"llama.cpp fine-tune" refers to the process of optimizing the LLaMA (Large Language Model Meta AI) model using specific command-line options available in the `llama.cpp` library to adapt the model for your particular dataset or task.
Here's a simple code snippet demonstrating the fine-tuning command in a basic context:
./llama.cpp --fine-tune --model-path path/to/your/model --data-path path/to/your/dataset
Understanding Llama.cpp
What is Llama.cpp?
Llama.cpp is a versatile library tailored for optimizing C++ programs, particularly in the context of machine learning. It enables developers to leverage advanced algorithms and techniques effortlessly. With its clean interface and robust performance, Llama.cpp streamlines the complexities of building and fine-tuning models, allowing users to focus on innovation rather than intricate implementation details.
Importance of Fine-Tuning in Llama.cpp
Fine-tuning is the process of adjusting a pre-trained model on new data to enhance its performance on a specific task. In the case of Llama.cpp, fine-tuning can significantly improve model accuracy and reduce training time. By customizing hyperparameters and leveraging unique datasets, developers can ensure that their models perform optimally for the intended application, thereby maximizing efficiency and effectiveness.
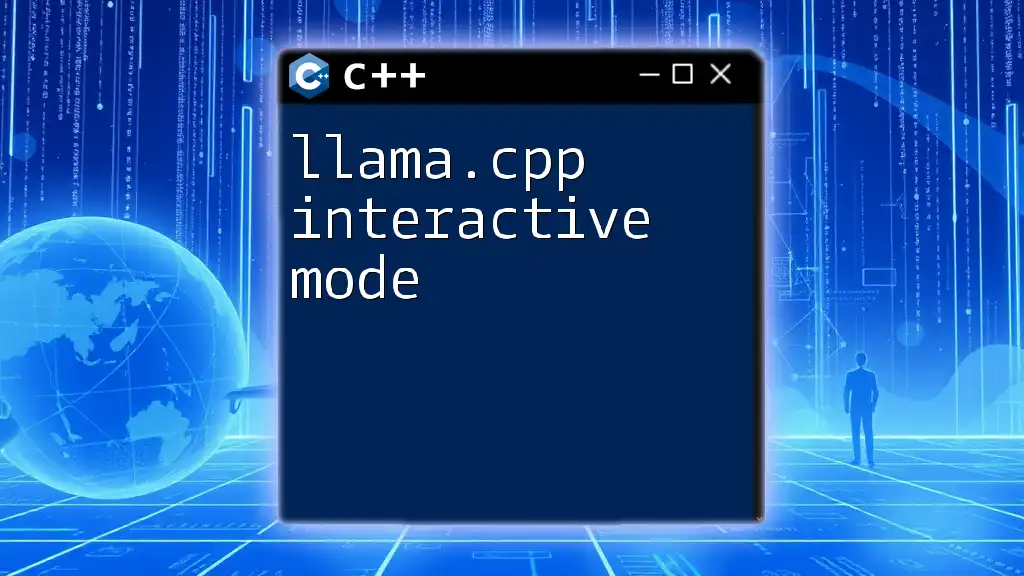
Getting Started with Llama.cpp Fine Tuning
Prerequisites
Before diving into the llama.cpp fine tune process, it's essential to have the right tools and knowledge. Ensure you have:
- A basic understanding of C++ programming.
- Familiarity with machine learning concepts.
- Access to a machine with sufficient resources (CPU/GPU) for model training.
Setting Up Your Environment
To start working with Llama.cpp, follow these installation steps:
-
Installation of Llama.cpp: You can typically install Llama.cpp via a package manager like `vcpkg` or compile it from source. For systems like Linux, use the command:
git clone https://github.com/your-repo/llama.cpp.git cd llama.cpp mkdir build && cd build cmake .. make
-
Configuring Dependencies: Make sure to install any required libraries, such as `Eigen` or `OpenBLAS`. These libraries are essential for matrix operations and optimized calculations.
