C++ pointer arithmetic allows you to perform arithmetic operations on pointer values to traverse arrays or access elements based on their memory addresses.
#include <iostream>
int main() {
int arr[] = {10, 20, 30, 40, 50};
int* ptr = arr; // Pointing to the first element
// Accessing elements using pointer arithmetic
for (int i = 0; i < 5; i++) {
std::cout << *(ptr + i) << " "; // Output: 10 20 30 40 50
}
return 0;
}
Understanding Pointers in C++
A pointer in C++ is a variable that stores the memory address of another variable. Pointers play a critical role in C++, allowing for efficient memory management and enabling the creation of dynamic data structures. To declare a pointer, you use the asterisk (`*`) symbol, indicating that the variable will hold a memory address rather than a regular value.
For example:
int* myPointer; // Declaration of a pointer to an integer
Types of Pointers
Understanding different types of pointers helps in managing memory effectively:
- Null Pointers: A pointer that doesn't point to any valid location, commonly initialized as `nullptr`.
- Void Pointers: A pointer that can point to any data type, yet lacks information about the data type it is pointing to.
- Smart Pointers: Introduced in modern C++, smart pointers manage memory automatically, reducing the risk of memory leaks. Common types include `std::unique_ptr` and `std::shared_ptr`.
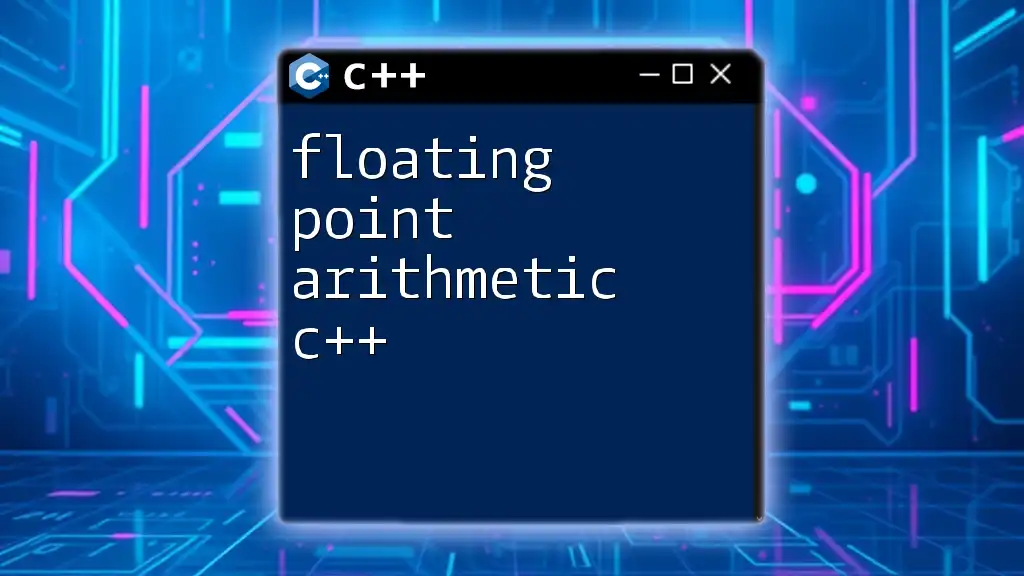
The Fundamentals of Pointer Arithmetic
Pointer arithmetic allows you to manipulate the addresses that a pointer holds. Each pointer type has a specific size that determines how many bytes are added or subtracted when you perform arithmetic operations. This is crucial for iterating through arrays or other data structures in C++.
Basic Operations
Pointer arithmetic primarily involves two operations: incrementing and decrementing pointers.
- Incrementing Pointers: When you increment a pointer (e.g., `ptr++`), it moves to the next memory location of the type it points to.
- Decrementing Pointers: Similarly, decrementing a pointer (e.g., `ptr--`) moves it to the previous memory location.
Memory and Address Calculation
To understand how pointer arithmetic works, you need to grasp the concept of memory layout. C++ uses both stack and heap for memory allocation, and pointers provide a way to navigate this memory.
When you increment or decrement a pointer, the memory address changes by the size of the data type it points to. For example, if you have an `int` pointer, moving forward by one will increase the address by `sizeof(int)` (typically 4 bytes on most systems).
This means that pointer calculations can be precise and are crucial for accessing data correctly.
