The `llama.cpp` GUI is an intuitive interface that simplifies the execution of C++ commands, enabling users to efficiently interact with the LLaMA language model.
Here’s a simple example to demonstrate how to initialize a model using `llama.cpp`:
#include "llama.h"
int main() {
LlamaModel model("path/to/model/file");
model.initialize();
model.run();
return 0;
}
Understanding Llama.cpp
What is Llama.cpp?
Llama.cpp is a powerful C++ library designed to facilitate efficient programming with an emphasis on ease of use, particularly in the development of GUI applications. Offering a variety of rich features, Llama.cpp allows developers to focus more on creativity and less on complex coding syntax. It stands out due to its simplicity and versatile functionality, making it an excellent choice for both beginners and seasoned programmers looking for rapid prototyping.
Installing Llama.cpp
To harness the power of Llama.cpp, you first need to install it. Here’s how you can get started:
Step-by-Step Installation Guide:
- System Requirements: Ensure you have a C++ compiler (like g++) and an IDE that supports C++ development.
- Downloading Llama.cpp: You can obtain the latest version of Llama.cpp from the official repository (insert URL here).
- Installation Commands: Open your terminal and run the following commands:
git clone https://github.com/username/llama.cpp.git
cd llama.cpp
make install
- Common Installation Issues: If you encounter issues such as missing dependencies, ensure all required libraries are installed. Refer to the documentation or community forums for troubleshooting tips.
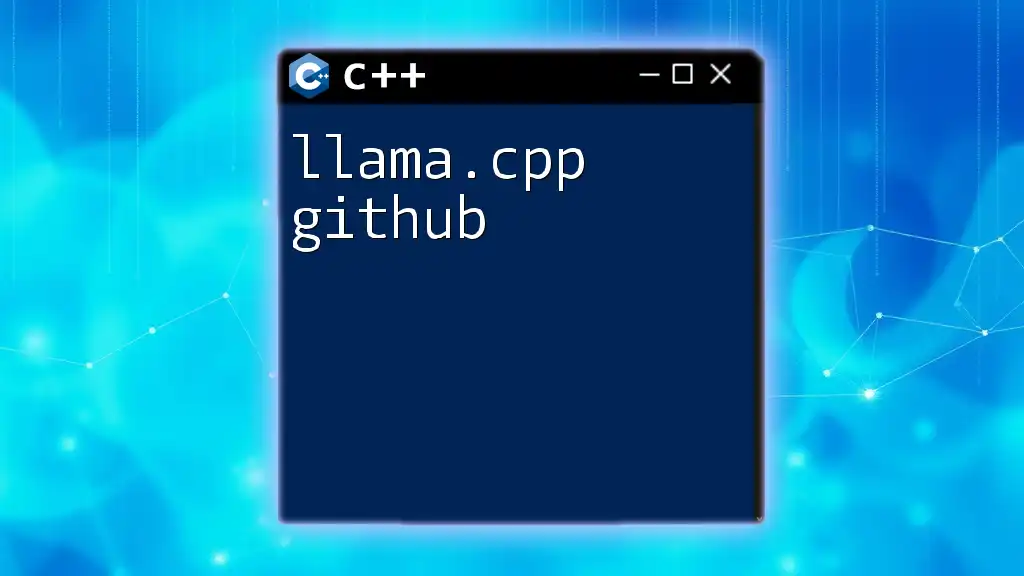
Getting Started with GUI Development
Basics of GUI Programming in C++
GUI programming in C++ involves creating graphical interfaces that users interact with through windows, buttons, and forms. It centers around event-driven programming, where the application responds to user actions.
Creating an engaging user experience (UX) is crucial for any application. It’s essential to keep a user-centric approach while designing your GUI, ensuring functionalities are intuitive and straightforward.
