C++ (often abbreviated as cpp) is a widely-used programming language known for its performance and flexibility, enabling developers to write efficient and high-level applications.
Here’s a simple example of a C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is CPP 2?
CPP 2 is a significant advancement in the C++ programming language, bringing essential enhancements and features that cater to modern programming needs. It builds upon the foundations laid by previous versions, ensuring compatibility while introducing new paradigms to improve efficiency and clarity in code.
Key Features of CPP 2
The features of CPP 2 are designed to provide developers with enhanced functionality over its predecessors. Here are some notable improvements:
- Enhanced Syntax and Semantics: CPP 2 focuses on readability and reducing boilerplate code, making it easier to implement features.
- Type Concepts: It infuses the language with the ability to define constraints on template parameters, enabling better type-checking and enhancing code safety.
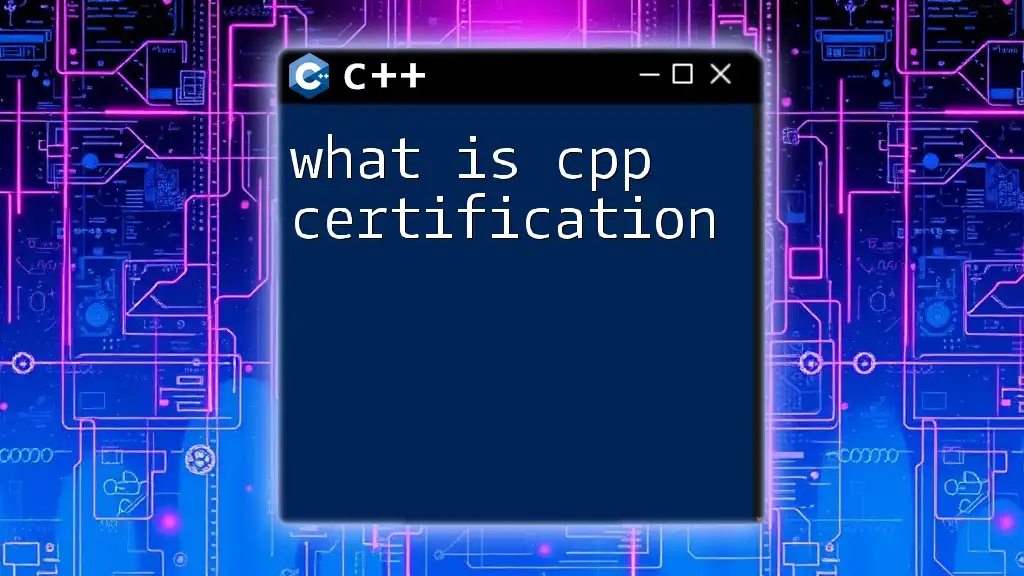
The Evolution of C++
From C to C++
C++ evolved from the C programming language, bringing forth powerful Object-Oriented Programming (OOP) concepts that shape its foundation today. C++ allowed for code reuse, encapsulation, and polymorphism — foundational OOP principles that enhance the code's maintainability.
Key Versions Leading to CPP 2
The journey from C++98 to CPP 2 has seen numerous updates that progressively modernized the language:
- C++98 established the core constructs,
- C++11 introduced features like auto keyword, range-based loops, and smart pointers to aid in memory management and reduce bugs,
- C++14 brought minor enhancements and fixes,
- C++17 introduced std::optional, std::variant, and parallel algorithms, setting the stage for even more profound changes in CPP 2.
