"Llama.cpp Lora training involves using C++ commands to efficiently fine-tune language models through the incorporation of low-rank adaptation."
Here’s a simple code snippet to illustrate a typical setup for Lora training in C++:
#include <lora.h>
int main() {
Lora loraModel("path/to/model");
loraModel.train("path/to/dataset", 100); // Train for 100 epochs
loraModel.save("path/to/save/model");
return 0;
}
What is Llama.cpp?
Llama.cpp is a robust library designed for high-performance machine learning tasks in C++. It is a powerful tool that enables developers to implement and leverage large language models with ease. Its key features include efficient memory management, speed optimizations, and a focus on usability that allows users to harness the power of cutting-edge AI technologies without the steep learning curve.
Importance of C++ in AI Development
C++ is often the language of choice for several AI and machine learning projects due to its performance efficiency and control over system resources. It provides the ability to optimize algorithms at a granular level, making it suitable for scenarios where every millisecond counts, such as real-time applications.
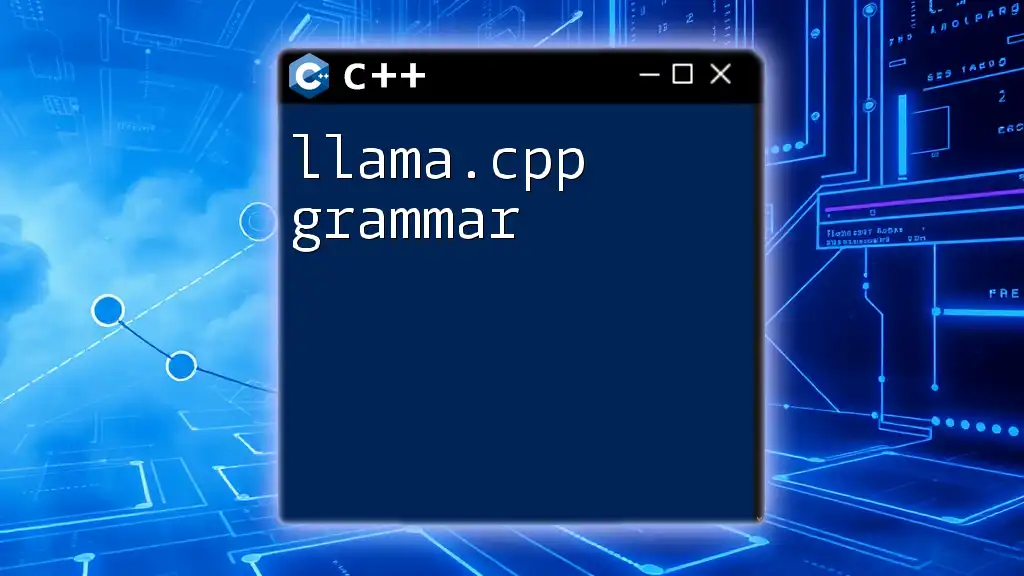
Understanding LoRA (Low-Rank Adaptation)
What is LoRA?
Low-Rank Adaptation (LoRA) is a technique used to fine-tune pre-trained neural networks by adding a small number of trainable parameters. This method significantly reduces the computational cost and memory footprint typically associated with large model training. By maintaining the core weights of the model while only updating a low-rank approximation, LoRA allows developers to adapt existing models for specific tasks efficiently.
Advantages of using LoRA in model training
- Efficiency: Minimal modifications to existing models lower the resource requirements for training.
- Speed: Training proceeds faster since fewer parameters are being tuned, facilitating quicker iteration.
- Performance Preservation: By leveraging the strengths of pre-trained models, LoRA maintains the integrity of the model's knowledge while adapting it to new tasks.
The Need for LoRA in Llama.cpp Training
Training large models often involves extensive computational resources and time. Traditional fine-tuning methods may not be feasible in many scenarios due to hardware constraints. LoRA provides an alternative approach that allows developers to achieve effective model adaptation without demanding excessive resources.
