A Makefile in C++ is a special file used by the `make` utility to automate the compilation of programs, defining rules and dependencies for building targets from source files.
Here's a simple example of a Makefile for a C++ project:
# Makefile example for a simple C++ project
CXX = g++
CXXFLAGS = -Wall -Wextra -g
TARGET = my_program
SRCS = main.cpp utils.cpp
OBJS = $(SRCS:.cpp=.o)
all: $(TARGET)
$(TARGET): $(OBJS)
$(CXX) $(CXXFLAGS) -o $@ $^
%.o: %.cpp
$(CXX) $(CXXFLAGS) -c $<
clean:
rm -f $(OBJS) $(TARGET)
This Makefile compiles the source files `main.cpp` and `utils.cpp` into an executable named `my_program`, and includes a clean target to remove the generated files.
What is a Makefile?
A Makefile is a special file used to control the build process of a project. It serves as a script for the make utility, which automates the compilation of source code into executable programs. Makefiles are critical in C++ development as they streamline the process of managing multiple source files, compiling them, and linking them together.
A Makefile consists of a series of rules that specify how to derive one file from another, essentially outlining how the project should be built.
History and Evolution of Makefiles
Makefiles have their origins in early software development when it was crucial to manage the compilation process of complex projects with multiple source files. Over time, they evolved from simple scripts into sophisticated build systems that support various compilation flags, dependencies, and even integration with version control systems.
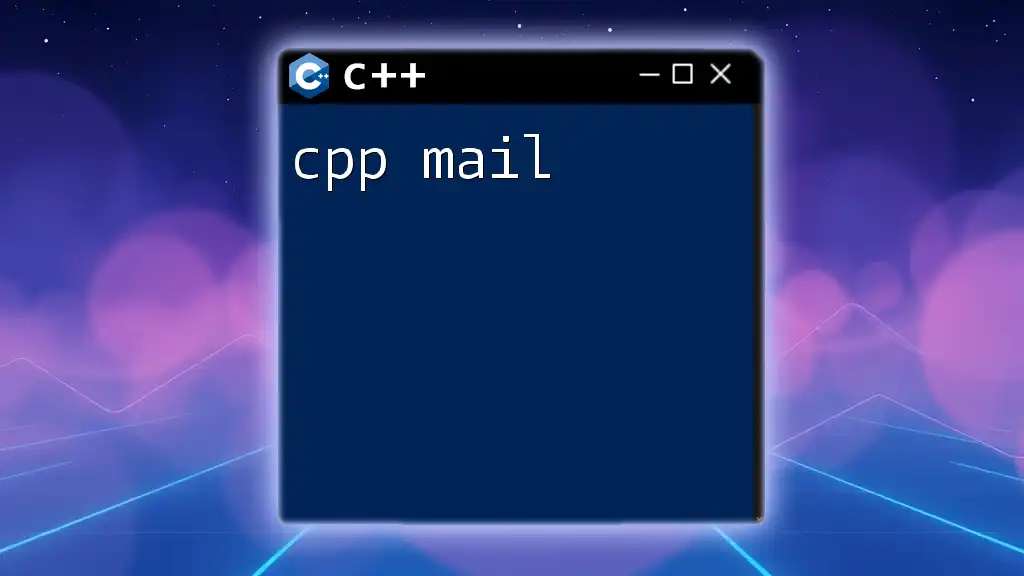
Fundamentals of Makefiles
The Make Command
The `make` command reads the content of a Makefile and executes the defined rules. It checks if the target (the file you want to build) needs to be updated by comparing timestamps between the target and its dependencies. If a dependency has changed more recently than the target, `make` executes the associated commands to update the target.
Key Components of a Makefile
Understanding the essential components of a Makefile is crucial for its effective utilization.
- Targets: These are the files that you want to build. A target can also be an action like `clean`.
- Dependencies: These are the files that must be updated before the target can be built.
- Commands: These are the instructions executed to create or update the target.
