In C++, a function can return a vector by defining the return type as `std::vector<Type>` and populating the vector before returning it. Here’s a code snippet illustrating this:
#include <vector>
std::vector<int> createVector() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
return myVector;
}
Understanding C++ Vectors
What is a Vector?
In C++, a vector is defined as a sequence container that encapsulates dynamic size arrays. Unlike traditional arrays that have a fixed size, vectors can grow and shrink as needed, dynamically allocating and deallocating memory. As part of the C++ Standard Library, vectors are implemented via the `std::vector` template, which provides a versatile and efficient way to manage collections of elements.
Why Use Vectors?
Vectors offer several advantages that make them preferable to arrays in many scenarios:
-
Dynamic Sizing: Vectors automatically adjust their size when elements are added or removed, eliminating the need for manual memory management.
-
Automatic Memory Management: When a vector goes out of scope, it automatically deallocates its memory, reducing the chances of memory leaks.
-
Rich Functionality: The `std::vector` class comes with a variety of member functions, like `push_back()`, `pop_back()`, and `size()`, which make operations on collections straightforward and efficient.
Common use cases for vectors include storing dynamic lists of items, managing collections that may change in size, and handling return values from functions in a clean and efficient manner.
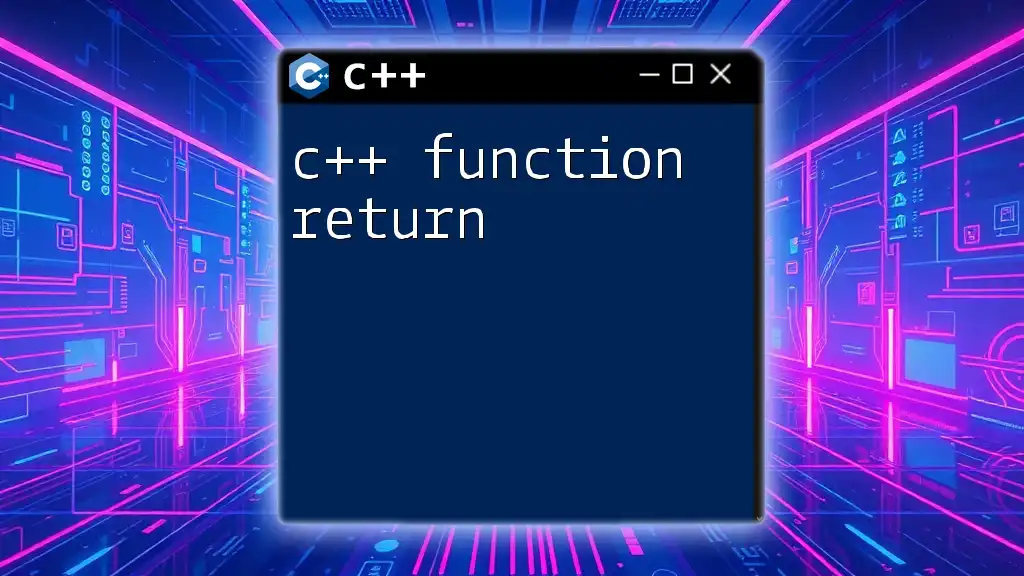
C++ Return Vector from Function
How to Return a Vector C++?
Returning a vector from a function is straightforward. The function's return type is defined as `std::vector<Type>`, where `Type` represents the datatype of the vector’s elements. By using this syntax, you can easily return a vector with any type of data, whether it be integers, floating-point numbers, or user-defined classes.
Returning a Vector by Value
When you return a vector by value, a new copy of the vector is created in memory. This means that while the original vector remains unchanged, the function caller gets a separate instance of the vector. Here’s an example of returning a vector by value:
#include <iostream>
#include <vector>
std::vector<int> createVector() {
std::vector<int> vec = {1, 2, 3, 4, 5};
return vec; // Returning vector by value
}
int main() {
std::vector<int> myVector = createVector();
// Output the vector elements
for (int i : myVector) {
std::cout << i << " ";
}
return 0;
}
In this code snippet, the function `createVector()` creates a vector and returns it. When the vector is returned, the calling code receives a copy of the vector. It’s important to note that returning by value copies the entire vector, which may lead to performance issues, especially with larger data sets. However, since C++11, optimization techniques like Return Value Optimization (RVO) and move semantics mitigate much of the performance overhead.
