An inline constructor in C++ allows you to define the constructor's implementation directly within the class definition, promoting ease of use and potentially improving performance.
Here's a code snippet demonstrating an inline constructor:
class MyClass {
public:
MyClass(int x) : value(x) {} // Inline constructor
int getValue() const { return value; }
private:
int value;
};
Understanding Constructors in C++
What is a Constructor?
In C++, a constructor is a special member function of a class that is automatically called when an object of the class is created. Constructors serve the purpose of initializing an object's attributes and allocating resources necessary for the object's functioning.
There are mainly three types of constructors:
- Default Constructor: Takes no parameters and initializes member variables to default values.
- Parameterized Constructor: Accepts parameters to set member variables differently during instantiation.
- Copy Constructor: Initializes an object using another object of the same class.
The significance of constructors in object-oriented programming cannot be understated—they set the groundwork for creating objects with defined properties and behaviors.
What is an Inline Function?
An inline function is a function for which the compiler attempts to generate the code directly into the caller's context, replacing the function call with the actual code. This can lead to performance enhancements, particularly in small functions where the overhead of a function call would outweigh the benefits of abstraction.
Benefits of inline functions include:
- Performance Improvement: Reduced function call overhead.
- Increased Execution Speed: Particularly significant during high-frequency calls.
However, it is crucial to be aware of the differences between inline functions and standard functions. Inlining is a request to the compiler, not a command; the compiler can choose to ignore the inline request based on its own heuristics.
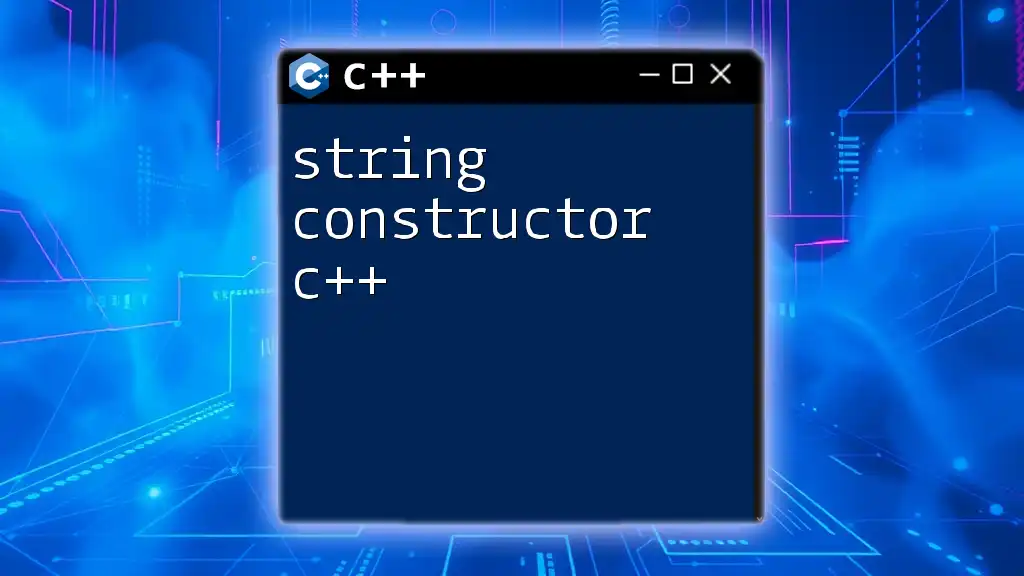
Inline Constructors in C++
Definition of Inline Constructors
An inline constructor is simply a constructor defined within the class definition itself, allowing for immediate initialization of class members when objects are created.
Syntax of an inline constructor typically looks like this:
class MyClass {
public:
MyClass() { /* constructor code */ }
};
Why Use Inline Constructors?
The primary advantages of using inline constructors rest on performance and code conciseness. Inline constructors can result in faster instantiation of objects since there is no overhead associated with function calls—this is particularly beneficial in scenarios involving multiple object creations.
Common misconceptions about inline constructors include beliefs that they always lead to better performance. While they may improve speed, it's essential to ensure that they do not compromise code readability or maintainability.
