A hashmap in C++ is a data structure that allows you to store key-value pairs for efficient data retrieval using hash functions.
Here's a simple code snippet demonstrating how to use `std::unordered_map` from the C++ Standard Library:
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> hashmap;
hashmap["apple"] = 1;
hashmap["banana"] = 2;
// Accessing value by key
std::cout << "The value associated with 'banana' is: " << hashmap["banana"] << std::endl;
return 0;
}
What is a Hashmap?
A hashmap is a data structure that implements an associative array, a structure that can map keys to values. Hashmaps are incredibly efficient because they provide a way to retrieve values using unique keys with average-case O(1) time complexity. This is significantly faster than other data structures like arrays or linked lists, which typically require linear time to search for an element.
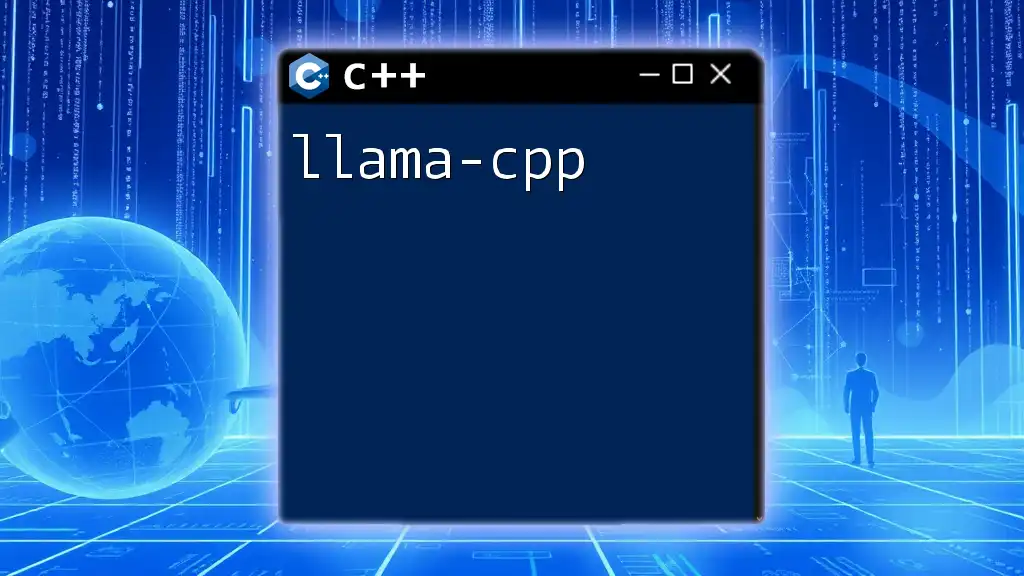
Importance of Hashmaps in C++ Programming
Hashmaps are essential in C++ programming due to their high-performance characteristics and versatility. They allow developers to store and manipulate data efficiently, making them invaluable for various programming tasks including:
- Fast data retrieval
- Frequency counting for items
- Implementing caches
By utilizing hashmaps, you can optimize your applications for performance and efficiency.
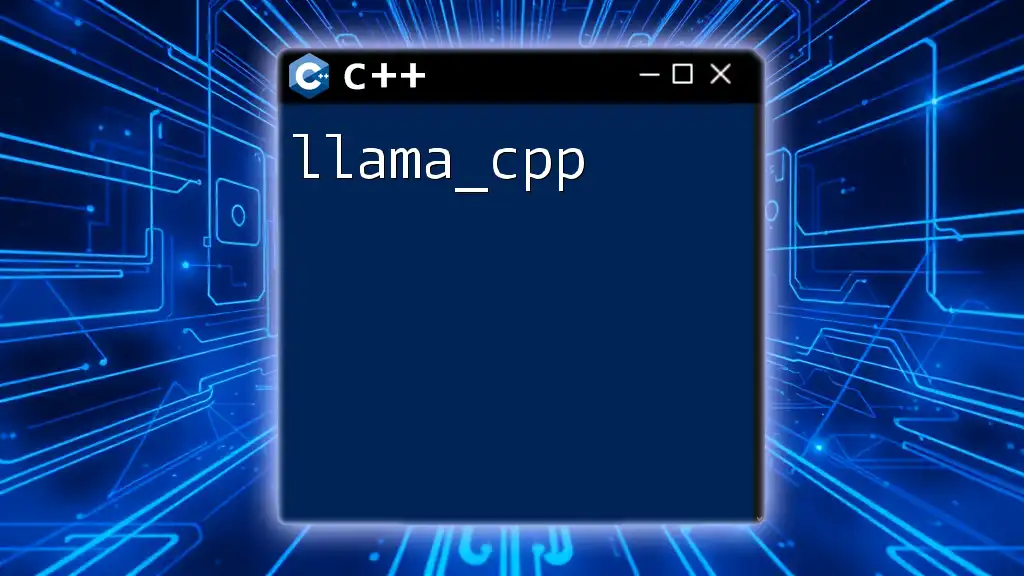
Understanding the Basics of C++ Hashmaps
Key Concepts
In understanding hashmap cpp, it's crucial to grasp a few fundamental concepts:
Keys and Values: In a hashmap, each value is associated with a unique key. The key serves as an identifier that lets you retrieve the corresponding value quickly.