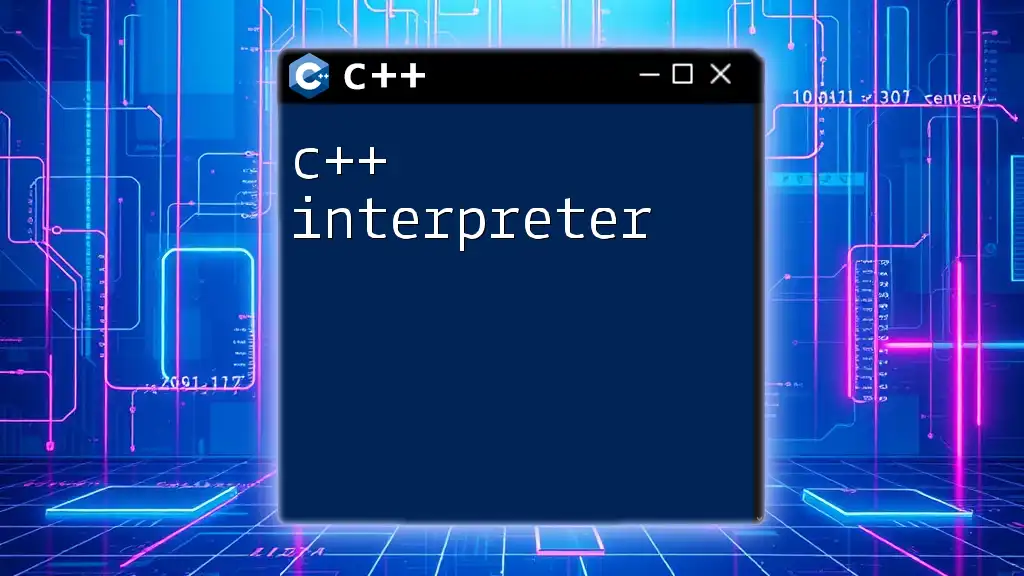
Detailed Pointer Arithmetic Operations
Adding and Subtracting Integers to Pointers
Adding an integer to a pointer effectively moves the pointer forward by a number of elements. This is particularly useful when dealing with arrays, as illustrated below:
int arr[] = {10, 20, 30, 40};
int* ptr = arr; // Points to the first element
ptr += 2; // Now points to arr[2], which is 30
Similarly, you can decrement a pointer to move backward:
ptr -= 1; // Now points to arr[1], which is 20
Subtracting Pointers
Pointer subtraction enables you to find the distance between two pointers. The distance is measured in terms of the number of elements between them, not bytes.
Here’s how you can do it:
int* ptr1 = &arr[1]; // Points to arr[1]
int* ptr2 = &arr[3]; // Points to arr[3]
int distance = ptr2 - ptr1; // Result: 2, as there are 2 elements in between
Pointer Comparison
Pointers can be compared using relational operators. For example, checking if one pointer points to a lower memory address than another:
if (ptr1 < ptr2) {
// Some logic when ptr1 points to an earlier location than ptr2
}
Pointer Arithmetic with Multi-dimensional Arrays
Navigating multi-dimensional arrays also relies on pointer arithmetic. For instance, to access elements in a 2D array, pointers can again be used to calculate specific memory addresses.
int arr[2][3] = {{1, 2, 3}, {4, 5, 6}};
int* ptr = &arr[0][0]; // Pointer to the first element
ptr += 4; // Now points to arr[1][1], which is 5
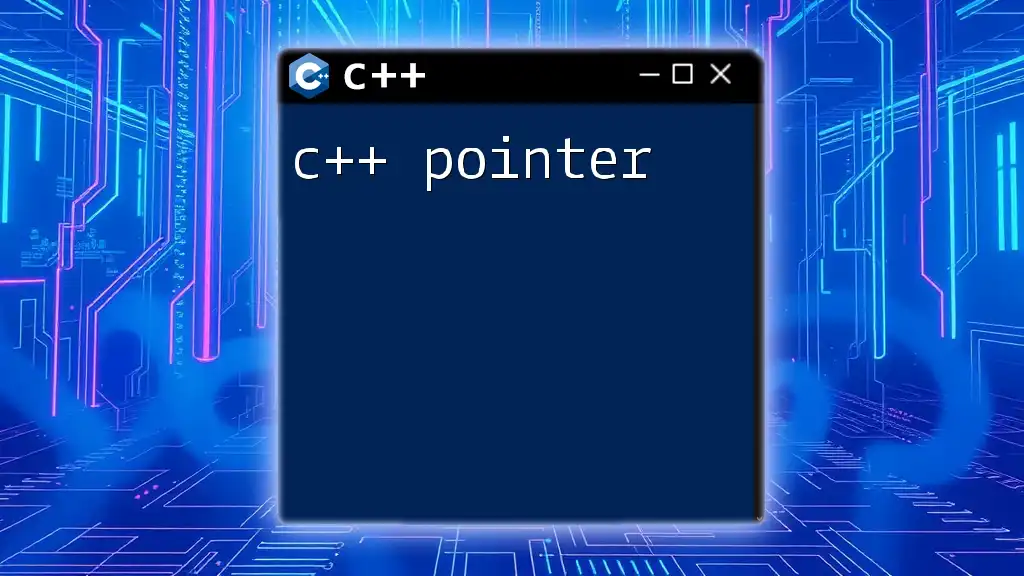
Best Practices for Using Pointer Arithmetic
While pointer arithmetic is powerful, it carries risks if not handled carefully. Here are some best practices to follow:
- Avoid Common Pitfalls: Make sure not to access out-of-bounds memory, as this leads to undefined behavior. Always validate pointer positions before performing arithmetic.
- Use Uninitialized Pointers Carefully: Accessing uninitialized pointers can cause program crashes or erratic behavior. Always initialize your pointers before use.
