In C++, floating point division can be performed by using the division operator `/` with at least one operand as a floating-point number (float or double), ensuring that the result is a floating-point value instead of an integer.
#include <iostream>
int main() {
double a = 5.0;
double b = 2.0;
double result = a / b; // performs floating point division
std::cout << "Result: " << result << std::endl; // Output: Result: 2.5
return 0;
}
Understanding Floating Point Numbers
What are Floating Point Numbers?
In C++, floating point numbers are a category of data types used to represent real numbers that can have a fractional part. Unlike integers, which can only represent whole numbers, floating point numbers can capture both the whole and decimal parts. C++ provides three primary floating point types: `float`, `double`, and `long double`. Each type varies in terms of precision and range, making it essential to choose the appropriate type based on your application's precision requirements.
Precision and Accuracy
Precision is vital when performing calculations in programming. Floating point numbers are subject to rounding errors due to their representation in binary format. The IEEE 754 standard is a widely adopted guideline that specifies how floating point numbers are stored and managed in computing systems.
Despite its advantages, floating point arithmetic may not always yield perfectly accurate results due to these internal representations. Understanding precision, as well as the potential pitfalls associated with floating point arithmetic, is crucial for developers.
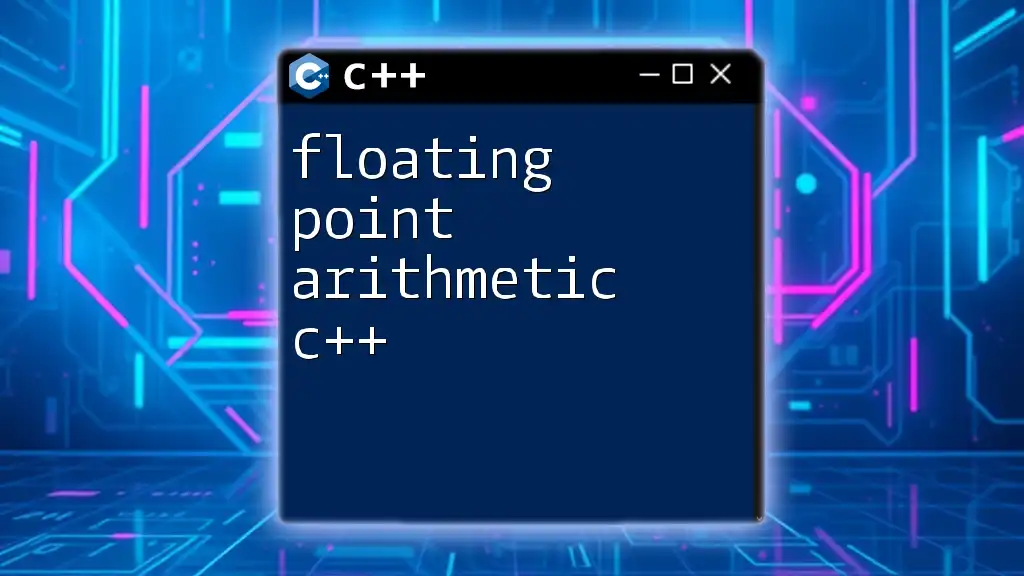
Basic Syntax of Floating Point Division in C++
C++ Division Operator
In C++, the division of floating point numbers is performed using the division operator `/`. The syntax for dividing two floating point variables is straightforward:
float a = 5.0f;
float b = 2.0f;
float result = a / b;
In this example, the value of `result` would be `2.5`. It’s essential to ensure that at least one of the variables (`a` or `b`) is declared as a floating type (e.g., `float`, `double`) so that the division does not perform integer division.
