The AWS SDK for C++ provides a set of APIs that allow developers to interact with various Amazon Web Services using C++ programming language.
Here's a simple example of how to initialize the SDK and create an S3 client:
#include <aws/core/Aws.h>
#include <aws/s3/S3Client.h>
int main() {
Aws::SDKOptions options;
Aws::InitAPI(options);
{
Aws::S3::S3Client s3_client;
// Use s3_client to interact with Amazon S3
}
Aws::ShutdownAPI(options);
return 0;
}
What is AWS SDK for C++?
The AWS SDK for C++ (aws-sdk-cpp) is a robust toolkit designed to enable developers to interact seamlessly with Amazon Web Services (AWS). This software development kit simplifies the process of connecting applications to AWS services, ranging from storage solutions like S3 (Simple Storage Service) to powerful computing resources such as EC2 (Elastic Compute Cloud). By leveraging the SDK, developers can focus on building applications while offloading the complexities of AWS integrations.
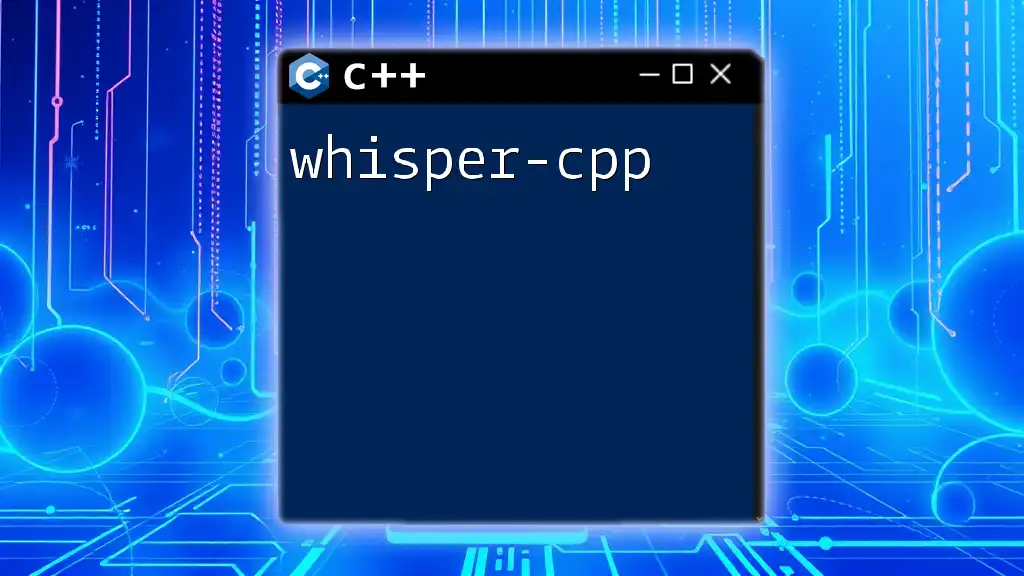
Benefits of Using AWS SDK for C++
Using the AWS SDK for C++ offers several significant advantages:
- Simplified Access to AWS Services: The SDK provides a convenient API layer for interacting with various AWS services, streamlining routine tasks such as retrieving, storing, and managing data.
- Cross-Platform Compatibility: The AWS SDK supports multiple platforms, including Windows, macOS, and Linux. This versatility enables developers to write code that remains consistent regardless of the operating system.
- Efficient Resource Management: Built-in utilities help manage connections and resources effectively, allowing for better handling of performance and error scenarios.
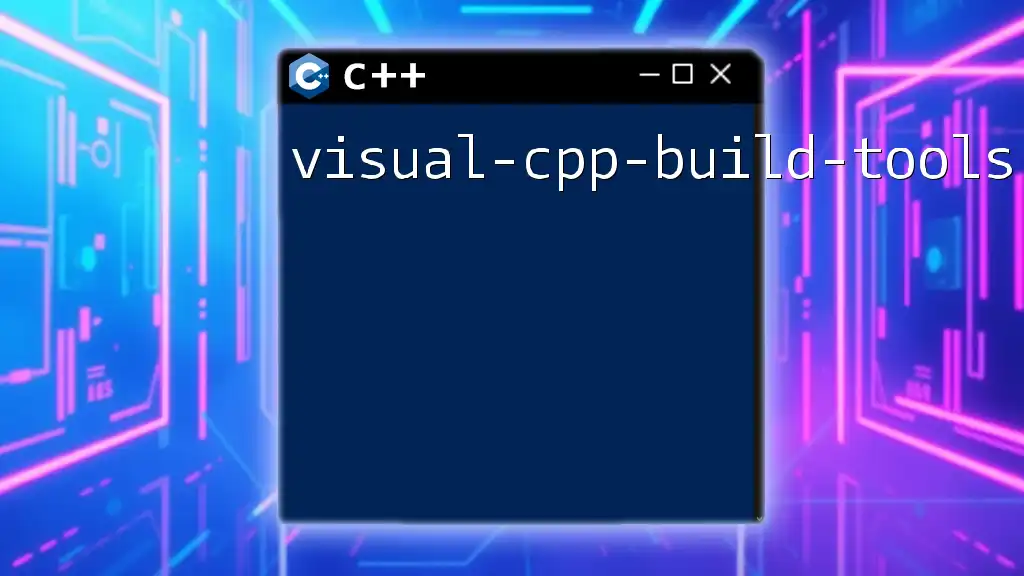
Getting Started with AWS SDK for C++
Prerequisites
Before diving into the AWS SDK for C++, it is essential to have a foundation in the following areas:
- Basic Knowledge of C++: Familiarity with C++ programming constructs is crucial.
