In C++, ranges provide a way to work with sequences of elements more intuitively and declaratively, allowing for easier manipulation through the standard library's range-based algorithms.
Here's a simple example demonstrating how to use ranges with the `std::views`:
#include <iostream>
#include <vector>
#include <ranges>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
auto even_numbers = numbers | std::views::filter([](int n) { return n % 2 == 0; });
for (int num : even_numbers) {
std::cout << num << " "; // Output: 2 4
}
return 0;
}
What are C++ Ranges?
C++ ranges are a powerful feature introduced in C++20 that modernizes the way developers work with sequences of data. Ranges provide an expressive and intuitive syntax for handling collections, allowing programmers to focus more on what they wish to achieve rather than the intricate details of iterators. With ranges, you can manipulate and interact with collections in a more readable way, resulting in cleaner and more maintainable code.
Comparison with traditional iterator-based loops: Traditional C++ programming often involves iterators and complicated loop structures to traverse collections. Ranges simplify this process, promoting a more functional programming style and enhancing clarity.
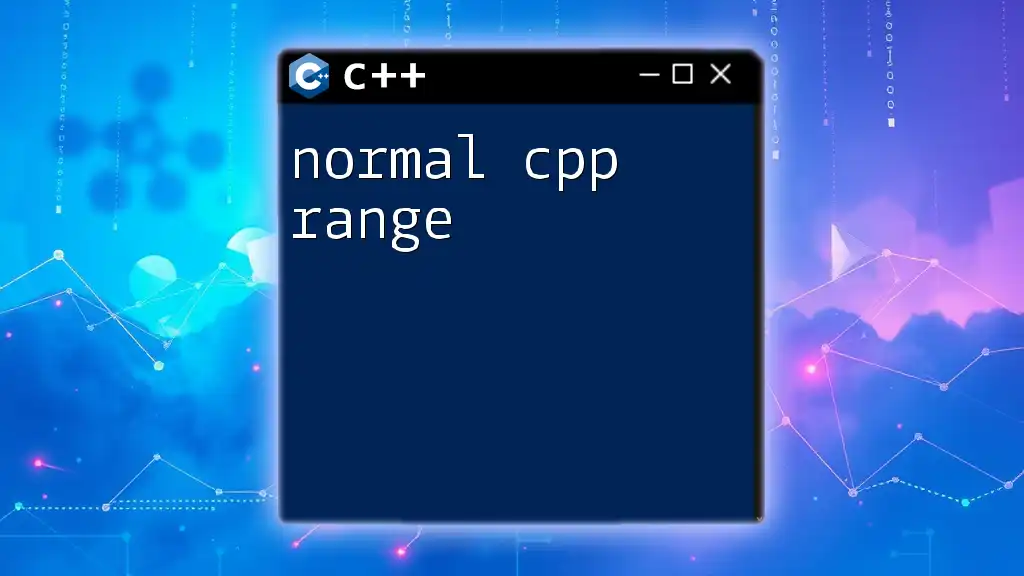
The Evolution of Ranges in C++
To appreciate C++ ranges, it's essential to recognize their historical context. Although the concept of ranges has been around in programming for a long time, C++ lacked formal support until the advent of C++20. The introduction of ranges represents a significant evolution in the language, aligning C++ with modern programming paradigms that emphasize elegance and efficiency.
By incorporating ranges into C++, the language embraces a more functional approach, enabling developers to write expressive code that is not only easier to understand but also faster to develop and debug.
