The `vector.cpp` file typically contains functions and operations related to the C++ Standard Library's `std::vector`, which is a dynamic array that allows for automatic resizing and efficient access to its elements.
Here's an example of how to declare and use a vector in C++:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5}; // Declare and initialize a vector
for (int number : numbers) {
std::cout << number << " "; // Output each element in the vector
}
return 0;
}
What is a Vector in C++?
In C++, a vector is a dynamic array that can grow or shrink in size, providing the flexibility to store a collection of elements without needing to define its size ahead of time. This feature makes vectors extremely useful, especially when dealing with data where the number of elements is not known in advance or can change during program execution.
The `vector` Library in C++
The vector library in C++ is part of the Standard Template Library (STL). It abstracts array management and offers numerous functionalities to make working with arrays easier. Utilizing the `vector` library not only saves time but also enhances code readability, maintainability, and performance.
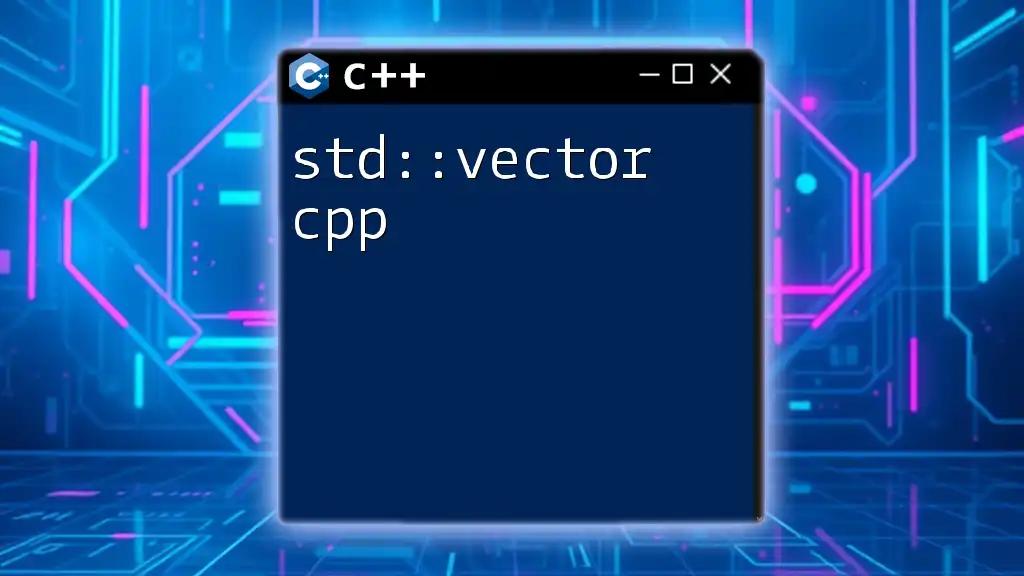
Basics of C++ Vector Syntax
C++ Vector Declaration
Declaring a vector in C++ is simple. You define a vector using the `std::vector` template with the type of elements you intend to store. The basic syntax for declaring a vector is:
std::vector<int> numbers;
In this example, we declare a vector named `numbers` that can store integers.
