The C++ quadratic equation solver calculates the roots of a quadratic equation in the form ax² + bx + c = 0 using the quadratic formula: x = (-b ± √(b² - 4ac)) / (2a).
Here's a simple code snippet that demonstrates this:
#include <iostream>
#include <cmath>
using namespace std;
int main() {
double a, b, c, discriminant, root1, root2;
cout << "Enter coefficients a, b and c: ";
cin >> a >> b >> c;
discriminant = b*b - 4*a*c;
if (discriminant > 0) {
root1 = (-b + sqrt(discriminant)) / (2 * a);
root2 = (-b - sqrt(discriminant)) / (2 * a);
cout << "Roots are real and different: " << root1 << " and " << root2 << endl;
} else if (discriminant == 0) {
root1 = root2 = -b / (2 * a);
cout << "Roots are real and the same: " << root1 << endl;
} else {
double realPart = -b / (2 * a);
double imaginaryPart = sqrt(-discriminant) / (2 * a);
cout << "Roots are complex: " << realPart << " + " << imaginaryPart << "i and "
<< realPart << " - " << imaginaryPart << "i" << endl;
}
return 0;
}
What is a Quadratic Equation?
A quadratic equation is a polynomial equation of the second degree, which can be represented in the standard form:
$$ ax^2 + bx + c = 0 $$
Here, \( a \), \( b \), and \( c \) are coefficients, and \( a \) cannot be zero. Quadratic equations are vital in various fields such as physics, engineering, economics, and statistics because they model many real-world problems. For example, the path of a projectile can be described using a quadratic equation.
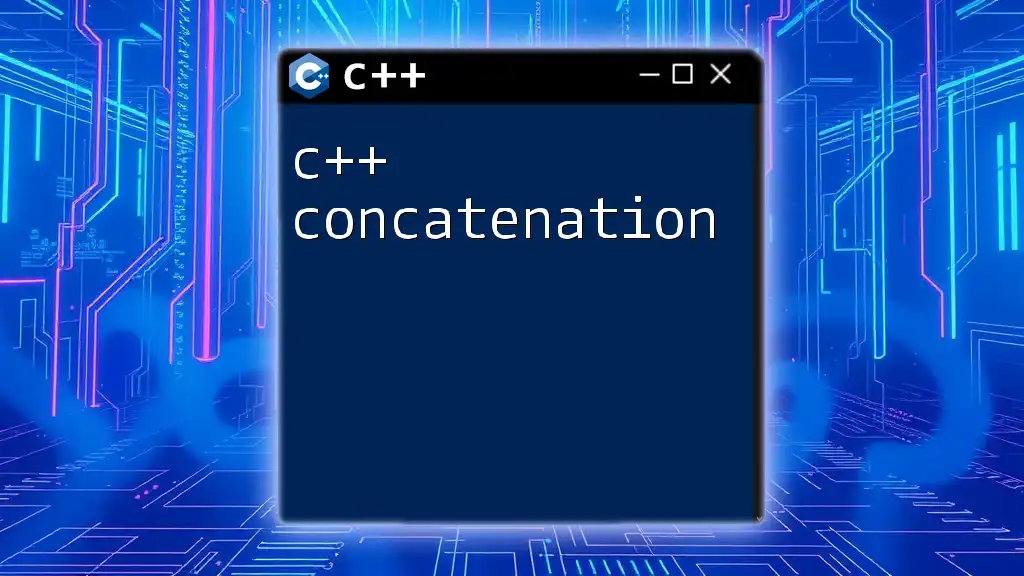
The Quadratic Formula
To find the roots (solutions) of a quadratic equation, we use the quadratic formula:
$$ x = \frac{{-b \pm \sqrt{{b^2 - 4ac}}}}{{2a}} $$
This formula allows us to calculate the values of \( x \) that satisfy the equation. Each component of the formula is important:
- \( a \): The coefficient of \( x^2 \).
- \( b \): The coefficient of \( x \).
- \( c \): The constant term.
- The square root \( \sqrt{{b^2 - 4ac}} \): This term is known as the discriminant.
