A destructor in C++ is a special member function that is automatically called when an object goes out of scope or is deleted, used to release resources allocated to the object.
class Example {
public:
Example() {
// Constructor
}
~Example() {
// Destructor
}
};
Understanding Destructors in C++
What is a Destructor?
A destructor is a special member function in a class that is called automatically when an object of that class goes out of scope or is explicitly deleted. The main purpose of a destructor is to free resources that the object may have acquired during its lifetime, ensuring that there are no memory leaks. Destructors play a crucial role in resource management, as they allow programmers to clean up after their objects.
Why Use Destructors?
Using destructors effectively is vital for several reasons:
- Memory Management: Dynamically allocated memory must be deallocated to avoid memory leaks. Destructors handle this task automatically.
- Resource Cleanup: When an object manages resources such as file handles, database connections, or network sockets, a destructor ensures that these resources are properly released.
- Preventing Memory Leaks: Failing to release memory can lead to memory leaks, which can severely impact performance and stability.
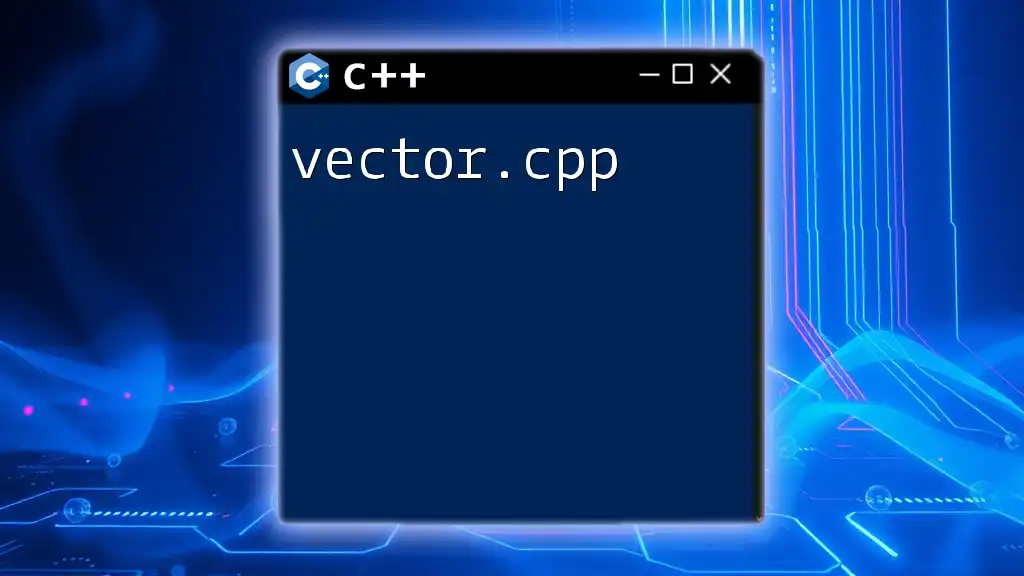
The Syntax of C++ Destructors
Basic Syntax and Structure
A destructor in C++ is declared using a tilde `~` followed by the class name. It does not have a return type and does not take parameters.
Here’s a simple example of a destructor declaration:
class Example {
public:
~Example() {
// Destructor code
}
};
In this case, `~Example()` is the destructor for the class `Example`. It will be invoked automatically when an object of this class is destroyed.
