Godot-cpp is a bindings library that allows developers to write Godot scripts in C++, enabling the use of C++ features and performance while integrating seamlessly with the Godot engine.
Here’s an example of a simple C++ script using Godot-cpp to create a basic node:
#include <Godot.hpp>
#include <Node.hpp>
namespace godot {
class MyNode : public Node {
GODOT_CLASS(MyNode, Node)
public:
static void _register_methods() {
register_method("_ready", &MyNode::_ready);
}
void _init() {}
void _ready() {
Godot::print("Hello from C++!");
}
};
}
Introduction to godot-cpp
What is godot-cpp?
godot-cpp is a set of C++ bindings for the Godot Engine, enabling developers to write game logic and functionality using C++. It provides a seamless way to integrate C++ code into Godot projects, giving access to the robust performance and features of the C++ language while leveraging Godot's powerful game development tools.
Why Use C++ in Godot?
Utilizing C++ in a Godot project comes with numerous advantages. Performance enhancements are one of the most significant benefits; C++ is known for its speed and efficiency, especially for complex calculations or real-time systems. It allows developers to implement high-performance logic without sacrificing the ease of use provided by Godot's visual environment. Additionally, using C++ grants access to a wide array of C++ features and libraries, enriching the capabilities of your game beyond what’s readily available in GDScript.
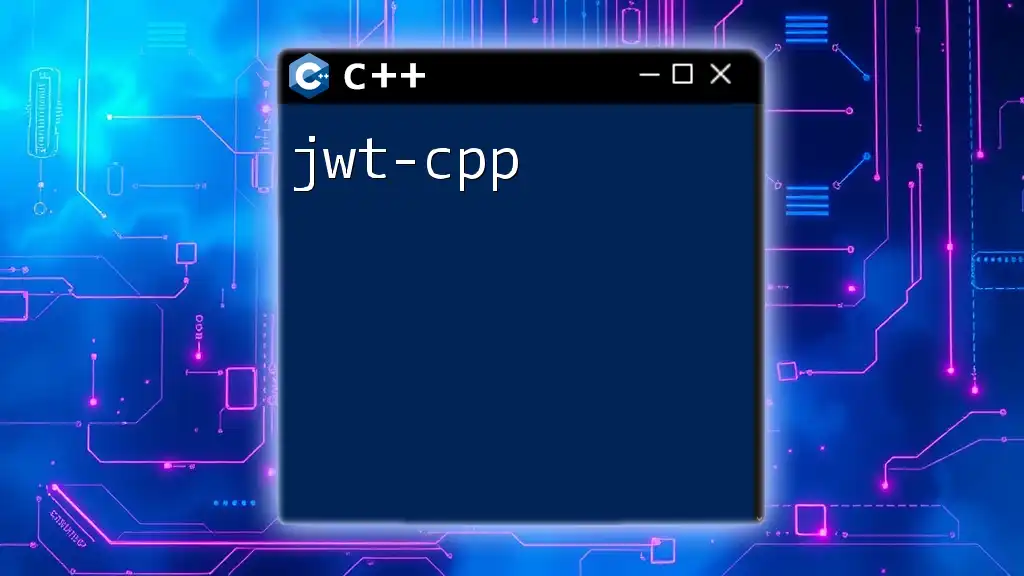
Setting Up godot-cpp
Installation Prerequisites
Before diving into godot-cpp, ensure you have the following software installed:
- Godot Engine: Make sure you have the latest stable version.
- CMake: A cross-platform build system is essential for compiling godot-cpp.
- A C++ compiler: Depending on your operating system, you can use MSVC on Windows, GCC on Linux, or Clang on macOS.
Clone the godot-cpp Repository
Fetching the godot-cpp source code is straightforward. You can clone the repository directly from GitHub. Open your terminal or command prompt and execute:
git clone https://github.com/godotengine/godot-cpp.git
This command will create a local copy of the library in a directory named `godot-cpp`.
Building the Godot C++ Bindings
After cloning, navigate into the `godot-cpp` directory. Building the bindings requires CMake. Run the following commands:
cd godot-cpp
mkdir build
cd build
cmake ..
cmake --build .
This process creates all necessary bindings for you to start with. If any errors occur, check the installation of your CMake and compiler, as these are common sources of issues.
