In C++, a fraction can be represented using a simple class that stores the numerator and denominator, allowing operations like addition and simplification. Here's a basic example:
class Fraction {
public:
int numerator, denominator;
Fraction(int num, int denom) : numerator(num), denominator(denom) {}
// Additional methods for operations can be added here
};
Understanding Fractions in C++
A fraction represents a part of a whole. In mathematics, a fraction consists of two main components: the numerator, which indicates how many parts we have, and the denominator, which indicates how many parts make up a whole. In programming, particularly with C++, handling fractions becomes crucial when precision is necessary, especially in calculations that involve rational numbers.
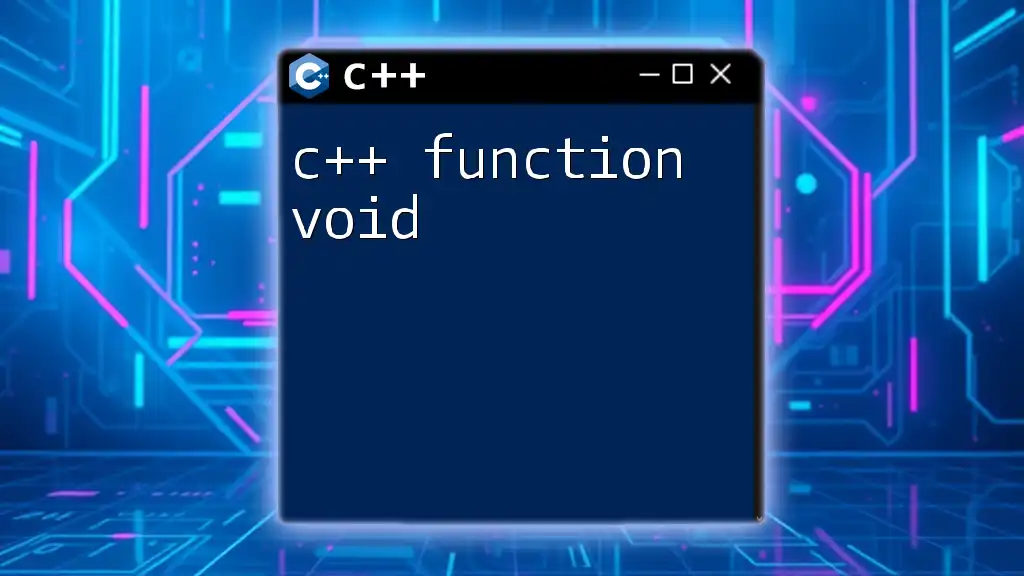
Why Use Fractions?
Using fractions in programming has several advantages:
- Precision in Mathematical Calculations: Fractions can represent numbers that integers or floating-point types struggle to accurately depict. For example, with fractions, 1/3 is exactly represented, while a floating-point approximation can lead to rounding errors.
- Avoiding Floating-Point Imprecision: Floating-point representations have inherent limitations; fractions bypass this by maintaining exact values.
- Enhancing Clarity in Code: By representing rational values directly with fractions, your code becomes clearer, making it easier to read and maintain.
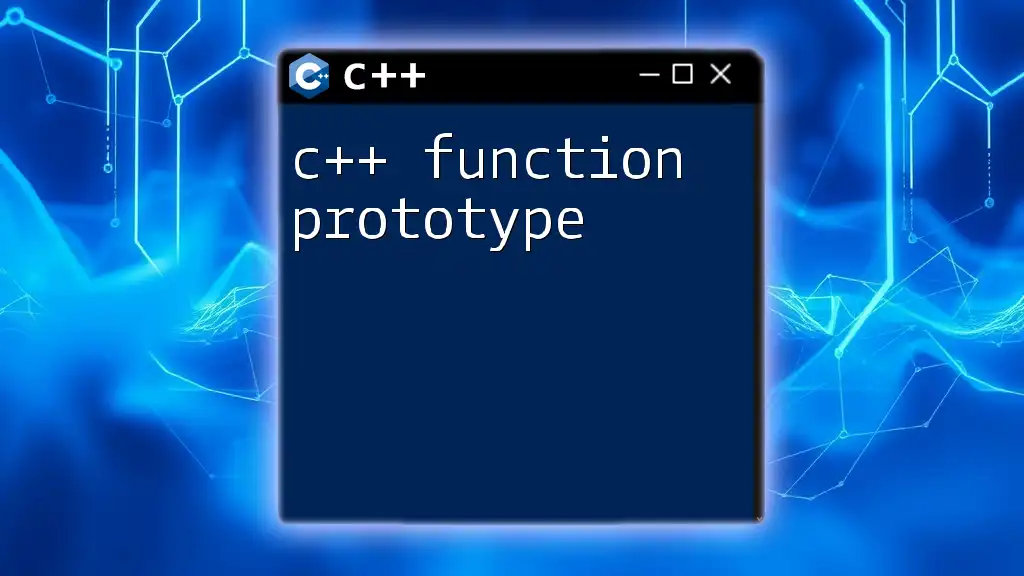
Implementing a Fraction Class in C++
To effectively handle fractions in C++, creating a dedicated Fraction class is an excellent approach. This class will encapsulate the functionalities needed for fraction arithmetic.
