In C++, you can calculate the square of a number using a simple function that multiplies the number by itself. Here's a code snippet to demonstrate this:
#include <iostream>
using namespace std;
int square(int num) {
return num * num;
}
int main() {
int number = 5;
cout << "The square of " << number << " is " << square(number) << endl;
return 0;
}
Understanding the Basics of C++
C++ is a powerful, high-level programming language widely used in various domains, including system/software development, game programming, and scientific computing. One of the key advantages of C++ is its efficiency, which makes it suitable for performing various mathematical computations, such as squaring numbers.
Why Learn C++ for Mathematical Operations
Learning C++ equips you with the tools to create efficient algorithms. Mathematical operations like squaring are fundamental in programming, serving as building blocks for more complex functionalities. By mastering these basic operations, you’ll be well on your way to tackling more intricate problems.

How to Square Something in C++
Squaring a number involves multiplying the number by itself. In programming, this fundamental operation can be easily implemented in various ways. Let's dive into the methods you can use to perform squaring in C++.
Simple Squaring Using Multiplication
One of the most straightforward methods to square a number in C++ is by using direct multiplication. This approach is clear and concise.
Code Snippet: Squaring Through Direct Multiplication
#include <iostream>
int main() {
int num = 5;
int square = num * num;
std::cout << "The square of " << num << " is " << square << std::endl;
return 0;
}
In the code above:
- We declare an integer `num` and assign it a value of `5`.
- The variable `square` is calculated by multiplying `num` by itself.
- The result is displayed using `std::cout`, which prints "The square of 5 is 25."
Using Functions to Square Numbers
Functions are a core concept in C++, allowing for code reusability and improved organization. Defining a function to square numbers can help streamline your code, especially when you need to perform this operation multiple times.
Creating a Square Function
Code Snippet: Function to Calculate Square
#include <iostream>
int square(int num) {
return num * num;
}
int main() {
int number = 6;
std::cout << "The square of " << number << " is " << square(number) << std::endl;
return 0;
}
Here’s what’s happening in this example:
- We define a function named `square`, which takes an integer parameter and returns its square.
- In the `main()` function, we call `square()` with `6` as an argument, and it returns `36`, which is then printed.
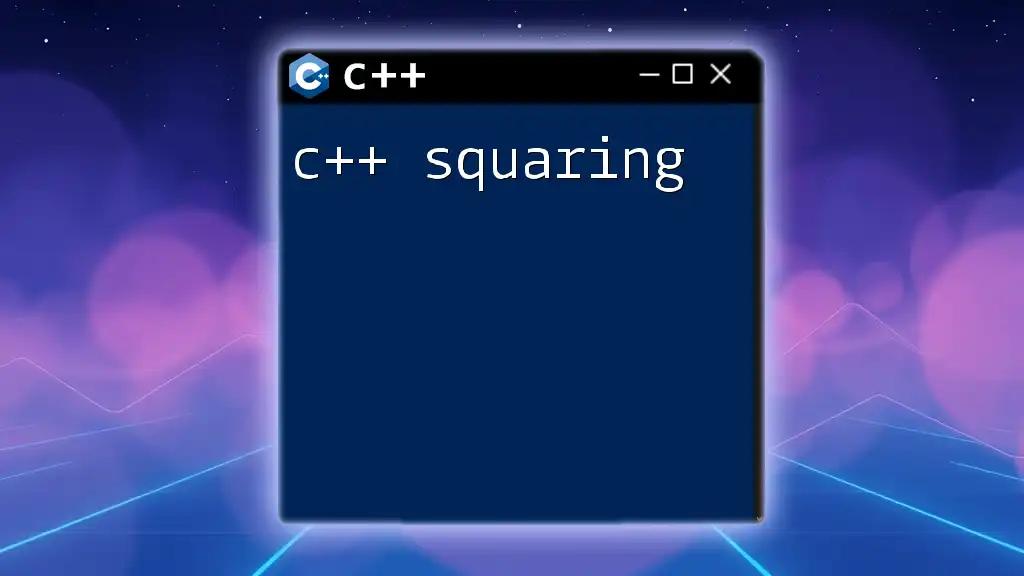
More Advanced Techniques in C++
In addition to basic multiplication, C++ offers various ways to accomplish squaring through library functions and templates.
