In C++, "require" isn't a built-in command; however, you can create a custom function to enforce specific conditions, which can be formally implemented through assertions to ensure certain criteria are met at runtime.
Here's an example code snippet using `assert` to illustrate this concept:
#include <cassert>
int main() {
int value = 10;
assert(value > 0 && "Value must be greater than zero");
return 0;
}
What Does C++ Require Mean?
In the context of C++, `require` refers to a concept that emphasizes the necessity of certain conditions to be satisfied prior to executing functions or templates. This concept has evolved with the introduction of concepts in C++20, where `requires` plays a crucial role in ensuring that types conform to specified criteria, thereby enhancing type safety and logical flow in your code.
Key distinctions exist between traditional programming conditions and the modern use of `requires`. The latter offers a robust mechanism for validating type compatibility, significantly improving null checks or type-based computations where type correctness before execution is paramount.
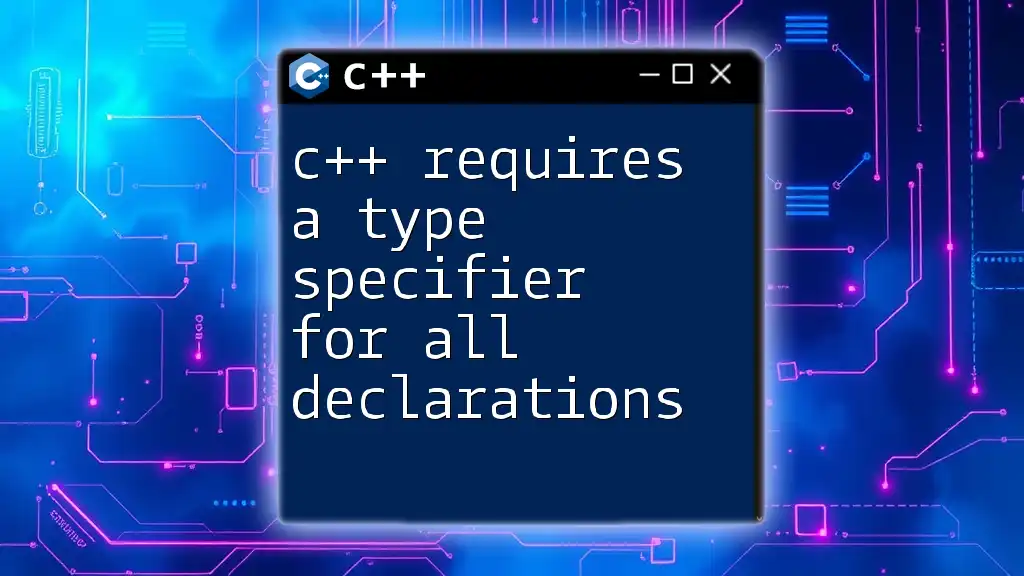
The Basics of C++ Requires
What is the Purpose of Requires?
The main purpose of the C++ requires clause is to define preconditions that must be met for a function to be executed. This is particularly useful in template programming, where different types might be passed to a function, and ensuring type safety beforehand can prevent runtime errors.
By using `requires`, you make it clear what types are acceptable, contributing to easier code readability and maintainability. For example, when you have a function that performs operations specifically designed for integral data types, utilizing `requires` ensures that you won't inadvertently pass floating-point numbers, which might lead to incorrect computations.
Syntax of C++ Requires
Understanding the grammar behind requires clauses is critical. The syntax primarily revolves around defining concepts that specify the conditions required for a template type.
The simplest way to declare a concept is:
template<typename T>
concept Integral = std::is_integral_v<T>;
In this code snippet, we define a concept named Integral which checks if type T is an integral type. The usage of `std::is_integral_v` from the STL (Standard Template Library) ensures that this condition is evaluated at compile time.