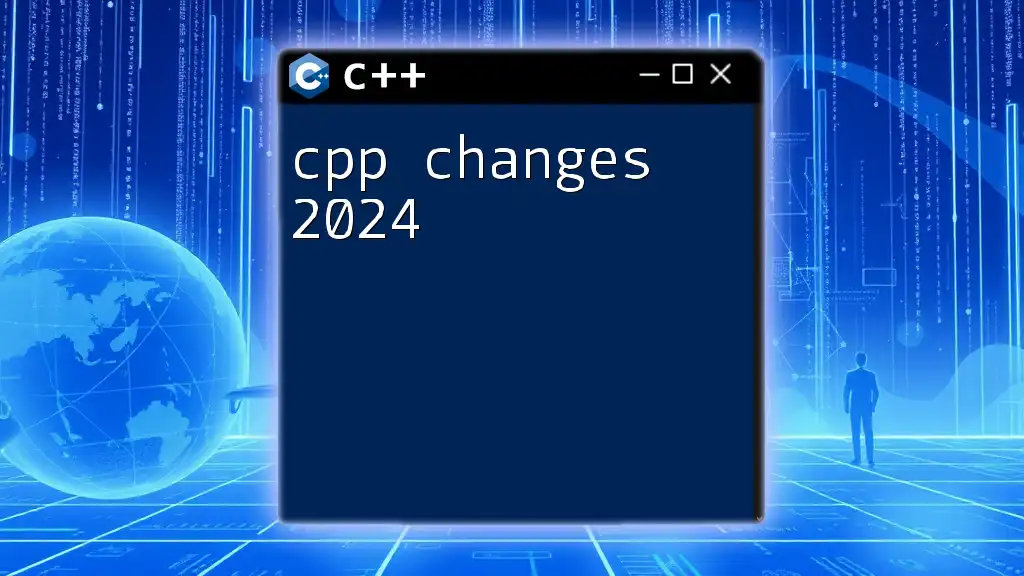
What are C++20 Ranges?
C++20 ranges offer a refined approach to dealing with sequences, focusing on expressiveness and usability. With the introduction of these ranges, developers can handle data in a way that aligns with contemporary programming practices.
Benefits of using ranges in modern C++ programming include:
- Increased readability: Code written with ranges is often clearer since the operations performed on the data are explicitly defined.
- Enhanced maintainability: With ranges, changes to how data is processed are easier to implement and understand.
- Simplified syntax: Less boilerplate code means programmers can write more with fewer lines.
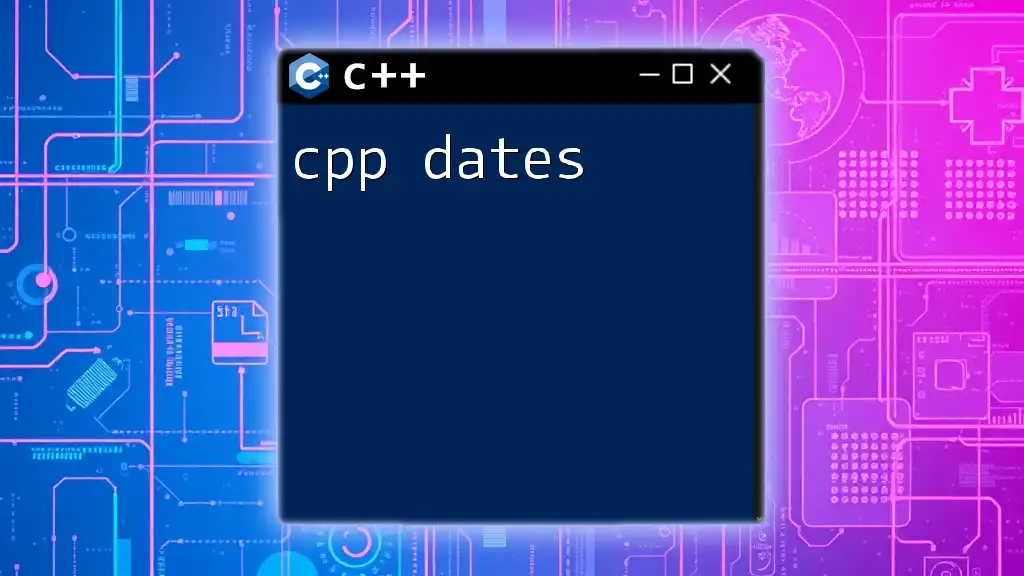
Key Features of C++20 Ranges
C++20 ranges come packed with features that make them an attractive choice for developers. Some of the standout characteristics include:
- Lazy evaluation: Ranges support lazy evaluation, which means that elements are processed only when necessary. This leads to performance benefits, especially when working with large datasets.
- Composability of range operations: Ranges enable you to combine multiple operations seamlessly. For example, you can filter, transform, and sort data in a single, coherent expression.
- Improved readability and maintainability: With less clutter in syntax, code utilizing ranges can be easier to read and maintain.