Using the Power Function from cmath
C++ includes a powerful library called `cmath`, which allows you to perform mathematical computations through various built-in functions, including the `pow()` function.
Code Snippet: Using pow() to Square a Number
#include <iostream>
#include <cmath>
int main() {
double num = 7.0;
double square = pow(num, 2);
std::cout << "The square of " << num << " is " << square << std::endl;
return 0;
}
In this example:
- We include the `cmath` library to access mathematical functions.
- The `pow()` function calculates the square of `num` by raising it to the power of `2`. This method is particularly advantageous when working with larger, more complex calculations.
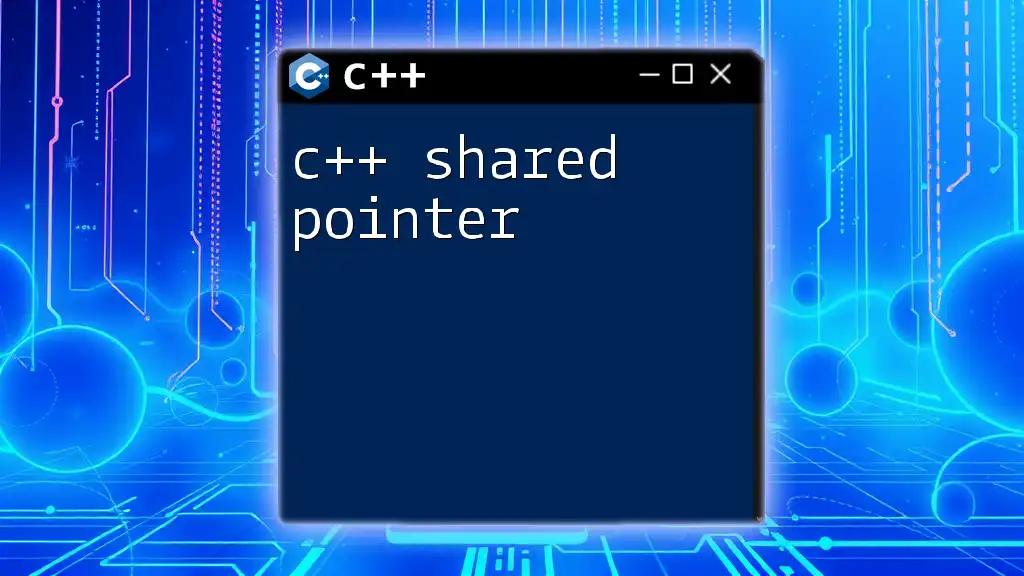
Squaring in C++ Using Templates
Templates in C++ allow functions and classes to operate with any data type. This feature offers tremendous flexibility, particularly when squaring numbers of different types, such as integers and floating points.
Code Snippet: Template Function for Squaring
#include <iostream>
template <typename T>
T square(T num) {
return num * num;
}
int main() {
std::cout << "Square of 5: " << square(5) << std::endl; // int
std::cout << "Square of 5.5: " << square(5.5) << std::endl; // double
return 0;
}
Here, we define a template function named `square`, which accepts any data type `T`. This allows us to square both integers and floating-point numbers using a single function, demonstrating the power and flexibility of C++ templates.
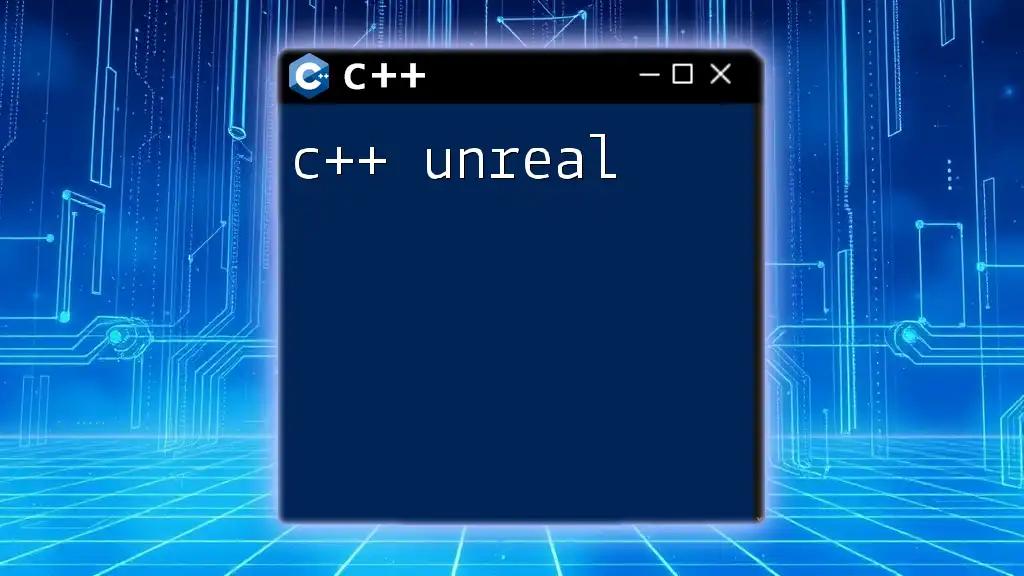
Practical Applications of Squaring in C++
Squaring numbers is not just a mathematical exercise; it has valuable applications in various fields.
