CPP training focuses on empowering individuals to efficiently utilize C++ commands through concise tutorials and practical examples.
Here’s a simple example of a C++ program that demonstrates the basic syntax of printing "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Getting Started with C++
Setting Up Your Development Environment
To dive into C++ training, the first step is to set up a robust development environment. This process involves choosing an Integrated Development Environment (IDE) that suits your needs. Popular choices include Visual Studio, Code::Blocks, and Eclipse. Installing a compiler is equally important; options like GCC (GNU Compiler Collection) and Clang are widely used among C++ developers.
Basic C++ Syntax
Understanding the basic syntax of a C++ program is crucial for beginners. A simple C++ program has a defined structure, which typically includes a main function and includes directives for libraries.
Here’s a classic Hello World example to illustrate this:
#include <iostream>
int main() {
std::cout << "Hello, World!";
return 0;
}
In this example:
- `#include <iostream>` is a preprocessor directive that includes the standard input-output library.
- `int main()` is the starting point of any C++ program.
- `std::cout` is used to output data to the console.
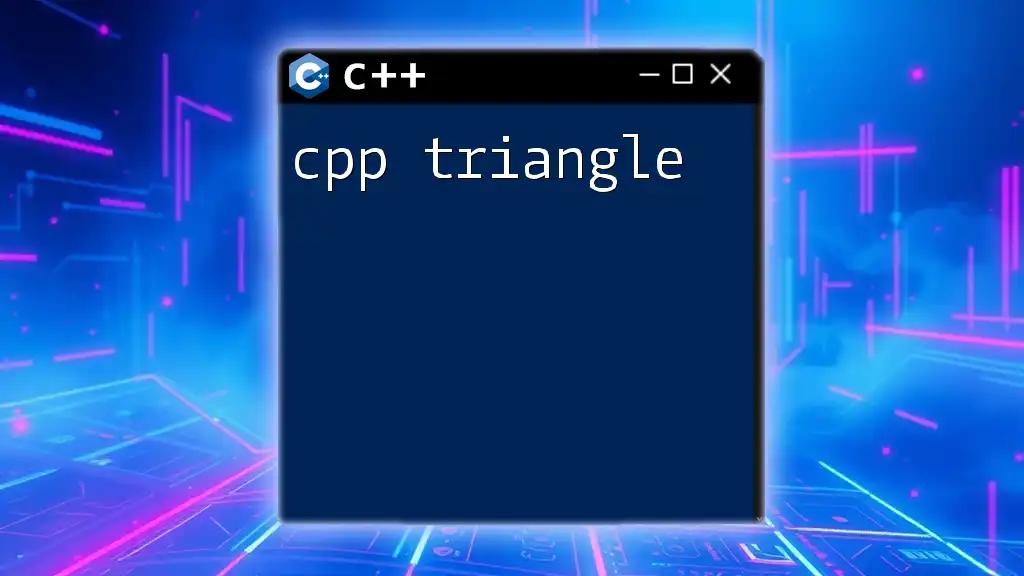
Core Concepts in C++
Data Types and Variables
C++ offers several fundamental data types, including:
- int: for integer values
- float: for floating-point numbers
- char: for single characters
Declaring and initializing variables is straightforward in C++. Here’s an example:
int age = 25;
float salary = 50000.50;
char grade = 'A';
Using the correct data type is essential as it affects memory allocation and operations you can perform on the variable.
Control Structures
Control structures allow for decision-making and repetitive tasks in C++. Conditional statements such as if and switch enable program control flow, while loops like for, while, and do-while are essential for iterations.
Here is an example of a for loop:
for (int i = 0; i < 5; i++) {
std::cout << i << " ";
}
This loop outputs the numbers 0 to 4, showcasing how control structures can efficiently manage repetitive tasks in your C++ training.
