In C++, naming conventions are a set of guidelines that dictate how identifiers such as variables, functions, and classes should be named to improve code readability and maintainability.
Here’s an example code snippet following common C++ naming conventions:
#include <iostream>
class MyClass {
public:
void myFunction() {
int localVariable = 10;
std::cout << "Value: " << localVariable << std::endl;
}
};
Understanding C++ Naming Conventions
What Are Naming Conventions?
Naming conventions are established guidelines that dictate how to name variables, functions, classes, and other entities in a programming language. The main goal of these conventions is clarity; they ensure that names are understandable and relevant to the context, allowing both the original programmer and others who might read or edit the code later to grasp its functionality quickly.
In C++, the effective use of naming conventions can significantly improve code readability and reduce the cognitive load on developers. When everyone adheres to a consistent naming scheme, collaborative coding becomes less error-prone and easier to manage.
Why Use Naming Conventions in C++?
Using proper naming conventions in C++ is crucial for various reasons:
- Enhancing code clarity and understanding: When names are meaningful, it's easier to deduce the purpose of variables or functions without needing to read through implementation details.
- Facilitating code maintenance and scalability: As projects grow, a consistent naming scheme makes it easier to locate and modify code.
- Avoiding naming conflicts and ambiguity: A well-thought-out convention reduces the chance of accidentally using the same name for different entities, which can lead to bugs that are difficult to trace.

Common C++ Naming Convention Standards
CamelCase
The CamelCase style capitalizes the first letter of each word while leaving no spaces between them. This method is typically used for class names and function names.
Example:
class MyClass {
public:
void DoSomething();
};
snake_case
In the snake_case style, words are separated by underscores, and all letters are typically in lowercase. This naming convention is often applied to variable names.
Example:
int my_variable = 5;
PascalCase
Similar to CamelCase, PascalCase capitalizes the first letter of each word, but it may be used in contexts distinct from CamelCase, such as when naming types or functions.
Example:
void ProcessData() {
}
kebab-case (not commonly used in C++)
The kebab-case style uses hyphens to separate words. While it's prevalent in file naming and URL construction, it is rarely, if ever, used in C++ due to language syntax constraints, which do not allow hyphens in identifiers.
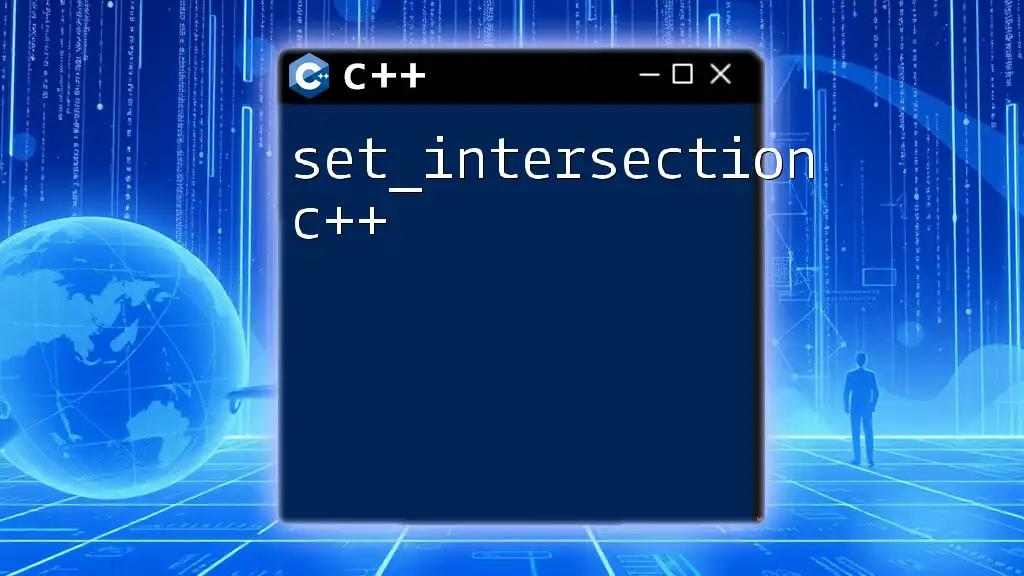
Naming Conventions for Different Elements in C++
Naming Variables
The guidelines for variable naming suggest that names should be descriptive while being concise. The general convention is to use snake_case for variables. It's essential to keep variable names meaningful and directly linked to their purpose.
Example:
float average_score;
Naming Functions
Functions should convey their actions through their names. A common practice in C++ is to use CamelCase or PascalCase for function definitions. The name should generally start with a verb.
Example:
void CalculateSum(int a, int b);
Naming Classes
Classes in C++ typically follow the CamelCase convention. Using nouns or noun phrases that describe the role of the class makes it easier for others to grasp its purpose at a glance.
Example:
class StudentProfile {
};
Naming Constants
Constants are usually named using UPPER_SNAKE_CASE, which capitalizes all letters and separates words with underscores. This visual distinction helps identify constants quickly.
Example:
const int MAX_USERS = 100;
Naming Enums
For enumerations, the convention is to name the enum type using CamelCase and its members using UPPER_SNAKE_CASE. This clarifies the relationship between the enum type and its member values.
Example:
enum Direction { NORTH, EAST, SOUTH, WEST };

