A CPP file is a source file used in C++ programming that contains code written in the C++ programming language, which can be compiled to create executable programs.
Here’s a simple example of a CPP file containing a basic "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What Is a CPP File?
Definition of a CPP File
A CPP file is a source code file used in programming with the C++ language. The term "CPP" stands for "C Plus Plus." This file is crucial for storing the code that developers write to implement logic and functionality in C++ applications. Essentially, a CPP file contains all the necessary instructions that the C++ compiler will translate into machine code, making it executable by a computer.
Characteristics of CPP Files
File Extension: One of the defining features of a CPP file is its .cpp file extension. This extension helps both programmers and compilers identify the file as containing C++ source code. When you see a file ending in .cpp, you know it is designed to be compiled with a C++ compiler.
Text Files: CPP files are plain text files. This means that they can be opened and edited with any standard text editor, allowing for easy code editing and manipulation without requiring any specialized software.
Compiler Relevance: CPP files work in tandem with compilers—tools that convert the C++ code written in CPP files into machine language that the computer can execute. This relationship is essential for software development and allows programmers to transform their human-readable code into the instructions necessary for a computer to perform tasks.
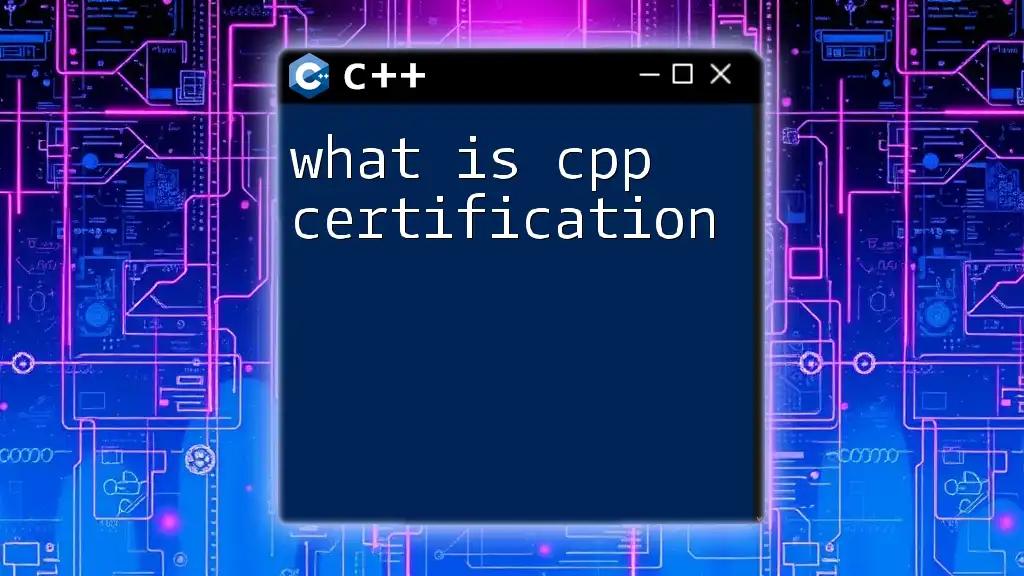
The Purpose of CPP Files
Source Code Storage
One of the primary purposes of CPP files is to serve as storage for the C++ source code. Each CPP file typically holds specific functions or classes, enabling modular programming. Modular programming is a practice that enhances code maintainability and readability by breaking down complex systems into smaller, manageable components.
Example: A simple C++ program may be stored in a CPP file as shown below:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this example, the CPP file contains the necessary instructions to output "Hello, World!" to the console. Each CPP file can be compiled separately or linked with other CPP files in larger projects, showcasing the flexibility of using CPP files for code organization.
Project Organization
CPP files play a vital role in organizing code, especially in larger projects. Effective organization can significantly improve the development process and help teams collaborate more efficiently. By using multiple CPP files, developers can separate different functionalities into distinct files. This separation of concerns allows programmers to focus on one aspect of the application at a time without getting overwhelmed by the entire codebase.
