The `llama.cpp` OpenAI API allows developers to efficiently interact with the LLaMA (Large Language Model Meta AI) models using concise C++ commands.
Here’s a simple code snippet demonstrating how to call an example API function:
#include <iostream>
#include "llama.cpp" // Assume llama.cpp has relevant API functions
int main() {
LlamaAPI api;
std::string response = api.call("What is the capital of France?");
std::cout << response << std::endl; // Output: Paris
return 0;
}
What is OpenAI API?
Understanding APIs in Programming
APIs, or Application Programming Interfaces, allow different software applications to communicate with each other. They act as a bridge between the front-end and back-end components, allowing developers to interact with complex systems using simple commands.
Key Terms:
- REST: A common architectural style for designing networked applications.
- HTTP requests: The method used to communicate with APIs.
- JSON: A lightweight format used for data interchange.
Overview of OpenAI API
OpenAI API provides developers access to powerful machine learning models, enabling them to implement advanced features such as natural language processing, image recognition, and more within their applications. Some popular use cases include:
- Creating chatbots that can lead human-like conversations.
- Automating content creation for blogs and social media.
- Enhancing user experiences in various applications by providing intelligent responses.
The OpenAI API supports multiple programming languages and platforms, making it versatile for various development environments.
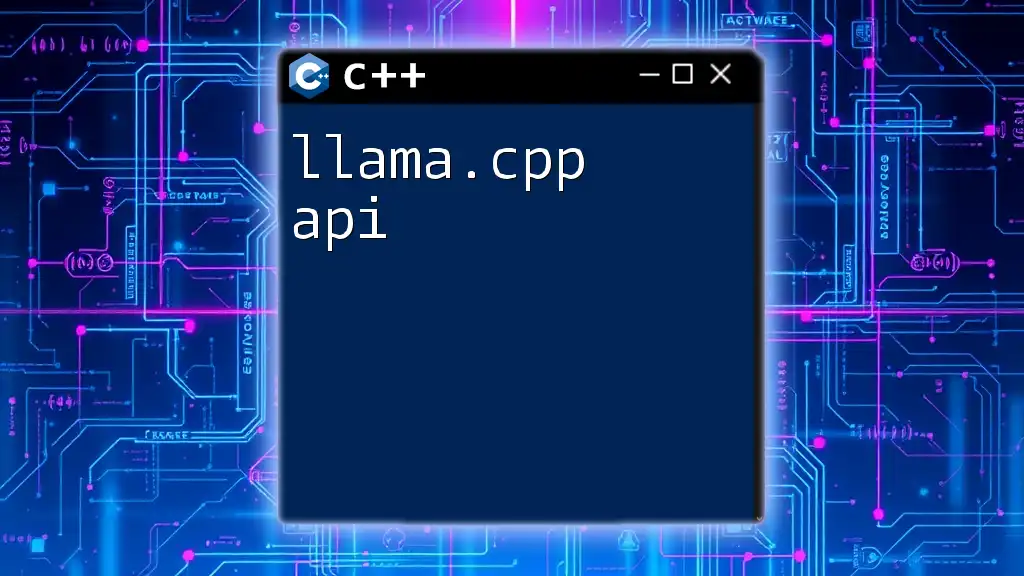
Introduction to llama.cpp
What is llama.cpp?
`llama.cpp` is a specialized library designed to simplify interactions with the OpenAI API using C++. Its main purpose is to streamline API calls, making it easier for developers to harness the power of OpenAI’s models without getting bogged down in the technical details.
Benefits of Using llama.cpp
The benefits of using `llama.cpp` include:
- Ease of Use: With its straightforward syntax, developers can start making API calls quickly without extensive setup.
- Performance Benefits: By optimizing requests and managing responses efficiently, `llama.cpp` enhances the overall speed of data retrieval.
- Integration with Existing C++ Projects: It is designed to be seamlessly integrated into current C++ projects, allowing for immediate functionality.