Here's an example of a simple Makefile structure:
target: dependencies
command
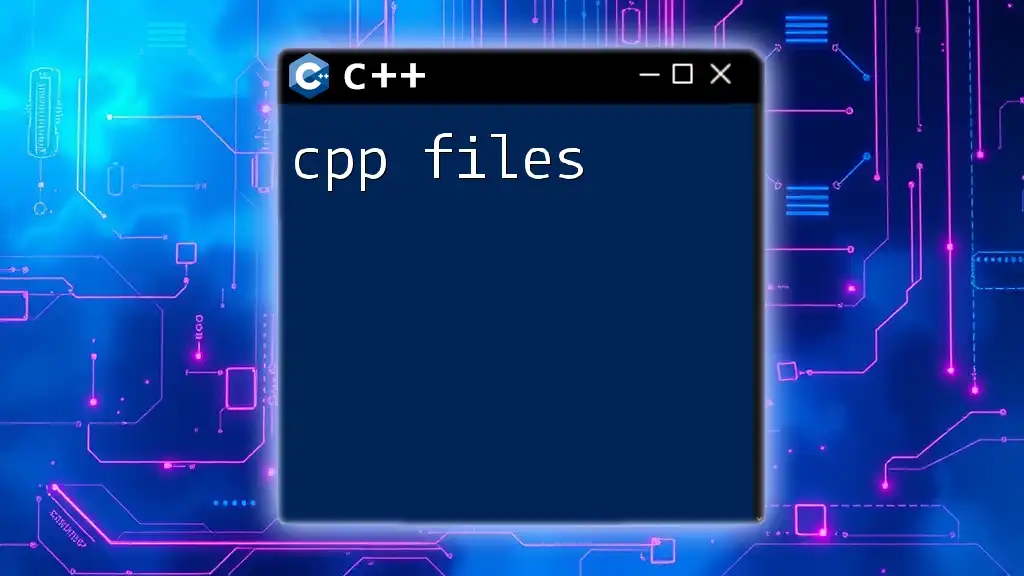
Structure of a C++ Makefile
Basic Makefile Syntax
A C++ Makefile typically includes compiler commands, source files, and the compilation rules needed to generate executable files. Below is an example of a basic C++ Makefile:
CC = g++
CXXFLAGS = -Wall -g
my_program: main.o helper.o
$(CC) -o my_program main.o helper.o
In this example, `CC` represents the compiler used (G++ in this case), while `CXXFLAGS` holds the compiler flags for warnings and debugging. This structure makes the Makefile clearer and more maintainable.
Variables in Makefiles
Variables play a significant role in Makefiles by allowing you to define and reuse values throughout your script.
Commonly Used Variables
- CC: The compiler to use (e.g., `g++` for C++).
- CXXFLAGS: Flags for all C++ files (e.g., `-Wall` for enabling all warnings).
- LDFLAGS: Flags for the linker.
You can define and use these variables as shown below:
CC = g++
CXXFLAGS = -Wall -g
Creating Targets
Building Executables
In a Makefile, targets specify how to create executables from object files. For instance, consider this target for an executable:
my_program: main.o helper.o
$(CC) -o my_program main.o helper.o
This rule tells the make utility to build the target `my_program` by linking the object files `main.o` and `helper.o` using the `g++` command defined in the `CC` variable.
Clean Up with Makefile
Including a clean-up target in your Makefile is significant to maintain a clutter-free directory. It helps remove all object files and executables that are generated during the build process. Here’s an example of a clean target:
clean:
rm -f *.o my_program
By running the command `make clean`, users can easily clear out old builds, ensuring that they start from a clean slate.
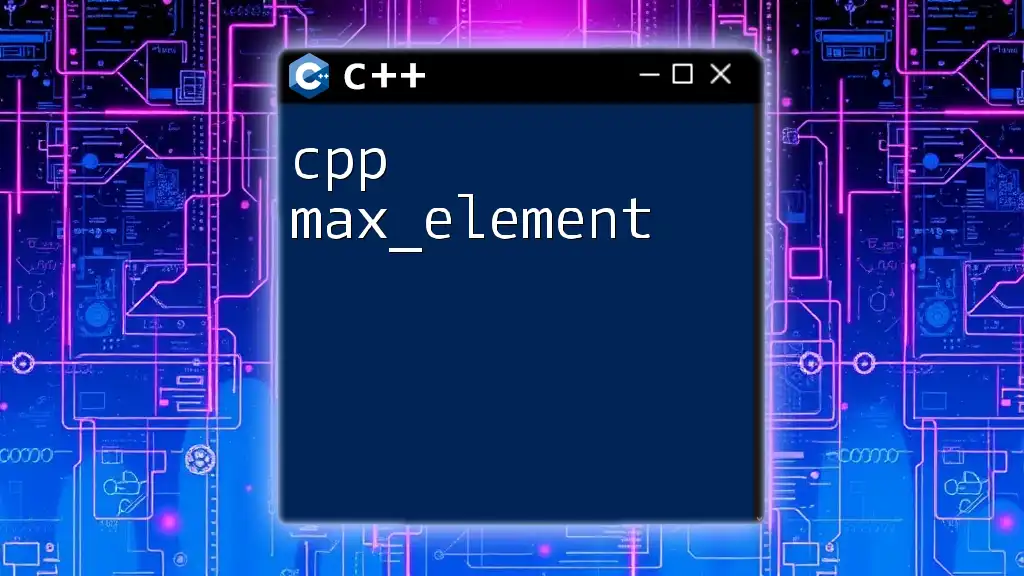
Advanced Makefile Techniques
Pattern Rules
Pattern rules allow you to define a template for building files without explicitly specifying every target. They can simplify your Makefile when you have multiple source files that follow a consistent naming convention.