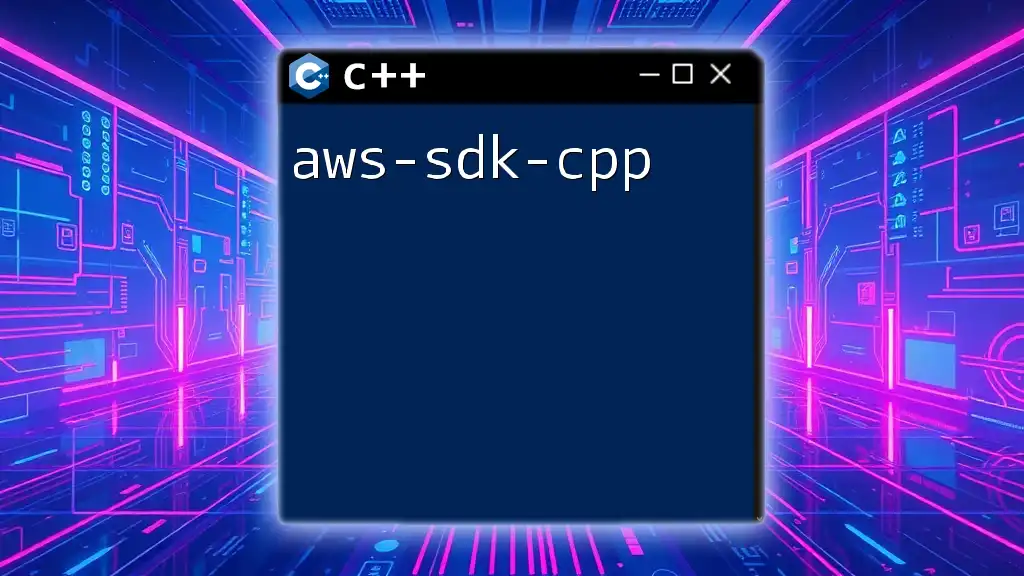
Integrating C++ with Godot
Creating a New Godot C++ Project
To utilize godot-cpp, first create a new C++ project within Godot. Start by launching Godot and create a new project. Organize your directory by creating a folder structure that maintains clarity between your Godot files and C++ source code.
Writing Your First C++ Script
Once your project is set up, you can start writing C++ scripts. Here’s how to create a simple script that prints a message to the console:
#include <Godot.hpp>
using namespace godot;
class MyNode : public Node {
GODOT_CLASS(MyNode, Node)
public:
static void _register_methods() {
register_method("_process", &MyNode::_process);
}
void _init() {}
void _process(float delta) {
Godot::print("Hello from C++!");
}
};
In this example, we define a class `MyNode` that inherits from Godot's Node class. The `_process` method, called every frame, outputs a message to the console.
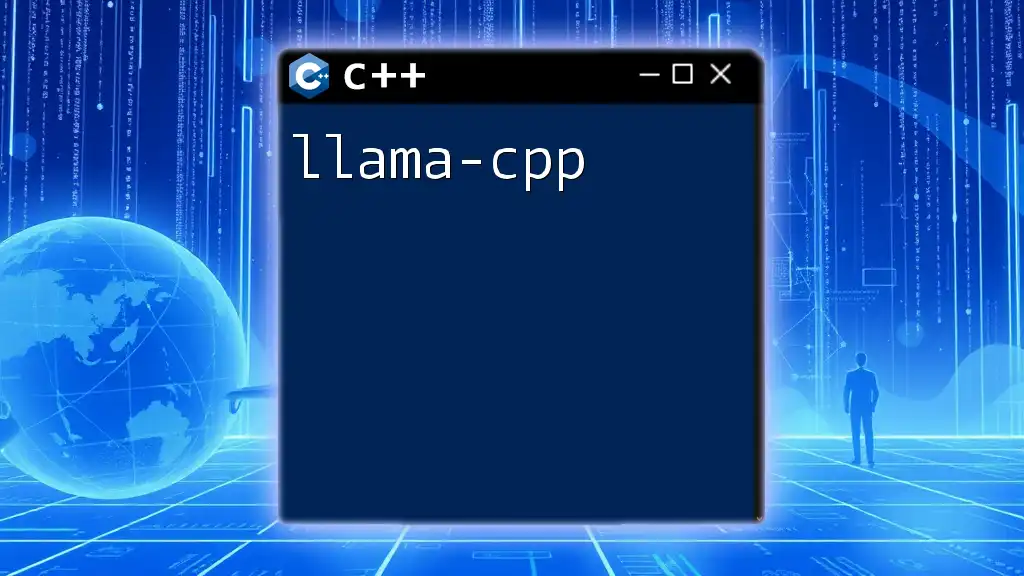
Godot C++ Scripting Basics
Understanding the Godot C++ API
The Godot C++ API is structured and intuitive. It mirrors the GDScript API closely, making it easier for developers transitioning from GDScript to C++. Critical classes such as `Node`, `Scene`, and `Godot` play fundamental roles in game object management and interactions.
