A `.cpp` file is a source code file in C++ that contains declarations and definitions in the C++ programming language, which can be compiled into an executable program.
Here's an example of a simple C++ program contained in a `.cpp` file:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is a .cpp File?
A .cpp file is a C++ source file where you can write your C++ code. The extension .cpp indicates that the file contains C++ program code, which the compiler can translate into machine language for execution. Each .cpp file serves as a container for functions, classes, and data that form the building blocks of your program.
The importance of the .cpp file in C++ programming cannot be overstated. When you develop software in C++, you will organize your code into manageable pieces, and .cpp files play a crucial role in this organization. They help in separating implementation from declarations, making your code cleaner and more modular.
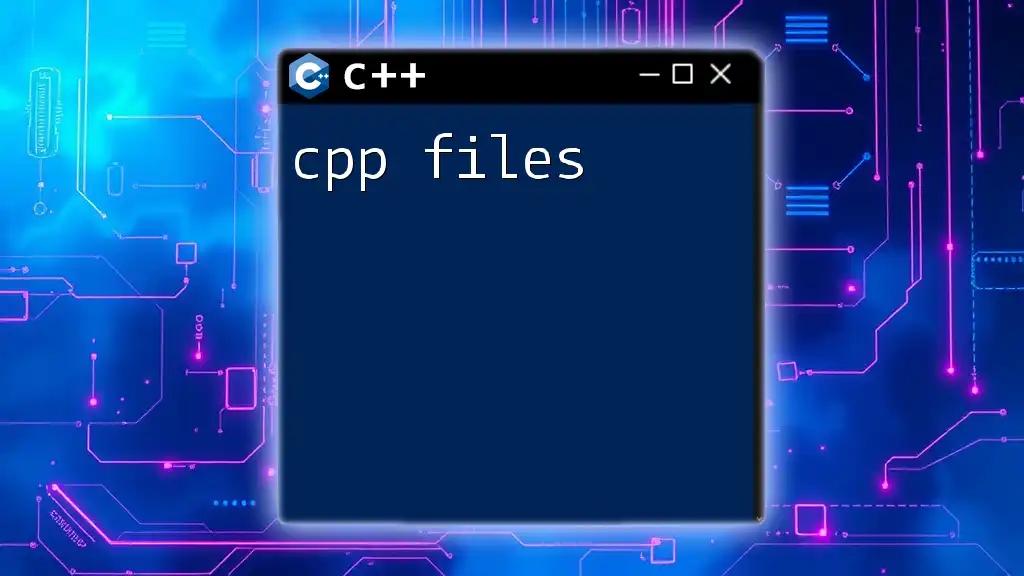
Why Use .cpp Files in C++ Programming?
Using .cpp files in your C++ projects offers several advantages:
- Modularity: By separating your code into different .cpp files, you create a modular structure, making it easier to maintain and update programs.
- Reusability: Functions and classes within .cpp files can be reused across multiple projects, promoting code reusability.
- Collaboration: When working in teams, multiple developers can work on different .cpp files simultaneously, streamlining collaboration.
Comparing with other file types, such as .h files (header files), we see that .cpp files typically contain the definitions and implementations of functions and classes, while .h files generally contain declarations and shared interfaces.

Creating a .cpp File
Setting Up Your Development Environment
Before you can start coding, you need a suitable development environment. Popular Integrated Development Environments (IDEs) and text editors that support C++ include:
- Visual Studio: Known for its powerful debugging tools and user-friendly interface.
- Code::Blocks: An open-source IDE that's lightweight and easy to use.
- CLion: A cross-platform IDE with intelligent coding assistance.
Make sure you have installed a C++ compiler, such as GCC or Clang, which you'll use to compile your .cpp files into executable programs.
Writing Your First .cpp File
The structure of a typical .cpp file is straightforward. It usually begins with including necessary libraries, followed by the main function, which is the starting point of any C++ program. Here’s an example of a simple program written in a .cpp file:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example:
- `#include <iostream>`: This line imports the standard input-output stream library, allowing the use of `std::cout`.
- `int main() { ... }`: This defines the main function that the C++ runtime looks for when executing the program.
- The line `std::cout << "Hello, World!" << std::endl;` outputs the string "Hello, World!" to the console.