Discriminant in Detail
The discriminant \( D = b^2 - 4ac \) tells us about the nature of the roots:
- If \( D > 0 \): The equation has two distinct real roots.
- If \( D = 0 \): The equation has exactly one real root (a repeated root).
- If \( D < 0 \): The equation has two complex roots.
Understanding the discriminant is crucial for accurately interpreting the solutions of the quadratic equation.
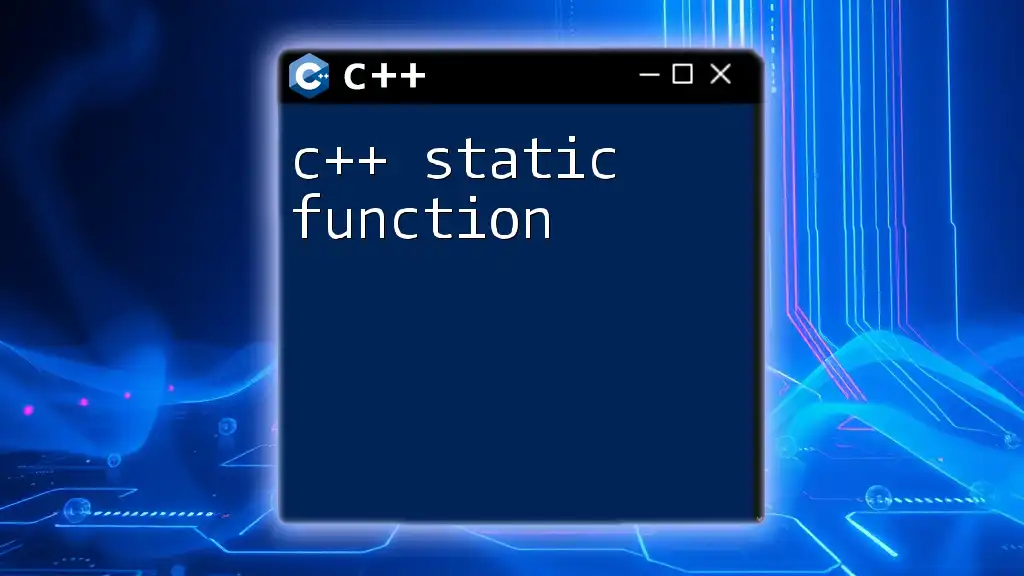
Setting Up Your C++ Environment
Before diving into coding, it's essential to have a suitable C++ development environment. Some popular Integrated Development Environments (IDEs) and compilers include:
- Code::Blocks: A user-friendly IDE for beginners.
- Visual Studio: Robust IDE with extensive features.
- Dev-C++: Lightweight and straightforward for quick projects.
Writing a Simple C++ Program
A basic C++ program structure includes headers, the `main()` function, and I/O commands. Here's a simple skeleton to start with:
#include <iostream>
using namespace std;
int main() {
// Your code here
return 0;
}
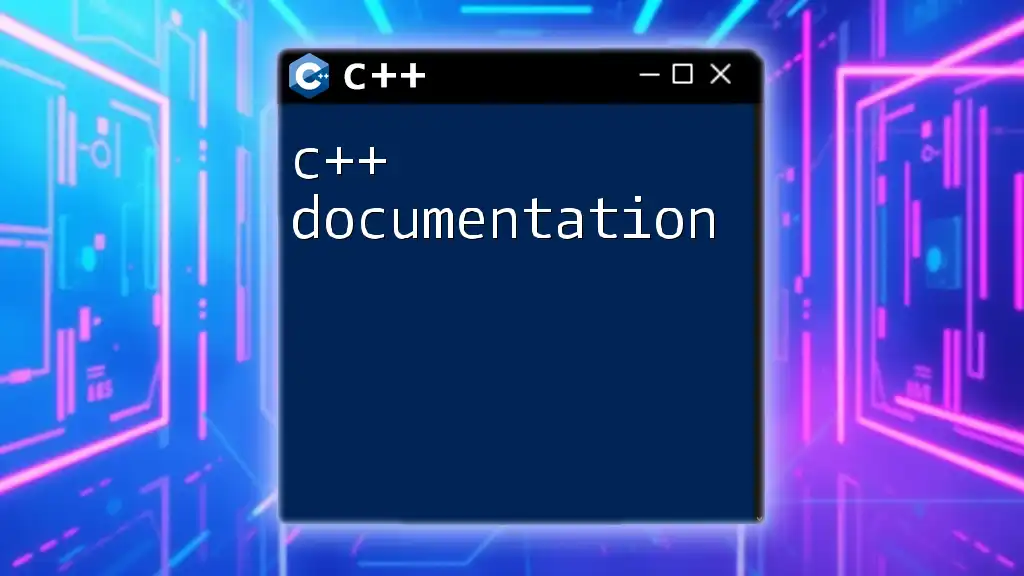
Implementing the Quadratic Formula in C++
Now, we can start coding to find the roots of a quadratic equation using C++.