Common Applications of Squaring in Programming
- Graphics Programming: Squaring is often used to calculate distances in 2D space, helping to determine object placements and movements.
- Physics Simulations: Squaring plays a vital role in calculating trajectories and forces.
- Machine Learning: Algorithms often employ squaring to calculate errors and optimize weights.
Example: Calculating Distance in a 2D Space
Code Snippet: Using Square to Calculate Distance
#include <iostream>
#include <cmath>
double distance(double x1, double y1, double x2, double y2) {
return sqrt(pow(x2 - x1, 2) + pow(y2 - y1, 2));
}
int main() {
std::cout << "Distance between points (1, 2) and (4, 6) is " << distance(1.0, 2.0, 4.0, 6.0) << std::endl;
return 0;
}
In this scenario:
- We are calculating the distance between two points (1,2) and (4,6).
- The equation utilizes squaring as a part of the distance formula in Euclidean space, demonstrating how essential squaring is in practical applications of C++.
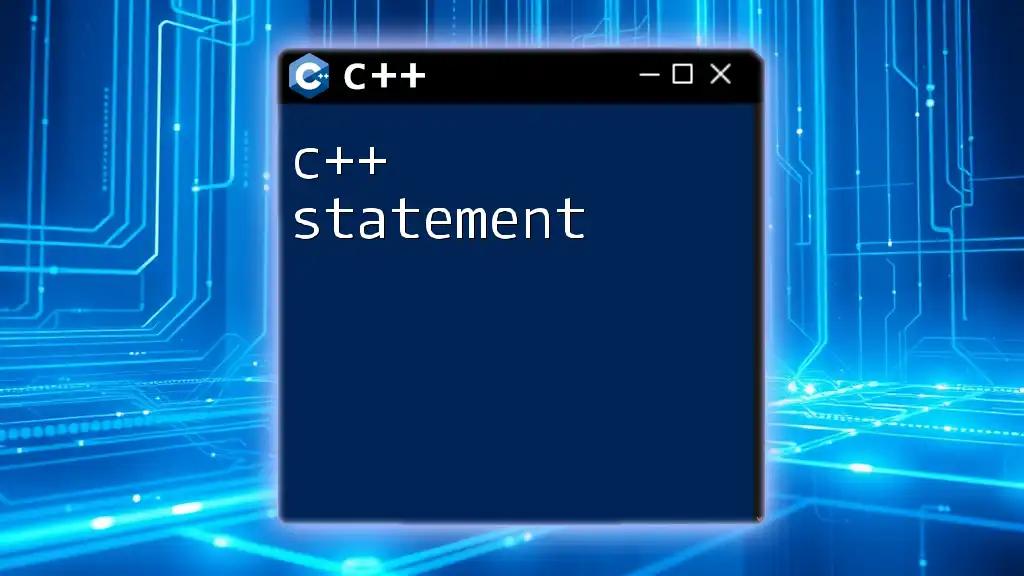
Conclusion
In this guide, we explored several methods to perform the C++ square operation, from simple multiplication and function definitions to using the powerful `cmath` library and templates. Understanding these techniques not only enhances your mathematical programming skills in C++ but also prepares you for more complex algorithms and applications in various fields. With this foundational knowledge, you're equipped to tackle more intricate programming challenges and maximize the efficiency of your C++ projects.