Floating-point numbers in C++ are numeric data types that represent real numbers, allowing for the representation of fractional values using a decimal point.
Here's a simple example demonstrating the declaration and use of floating-point numbers in C++:
#include <iostream>
int main() {
float pi = 3.14f; // Single precision floating-point
double e = 2.718281828459; // Double precision floating-point
std::cout << "Value of Pi: " << pi << "\n";
std::cout << "Value of e: " << e << "\n";
return 0;
}
Understanding Floating Point Numbers in C++
What is a Floating Point Number?
Floating point numbers are a crucial concept in programming that allow for the representation of real numbers in a way that can accommodate a vast range of values. Unlike integer types, which can only represent whole numbers, floating point numbers can represent fractions and decimals. This is particularly important in calculations that require precision and scalability, such as scientific computations or financial applications.
Why Use Floating Point Numbers?
Floating point numbers are essential for various applications where precision and range matter. They are crucial in:
- Scientific calculations, where large and small values often arise.
- Graphics programming, where coordinate positions and colors might require decimal precision.
- Any situation where errors from rounding whole numbers can lead to significant discrepancies.
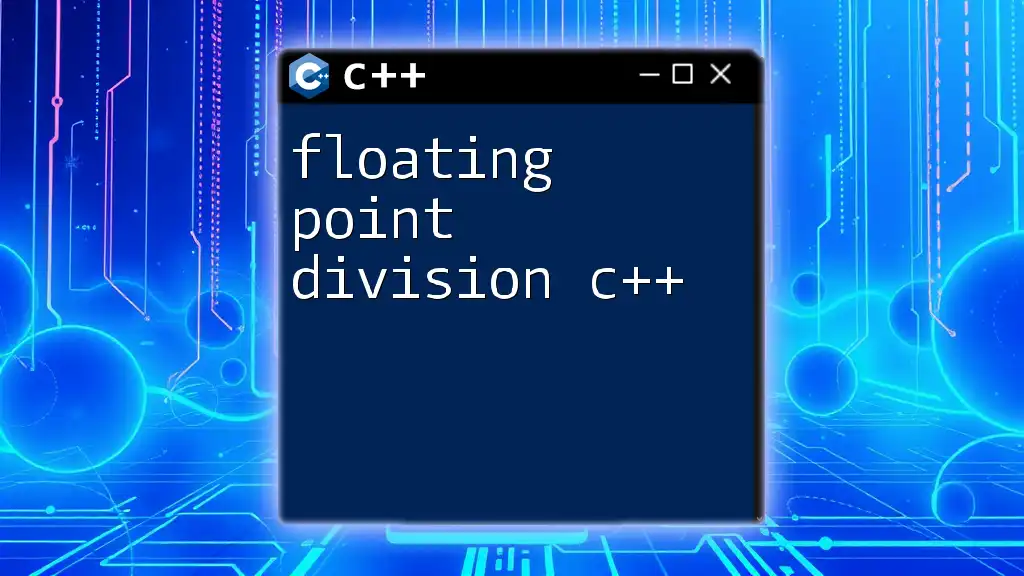
Types of Floating Point Numbers in C++
The float Data Type
In C++, the `float` data type is used to represent single-precision floating point numbers. It typically occupies 4 bytes in memory and provides a precision of about 6 to 7 decimal digits.
Here's an example of declaring and using a `float` number:
float myFloat = 3.14f;
std::cout << myFloat << std::endl; // Output: 3.14
The double Data Type
The `double` data type is utilized for double-precision floating point numbers, which occupy 8 bytes and provide a much higher precision of about 15 to 16 decimal digits. This is preferred when calculations require more accuracy.
Example of declaring and using a `double` number:
double myDouble = 3.14159265359;
std::cout << myDouble << std::endl; // Output: 3.14159265359
The long double Data Type
For situations requiring even greater precision, C++ offers the `long double` type, usually taking up 12 or 16 bytes of memory depending on the compiler and hardware. This data type provides extended precision beyond that of `double`.