Commonly Used Classes and Functions
Understanding frequently utilized classes is crucial for effective game scripting in C++. Classes such as `Spatial`, `CanvasItem`, and `Resource` form the backbone of Godot’s node system. For example, handling user input can be managed through functions tied to the `Input` class.
Signals and Custom Signals
Signals are a powerful feature that enables communication between different objects. You can define custom signals in your C++ scripts, allowing you to create responsive gameplay elements. Here is an example of a custom signal:
signal my_signal;
void my_function() {
emit_signal("my_signal");
}
Connect this signal to another method using Godot’s signal system to trigger actions in response.
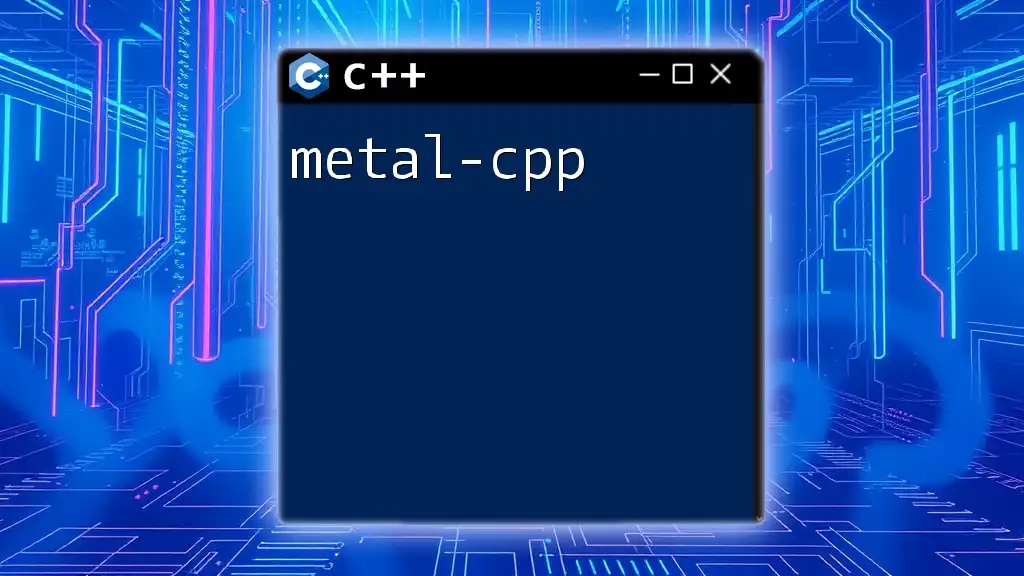
Advanced Features in godot-cpp
Using Godot's Built-in Types in C++
Godot provides several built-in types, such as `Variant`, `Array`, and `Dictionary`, which simplify data handling in a game. For example, using a `Variant` can ease the process of storing different data types:
Variant myVariant = 42; // Storing an integer
Array myArray;
myArray.append("Hello");
myArray.append(3.14);
This versatility allows for dynamic gameplay scenarios where data types may vary.
Integrating Third-Party Libraries with godot-cpp
Using third-party libraries can significantly enhance your game's functionality. When integrating libraries such as SDL for multimedia or OpenCV for advanced image processing, make sure to utilize CMake effectively to link these libraries during the build process.
Performance Optimization Tips
To optimize performance when writing C++ code for Godot, consider the following practices:
- Avoid deep hierarchies of nodes; flatten your node structure where possible.
- Use pools efficiently to manage object creation and destruction, minimizing overhead.
- Profile code using Godot’s built-in profiler to identify bottlenecks and optimize accordingly.