Basic Structure of the Fraction Class
Let's dive into the structure of the Fraction class. We'll need to define private member variables for the numerator and denominator:
class Fraction {
private:
int numerator;
int denominator;
public:
Fraction(int num, int denom);
// Other functionalities will follow...
};
Constructor for the Fraction Class
A constructor initializes a fraction object. It’s vital to validate that the denominator is not zero, as this would result in an invalid fraction.
Fraction::Fraction(int num, int denom) {
// Validate the denominator
if (denom == 0) {
throw std::invalid_argument("Denominator cannot be zero.");
}
numerator = num;
denominator = denom;
}
Simplifying Fractions
To maintain accuracy, fractions should often be simplified. We can achieve this using the greatest common divisor (GCD) algorithm:
int gcd(int a, int b) {
return (b == 0) ? a : gcd(b, a % b);
}
void simplify() {
int gcdValue = gcd(numerator, denominator);
numerator /= gcdValue;
denominator /= gcdValue;
}
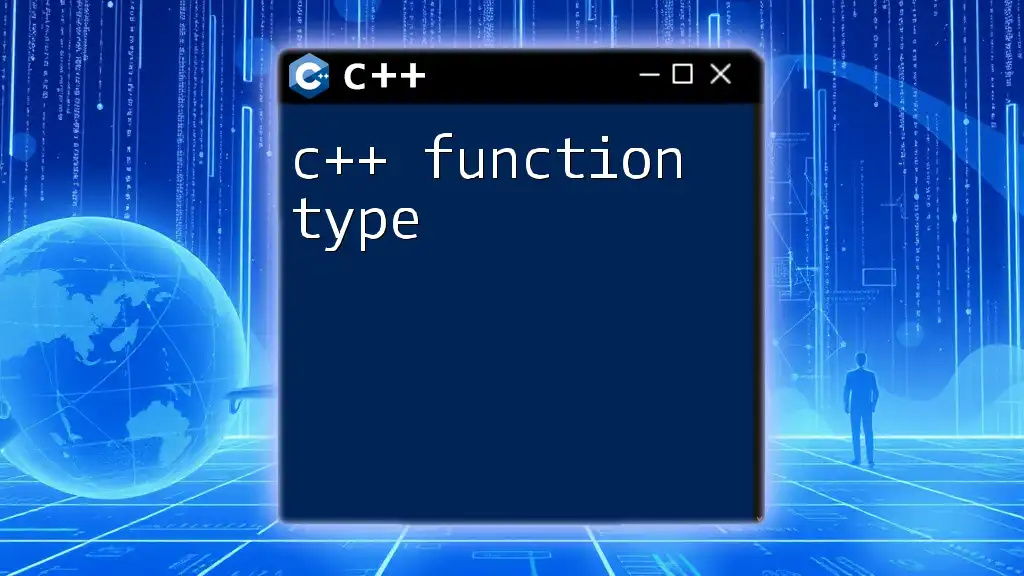
Basic Operations with Fractions
Once our Fraction class is set up, we can perform various arithmetic operations.