Creating the C++ Program
Begin by prompting the user to input the coefficients \( a \), \( b \), and \( c \):
#include <iostream>
#include <cmath> // For sqrt()
using namespace std;
int main() {
double a, b, c;
cout << "Enter coefficients a, b and c: ";
cin >> a >> b >> c;
// Additional code will go here...
}
Calculating the Discriminant
Next, calculate the discriminant and display its value:
double discriminant = b*b - 4*a*c;
cout << "Discriminant: " << discriminant << endl;
Finding the Roots
Now we can determine the roots based on the value of the discriminant.
Case 1: Real and Distinct Roots
If \( D > 0 \), the formula produces two distinct real roots. Here’s how to handle this case:
if (discriminant > 0) {
double root1 = (-b + sqrt(discriminant)) / (2*a);
double root2 = (-b - sqrt(discriminant)) / (2*a);
cout << "Roots are real and different." << endl;
cout << "Root 1: " << root1 << endl;
cout << "Root 2: " << root2 << endl;
}
Case 2: Real and Repeated Roots
If \( D = 0 \), there’s only one distinct root:
else if (discriminant == 0) {
double root = -b / (2*a);
cout << "Roots are real and the same." << endl;
cout << "Root: " << root << endl;
}
Case 3: Complex Roots
For \( D < 0 \), the roots are complex. We can compute and display them with the following code:
else {
double realPart = -b / (2*a);
double imaginaryPart = sqrt(-discriminant) / (2*a);
cout << "Roots are complex and different." << endl;