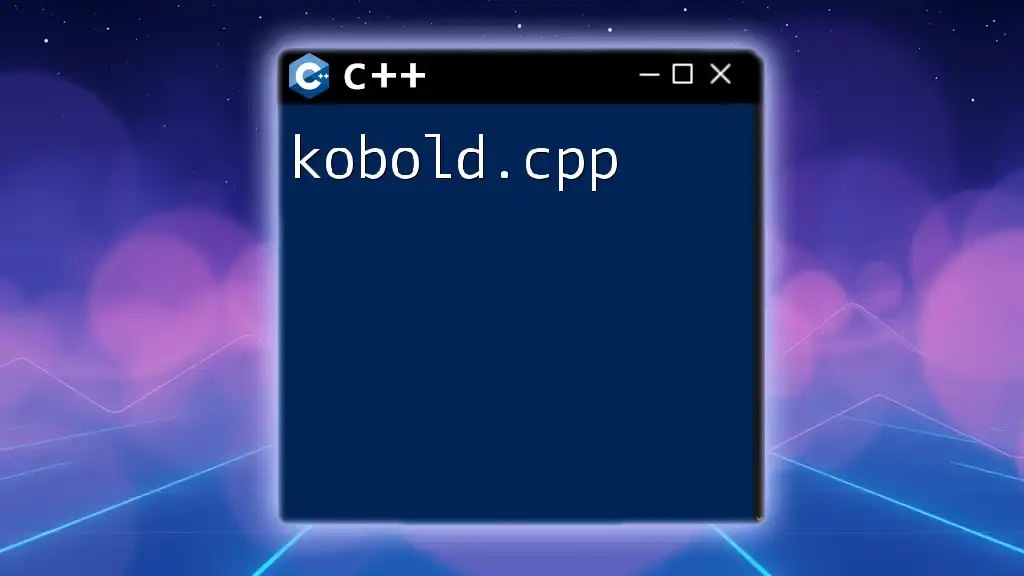
Debugging and Testing C++ Scripts
Setting Up Debugging in Godot
Godot’s debugger offers a comprehensive interface for diagnosing issues. To debug C++ scripts, ensure your project is compiled in Debug mode and utilize breakpoints to monitor the execution flow.
Unit Testing in C++
Unit testing is crucial for ensuring code reliability. By integrating a testing framework into your godot-cpp project, you can create tests that run automatically, helping maintain code quality. Leveraging libraries like Google Test can streamline this process while offering robust functionality.
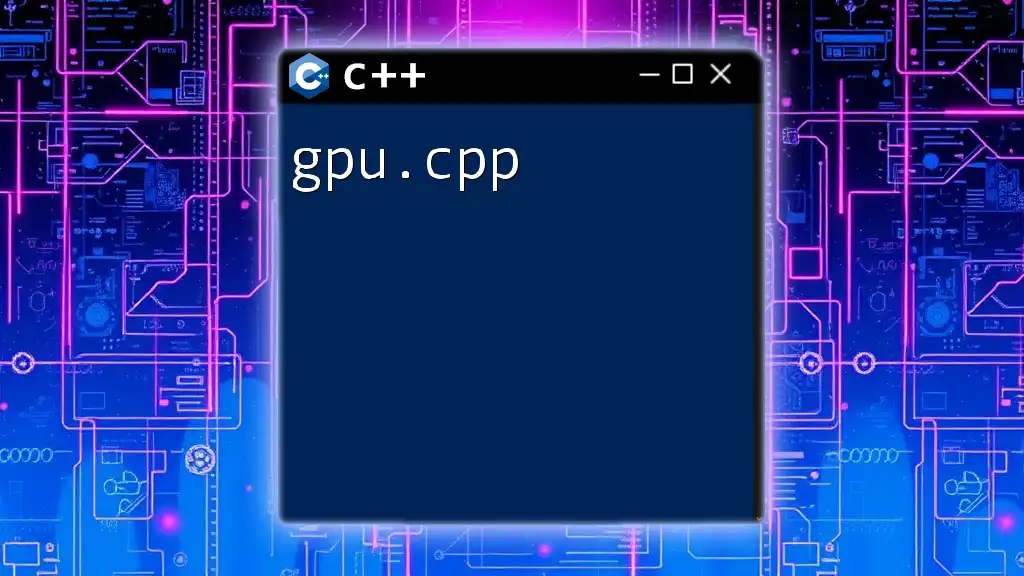
Real-World Examples and Case Studies
Case Study: Simple Game Implementation
Consider creating a simple 2D platformer using godot-cpp. This game could utilize C++ for handling physics calculations, player movement, and AI behavior. By separating these functionalities into distinct classes, you can manage complexity while maximizing performance.
Example Projects and Resources
Explore open-source projects that utilize godot-cpp on platforms such as GitHub. Engaging with existing codebases allows for practical learning and experience. The Godot community also offers extensive resources through forums and Discord channels, promoting collaboration and knowledge sharing.
