In C++, the `std::make_unique` function is used to create a unique pointer to an object, ensuring proper memory management and preventing memory leaks by automating the deallocation of the object when it goes out of scope.
#include <memory>
int main() {
auto myUniquePtr = std::make_unique<int>(42); // Creates a unique pointer to an integer with value 42
return 0;
}
Understanding Smart Pointers
What Are Smart Pointers?
Smart pointers are automated memory management tools that help developers manage dynamic memory more effectively and safely. Unlike raw pointers, which require manual memory deallocation using `delete`, smart pointers automatically handle resource cleanup when they are no longer in use.
The primary types of smart pointers in C++ include:
- `std::unique_ptr`: Represents exclusive ownership of an object, ensuring that only one `unique_ptr` can point to a resource at a time.
- `std::shared_ptr`: Allows multiple pointers to share the ownership of a single resource, maintaining a reference count to manage its lifetime.
- `std::weak_ptr`: A companion to `shared_ptr` that provides access to an object without affecting its reference count, preventing cyclic dependencies.
Why Use Smart Pointers?
Using smart pointers offers numerous benefits over traditional raw pointers. These benefits include:
- Memory Management Benefits: Smart pointers automatically deallocate memory when they go out of scope, significantly reducing the likelihood of memory leaks.
- Avoiding Memory Leaks: By automatically managing a pointer's lifetime, smart pointers help prevent scenarios where memory remains allocated after it is no longer needed.
- Exception Safety: Smart pointers ensure that resources are released even when exceptions occur, increasing the robustness of your code.
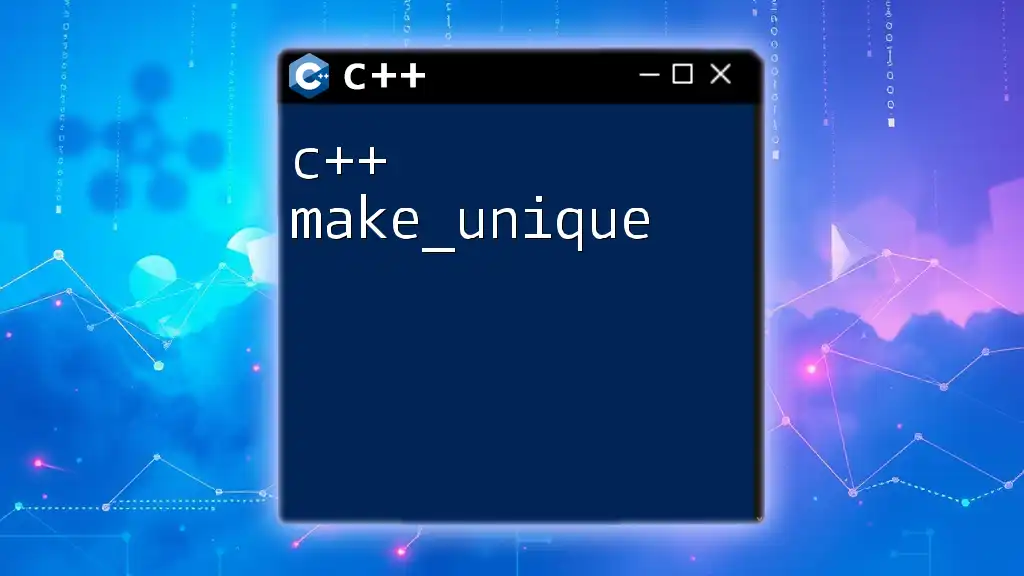
What is `std::make_unique`?
Introduction to `std::make_unique`
`std::make_unique` is a factory function introduced in C++14 that creates a `std::unique_ptr`. It allocates memory for an object and returns ownership of that memory in a `unique_ptr`. This helps prevent common memory management errors, such as memory leaks, which can occur when using raw pointers directly.
Key Difference: While you could allocate memory using `new` and then wrap it in a `std::unique_ptr`, `make_unique` simplifies this process by condensing both steps into one clean function call.
Syntax of `std::make_unique`
The syntax for using `std::make_unique` involves specifying the type and any constructor arguments:
std::unique_ptr<Type> ptr = std::make_unique<Type>(args...);
Here is a simple example that demonstrates its use:
auto uniquePtr = std::make_unique<int>(42); // Creates a unique_ptr managing an integer
In this example, `uniquePtr` is a unique pointer to an integer initialized with the value `42`. The memory will automatically be deallocated when `uniquePtr` goes out of scope.