Hash Function: A hash function converts a given key into a hash code, which is an index to store the corresponding value in the hashmap. This process is fundamental to the efficiency of hashmaps, as it minimizes search time.
Common Terms in C++ Hashmaps
Understanding certain terms will help clarify hashmap functionalities:
- Collision: This occurs when two distinct keys hash to the same index.
- Load Factor: This represents the ratio of the number of elements to the number of buckets in the hashmap. A higher load factor can lead to more collisions.
- Rehashing: As elements are added and the load factor increases, rehashing reallocates the hashmap to ensure optimal performance.
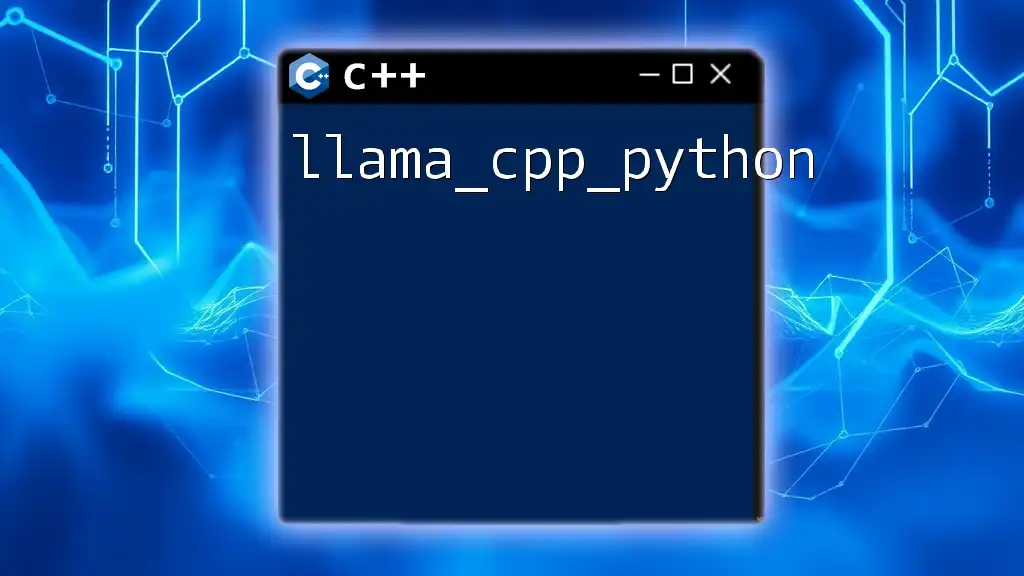
Implementing Hashmaps in C++
Using C++ Standard Library's `std::unordered_map`
C++ provides the `std::unordered_map` as part of its Standard Template Library (STL). This structure allows for easy implementation of hashmaps.
Creating a basic hashmap:
To get started, here’s how you can create a simple hashmap in C++:
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> hashmap;
hashmap["apple"] = 1;
hashmap["banana"] = 2;
return 0;
}
In this example, we create a hashmap that associates string keys with integer values. You can easily extend this to store a variety of data types.
Accessing elements: Accessing a value based on its key is straightforward. For instance:
int appleValue = hashmap["apple"]; // Returns 1
Adding and removing elements: Adding a new key-value pair is done using the assignment operator, while removing an element can be achieved with the `erase` method:
hashmap["orange"] = 3; // Add new element
hashmap.erase("banana"); // Remove "banana"
Custom Hash Functions
In some cases, you may need custom hash functions that tailor to your specific data types or requirements. This is particularly useful when dealing with complex keys.