Scope and Context in Naming Conventions
Local vs. Global Variables
The naming conventions for local and global variables can differ. It's generally advisable to prefix global variables with "g_" to indicate their scope, minimizing confusion with local variables.
Example:
int g_globalValue; // Global
void function() {
int localValue; // Local
}
Namespace Considerations
Namespaces are a vital feature in C++, helping to organize code and prevent name collisions. It’s prudent to use the namespace name as a prefix when naming classes and functions within that context.
Example:
namespace Math {
class Calculator {
};
}
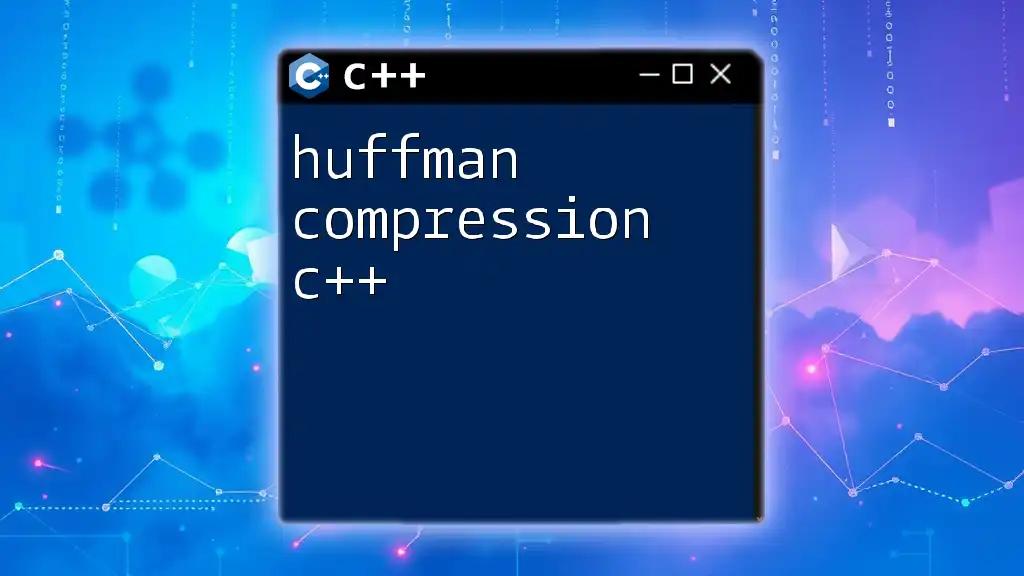
Best Practices for Naming in C++
Consistency is Key
Consistency in your naming conventions cannot be overstated. When the same conventions are followed uniformly across a codebase, it leads to a more coherent understanding of the code, making it easier for others to pick it up and contribute. Tools like linters can help enforce consistent naming schemes.
Avoiding Reserved Words
In C++, certain words are reserved for the language syntax, such as `int`, `float`, `return`, etc. Using reserved words as identifiers can lead to syntax errors and confusing code. Ensure any chosen names are distinct and descriptive.
Adopting a Naming Convention Early
Setting a naming convention at the project's beginning is highly beneficial. Doing so not only streamlines collaboration but also enhances the overall quality of the code. It's essential to discuss and agree on these conventions in a team setting to ensure everyone is on the same page.

