Floating point arithmetic in C++ allows for the representation and manipulation of real numbers using decimal points, enabling calculations that require fractional values.
#include <iostream>
int main() {
float a = 5.5f, b = 2.0f;
float sum = a + b;
std::cout << "The sum is: " << sum << std::endl; // Output: The sum is: 7.5
return 0;
}
What are Floating Point Numbers?
Floating point numbers represent real numbers within a certain range of values, allowing for the representation of fractions as well as very large and very small numbers. In C++, floating point types are primarily used for calculations that require a decimal representation.
The main difference between floating point and integer types lies in their ability to represent fractional values. While integers can only hold whole numbers (e.g., -1, 0, 1), floating point types can represent numbers like 3.14, -2.5, or 0.001.
Floating point numbers in C++ adhere to the IEEE 754 standard, which defines how these numbers are stored in memory. There are two major categories of floating point types:
- Single precision (`float`): Typically 32 bits in size, providing approximately 7 decimal digits of precision.
- Double precision (`double`): Typically 64 bits, offering about 15 decimal digits of precision.
Understanding the nuances of these types is essential for accurate calculations and avoiding unintended errors.
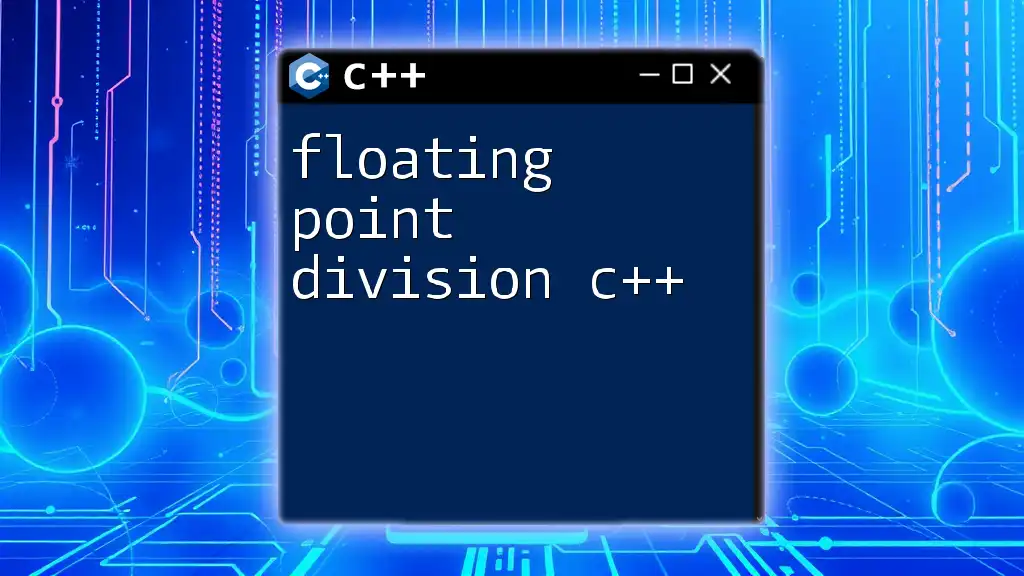
Representing Floating Point Numbers in C++
In C++, representing floating point numbers is straightforward with specific data types. You can declare variables using the following syntax:
float a = 3.14f; // float literal
double b = 2.718281828459; // double literal
When declaring floating point literals, it is important to use the suffix (`f` for float) to specify the type explicitly. This ensures that the number is correctly treated as a float rather than a double.
C++ also provides the `<limits>` library, which is useful for determining the numeric limits and precision for floating point types.
For example:
#include <limits>
std::cout << "Max float: " << std::numeric_limits<float>::max() << std::endl;
This snippet uses the numeric limits to show the maximum value a `float` type can hold. Understanding these limits is crucial when dealing with computations that can reach extreme values.