To create a custom hash function, follow this example:
struct CustomHash {
std::size_t operator()(const std::string& key) const {
return std::hash<std::string>()(key);
}
};
By defining a custom hash struct, you can now utilize it for more specialized needs when creating hashmaps.
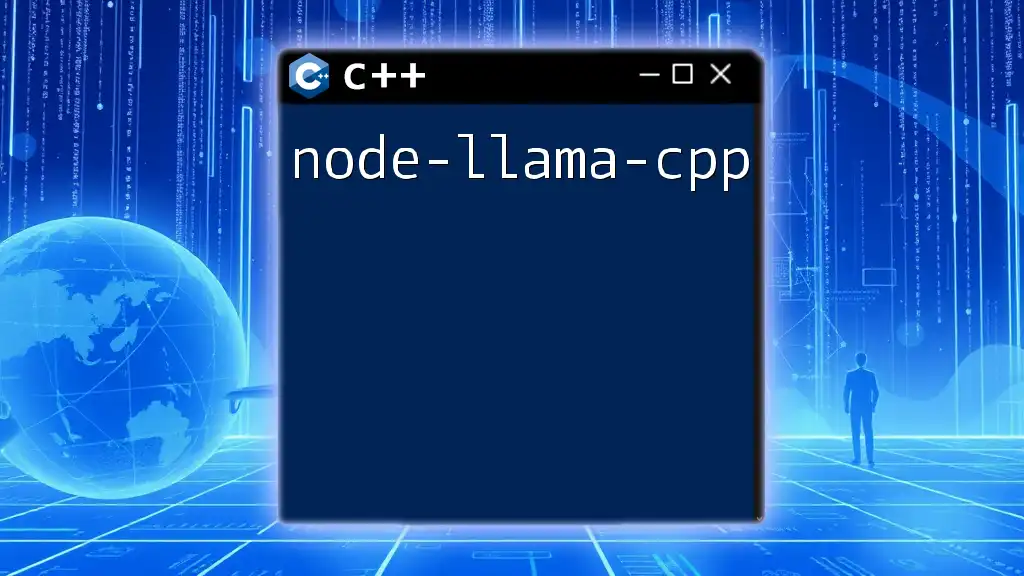
Advanced Techniques with C++ Hashmaps
Handling Collisions
Collisions are an unavoidable aspect of using hashmaps. Two main techniques to handle them are chaining and open addressing.
-
Chaining: This technique involves storing multiple values at the same index in a linked list. If a collision occurs, multiple values can still slot into that one index without loss.
-
Open Addressing: In this technique, instead of storing multiple values, if a collision occurs, you look for another empty slot following a specific probing sequence.
Performance Considerations
Hashmap performance can degrade under certain circumstances. It's essential to consider:
-
Time Complexity: The average-case time complexity for lookup, insert, and delete operations is O(1). However, in the worst-case scenario (e.g., many collisions), this can degrade to O(n).
-
Load Factor: A high load factor means you're nearing the limits of your hashmap. It’s advisable to resize or rehash the hashmap periodically to maintain performance.
Best Practices for Using Hashmaps in C++
To leverage hashmaps effectively, consider the following best practices:
-
Choosing the Right Hash Function: A good hash function minimizes collisions and ensures even distribution across buckets.
-
Memory Management: Be conscious of memory usage, especially as you store more items. Optimize the initial capacity to suit the expected load to avoid excessive rehashing.
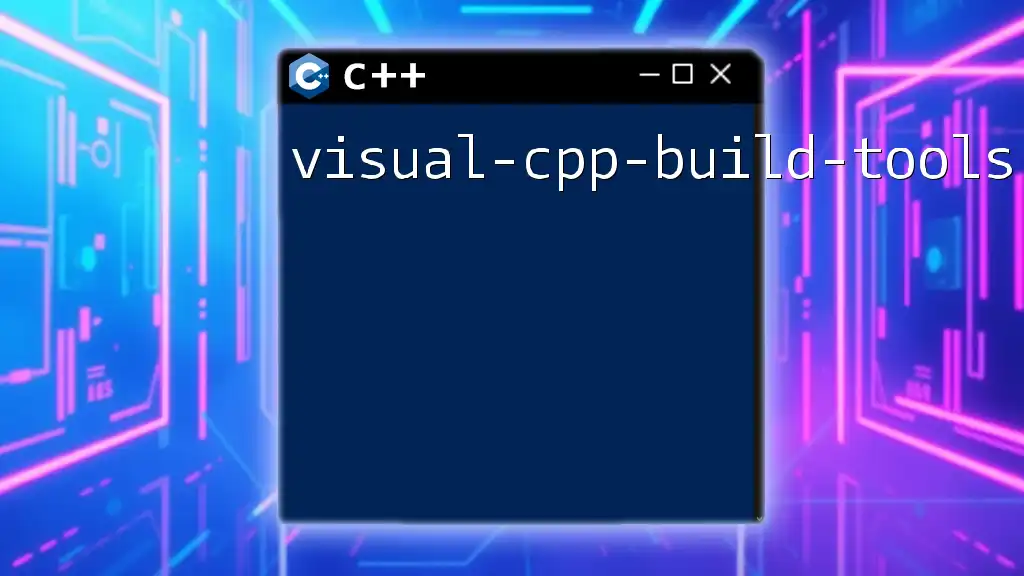
Real-World Applications of C++ Hashmaps
Hashmaps find relevance across various scenarios within software development. Here are key applications:
Use Cases in Software Development
-
Caching Mechanisms: Hashmaps can store results of expensive function calls to enhance performance, allowing you to retrieve results instantly.