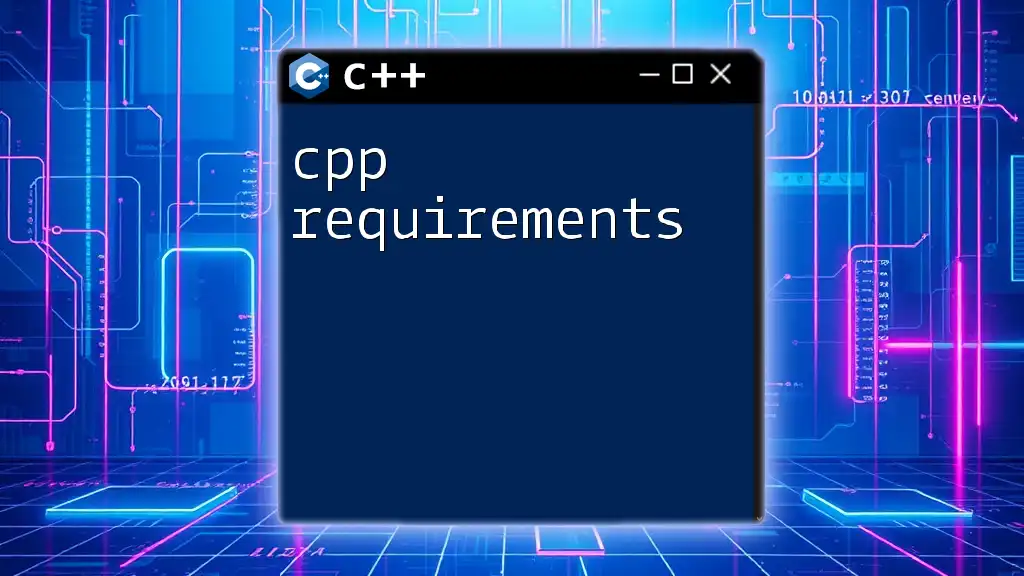
Practical Applications of C++ Requires
Designing Templated Functions
One of the fantastic aspects of using C++ requires is the clarity it brings when designing templated functions. By enumerating the types that the function can handle, you can ensure type correctness right at the point of instantiation.
For instance, consider a templated function:
template<typename T>
requires Integral<T>
T add(T a, T b) {
return a + b;
}
In this example, the `add` function is designed to only accept integral types as parameters. Any attempt to call `add` with a non-integral type will result in a compilation error, not only providing immediate feedback during development but also creating a safer codebase.
Concept Checking with Requires
Concept checking is another powerful feature of C++ requires that supports the checks of conditional requirements that must be met. This is particularly useful in library development where functions may need to have constraints over their input parameters.
Consider this example:
template<typename T>
void process(T value) requires Integral<T> {
// Function logic here
}
In this snippet, the `process` function can only operate if the type T is integral. This ensures that any operations within the function that assume an integral type can be performed safely.
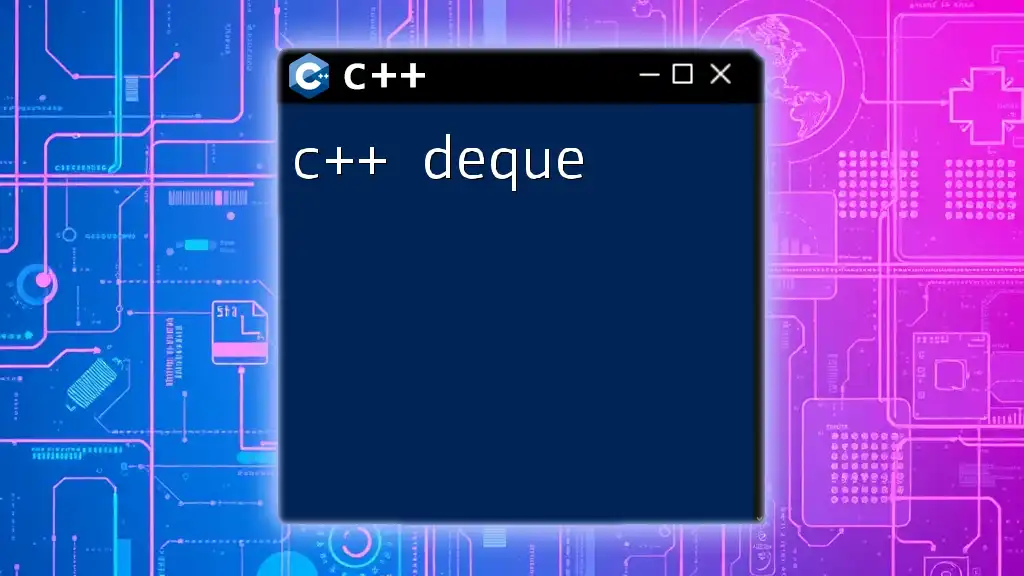
Advanced Usage of C++ Requires
Combining Requires with Other Features
While C++ requires shines on its own, it can also be combined with other C++ features like `std::enable_if` to create even more complex type constraints. This flexibility allows you to integrate older style practices while leveraging the newer `requires` mechanism.
