C++17 with CMake simplifies the process of managing project builds by leveraging modern features like improved initialization and templates, as shown in the following example of a basic CMakeLists.txt configuration:
cmake_minimum_required(VERSION 3.8)
project(MyCPP17Project)
set(CMAKE_CXX_STANDARD 17)
set(CMAKE_CXX_STANDARD_REQUIRED ON)
add_executable(my_app main.cpp)
Setting Up Your Development Environment
Installing C++ Compiler
To get started with C++17 and CMake, the first step is to install a C++ compiler suitable for your platform. There are several popular compilers to choose from, including GCC, Clang, and MSVC. Each compiler has its unique features and compatibility, but they all support C++17.
Installation Steps
-
Linux: On Ubuntu or other Debian-based systems, you can install GCC with:
sudo apt install g++
-
macOS: Use Homebrew to install GCC or Clang:
brew install gcc
-
Windows: You can install MSVC through Visual Studio Installer or install MinGW for GCC support.
Installing CMake
After setting up your compiler, the next crucial step is to install CMake. CMake is a powerful build system that automates the process of building and managing C++ projects.
How to Install CMake
Installation may vary based on the operating system:
-
Linux: On Ubuntu, run:
sudo apt install cmake
-
macOS: Again, use Homebrew:
brew install cmake
-
Windows: Download the installer from the CMake website or use vcpkg.
Verifying CMake Installation
Once CMake is installed, you can verify the installation by checking the version:
cmake --version
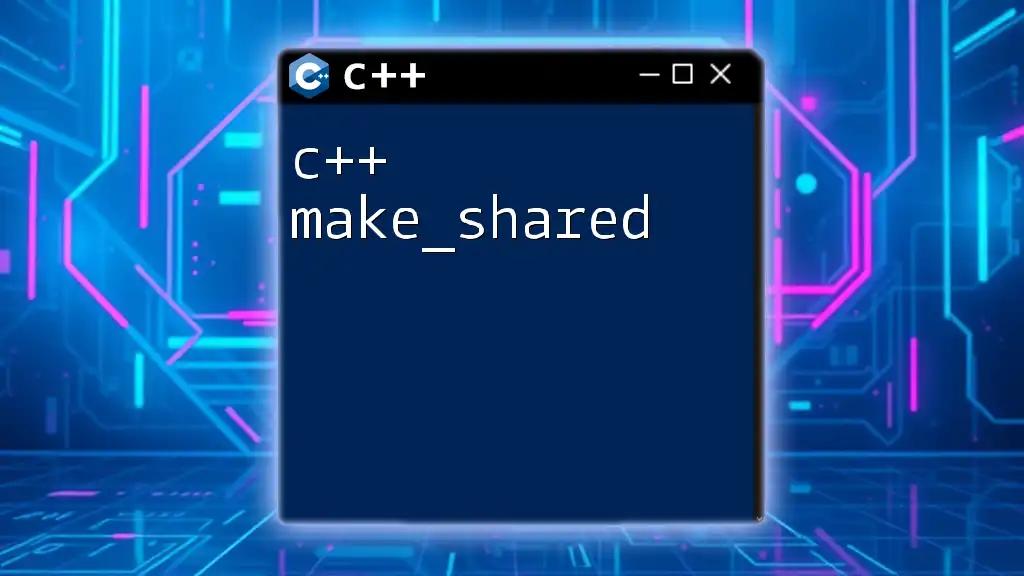
Creating Your First CMake Project
Project Folder Structure
Establishing a clean and organized project structure is essential for any C++17 CMake project. Here's a recommended folder hierarchy:
/YourProjectName
/src
/include
/build
- src: Contains your C++ source files.
- include: Houses your header files.
- build: The directory where CMake generates build files.