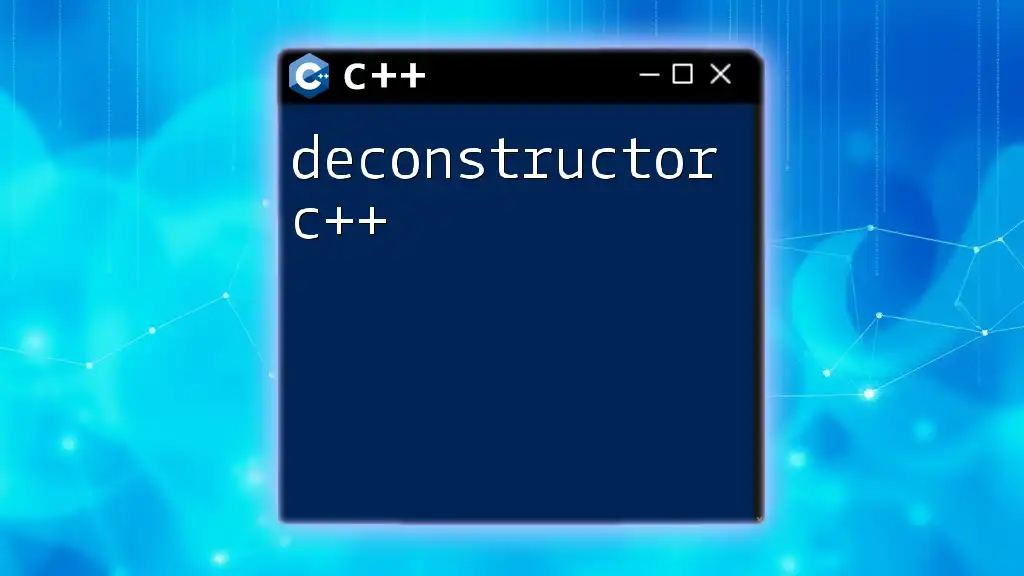
Types of Destructors
Default Destructor
The default destructor is automatically provided by the C++ compiler if no destructors are defined in a class. It performs member-wise deletion of member objects and is used when all members can be automatically cleaned.
User-Defined Destructor
Developers can create custom destructors to handle specific cleanup operations. When a user-defined destructor is provided, it takes precedence over the default destructor.
Here's how to create a user-defined destructor:
class Resource {
public:
Resource() {
// Allocation of resource
}
~Resource() {
// Release the resource
}
};
In this example, the user-defined destructor performs cleanup when an object of the class `Resource` is destroyed.
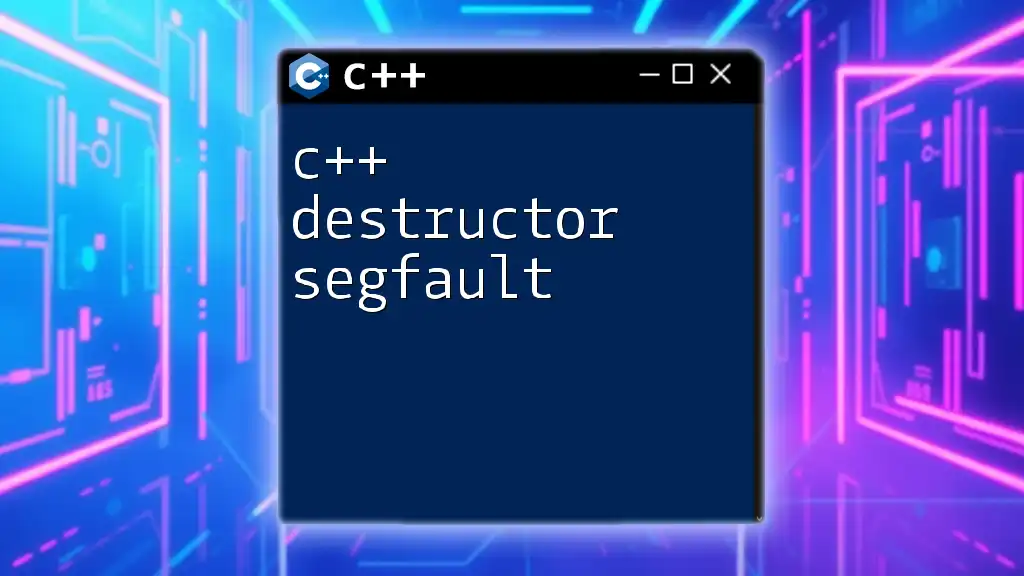
Working with Destructors in C++
Destructor in Class C++
Destructors can be seamlessly integrated into class definitions. When an object instantiated from a class is no longer needed, the destructor is called to perform cleanup.