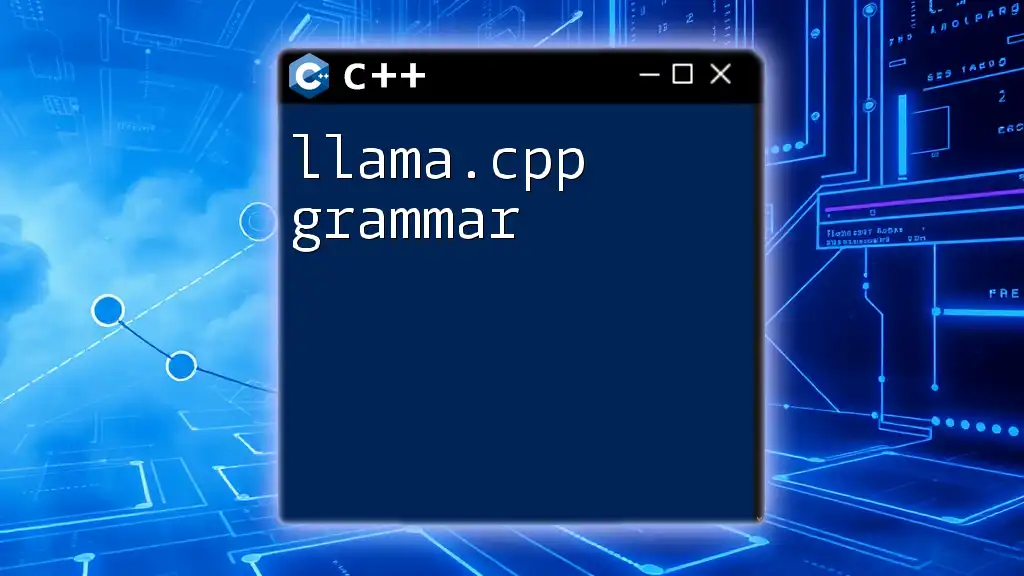
Getting Started with llama.cpp
Prerequisites
Before diving into using `llama.cpp`, ensure you have the following:
- A basic understanding of C++ programming.
- An installed C++ compiler (like g++).
- Access to the internet to communicate with OpenAI's servers.
To get started with `llama.cpp`, follow these installation instructions:
- Install llama.cpp: Clone the repository or use a package manager if available.
- Dependencies: Ensure you have any required libraries, which will generally be documented in the repository.
Basic Structure of llama.cpp Code
Once you have installed `llama.cpp`, it's essential to set up the code structure. Begin with the basic include statements and initialization:
#include <iostream>
#include "llama_api.h"
int main() {
// Your initialization code here
return 0;
}
This serves as a simple entry point for your application, indicating where the execution will start.
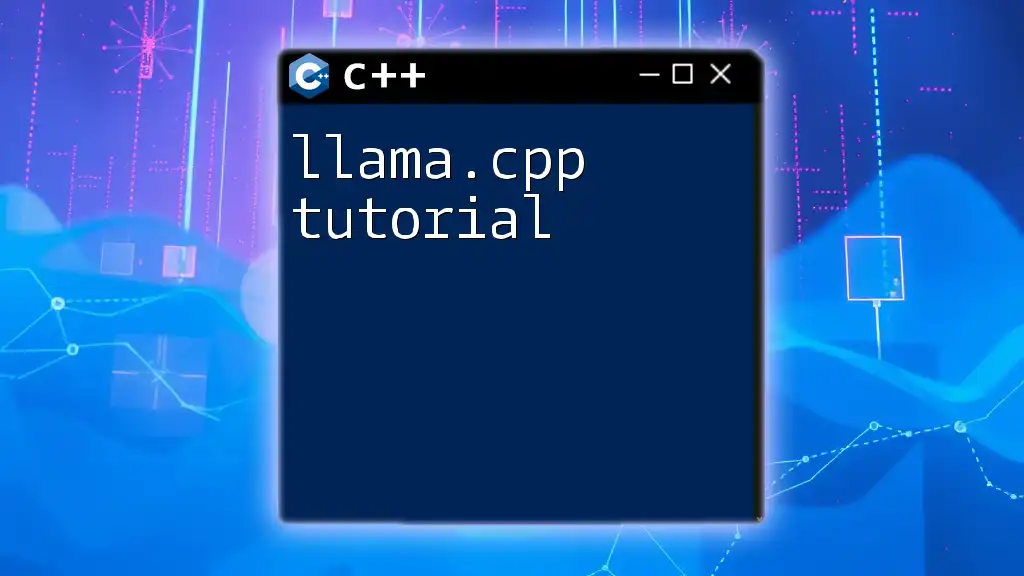
Making Your First API Call
Setting Up the API Key
To communicate with the OpenAI API, you'll need a valid API key. Here’s how you can obtain and securely store your API key:
- How to Obtain: Sign up on the OpenAI website and create your API key from the dashboard.
