A constructor in C++ is a special member function that is automatically called when an object of a class is created, allowing for the initialization of the object's properties.
Here’s a simple code snippet demonstrating a constructor in C++:
#include <iostream>
using namespace std;
class MyClass {
public:
int x;
// Constructor
MyClass(int val) {
x = val;
}
};
int main() {
MyClass obj(10); // Create an object of MyClass
cout << obj.x; // Output: 10
return 0;
}
What is a Constructor in C++?
Definition of a Constructor
A constructor is a special member function in C++ that is automatically invoked when an object of a class is created. Its primary role is to initialize the object, often establishing the initial values of its member variables. Constructors differ from regular member functions in that they share the same name as the class and do not have a return type, not even `void`. They are essential in enforcing good programming practices by ensuring objects are created in a defined state.
Types of Constructors in C++
Default Constructor
A default constructor is one that does not take any arguments. It is invoked when an object is created without any parameters. If no constructors are defined in a class, C++ automatically generates a default constructor, which initializes the object with default values.
class Example {
public:
Example() {
// Default constructor
std::cout << "Default constructor called!" << std::endl;
}
};
int main() {
Example obj; // Calls the default constructor
return 0;
}
In this code, when `Example obj;` is executed, the console will display "Default constructor called!" indicating that the default constructor was successfully invoked.
Parameterized Constructor
A parameterized constructor allows you to provide initial values for the object at the time of its creation. This flexibility enables you to customize the state of different objects from the beginning.
class Example {
public:
int value;
Example(int v) { // Parameterized constructor
value = v;
std::cout << "Parameterized constructor called with value: " << value << std::endl;
}
};
int main() {
Example obj(10); // Calls the parameterized constructor with 10
return 0;
}
In this example, the output will be "Parameterized constructor called with value: 10" since we've initialized `value` with the specified argument.
Copy Constructor
A copy constructor is used to create a new object by copying an existing object. In cases where the class contains pointers or dynamically allocated resources, the copy constructor is critical for resource management.
class Example {
public:
int* ptr;
Example(int v) {
ptr = new int(v); // Dynamically allocate memory
}
// Copy constructor
Example(const Example &obj) {
ptr = new int(*obj.ptr); // Deep copy to prevent shallow copy issues
}
~Example() {
delete ptr; // Destructor to free memory
}
};
int main() {
Example obj1(10); // Calls parameterized constructor
Example obj2 = obj1; // Calls copy constructor
std::cout << "Object 2 value: " << *obj2.ptr << std::endl;
return 0;
}
In this case, when `obj2` is created as a copy of `obj1`, the copy constructor is invoked. This deep copies the value of `ptr`, ensuring that each object manages its own memory safely.
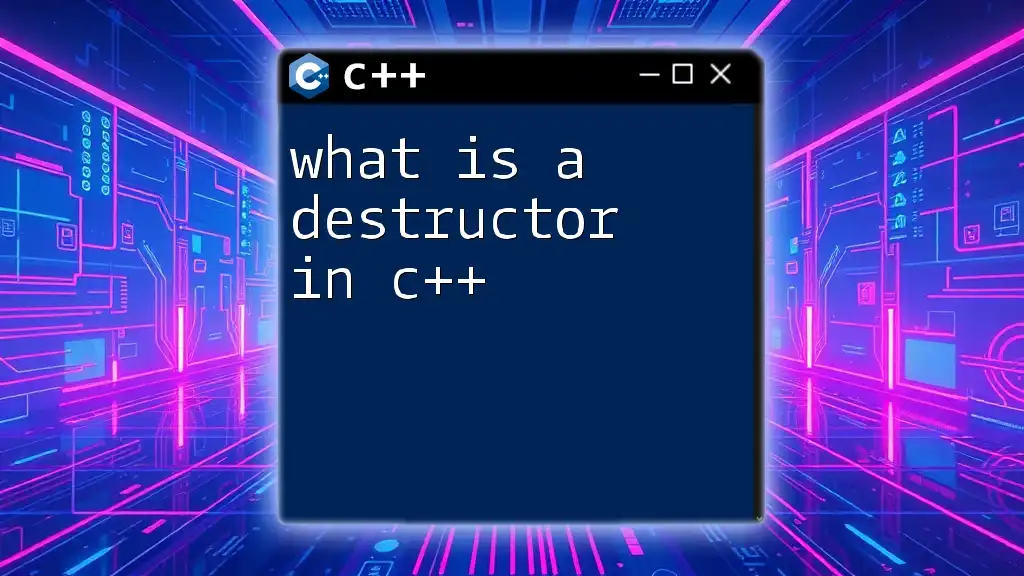
How to Make a Constructor in C++
Syntax of a Constructor
The basic syntax of a constructor in C++ is simple. It shares the name with its class and does not include a return type.