Here's a basic example of a class with a destructor:
class MyClass {
public:
MyClass() {
// Constructor logic
std::cout << "Object created" << std::endl;
}
~MyClass() {
// Destructor logic
std::cout << "Object destroyed" << std::endl;
}
};
In this example, the output "Object created" appears when an instance of `MyClass` is created, and "Object destroyed" appears when the instance goes out of scope.
C++ Destructor Example
Let's expand the previous example to illustrate the destructor's role in resource management:
class FileHandler {
private:
FILE* filePtr;
public:
FileHandler(const char* filename) {
filePtr = fopen(filename, "r");
if (!filePtr) {
std::cerr << "File could not be opened" << std::endl;
}
}
~FileHandler() {
if (filePtr) {
fclose(filePtr);
std::cout << "File closed successfully" << std::endl;
}
}
};
In this `FileHandler` class, the destructor is responsible for closing the file when the `FileHandler` object is destroyed, preventing resource leaks.
Handling Dynamically Allocated Memory
When objects allocate memory dynamically, destructors must handle the deallocation to prevent memory leaks. Here's an example:
class DynamicArray {
private:
int* arr;
int size;
public:
DynamicArray(int s) {
size = s;
arr = new int[size]; // Dynamic allocation
}
~DynamicArray() {
delete[] arr; // Free the allocated memory
}
};
In this `DynamicArray` class, the destructor ensures that the memory allocated for the array is freed when the object is destroyed.
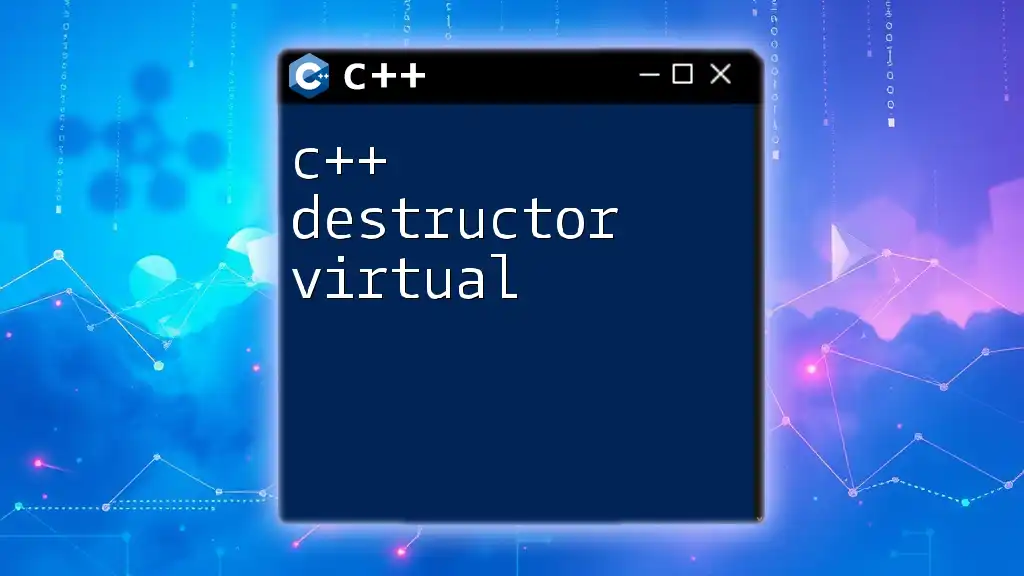
Common Misunderstandings About Destructors
Destructor vs. Constructor
A constructor initializes an object when it is created, while a destructor cleans up when the object is destroyed. Constructors are called explicitly upon the creation of an object, whereas destructors are called implicitly when an object goes out of scope or is deleted.
Virtual Destructors
In cases of inheritance and polymorphism, using virtual destructors is fundamental. When a base class pointer points to a derived class object, the destructor is crucial for ensuring the derived class's destructor is called correctly.