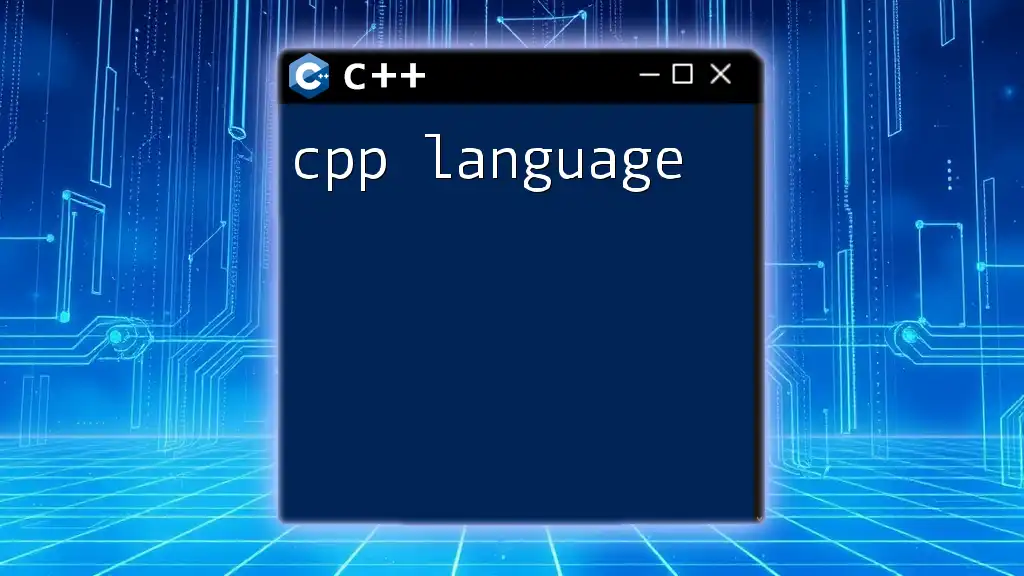
Ranges vs. Iterators
It's crucial to understand how ranges differ from traditional iterators. While both allow for sequential access to data, ranges abstract away the complexity involved with iterators.
When to use ranges over iterators:
- If you prioritize readability and conciseness, ranges should be your preferred choice.
- For functional programming patterns, ranges provide a cleaner way to express operations.
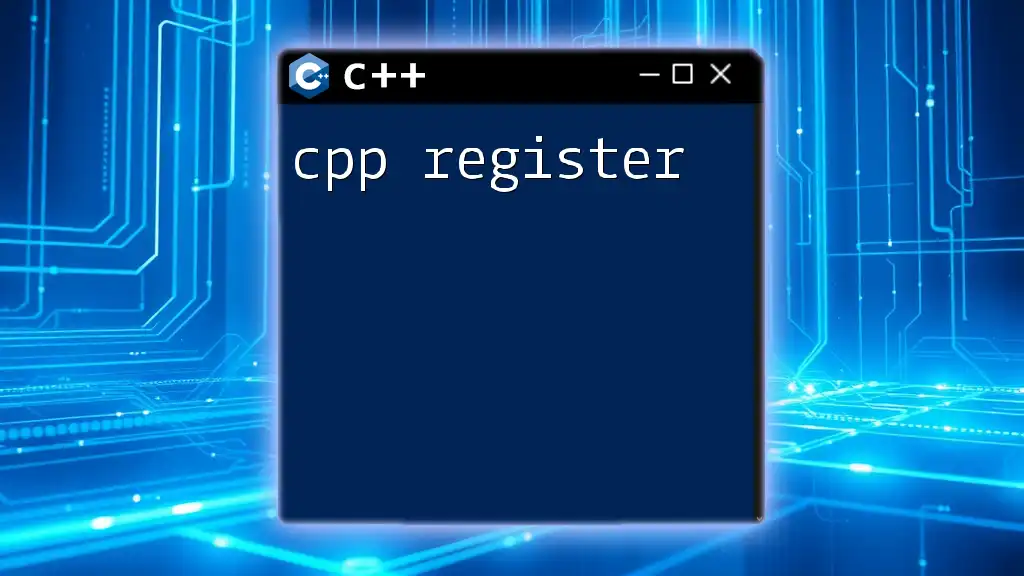
Components of C++ Ranges
To understand C++ ranges better, it's helpful to get acquainted with two essential components: view and container.
View vs. Container
Views: Views in C++ ranges are lightweight representations of sequences that allow for on-the-fly transformations without actually altering the underlying data. This characteristic lets developers work with abstracted data efficiently.