Returning a Vector by Reference
An alternative approach is to return a vector by reference. This means that the function returns a reference to the original vector, allowing modifications to it without creating a duplicate. Here’s an example:
#include <iostream>
#include <vector>
std::vector<int>& getGlobalVector() {
static std::vector<int> globalVec = {10, 20, 30};
return globalVec; // Returning vector by reference
}
int main() {
std::vector<int>& myVector = getGlobalVector();
// Modify the global vector
myVector.push_back(40);
for (int i : myVector) {
std::cout << i << " ";
}
return 0;
}
In this example, `getGlobalVector()` returns a reference to a static vector `globalVec`. Here, it’s crucial to note the use of the `static` keyword. If you didn't use `static`, the vector would be destroyed when the function exits, leading to undefined behavior when trying to access it later.
The flexibility to return by reference has its advantages, but it can also lead to potential issues, especially if the object being referred to goes out of scope. Thus, while it is memory-efficient, caution is required.
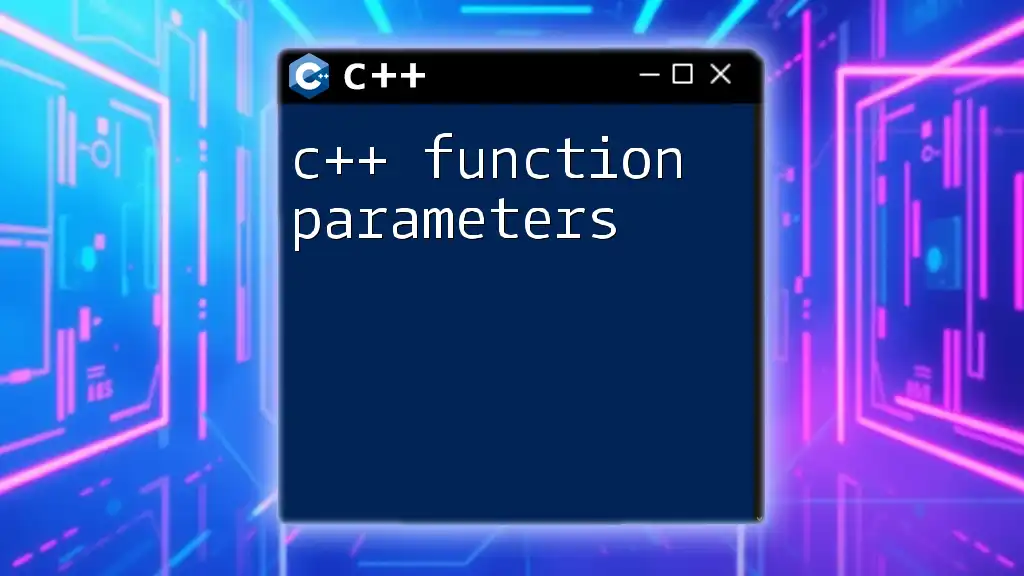
C++ Returning Vector – Best Practices
Memory Management
When returning vectors from functions, understanding memory management is vital. Local vectors are automatically deallocated when they go out of scope, and while returning by reference avoids unnecessary copying, it requires careful handling to ensure that the vector remains valid.
If you need to return a vector from a function, consider whether a local vector suffices or if you should use a static vector. It’s essential to strike a balance between performance and safety.
Performance Considerations
Returning large vectors by value can be costly in terms of performance due to the overhead of copying. C++11 introduced move semantics, allowing the efficient transfer of resources without copying. Instead of copying the vector, we can "move" it:
std::vector<int> moveVector() {
std::vector<int> vec = {1, 2, 3, 4, 5};
return std::move(vec); // Move semantics
}
Using `std::move`, we indicate that the contents of `vec` can be transferred to the caller without creating a copy. This is highly efficient for large containers and significantly improves performance when returning large vectors.