Here’s an example of using pattern rules for compiling multiple C++ files:
%.o: %.cpp
$(CC) $(CXXFLAGS) -c $< -o $@
In this rule, `%.o` represents any object file corresponding to a source file `%.cpp`. The `$<` is an automatic variable that refers to the first prerequisite, while `$@` corresponds to the target.
Conditional Statements in Makefiles
Conditional statements can make your Makefile more dynamic by executing specific commands based on certain conditions, such as the presence of files or environment variables.
For example:
ifeq ($(DEBUG), true)
CXXFLAGS += -g
endif
This condition checks if a variable named `DEBUG` is set to `true`, and if so, it appends the `-g` flag to `CXXFLAGS`.
Including Other Makefiles
For larger projects, it’s best practice to organize your Makefiles logically. You can use the `include` directive to incorporate other Makefiles, allowing for a modular design. This is useful to keep common rules or variables in a separate file.
include common.mk
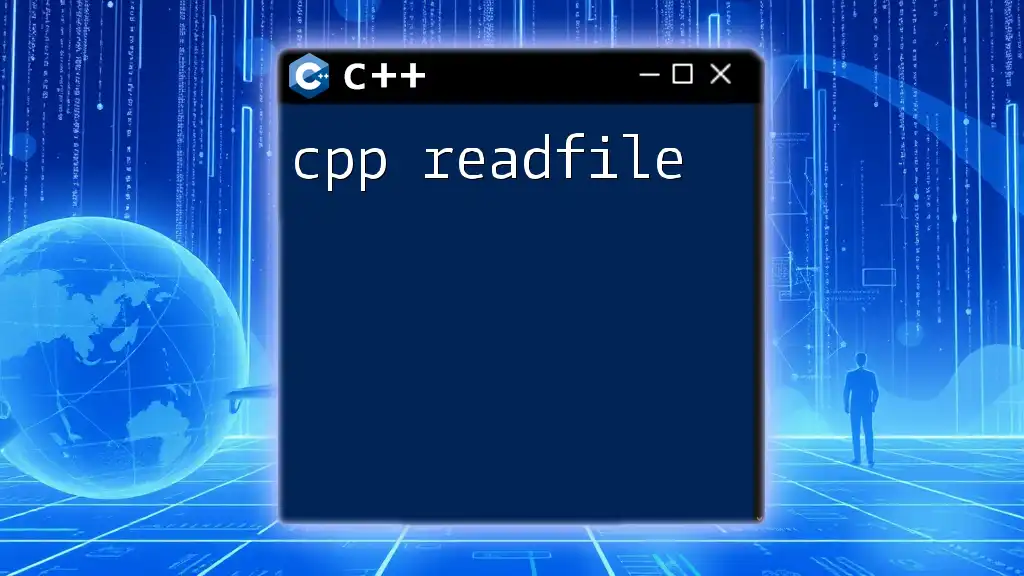
C++ Project Organization with Makefiles
Directory Structure Best Practices
While creating a C++ Makefile, organizing your project directory efficiently enhances maintainability. A recommended structure includes separate folders for source files, headers, and object files, like so:
/my_project
/src
main.cpp
helper.cpp
/include
helper.h
/bin
/obj
This layout keeps different types of files structured, making it easier to manage as the project grows.
Version Control Integration
Integrating your Makefile with version control systems like Git aids in creating consistent builds. By defining a clean target and ensuring no generated files are committed, you help maintain a clean repository and reduce merge conflicts.