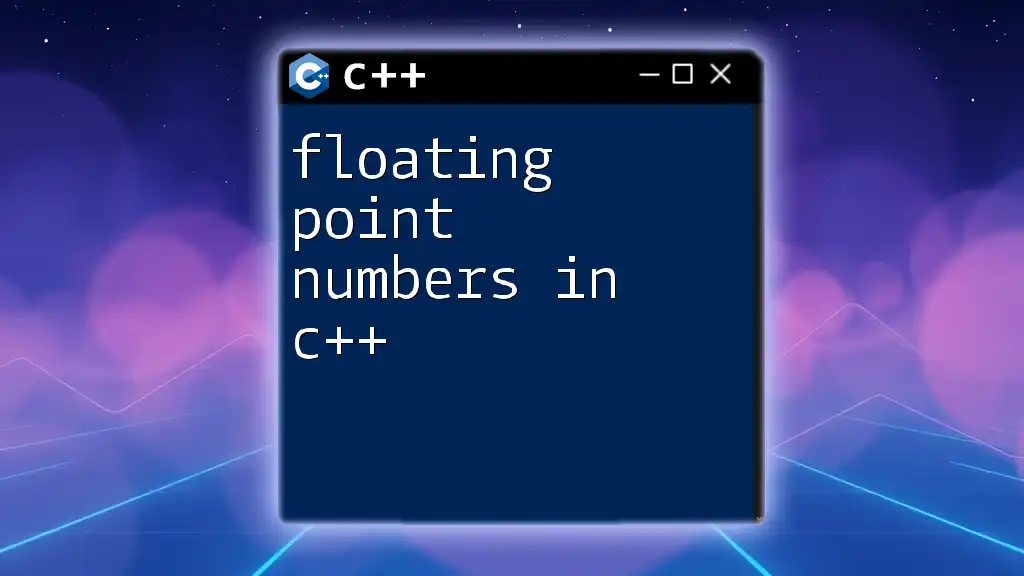
Basic Operations on Floating Point Numbers
Performing arithmetic operations with floating point numbers in C++ is similar to integer operations; however, precision issues may arise.
For example, when adding two floating point numbers, the result may not be as expected:
float x = 1.0f, y = 0.1f;
float sum = x + y; // possible precision issues
In floating point arithmetic, small rounding errors can accumulate, leading to unexpected results.
When it comes to multiplication and division, it is essential to handle potentially problematic situations, such as division by zero:
float result = x / y; // valid; but check for zero
While division is valid here, always remember to check if the divisor is zero to prevent runtime errors.
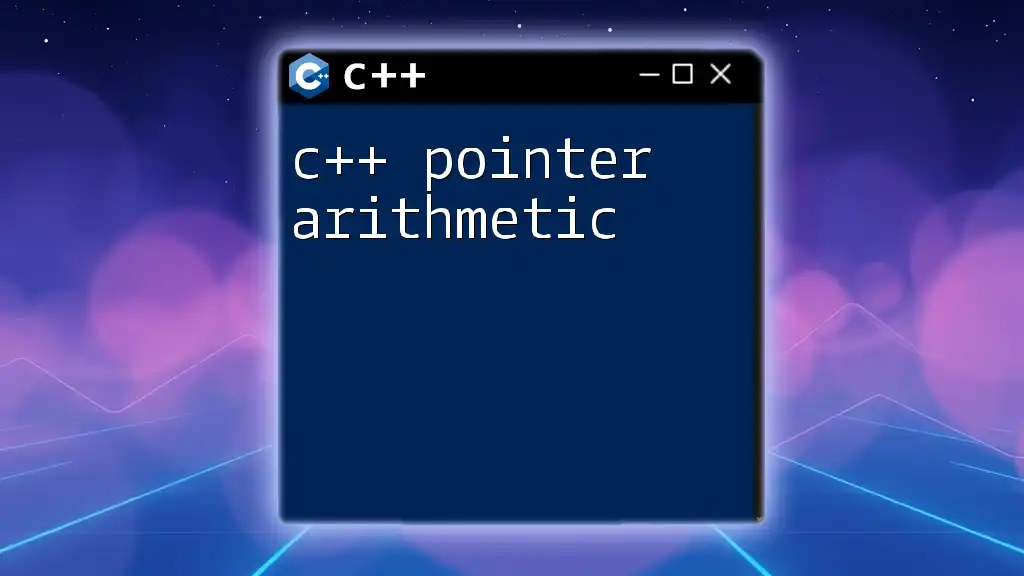
Precision and Rounding Errors
One of the most significant challenges with floating point arithmetic in C++ is rounding errors. These errors can occur because some decimal fractions cannot be represented exactly in binary, leading to inaccuracies in subsequent calculations.
For instance, consider the following example:
float a = 0.1f + 0.2f;
if (a == 0.3f) {
std::cout << "Equal!" << std::endl; // may not print
}
Even though mathematically, 0.1 + 0.2 equals 0.3, the way that floating point arithmetic is handled may cause the comparison to fail due to minor discrepancies in the representation of these values.
To mitigate such issues, it is advisable to use a tolerance level when comparing floating point numbers:
const float EPSILON = 0.00001f;
if (fabs(a - 0.3f) < EPSILON) {
std::cout << "Approximately Equal!" << std::endl;
}
By introducing a small `EPSILON` value, we acknowledge that values can be very close to each other without being exactly equal, thereby improving the reliability of your comparisons.
