Metal-C++ is a C++ programming framework that provides a high-performance interface for leveraging Apple’s Metal graphics API, enabling developers to write GPU-accelerated code in a familiar C++ syntax.
Here’s a simple example of how you can use Metal-C++ to create a basic compute kernel:
#include <metal_stdlib>
using namespace metal;
kernel void computeKernel(device float *input [[ buffer(0) ]],
device float *output [[ buffer(1) ]],
uint gid [[ thread_position_in_grid ]]) {
output[gid] = input[gid] * 2.0;
}
Setting Up Your Environment
To start programming with Metal-C++, it’s essential to have the right tools and environment set up.
Required Tools and Software
Before diving into code, ensure you have the following installed on your machine:
- Xcode: This is Apple’s integrated development environment (IDE) and is necessary for compiling and building your Metal-C++ applications. Make sure to install the latest version.
- Metal SDK: Metal is included with Xcode, but ensure you have the latest updates which provide enhancements and bug fixes.
Creating a Metal-C++ Project
- Open Xcode and create a new project.
- Select "macOS" and then choose "App".
- Name your project and set the language to C++. This ensures that your project can utilize metal-cpp features.
- After the project is created, navigate to the "Build Settings" and ensure the Metal API is enabled.
By following these steps, you will have a foundation ready for building your Metal-C++ applications.
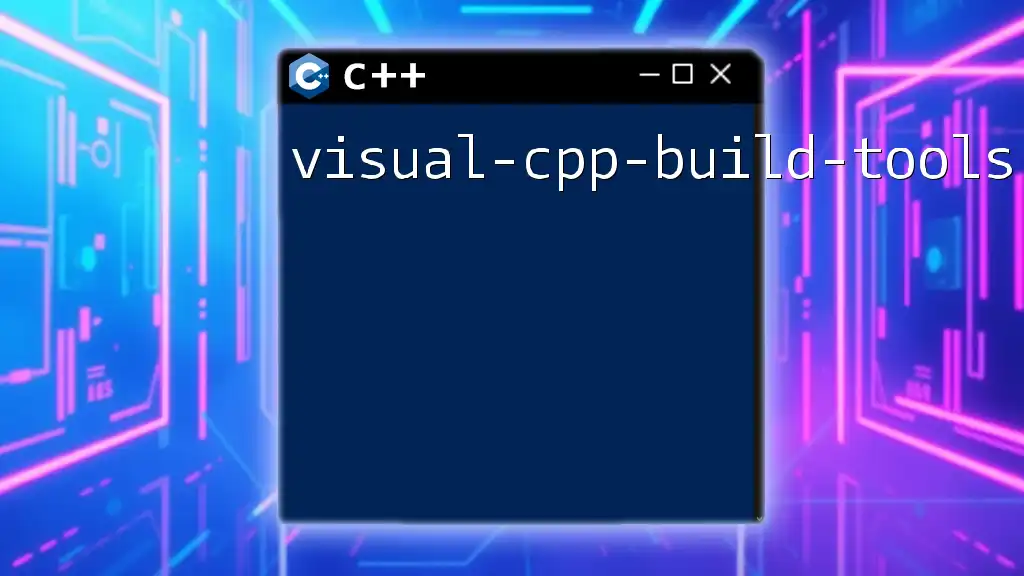
Understanding the Basics of Metal-C++
Core Concepts of Metal
Metal is a low-level graphics API developed by Apple that allows developers to interact directly with the GPU. Understanding its core aspects is crucial before venturing into Metal-C++ development.
-
What is Metal?
- Metal provides low-overhead access to the graphics processing unit (GPU), enabling high-performance rendering and data-parallel computation.
-
Overview of the Metal API architecture
- Metal has a clean, efficient structure that allows developers to submit commands to the GPU quickly, which is vital for rendering tasks and complex calculations.
C++ Integration
C++ integration in Metal development provides added advantages. It allows for more complex data structures, object-oriented programming, and powerful algorithms, making your application more maintainable and scalable.
- Pros of using C++ for Metal programming
- Enhanced performance and speed due to compiled code.