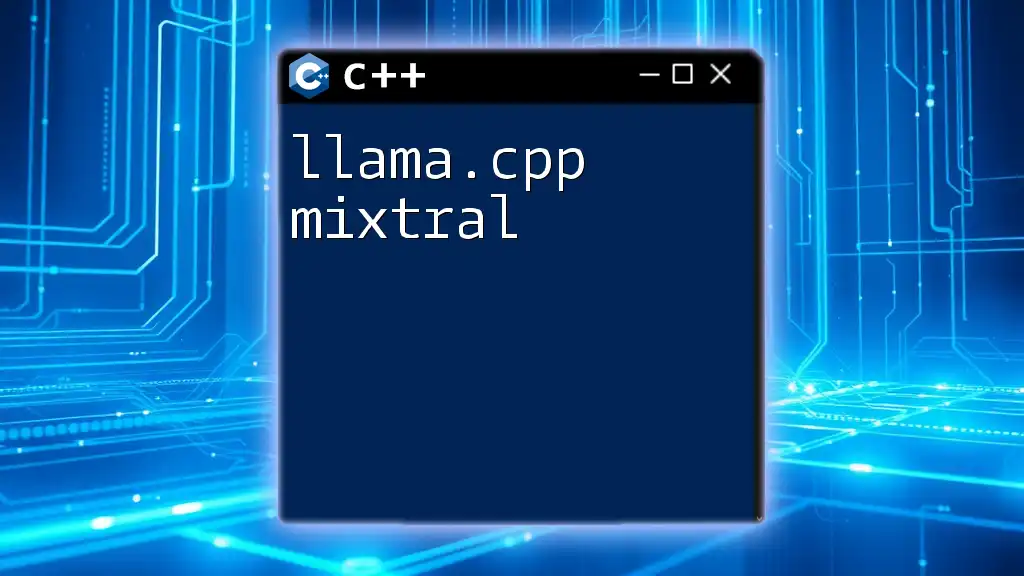
Setting Up Your Environment for Llama.cpp LoRA Training
Prerequisites
Before diving into Llama.cpp LoRA training, there are several prerequisites to consider:
- Software: Ensure you have a C++ compiler (like g++) and CMake installed.
- Libraries: Depending on your project, you may need libraries such as Eigen or Armadillo for matrix operations.
- Hardware: A multi-core CPU with sufficient RAM is recommended to handle the training tasks efficiently.
Installation Steps
To install Llama.cpp, follow these steps:
-
Clone the Llama.cpp repository from GitHub using the command:
git clone https://github.com/username/repository.git
-
Navigate into the cloned directory:
cd repository
-
Build the project using CMake:
mkdir build && cd build cmake .. make
Configuring the Environment
A well-configured C++ project structure enhances collaboration and maintenance. Ensure to organize your project files and include a clear README detailing setup instructions. A common structure might look like:
/project-root
/src
/include
/data
CMakeLists.txt
README.md
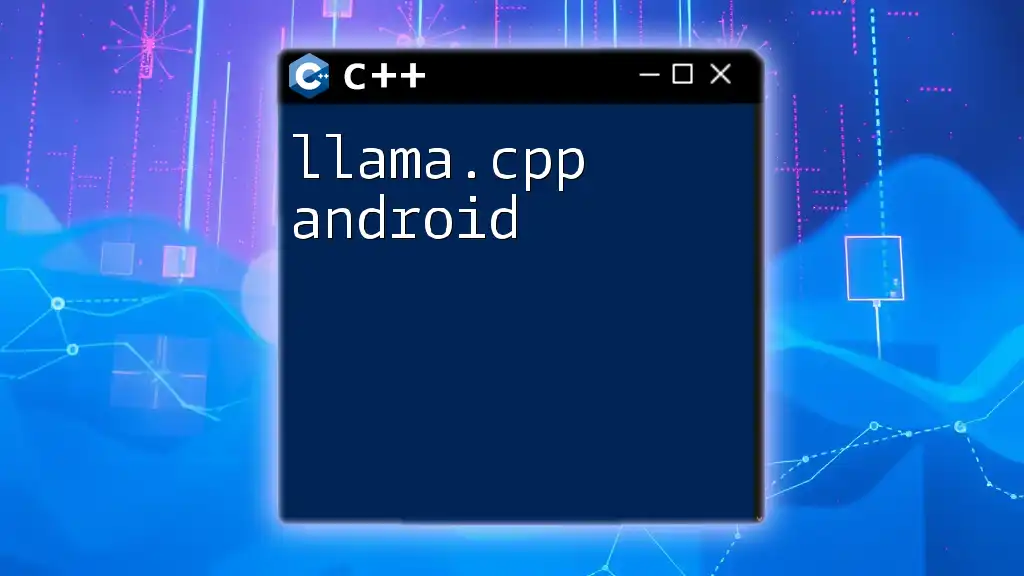
Training with Llama.cpp and LoRA
Preparing Your Data
For successful LoRA training, your data must be well-prepared. Key considerations include:
- Dataset size: Large datasets yield better performance, but ensure they are manageable given your hardware capabilities.