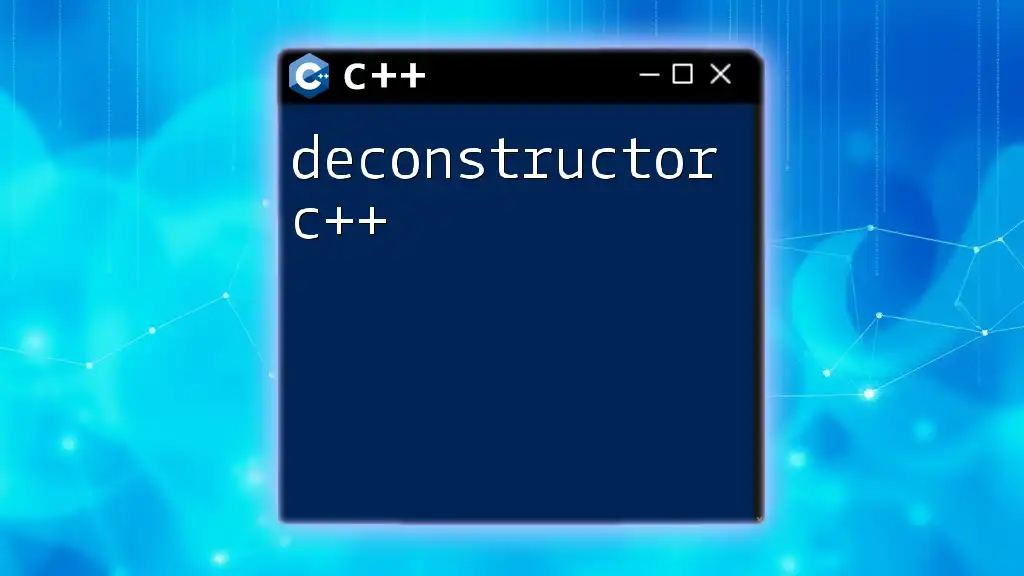
Defining Inline Constructors
Syntax of Inline Constructors
The general syntax for defining an inline constructor includes placing the constructor's code within the class definition. Here’s a straightforward example:
class MyClass {
public:
MyClass() { /* constructor code */ }
};
Inline Constructor with Parameters
Parameterized inline constructors allow for dynamic object initialization with customizable values. Here’s an example:
class MyClass {
public:
MyClass(int x) { /* constructor code with parameter x */ }
};
By utilizing this feature, you can create objects with specific configurations directly upon instantiation, leading to cleaner and more efficient object creation processes.
Inline Constructor with Initializer List
Initializer lists provide an elegant way to initialize member variables directly. They’re particularly useful in situations where you want to pass parameters that need to be assigned during construction. Here’s an example showcasing an inline constructor using an initializer list:
class MyClass {
private:
int value;
public:
MyClass(int x) : value(x) { /* constructor code */ }
};
In this case, the member variable `value` is directly assigned the parameter `x`, enhancing performance, particularly for complex data types where default constructors might otherwise be invoked.
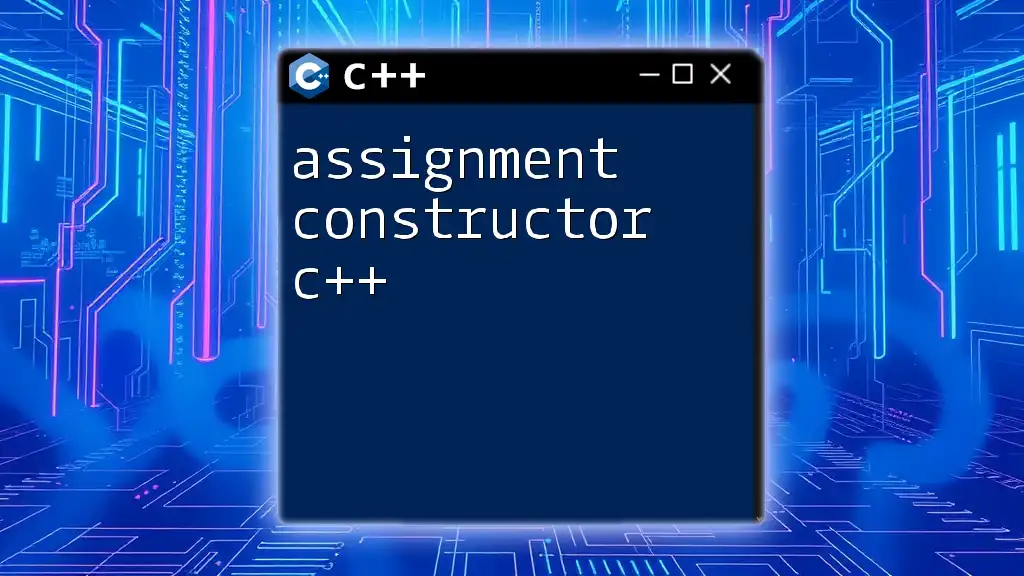
Best Practices for Using Inline Constructors
When to Use Inline Constructors?
