In C++, the equality operator (`==`) is used to compare two values for equality, returning `true` if they are equal and `false` otherwise.
#include <iostream>
int main() {
int a = 5;
int b = 5;
if (a == b) {
std::cout << "a is equal to b" << std::endl;
} else {
std::cout << "a is not equal to b" << std::endl;
}
return 0;
}
Understanding the Equals Operator in C++
What is the Equals Operator?
The equals operator in C++ is denoted by the symbol `=`. Its primary function is to assign values to variables. Understanding this operator is crucial as it serves as the fundamental building block for variable manipulation and data handling in C++.
The Role of the Equals Operator in Variable Assignment
The equals operator allows you to create a relationship between a variable and a value, effectively storing that value in the location allocated for the variable in memory.
For example, consider the following code:
int x = 10; // Assigning the value 10 to x
In this case, `10` is assigned to the variable `x`. This means that whenever you reference `x` later in your program, it directly reflects the value `10`.
The Equals Operator versus Comparison Operators
It is essential to differentiate between the equals operator (`=`) and the comparison operator (`==`). The equals operator is used for assignment, while the comparison operator checks whether two values are equal.
Using them incorrectly can lead to subtle bugs in your code that are often difficult to track down. Here’s a simple illustration of their differences:
int a = 5; // Assignment using the equals operator
if (a == 5) { // Comparison using the equality operator
// Code to execute if a is equal to 5
}
Notably, beginners often confuse these two operators, leading to logic errors where they intend to compare values but accidentally assign them instead.
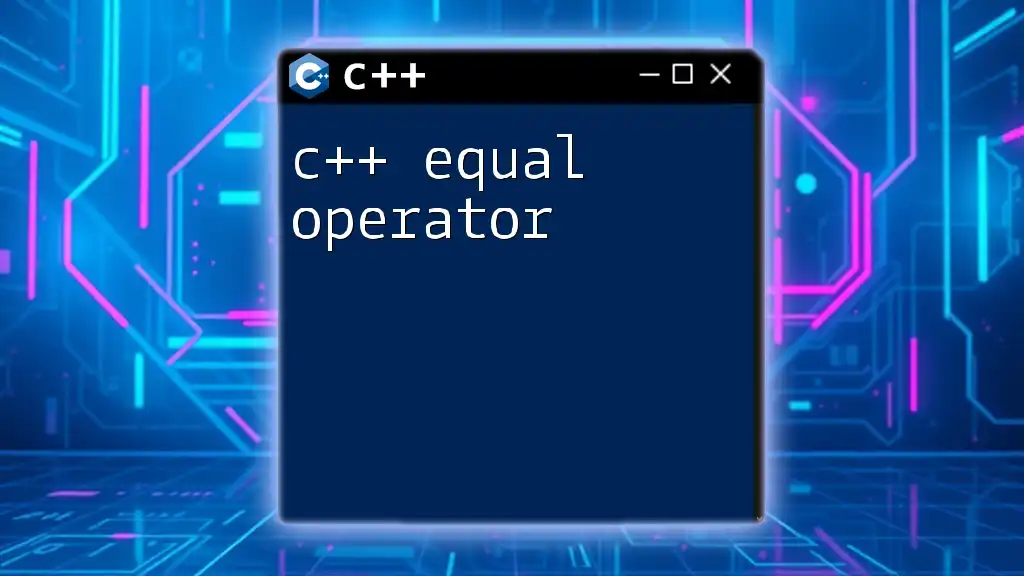
Using the Equals Operator in Different Scenarios
Assigning Values to Different Data Types
The equals operator can be used with various data types, including integers, floats, characters, and booleans. Understanding how to utilize this operator effectively with different types ensures robust programming.
Here are a few examples showcasing assignments across different data types:
float price = 49.99f; // Assigning a float
char initial = 'A'; // Assigning a char
bool isAvailable = true; // Assigning a boolean
Each of these examples highlights how the equals operator serves to link a variable with a specific value of the respective type.
Assigning Values to Objects
When it comes to Object-Oriented Programming (OOP) in C++, the equals operator also assigns values to class instance variables. Like primitive types, when utilized with instances of classes, the equals operator sets specific values to the object's attributes.
For example, consider the following code snippet:
class Person {
public:
std::string name;
int age;
};
// Creating an object and assigning values
Person john;
john.name = "John Doe"; // Assigning values to object's attributes
john.age = 30;
In this scenario, `john.name` and `john.age` are assigned specific values demonstrating how objects manage their internal states.