Setting Up Your First GUI with Llama.cpp
Creating your first GUI application with Llama.cpp is straightforward. Here’s how you can do it:
Creating a Simple Llama.cpp Application: Start a new project directory and create a main.cpp file. In this file, initialize a window and add components like buttons and labels as follows:
#include <llama/gui.h>
int main() {
Llama::GUI gui; // Initialize GUI framework
gui.createWindow("My First Llama.cpp GUI Application", 800, 600); // Create a window
Llama::Button button("Click Me"); // Create a button
button.setPosition(100, 100); // Position button
gui.addWidget(button); // Add button to window
gui.run(); // Start the GUI loop
return 0;
}
This code initializes a simple GUI application with a window and a button. You can expand this by adding more UI elements to enhance interactivity.
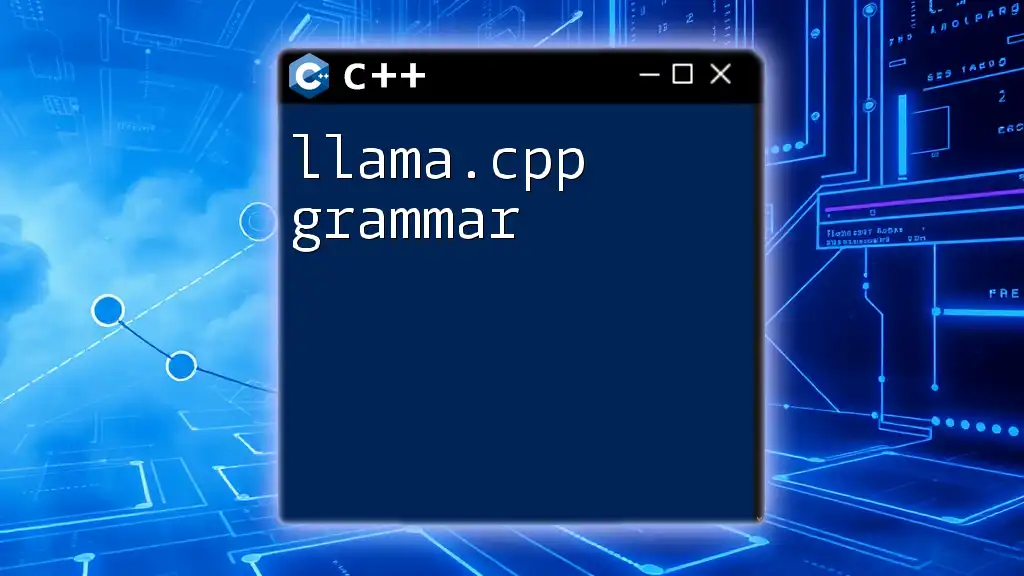
Llama.cpp GUI Features
Key Components of Llama.cpp GUI
Widgets in Llama.cpp consist of various interactive elements that make your GUI functional and interactive. Common widgets include labels, buttons, and text fields.
Here’s how to create and customize widgets:
Llama::Label label("Hello, Llama!"); // Create a label
label.setFontSize(20); // Set font size
label.setColor(Llama::Color::Blue); // Set text color
gui.addWidget(label); // Add label to window
Layouts and Design play a crucial role in arranging your GUI components effectively. Llama.cpp supports various layout types:
Llama::GridLayout layout; // Create a grid layout
layout.addWidget(button, 0, 0); // Add widget at (0, 0)
layout.addWidget(label, 0, 1); // Add label at (0, 1)
gui.setLayout(layout); // Assign layout to GUI
These snippets demonstrate how to create structured and organized designs, enhancing usability.
Advanced GUI Features
Event Handling in Llama.cpp allows the application to respond to user interactions. To implement a button click event, you can use:
button.onClick([]() {
std::cout << "Button clicked!" << std::endl; // Print message on click
});
This enhances interactivity, providing immediate feedback to user actions.
