In C++, an `auto` pointer allows the compiler to automatically deduce the type of a variable, simplifying code by removing the need for explicit type declarations.
Here's a simple example of using `auto` with pointers in C++:
#include <iostream>
int main() {
auto ptr = new int(42); // Automatically deduce ptr as an int*
std::cout << *ptr << std::endl; // Outputs: 42
delete ptr; // Clean up dynamically allocated memory
return 0;
}
Understanding C++ Auto Pointer
Definition of Auto Pointer
An auto pointer is a type of smart pointer that provides automatic memory management by ensuring that dynamically allocated memory is released when the auto pointer goes out of scope. This construct was part of earlier C++ standards, specifically designed to simplify memory handling by automatically destroying the object it points to when the auto pointer itself is destroyed.
Importance of Pointers in C++
Pointers are fundamental to C++ programming, allowing direct access to memory, dynamic memory allocation, and the manipulation of data structures. However, managing raw pointers requires careful handling to prevent memory leaks and dangling pointers. This is where smart pointers, like auto pointers, come into play, providing a safer alternative.

Evolution of Smart Pointers
From Raw Pointers to Smart Pointers
Raw pointers, while powerful, come with significant downsides, such as the risk of memory leaks or corruption due to manual memory management. As a result, C++ introduced smart pointers, designed to automate memory control and reduce the risk of errors.
The Role and Type of Smart Pointers
Smart pointers act as wrappers for raw pointers, controlling the lifecycle of the object they manage. The three primary types of smart pointers in C++ include:
- `unique_ptr`: A smart pointer that ensures exclusive ownership of an object and cannot be copied.
- `shared_ptr`: A smart pointer that allows multiple pointers to share ownership of the same object through reference counting.
- `weak_ptr`: A smart pointer that provides a non-owning reference to an object managed by a `shared_ptr`, helping to resolve circular dependencies.
Note: While `auto_ptr` served a role in early C++, it has since been deprecated, giving way to more robust alternatives like `unique_ptr`.
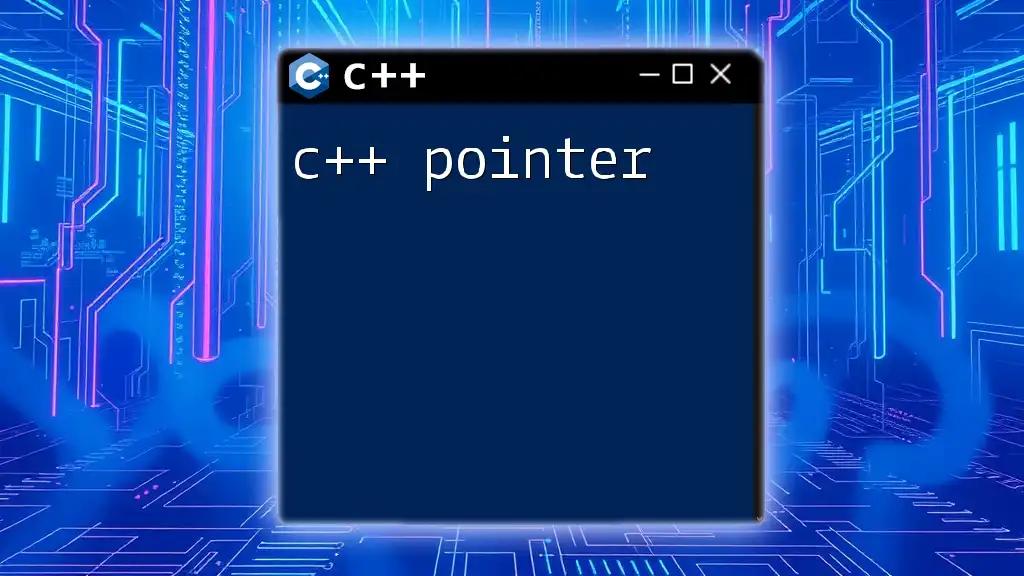
Understanding `auto_ptr`
What Is `auto_ptr`
The `auto_ptr` class is used to manage dynamically allocated memory automatically. It transfers ownership of a dynamically allocated object, which ensures that the object is deleted once the `auto_ptr` goes out of scope.
Syntax and Basic Usage
Here's how `auto_ptr` is generally declared and used:
std::auto_ptr<Type> ptr(new Type);
This line creates an `auto_ptr` that takes ownership of a dynamically allocated object of type `Type`.
Features of `auto_ptr`
One of the key features of `auto_ptr` is its ownership transfer semantics. When you copy an `auto_ptr`, the original pointer is set to `nullptr`, and the new `auto_ptr` takes over ownership of the allocated memory.