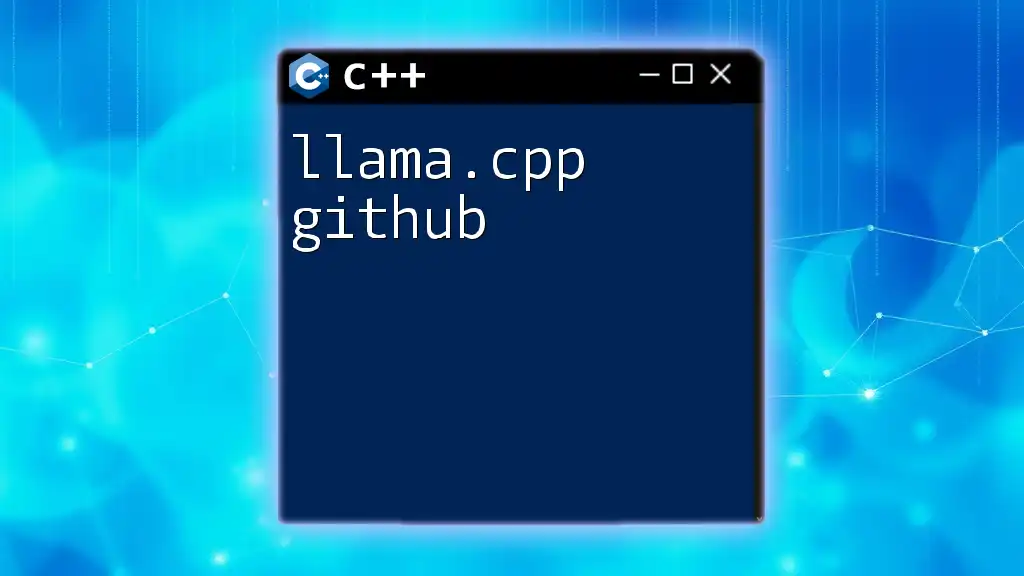
The Fine-Tuning Process
Data Preparation
Quality data is crucial for effective fine-tuning. Start by collecting relevant datasets that reflect the specific tasks you want your model to excel in. Preprocess the data to ensure it's clean and formatted correctly. Here’s a simple preprocessing pipeline outline:
- Loading Data: Use libraries like `pandas` to load and manipulate your datasets.
- Cleaning Data: Remove any inconsistencies or irrelevant information.
- Transforming Data: Normalize and scale your data for better convergence during training.
For example, a basic C++ snippet to handle data loading might look like:
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
std::vector<std::string> loadDataset(const std::string& filepath) {
std::vector<std::string> data;
std::ifstream file(filepath);
std::string line;
while (std::getline(file, line)) {
data.push_back(line);
}
return data;
}
Fine-Tuning Techniques
Learning Rate Adjustment
Adjusting the learning rate is a vital aspect of fine-tuning. A learning rate that is too high may lead to convergence issues, while one that is too low can slow down the training process. For instance, you could experiment with values like 0.01 or 0.0001 to find the optimal setting for your task.
Batch Size Considerations
Batch size plays a crucial role in model training. A larger batch size can lead to faster training times but may require more memory, while a smaller batch size can add noise but can also provide better generalization. Consider testing different batch sizes to determine what works best for your specific model and dataset. You can adjust the batch size like this:
int batch_size = 32; // Example batch size
Regularization Methods
Employing regularization techniques can help prevent overfitting. Some common methods include:
- L1 Regularization: Adds a penalty equal to the absolute value of the magnitude of coefficients.
- L2 Regularization: Adds a penalty equal to the square of the magnitude of coefficients.
- Dropout: Randomly sets a fraction of input units to 0 at each update during training, which helps prevent co-adaptation of hidden units.
Implementing Fine Tuning in Llama.cpp
Step-by-Step Fine-Tuning with Code Examples
Loading Your Dataset
Once your data is prepared, it can be loaded into Llama.cpp. Below is an example code snippet:
#include "llama.h" // Include the necessary Llama.cpp library
LlamaModel model;
model.loadData("path/to/dataset.txt");
Training the Model
Fine-tuning involves retraining the model with your specific dataset to optimize performance. A typical training loop may look like this:
for (int epoch = 0; epoch < num_epochs; ++epoch) {
model.train(training_data, batch_size, learning_rate);
if (epoch % eval_interval == 0) {
model.evaluate(validation_data);
}
}
In this example, the model is trained over several epochs, and evaluation is done periodically to monitor performance.
Evaluating Performance
Metrics for Success
Measuring the success of fine-tuning is critical. Common metrics include:
- Accuracy: Proportion of correct predictions.
- Precision and Recall: Useful for classification tasks.
- F1 Score: The harmonic mean of precision and recall, providing a single score.
Analyzing Results
Once you've evaluated your model, it's essential to interpret the results. Use visualization tools to analyze performance metrics. Creating plots of validation loss over epochs can help identify if your model is overfitting or underfitting.