- Understanding AWS Services: Having an overview of the various AWS services will support effective integration.
- Required Tools: Ensure that you have essential tools installed, like CMake and a C++ compiler (such as gcc or clang).
Installing AWS SDK for C++
Using Package Managers
The simplest way to install the AWS SDK for C++ is via package managers:
- Homebrew (macOS): Use `brew install aws-sdk-cpp`.
- apt (Ubuntu/Debian): Leverage `sudo apt-get install libaws-sdk-cpp-dev`.
- vcpkg (Windows): Utilize `vcpkg install aws-sdk-cpp`.
Building from Source
Alternatively, you can build the SDK directly from the source:
- Clone the repository from GitHub:
git clone https://github.com/aws/aws-sdk-cpp.git
- Create a build directory and navigate into it:
cd aws-sdk-cpp mkdir build && cd build
- Run the CMake configuration:
cmake .. -DBUILD_ONLY="s3;ec2" # Specify the AWS services you wish to include
- Finally, compile the SDK:
make
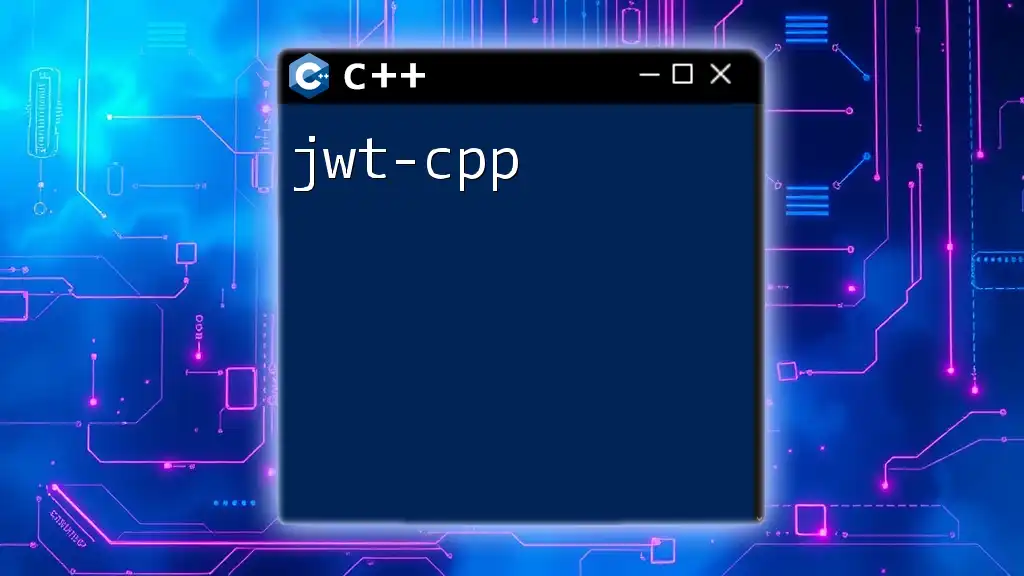
Key Components of AWS SDK for C++
Main Libraries
The AWS SDK for C++ encompasses various libraries, each tailored to specific functionalities:
Core Libraries
Core libraries include essential components shared among various services. Understanding these libraries is foundational as they manage lower-level operations and dependency management.
Service-Specific Libraries
The SDK also offers service-specific libraries, such as:
- S3 for storage
- DynamoDB for database access
- EC2 for compute resources
Configuration and Initialization
Setting Up the SDK
Before utilizing the SDK, configuring your AWS credentials is necessary. You can achieve this by creating the credentials file at `~/.aws/credentials`.