- Store Securely: Store your API key in a secure way, ensuring it isn't hard-coded in your main script.
Here’s how you can define your API key in your code:
const std::string API_KEY = "your_api_key_here";
Crafting Your First Request
With your API key in place, you can now create your first request to the OpenAI API. Understanding the request structure is crucial:
auto response = llama_api::callOpenAI(API_KEY, "Hello, how do I use OpenAI API with llama.cpp?");
std::cout << response << std::endl;
In this code snippet, you are calling the OpenAI API with a greeting string, and the response is printed to the console.
Understanding the Response
After making a request, you'll receive a response in JSON format. Here’s an example JSON response:
{
"id": "1",
"object": "text_completion",
"created": 1622007126,
"choices": [
{
"text": "You can use the OpenAI API with llama.cpp...",
"index": 0,
"logprobs": null,
"finish_reason": "stop"
}
]
}
Take note of the key fields within this response, such as `text`, which contains the generated content.
Error Handling and Debugging
Errors can occur during your API calls, so it's vital to implement error handling strategies. Ensure you check the status of your response and provide user-friendly feedback when something goes wrong.
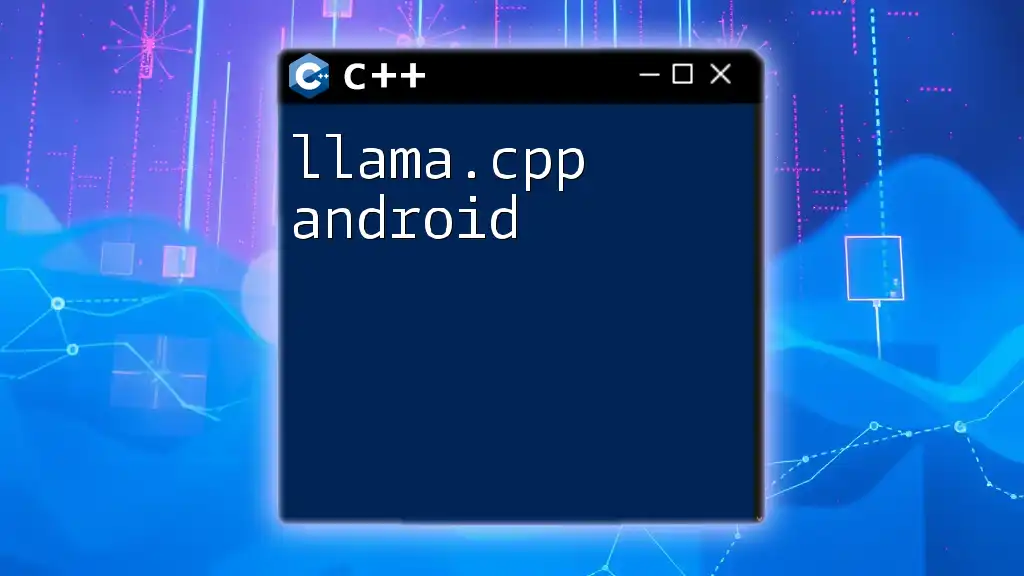
Advanced Features of llama.cpp
Customizing the API Requests
One of the strengths of `llama.cpp` is its ability to customize API requests. You can modify several parameters to optimize your interactions with the OpenAI API, including temperature, max tokens, and more.
