C++17 ranges simplify the process of working with sequences by providing a more intuitive way to manipulate collections with a range-based approach, allowing developers to perform operations like filtering and transforming effortlessly.
Here’s a code snippet demonstrating the use of ranges in C++17:
#include <iostream>
#include <vector>
#include <ranges>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5, 6};
auto even_numbers = numbers | std::views::filter([](int n) { return n % 2 == 0; })
| std::views::transform([](int n) { return n * n; });
for (int n : even_numbers) {
std::cout << n << " ";
}
return 0;
}
What are Ranges?
In modern C++ programming, ranges serve as a powerful abstraction for working with sequences of values. They allow you to express operations such as iteration, filtering, and transforming in a more intuitive way. Ranges help in making your code less error-prone and easier to read, encapsulating the details of iterators and algorithms while providing a clean and expressive syntax.
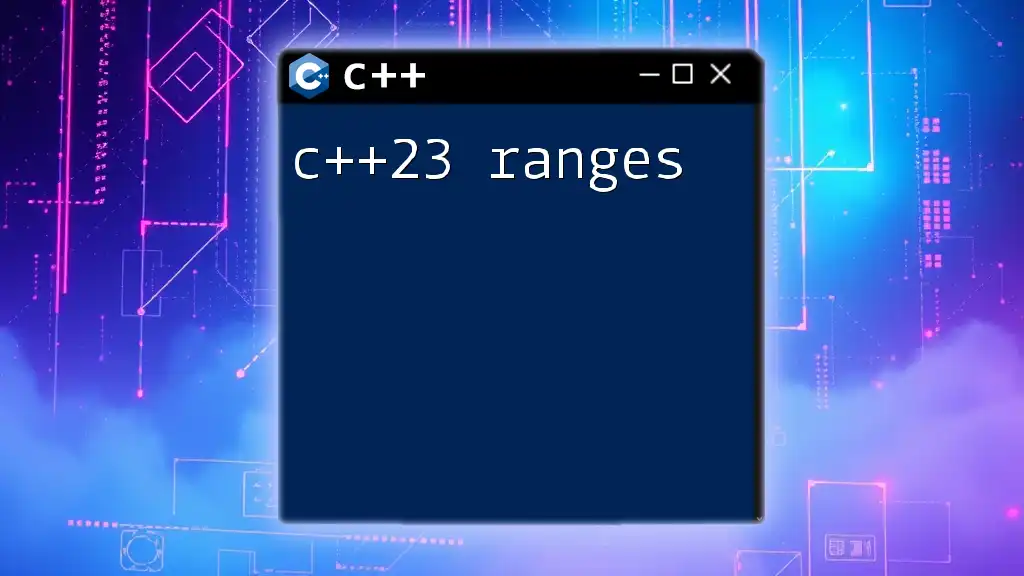
Why Ranges?
The advent of C++17 brought significant enhancements to the C++ Standard Library, one of which is the concept of ranges. Using ranges makes code more concise and expressive, as you can immediately understand the intent behind operations. This is especially useful for beginners and can also greatly benefit seasoned developers looking to improve code maintainability and readability.
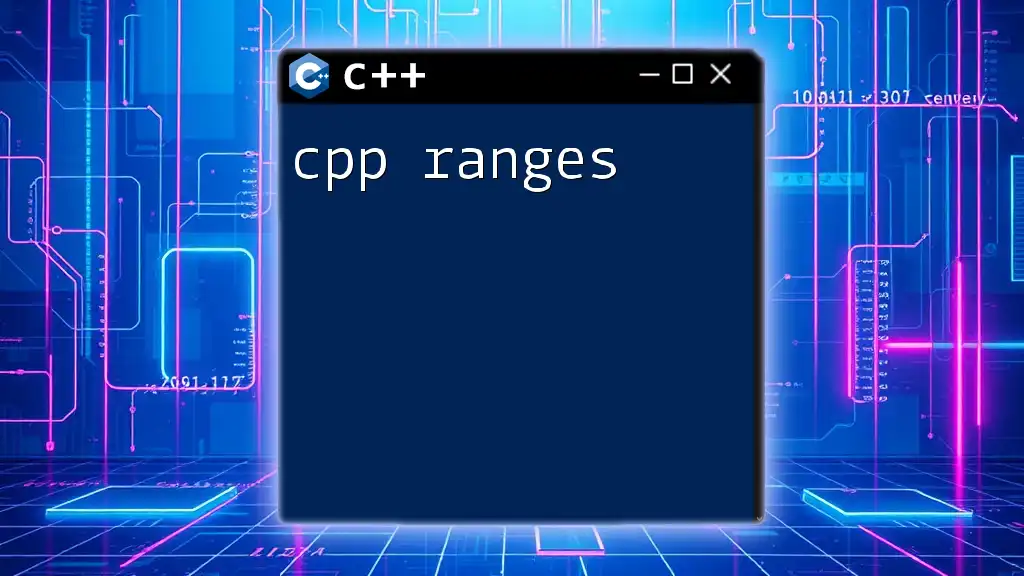
Core Concepts of Ranges
Range Types
C++17 defines several types of ranges, each suited for different use cases:
- Input Ranges: Allow traversing elements in a single pass.