Writing a Simple C++17 Program
Let’s create a simple C++ program that outputs "Hello, World!" to the console. Create a file named `main.cpp` inside the `src` folder with the following content:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up CMakeLists.txt
The heart of any CMake project lies in the `CMakeLists.txt` file. In your project's root directory, create a `CMakeLists.txt` file with the following contents:
cmake_minimum_required(VERSION 3.10)
project(HelloWorld)
add_executable(hello_world src/main.cpp)
This basic configuration specifies the minimum CMake version, sets the project name, and defines the executable to be built from `main.cpp`.
Building the Project with CMake
Now you’re ready to build your project. Navigate to the `build` directory within your project structure and run the following commands:
mkdir build
cd build
cmake ..
make
- The `cmake ..` command configures the project.
- The `make` command compiles the code.
If everything goes smoothly, you will have an executable named `hello_world` created in your `build` directory.
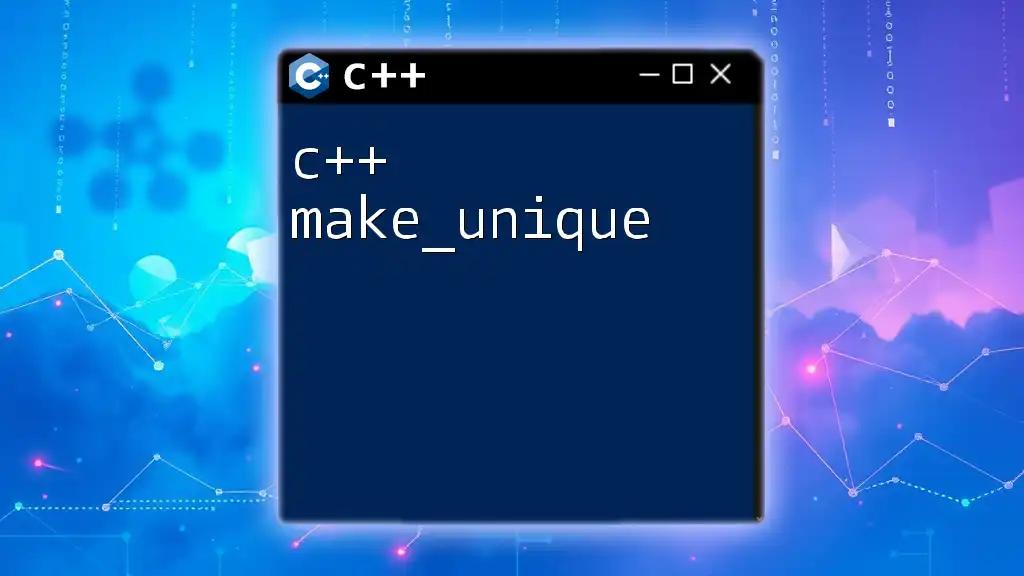
Exploring C++17 Features
New Language Features
C++17 introduced several significant language features that enhance the language's usability and performance.
Structured Bindings
One of the exciting features is Structured Bindings, which allows you to unpack tuple-like objects into multiple variables easily. Here's a simple example:
#include <tuple>
std::tuple<int, double> getValues() {
return {1, 2.5};
}
int main() {
auto [x, y] = getValues(); // x is 1, y is 2.5
}
Inline Variables
C++17 also allows you to declare inline variables, which can simplify your code and reduce the need for header guards.
Standard Library Improvements
C++17 brings improvements to the Standard Library, making it more powerful and versatile.
Optional and Variant Types
The introduction of `std::optional` and `std::variant` is significant for improving code safety and expressiveness.
For example, `std::optional` can be used to indicate that a variable may or may not hold a value:
#include <optional>
std::optional<int> maybeValue;
if (maybeValue) {
// Optional value is present
}
Parallel Algorithms
C++17 has introduced Parallel Algorithms that simplify parallel programming in C++. You can now use `std::for_each` with execution policies.