Addition
To add two fractions, follow the basic rule:
\[ \frac{a}{b} + \frac{c}{d} = \frac{ad + bc}{bd} \]
Here's the implementation:
Fraction operator+(const Fraction &other) {
return Fraction(numerator * other.denominator + other.numerator * denominator,
denominator * other.denominator);
}
Subtraction
For subtracting fractions, the rule is:
\[ \frac{a}{b} - \frac{c}{d} = \frac{ad - bc}{bd} \]
The code for subtraction looks like this:
Fraction operator-(const Fraction &other) {
return Fraction(numerator * other.denominator - other.numerator * denominator,
denominator * other.denominator);
}
Multiplication
When multiplying fractions, simply multiply the numerators and denominators:
\[ \frac{a}{b} \times \frac{c}{d} = \frac{ac}{bd} \]
Here's how you can implement multiplication:
Fraction operator*(const Fraction &other) {
return Fraction(numerator * other.numerator, denominator * other.denominator);
}
Division
Dividing fractions involves flipping the second fraction and multiplying:
\[ \frac{a}{b} \div \frac{c}{d} = \frac{a}{b} \times \frac{d}{c} = \frac{ad}{bc} \]
The implementation for division is as follows:
Fraction operator/(const Fraction &other) {
if (other.numerator == 0) {
throw std::invalid_argument("Cannot divide by zero.");
}
return Fraction(numerator * other.denominator, denominator * other.numerator);
}
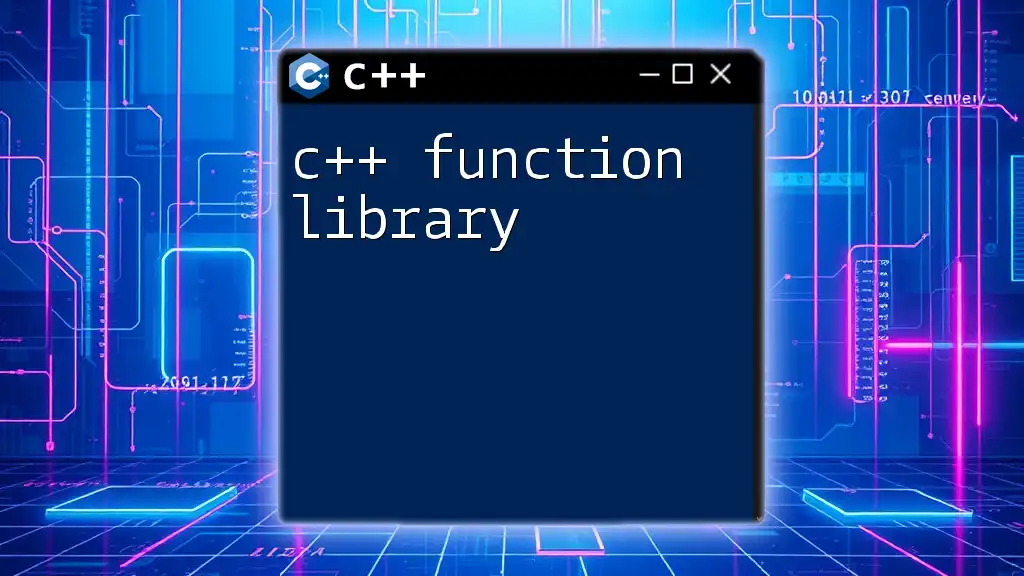
Comparing Fractions
Equal and Not Equal Comparison
To compare two fractions for equality:
bool operator==(const Fraction &other) {
return (numerator * other.denominator == other.numerator * denominator);