Initializing a Vector
Vectors can be initialized upon declaration, allowing you to specify the initial elements. The syntax for initializing a vector with predefined values is as follows:
std::vector<int> nums = {1, 2, 3, 4, 5};
This creates a vector `nums` containing the integers 1 through 5. You can also initialize it with a specific number of default-initialized elements:
std::vector<int> defaultVector(10); // Creates a vector of size 10 with default values (0)
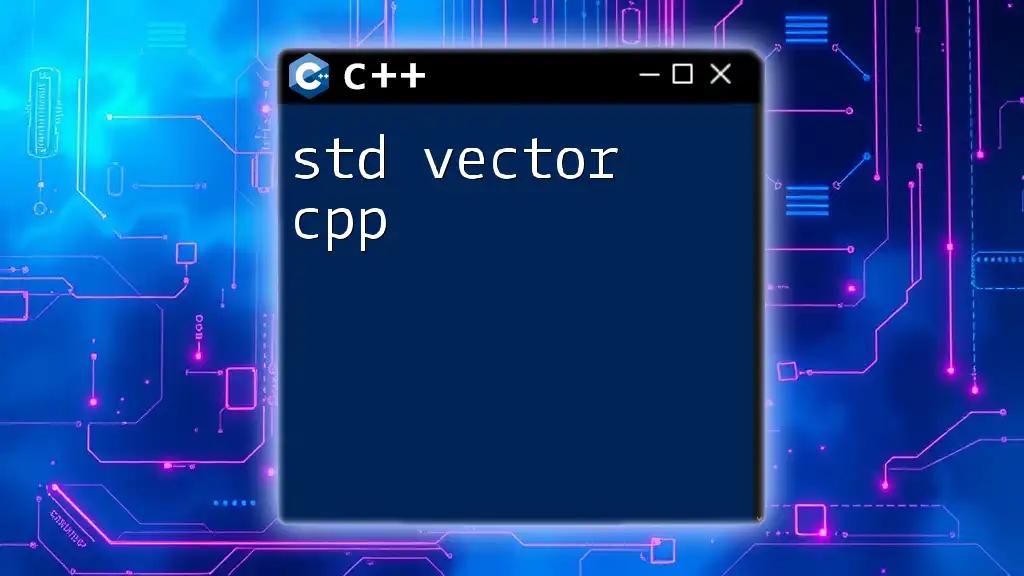
Key C++ Vector Methods
Overview of C++ Vector Methods
C++ vectors come equipped with various methods that allow you to manipulate the vector easily. Understanding these methods is crucial for effective programming.
Common Methods in C++ Vectors
- `push_back()`
The `push_back()` method allows you to add a new element to the end of a vector. This operation efficiently increases the vector's size. For example:
std::vector<int> myVector;
myVector.push_back(10);
myVector.push_back(20);
- `pop_back()`
Conversely, `pop_back()` removes the last element from the vector, effectively decreasing its size. Here’s how it works:
myVector.pop_back(); // Removes 20, leaving myVector with just 10
- `size()`
The `size()` method returns the current number of elements in the vector:
size_t vectorSize = myVector.size(); // Returns 1
- `empty()`
To check if a vector is empty, use the `empty()` method. This can be useful in various conditions:
if (myVector.empty()) {
std::cout << "Vector is empty!" << std::endl;
}
Manipulating Vector Elements with Functions
Accessing vector elements can be done using the following methods:
- `at()` method: Safer access that checks bounds.
int value = myVector.at(0); // Returns the first element
- `operator[]`: More straightforward but does not check bounds.
int value = myVector[0]; // Returns the first element
Iterating through a vector can be accomplished using a loop, like so:
for (size_t i = 0; i < myVector.size(); ++i) {
std::cout << myVector[i] << " ";
}
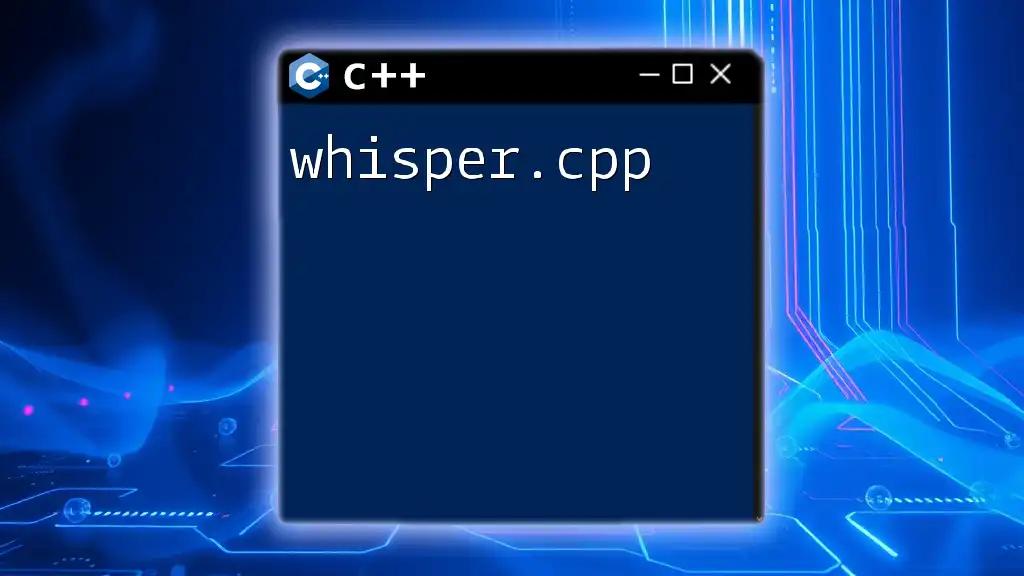
Advanced C++ Vector Functions
Sorting Vectors
One powerful functionality of vectors is sorting. The `std::sort()` function from the `<algorithm>` header can be used effectively on vectors:
#include <algorithm>
std::vector<int> myVector = {4, 2, 3, 1};
std::sort(myVector.begin(), myVector.end()); // Vector now sorted to {1, 2, 3, 4}
Searching in Vectors
You can search for elements within a vector using the `std::find()` function:
#include <algorithm>
auto it = std::find(myVector.begin(), myVector.end(), 3);
if (it != myVector.end()) {
std::cout << "Found 3!" << std::endl;
}
Vector Resizing and Capacity Management
- `resize()`: This method changes the size of the vector to the specified count. If the new size is larger, new elements are initialized.
myVector.resize(5); // Vector size becomes 5 (new elements initialized to 0)
- `reserve()`: This method lets you manage memory beforehand, increasing the vector's capacity without changing the size of the vector.
myVector.reserve(100); // Reserves space for 100 elements
Clearing Vectors
To remove all elements from a vector and free the memory used, you can use the `clear()` function:
myVector.clear(); // myVector is now empty

Practical Use Cases for Vectors in C++
When to Use Vectors Instead of Arrays
Vectors are preferred over raw arrays because they can dynamically resize according to your needs. This flexibility simplifies memory management and reduces the chance of buffer overflows.
For example, if you are constructing a list of user inputs and the total number of entries remains uncertain, vectors are the ideal choice.
Vectors with User-Defined Data Types
Vectors can also store user-defined types, like classes or structures:
class Person {
public:
std::string name;
int age;
Person(std::string n, int a) : name(n), age(a) {}
};
std::vector<Person> people;
people.emplace_back("Alice", 30);
people.emplace_back("Bob", 25);
Multidimensional Vectors in C++
You can create vectors of vectors, effectively forming a 2D structure. Here’s an example:
std::vector<std::vector<int>> matrix(3, std::vector<int>(3, 0)); // 3x3 matrix initialized with 0
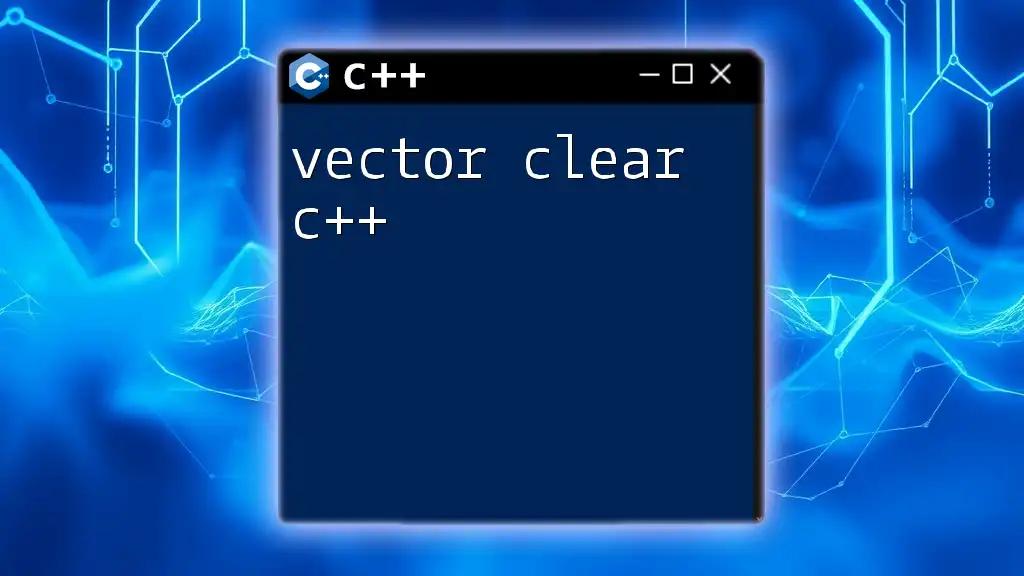
Performance Considerations with C++ Vectors
Vector Memory Management
Vectors manage their memory, allocating more space as needed when items are added. This dynamic nature can lead to performance benefits but may incur overhead if resizing occurs frequently.