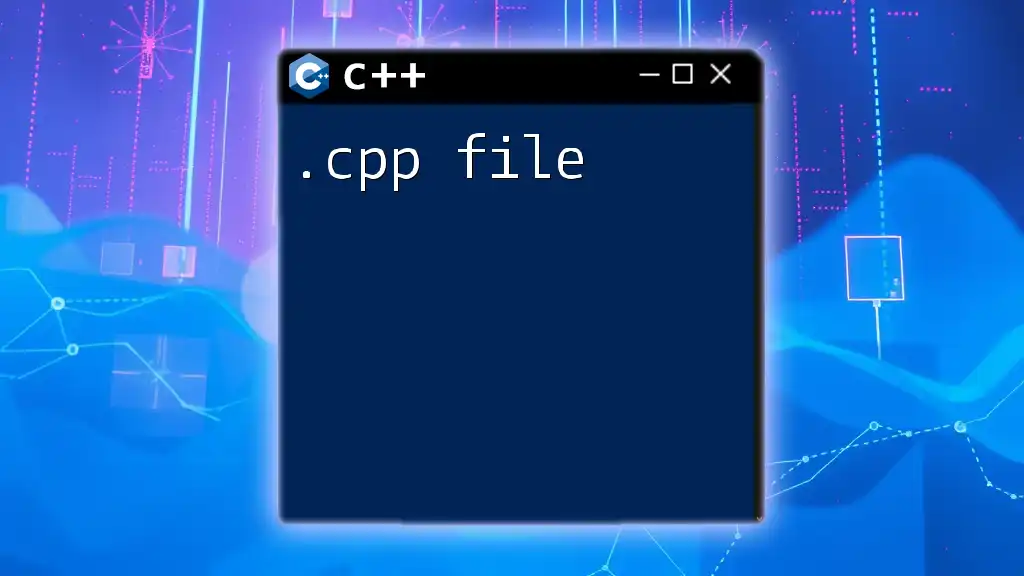
Debugging and Troubleshooting Makefiles
Common Errors and Solutions
Errors in a Makefile often arise due to incorrect targets or missing files. For instance, if `make` complains about missing `.o` files, ensure that the corresponding `.cpp` files exist and the paths are correct.
Using Verbose Mode
Verbose mode is crucial for debugging your Makefile. It provides detailed output so you can see precisely what commands are being executed. To enable verbose output, you can run the following command:
make --debug
This command will give insights into dependencies and the commands being executed, helping you to track down issues more effectively.
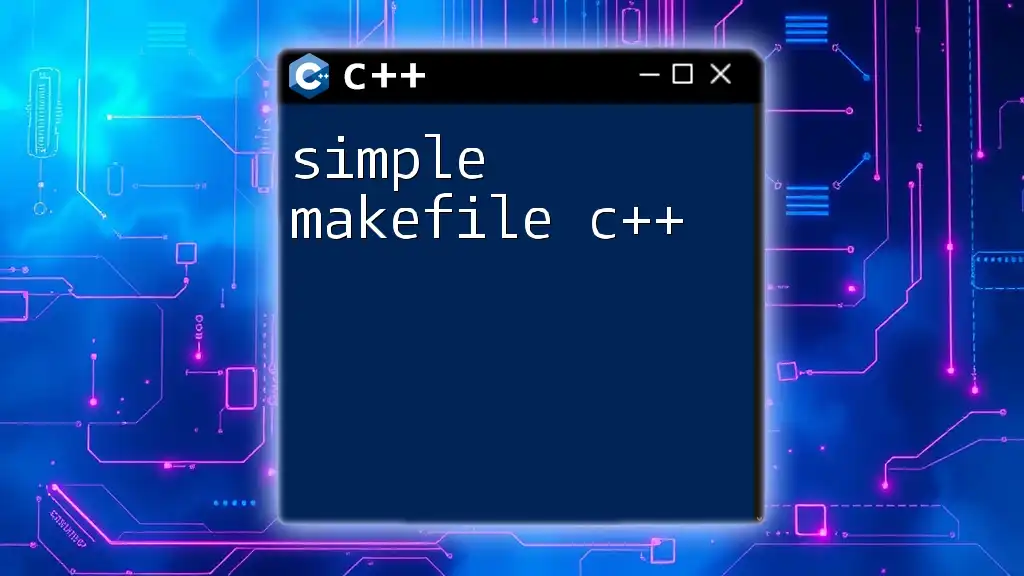
Conclusion
C++ Makefiles are a powerful way to streamline the process of building projects. They enable developers to manage complex builds, making it easier to handle multiple source files, dependencies, and compilation commands. As you gain more experience with C++ Makefiles, you'll find that experimenting with advanced techniques and regularly refining your Makefiles can significantly enhance your development process.