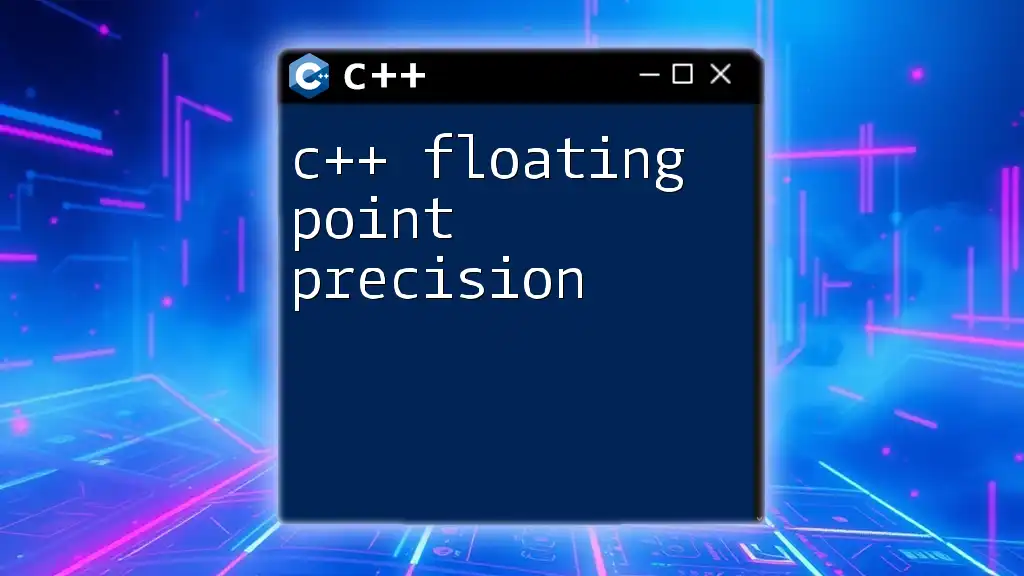
Advanced Floating Point Operations
C++ provides a variety of mathematical functions from the `<cmath>` library that work seamlessly with floating point numbers. These functions are essential for advanced computations in various fields such as physics, engineering, and computer graphics.
Common functions include:
- Trigonometric functions: `sin()`, `cos()`, `tan()`
- Exponential functions: `exp()`, `pow()`
- Root functions: `sqrt()`
Here’s an example of using the sine function:
#include <cmath>
double angle = 45.0; // in degrees
double radians = angle * (M_PI / 180.0); // converting degrees to radians
std::cout << "Sin: " << sin(radians) << std::endl;
In this code snippet, we convert degrees to radians to calculate the sine of an angle, which is crucial in many mathematical and physical applications.
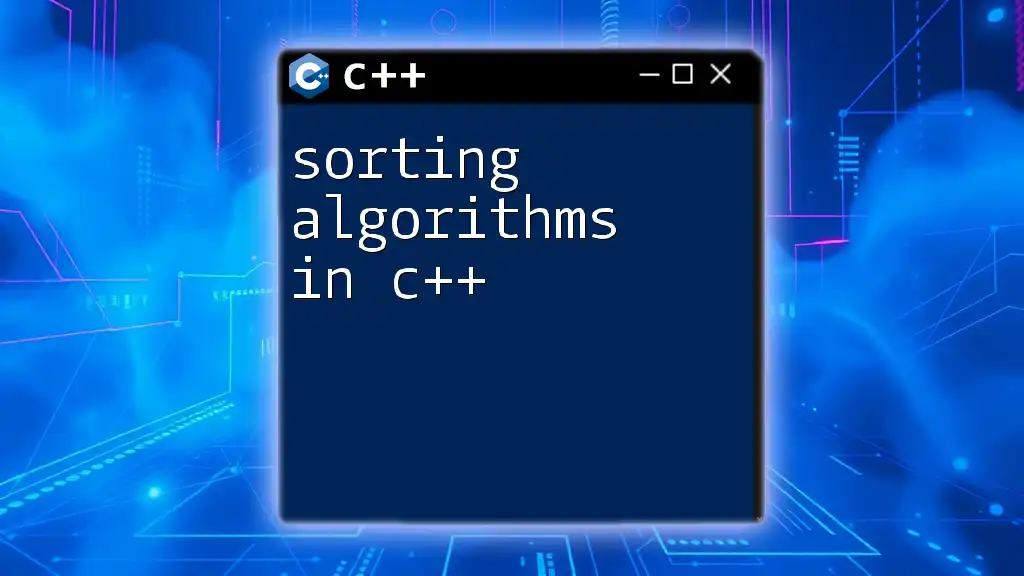
Performance Considerations
When working with floating point arithmetic in C++, performance may be impacted compared to integer arithmetic, primarily due to the complexity of floating point operations.
Profiling the performance of floating point operations can provide insights into how efficiently your application runs. Utilize specific tools for performance analysis, such as gprof or Valgrind, which can help you identify bottlenecks in the code.
Consider monitoring hotspots where floating point operations are heavily utilized, especially in graphics rendering or data simulation tasks.