Here’s a quick example that multiplies each element in a vector by 2 in parallel:
#include <algorithm>
#include <execution>
#include <vector>
std::vector<int> v{1, 2, 3, 4, 5};
std::for_each(std::execution::par, v.begin(), v.end(), [](int& n) { n *= 2; });
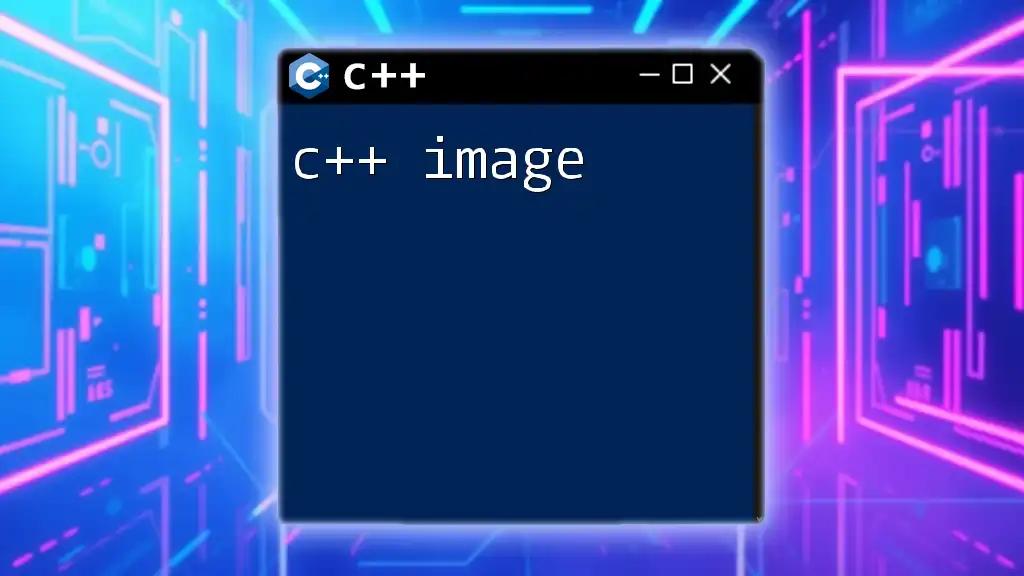
Advanced CMake Features
Adding Libraries
CMake lets you manage libraries easily, whether they are static or shared. For instance, to create a static library, you can modify your `CMakeLists.txt` like this:
add_library(mylib STATIC src/mylibrary.cpp)
Then link your library with an executable:
add_executable(hello_world src/main.cpp)
target_link_libraries(hello_world mylib)
Using CMake Modules
CMake allows for easy integration of external libraries with commands like `find_package()`. For example, integrating Boost might look like this:
find_package(Boost REQUIRED)
target_link_libraries(hello_world Boost::Boost)
Custom Build Targets
CMake also allows the definition of custom commands and targets for your projects. This functionality is useful for running tests or other scripts. Integrating tests can be managed easily with CTest.
