In C++, a `map` is an associative container that stores elements in key-value pairs, allowing for efficient retrieval of values based on their unique keys.
Here’s a simple code snippet demonstrating how to use a `std::map` in C++:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> fruitCount;
fruitCount["apples"] = 5;
fruitCount["bananas"] = 3;
// Accessing and displaying values
for(const auto& pair : fruitCount) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
Understanding Maps in C++
What is a Map?
In C++, a map is an associative container that stores elements in the form of key-value pairs. Each unique key maps to a specific value, allowing for efficient data management and retrieval. Maps are crucial for situations where you need to associate data in a structured way, making them invaluable for numerous programming tasks.
Key Features of Maps
- Associative Container: Maps allow you to access data using a defined key rather than an index. This provides flexibility in data management.
- Ordered Data: Internally, C++ maps use a self-balancing binary search tree (usually Red-Black Tree), which ensures that the keys are always sorted. This characteristic allows for efficient data retrieval.
- Uniqueness of Keys: In a map, each key must be unique. If you attempt to insert a key that already exists, the new value will overwrite the existing value.
Syntax of a Map
To declare a map in C++, you can use the following syntax:
#include <map>
std::map<KeyType, ValueType> myMap;
In this statement, `KeyType` and `ValueType` represent the data types of the keys and values. C++ offers various types of maps, including `std::map`, `std::multimap`, and `std::unordered_map`, each serving different needs regarding key uniqueness and storage efficiency.
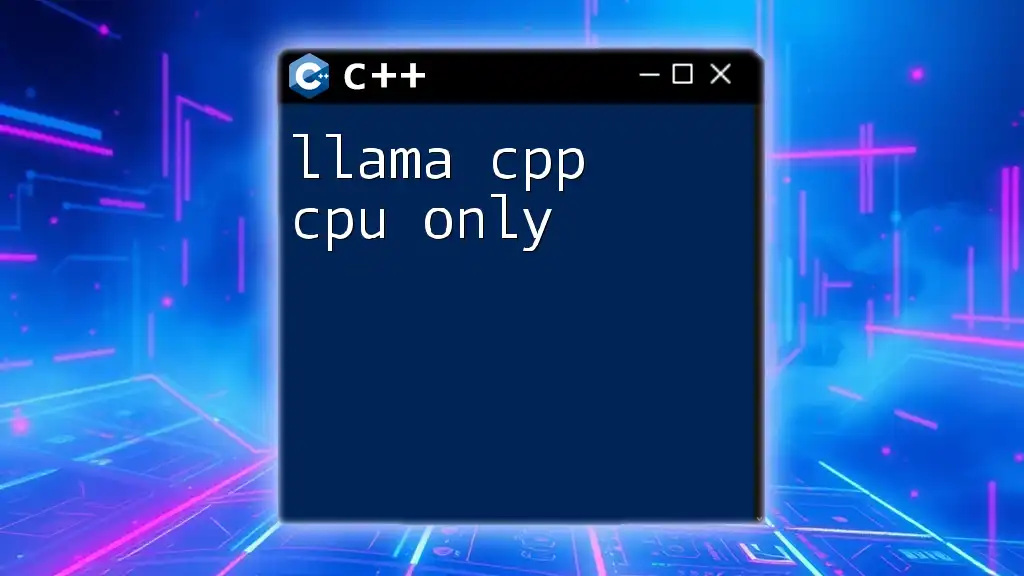
Working with Maps in C++
Inserting Elements
Inserting elements into a map can be accomplished in several ways. The most common method is using the `insert()` function. For example:
myMap.insert(std::pair<KeyType, ValueType>(key, value));
Alternatively, you can use the `[]` operator, which will automatically create a new entry if the key does not exist:
myMap[key] = value;
Accessing Map Elements
Accessing elements in a map is straightforward. You can retrieve a value using its corresponding key as shown here:
ValueType value = myMap[key];
However, care must be taken when accessing keys that may not exist in the map, as doing so can lead to unintended behavior or errors. It’s best to check if the key is present by using the `find()` method or leveraging the iterator.
Iterating Over Maps
To loop through the elements of a map, you can use a range-based for loop with iterators. Here’s how you can do it:
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
This loop goes through each key-value pair in the map and allows you to perform operations on them as required.
