A C++ function pointer is a variable that holds the address of a function, allowing you to call that function indirectly, and can be used to implement callback mechanisms.
Here's a simple example:
#include <iostream>
// Function to be pointed to
void greet() {
std::cout << "Hello, World!" << std::endl;
}
int main() {
// Declare a function pointer
void (*funcPtr)() = &greet;
// Call the function using the pointer
funcPtr();
return 0;
}
Introduction to C++ Function Pointers
What are Function Pointers?
A function pointer is a special type of pointer that stores the address of a function. In C++, functions are first-class citizens, meaning they can be treated like variables. This allows us to create pointers that point to functions, enabling dynamic function calls and higher flexibility in program design. Understanding function pointers is vital since they play an integral role in callback mechanisms and event handling in modern software development.
Applications of Function Pointers in C++
Function pointers are widely used in scenarios such as:
- Callbacks: Enabling functions to be passed as arguments for execution later.
- Event handling: Managing user-defined events in graphical user interfaces.
- Dynamic dispatch: Facilitating polymorphism in function calling.
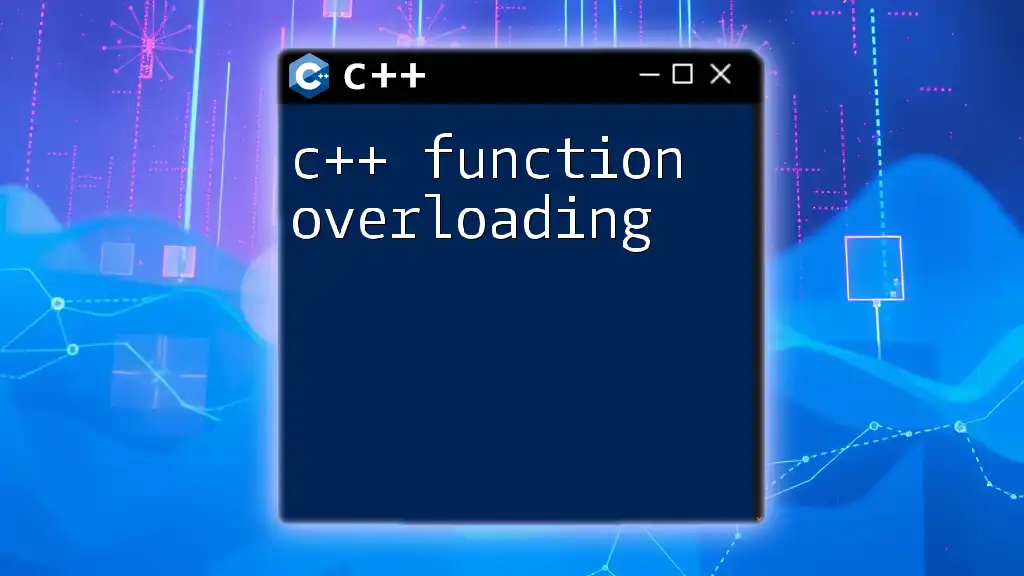
Understanding Pointers in C++
Basics of Pointers in C++
Pointers are variables that hold memory addresses. In C++, a pointer is declared with an asterisk `*`, which differentiates it from regular variables. For example, a pointer to an integer is declared as follows:
int* ptr;
This declaration indicates that `ptr` can hold the address of an integer variable.
Difference Between Pointers and Function Pointers
While standard pointers point to data types (like integers or characters), function pointers point to the address of a function. The syntax for declaring a function pointer is different and specifies not only the return type of the function but also its parameter types. Understanding this distinction is crucial for effective programming in C++.