When to Avoid Returning Vectors
While returning vectors can be very useful, there are specific scenarios where it may not be the most effective option. Here are a few considerations:
-
Memory Constraints: If your application frequently handles large data sets, consider using pointers or references instead to minimize memory usage.
-
Data Lifespan: If the data will not be needed beyond the function scope, consider modifying an argument passed by reference instead of returning.
-
Alternative Data Structures: Sometimes, alternatives such as `std::array` or even other container types might be more appropriate based on the specific requirements of your application.
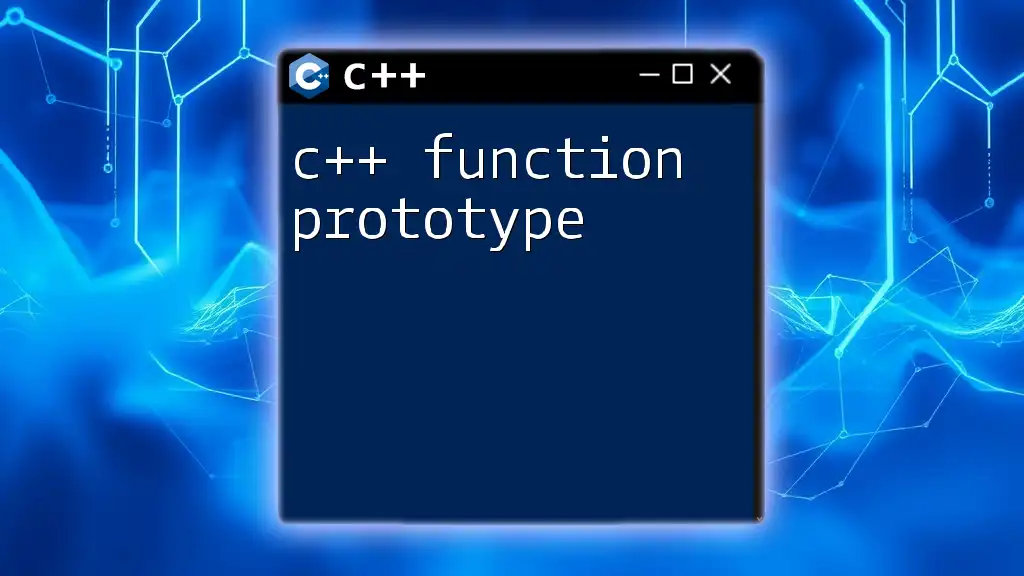
Examples and Use Cases
Use Case: Creating a Vector of Squares
Let’s consider a practical example where we create a function that generates a vector containing square numbers up to a specified integer:
#include <iostream>
#include <vector>
std::vector<int> squares(int n) {
std::vector<int> vec;
for (int i = 1; i <= n; ++i) {
vec.push_back(i * i);
}
return vec;
}
int main() {
std::vector<int> squareNumbers = squares(5);
for (const auto& sq : squareNumbers) {
std::cout << sq << " ";
}
return 0;
}
In this example, the `squares()` function generates square numbers from `1` to `n` and returns the resulting vector. This highlights the simplicity of using vectors for generating dynamic, calculated collections in C++.