Modularity: Modularity is a principle that involves creating independent modules of code that work together. By storing related functions or classes in separate CPP files, developers can manage and maintain their codebase easily.
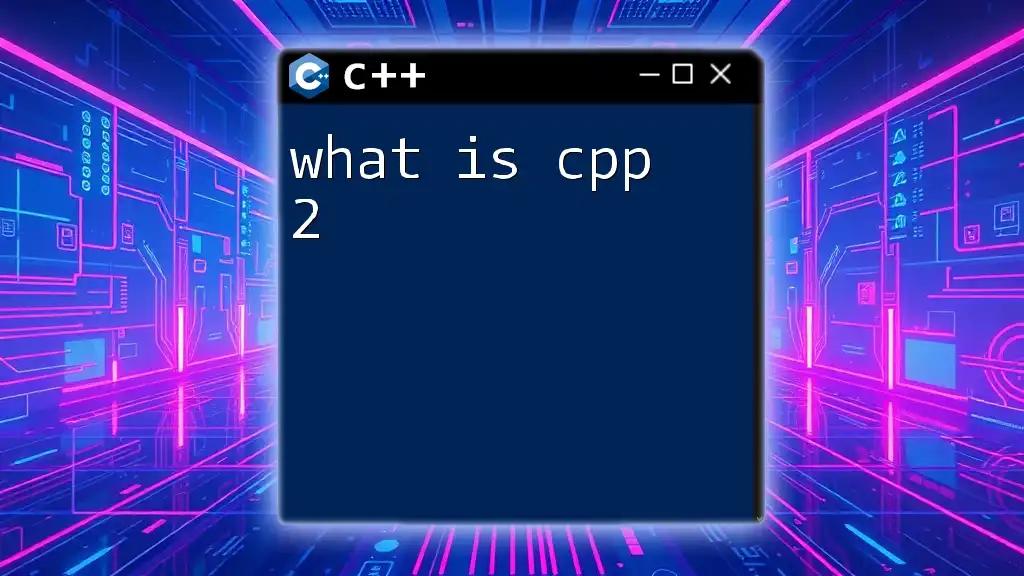
CPP File Structure
Basic Components of a CPP File
Understanding the basic components of a CPP file is essential for effective programming in C++.
Preprocessor Directives: These directives, which begin with a '#' symbol, instruct the preprocessor to include header files or define macros before compilation begins. For example, the directive `#include <iostream>` includes the I/O stream library, which enables the use of input and output operations in your program.
Main Function: Most C++ programs start with the `main()` function. This is the entry point of any C++ program. The structure typically looks like this:
int main() {
// Your code here
return 0;
}
This function must return an integer; returning `0` usually indicates successful execution of the program.
Namespaces: C++ uses namespaces to avoid name collisions. The `using namespace std;` directive allows the code to utilize names from the C++ Standard Library without needing to prefix them with `std::`.
Code Comments and Documentation
Writing comments in CPP files is pivotal for keeping code understandable. Comments not only help others read your code but can also remind you of your logic after some time has passed.
Example: There are different types of comments you can use in CPP files:
// This is a single-line comment
/* This is a
multi-line comment */
Using comments effectively enhances code readability and allows developers to convey the purpose of specific code sections.