For instance:
template<typename T, typename = std::enable_if_t<Integral<T>>>
void safeMultiply(T a, T b) {
// safety logic
}
In this example, we use `std::enable_if` to ensure that the function can only execute if T is an integral type. While this is valid, using `requires` is often a more readable and cleaner alternative.
Performance Implications of Using Requires
The use of C++ requires can lead to significant efficiency improvements, especially in larger codebases where compile-time checks reduce the risk of runtime failures. By constraining types and conditions upfront, the compiler can optimize the generated code, ultimately resulting in better performance.
However, it’s essential to recognize potential trade-offs; overusing heavy constraints or excessively complex concepts could lead to longer compile times. Hence, developers must find a balance to harness the full power of C++ requires without sacrificing efficiency.
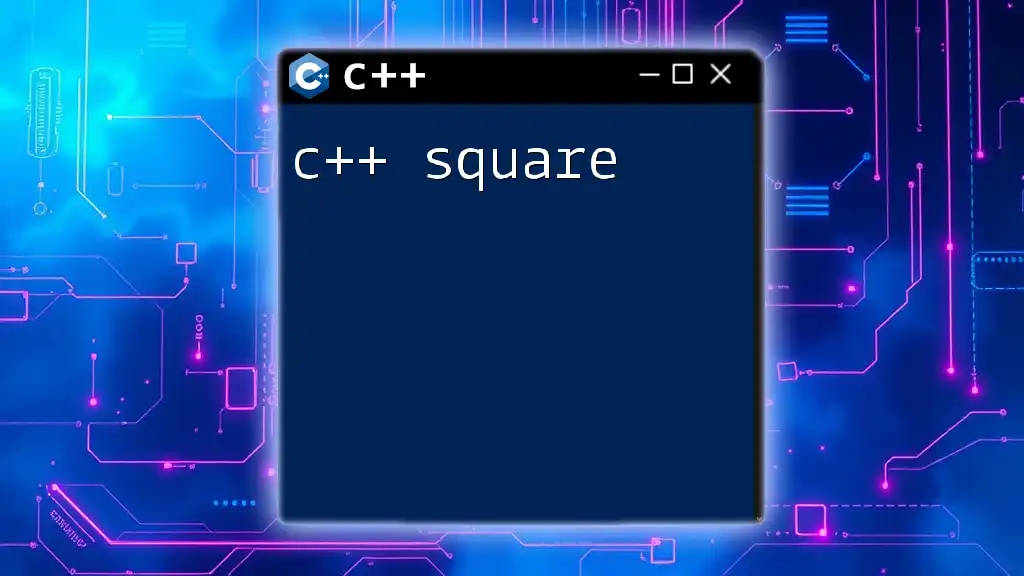
Common Pitfalls and Best Practices
Common Mistakes Made with Requires
One area where developers may falter is the misunderstanding of concepts themselves, leading to incorrectly defined requires clauses. For example, failing to specify appropriate conditions or using concepts that are too broad could render the function usable with unwanted types.
Always ensure that your constraints are as specific as necessary to prevent misuse while keeping the function flexible.
Best Practices for Utilizing C++ Requires
To get the most out of C++ requires, here are some best practices:
- Keep concepts simple: Start with basic constraints before building more complex ones.
- Be explicit with conditions: Define requirements clearly, which enhances code readability.
- Document your concepts: Commentary on the purpose of each concept can save time for future developers.