Final Thoughts
In summary, naming convention in C++ is more than just a matter of style; it’s a fundamental aspect of writing clean, maintainable, and effective code. Adopting recognized naming practices can significantly enhance the readability of your code and reduce errors, especially in larger projects or collaborative environments.

Conclusion
It's essential to implement standardized naming conventions in your C++ projects not only to enhance clarity but also to ease maintenance challenges as your code evolves over time. Adopting these guidelines will undoubtedly contribute to your growth as a proficient C++ developer.

Code Snippet Repository
The following snippets offer a quick reference to varying naming conventions across different C++ code elements, illustrating how consistent naming can enhance codebases.
class Shape {
public:
double area();
};
int main() {
int my_variable = 7;
Shape shape;
double result = shape.area();
}
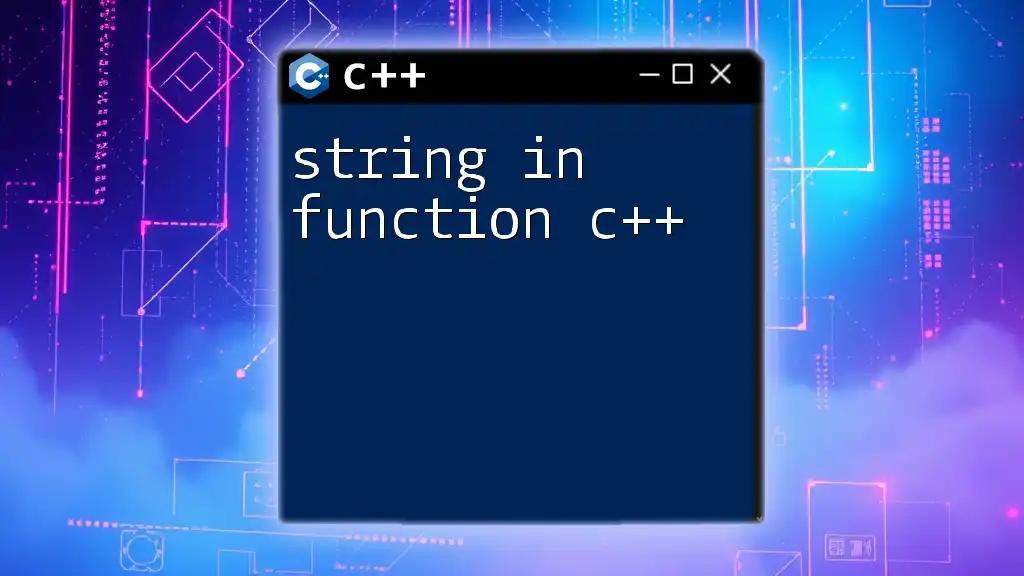
FAQs about C++ Naming Conventions
-
What is the purpose of naming conventions? Naming conventions improve code readability and maintainability, making it easier to understand and collaborate on projects.
-
Should I use CamelCase or snake_case? The choice often depends on personal or team preferences, but consistency is what matters most.
-
How do naming conventions affect team projects? Consistent naming conventions help prevent misunderstandings and errors, fostering a more productive team environment.
Understanding and applying proper naming conventions in C++ is an invaluable skill that will enhance the quality of your code and contribute to your success as a programmer. Use these guidelines to create clean, comprehensible, and maintainable C++ code!