Initializing the SDK
Initialization is a crucial step in any application. Use the `Aws::InitAPI()` function to get started and the `Aws::ShutdownAPI()` function to clean up resources later:
#include <aws/core/Aws.h>
Aws::SDKOptions options;
Aws::InitAPI(options);
{
// Your code here for AWS service interactions
}
Aws::ShutdownAPI(options);
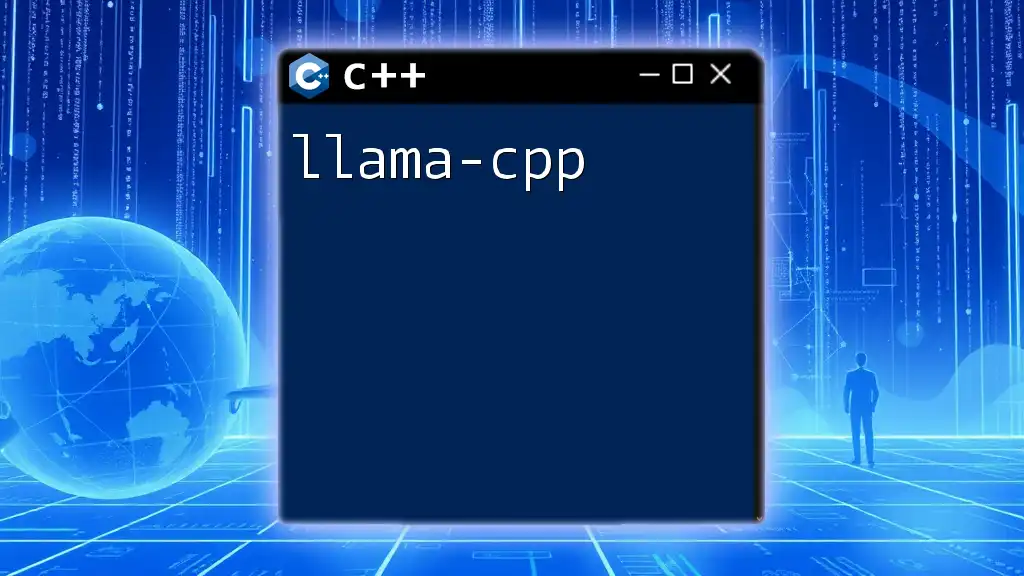
Working with AWS Services
Accessing Amazon S3
Setting Up the S3 Client
To work with Amazon S3, the first step is to initialize the S3 client:
#include <aws/s3/S3Client.h>
Aws::S3::S3Client s3_client;
Uploading Files to S3
Once the client is set up, you can upload files to S3. Here is a code snippet exemplifying how to upload a file to a designated bucket:
#include <aws/s3/model/PutObjectRequest.h>
Aws::S3::Model::PutObjectRequest object_request;
object_request.SetBucket("my-bucket");
object_request.SetKey("myfile.txt");
// Replace 'body_stream' with your input stream for the file
object_request.SetBody(body_stream);
// Perform the upload
auto outcome = s3_client.PutObject(object_request);
if (outcome.IsSuccess()) {
std::cout << "File uploaded successfully!" << std::endl;
} else {
std::cerr << "Error uploading file: " << outcome.GetError().GetMessage() << std::endl;
}
Managing DynamoDB Data
Creating a DynamoDB Client
Next, if you're working with DynamoDB, create a DynamoDB client as follows:
#include <aws/dynamodb/DynamoDBClient.h>
Aws::DynamoDB::DynamoDBClient dynamo_client;
Adding Items to DynamoDB
To add items to a DynamoDB table, you can use the following example, illustrating how to put an item into a table:
#include <aws/dynamodb/model/PutItemRequest.h>
Aws::DynamoDB::Model::PutItemRequest request;
request.SetTableName("my_table");