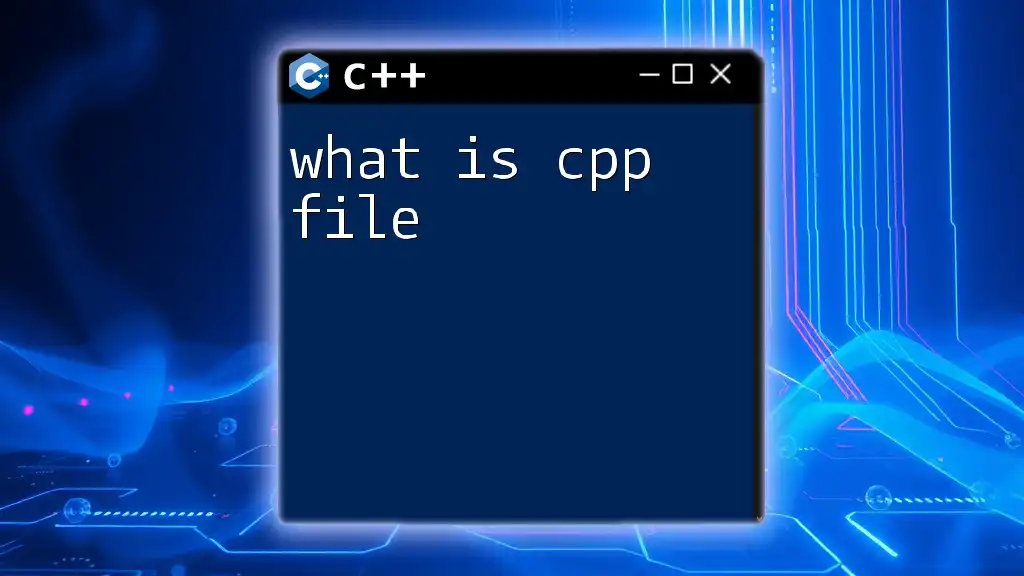
Major Enhancements in CPP 2
Introduction of Concepts
One of the standout features of CPP 2 is Concepts. These define a set of requirements that template parameters must satisfy, enhancing code safety and readability.
For example, consider the following use of Concepts:
template<typename T>
concept Integral = std::is_integral<T>::value;
template<Integral T>
void foo(T t) { /* Implementation here */ }
This snippet defines a concept named `Integral`, allowing developers to impose constraints directly in the type definitions, thus catching errors at compile time rather than at runtime.
Ranges and Algorithms
Another significant feature in CPP 2 is the introduction of the Ranges library. Ranges simplify handling collections by allowing a more readable and declarative style of coding.
Here’s an example illustrating how to use Ranges for transformations:
#include <iostream>
#include <vector>
#include <algorithm>
#include <ranges>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
auto transformed = vec | std::views::transform([](int n) { return n * 2; });
for(auto val : transformed) {
std::cout << val << " ";
}
return 0;
}
In this code, we demonstrate how Ranges combined with views permits elegant transformations without altering the original collection. Such expressive syntax reduces the complexity and improves the readability of the code.
Improved Attributes
Attributes in CPP 2 enhance code optimization and provide greater clarity. They allow developers to communicate intentions directly to the compiler, potentially increasing performance.
For example, using the `[[nodiscard]]` attribute:
[[nodiscard]] int compute_value() { /* Implementation here */ }
This indicates that the return value of `compute_value()` should not be ignored. It acts as a safety feature that encourages developers to handle important output values properly.
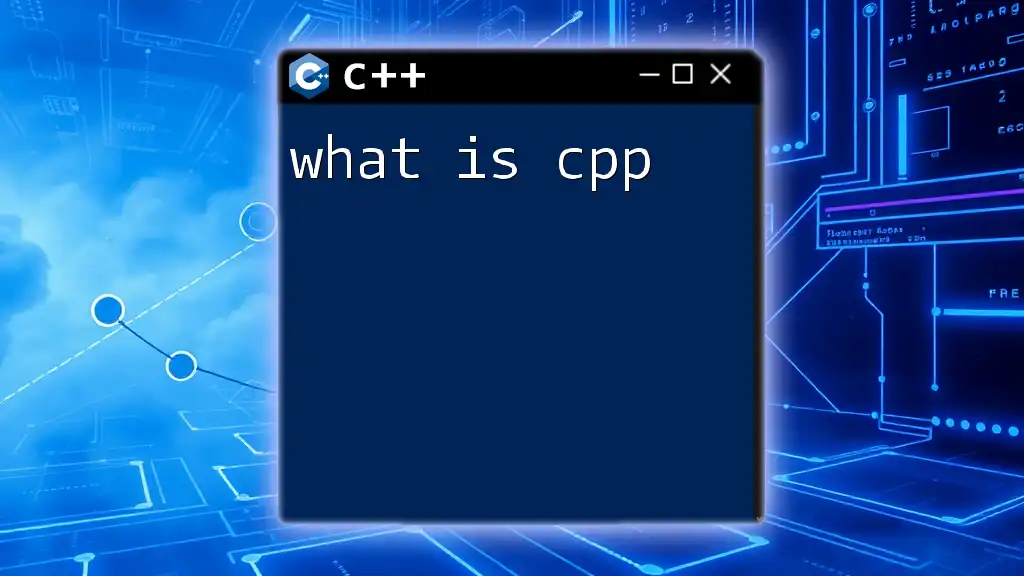
Performance Improvements
Enhanced Compile Times
CPP 2 introduces several optimizations contributing to significantly reduced compile times. Template specialization and better organization of the standard library are just a few changes that enhance compilation efficiency.