class ClassName {
public:
ClassName(parameters) {
// Constructor body
}
};
This structure is straightforward, allowing you to define different constructors based on the parameters you wish to accept.
Creating a Simple Class with a Constructor
Let’s walk through defining a class with various types of constructors.
class Person {
public:
std::string name;
int age;
// Default constructor
Person() {
name = "Unknown";
age = 0;
}
// Parameterized constructor
Person(std::string n, int a) {
name = n;
age = a;
}
void display() {
std::cout << "Name: " << name << ", Age: " << age << std::endl;
}
};
int main() {
Person person1; // Calls default constructor
Person person2("Alice", 30); // Calls parameterized constructor
person1.display(); // Name: Unknown, Age: 0
person2.display(); // Name: Alice, Age: 30
return 0;
}
In this code, `person1` uses the default constructor, initializes the `name` to "Unknown," and `age` to 0. On the other hand, `person2` utilizes the parameterized constructor to initialize its values.
Constructor Overloading in C++
Constructor overloading allows you to define multiple constructors with different parameters within the same class. This capability offers versatility in object construction.
class Rectangle {
public:
int length;
int width;
// Default constructor
Rectangle() {
length = 0;
width = 0;
}
// Parameterized constructor
Rectangle(int l, int w) {
length = l;
width = w;
}
};
int main() {
Rectangle rect1; // Calls default constructor
Rectangle rect2(10, 20); // Calls parameterized constructor
std::cout << "Rectangle 1: " << rect1.length << " x " << rect1.width << std::endl;
std::cout << "Rectangle 2: " << rect2.length << " x " << rect2.width << std::endl;
return 0;
}
Here, the program creates `rect1` with the default values and `rect2` with specified dimensions, showcasing the benefit of employing multiple constructors.
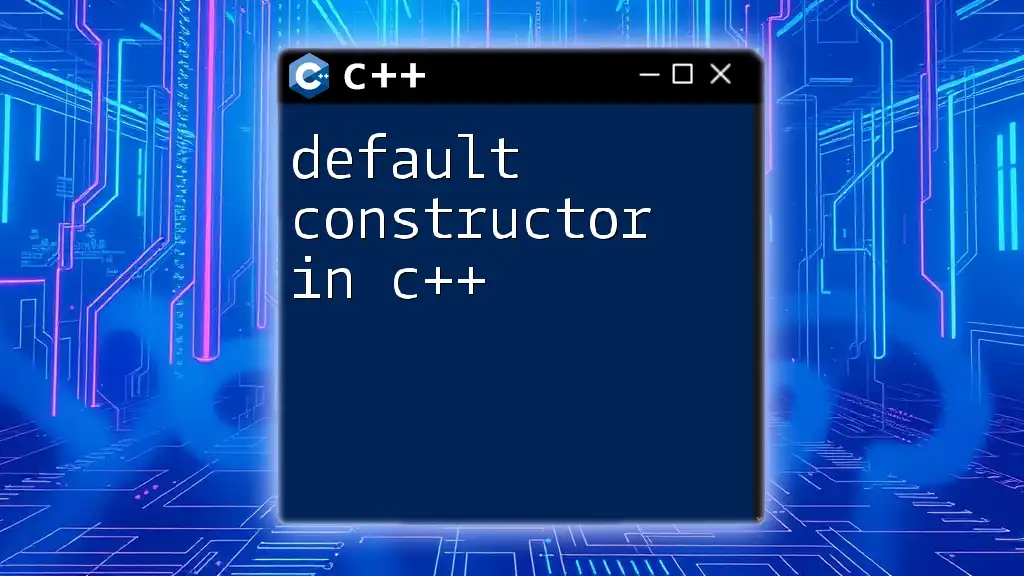
Best Practices for Using Constructors in C++
Avoiding Common Pitfalls
When working with constructors, avoid common mistakes such as:
- Initialization Issues: Ensure that you initialize all member variables to prevent garbage values. A failure to do so could lead to unpredictable behavior.