- Preprocessing: Data must be cleaned and possibly augmented to improve model robustness.
An example of basic data preprocessing in C++ might look like this:
#include <vector>
#include <string>
void preprocessData(std::vector<std::string>& data) {
for(auto& str : data) {
// Perform cleaning, like removing punctuation
str.erase(remove_if(str.begin(), str.end(), ::ispunct), str.end());
}
}
Fine-Tuning with LoRA
Step-by-Step Guide
To implement LoRA in Llama.cpp effectively, follow this guide:
-
Initialize the model and LoRA: Load your pre-trained model and initialize the LoRA parameters.
Model model = LoadModel("path/to/pretrained/model"); LoRA lora(model, r); // 'r' is the rank for LoRA
-
Set up the optimizer: Using an optimizer like Adam or SGD is critical for effective training.
Optimizer optimizer = CreateOptimizer(model.parameters(), learning_rate);
-
Run the training loop: Iterate over your data and update the model parameters.
while (training) { model.forward(input); optimizer.step(); // Calculate and log loss here }
Model Configuration Parameters
When configuring your training process, consider critical parameters such as:
- Learning Rate: Start with common values (e.g., 0.001) and adjust based on training performance.
- Batch Size: Determine based on memory capacity; larger batch sizes can accelerate training but require more GPU memory.
Running the Training Process
Executing the training script requires running your main code:
./path/to/your/executable
Monitor the terminal for training progress and be vigilant about error messages or warnings. Carefully examine your logs for any anomalies, as they can indicate issues with data or model configuration.
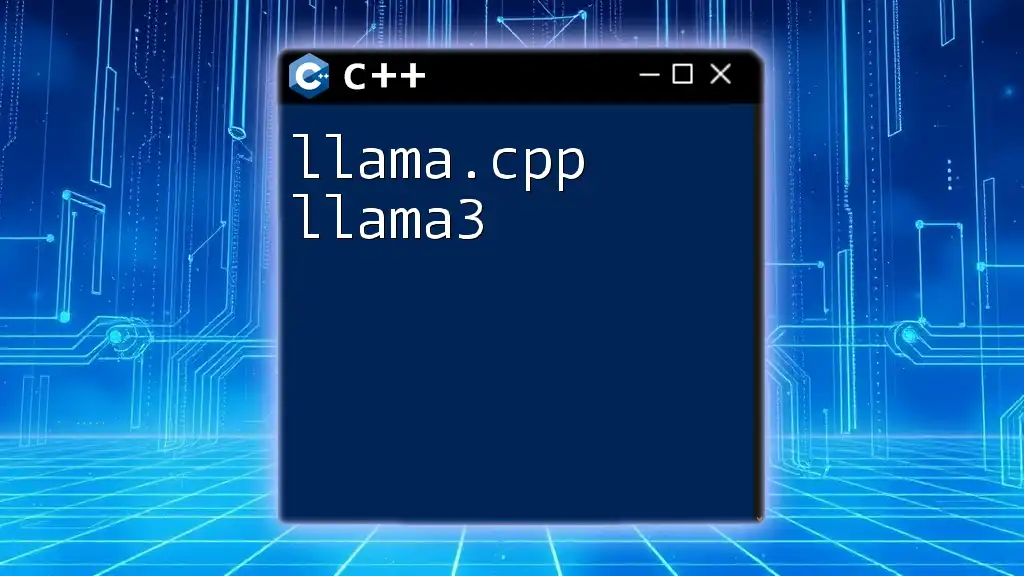
Evaluating Your Trained Model
Model Performance Assessment
Once training is complete, assess the model's performance using metrics appropriate for your task (e.g., accuracy for classification, BLEU score for language generation). A strong model should demonstrate high performance on your validation set compared to the training set to ensure it generalizes well.