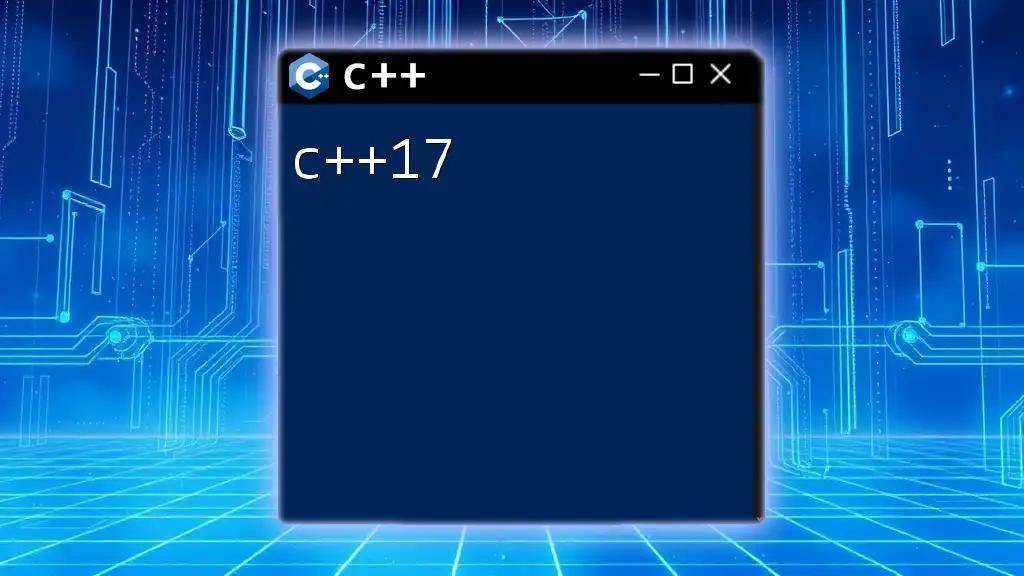
Best Practices in CMake
Keep CMakeLists.txt Clean
A clean `CMakeLists.txt` is crucial for maintainability. Use comments, blank lines, and well-structured layouts to keep your configuration readable. Modularizing your CMake files into separate directories can also help manage complexity.
Version Control with CMake
When using version control systems, it's essential to include only necessary files. Typically, you should avoid checking in the `build` directory since it contains generated files. Instead, add this to your `.gitignore`:
/build/
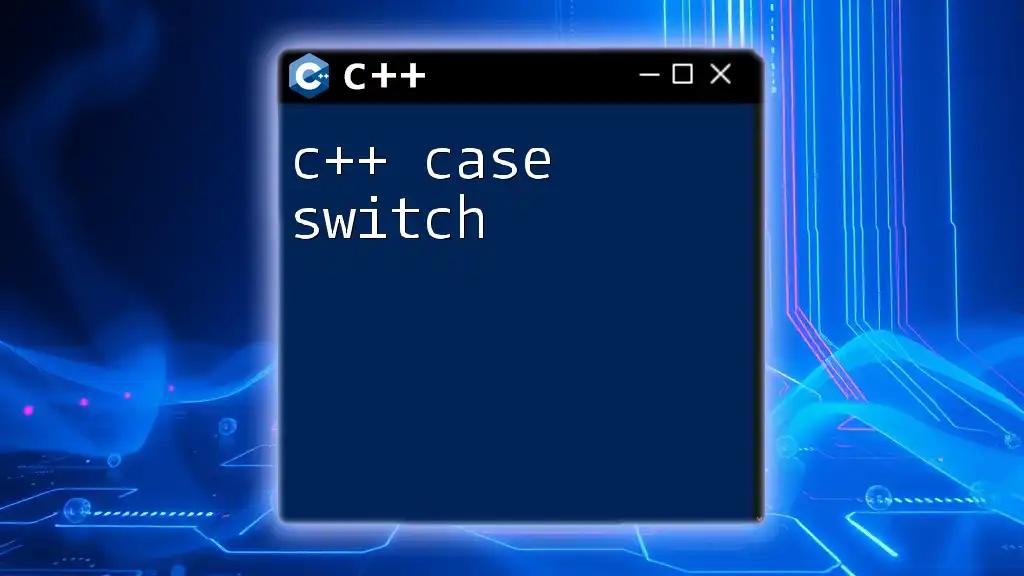
Conclusion
C++17, combined with CMake, offers a powerful framework for development, enabling developers to leverage modern features and an effective build system. By understanding the basics of CMake and exploring the innovative features of C++17, you are well on your way to creating robust and efficient C++ applications.