When considering the implementation of inline constructors, keep in mind a few guidelines:
- Favor Small Classes: Inline constructors shine in small, lightweight classes where the overhead of function calls can significantly detract from performance.
- Encapsulation: Ensure that the inline constructor encapsulates the class’s state and initiates essential members without exposing too much internal logic.
Common Mistakes to Avoid
While inline constructors can simplify object construction, it's important to watch out for potential pitfalls:
- Overcomplication of Code: Overusing inline constructors for large classes can lead to bloated class definitions that are hard to read and maintain.
- Performance Misjudgment: Not all constructors benefit from inlining—as your objects grow in complexity or size, the compiler may indeed choose to split them into separate calls impacting performance.
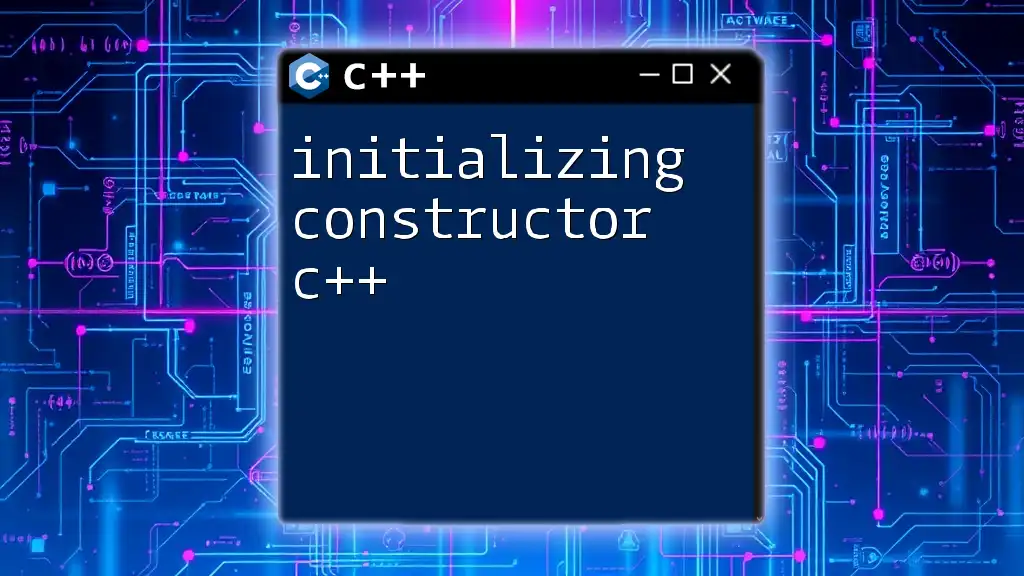
Examples of Inline Constructors in Use
Example 1: Basic Usage
Let’s take a look at a simple class that utilizes an inline constructor effectively:
#include <iostream>
class Point {
private:
int x, y;
public:
Point(int x, int y) : x(x), y(y) {}
void display() { std::cout << "(" << x << ", " << y << ")"; }
};
int main() {
Point p(10, 20);
p.display(); // Output: (10, 20)
return 0;
}
In this illustration, the `Point` class utilizes a parameterized inline constructor that initializes x and y upon creating an object.
Example 2: Complex Object Initialization
Here’s a more intricate example involving a class that holds a student’s information:
#include <iostream>
#include <string>
class Student {
private:
std::string name;
int age;
public:
Student(const std::string &n, int a) : name(n), age(a) {}
void display() {
std::cout << "Name: " << name << ", Age: " << age << std::endl;
}
};
int main() {
Student s("Alice", 20);
s.display(); // Output: Name: Alice, Age: 20
return 0;
}
The `Student` class demonstrates a parameterized inline constructor that initializes two key attributes—name and age—efficiently.