Example declaration and usage:
long double myLongDouble = 3.14159265358979323846L;
std::cout << myLongDouble << std::endl; // Output: 3.14159265358979323846
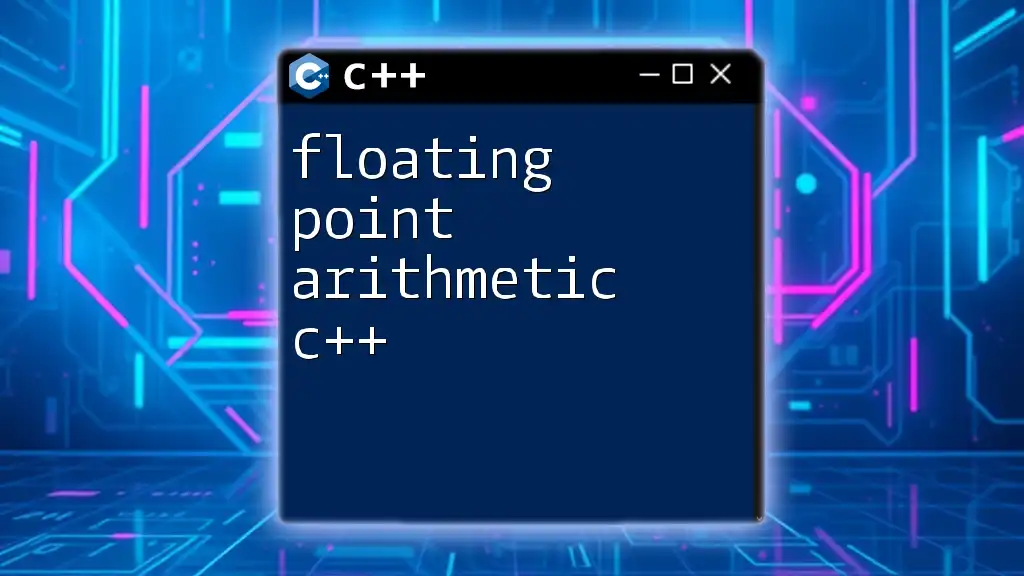
Floating Point Representation in C++
IEEE 754 Standard
The representation of floating point numbers in C++ is governed by the IEEE 754 standard, which defines how these numbers are stored in binary format. This standard divides a floating point number into three components: the sign bit, the exponent, and the mantissa.
- Sign Bit: Indicates whether the number is positive or negative.
- Exponent: Represents the scale of the number, determining how many places the decimal point moves.
- Mantissa: Contains the significant digits of the number.
Understanding this representation is essential when dealing with floating point arithmetic and ensures that your computations are consistent across different systems.
Precision and Range of Floating Point Numbers
Precision is one of the most critical aspects of floating point numbers. The different types (`float`, `double`, and `long double`) have varying levels of precision.
It's important to note that operations involving floating point numbers can lead to unexpected results due to their limited precision. For instance:
float f1 = 1.0f / 3.0f; // Result might not be exact
std::cout << f1 << std::endl; // Output may vary slightly
In contrast, using a `double` might yield a more accurate result:
double d1 = 1.0 / 3.0;
std::cout << d1 << std::endl; // More precise output
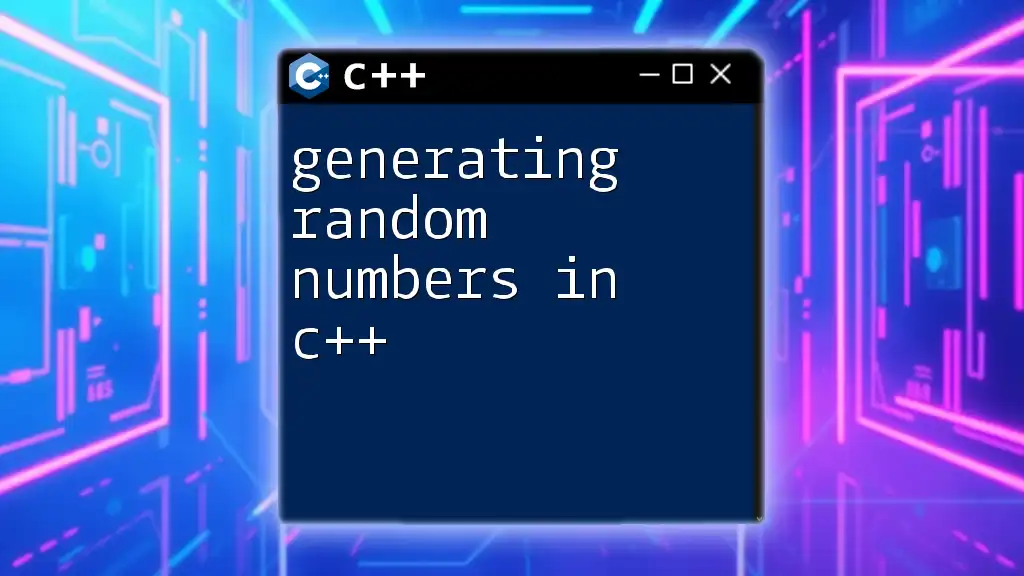
Operations with Floating Point Numbers
Basic Arithmetic Operations
Floating point numbers support standard arithmetic operations like addition, subtraction, multiplication, and division. Here's an illustration:
float a = 5.0f;
float b = 2.0f;
float c = a + b; // Example of addition
std::cout << c << std::endl; // Output: 7.0
Comparison of Floating Point Numbers
Comparing floating point numbers can be tricky due to precision errors. It is generally recommended to avoid direct comparisons. For instance:
float x = 0.1f + 0.2f;
float y = 0.3f;
if (fabs(x - y) < 0.0001) {
std::cout << "x and y are considered equal" << std::endl; // Safe comparison
}
Using a threshold, or epsilon, to determine equality is a best practice in floating point arithmetic.