Here’s an example illustrating virtual destructors:
class Base {
public:
virtual ~Base() {
std::cout << "Base Destructor" << std::endl;
}
};
class Derived : public Base {
public:
~Derived() override {
std::cout << "Derived Destructor" << std::endl;
}
};
In this example, if a `Derived` object is deleted through a `Base` pointer, both the `Derived` and `Base` destructors will be invoked, ensuring proper cleanup.
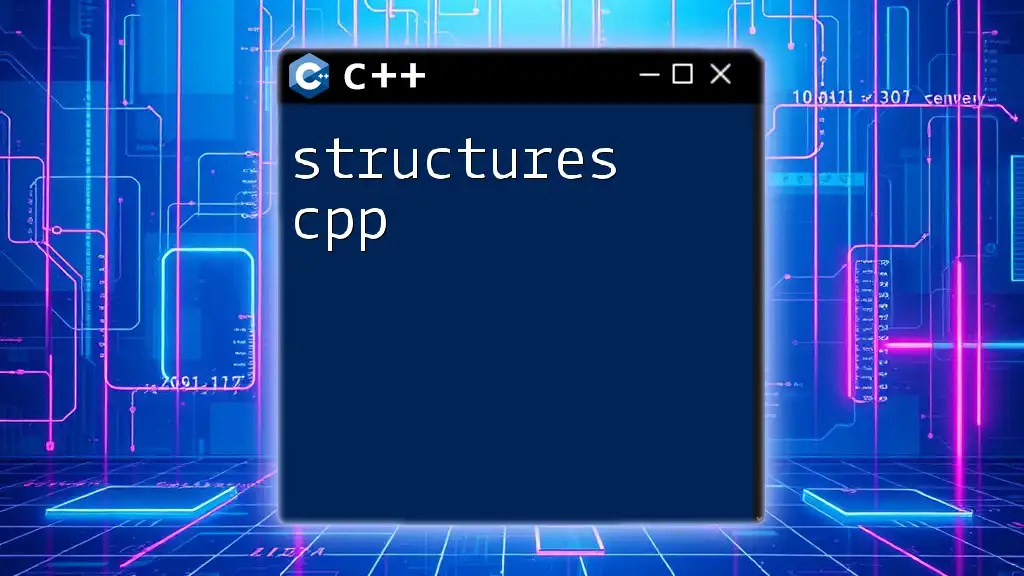
Best Practices for Using Destructors
Avoiding Memory Leaks
To avoid memory leaks:
- Ensure every `new` has a corresponding `delete`.
- Use smart pointers (`std::unique_ptr` and `std::shared_ptr`) when possible to manage resource ownership automatically.
Here’s an example using a smart pointer:
#include <memory>
class SmartArray {
private:
std::unique_ptr<int[]> arr;
public:
SmartArray(int size) : arr(new int[size]) {}
// No explicit destructor needed as unique_ptr handles it
};
Destructor Class C++
When designing class hierarchies, it’s essential to implement appropriates destructors for both base and derived classes to prevent resource leaks and undefined behavior in object destruction.