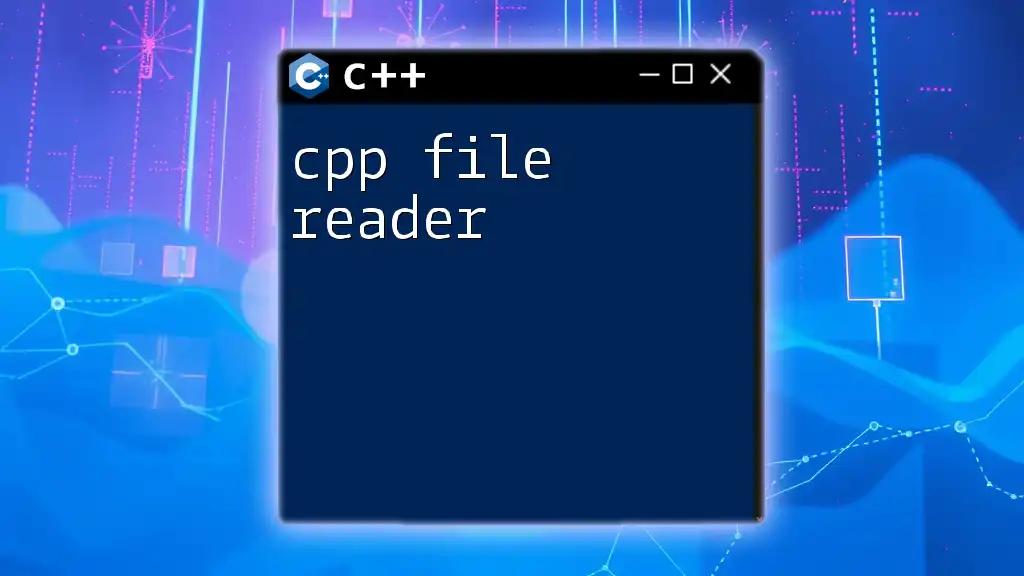
Compiling and Running .cpp Files
Compilation Process Explained
When you write code in a .cpp file, the compiler translates your human-readable code into machine-readable code through a process known as compilation. This involves several steps, including preprocessing, compiling, assembling, and linking.
How to Compile a .cpp File
The command used to compile a .cpp file can vary depending on your compiler. For example, using GCC, you can compile your program with the following command:
g++ -o hello hello.cpp
In this command:
- `g++` is the compiler.
- `-o hello` specifies the output filename (in this case, `hello`).
- `hello.cpp` is the source file you are compiling.
Running Your Compiled Program
After your .cpp file compiles successfully, you will have an executable file. You can run it from the command line as follows:
./hello
Executing this command will display the output of your program, which should show "Hello, World!" on the console, assuming you used the above initial code sample.
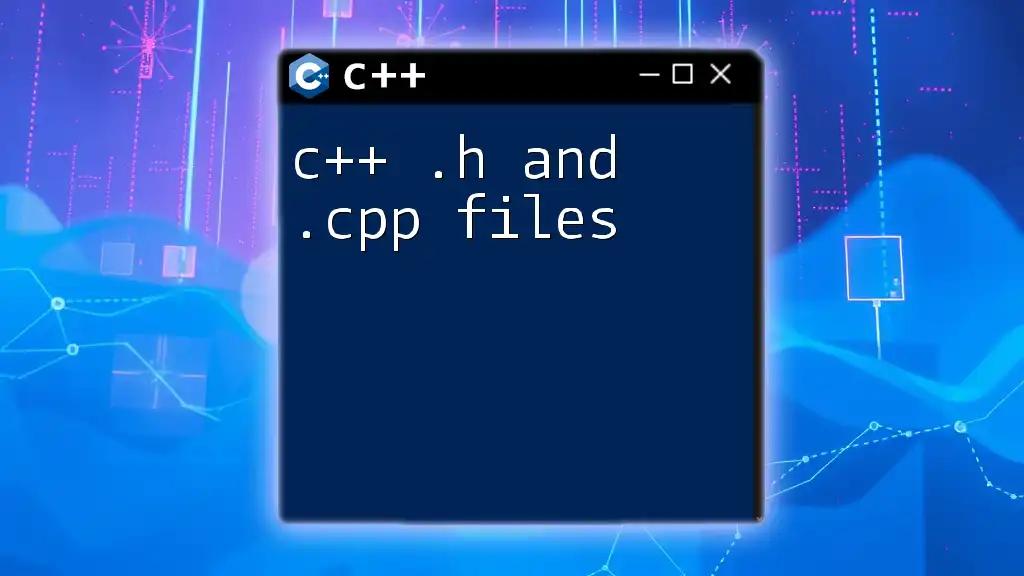
Best Practices for .cpp Files
Organizing Your Code
To maintain readability and organization in your .cpp files, consider structuring your code with clear logical sections. Use whitespace and indentation to improve visibility and break complex code into manageable parts.