- Forgetting to Initialize Member Variables: Always initialize your member variables, even in the copy constructor.
- Circular Dependencies: Be cautious of circular dependencies when using copy constructors, especially with pointers.
When to Use Constructors
Constructors should be utilized whenever you create a new object. They establish initial states, manage resources, and can prevent the possibility of uninitialized memory in your program. Whenever you have class data that needs to be set, consider using a constructor to handle those assignments efficiently.
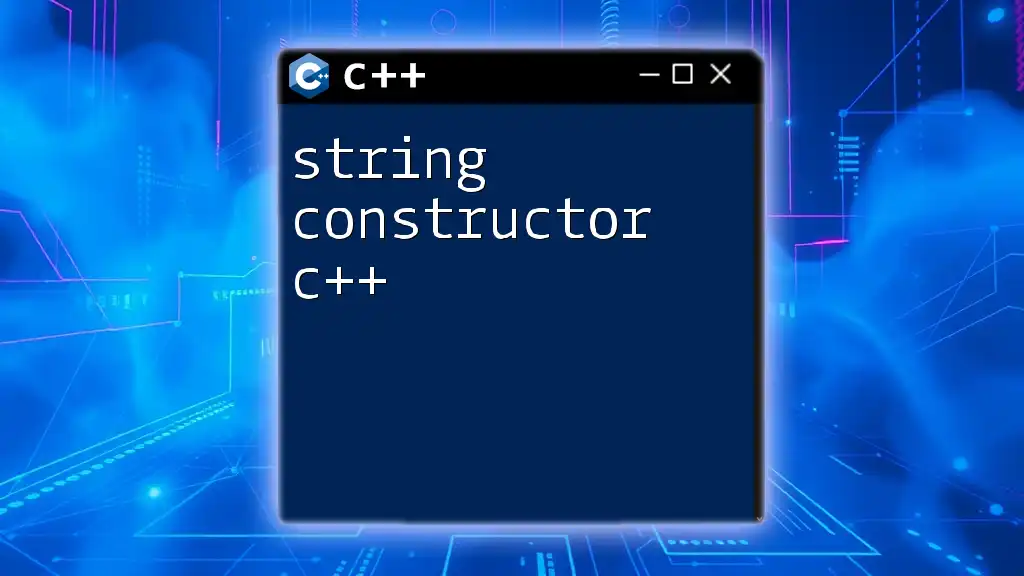
Conclusion
In summary, understanding what a constructor is in C++ is fundamental for anyone delving into object-oriented programming in this language. Constructors set the stage for how your objects behave and interact. By harnessing the power of default, parameterized, and copy constructors, you gain fine control over your objects' lifecycles. Experiment with these concepts and solidify your programming foundation by exploring more C++ topics in depth.