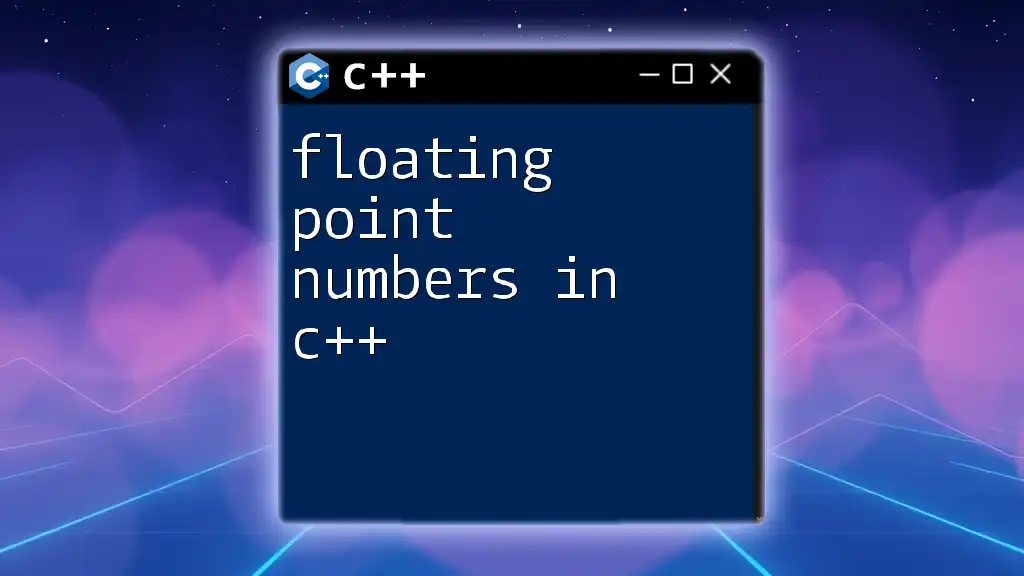
Common Issues in Floating Point Division
Division by Zero
One of the most critical issues developers face while performing floating point division is division by zero. Attempting to divide by zero can lead to runtime errors, which can crash your program or produce undefined behavior.
To handle this scenario gracefully, incorporate checks in your code:
if (b != 0) {
float result = a / b;
} else {
// Handle the error
std::cerr << "Error: Division by zero!" << std::endl;
}
This code snippet ensures that your application does not attempt to divide by zero, which is a crucial part of error handling in any reliable software.
Floating Point Precision Errors
Floating point precision can lead to unexpected results, particularly when performing arithmetic operations. For instance:
float x = 0.1f;
float y = 0.2f;
if ((x + y) == 0.3f) {
// This might not evaluate as true
}
In this example, the summation of `x` and `y` may not equal `0.3` due to floating point precision errors. Developers should strive to minimize these errors by following best practices, such as using `double` instead of `float` when higher precision is necessary.
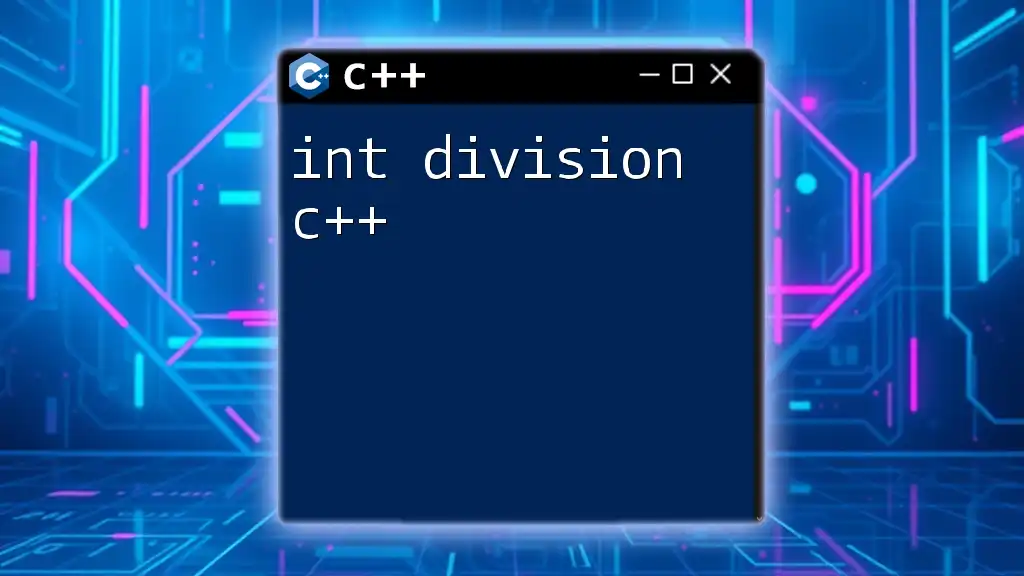
Advanced Floating Point Division Techniques
Using Standard Libraries and Functions
Standard libraries can facilitate more robust floating point arithmetic. While the basic `/` operator is often sufficient, functions like `std::fdiv` (if applicable) can help enhance precision and provide additional functionality.