For example, to set a custom temperature and token limit, you can do this:
llama_api::Request request;
request.setTemperature(0.7);
request.setMaxTokens(100);
auto response = llama_api::callOpenAI(API_KEY, request);
This customization can lead to more controlled and relevant responses depending on your application’s needs.
Optimizing Performance
To ensure efficient code execution, consider these optimization tips:
- Batch requests if possible to minimize network overhead.
- Use efficient data structures to handle responses, which helps in faster parsing and processing of data.
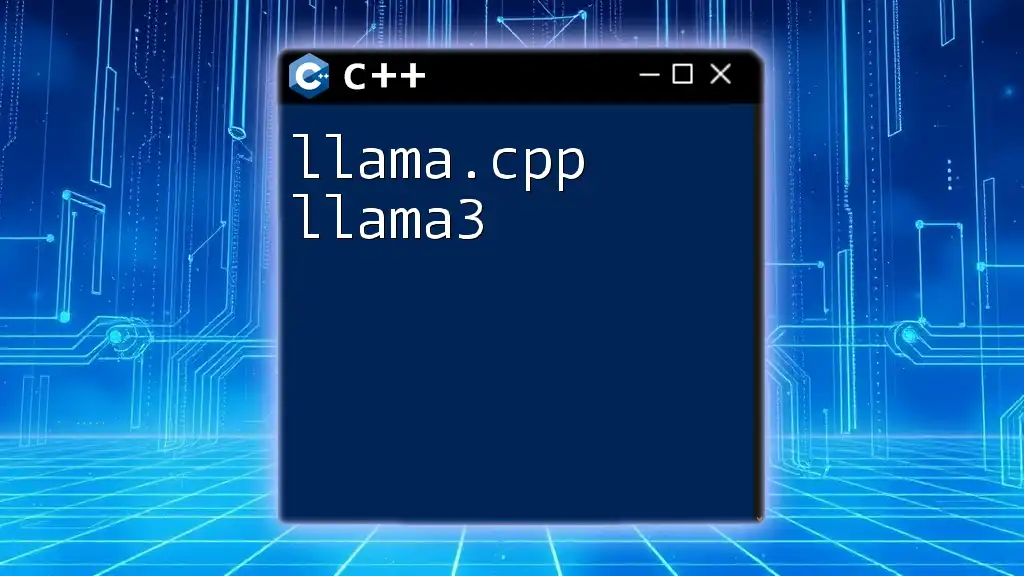
Best Practices for Using OpenAI API with llama.cpp
Handling Rate Limits
OpenAI imposes rate limits on API usage, which can affect application performance. To effectively manage these limitations:
- Understand the Limits: Familiarize yourself with the limits set by OpenAI.
- Implement Backoff Strategies: If you receive a rate limit error, implement a backoff strategy to retry requests after a delay.
Real-World Applications
The powerful combination of `llama.cpp` and the OpenAI API paves the way for innovative applications across various industries. Some real-world examples include:
- Chatbots: Enable businesses to automate customer service using intelligent dialogue systems.
- Content Generation: Tools that help marketers create engaging copy for websites, social media, or advertisements.
- Personalized Recommendations: Applications that analyze user preferences and suggest tailored content.