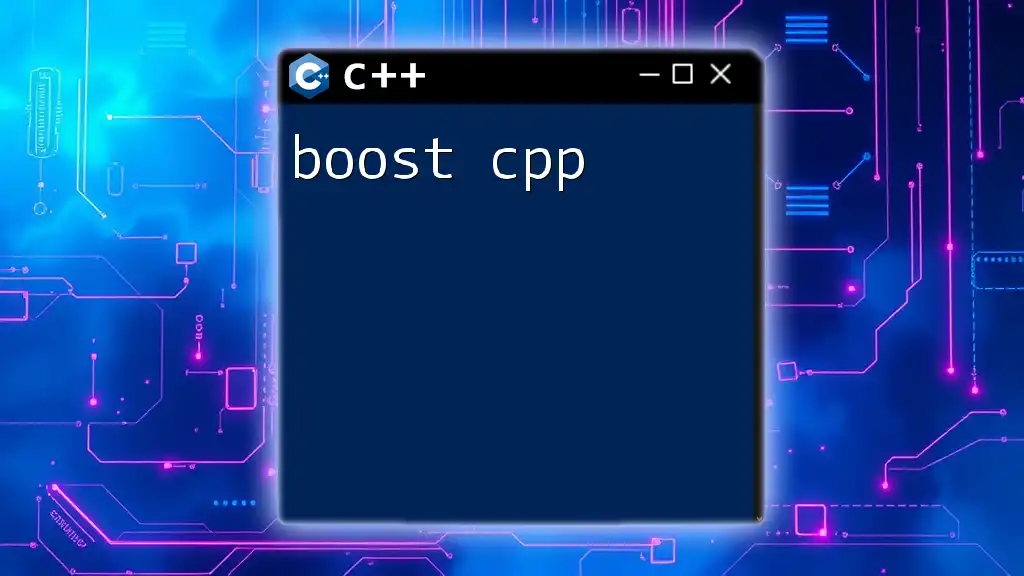
Conclusion
Recap of Key Points
The godot-cpp library empowers developers to combine the flexibility of Godot’s engine with the efficiency of C++. By following the guide outlined above, you are well on your way to leveraging the robust capabilities of C++ in your game projects.
Next Steps for Aspiring Developers
As you continue your journey with godot-cpp, immerse yourself in additional tutorials and documentations. Practice by implementing small projects that incrementally challenge your understanding of C++. Engaging with communities and contributing to open-source projects can also enhance your learning experience while building valuable connections in the industry.
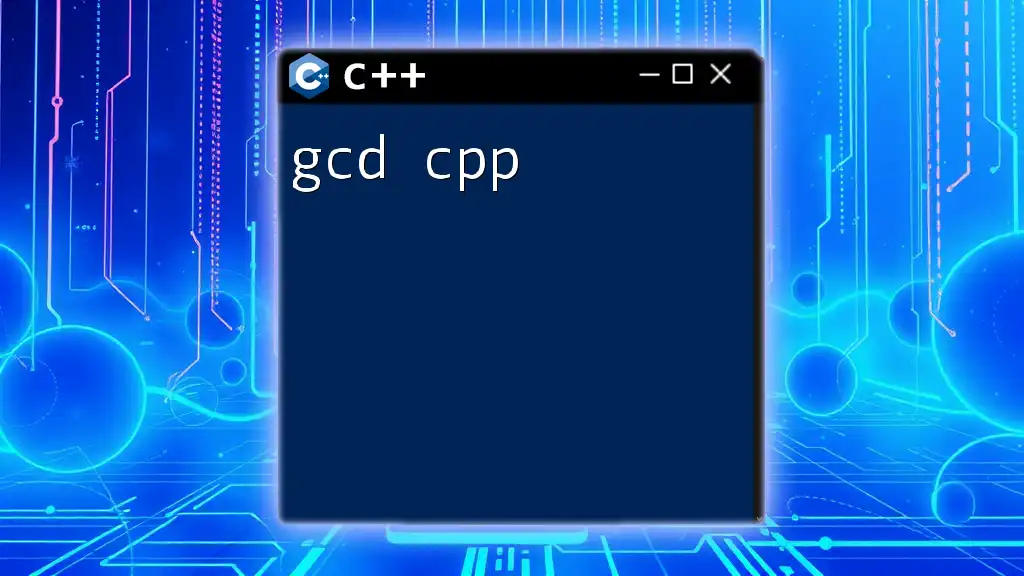
Additional Resources
Explore the Godot Engine Documentation for thoroughly detailed information on using C++. Online courses and recommended books can also provide deeper insights, helping strengthen your C++ skills and game development expertise.