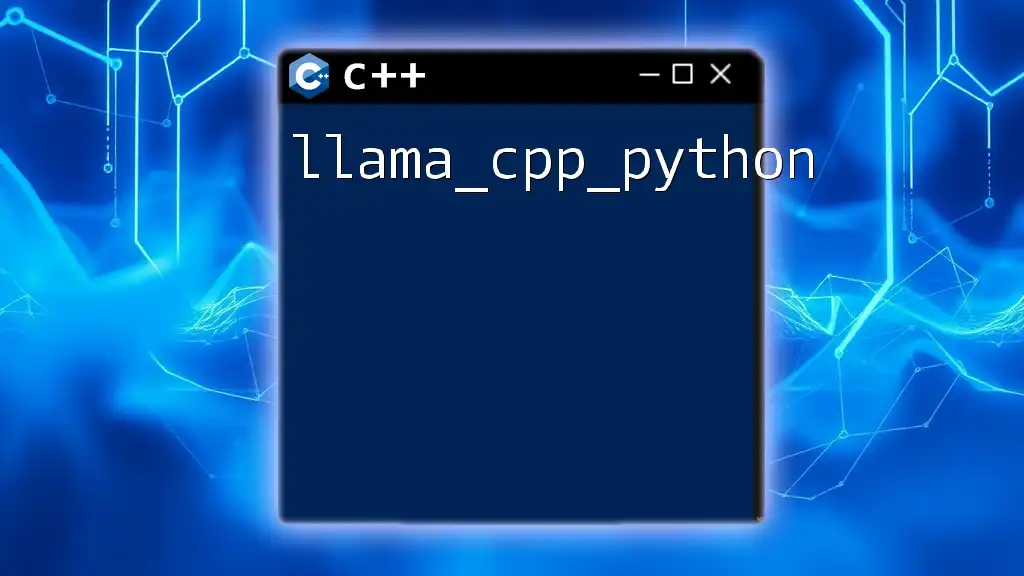
Introduction to ICP (Iterative Closest Point)
What is ICP?
Iterative Closest Point (ICP) is an algorithm used extensively in computer vision and robotics for aligning 3D models or point clouds. It works by iteratively minimizing the difference between two sets of points, generally through assessing the distances between points in one point cloud to their nearest counterparts in another.
The Role of Maps in ICP
In the context of ICP, maps become particularly useful for efficiently searching and linking corresponding points. Using maps allows you to store point correspondences, which is essential for accurate transformations required to align the datasets.
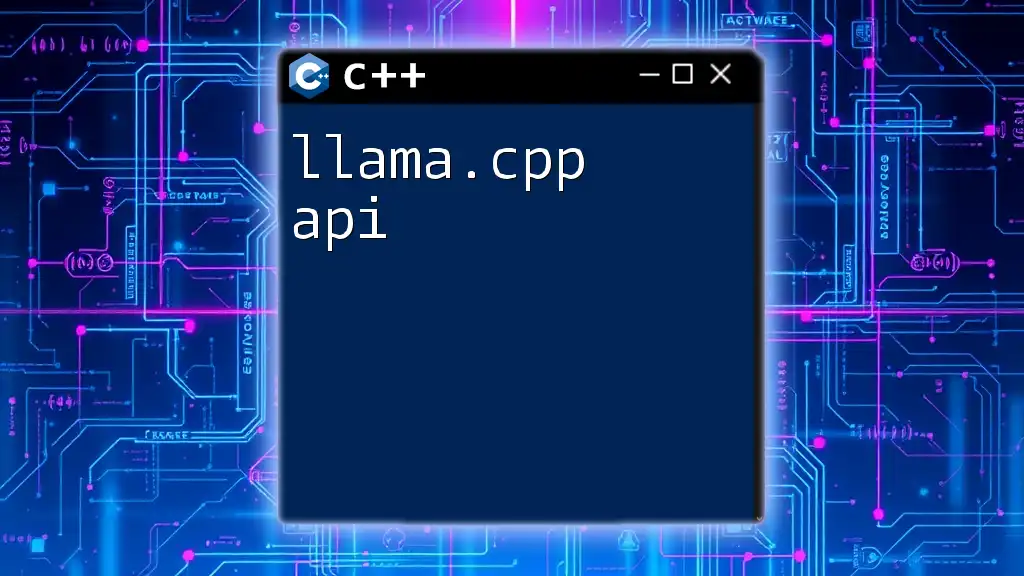
Implementing Map with ICP
Steps to Implement ICP with Maps
The ICP algorithm can be implemented using maps through the following steps:
- Initialize source and target point clouds.
- Create a map to store the nearest point correspondences.
- Iteratively find the nearest points and update the map.
- Apply the transformations based on the map entries and refine until convergence.