Containers: Traditional containers (like `std::vector`, `std::list`, etc.) store data and own their elements. In contrast, views do not own the data but provide a way to access it through a series of transformations.
By understanding these components, you can effectively choose when to use views and when to rely on standard containers.
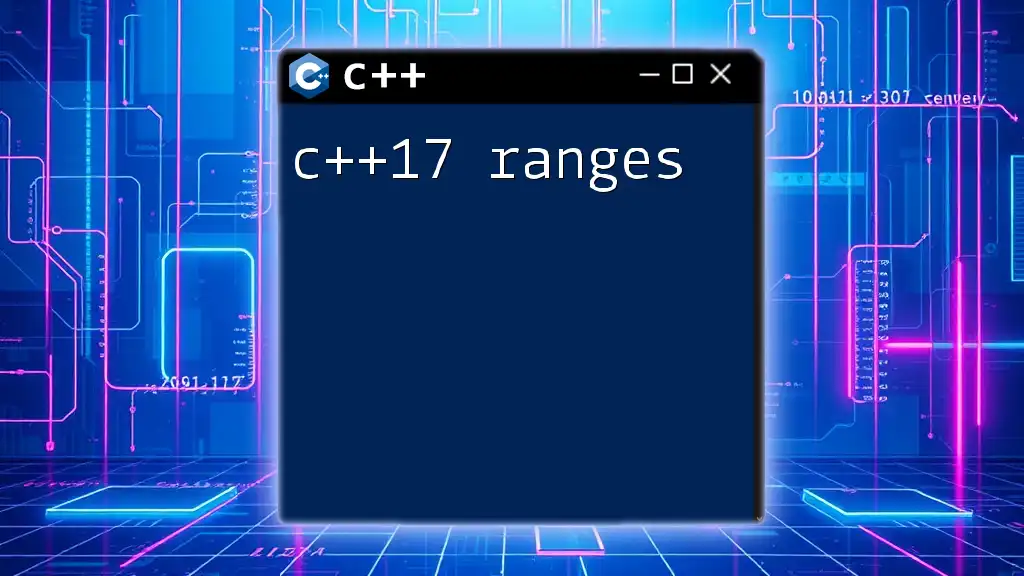
Creating Ranges
Creating ranges in C++ is straightforward. You can leverage the existing standard library containers to create your ranges easily. For instance, you can define a range from a vector of integers and apply transformations like this:
#include <vector>
#include <ranges>
std::vector<int> numbers = {1, 2, 3, 4, 5};
auto range = numbers | std::views::transform([](int n) { return n * 2; });
In the above example, `numbers` is transformed using a lambda function that doubles each element. This is a simple yet powerful way to manipulate sequences without manual iteration.
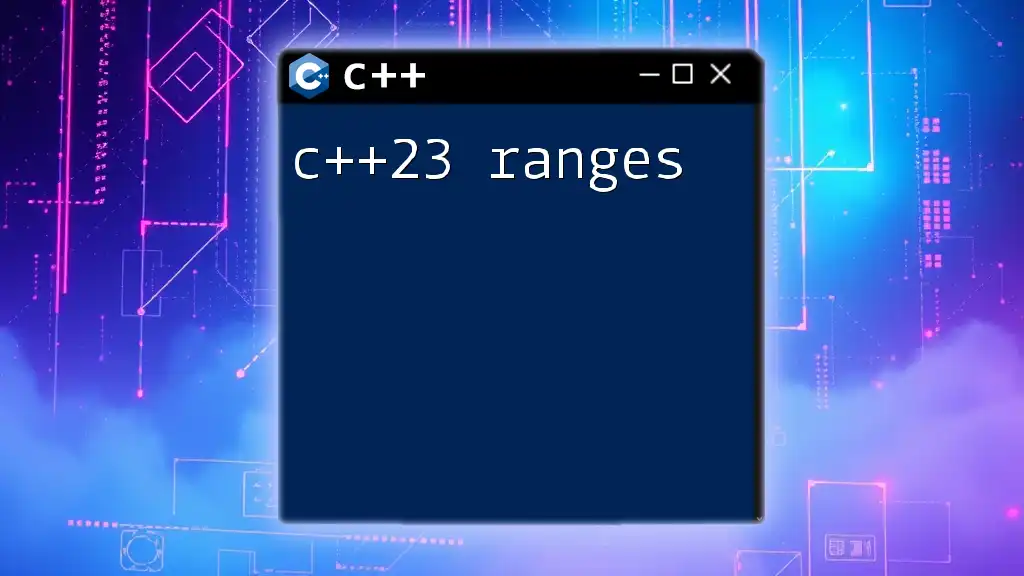
Common Range Algorithms
C++ ranges are compatible with standard algorithms, which allows you to harness a broad array of functionalities seamlessly. You can utilize algorithms like `std::ranges::sort`, `std::ranges::find`, and more.