- Features such as classes and templates can make developing complex graphics applications more manageable.
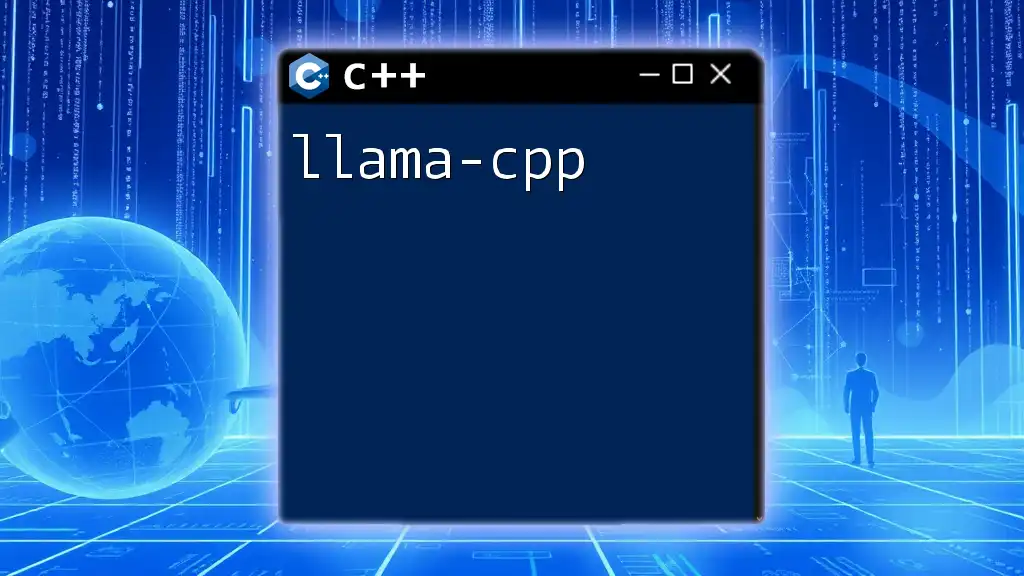
Building Your First Metal-C++ Application
Creating a Metal-C++ application is an exciting venture. Here’s how to get started with a basic application that renders a simple triangle.
Creating a Basic Metal Application
-
Initialize the Metal environment by including the necessary headers:
#include <Metal/Metal.h>
-
Initializing Metal in C++
id<MTLDevice> device = MTLCreateSystemDefaultDevice();
This line creates a Metal device, which acts as an interface between your application and the GPU.
-
Setting Up a Metal View
- You can create a Metal view that serves as a rendering surface. Keep in mind that you may need to subclass `NSView` for macOS applications or `UIView` for iOS applications, integrating Metal layer properties.
Rendering a Simple Triangle
The first graphical output most developers aim for is rendering a triangle. Below are essential steps to achieve this:
-
Vertex Data Preparation
- Define the vertices of the triangle in your C++ code.
float vertexData[] = { 0.0f, 1.0f, // Top vertex -1.0f, -1.0f, // Bottom left vertex 1.0f, -1.0f // Bottom right vertex };
-
Creating Buffers
- Allocate memory for the vertex data using Metal's buffer API.
id<MTLBuffer> vertexBuffer = [device newBufferWithBytes:vertexData length:sizeof(vertexData) options:MTLResourceStorageModeShared];
-
Loading a Shader
- Metal programs use shaders for rendering. You can create vertex and fragment shaders in the Metal Shading Language (similar to C-like syntax) and load them into your application. Compiling these shaders in Xcode will help avoid runtime errors.
-
Setting Pipeline State and Rendering
- Create a pipeline state object defining how the GPU should render your triangle. This pipeline state includes information about your shaders and any state configurations.