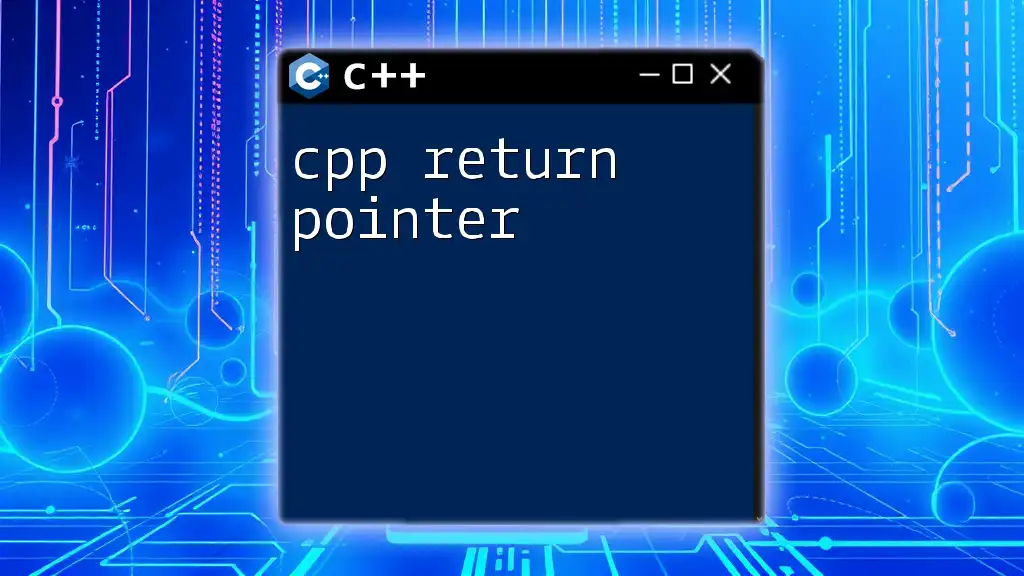
What is a Function Pointer in C++?
Defining Function Pointers
A function pointer is declared in a specific format that specifies the return type and the type of parameters the function expects. Here is the basic syntax for declaring a function pointer:
returnType (*pointerName)(parameterType1, parameterType2, ...);
For instance, if you have a function that takes two integers and returns an integer, the declaration would look like this:
int (*funcPtr)(int, int);
Pointer to Function in C++
When you define a function, it automatically has an address in memory, which can be retrieved using a function pointer. To assign a function to a pointer, you simply set the pointer to the function’s name, without parentheses. For example:
int add(int a, int b) {
return a + b;
}
// Function pointer declaration
int (*funcPtr)(int, int) = add;
In the example above:
- `add` is a function that performs addition.
- `funcPtr` is a pointer that points to the `add` function.
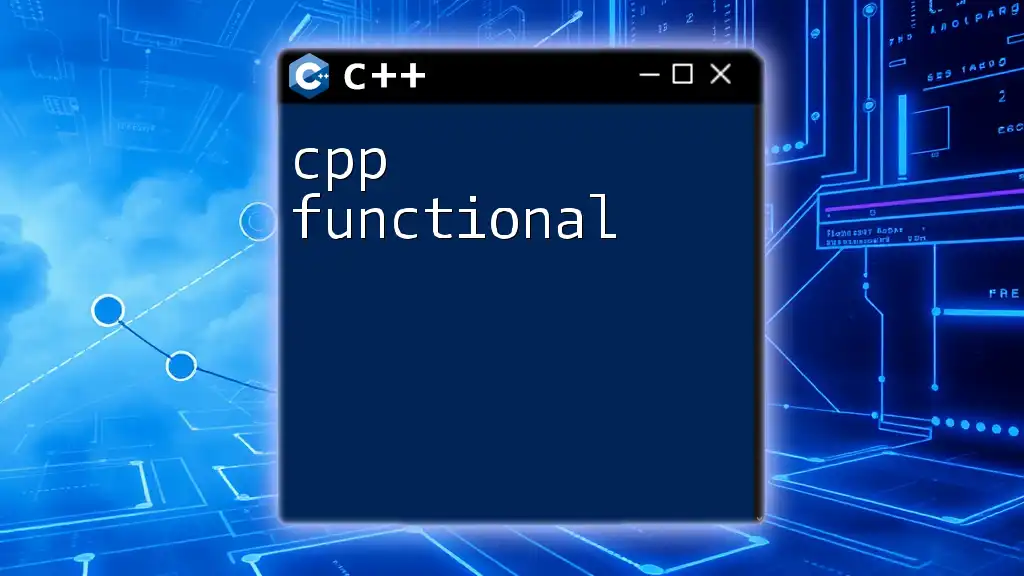
How to Use C++ Function Pointers
Declaring and Initializing Function Pointers
Declaring function pointers can initially seem complex, but once you understand the syntax, it becomes clear. To declare a pointer that points to a function returning `void` and taking no parameters, you would do the following:
void greet() {
std::cout << "Hello, World!" << std::endl;
}
// Function pointer declaration
void (*greetPtr)() = greet;
Here, `greetPtr` points to the `greet` function.