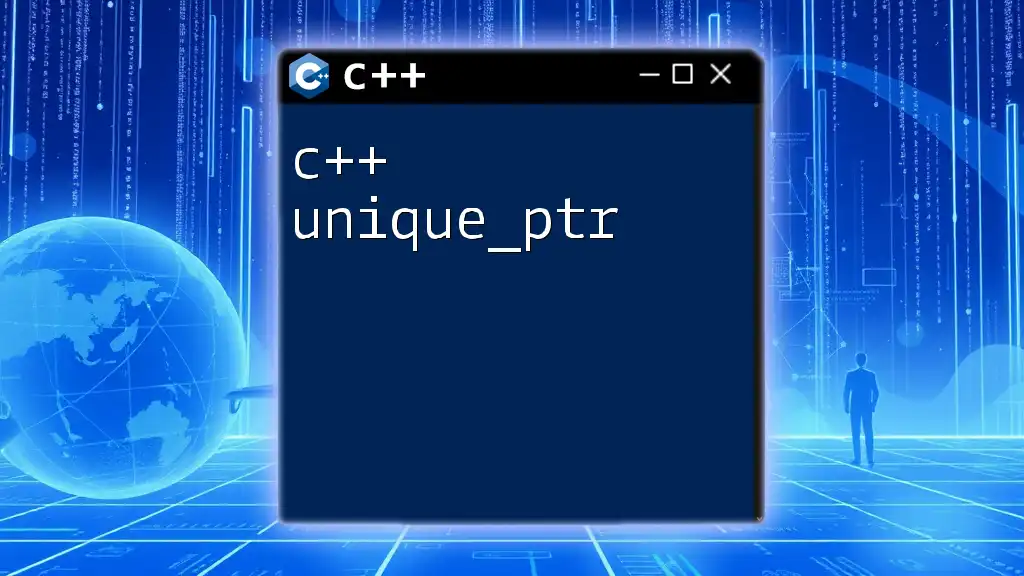
Benefits of Using `std::make_unique`
Automatic Resource Management
One of the most significant benefits of `std::make_unique` is its automatic resource management. When a `unique_ptr` is created using `make_unique`, it automatically takes over the management of the memory allocated for the object. When the `unique_ptr` goes out of scope (e.g., when the function ends), the memory is automatically released without any extra overhead, minimizing the risk of memory leaks.
Exception Safety
Exception safety is another key advantage. If an exception occurs after the allocation but before the pointer is used, the memory will still be properly released. Consider the following example:
void functionWithException() {
auto ptr = std::make_unique<int>(10);
throw std::runtime_error("Exception occurred");
// ptr will be automatically cleaned up
}
In this example, even though an exception is thrown, the memory managed by `ptr` is automatically released, preventing a memory leak.
Enhanced Readability and Maintainability
Using `std::make_unique` improves code readability and maintainability. The intent is clear: you are creating a unique pointer to manage a particular resource. In contrast, using raw pointers requires a more verbose and error-prone approach. Here’s a comparison:
// Raw pointer approach
int* rawPtr = new int(42);
// ... perform operations on rawPtr
delete rawPtr; // Manual memory deallocation
// Unique_ptr approach
auto uniquePtr = std::make_unique<int>(42); // Memory management handled automatically
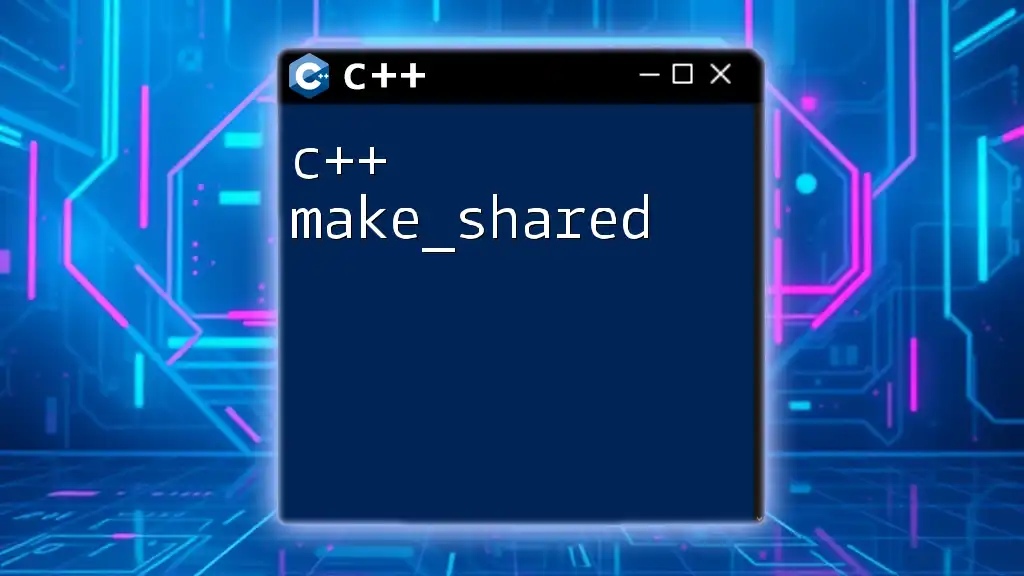
Common Use Cases for `std::make_unique`
Dynamic Memory Allocation
`std::make_unique` is particularly beneficial for dynamic memory allocation. It simplifies the creation and management of objects allocated on the heap, allowing for cleaner code without the overhead of manual memory management.