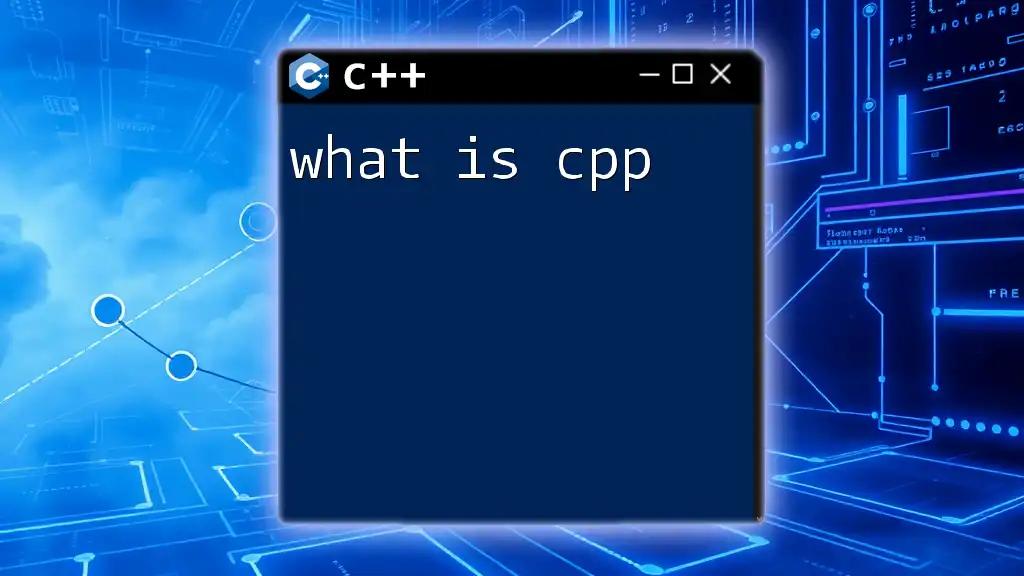
Working with CPP Files
Compiling CPP Files
Compiling CPP files is a crucial step in turning your code into an executable program. The compilation process involves transforming your source code written in a CPP file into machine code.
Command-Line Compilation: Most developers use command-line tools like g++ to compile CPP files. The basic command looks like this:
g++ -o hello hello.cpp
In this command, `g++` is the GNU C++ compiler, `-o hello` specifies the name of the output file, and `hello.cpp` is the CPP file to compile. Once this command runs successfully, it produces an executable file named `hello` that you can run to see your program in action.
IDE Compiling: Many Integrated Development Environments (IDEs) also include built-in compilers, streamlining the process so that developers can compile and run their code with a simple click of a button.
Debugging CPP Files
Debugging is an essential part of programming, particularly when working with CPP files. Common strategies for debugging include using debugging tools that allow you to step through code and inspect variables at runtime.
Effective debugging might involve:
- Print Statements: Adding print statements throughout the code to track variable values and flow.
- Debuggers: Utilizing debugging tools like gdb, integrated within IDEs, can significantly streamline this process by allowing breakpoints and variable inspection.
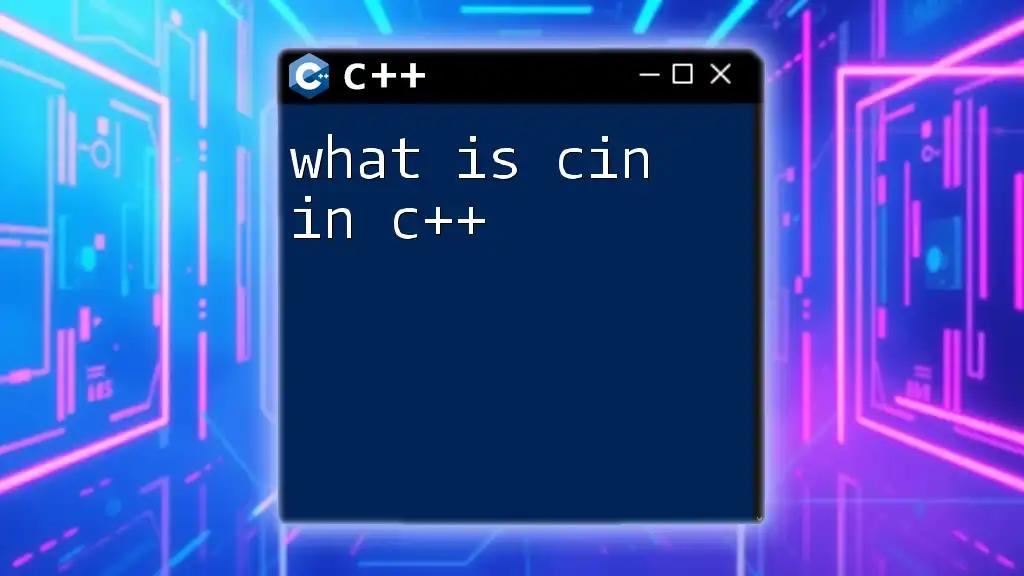
Best Practices for Writing CPP Files
Naming Conventions
Choosing meaningful names for CPP files is crucial for understanding the scope of the code without needing to open the file. Effective naming helps other developers (and your future self) grasp the purpose of a file at a glance. Use clear, descriptive names that reflect the functionality of the code within.