Example Code Snippet
Here is a simple example of how maps can be used within the ICP framework to map points from a source to a target point cloud:
std::map<Point3D, Point3D> pointMap;
// Assume Point3D is a struct representing 3D point coordinates
for (const auto& sourcePoint : sourceCloud) {
Point3D nearestPoint = findNearestPoint(sourcePoint, targetCloud);
pointMap[sourcePoint] = nearestPoint;
}
Key Functions in ICP
Within the ICP algorithm, certain functions facilitate the use of maps for nearest neighbor searches and mapping points. Here’s an example that shows how you might update your map with corresponding points:
void updateMap(std::map<Point3D, Point3D>& myMap, Point3D source, Point3D target) {
myMap[source] = target; // Map source point to its nearest target
}
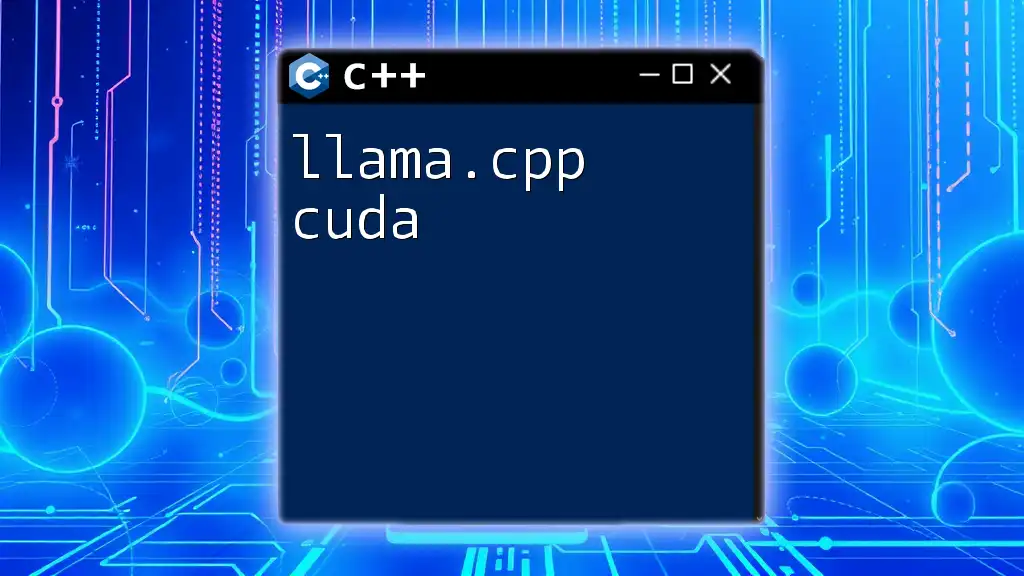
Optimizing the Use of Maps in ICP
Performance Considerations
When implementing maps in ICP, it’s crucial to consider performance. Maps, particularly `std::map`, have a logarithmic time complexity for insertions, deletions, and look-ups. However, if frequent access and search operations are required, `std::unordered_map` may be more suitable due to its average constant time complexity.
Tips for Efficiency
To improve the efficiency of maps within your ICP implementation, consider the following tips:
- Use unordered_map for faster lookups when key order is not a priority.
- Minimize redundant computations by caching results in the map.
- Regularly assess the size of your map to manage memory effectively.
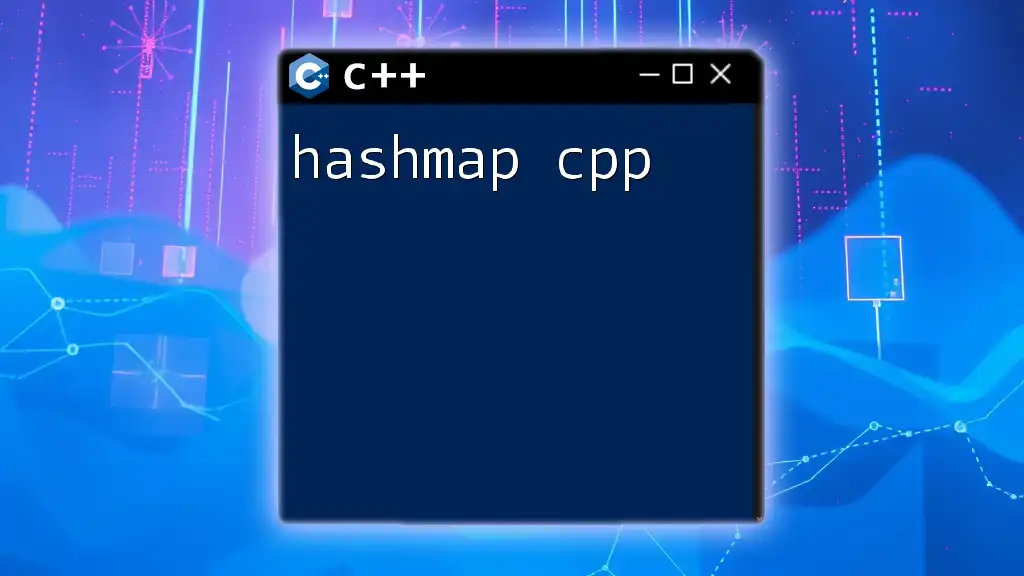
Conclusion
Combining maps in C++ with ICP offers a robust strategy for managing complex data relationships essential for accurate 3D point cloud registration. By leveraging the strengths of C++ maps, you can enhance the efficiency of your ICP implementations, making them more effective and manageable.