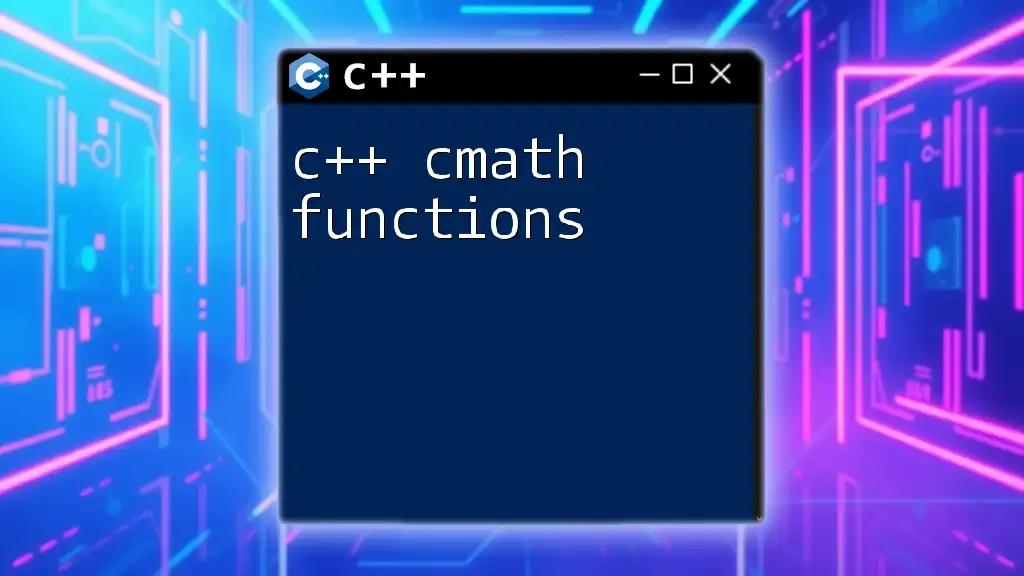
Resources
For those looking to deepen their understanding, consider exploring books specifically focused on C++17 features and CMake. The official CMake documentation and C++17 standard library documentation will serve as invaluable references during your coding journey.
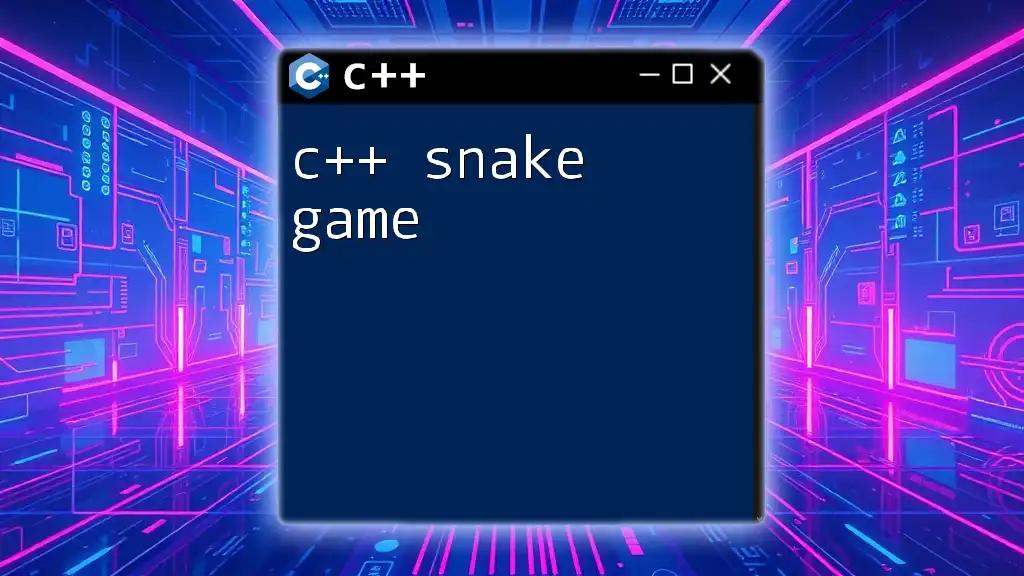
Call to Action
Now is the time to dive into C++17 with CMake. Start creating your projects, and feel free to share your experiences or any projects you've developed in the comments section below! Happy coding!