Code Organization
Organizing your code within CPP files contributes to clarity and maintainability. It is best to group related functions and classes together, and separate those that serve different roles. Consistent indentation and spacing improve readability and make it easier to identify the structure of your program.
Consistent Style
Adopting a consistent coding style is essential for teamwork and code reviews. Use linters or formatters to ensure that your code adheres to defined styling guidelines. Maintain consistent spacing, indentation levels, and naming conventions to foster a clean code environment that is easier to read and understand.
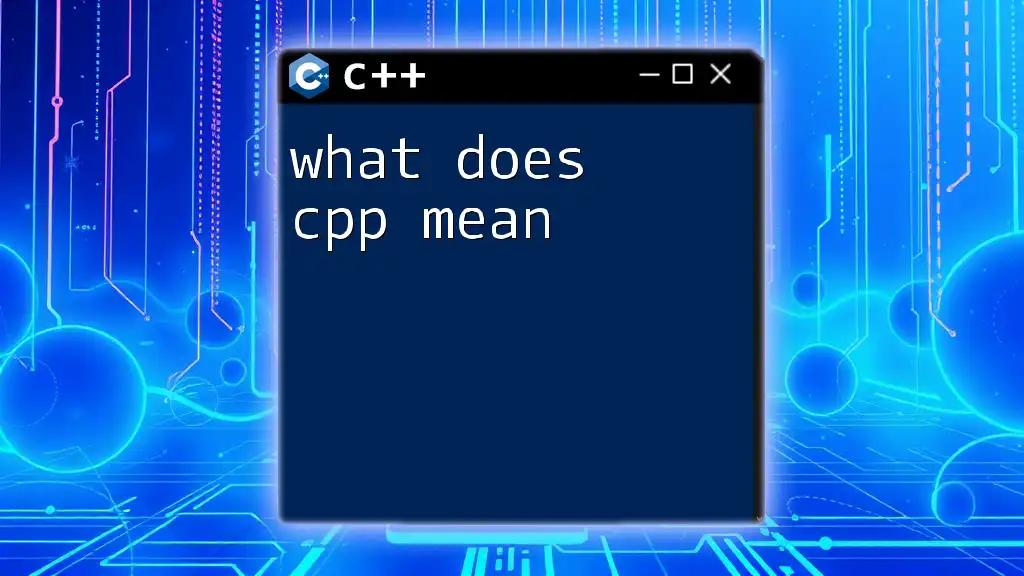
Conclusion
In conclusion, understanding what a CPP file is plays a fundamental role in mastering C++ programming. These text files are the foundation upon which C++ applications are built, and grasping their structure and purpose is vital for any aspiring developer. By practicing the organization, compilation, and debugging of CPP files, programmers can significantly enhance their productivity and the quality of their code. Explore more resources and articles in our blog to further your C++ learning journey!

Additional Resources
Further exploring compilers and IDEs, as well as engaging with community forums and suggested learning materials, can help reinforce your understanding of CPP files and best practices in C++ programming.