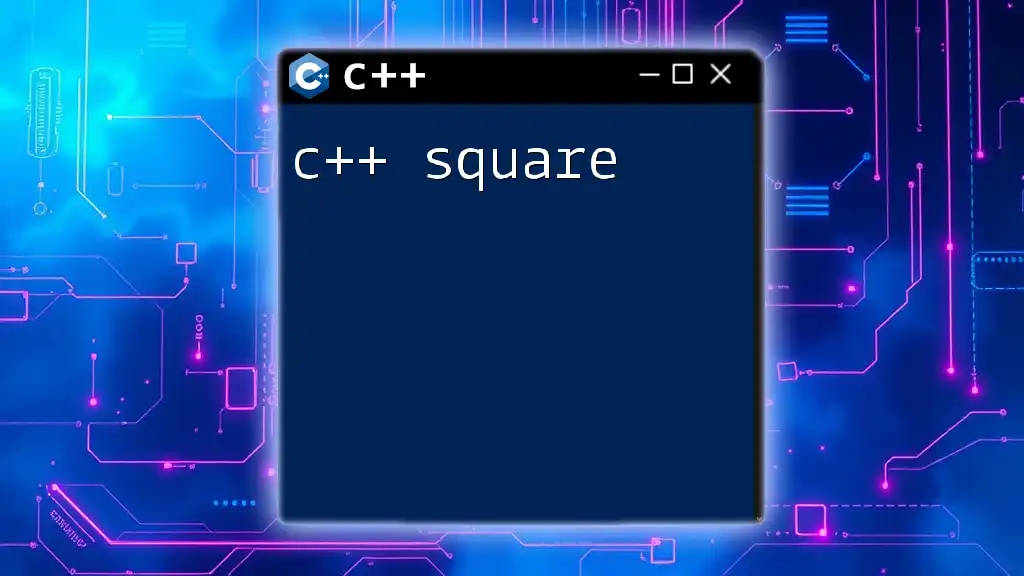
The Equals Operator and Memory Management
Understanding Lvalue and Rvalue Assignment
In C++, the concepts of lvalue and rvalue are pivotal to understanding how assignment works under the hood. An lvalue refers to a variable that has an identifiable location in memory (like `x` in `int x = 10;`), while an rvalue is typically a temporary object or value that does not have a persistent memory location.
Here’s a concrete example:
int x = 10; // x is an lvalue
int y = x; // x is used as an rvalue
In this case, `x` serves as an lvalue because its value can be referenced and modified, while `10` is an rvalue, which is transient and cannot be modified.
Understanding how these concepts interplay with the equals operator helps you write more efficient and effective C++ code, especially concerning memory management.
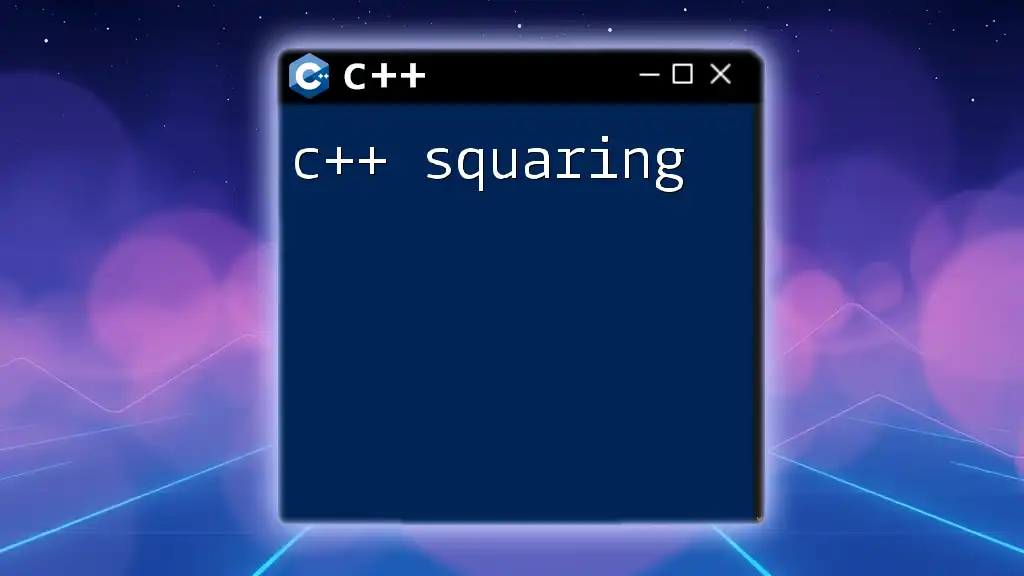
Operators Overloading with the Equals Operator
What is Operator Overloading?
Operator overloading allows you to define how operators function with user-defined types. In C++, you can overload the equals operator to customize how assignment operates for your custom classes.
Example: Overloading the Equals Operator
When you overload the equals operator, you can make your class instances behave just like built-in types for assignment. Here’s an example of how to achieve this:
class MyNumber {
public:
int value;
// Overloading the equals operator
bool operator=(const MyNumber& other) {
this->value = other.value; // Assigning values
return true; // Returning true for successful assignment
}
};
This example demonstrates how the class `MyNumber` can have its own method of handling assignment, making it more intuitive and aligned with standard practices.