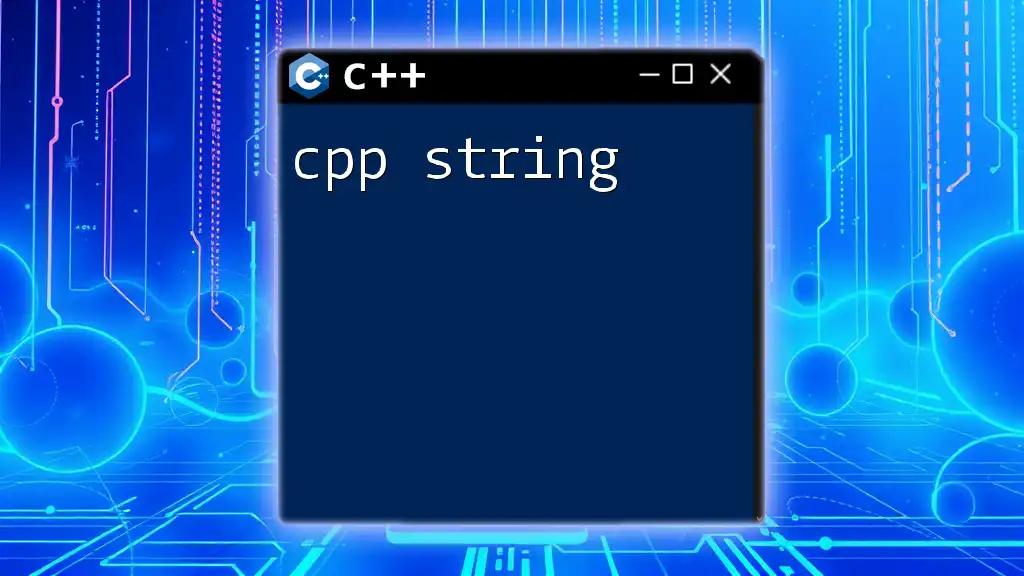
Functions in C++
Defining and Calling Functions
Functions are the building blocks of a C++ program, allowing you to modularize code into reusable segments. The basic syntax involves a return type, function name, and parameters (if any).
Here’s an example of a simple function:
void greet() {
std::cout << "Welcome to C++ Training!";
}
To call this function within your `main`, simply type `greet();`.
Function Parameters and Return Types
Understanding the function parameters and return types enhances the versatility of functions. Parameters allow you to pass values into functions which can then be operated upon.
Here’s an example of a function with parameters and a return type:
int add(int a, int b) {
return a + b;
}
This function takes two integers, adds them, and returns the result. Calling `add(5, 3)` will yield `8`.
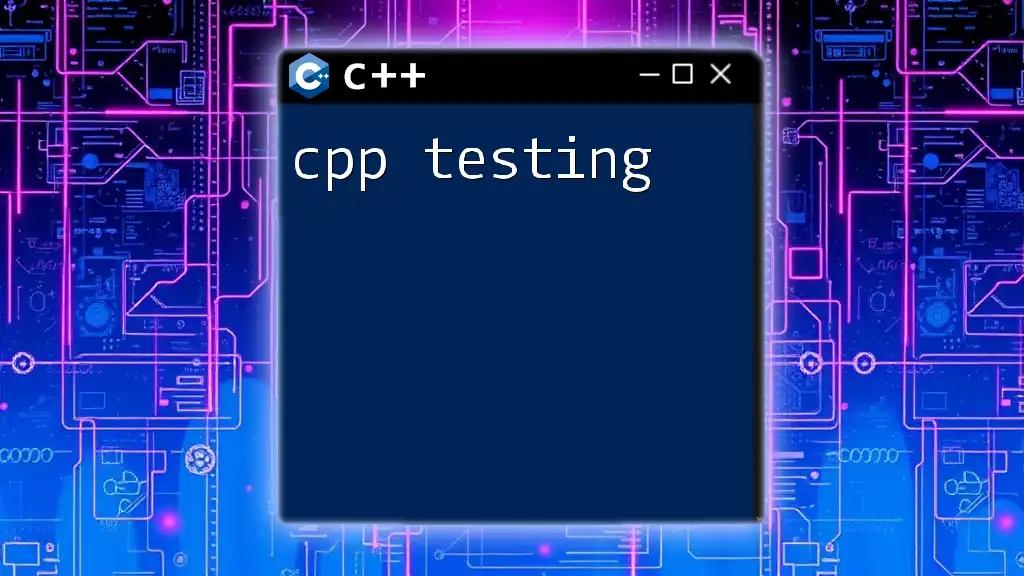
Object-Oriented Programming in C++
Understanding Classes and Objects
C++ is an object-oriented programming (OOP) language, which means it supports concepts such as classes and objects. A class acts as a blueprint for creating objects, encompassing attributes and methods.