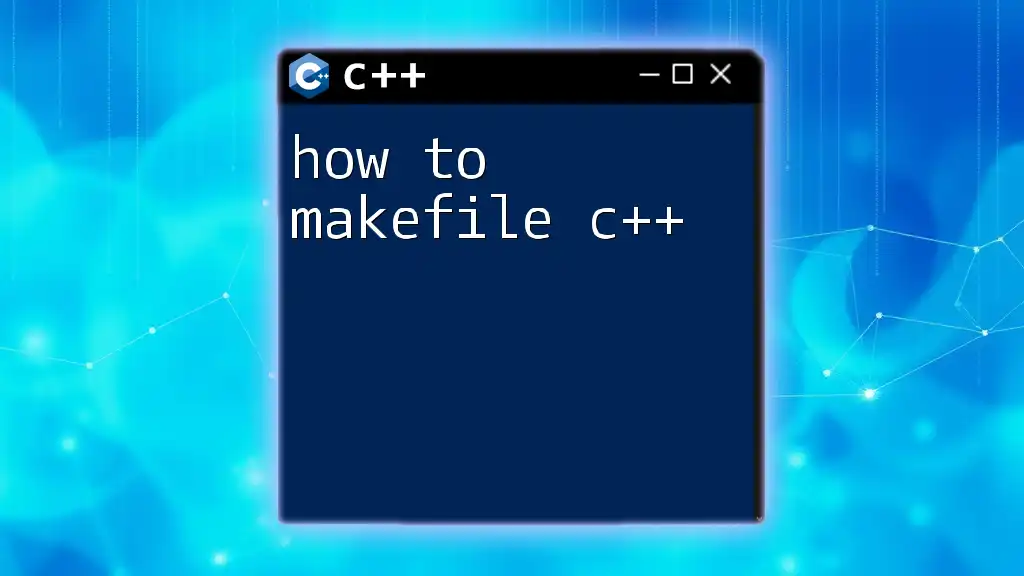
Additional Resources
For more detailed information on C++ Makefiles, consider the official documentation for the GNU Make utility, as well as numerous community-driven forums and tutorials available online.
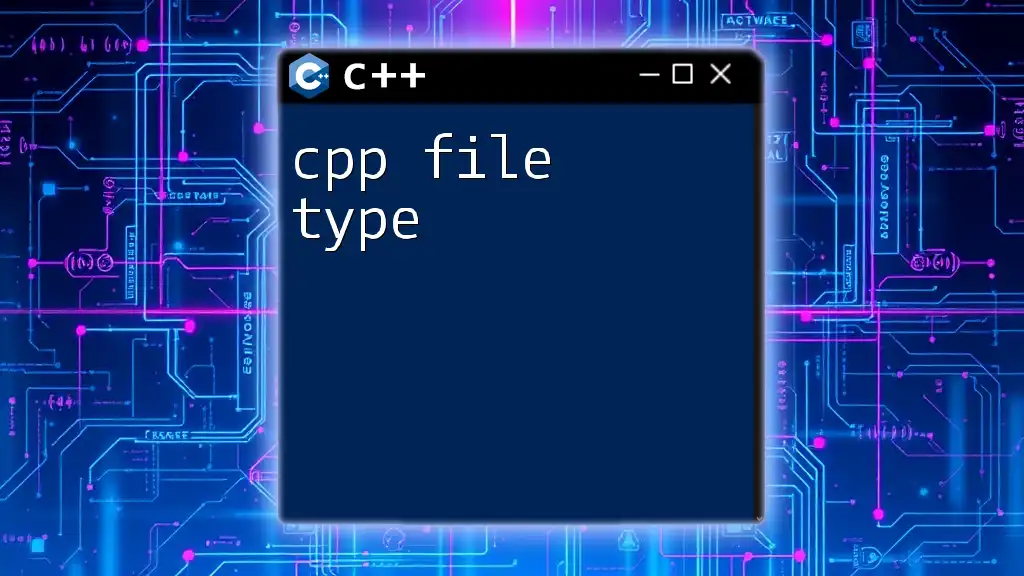
FAQs about C++ Makefiles
What is the difference between a Makefile and a build system?
While a Makefile is a specific file format used by the `make` tool to define the build process, a build system encompasses the broader environment and tools used for building software projects. Build systems can include frameworks like CMake, which offer more features and flexibility than traditional Makefiles.
How do I incorporate library dependencies in my Makefile?
To incorporate library dependencies, you can specify the libraries using the `-l` option in your linker command. For example:
my_program: main.o helper.o
$(CC) -o my_program main.o helper.o -lmylibrary
In this example, `-lmylibrary` tells the linker to link against the specified library.
Are there alternatives to Makefiles for C++ projects?
Yes, alternatives like CMake, SCons, and Ninja provide more features than traditional Makefiles, such as better support for cross-platform builds, advanced dependency management, and an easier learning curve for more novice programmers. Exploring these tools can yield significant benefits as your projects grow in complexity.