Code Snippet: Below is a simple example demonstrating ownership transfer using `auto_ptr`.
#include <iostream>
#include <memory>
void auto_ptr_example() {
std::auto_ptr<int> ptr1(new int(10)); // Creating an auto_ptr
std::cout << "ptr1 points to: " << *ptr1 << std::endl;
std::auto_ptr<int> ptr2 = ptr1; // Ownership transfer
std::cout << "ptr1 after transfer: " << ptr1.get() << std::endl; // Undefined behavior
std::cout << "ptr2 points to: " << *ptr2 << std::endl;
}
In this code, when `ptr2` is created from `ptr1`, `ptr1` is rendered unusable, which can lead to undefined behavior if we try to access it afterward.

Limitations of `auto_ptr`
Ownership Management Issues
The primary drawback of `auto_ptr` lies in its ownership management. When ownership is transferred, the original pointer is left in an undefined state. This behavior can lead to subtle bugs and makes it difficult to track resource ownership throughout the code.
Deprecated Status
In C++11, `auto_ptr` was marked as deprecated due to these management issues. Consequently, developers began to shift towards using `unique_ptr`, which provides a more robust and clearer ownership model, preventing such pitfalls.

Transitioning to `unique_ptr`
Why Use `unique_ptr` Instead of `auto_ptr`
The advantages of `unique_ptr` over `auto_ptr` include:
- Security: Unlike `auto_ptr`, `unique_ptr` does not allow copying, which prevents ownership from being inadvertently transferred.
- Clarity: `unique_ptr` provides explicit control over resource management, making ownership clearer in code.
Basic Usage of `unique_ptr`
Here’s a sample code showcasing how `unique_ptr` works with clear ownership transfer:
#include <iostream>
#include <memory>
void unique_ptr_example() {
std::unique_ptr<int> ptr1(new int(20)); // Creating a unique_ptr
std::cout << "ptr1 points to: " << *ptr1 << std::endl;
std::unique_ptr<int> ptr2 = std::move(ptr1); // Moving ownership
// ptr1 is now null
std::cout << "ptr1 after move: " << ptr1.get() << std::endl; // This will print '0' or null
std::cout << "ptr2 points to: " << *ptr2 << std::endl;
}
In this code, `ptr1` is moved to `ptr2`, making `ptr1` null and ensuring that memory is managed correctly without the risk of double deletion or undefined behavior.
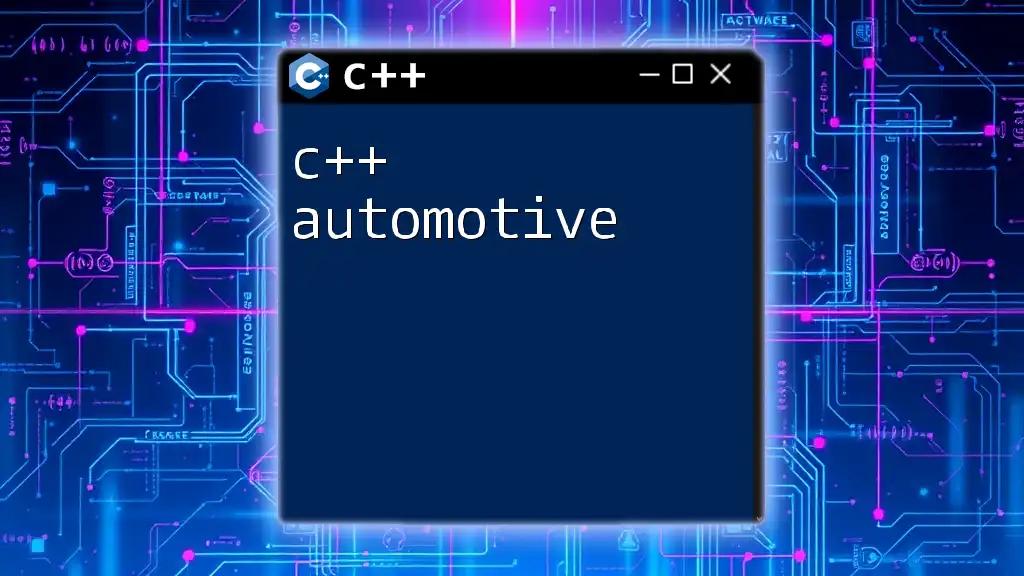
Best Practices for Using Smart Pointers
When to Use Smart Pointers
Smart pointers, such as `unique_ptr` and `shared_ptr`, should be preferred over raw pointers whenever possible. Their automatic management of memory makes them ideal for situations where ownership needs to be clear and predictable.
Common Pitfalls to Avoid
While smart pointers simplify management, pitfalls exist:
- Circular References: When using `shared_ptr`, it's essential to avoid situations where two or more pointers’ ownership references back to one another, leading to memory leaks.
