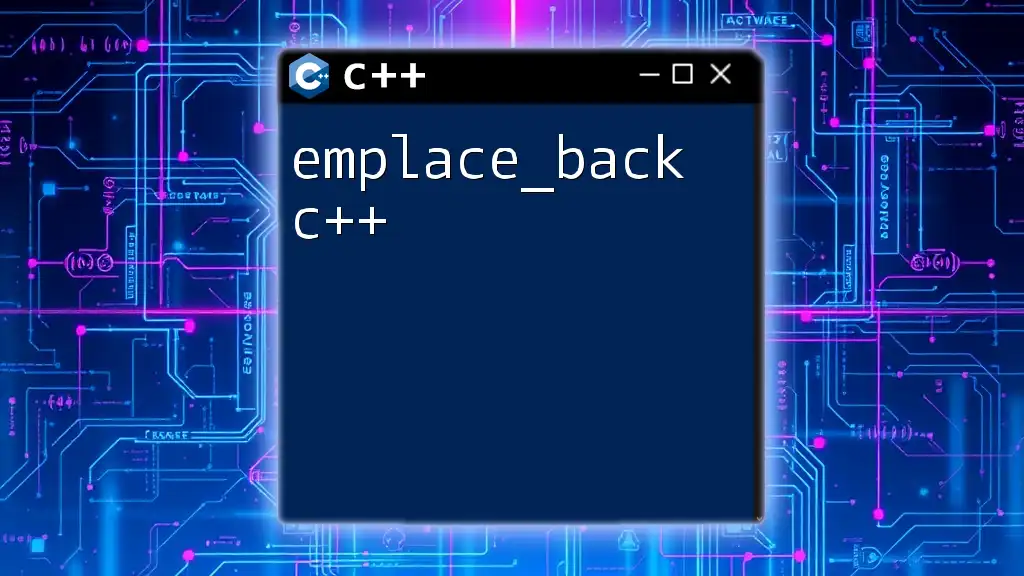
Additional Resources
For further reading and tutorials, consider exploring the C++ documentation on emplace and related topics. Various books and online courses are also available to deepen your understanding and practical skills in mastering C++.