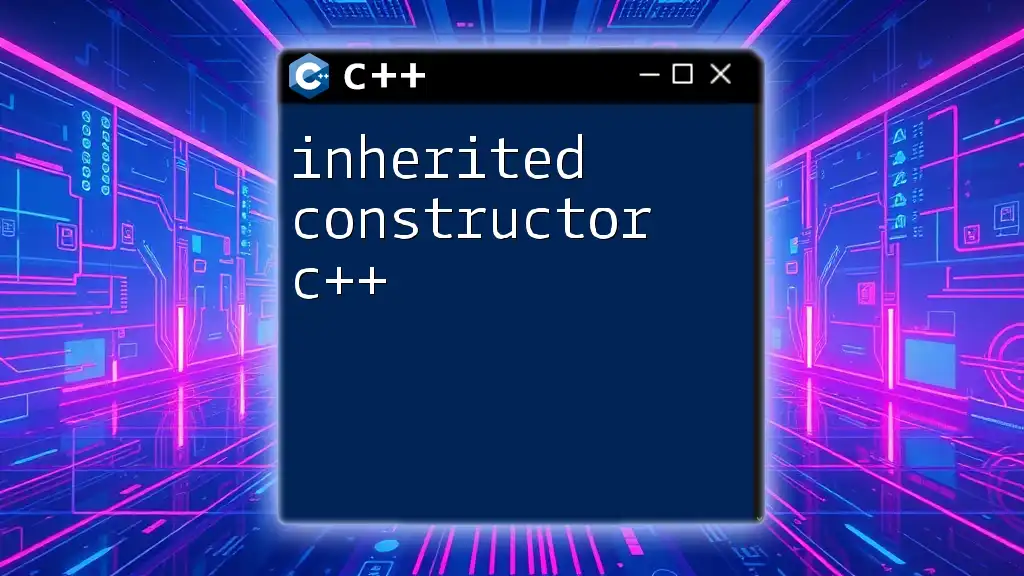
Performance Considerations
Impact of Inline Constructors on Performance
The decision to use inline constructors can significantly impact performance, especially in scenarios with numerous object instantiations. By eliminating the function-call overhead, inline constructors promote faster execution, making them highly beneficial in performance-intensive applications.
When Inline Constructors Might Not Be Ideal
However, there are scenarios where inline constructors might not be the best choice:
- Complexity and Size: As classes grow in complexity, inlining can lead to increased binary size if overused.
- Linking Issues: Excessive inlining can lead to complicated linking operations in larger projects, potentially resulting in maintenance challenges.
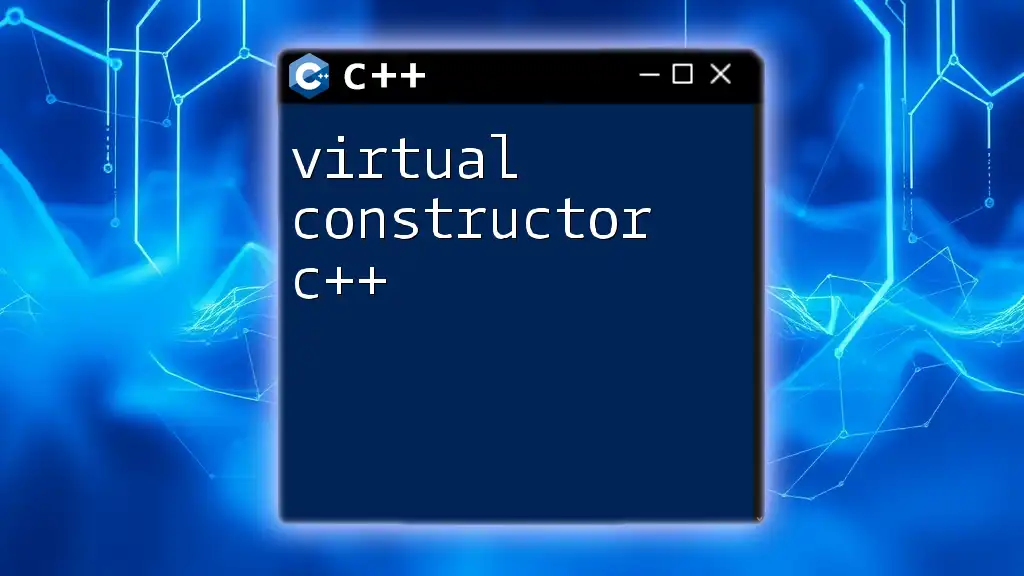
Conclusion
Inline constructors in C++ offer a powerful tool for developers aiming to enhance performance and simplify object creation in their applications. Understanding when and how to implement inline constructors effectively can lead to more efficient C++ code.
As you explore the vast landscape of C++, consider integrating inline constructors into your regular coding practices, and watch your object-oriented programming approach flourish. For further exploration, seek out additional resources and course materials that can deepen your understanding of C++ programming best practices.