This improvement not only fosters a smoother development process but also amplifies productivity by minimizing waiting times during the build process.
Memory Management
CPP 2 also brings innovations in memory management. Enhanced support for smart pointers allows developers to manage memory more effectively and reduce the likelihood of leaks or dangling pointers. Here’s an example of using smart pointers:
#include <memory>
struct Node { /* Implementation here */ };
std::shared_ptr<Node> ptr = std::make_shared<Node>();
This example showcases how smart pointers can improve safety by automating memory management tasks, thus promoting cleaner and more reliable code.
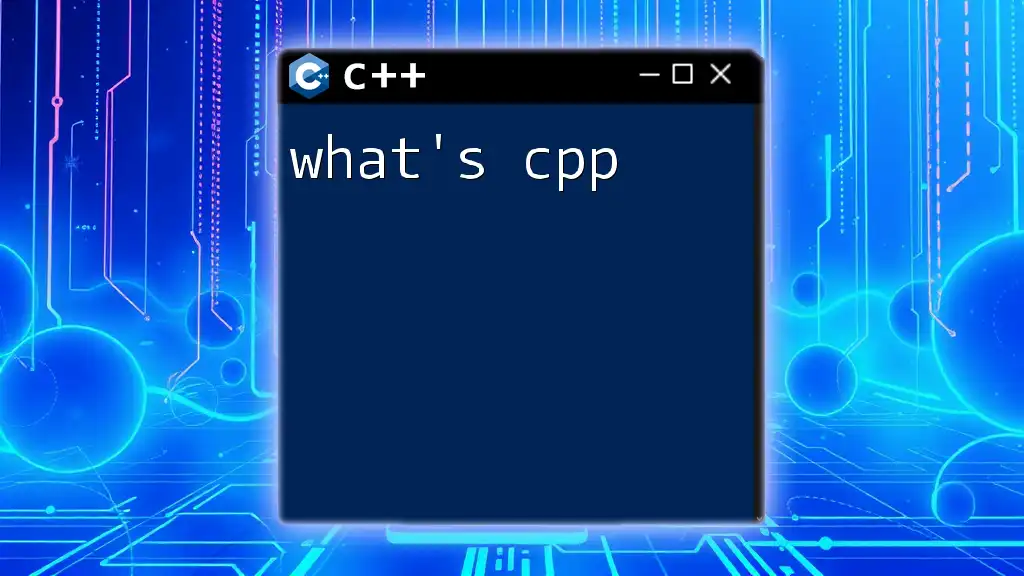
Practical Applications of CPP 2
Use Cases in Software Development
CPP 2 is pivotal in a variety of software development scenarios. Its advanced features are leveraged in industry applications such as:
- Game Development: Offering high-performance and low-level access.
- System Software: Allowing developers to write robust and efficient system-level programs.
- Data Analysis Tools: Facilitating fast and manageable data operations.
These applications benefit immensely from the improvements in efficiency and clarity offered by CPP 2, making it a valuable choice for modern software solutions.
Tutorials and Resources
For those interested in harnessing the power of CPP 2, numerous resources are available. Online courses, comprehensive documentation, and vibrant community forums provide guidance and support. Engaging with these resources can significantly boost your understanding and application of CPP 2.