// Construct your item to put in the request
Aws::DynamoDB::Model::AttributeValue value;
value.SetS("Sample Item");
request.AddItem("PrimaryKey", value);
auto outcome = dynamo_client.PutItem(request);
if (outcome.IsSuccess()) {
std::cout << "Item added successfully!" << std::endl;
} else {
std::cerr << "Error adding item: " << outcome.GetError().GetMessage() << std::endl;
}
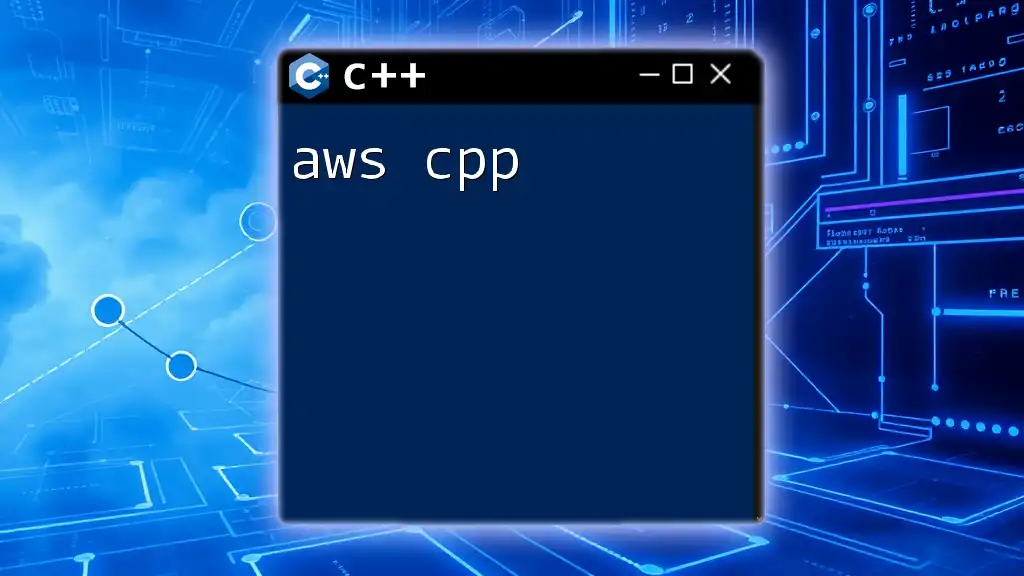
Error Handling and Debugging
Understand Error Codes
Error handling is vital for application stability. Familiarize yourself with common error codes in the AWS SDK for C++. You can retrieve detailed error messages using `Aws::Client::AWSError`, which provides insight into issues like connection problems or invalid parameters.
Debugging Tips
The SDK offers logging features that can help debug issues effectively. You can enable verbose logging by initializing the logging system as shown below:
Aws::Utils::Logging::InitializeAWSLogging(
Aws::MakeShared<Aws::Utils::Logging::DefaultLogSystem>(
"aws_sdk_logfile.log", Aws::Utils::Logging::LogLevel::Debug));
This allows for detailed logs, making it easier to trace problems in your AWS interactions.
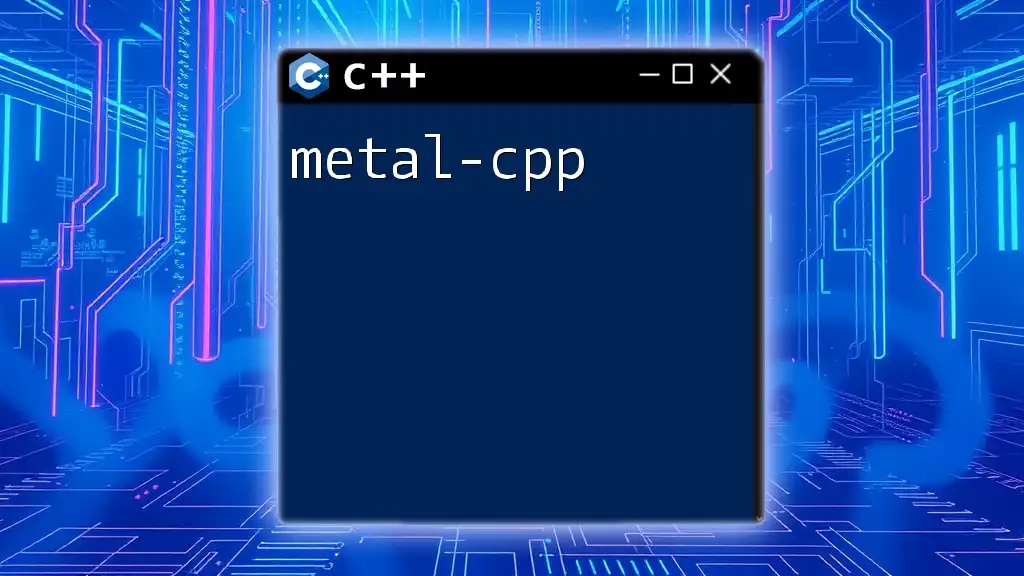
Best Practices
Code Organization
Maintaining a well-structured codebase is essential when working with AWS SDK for C++. Organize your project effectively to allow easy navigation and management of AWS service interactions.
Security Considerations
When implementing solutions with AWS, understand the significance of IAM roles and policies. Practicing diligent security measures—such as using environment variables for credentials—enhances the security of your applications and minimizes exposure to unauthorized access.