cout << "Root 1: " << realPart << " + " << imaginaryPart << "i" << endl;
cout << "Root 2: " << realPart << " - " << imaginaryPart << "i" << endl;
}
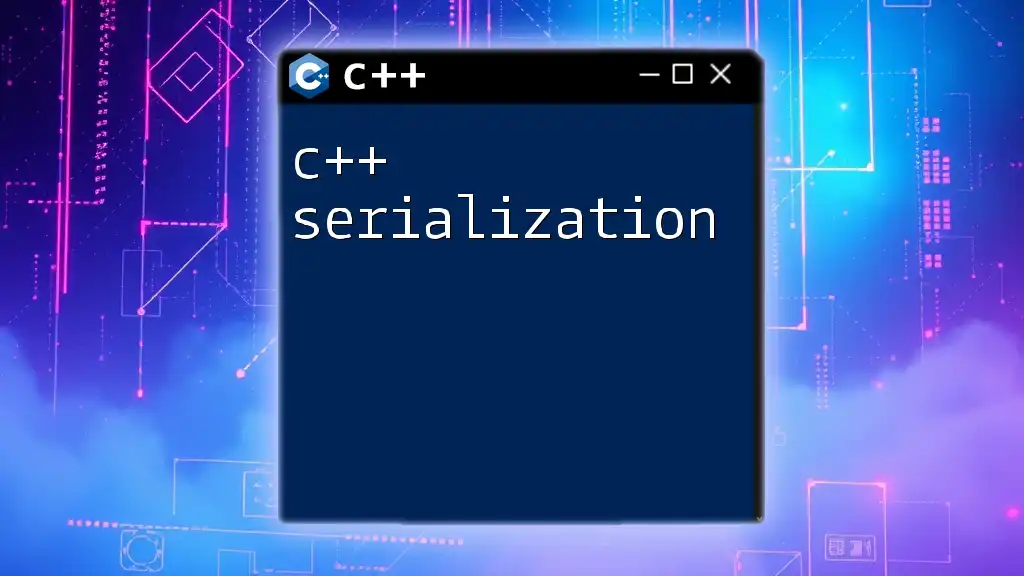
Complete C++ Program Example
Here’s the complete code snippet that integrates all the elements explained:
#include <iostream>
#include <cmath>
using namespace std;
int main() {
double a, b, c;
cout << "Enter coefficients a, b and c: ";
cin >> a >> b >> c;
double discriminant = b*b - 4*a*c;
cout << "Discriminant: " << discriminant << endl;
if (discriminant > 0) {
double root1 = (-b + sqrt(discriminant)) / (2*a);
double root2 = (-b - sqrt(discriminant)) / (2*a);
cout << "Roots are real and different.\nRoot 1: " << root1 << "\nRoot 2: " << root2 << endl;
}
else if (discriminant == 0) {
double root = -b / (2*a);
cout << "Roots are real and the same.\nRoot: " << root << endl;
}
else {
double realPart = -b / (2*a);
double imaginaryPart = sqrt(-discriminant) / (2*a);
cout << "Roots are complex and different.\nRoot 1: " << realPart << " + " << imaginaryPart << "i\nRoot 2: " << realPart << " - " << imaginaryPart << "i" << endl;
}
return 0;
}
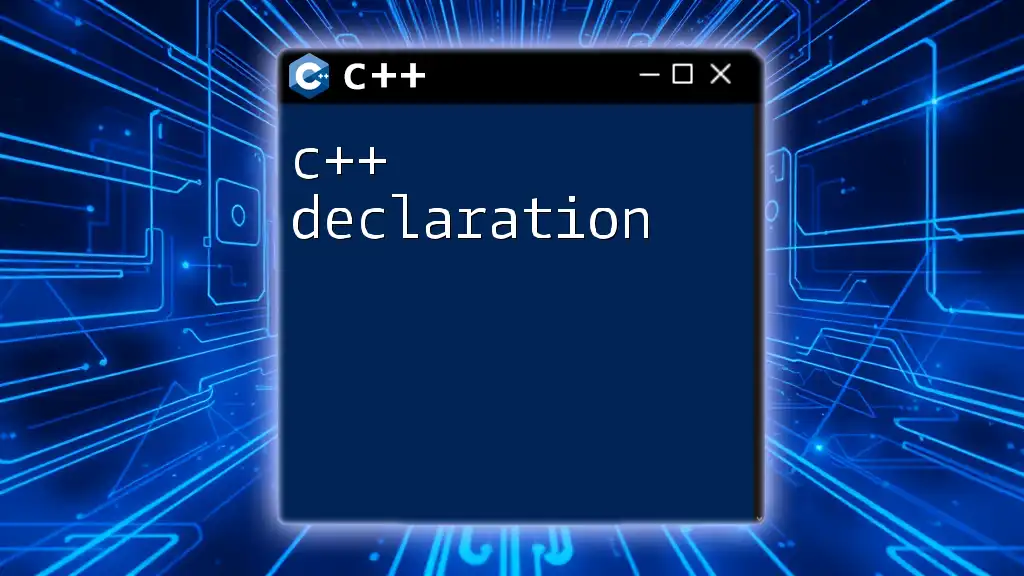
Summary of Key Points
This guide has covered the essential aspects of solving a C++ quadratic equation using the quadratic formula. Understanding the discriminant helps us determine the nature of the roots, while implementing the formula in C++ showcases the elegance of programming in solving mathematical problems.