- Output Ranges: Designed for producing a sequence of values.
- Forward Ranges: Permit multiple passes through the data.
- Bidirectional Ranges: Can be traversed in both directions.
- Random Access Ranges: Allow direct access to elements using offsets.
Components of Ranges
View
A view is a lightweight, non-owning wrapper around a range that allows you to apply transformations or filters without copying the underlying data. Built-in views in C++ include `std::views::filter` for filtering elements and `std::views::transform` for applying transformations.
Adapters
Adapters allow you to modify or combine views. They change how a range is iterated or accessed. Examples include `std::views::take`, which limits the number of elements retrieved, and `std::views::drop`, which skips a specified number of elements.
Actions
Actions are operations that modify the original range. For instance, `std::ranges::sort` rearranges elements, while `std::ranges::copy` copies elements from one range to another.
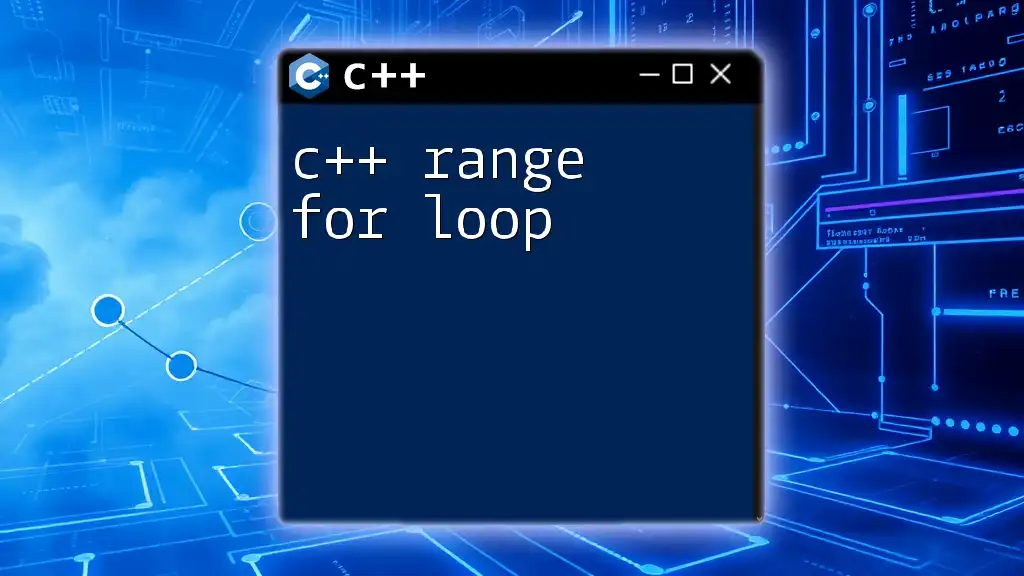
Working with C++17 Ranges
Basic Usage
To illustrate the basic use of ranges, consider creating a range from a `std::vector`. The following code snippet demonstrates how to create a transformed range:
#include <iostream>
#include <vector>
#include <ranges>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
auto range = nums | std::views::transform([](int n) { return n * n; });
for (int n : range) {
std::cout << n << ' '; // Output: 1 4 9 16 25
}
}
In this code, the `transform` operation squares each number in the original vector, demonstrating the power and simplicity of using ranges.
Filtering with Ranges
Filtering is one of the most common operations where ranges shine. Here’s how to filter even numbers from a collection:
#include <iostream>
#include <vector>
#include <ranges>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
auto even_nums = nums | std::views::filter([](int n) { return n % 2 == 0; });
for (int n : even_nums) {
std::cout << n << ' '; // Output: 2 4
}
}
This example demonstrates how the `filter` view allows you to concisely express the intent to iterate through only the even numbers.