}
bool operator!=(const Fraction &other) {
return !(*this == other);
}
Less Than and Greater Than Comparison
You can also compare using less than and greater than operators:
bool operator<(const Fraction &other) {
return (numerator * other.denominator < other.numerator * denominator);
}
bool operator>(const Fraction &other) {
return (numerator * other.denominator > other.numerator * denominator);
}
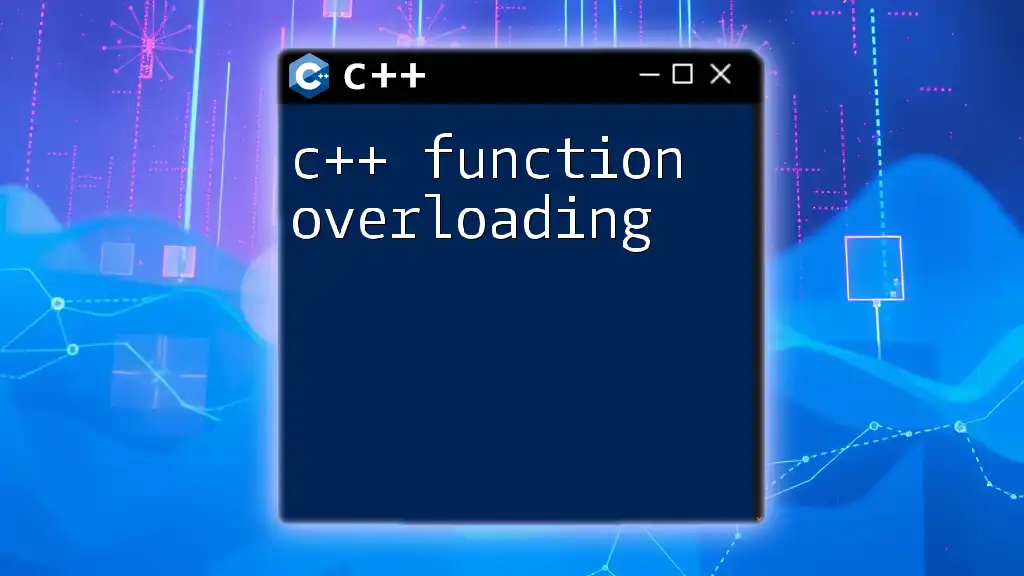
Handling Input and Output
Overloading the Stream Operators
To manage input and output in a smart way, you can overload the `<<` and `>>` operators:
friend std::ostream& operator<<(std::ostream &out, const Fraction &frac) {
out << frac.numerator << "/" << frac.denominator;
return out;
}
friend std::istream& operator>>(std::istream &in, Fraction &frac) {
char sep;
in >> frac.numerator >> sep >> frac.denominator;
if (frac.denominator == 0) {
throw std::invalid_argument("Denominator cannot be zero.");
}
return in;
}
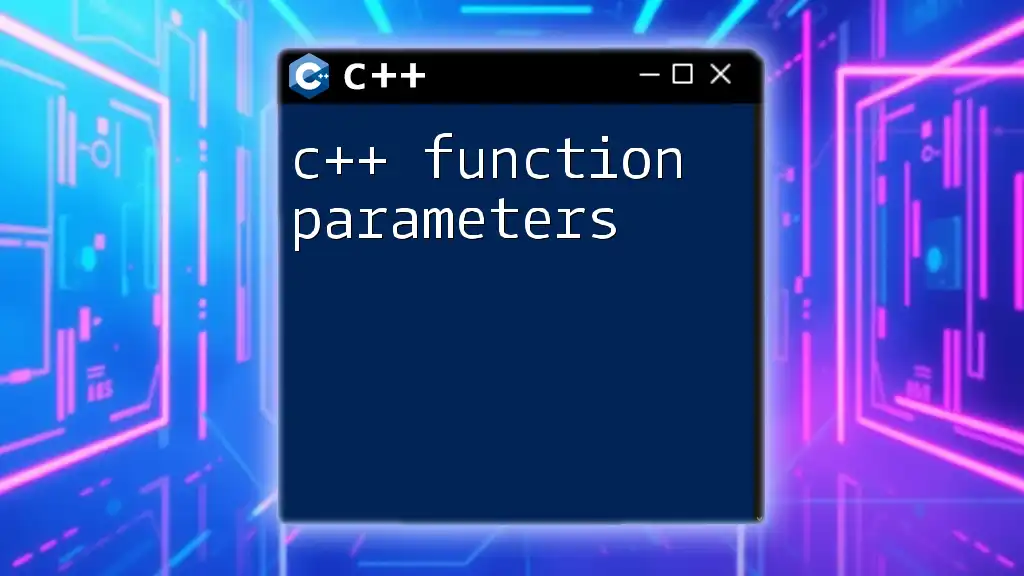
Best Practices for Using the Fraction Class
Tips for Rounding and Precision
While fractions inherently avoid many issues that arise with floating-point arithmetic, it’s still important to understand when and how to convert fractions to floating-point numbers for specific use cases. Always be cautious of rounding parity.
Error Handling
Despite the robust design, errors can occur. Implement proper exception handling. For instance, ensure that your application gracefully handles a zero denominator throughout all places in the code.