Custom Division Functions
Creating custom functions for division can improve error handling and accuracy. You can define a utility function such as:
float safeDivide(float numerator, float denominator) {
if (denominator == 0.0f) {
throw std::invalid_argument("Denominator cannot be zero.");
}
return numerator / denominator;
}
This `safeDivide` function not only checks for division by zero but also throws an exception, elevating the control flow for better error handling.
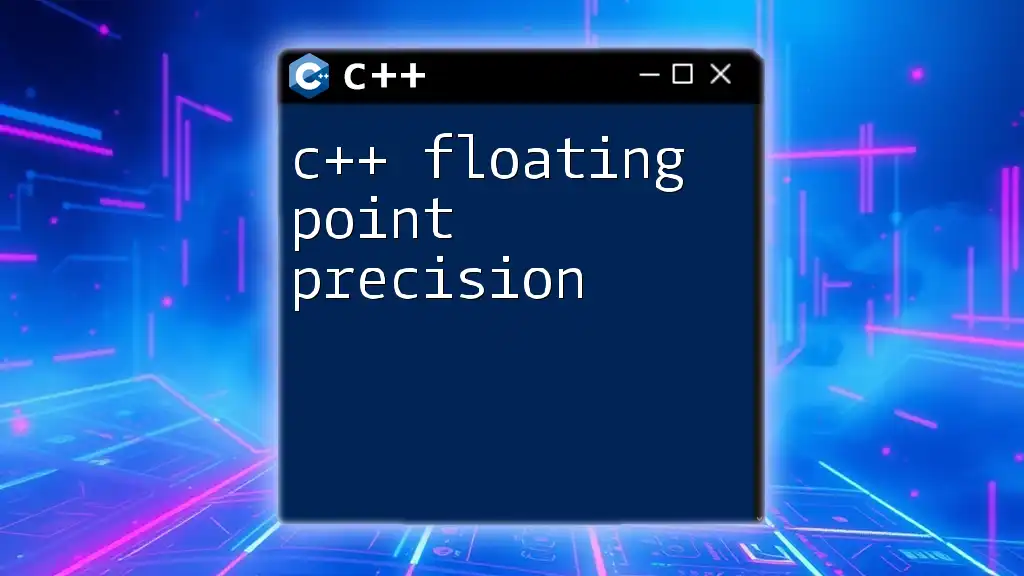
Best Practices for Floating Point Division
Choosing Appropriate Data Types
Selecting the right floating point data type is crucial. Here is a brief overview:
- float: Single-precision (typically 32 bits); suitable for applications where memory savings are important, and precision can be compromised.
- double: Double-precision (typically 64 bits); recommended for most applications requiring numerical calculations due to its enhanced precision.
- long double: Extended precision; useful in specialized applications where even higher precision is required.
Using a type fit for your application's requirements ensures better performance and reliability.
Error Handling Techniques
Strong error handling is a cornerstone of robust software. Utilizing exceptions can help capture issues during division operations. For instance, using `try-catch` blocks can be beneficial:
try {
float result = safeDivide(a, b);
} catch (const std::invalid_argument& e) {
std::cerr << e.what() << std::endl;
}
Commenting and Documentation
Always strive to make your code understandable. Clear comments and documentation are essential for clarity—not just for others but also for your future self. Documenting the purpose of division operations, especially custom functions, will significantly aid in maintenance and debugging later.