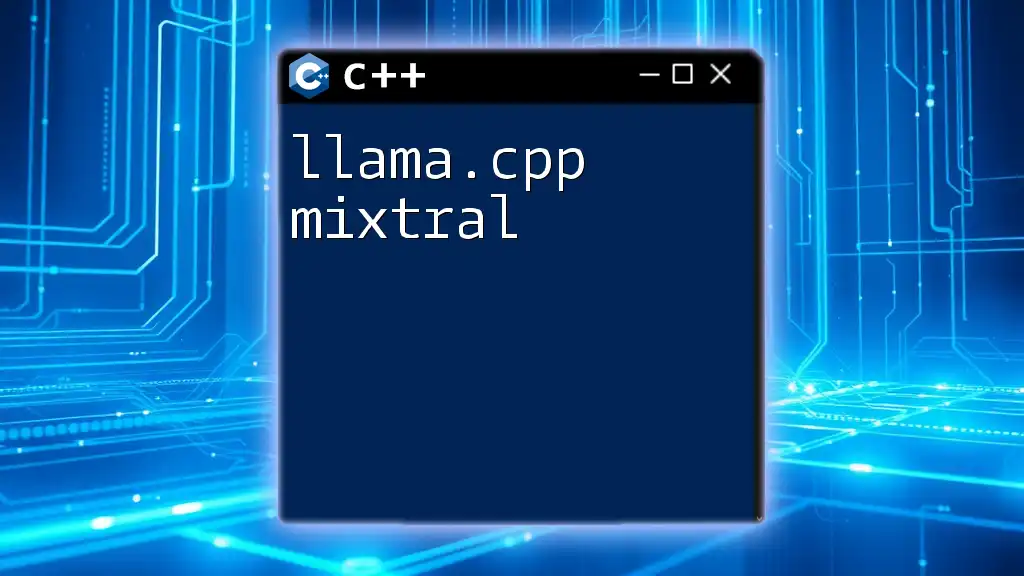
Troubleshooting Common Issues
Common Errors and Solutions
During the llama.cpp fine tune process, you may encounter several errors, such as:
- Out of Memory: This can happen with larger batch sizes. Try reducing the batch size or upgrading your hardware.
- Diverging Loss: If your loss value increases, consider lowering your learning rate.
Best Practices for Smooth Fine Tuning
To facilitate a smoother fine-tuning process, follow these best practices:
- Regularly save your model checkpoints to avoid data loss during training.
- Monitor metrics meticulously to detect issues early.
- Engage with the Llama.cpp community for insights and troubleshooting tips.
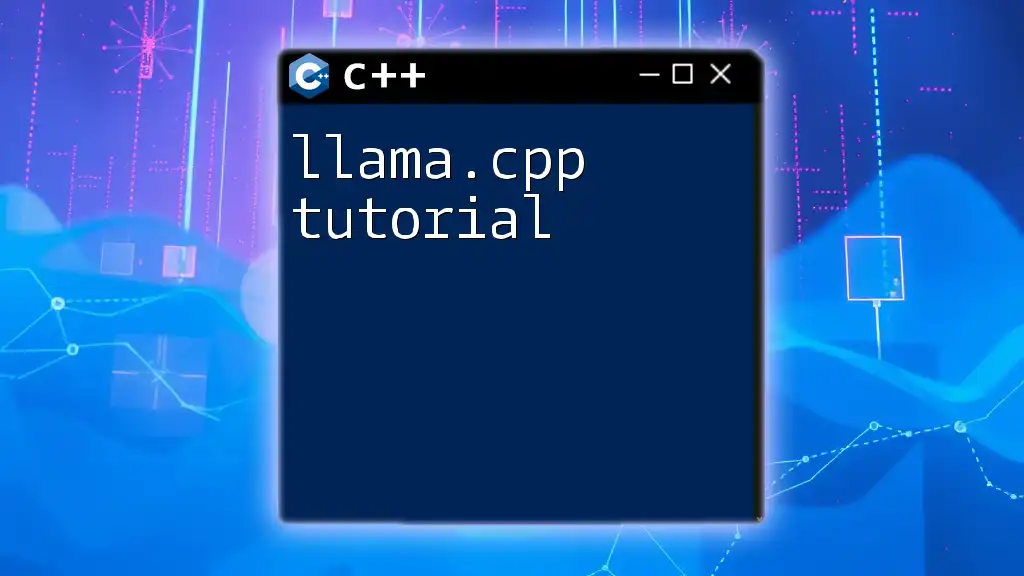
Conclusion
In summary, fine-tuning with Llama.cpp allows developers to customize their models effectively, optimizing them for specific applications. Exploring different techniques and maintaining good practices can lead to significant improvements in performance. As you embark on your journey with Llama.cpp fine-tuning, don't hesitate to experiment and iterate on your models!