Debugging Pointers
Debugging pointer-related issues can often be tricky. Utilizing tools like Valgrind can help identify memory leaks or improper access to memory locations. Monitoring allocation and deallocation diligently ensures your code runs smoothly.
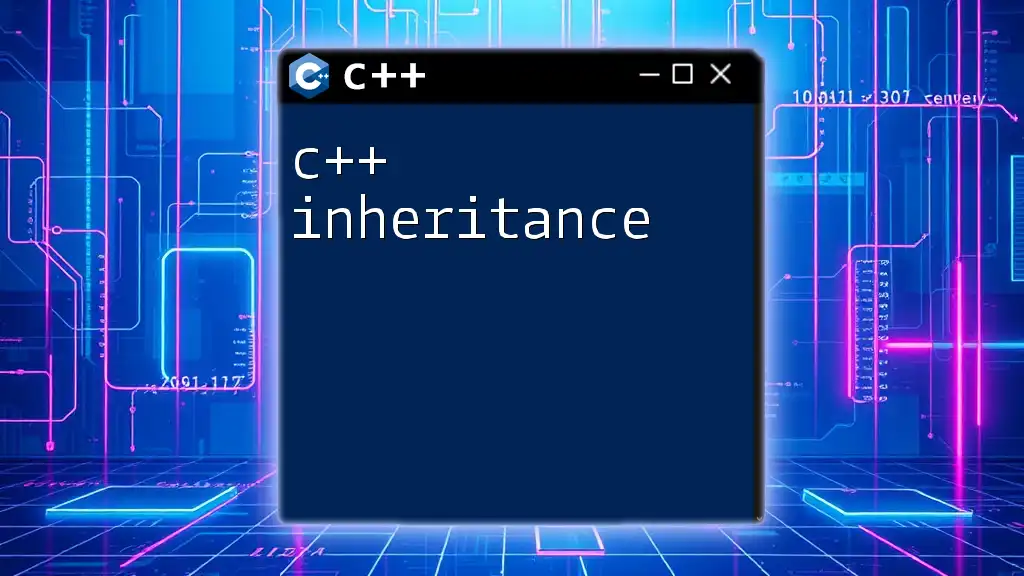
Real-world Examples of Pointer Arithmetic
In practice, pointer arithmetic is frequently used in dynamic arrays and complex data structures like linked lists.
Dynamic Arrays
Using pointer arithmetic can enable resizing and resizing operations in dynamic arrays, which is fundamental in various applications, from simple programs to complex systems.
Data Structures
Let’s consider a linked list. Here’s a code snippet for traversing a linked list using pointers:
struct Node {
int data;
Node* next;
};
Node* head; // Pointer to the first node
Node* current = head; // Start at the head
while (current != nullptr) {
// Process current node
current = current->next; // Move to the next node
}
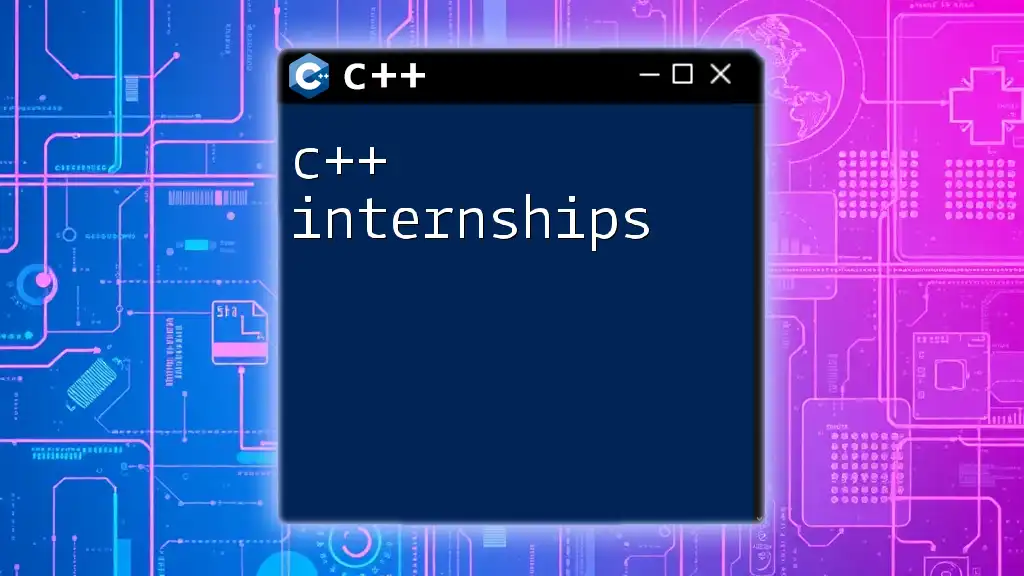
Conclusion
Understanding C++ pointer arithmetic is essential for any programmer looking to master the language. It allows for efficient memory manipulation, essential for dynamic data structures and optimized algorithms. By mastering pointer arithmetic, you’ll gain greater control over memory and increase the performance of your C++ programs. Continue to practice these concepts to solidify your understanding and apply them in various coding scenarios.