Using Comments Effectively
Comments are essential in C++ for providing clarity and context to the code. There are two types of comments:
- Single-line comments start with `//` and apply to everything on that line.
- Multi-line comments are enclosed between `/` and `/` and can span several lines.
Using comments wisely can help others (and your future self) understand the reason behind your code decisions.
Debugging Tips for .cpp Files
Debugging is a crucial part of programming. Common issues to look out for include syntax errors, logical errors, and runtime errors. To debug effectively, consider the following practices:
- Use a debugger tool integrated into your IDE.
- Print statements can help track variable values and program flow during execution.
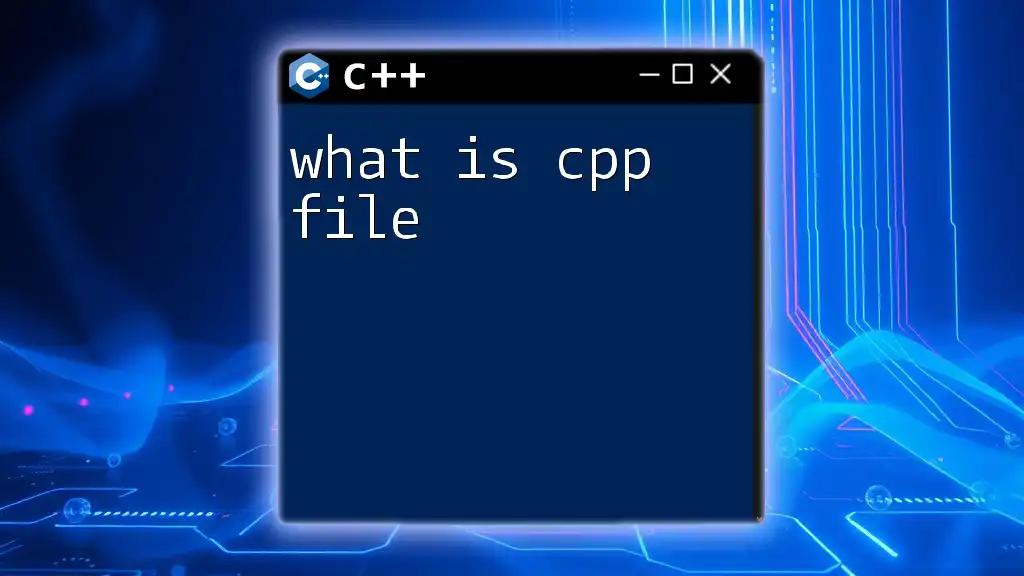
Advanced Features of .cpp Files
Header Files and .cpp Files
When coding in C++, you'll often use header files (.h) alongside your .cpp files. Header files declare functions and classes, while .cpp files contain their implementations. You include a header file in your .cpp file using the syntax:
#include "myHeader.h"
This statement allows the compiler to understand the prototypes you are using in your .cpp file.
Namespaces in .cpp Files
Namespaces prevent name conflicts by grouping related classes, functions, and variables. For example, using the `std` namespace prevents ambiguity when using standard library functions. You can utilize namespaces as follows:
#include <iostream>
using namespace std;
int main() {
cout << "Using namespaces!" << endl;
return 0;
}
Classes and Objects in .cpp Files
C++ is an object-oriented language, and classes are the blueprint for creating objects. Here's a simple example of defining a class in a .cpp file:
#include <iostream>
class Dog {
public:
void bark() {
std::cout << "Woof! Woof!" << std::endl;
}
};
In this code, we define a class `Dog` with a public method `bark()`, which outputs "Woof! Woof!" when called.