For example, consider this code snippet that utilizes `std::ranges::sort` to sort a vector:
#include <vector>
#include <ranges>
std::vector<int> numbers = {5, 1, 4, 2, 3};
std::ranges::sort(numbers);
This code sorts the `numbers` vector in ascending order without needing to specify any iterators manually. It makes the intention clear and the operation concise.
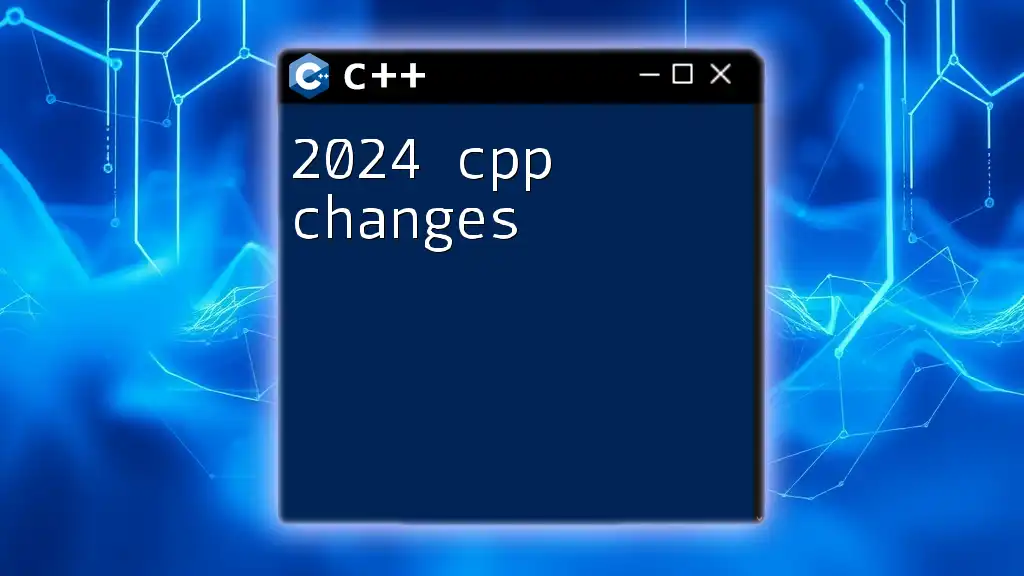
Chaining Operations with Ranges
One of the most powerful capabilities of C++ ranges is the ability to chain multiple operations together efficiently. You can filter elements, transform values, and more in a single, readable expression.
Here's an example of chaining operations to create a new range of squared even numbers:
auto even_numbers = numbers
| std::views::filter([](int n) { return n % 2 == 0; })
| std::views::transform([](int n) { return n * n; });
In this snippet, we first filter the even numbers from the `numbers` vector and then transform them by squaring each value. This approach significantly reduces complexity and improves clarity.