Transforming with Ranges
Transformations can also be applied seamlessly with ranges. Here’s an example that shows how to double each number:
#include <iostream>
#include <vector>
#include <ranges>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
auto doubled = nums | std::views::transform([](int n) { return n * 2; });
for (int n : doubled) {
std::cout << n << ' '; // Output: 2 4 6 8 10
}
}
This code snippet showcases how easily one can apply a transformation on each element of a range using `std::views::transform`.
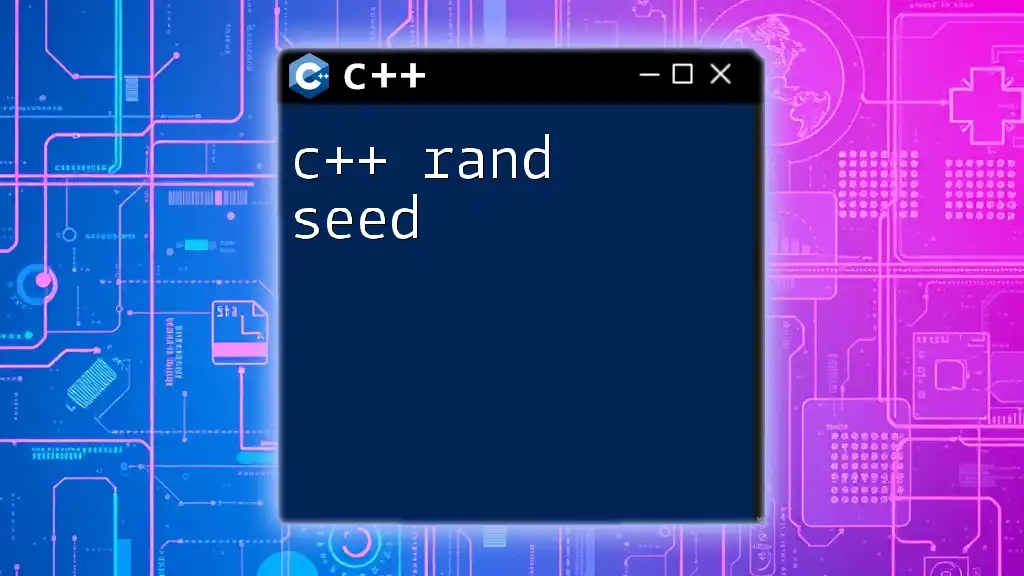
Advanced Range Techniques
Combining Ranges
One of the immense strengths of C++17 ranges is the ability to combine multiple operations elegantly. By chaining together views, you can create sophisticated data pipelines. Here’s an example combining filtering and transformation:
#include <iostream>
#include <vector>
#include <ranges>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5, 6};
auto result = nums | std::views::filter([](int n) { return n % 2 == 0; })
| std::views::transform([](int n) { return n * n; });
for (int n : result) {
std::cout << n << ' '; // Output: 4 16 36
}
}
This snippet demonstrates how you can first filter even numbers and then square each of them, achieving a highly expressive and concise code structure.
Ranges and Algorithms
Ranges integrate smoothly with the existing algorithms in the Standard Library. This means you can apply traditional algorithms to ranges, enabling a rich set of functionalities. For example, consider sorting a range:
#include <iostream>
#include <vector>
#include <ranges>
#include <algorithm>
int main() {
std::vector<int> nums = {5, 2, 3, 1, 4};
std::ranges::sort(nums);
for (int n : nums) {
std::cout << n << ' '; // Output: 1 2 3 4 5
}
}
This example shows that sorting becomes straightforward and easy to implement, all while working within the range paradigm.