-
Counting Frequencies of Elements: For example, in a text processing application, hashmaps can efficiently count occurrences of words.
Case Study: Analyzing Student Grades
Let’s consider a simple example where a hashmap is used to store student grades:
std::unordered_map<std::string, int> studentGrades;
studentGrades["John"] = 85;
studentGrades["Doe"] = 90;
for (const auto& grade : studentGrades) {
std::cout << grade.first << " scored " << grade.second << std::endl;
}
In this case, the hashmap allows quick access and output of each student's grade.
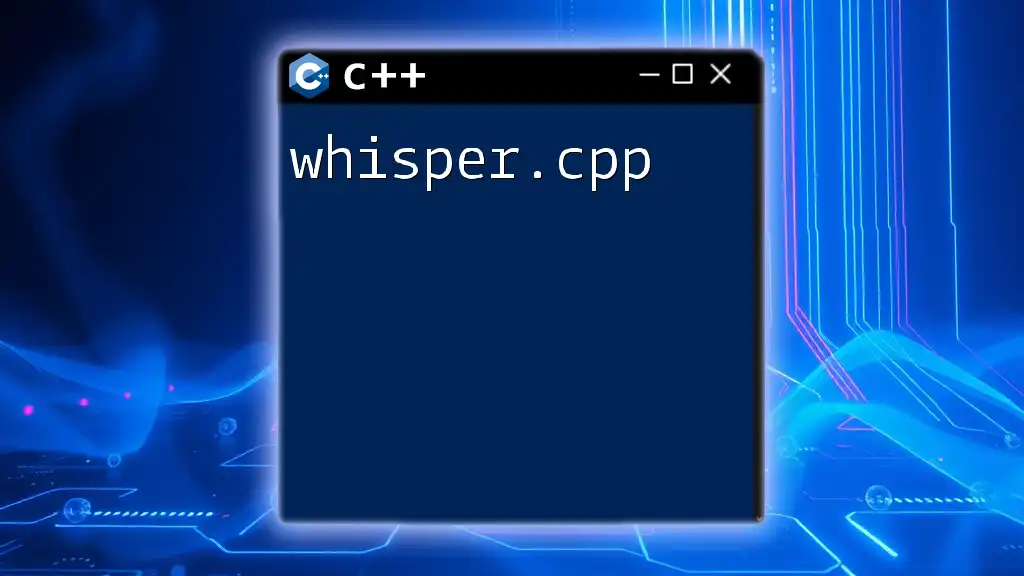
Comparing Hashmaps with Other C++ Data Structures
When it comes to data structure selection, understanding the differences is key:
-
Hashmap vs. Vector: Vectors offer dynamic resizing but require linear search for key-based access, whereas hashmaps provide faster access but use more memory.
-
Hashmap vs. Set: Sets are useful for unique elements and provide no direct key-value association, while hashmaps allow for paired entries with faster retrieval.
-
Hashmap vs. Map: While `std::map` is ordered and has logarithmic access time, `std::unordered_map` delivers average constant time complexity.