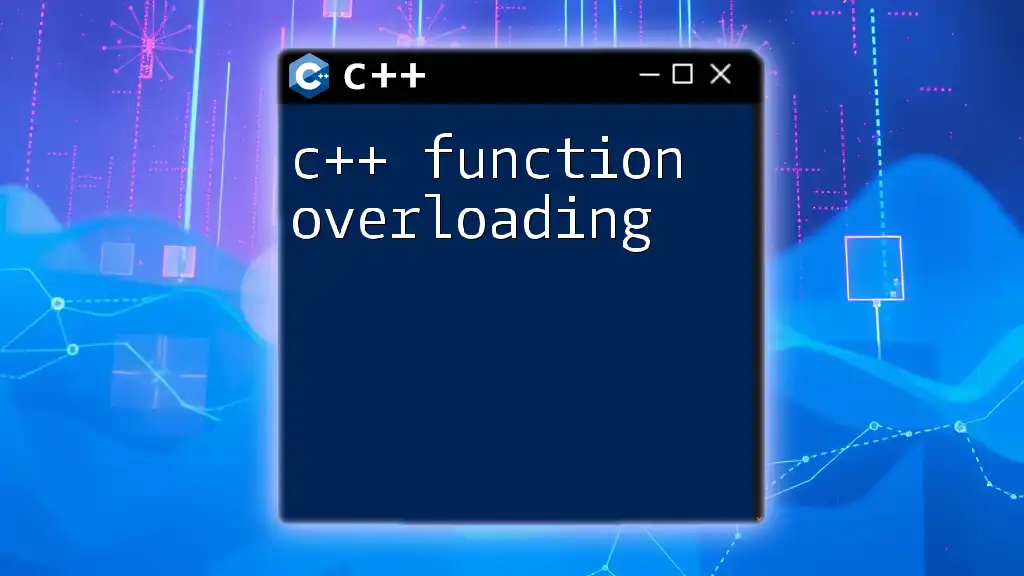
Conclusion
Understanding the nuances of returning vectors from functions in C++ is essential for any developer looking to leverage the power and flexibility of the Standard Library. Embracing the methods discussed — returning by value versus reference, practicing proper memory management, and considering performance implications — allows for the effective use of vectors in your coding projects.
Experimenting with your functions will deepen your understanding and help you master one of the most critical elements of C++ programming. With practice, you’ll unlock the full potential of vectors in your applications, enhancing both usability and performance.
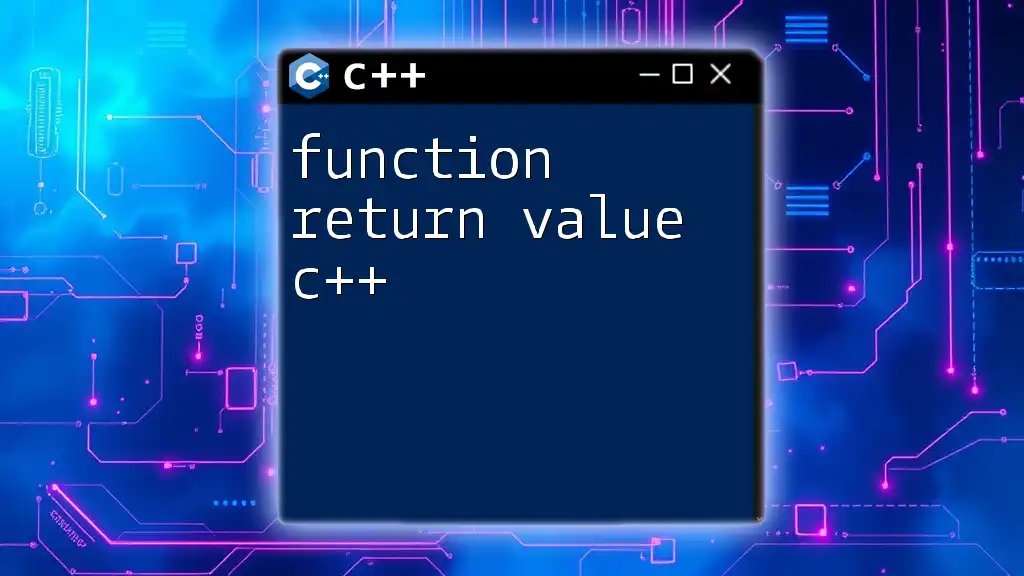
FAQs about C++ Return Vector
Common Questions
-
Can you return an empty vector from a function? Yes, a function can return an empty vector without any issues. An empty vector is a valid state and can be initialized and returned like any other vector.
-
What happens if the function returning a reference goes out of scope? If the function returns a reference to a local variable that goes out of scope, it will lead to undefined behavior when the reference is accessed, resulting in potential crashes or data corruption.
-
How does C++11 and beyond impact returning vectors? C++11 introduced move semantics, which greatly optimized the performance of returning vectors by allowing the ownership of resources to be transferred without copying, reducing overhead in many scenarios.
In conclusion, mastering how to effectively use and return vectors will set the foundation for writing more efficient and maintainable C++ code.