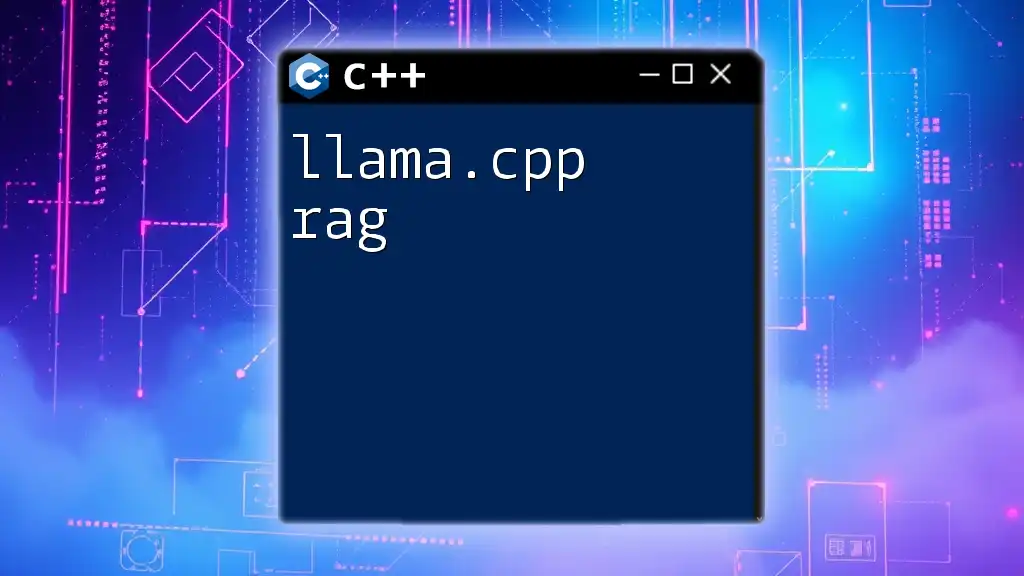
Custom Range Views
Creating custom range views allows you to tailor sequences to your specific needs. You can define your own transformations to facilitate custom data processing. Here’s how to create a simple custom range view:
template<typename R>
auto custom_view(R&& range) {
return range | std::views::transform([](auto &&elem) { return elem + 10; });
}
In this function, when you pass a range, it creates a view that adds 10 to each element. This flexibility allows you to build reusable, modular code easily.
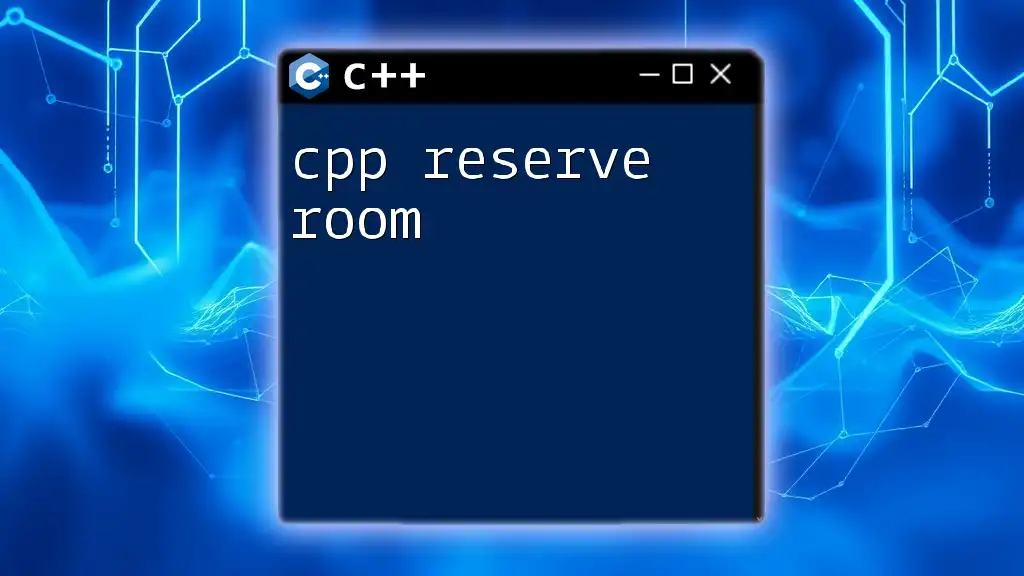
Lazy vs. Eager Evaluation
Lazy evaluation is a significant advantage of using C++ ranges. Elements are evaluated only when needed, which can result in substantial performance gains, especially with complex data manipulations.
However, it's important to recognize the trade-offs. Lazy evaluation can lead to iterative overhead in certain cases where all elements need to be processed. Understanding when to utilize lazy versus eager evaluation enables more efficient coding practices.