Styling Your Application is essential for branding and aesthetics. Customizing the appearance of widgets can significantly impact user engagement. Here’s a snippet to change the style of a button:
button.setBackgroundColor(Llama::Color::Green); // Set background color
button.setBorderRadius(10); // Add rounded corners
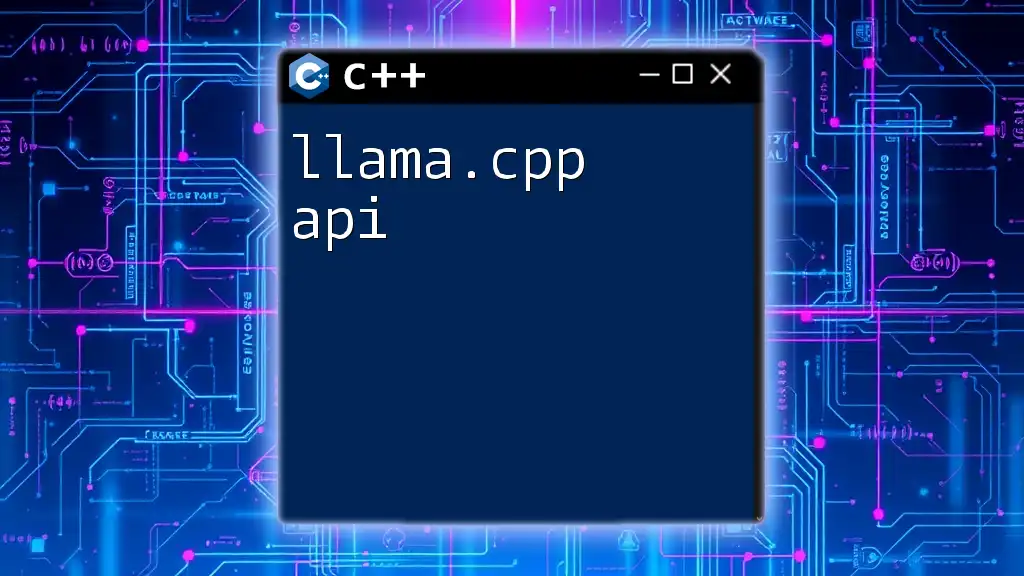
Enhancing the User Experience
Incorporating Multimedia Elements
Incorporating multimedia elements breathes life into your application. For example, to load and display an image, you might use:
Llama::Image image("path/to/image.png");
gui.addImage(image); // Add image to the GUI
Adding multimedia attributes not only enhances aesthetics but also improves user engagement through richer content.
Accessibility and User-Friendliness
Designing for Accessibility is not just a compliance issue; it's about reaching a broader audience. Consider color contrast, font sizes, and navigational aids to ensure that all users, including those with disabilities, can use your application.
Tips for Making Your Llama.cpp GUI More User-Friendly:
- Provide clear labels and buttons.
- Use tooltips to offer context-sensitive help.
- Implement keyboard shortcuts for quicker navigation.
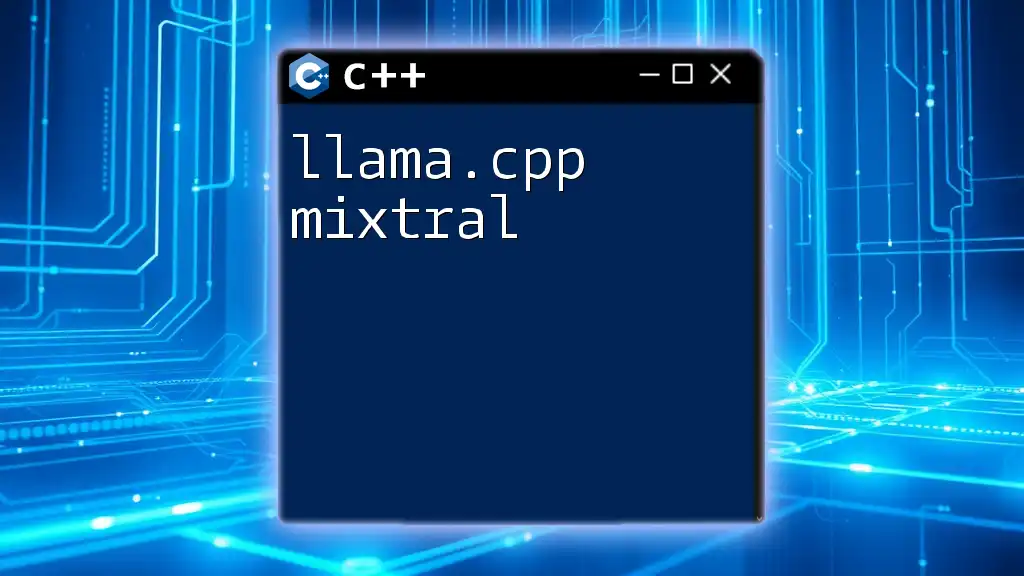
Deploying Your Llama.cpp GUI Application
Packaging Your Application
For packaging your application for distribution, utilize tools like CMake or Bundler. The process helps ensure that your application includes all necessary dependencies, making it easy for users to install and run without additional setup.