Time Complexity of Common Vector Operations
Understanding time complexity helps in optimizing vector usage:
- Push and Pop: Average O(1)
- Access: O(1)
- Insertion/Deletion (at arbitrary positions): O(n)
Best Practices for Using Vectors
To enhance performance and readability, consider the following tips:
- Initialize with a size or reserve space when the size is known.
- Prefer `emplace_back()` over `push_back()` for user-defined types to avoid unnecessary copies.
- Avoid frequent resizing and popping operations in performance-critical code.
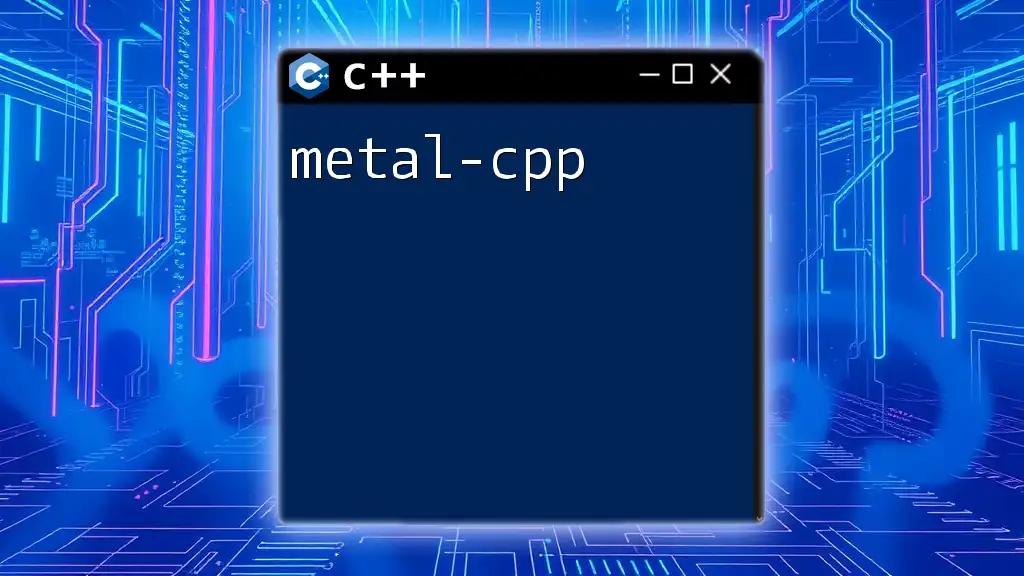
Conclusion
C++ vectors are a robust and essential tool for any programmer. They provide dynamic sizing functionality, rich methods for data manipulation, and efficient memory management. Whether working with simple data types or complex structures, mastering vectors can significantly enhance your programming capabilities. Explore and practice using vectors to see their full potential in your C++ applications.