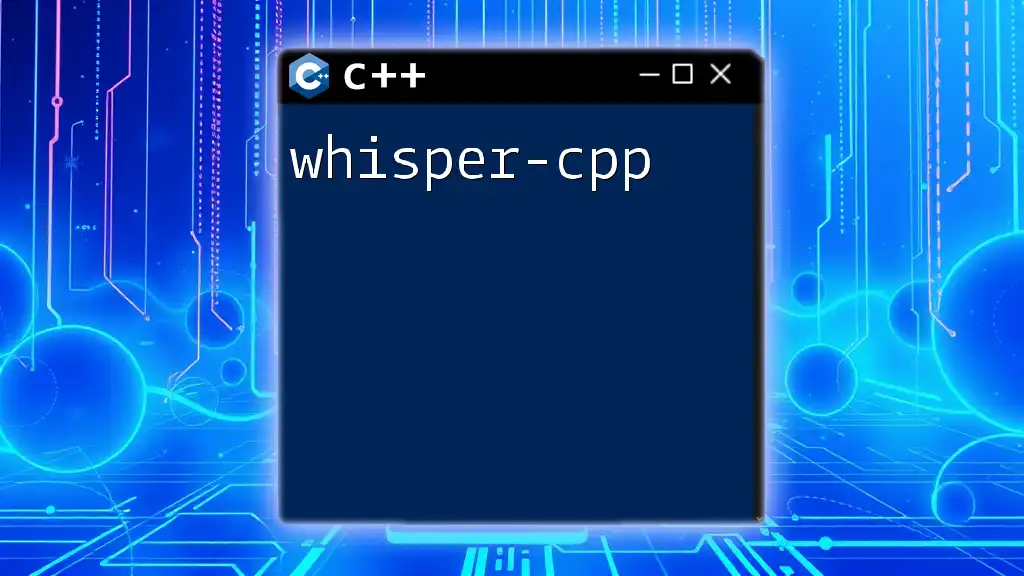
Debugging Tips for C++ Hashmaps
Common Issues
When working with hashmaps, you may encounter:
- Hash collisions, which can slow down the retrieval time.
- Performance issues as the load factor increases, necessitating a rehash.
Tools for Visualization and Testing
Using debugging tools can help you visualize the hashmap's behavior, identify performance bottlenecks, and provide insight into how data is being stored and accessed.
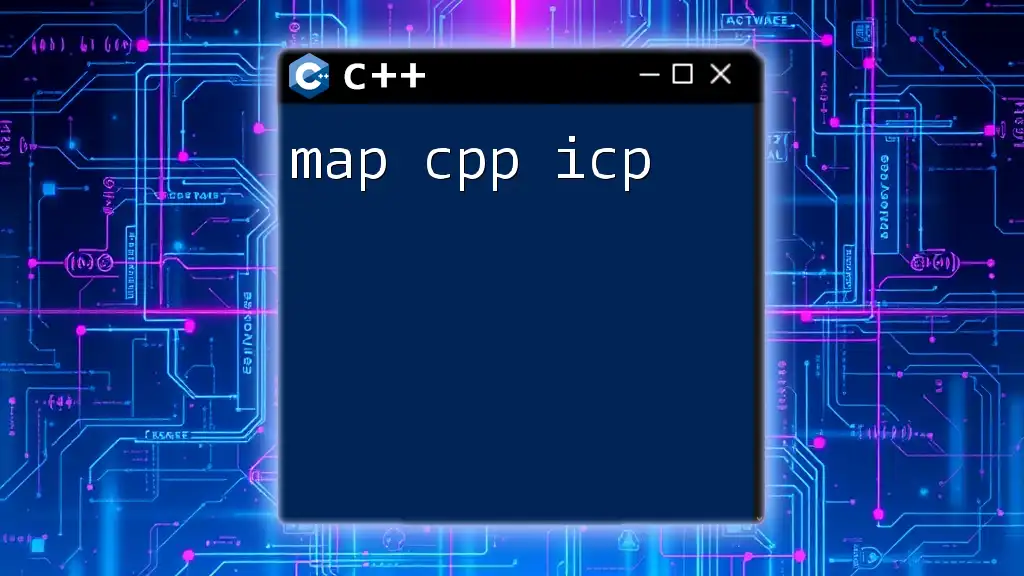
Conclusion
In conclusion, mastering hashmap cpp is a valuable skill in C++ programming. By understanding the concepts, implementation techniques, and best practices, you can effectively leverage this powerful data structure. The speed and efficiency provided by hashmaps open up new avenues for optimized coding practices, making them indispensable in your programming toolkit.