Calling Functions Using Pointers
Once a function pointer is initialized, you can use it to call the function it points to. Use the same syntax you would for calling a regular function, but with the function pointer and parentheses:
greetPtr(); // Calls the greet function
This invokes the `greet` function and outputs `Hello, World!`.
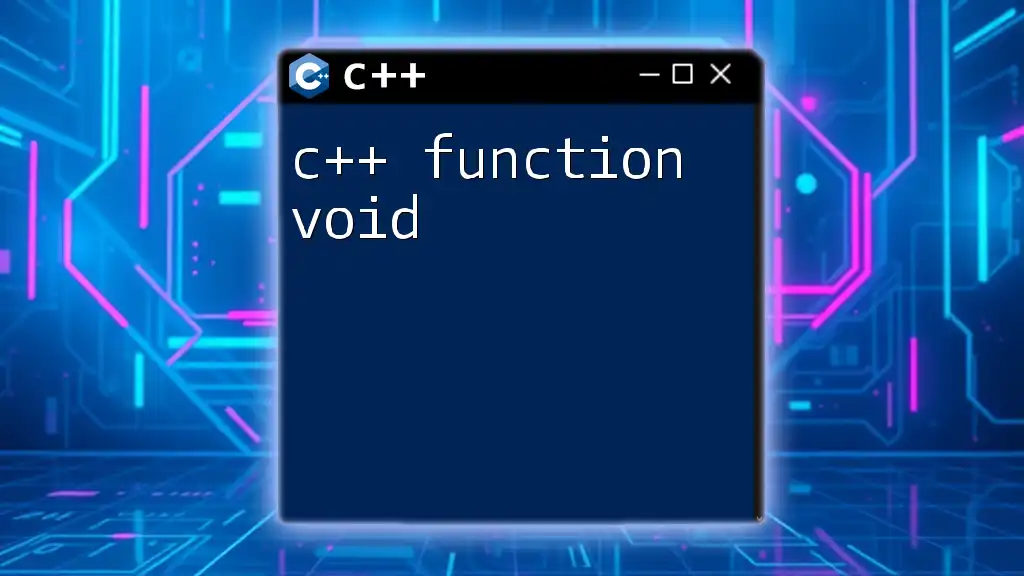
Advanced Usage of Function Pointers in C++
Pointers to Functions with Different Signatures
Function pointers can be adapted for different function signatures, allowing you to call various functions flexibly. For example, consider the following multiplication function:
double multiply(double x, double y) {
return x * y;
}
// Function pointer declaration for multiply
double (*multPtr)(double, double) = multiply;
Passing Function Pointers as Arguments
Function pointers can also be passed as arguments to other functions, increasing the flexibility of your code. Below is an example of how this works:
void executeOperation(int (*operation)(int, int), int a, int b) {
std::cout << "Result: " << operation(a, b) << std::endl;
}
executeOperation(add, 5, 3); // Result: 8
In this example, `executeOperation` takes a function pointer as its first argument, which can be used to execute different operations based on what function you pass to it.