Consider the following class definition for a Car:
class Car {
public:
string brand;
void honk() {
std::cout << "Beep beep!";
}
};
In this example, `Car` is a class with an attribute `brand` and a method `honk()`. Defining classes paves the way for encapsulating data and functions, making your code more organized.
Inheritance and Polymorphism
Inheritance allows a class to inherit properties from another class. The base class can define common features, while derived classes can extend or customize functionality.
Polymorphism, on the other hand, allows methods to behave differently based on the object calling them. Here is a basic example:
class Vehicle {
public:
virtual void start() {
std::cout << "Vehicle Starting";
}
};
class Bike : public Vehicle {
public:
void start() override {
std::cout << "Bike Starting";
}
};
In this case, the `start()` method behaves differently depending on whether it's invoked by a `Vehicle` or a `Bike`, demonstrating the power of OOP principles in your C++ training.
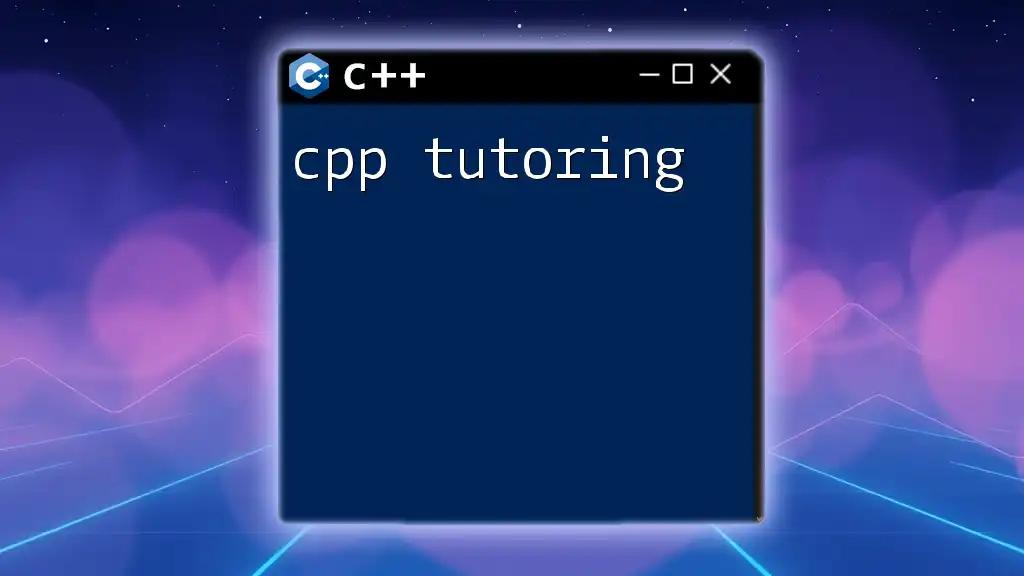
Advanced C++ Topics
Pointers and Memory Management
Pointers allow you to store the address of another variable, providing powerful capabilities to manipulate data directly in memory.