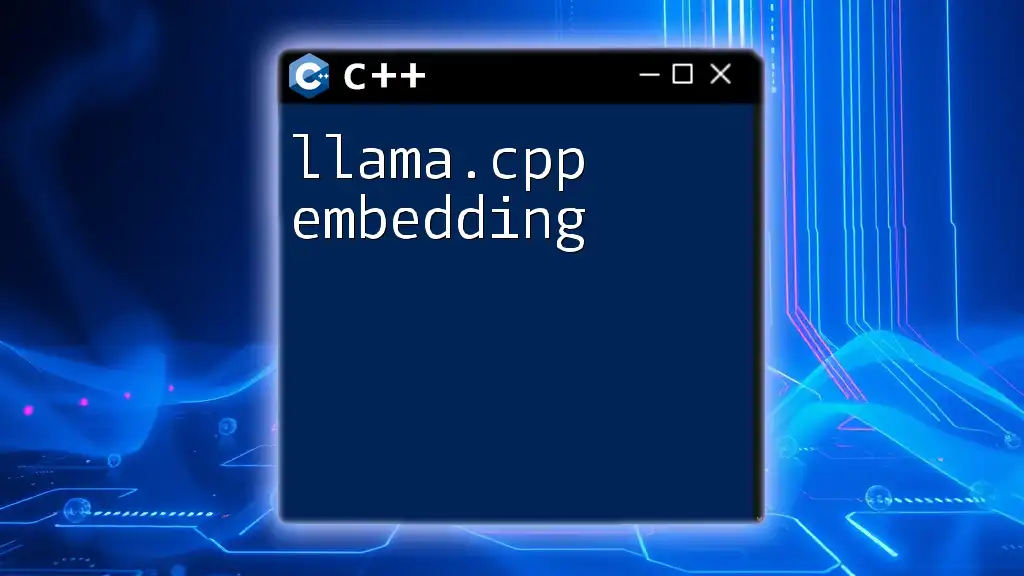
Conclusion
In conclusion, utilizing the llama.cpp openai api opens a door to leveraging advanced AI capabilities via C++. By simplifying the complexities of API interactions, it allows developers to create robust applications seamlessly. Whether you're creating sophisticated chatbots or enhancing existing features, `llama.cpp` provides an excellent foundation to build upon.
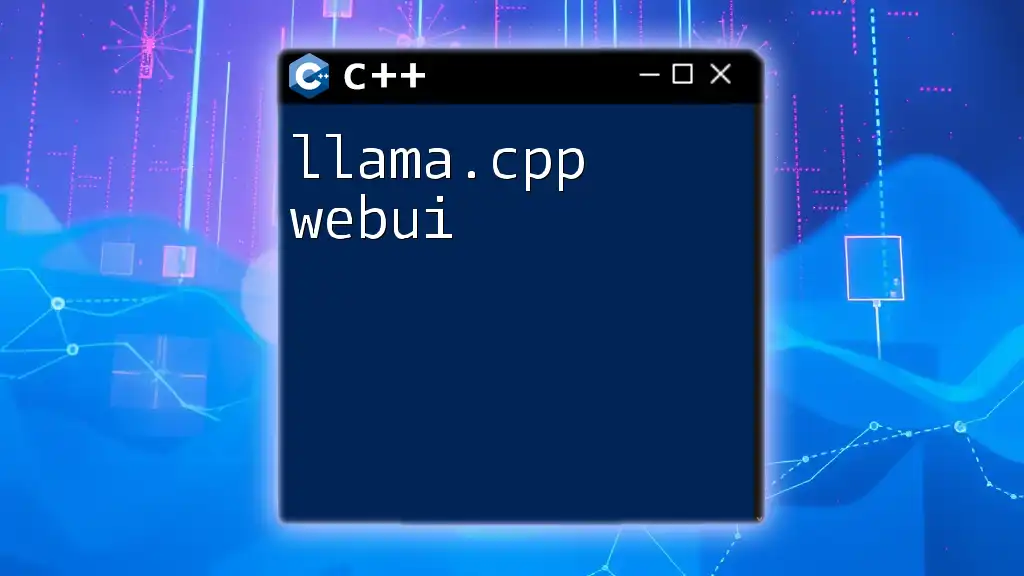
Additional Resources
For further learning and exploration, check out the official documentation of the OpenAI API and the `llama.cpp` GitHub repository for community support, sample code, and best practices. Join relevant forums or online groups to connect with fellow developers to share experiences and enrich your skills in this exciting area of technology.