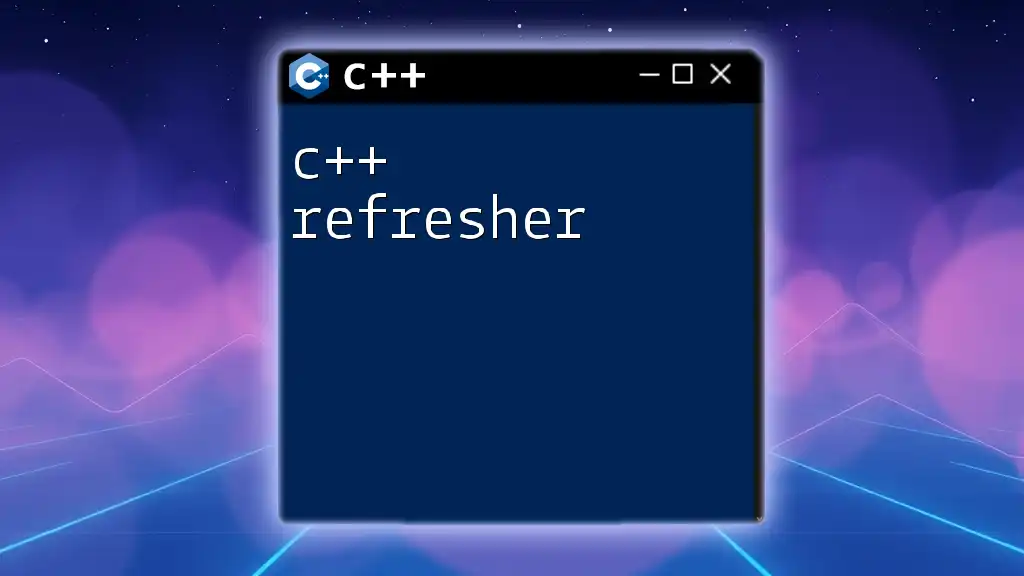
Limitations of C++ Requires
While C++ requires is powerful, it has limitations. One notable limitation is that it cannot replace runtime checks. There are certain scenarios where type checks must occur during execution, and a `requires` clause won’t suffice.
Moreover, complex types or conditions can lead to difficulties in debugging compile-time errors, potentially confusing developers less familiar with template metaprogramming.
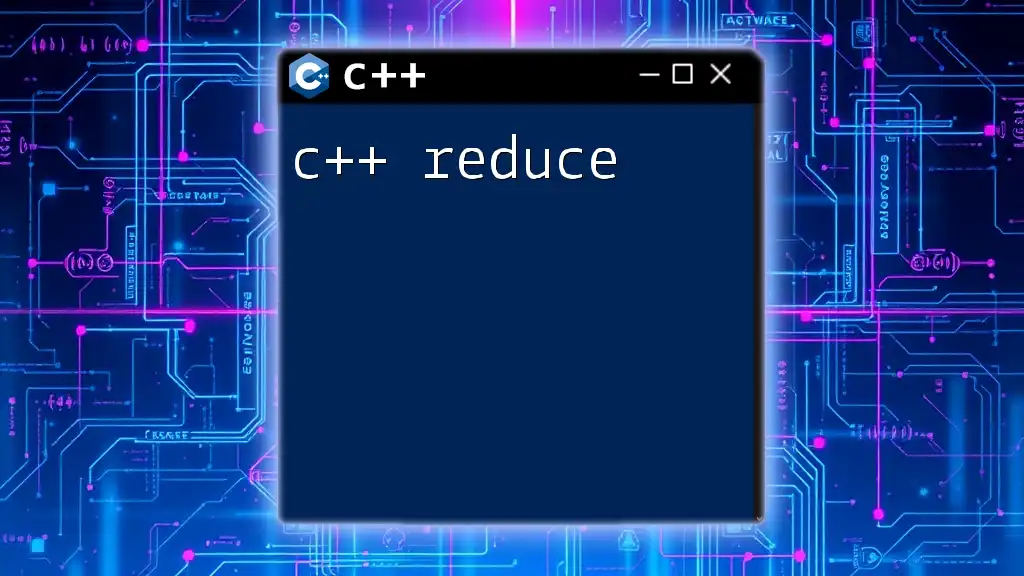
Conclusion
C++ requires is a transformative feature that elevates the safety and clarity of C++ programming. By imposing precondition validations at compile time, it minimizes runtime errors and enhances your code quality. Embracing this feature will enable you to craft more robust, type-safe functions and templates, and ultimately lead to better software performance.
As you gain more experience with C++ requires, experimentation and practice will be key. Don’t hesitate to integrate this powerful feature into your projects and see firsthand the benefits it brings to your development workflow.