Troubleshooting Common Issues
Despite careful setup and execution, issues may arise. Common problems include:
- Overfitting: If training accuracy is high but validation accuracy is low, consider regularization techniques or reducing model complexity.
- Resource Exhaustion: If you encounter out-of-memory errors, reduce batch size or optimize data loading.
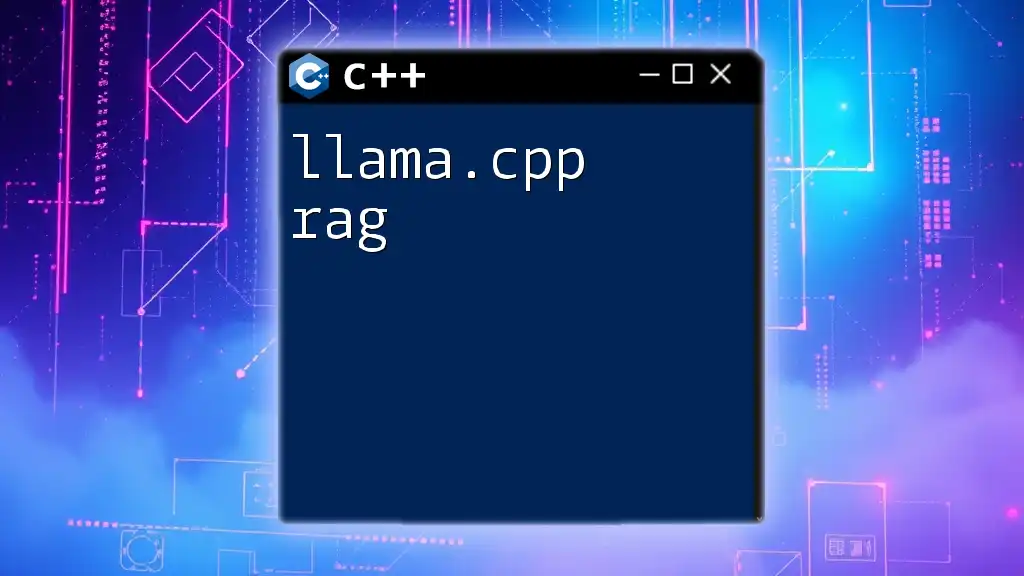
Conclusion
In summary, llama.cpp lora training provides a streamlined approach to fine-tuning large models efficiently. By understanding both Llama.cpp and LoRA, developers can adapt powerful pre-trained models for their unique needs while minimizing resource requirements. Experimentation is key, and readers are encouraged to explore the potential of this technology further.