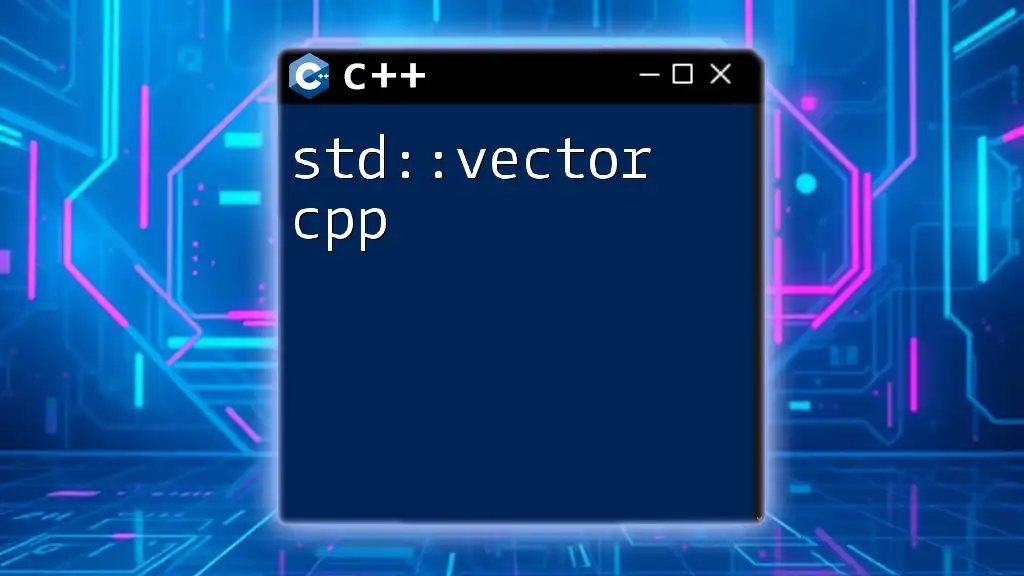
Debugging Destructors
Common Errors and How to Resolve Them
Common errors associated with destructors include:
- Dangling pointers: Accessing an object after it has been destroyed can cause undefined behavior.
- Double deletion: Attempting to delete the same pointer twice leads to crashes.
Carefully managing pointers and using smart pointers greatly reduces these issues.
Tools for Debugging C++ Destructors
Utilizing tools such as Valgrind can help detect memory leaks and improper memory management. These tools provide comprehensive reporting on memory usage, allowing developers to identify and fix destructor-related problems efficiently.
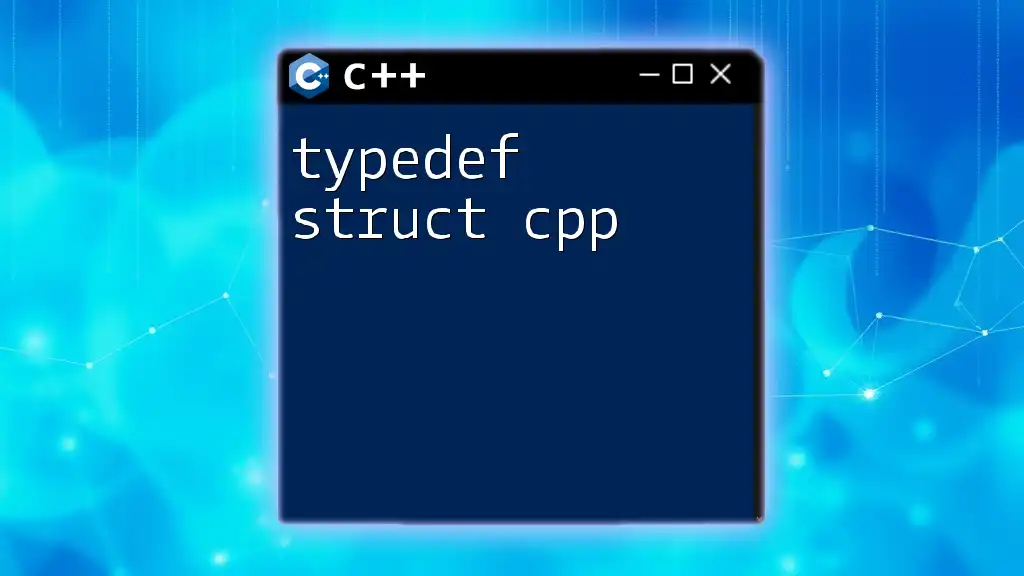
Conclusion
In summary, destructors are an essential feature in C++ programming for resource management, helping to maintain stability and performance by preventing memory leaks. Understanding the differences between default, user-defined, and virtual destructors, as well as following best practices, is crucial for effective C++ programming.
Embrace the concepts discussed and practice implementing destructors in your applications to ensure you’re managing resources responsibly.