Understanding memory management is crucial to prevent issues such as memory leaks. Here’s an example of dynamic memory allocation:
int* ptr = new int; // dynamically allocated memory
*ptr = 10; // assign value
delete ptr; // free allocated memory
Using `new` allocates memory dynamically, while `delete` is used to free that memory, ensuring your application runs efficiently.
Templates and STL (Standard Template Library)
Templates allow you to create functions and classes that can operate on any data type. This is useful for writing generic code that can be reused across various types.
The Standard Template Library (STL) provides a collection of generic classes and functions. It includes useful containers like `vector`, `list`, and `map`. Here’s an example of using a vector:
#include <vector>
std::vector<int> vec = {1, 2, 3, 4};
for(int i : vec) {
std::cout << i << " ";
}
This example demonstrates how to create a dynamic array and iterate through its elements, showcasing the versatility and efficiency of STL resources which can significantly enhance your C++ training.
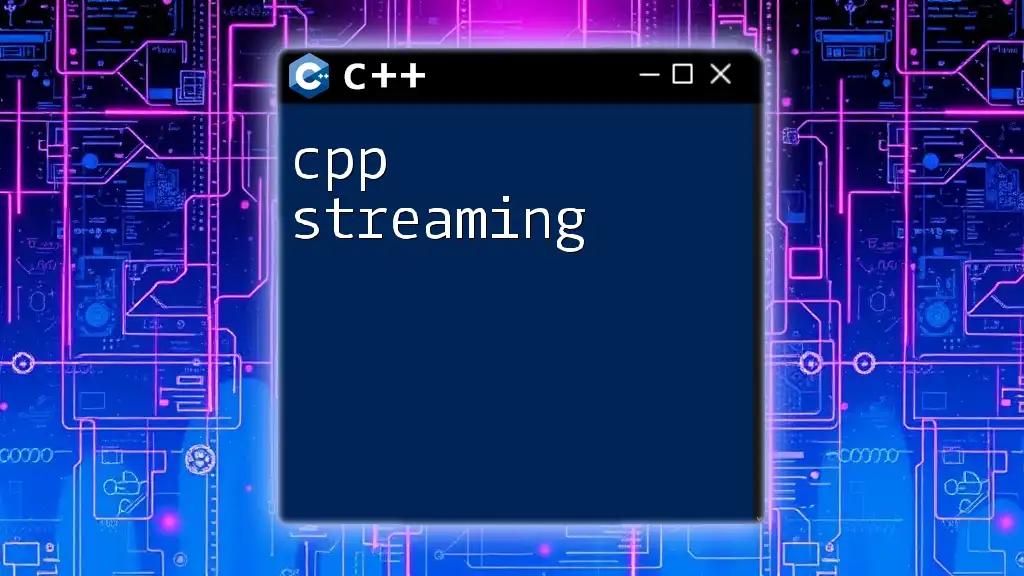
Best Practices in C++
Writing Clean and Maintainable Code
Maintaining clean code practices is imperative for successful programming. Incorporate comments to explain complex code segments, use clear naming conventions, and keep your functions as concise as possible. This makes your code more readable and easier for others (and yourself) to maintain.
Common Pitfalls and How to Avoid Them
As you progress in your C++ training, being aware of common pitfalls, such as memory leaks and dangling pointers, is vital. Always ensure to free dynamically allocated memory and avoid returning addresses of local variables from functions.