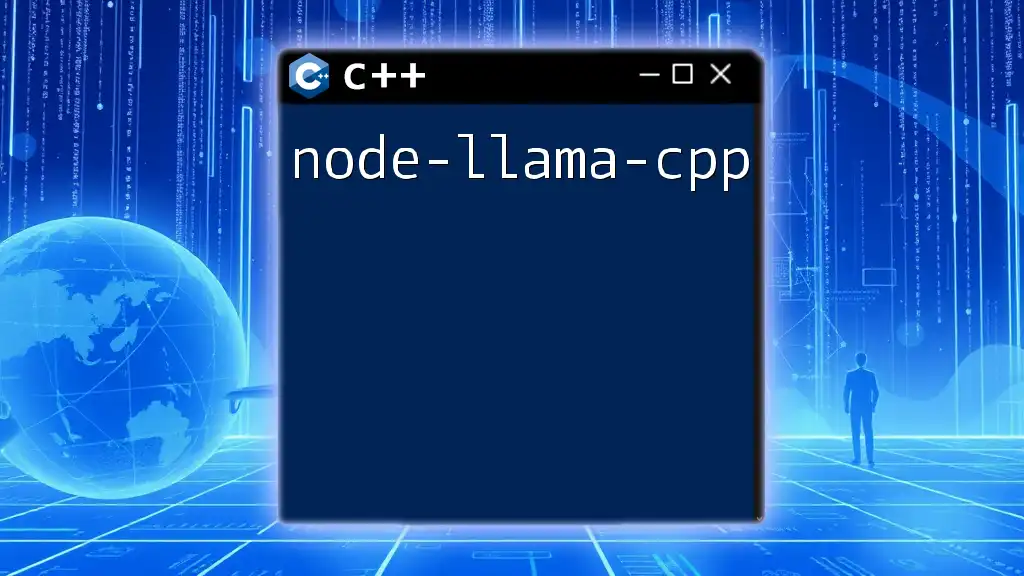
Exploring Advanced Metal-C++ Features
Once you grasp the basics, you can explore some of the more advanced features of Metal-C++.
Shader Programming
Shader programming is crucial for controlling the rendering pipeline. Metal-C++ allows you to write and use shaders efficiently.
- Writing GLSL to Metal Shaders
- If you're familiar with GLSL, know that transitioning to Metal’s shading language is modular and relatively straightforward.
- Compiling and loading shaders in C++
- Use the Metal API to compile your shaders within your C++ project and bind them to the rendering pipeline.
Memory Management in Metal-C++
Efficient memory management enhances performance. Metal-C++ requires developers to understand resource allocation strategies:
- Use GPU resources effectively by managing memory allocation and transfers between CPU and GPU.
- Use buffer access policies wisely to avoid bottlenecks.
Synchronization and Performance Optimization
Performance is a priority when programming with metal-cpp.
- Thread management with C++ can help distribute rendering tasks efficiently.
- Implement Render Passes and Command Buffers to batch rendering commands and minimize GPU stalls.
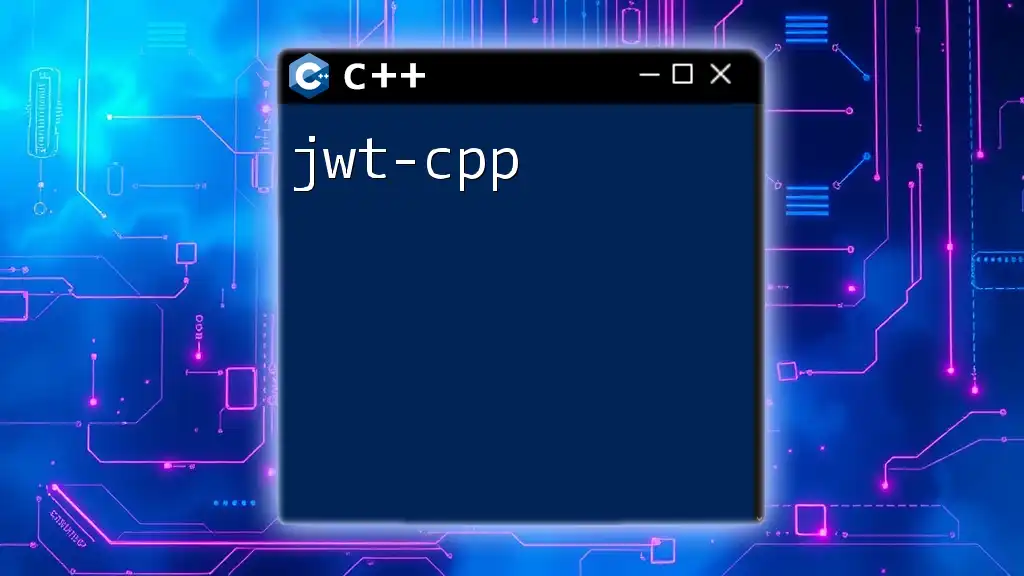
Interfacing Metal-C++ with Other Technologies
Combining Metal with OpenGL
For those transitioning from OpenGL, Metal-C++ can seamlessly complement existing applications.