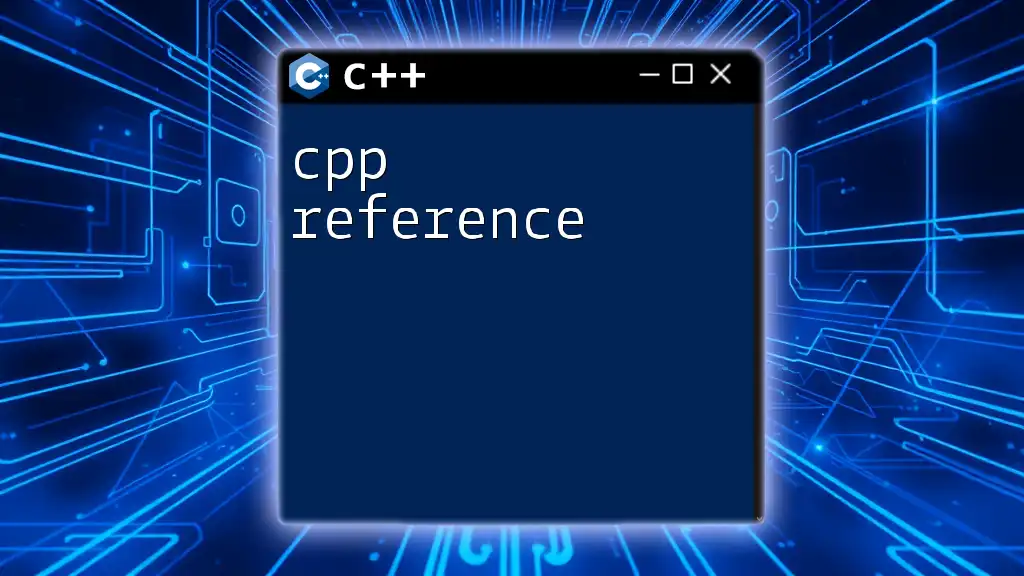
Real-world Examples
C++ ranges shine particularly in application development where managing collections is commonplace. For example, when processing data streams or filtering large datasets, C++ ranges can optimize performance and maintain clarity.
A concrete example is in sorting user input data or processing results from a database. As ranges facilitate clear and concise data manipulation, they can significantly enhance the overall development experience.
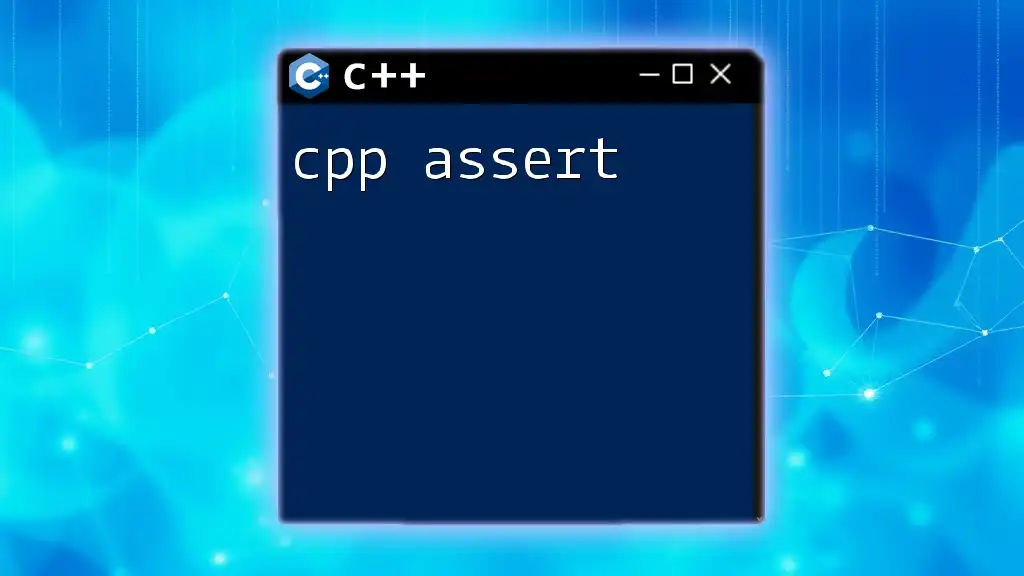
Best Practices for Using Ranges
To maximize the benefits of C++ ranges, it's essential to follow best practices:
- Prioritize readability by choosing meaningful names for views and transformation functions.
- Ensure sufficient testing for custom range views to guarantee they correctly process data.
- Utilize standard algorithms alongside ranges, making the most of existing functionalities while enhancing expressiveness.