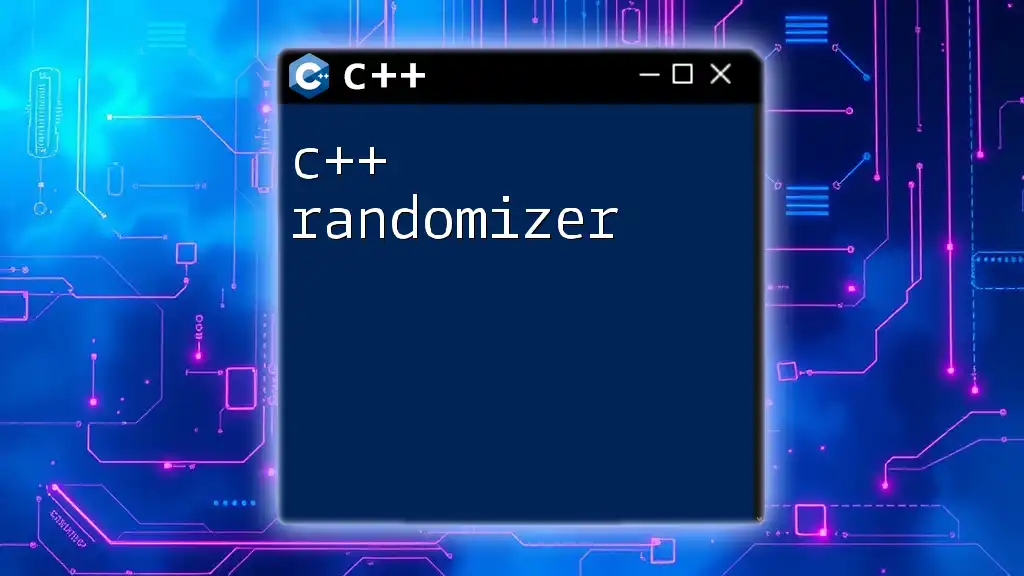
Best Practices
Performance Considerations
While ranges can greatly improve code clarity, it's essential to understand their performance implications. In some scenarios, using ranges could introduce overhead compared to traditional loops and iterators, especially regarding temporary objects. Consider these trade-offs carefully when performance is a critical concern.
Readability and Maintainability
Ranges inherently enhance the readability of code. They allow you to express complex operations in fewer lines, making your intent clear. When you combine multiple operations into a single pipeline, it significantly reduces mental overhead for anyone maintaining or reviewing the code.
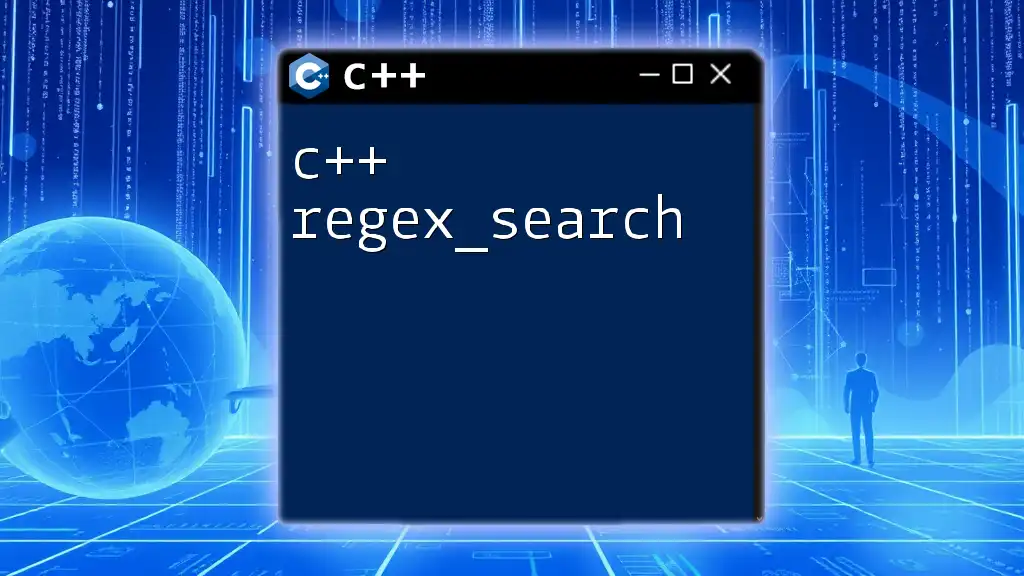
Conclusion
C++17 ranges are a powerful tool designed to simplify and enhance the way you interact with data sequences. They encapsulate complex operations and provide expressive syntax that leads to cleaner code. Embracing ranges can not only speed up development but also yield significant improvements in maintainability.