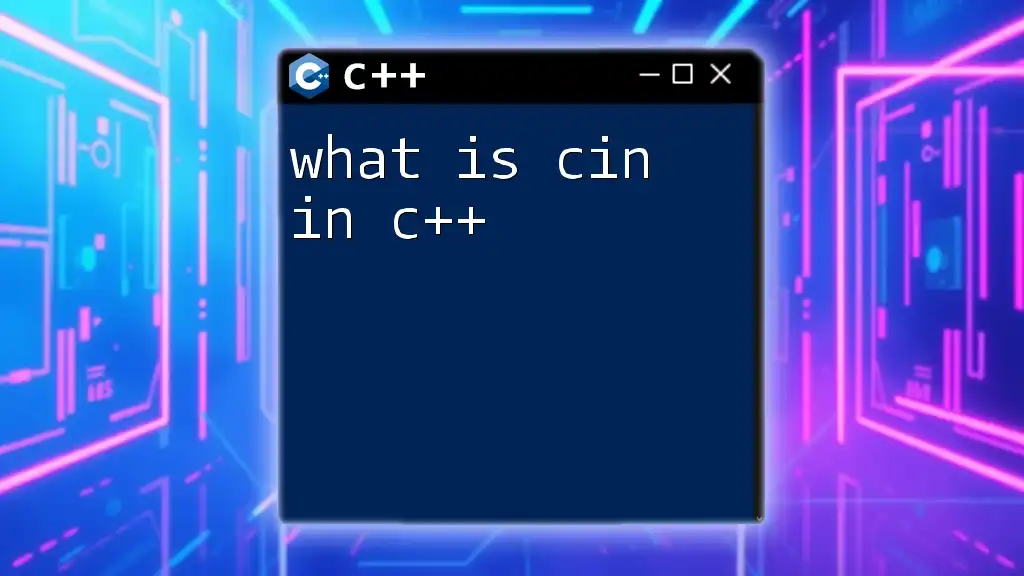
Conclusion
Understanding what is CPP 2 is crucial for modern programmers looking to leverage the strengths of C++. Its advancements deliver enhanced functionality and safety, catering to the evolving needs of the software development landscape. Embracing CPP 2 can optimize coding practices and improve overall project outcomes.
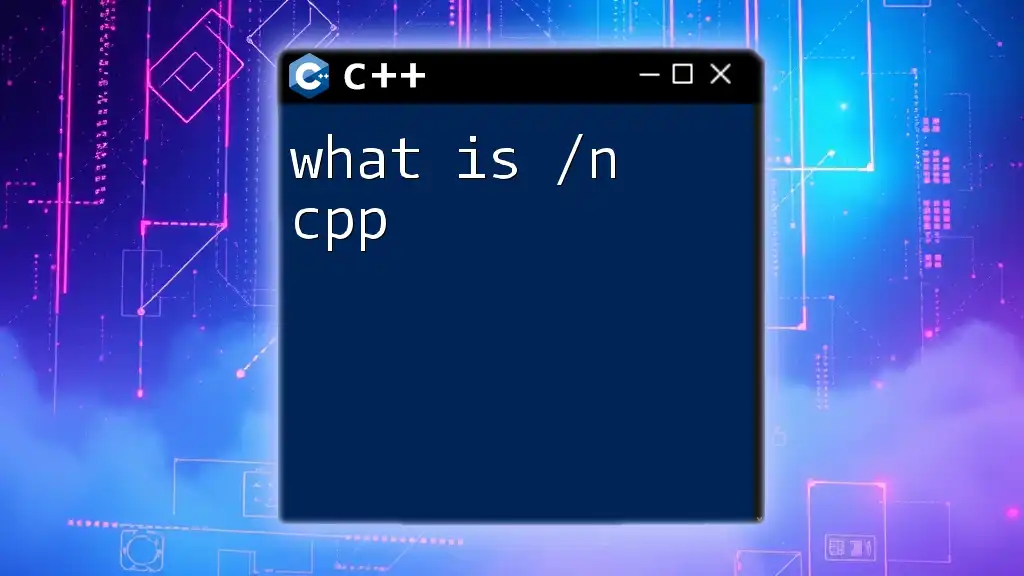
Call To Action
We invite you to share your experiences with CPP 2 or join our training program. As you navigate the intricacies of modern C++, remember that continuous learning is key to mastering these powerful new features. For additional reading, check out the resources available on our website, and embark on your journey to becoming proficient in CPP 2!