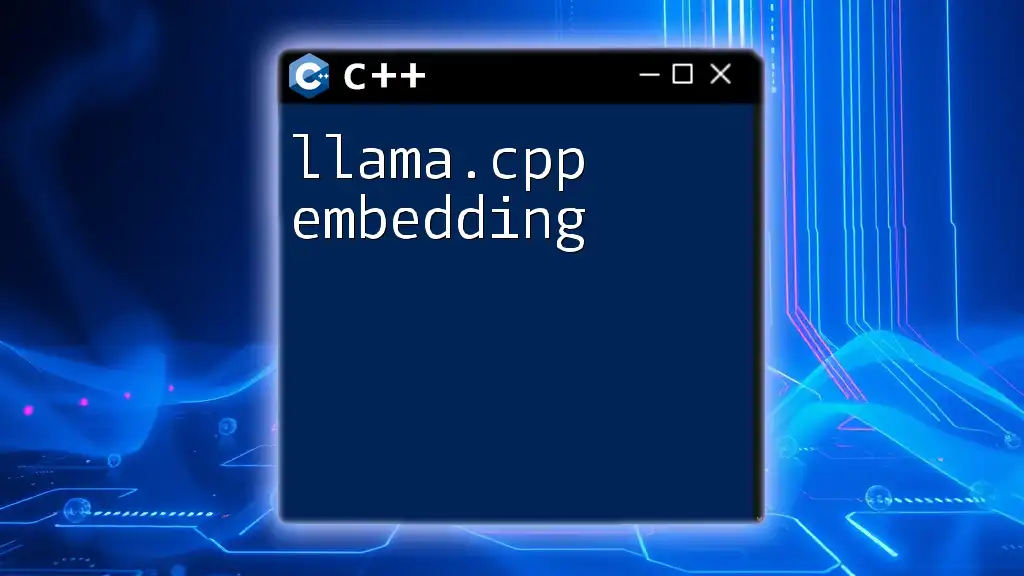
Further Resources
Recommended Reading and Courses
For those looking to deepen their understanding, check out:
- Books on C++ for machine learning.
- Online courses that cover the intersection of AI and C++ programming.
Online Communities and Support
Engage with fellow learners and professionals through forums and communities dedicated to Llama.cpp and machine learning. Platforms such as GitHub discussions or specialized AI forums can be invaluable for sharing insights and getting assistance.
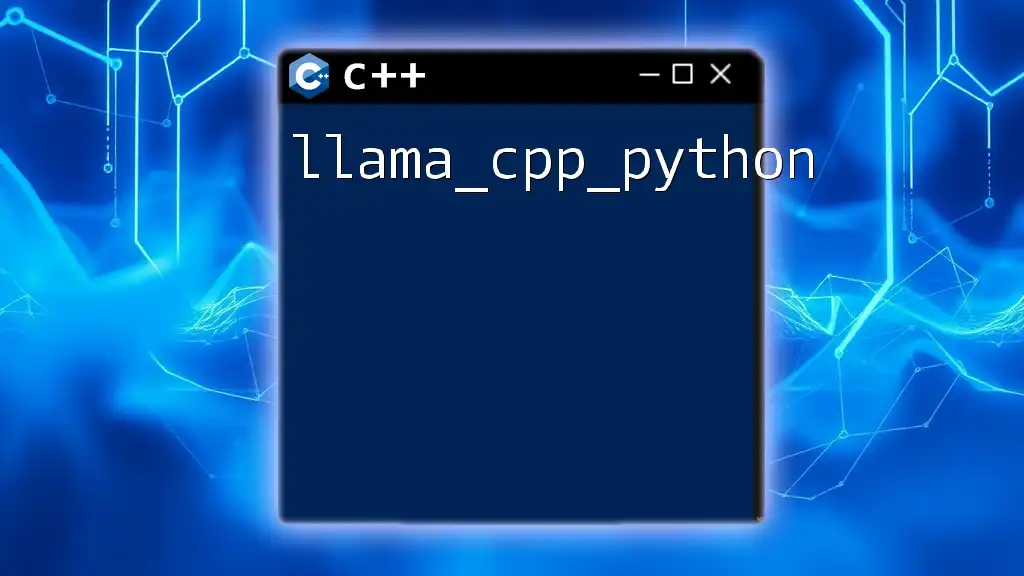
Call to Action
Have you tried llama.cpp lora training yet? Share your experiences and any challenges you faced in the comments below! Join the discussion and contribute to the growing community surrounding these powerful tools.