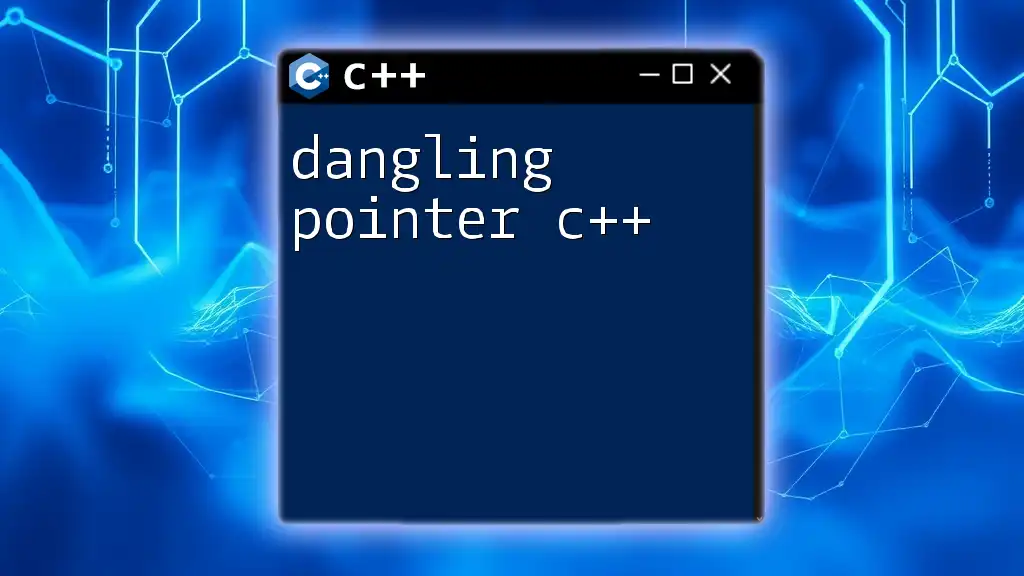
Conclusion
In summary, floating point arithmetic in C++ is a powerful tool for representing and manipulating real numbers. However, it comes with challenges, such as rounding errors and precision issues, that developers must be aware of. By following best practices, such as using tolerance levels for comparisons and being informed about the intrinsic limitations of floating point types, you can harness the potential of floating point arithmetic effectively.
Deeply understanding floating point arithmetic in C++ not only enhances your coding skills but also prepares you for more complex programming tasks in various applications. As you continue to explore this topic, remember that knowledge of floating point operations can significantly optimize your computational workflows.
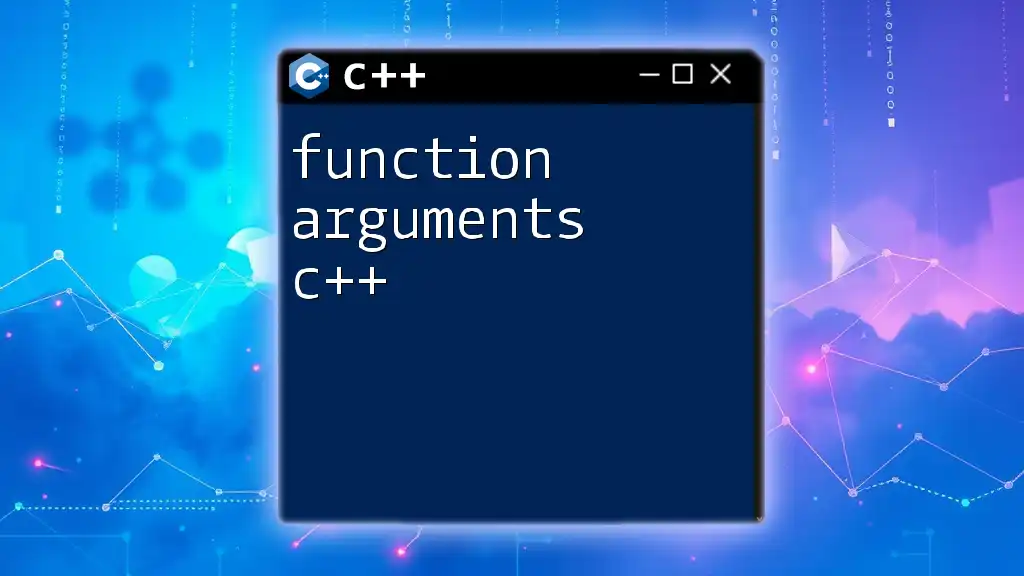
Additional Resources
For those interested in diving deeper into floating point arithmetic and its applications in C++, consider exploring recommended books, online tutorials, and the official documentation provided by the C++ standards committee. Understanding the theory behind floating point representation will further enhance your programming acumen and problem-solving capabilities.