Cross-Platform Compatibility
To ensure your application runs smoothly across different operating systems, conduct thorough testing. Use conditional compilation techniques in C++ to handle OS-specific functionalities seamlessly:
#ifdef _WIN32
// Windows specific code here
#else
// Code for other operating systems
#endif
This approach allows your application to maintain functionality no matter the user's platform.
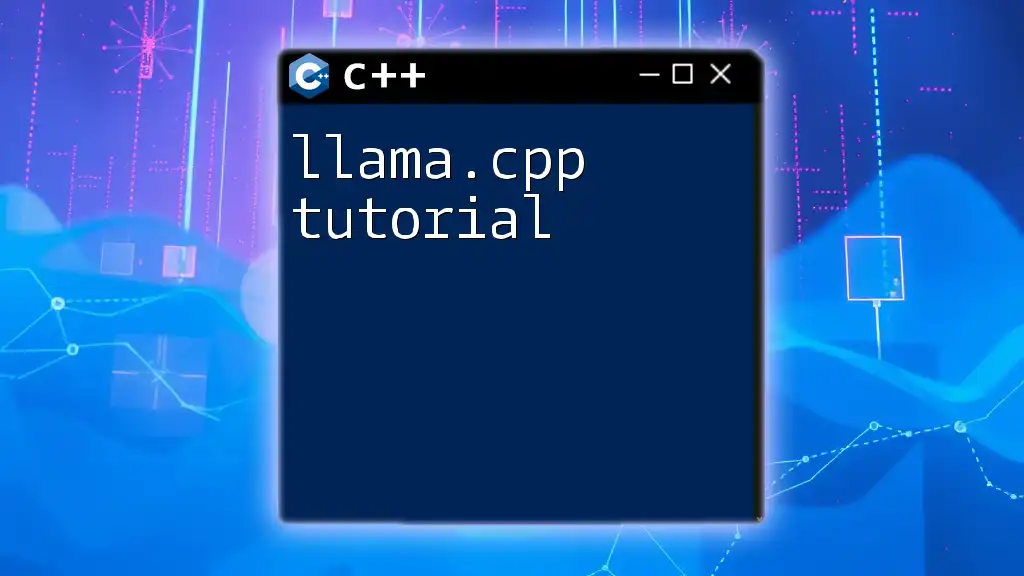
Best Practices in Llama.cpp GUI Development
Developing with Llama.cpp requires attention to detail and adherence to best practices. Code organization and maintainability are crucial. Structure your files clearly, using separate files for different components, enhancing readability.
Documentation is vital—not just for you but also for anyone who might work on the project in the future. Provide clear comments and maintain a README file that outlines how to run the application.
During development, efficient debugging strategies can save you time. Utilize debugging tools and verbose error messages to quickly identify and rectify issues.