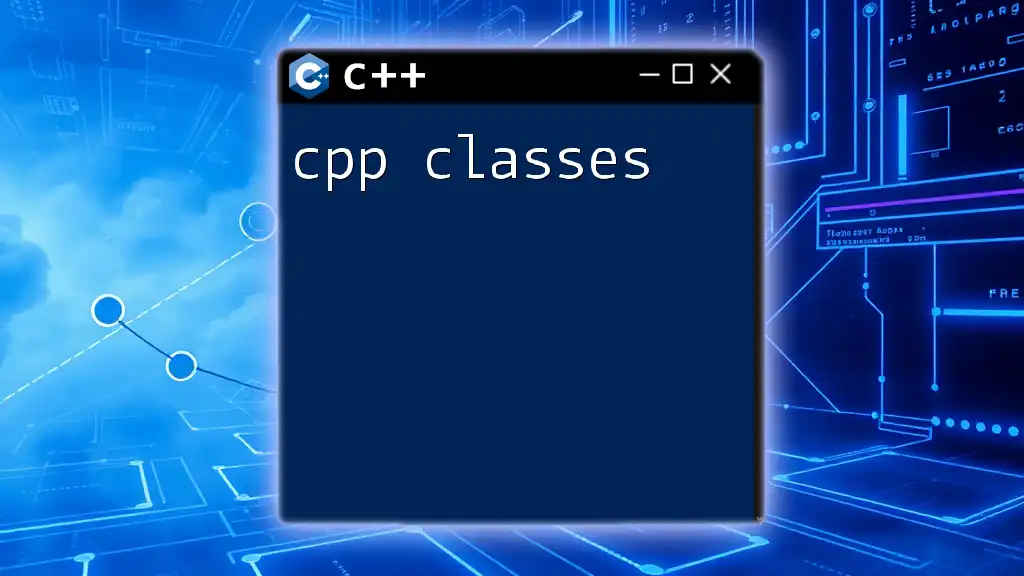
The Future of C++ Ranges
As C++ continues to evolve, so will the support for ranges and their usage patterns. Developers are encouraged to adopt ranges for modern programming practices to take advantage of improved performance and readability. Keeping up with ongoing developments in C++ standards will ensure that you can leverage new features and paradigms as they are made available.
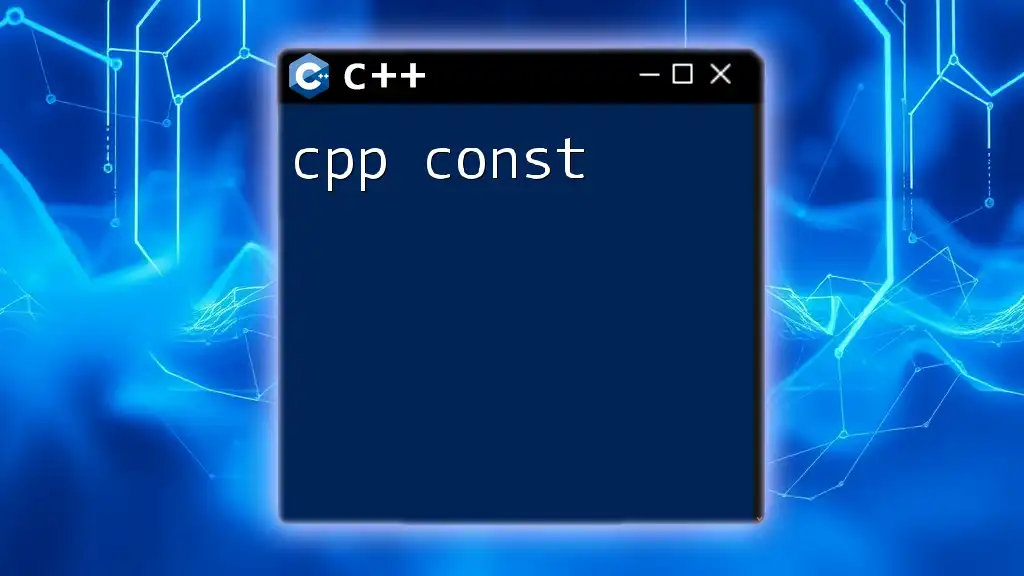
Further Reading and References
For those looking to expand their understanding of C++ ranges, numerous resources are available. Refer to the official documentation for deeper insights, tutorials for practical applications, and community resources for ongoing discussions.
As you explore the transforming landscape of C++ programming, engage with forums and practice with examples to become proficient in using C++ ranges effectively in your projects.