- Understanding how to pass data between OpenGL and Metal provides added flexibility and can enhance performance in hybrid applications.
Integration with UIKit and Swift
Knowing how to bridge your Metal-C++ code with Objective-C and Swift opens doors to iOS development:
- Utilize Objective-C++ to combine C++ and Objective-C code within your application seamlessly.
- Implementing Metal-C++ in a Swift-based application involves bridging headers and using Type safety features.
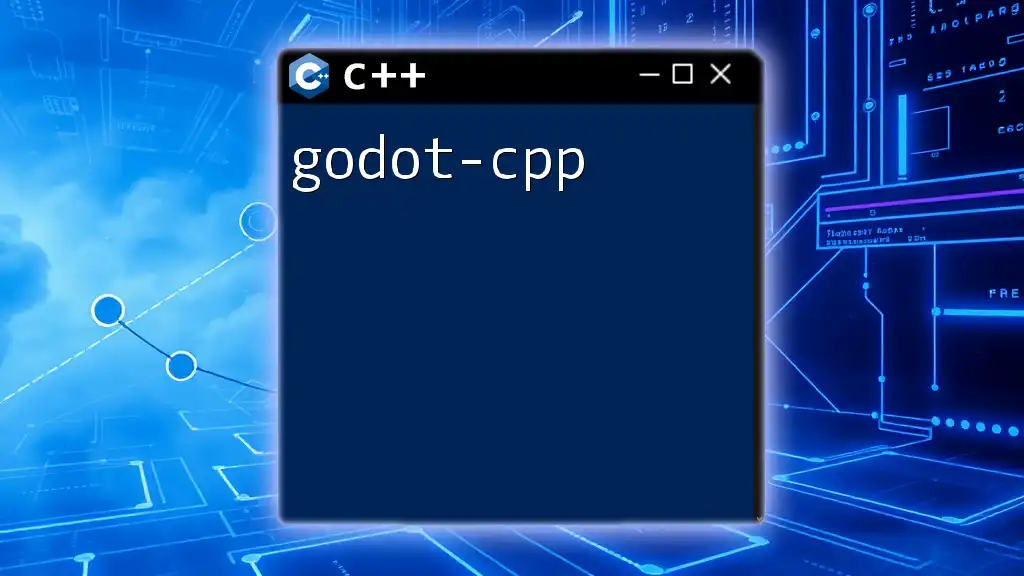
Debugging and Testing Metal-C++ Applications
Best Practices for Debugging
Debugging in Metal-C++ can initially seem daunting but adopting the right strategies can help:
- Common pitfalls include improper device initialization and buffer mismanagement. Always double-check the state of your GPU and pipeline.
- Utilize Xcode’s debugger and GPU frame capture tools to identify issues rapidly.
Performance Profiling
Boosting the efficiency of your Metal-C++ applications is essential.
- Xcode Instruments allows you to profile your application concerning CPU and GPU performance. Monitor the frame rate, memory usage, and the overall rendering time to identify bottlenecks.