For instance, you can create a simple structure like this:
struct MyStruct {
int data;
MyStruct(int d) : data(d) {}
};
auto myStructPtr = std::make_unique<MyStruct>(5);
In this case, `myStructPtr` is a `unique_ptr` that takes ownership of a `MyStruct` object initialized with `5`.
Managing Arrays and Custom Types
`std::make_unique` can also be used effectively with arrays, making it possible to manage dynamically allocated arrays with unique ownership. For example:
auto arrPtr = std::make_unique<int[]>(10); // Array of 10 integers
Here, `arrPtr` manages an array of integers, and memory will be released automatically when it goes out of scope.
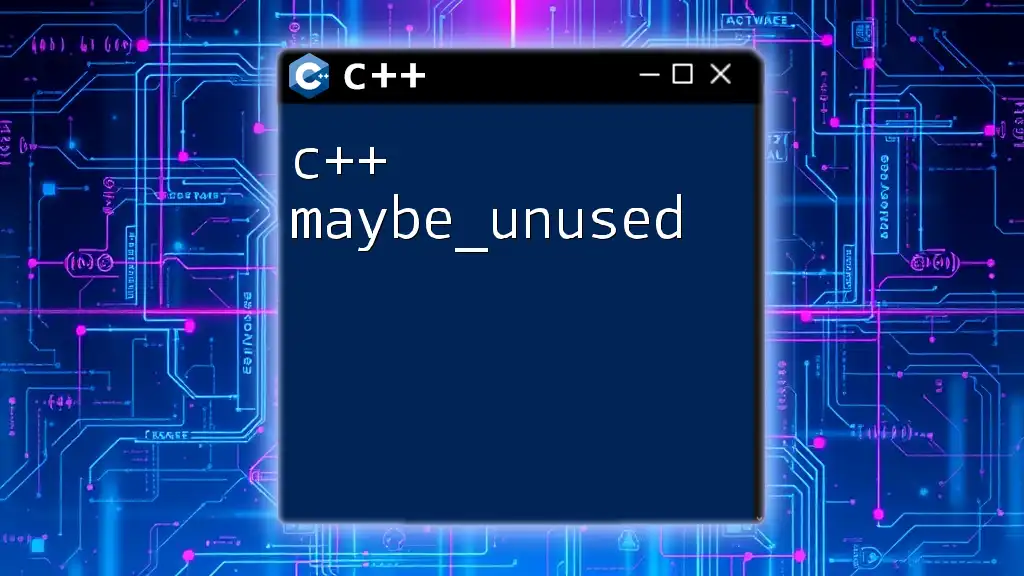
When Not to Use `std::make_unique`
Limitations and Considerations
While `std::make_unique` is powerful, there are scenarios where it may not be the best fit. For instance, when you need to share ownership of a resource, `std::shared_ptr` is more appropriate. This is especially relevant in large applications where the same resource might be used in multiple contexts.
Legacy Code and Compatibility Issues
For projects written in earlier versions of C++, `std::make_unique` may not be available. In such cases, you can resort to using raw pointers or `std::unique_ptr` directly with `new`, but you must remember to manually manage memory cleanup.