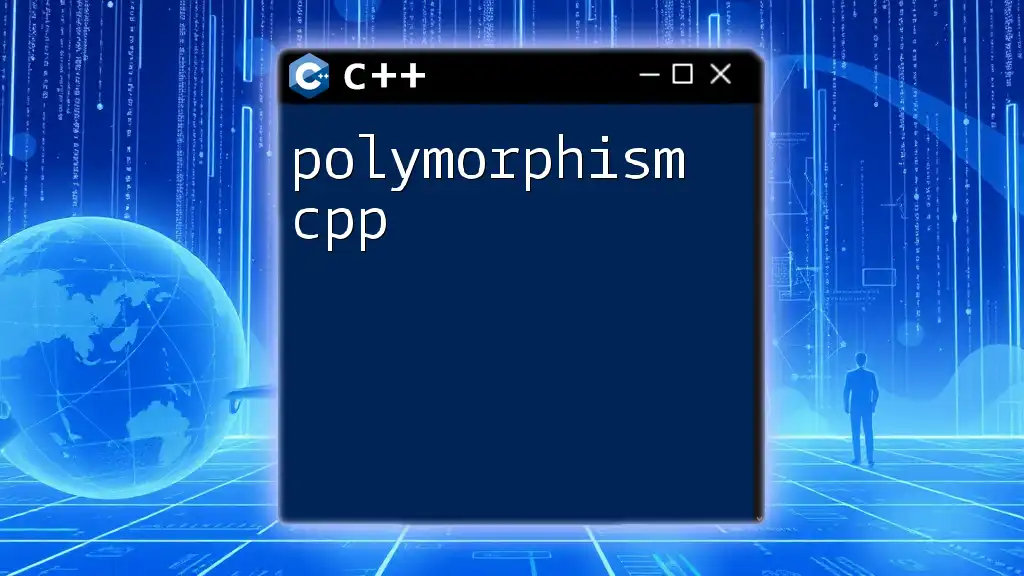
Additional Resources
Consider diving deeper with books, online courses, and useful articles that focus specifically on hashmaps and their applications in C++. Online forums and coding communities can also offer support and additional learning opportunities.
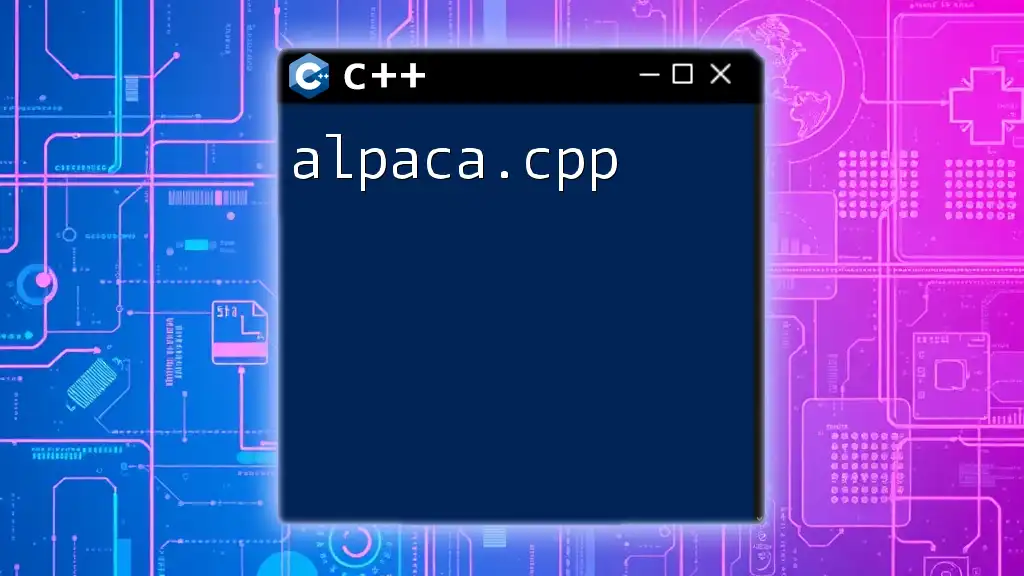
FAQs about C++ Hashmaps
-
What is the difference between `std::unordered_map` and `std::map`?
- `std::unordered_map` offers average constant time complexity for lookup, while `std::map` provides ordered entries with logarithmic complexity.
-
How to choose the right data structure for a specific use case?
- Assess your needs based on access speed, data ordering requirements, and memory usage to select the most appropriate structure.
-
Can Hashmaps store different data types?
- Generally, hashmaps in C++ are designed to work with a single key-value type. However, with structures or variant types, it is possible to store mixed data.