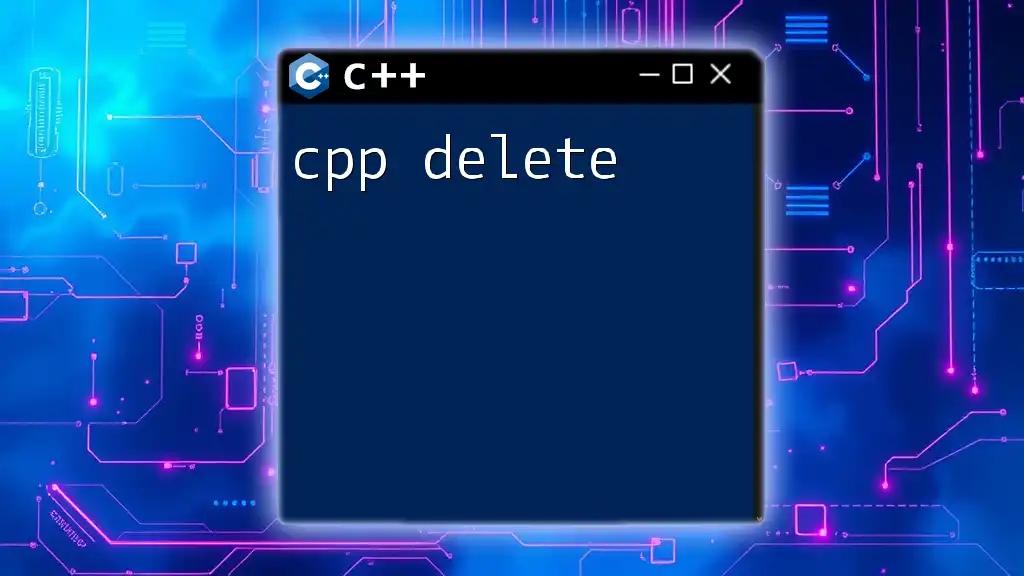
Recap of Key Points
In this article, we explored the vital role of .cpp files in C++ programming. From defining what a .cpp file is, to compiling and running your code, we covered the essential aspects to help you become more proficient. We also touched on best practices and advanced features that enhance your programming skills.
Further Learning Resources
For those looking to deepen their understanding of C++, consider the following resources:
- Comprehensive C++ books, such as "C++ Primer" and "Effective Modern C++".
- Online courses from platforms like Coursera or Udemy.
- Engaging with online communities on Reddit or Stack Overflow for Q&A and shared learning.
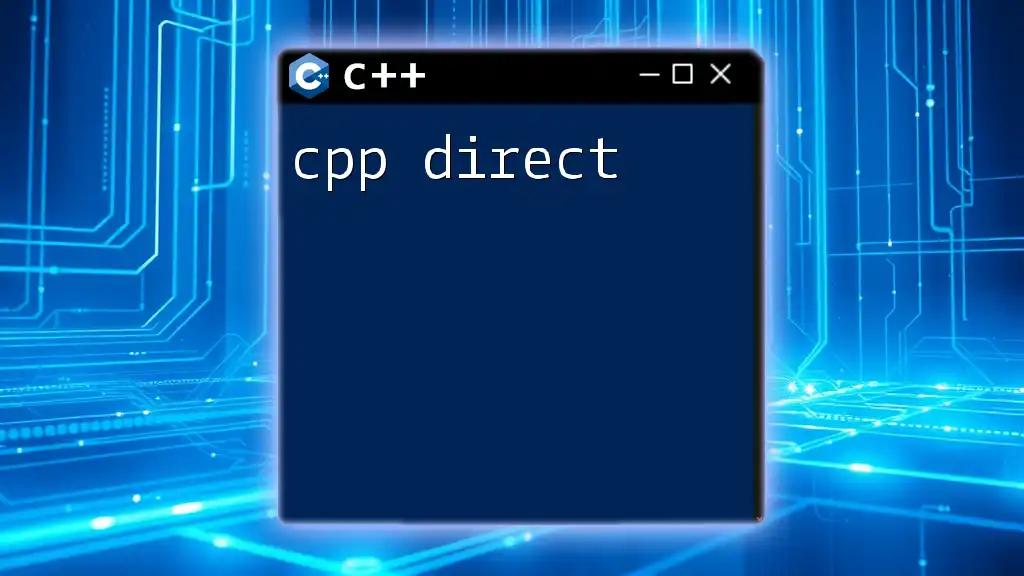
Call to Action
Now that you've learned about .cpp files, it's time to put your knowledge into practice. Start by creating and compiling your .cpp files. Don’t hesitate to share your experiences or pose questions in the comments section below. Happy coding!