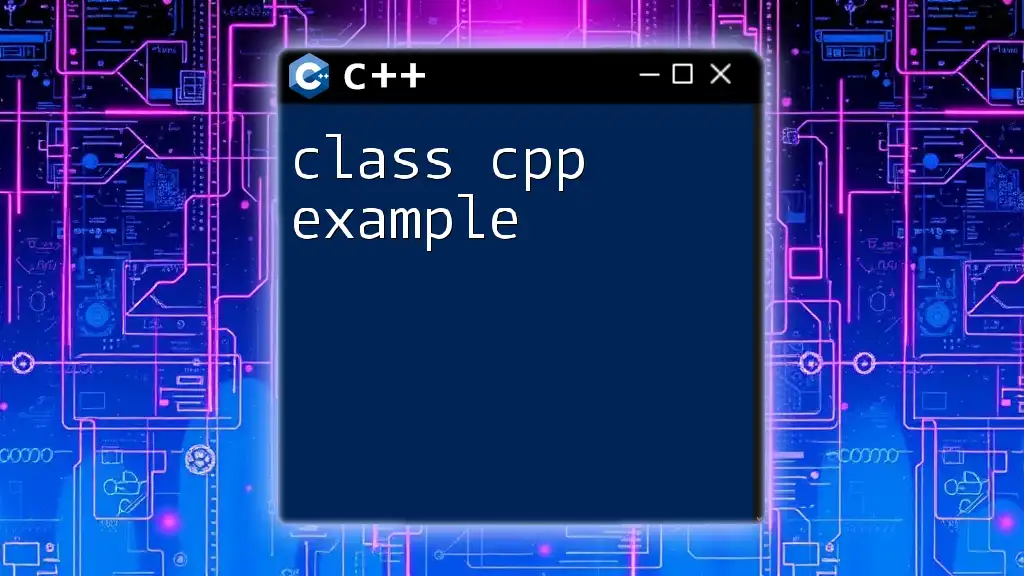
Conclusion
The AWS SDK for C++ empowers developers with a robust framework to integrate and manage AWS services effortlessly. With the right setup and understanding of its core components, transitioning your C++ applications to use cloud resources becomes an achievable task. Embrace the SDK and start exploring the vast possibilities of AWS to enhance your projects with state-of-the-art cloud technology.
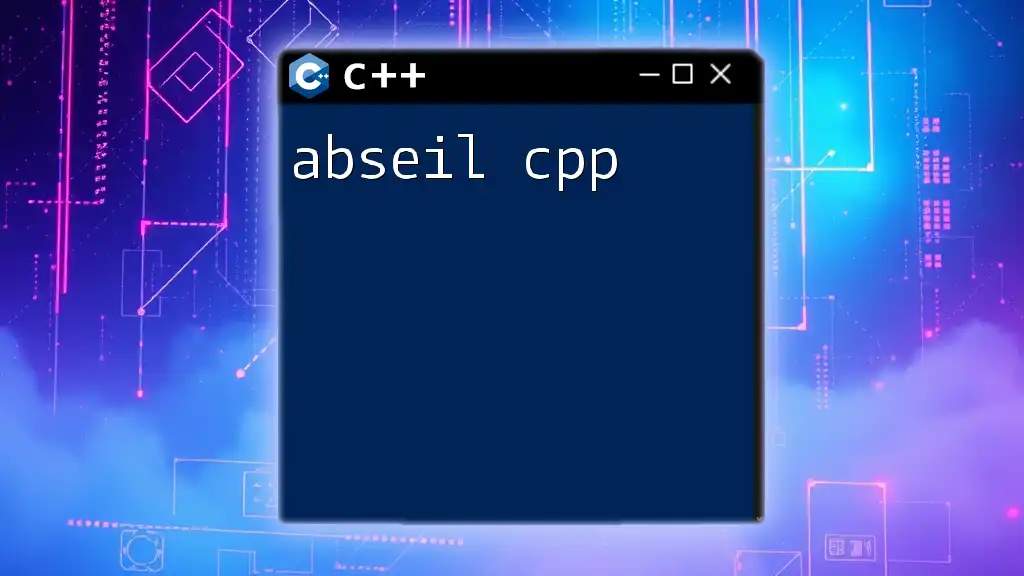
Additional Resources
To further deepen your knowledge, be sure to explore the official AWS SDK for C++ documentation, which offers comprehensive guidelines and examples. Engage with community forums for shared knowledge, or follow suggested learning paths to master AWS services alongside C++.