- Memory Leaks: Always ensure that smart pointers are used correctly to prevent unintentional memory leaks; for instance, avoid mixing raw pointers and smart pointers.
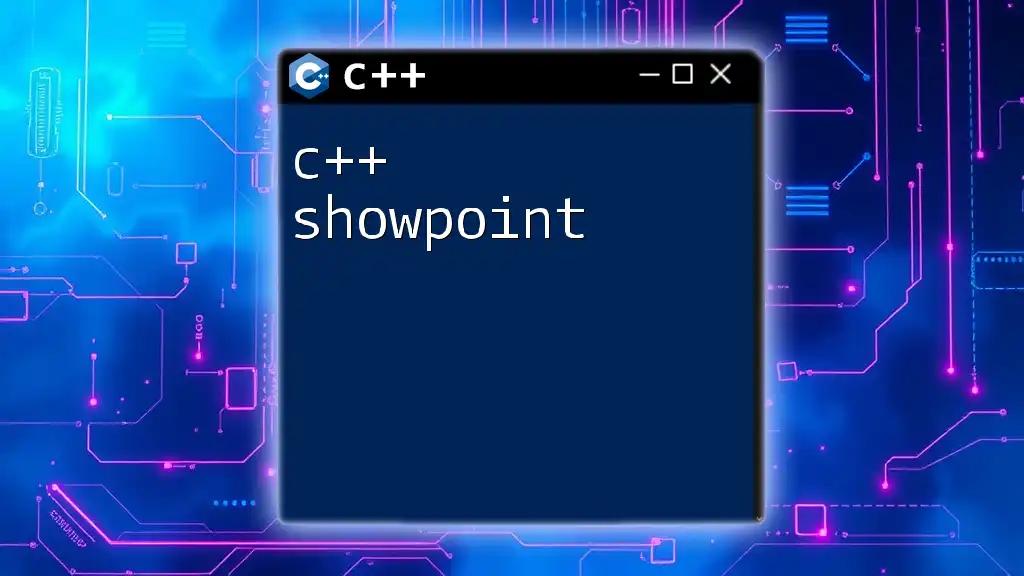
Conclusion
The transition from `auto_ptr` to `unique_ptr` represents a significant evolution in C++ memory management practices. By understanding and embracing these advancements, developers can improve their coding practices, promoting safer and more efficient memory handling in their applications. Utilizing modern constructs such as `unique_ptr` ensures improved clarity and reliability in resource management.
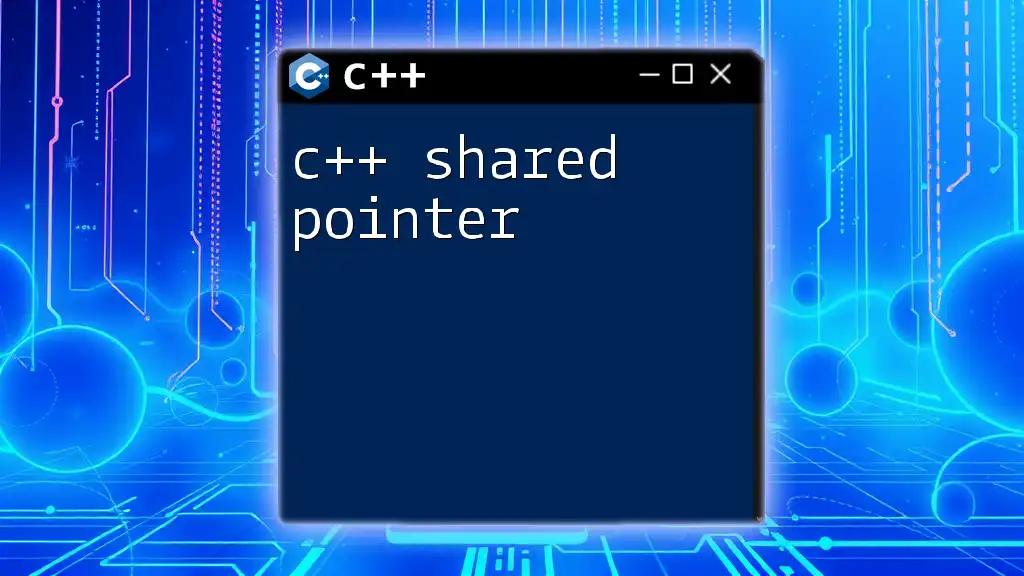
Additional Resources
For those interested in further exploring C++ memory management and smart pointers, consider the following resources:
- Recommended books and online courses for deeper learning.
- Links to official C++ documentation and smart pointer references that provide in-depth technical details.