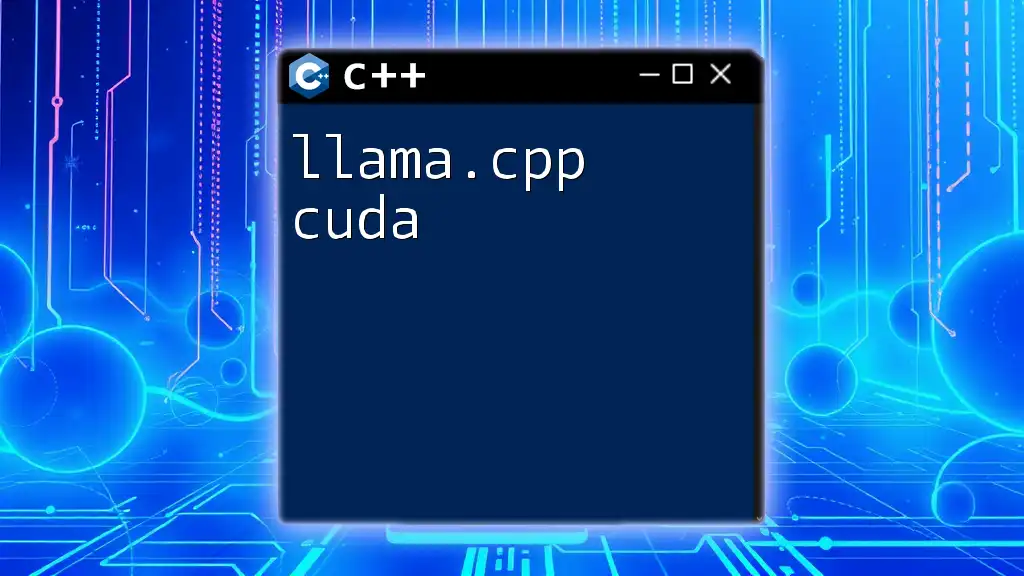
Conclusion
Using Llama.cpp for GUI development can significantly streamline the process, making it accessible to developers at all levels. The library’s intuitive design and robust features allow for creativity and innovation in C++ development. Explore Llama.cpp and harness its functionalities to create engaging and powerful GUI applications.
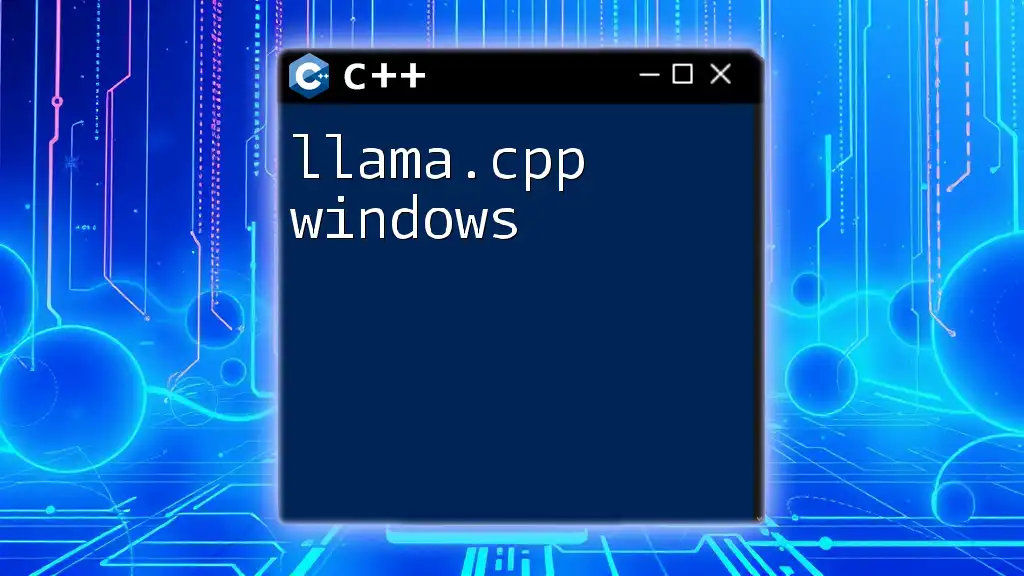
Call to Action
Have you started building your projects using Llama.cpp GUI? Share your creations, experiences, and queries with the community! Engage in forums and explore additional resources to further deepen your understanding of C++ GUI development.