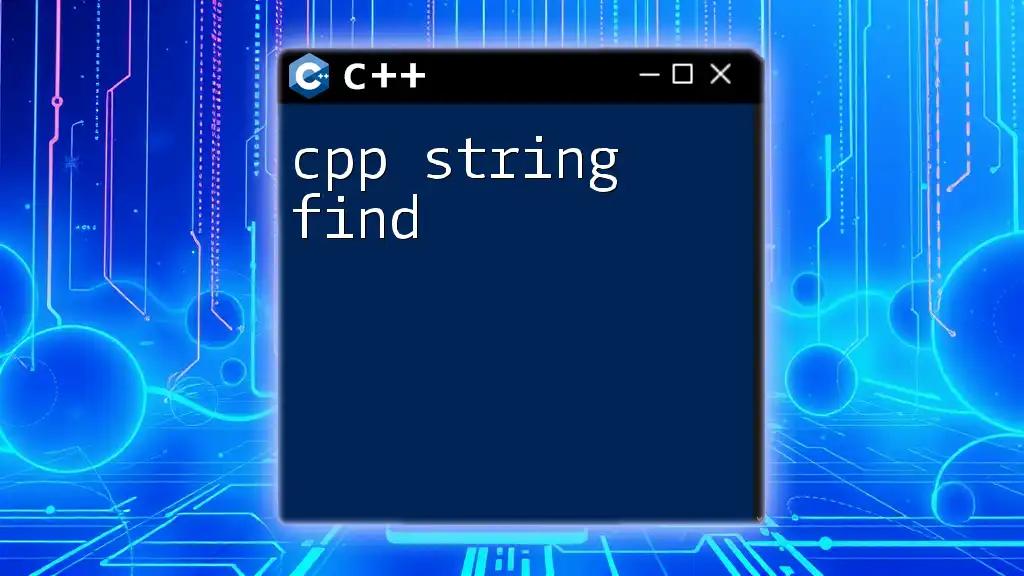
Conclusion
Through this C++ training guide, you have delved into the foundational concepts of C++, explored its object-oriented features, and ventured into more advanced topics such as pointers and templates. Each section serves to build upon the last, giving you a well-rounded understanding of what C++ has to offer.
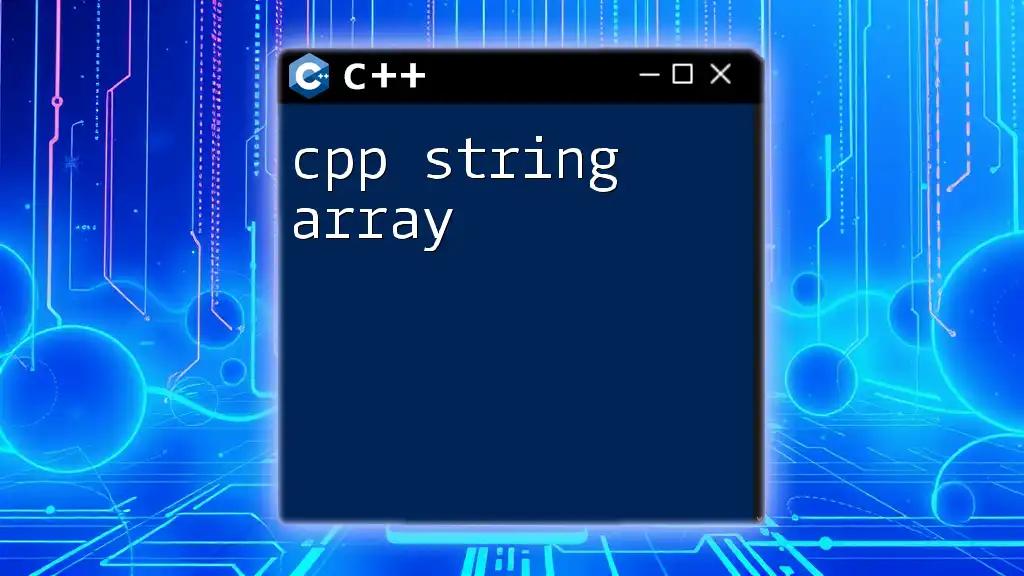
Call to Action
If you are excited about mastering C++, consider joining our specialized C++ training program. Our course is designed to guide you through practical examples, projects, and the deep insights necessary for becoming proficient in C++. Sign up today to start your journey towards becoming a skilled C++ developer!