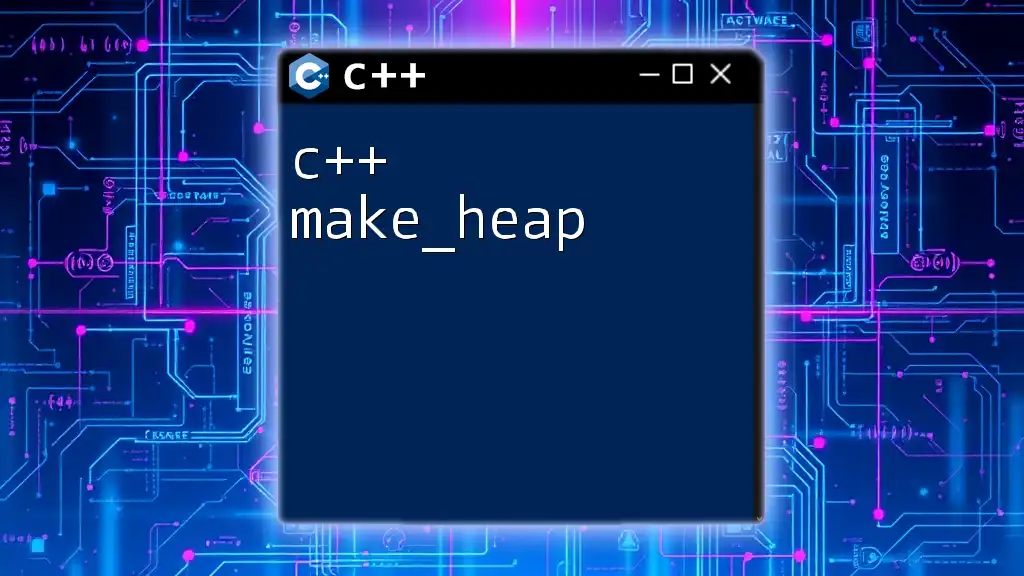
Best Practices for Using `std::make_unique`
Consistency in Code Style
Maintaining a consistent coding style is crucial for team projects. Encourage the use of `std::make_unique` throughout your codebase to unify memory management approaches. This consistency makes it easier for developers to read and understand the code.
When to Prefer Smart Pointer to Raw Pointers
Use smart pointers like `std::make_unique` whenever you would otherwise consider using raw pointers, particularly when dynamic memory allocation is involved. In scenarios where specific ownership semantics are not required, prefer using `std::vector` or similar containers, which manage their own memory.
Combining `make_unique` with STL Containers
You can also store unique pointers in STL containers, further enhancing their utility. Here's an example:
std::vector<std::unique_ptr<MyStruct>> vec;
vec.push_back(std::make_unique<MyStruct>(10));
This example demonstrates how to manage a collection of unique pointers, making memory management both convenient and safe.
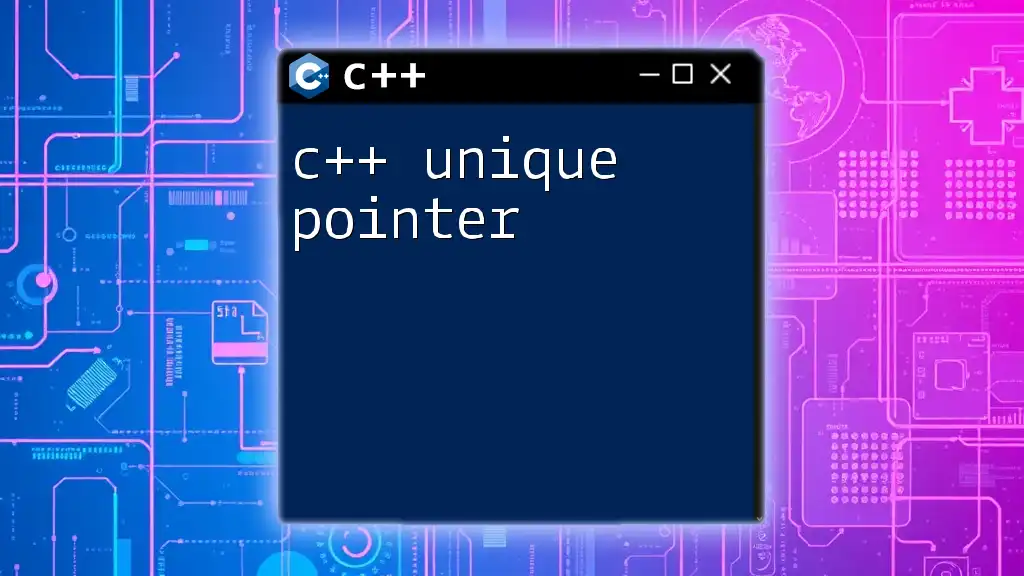
Conclusion
In summary, `std::make_unique` is an invaluable tool in modern C++ programming, providing robust and efficient memory management. By leveraging this function, developers can write safer, more maintainable code while avoiding common pitfalls related to raw pointer management. Embrace smart pointers in your projects, practice their usage, and enhance your C++ programming skills.