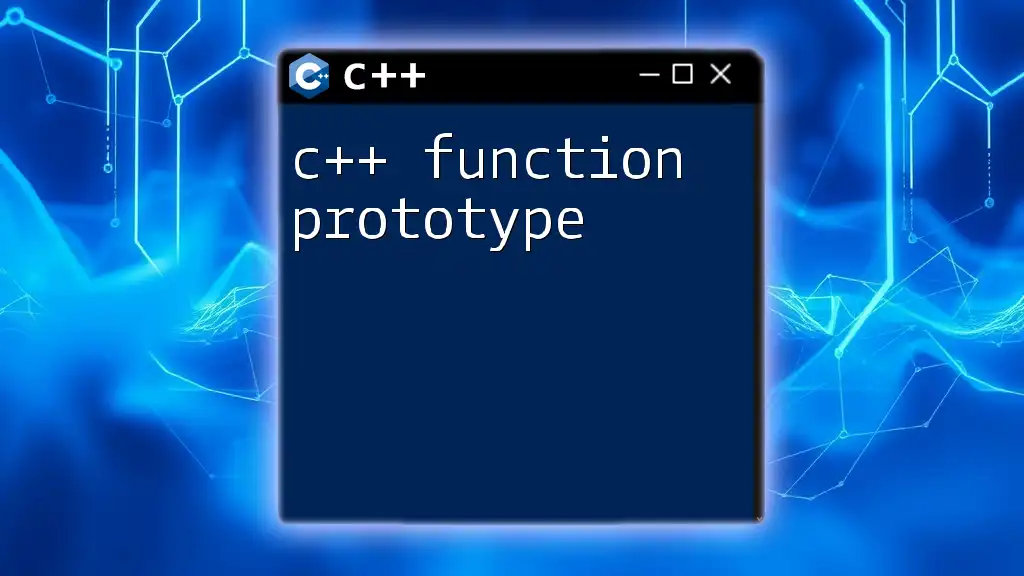
Key Benefits of Using Function Pointers in C++
Using function pointers in C++ offers several advantages:
Increased Flexibility and Reusability
By decoupling function definitions from their calls, function pointers make your code more modular and reusable. This is especially useful in larger codebases where functions can be substituted or redefined without changing the overall structure.
Handling Callbacks and Events
Function pointers are essential for implementing callbacks, which allow certain functions to be called in response to specific events. For instance, in a GUI application, you can set up callback function pointers for various user interactions, like button clicks or text input.
Improved Performance in Certain Scenarios
In high-performance applications, using function pointers can reduce function call overhead. Rather than using elaborate conditionals or switches to handle function calls, simply assign the required function to a pointer, thus optimizing execution speed.
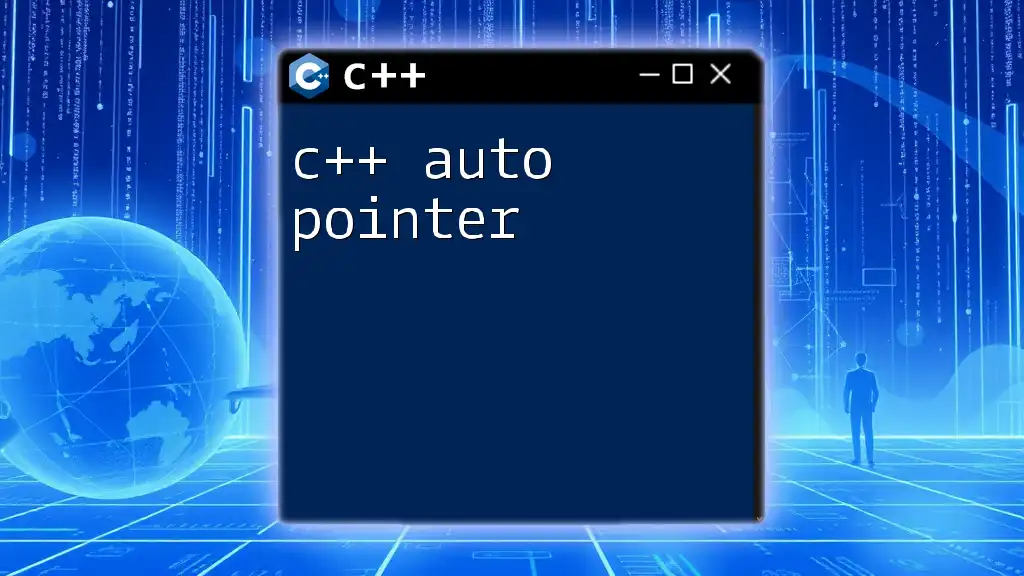
Common Mistakes with Function Pointers
When working with function pointers, programmers often make some common mistakes:
Mistakes to Avoid with Function Pointer Syntax
- Forgetting to match the function pointer signature with the function definition.
- Misusing parentheses in declarations, which can lead to compilation errors.
Debugging Function Pointer Issues
Debugging function pointer related issues can be challenging. Ensure that the pointer is properly initialized before use and check the function signature matches the pointer type. Print debugging statements can be helpful to verify address values.