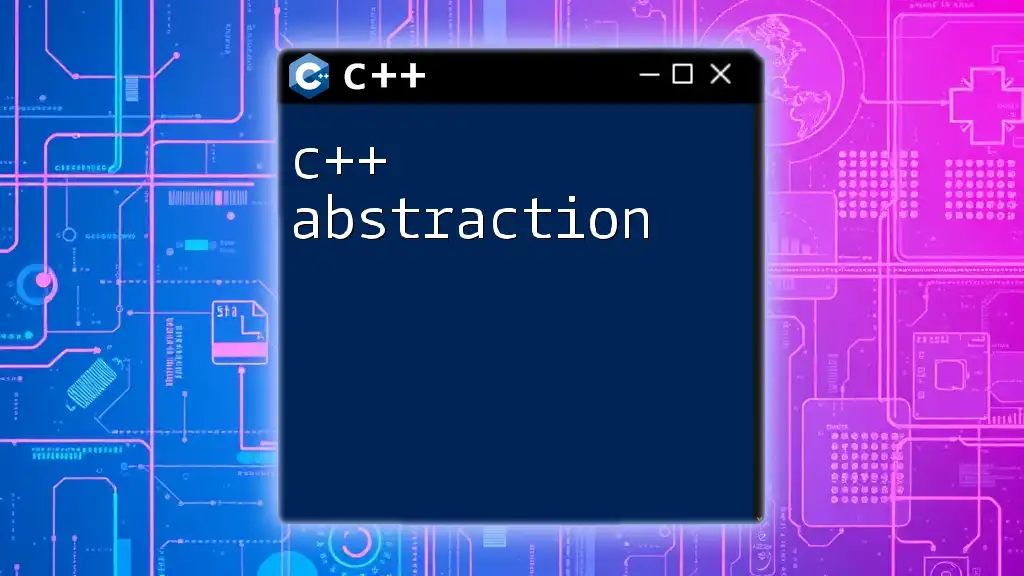
Encouragement to Practice
I encourage you to modify the provided code. Experiment with different coefficients and explore additional problems related to quadratic equations. The more you practice, the more proficient you will become in using C++ for mathematical computations.
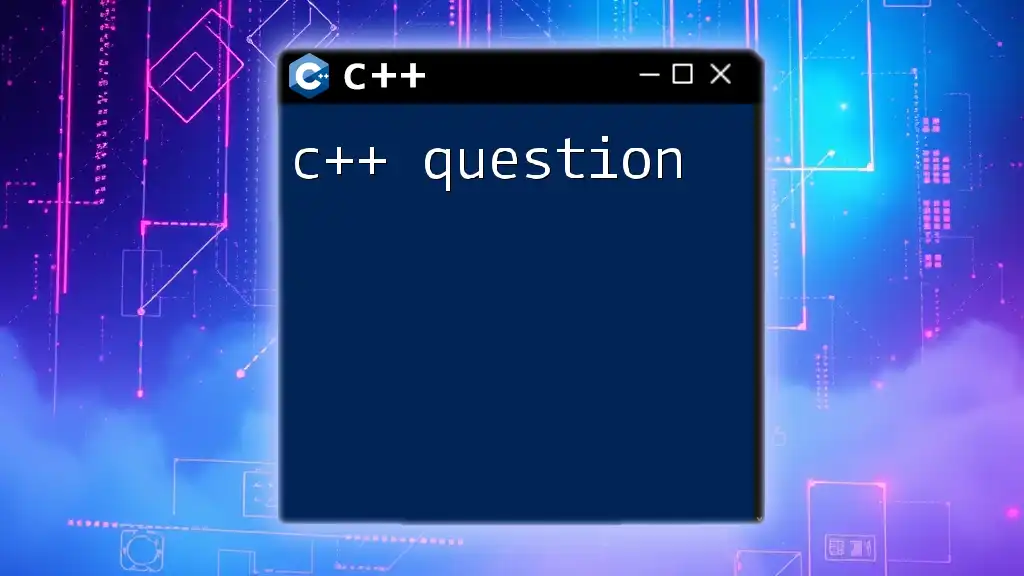
Additional Resources
For further reading, consider these resources:
- Books on C++ programming and mathematical modeling.
- Online courses focused on algorithm design and numerical methods.
- Community forums and groups dedicated to C++ programming, where you can ask questions and share insights.
Happy coding!