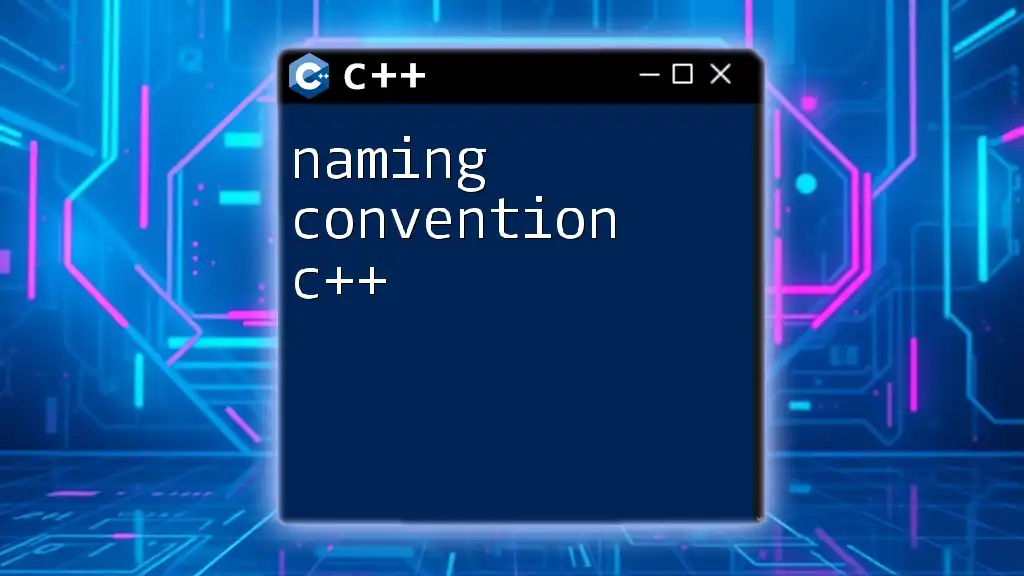
Real-life Applications of Floating Point Division
Use Cases
Floating point division is ubiquitous in various programming scenarios, such as graphics rendering, scientific computations, and financial calculations. For instance, in graphics programming, division is often necessary for perspective projection calculations.
Case Study
Consider a simulation application that models the trajectory of a projectile. Accurate calculations to determine velocity, distance, and time rely heavily on floating point divisors to ensure realistic simulation results. Using precision-aware floating point division is crucial for achieving realistic outcomes in such instances.
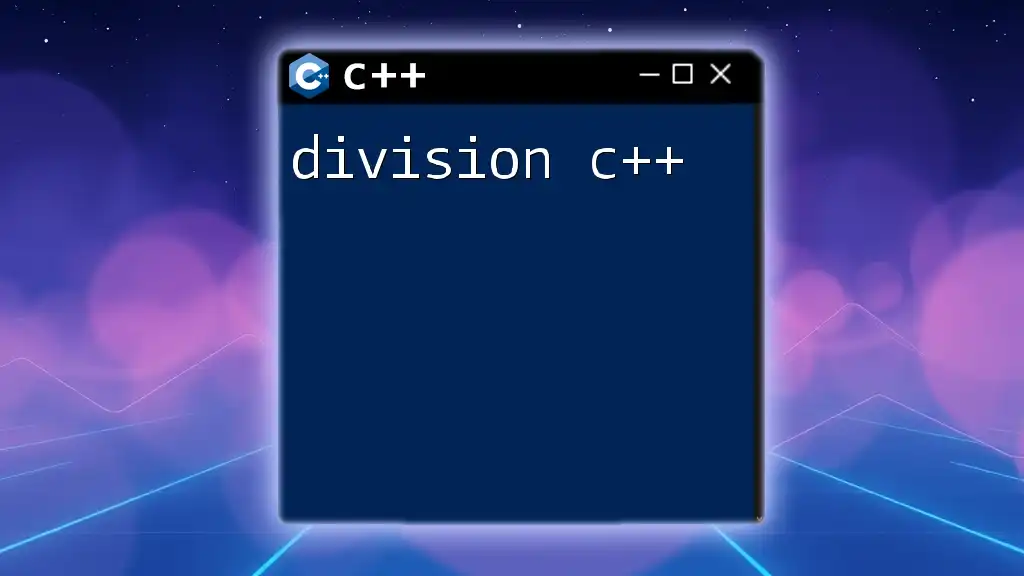
Conclusion
Floating point division in C++ is an essential aspect of numerical computing. Understanding how to correctly utilize floating point arithmetic, handle common issues like division by zero and precision errors, and follow best practices is critical for any developer. This knowledge not only aids in creating reliable applications but also builds a foundation for further exploration into the complexities of numerical programming.
As you continue your journey in mastering floating point division and C++, various resources such as online tutorials and documentation can provide deeper insights and advanced techniques. Embrace the learning process, and the benefits will be substantial in your programming endeavors.