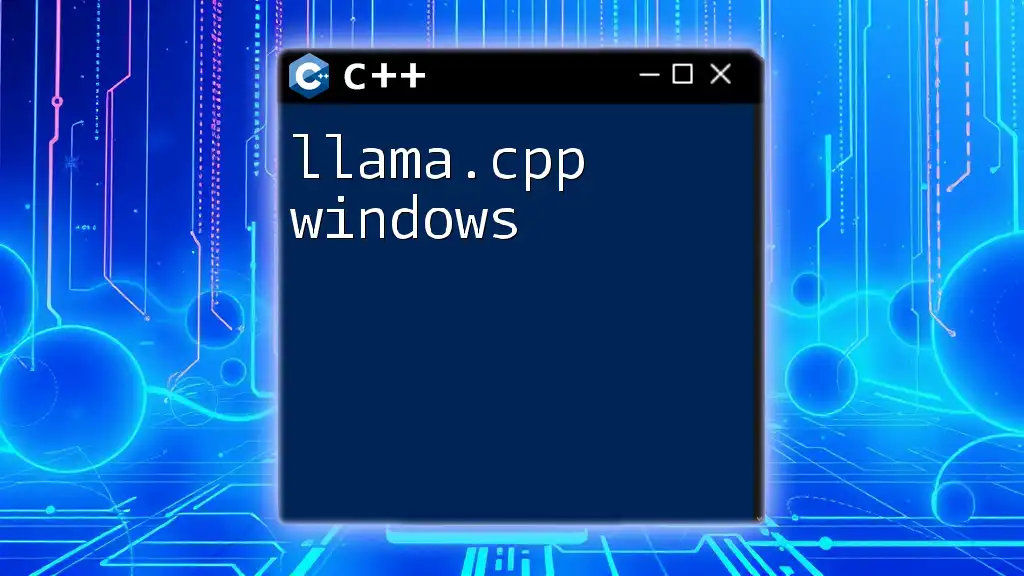
Additional Resources
For further learning, consider exploring books, tutorials, and online courses that delve deeper into Llama.cpp and fine-tuning techniques. Engaging with community forums can also provide valuable insights and support as you enhance your skills.
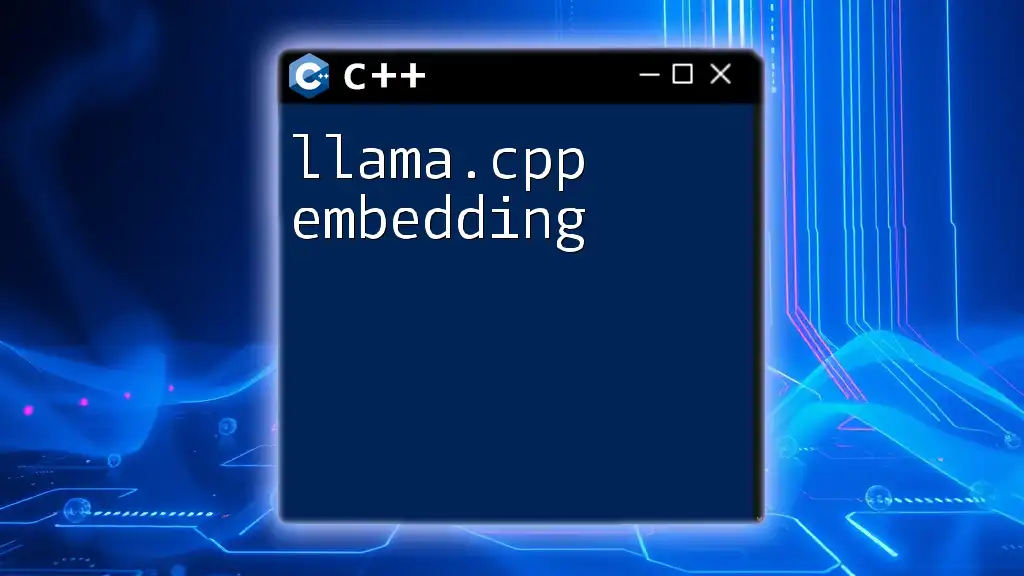
Call to Action
Join our platform to explore more about using C++ commands effectively. Share your experiences with llama.cpp fine tune and let’s grow our community together!