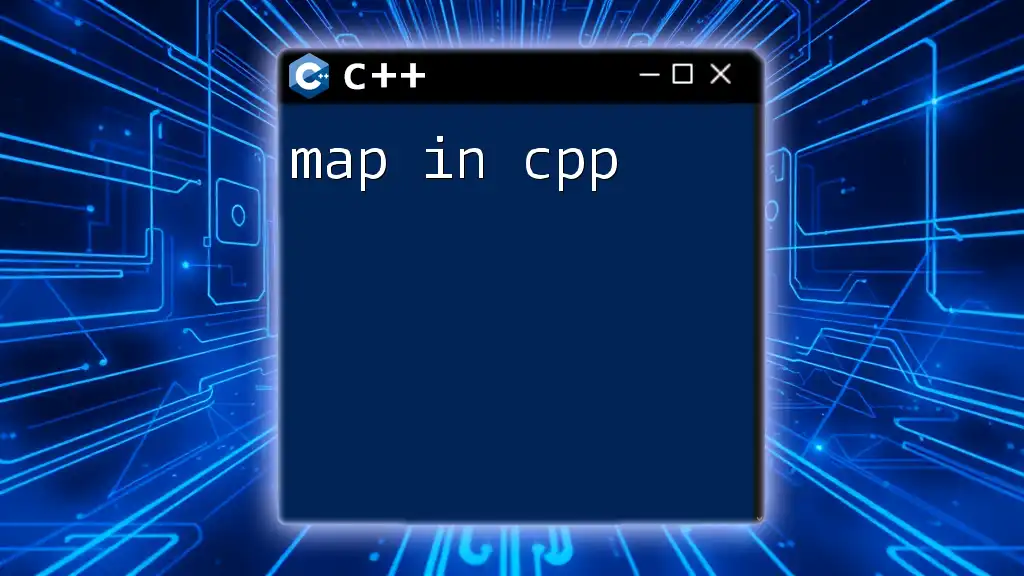
Further Resources
For those interested in diving deeper into the world of C++ maps and ICP, several resources are available, including specialized books, online tutorials, and courses focusing on these topics in detail.
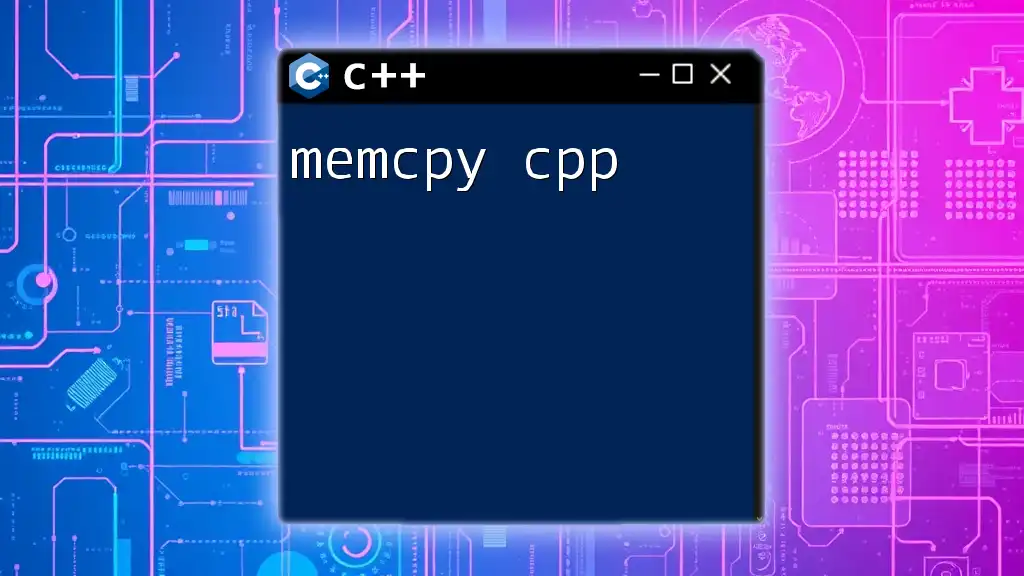
Call to Action
We invite you to take your understanding of C++ to the next level by experimenting with maps and the ICP algorithm. Share your experiences, ask questions, and don’t forget to check back for more concise tutorials tailored to boost your C++ skills.