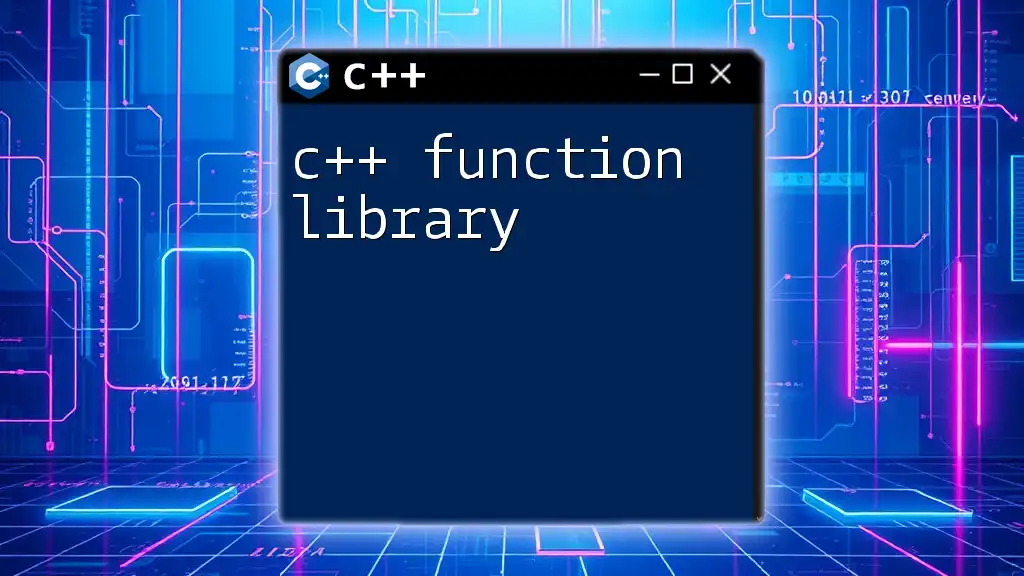
Conclusion
In summary, C++ function pointers are a powerful feature that enhances the flexibility and modularity of programs. They enable callbacks, event handling, and even optimize performance when used judiciously. By mastering function pointers, you equip yourself with a vital tool for advanced C++ programming.
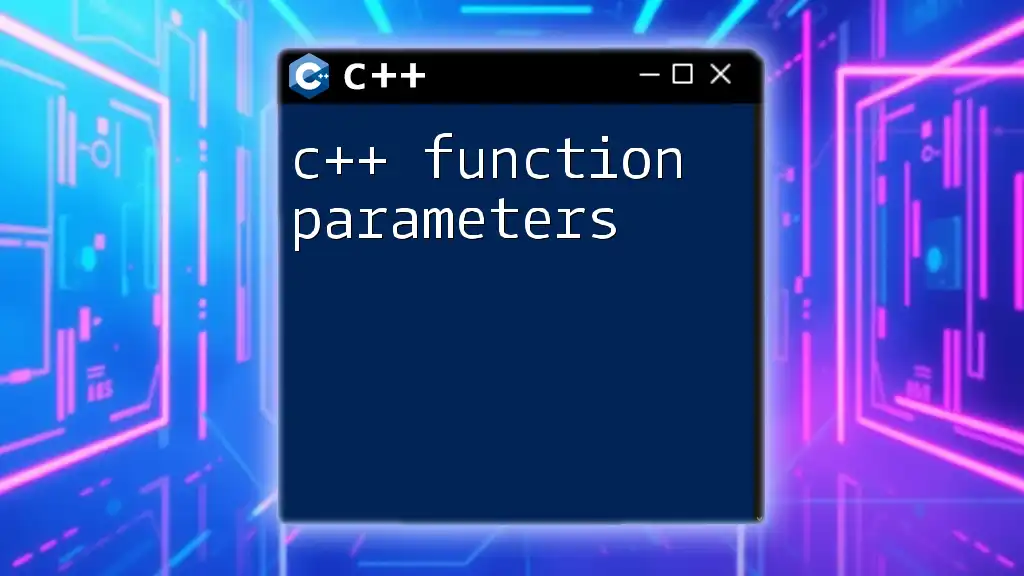
Additional Resources
For those eager to deepen their knowledge, consider checking out some highly recommended books and online tutorials specifically focused on C++. Engaging with community forums can also provide real-world insights and troubleshooting help, facilitating a smoother learning curve as you explore the world of function pointers in C++ programming.