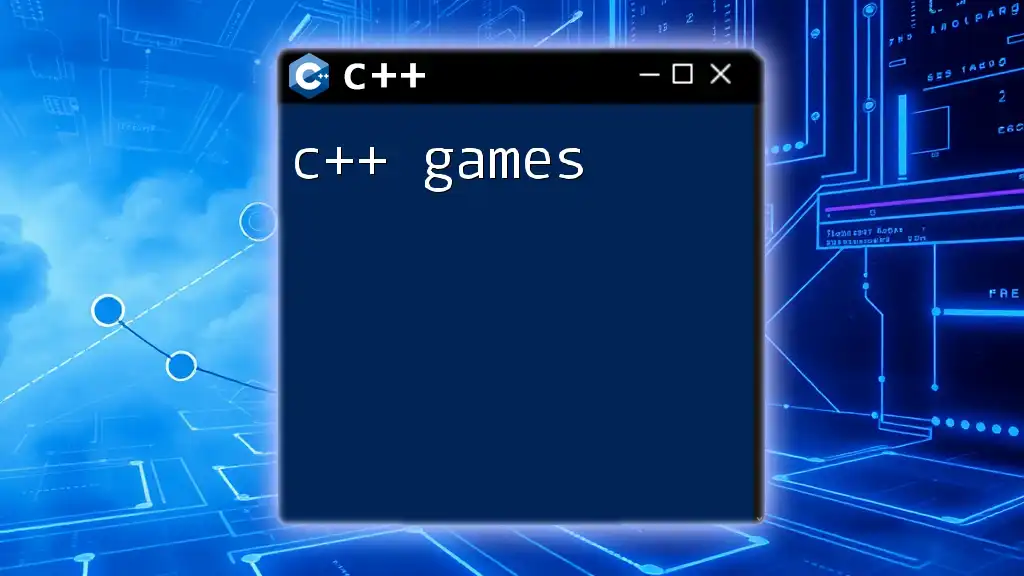
Additional Resources
To continue your journey with C++17 ranges, consider exploring the official C++ documentation and online resources such as C++ reference sites. Additionally, you can check out books dedicated to modern C++ practices or enroll in online courses that provide hands-on experience with C++17 features.
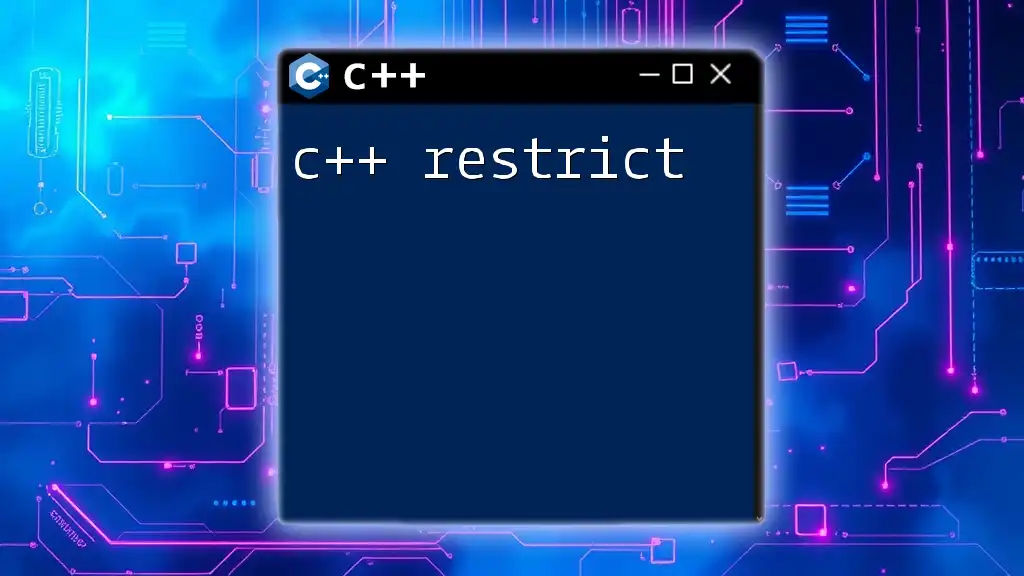
Frequently Asked Questions (FAQs)
What compilers support C++17 ranges?
The most popular compilers, including GCC, Clang, and MSVC, support C++17 features and therefore ranges. Ensure your compiler is updated to take advantage of the latest enhancements.
How can I practice using C++17 ranges?
One effective way to practice is by solving problems on platforms like LeetCode or HackerRank, specifically focusing on collections and algorithms. You can implement solutions using ranges to reinforce your understanding.
Are ranges available in earlier versions of C++?
Ranges are a feature unique to C++17. For earlier versions, you would need to rely on the traditional iterator and algorithm approach, which can be more verbose and less intuitive. However, many concepts can be implemented manually using iterators if needed, though with more boilerplate code.