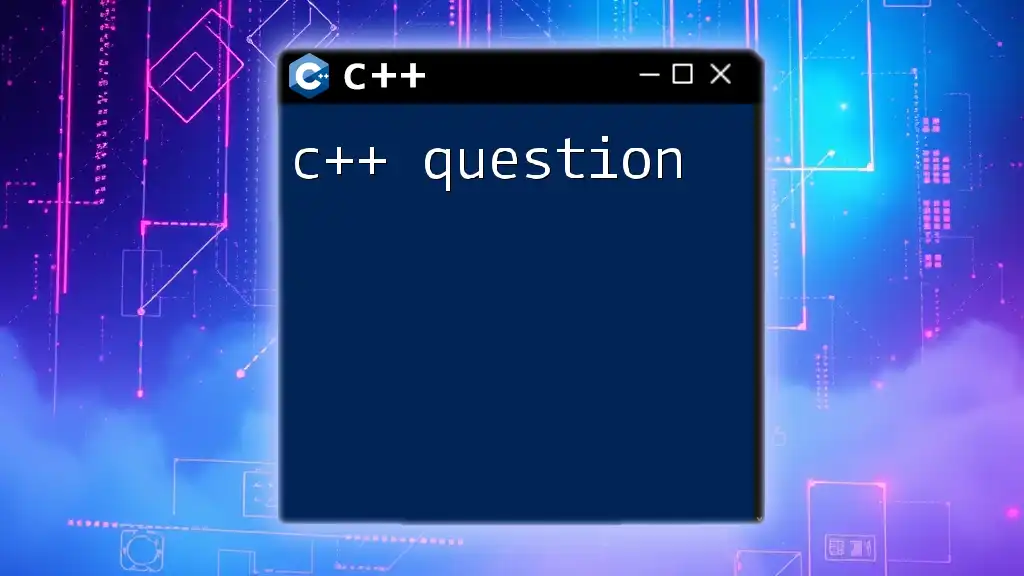
Best Practices when Using the Equals Operator
Common Pitfalls and How to Avoid Them
One frequent mistake among C++ programmers, especially newcomers, is confusing the equals operator (`=`) with the equality operator (`==`). This confusion often leads to logic errors, resulting in unexpected behavior. Always double-check your code to ensure you’re using the correct operator for the intended action.
When to Use Const with the Equals Operator
Using `const` with the equals operator, particularly in function parameters, enhances code safety and prevents modifications to unintended data. Here’s an illustration of good practice:
void assignValue(const int& val) {
int x = val; // Safe assignment, val remains unchanged
}
In this scenario, by marking `val` as `const`, you denote that the reference should not be altered within `assignValue`, thus enforcing better coding discipline.
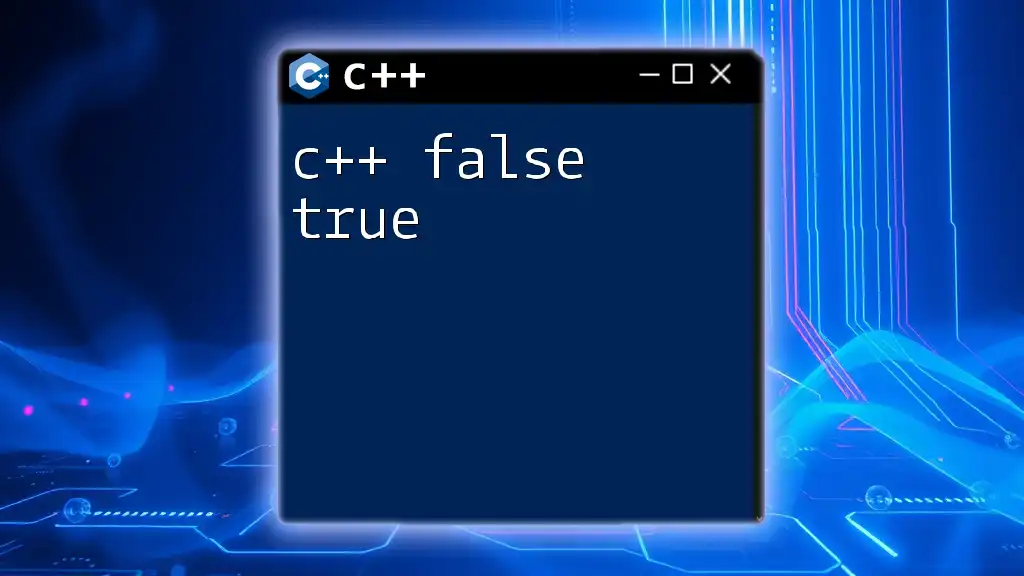
Conclusion
In summary, understanding the C++ equals operator is essential for any aspiring programmer. It plays a fundamental role in variable assignment, interacts with various data types, and facilitates object-oriented programming through operator overloading. By following the best practices outlined in this guide, you can avoid common pitfalls and enhance your overall proficiency in C++.
Knowledge of the equals operator not only improves your C++ skills but also lays a strong foundation for tackling more complex programming challenges. Dive deeper into C++ tutorials and exercises to solidify your understanding and expertise.