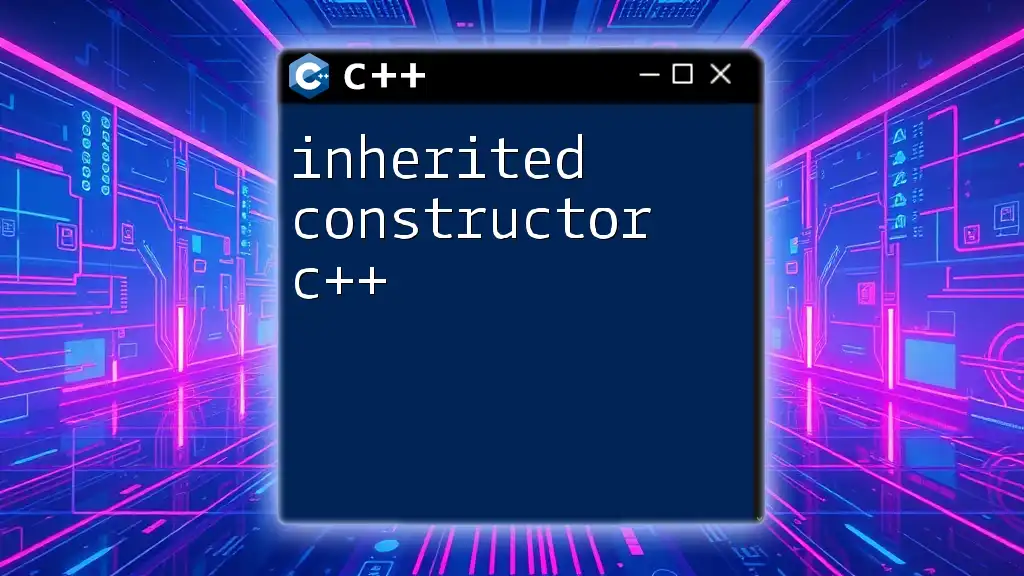
FAQs about C++ Constructors
What is the difference between a constructor and a method in C++?
- A constructor initializes an object upon creation and has the same name as the class, while a method is a regular function that performs an action on the object and can be called at any time after the object is created.
Can we create a constructor with no parameters?
- Yes, creating a constructor with no parameters is known as a default constructor. It is often used to initialize members with default values.
What happens if you don’t create a constructor in C++?
- If no constructors are defined, the compiler generates a default constructor automatically, which initializes member variables with default values.
Why is the copy constructor important in C++?
- The copy constructor is crucial for avoiding shallow copies, which can lead to issues like double-free errors in dynamic memory management. A proper copy constructor ensures that resources are appropriately copied, maintaining the integrity of both the original and the copied object.
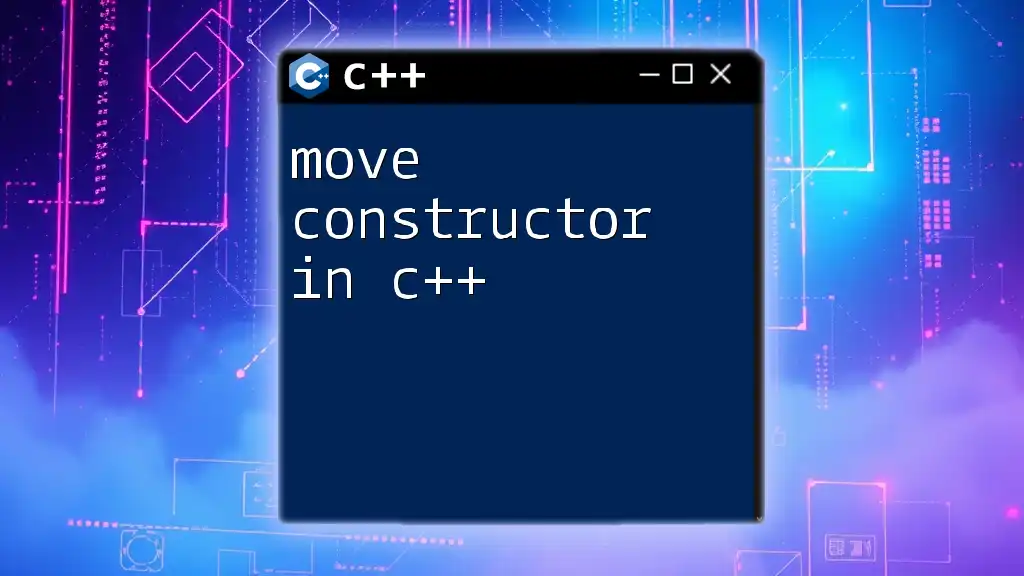
Additional Resources
For further reading and to enhance your understanding of C++ and object-oriented programming, consider exploring additional tutorials, documentation, and practice problems related to constructors in C++. These resources can help solidify your knowledge and make you a more proficient C++ programmer.