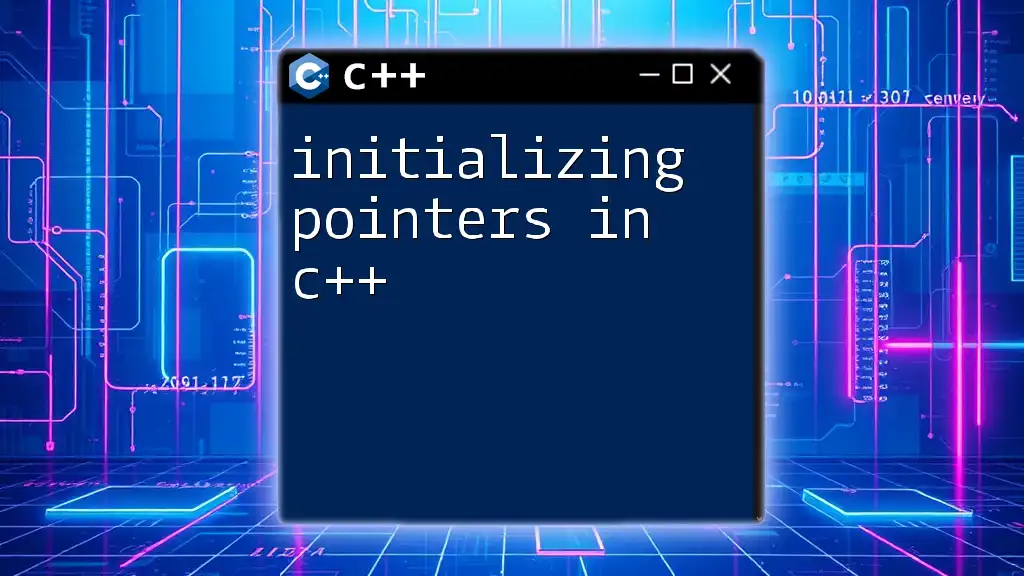
Common Pitfalls and Best Practices
Precision Loss in Calculations
Precision loss is a common issue when performing arithmetic operations with floating point numbers. It can lead to subtle bugs and inaccuracies in programs. For example, adding very small floating point numbers can yield unexpected results due to rounding errors.
Avoiding Floating Point Errors
To minimize floating point errors, consider these best practices:
- Be mindful of the operations you perform and the types you use.
- Use higher precision types when necessary (prefer `double` or `long double` when dealing with very precise calculations).
- Utilize format specifiers to control output precision. For example:
#include <iomanip>
std::cout << std::setprecision(5) << myDouble << std::endl; // Controls output precision
These practices will help you manage precision and ensure your computations yield reliable results.
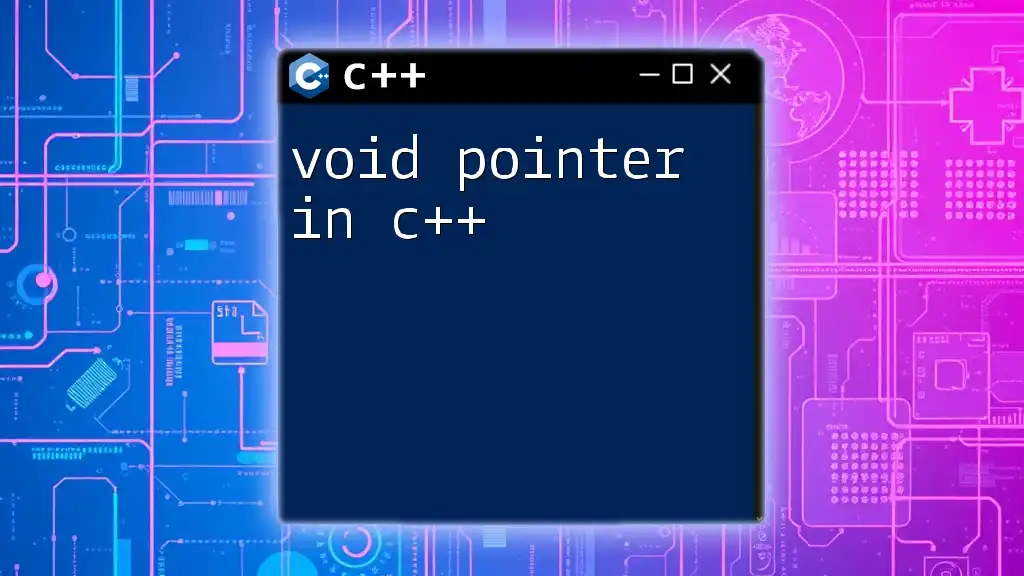
Conclusion
Understanding floating point numbers in C++ is essential for programmers dealing with real numbers, especially in contexts requiring precision and scalability. As you delve deeper into the complexities of computations, being aware of the types available, their representation, and common pitfalls will empower you to create more robust and accurate applications. Take the knowledge you've gained, apply it, and continue exploring further into the fascinating world of C++.