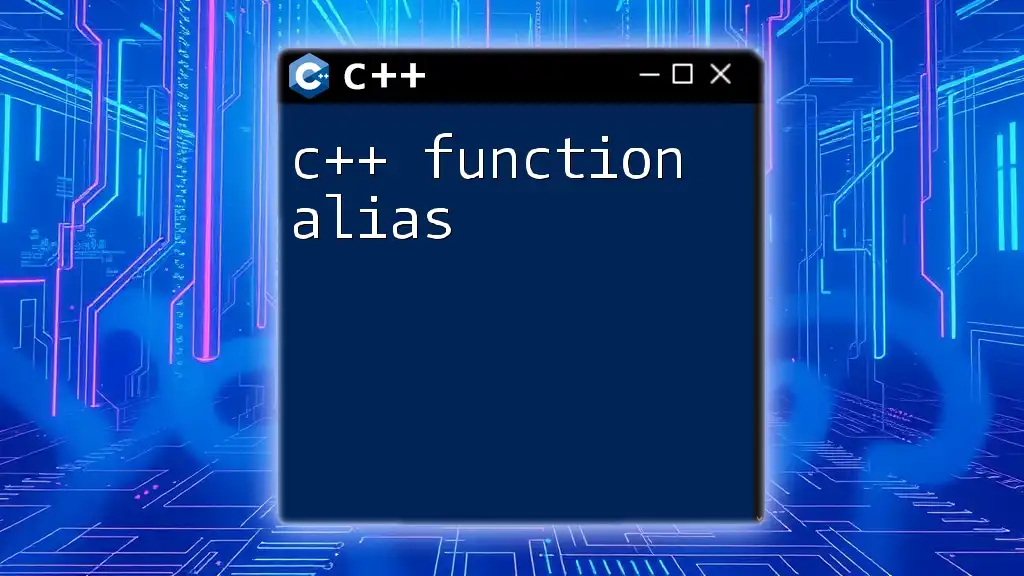
Conclusion
The C++ fraction implementation outlined here provides a solid foundation for handling rational numbers. With features allowing for addition, subtraction, multiplication, and division, along with comprehensive comparison methods, this class can significantly enhance mathematical precision in your programs. I encourage you to implement and experiment with this Fraction class in your projects to fully appreciate its utility in C++.
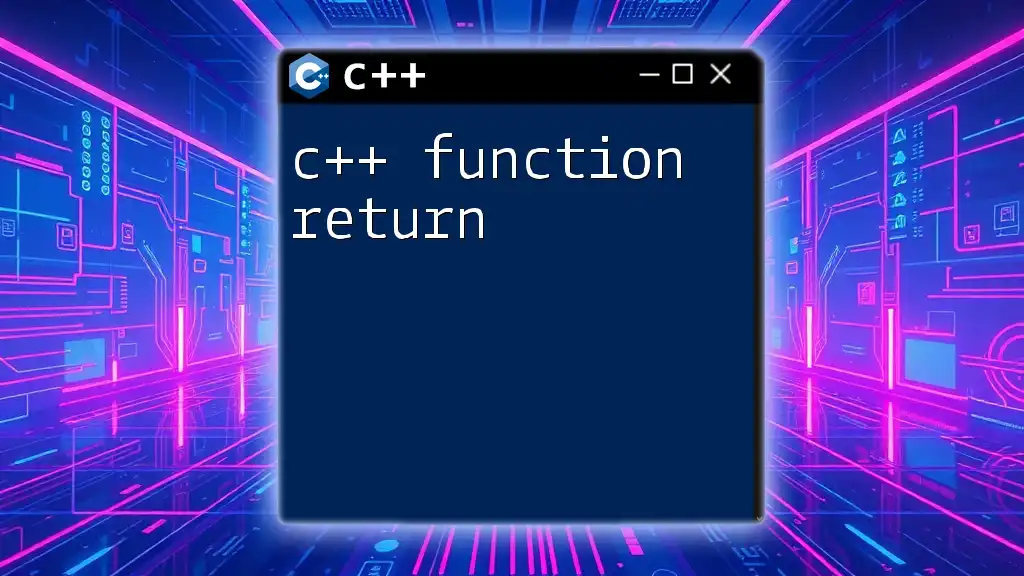
Additional Resources
For further reading, consider diving into topics such as rational number libraries or more advanced mathematical concepts in C++. Understanding the underlying principles of handling rational numbers will lead to better programming practices and ultimately improve your projects. Feel free to comment or ask questions for further clarity in this area.