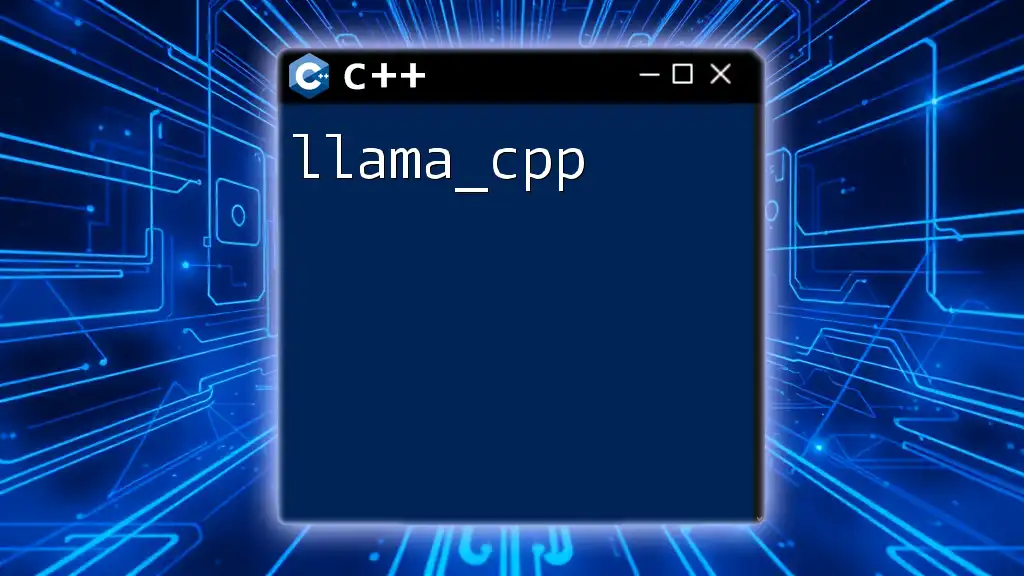
Conclusion
Recapping key points, Metal-C++ serves as a powerful tool for graphics programming, providing developers with significant benefits over traditional approaches. Metal-C++ will continue to evolve and embrace new features, keeping it at the forefront of graphics programming. For those looking for further resources, numerous online courses, books, and community forums exist to deepen your understanding and skills.
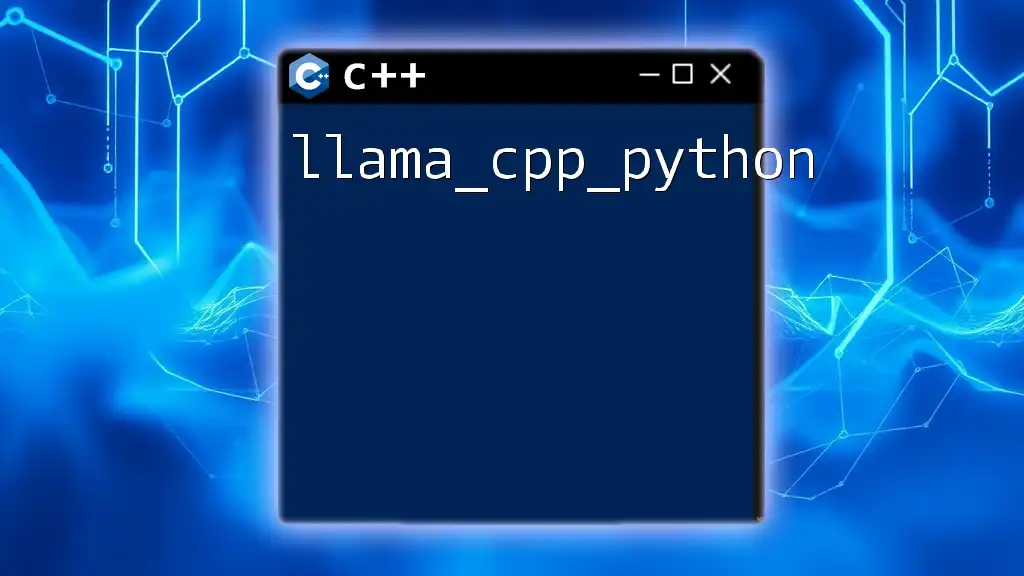
Frequently Asked Questions (FAQs)
- Common questions often revolve around compatibility issues or shader programming tips. Engaging with the community can provide additional insight and troubleshooting support.
- Always consult the official Metal documentation for the latest updates and examples tailored for Metal-C++.
By following this guide, you are well on your way to mastering metal-cpp and unleashing its potential in your graphics applications. Happy coding!